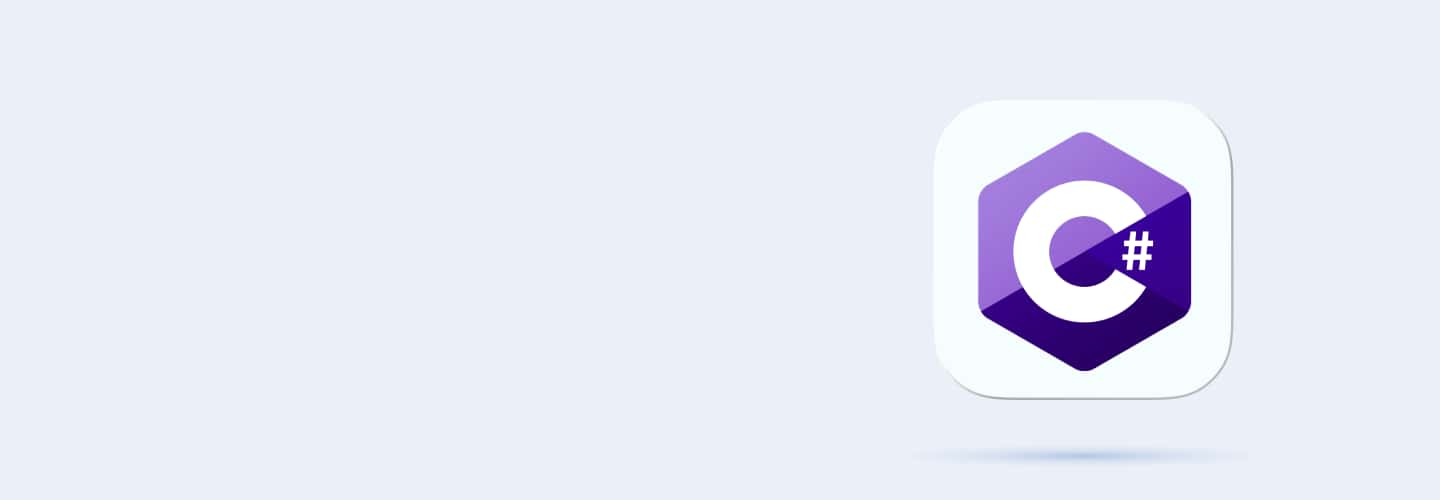
Q1
Q1 What is C# primarily used for in the .NET ecosystem?
System Programming
Web Development
Mobile Apps
All of the above
Q2
Q2 Who developed C#?
Microsoft
Apple
IBM
Q3
Q3 Which of the following is a feature of C#?
Platform dependent
Garbage collection
Weakly typed
None of the above
Q4
Q4 In which year was C# first released?
2000
2002
2005
2008
Q5
Q5 What type of language is C#?
Procedural
Functional
Object-oriented
All of the above
Q6
Q6 Which of the following best describes the .NET framework?
A hardware component
An operating system
A development platform
A database management system
Q7
Q7 Which language influenced the development of C# the most?
Java
Python
C++
PHP
Q8
Q8 What is the primary runtime used to execute C# programs?
JVM
CLR
Python Interpreter
None of the above
Q9
Q9 Which of the following is the correct way to start a C# console application?
public static void Main() { }
public void Main() { }
static void Main() { }
void Main() { }
Q10
Q10 If you receive a 'namespace not found' error, what is the likely cause?
Incorrect file extension
Missing 'using' directive
Typo in the class name
Missing semicolon
Q11
Q11 Which keyword is used to define a constant in C#?
constant
const
readonly
static
Q12
Q12 What is the correct syntax for a single-line comment in C#?
// comment
/* comment */
<!-- comment -->
# comment
Q13
Q13 Which of the following is a valid identifier in C#?
1variable
_variable
variable#
@variable
Q14
Q14 How do you declare an integer variable in C#?
int number;
integer number;
var number;
int: number;
Q15
Q15 Which keyword is used to prevent a class from being inherited in C#?
sealed
static
final
private
Q16
Q16 What is the output of 'Console.WriteLine(10 % 3);' in C#?
1
2
3
0
Q17
Q17 How do you define a method in C#?
void MethodName() { // code }
method MethodName() { // code }
void MethodName[] { // code }
function MethodName() { // code }
Q18
Q18 How do you write a 'for' loop in C#?
for (int i = 0; i < 10; i++) { }
for (int i = 0; i < 10) { }
for i in range(10): { }
for i = 0 to 9 { }
Q19
Q19 How do you create a new instance of a class in C#?
MyClass obj = new MyClass();
MyClass obj();
MyClass obj = MyClass();
new MyClass obj;
Q20
Q20 You get a 'missing semicolon' error.
What is the likely cause?
A line of code is missing a semicolon at the end
Incorrect class definition
Invalid variable declaration
Incorrect use of a keyword
Q21
Q21 You encounter a 'syntax error' in your C# code.
What could be the possible reason?
Missing brackets
Misspelled keywords
Incorrect use of operators
All of the above
Q22
Q22 Which of the following is a value type in C#?
String
Object
Int
Array
Q23
Q23 What is the default value of a boolean variable in C#?
true
false
0
null
Q24
Q24 Which of the following is a reference type in C#?
int
float
double
string
Q25
Q25 What is the size of an 'int' data type in C#?
2 bytes
4 bytes
8 bytes
16 bytes
Q26
Q26 What is the difference between 'int' and 'double' in C#?
'int' is for integer values, 'double' is for floating-point values
'int' is for small numbers, 'double' is for large numbers
'int' is a reference type, 'double' is a value type
'int' is an immutable type, 'double' is a mutable type
Q27
Q27 Which data type would you use for a variable that needs to store a large text?
char
string
StringBuilder
Text
Q28
Q28 How would you define a constant integer in C#?
constant int x = 10;
const int x = 10;
readonly int x = 10;
static int x = 10;
Q29
Q29 Which of the following is the correct way to assign a value to a variable in C#?
int x = 10;
int x == 10;
int x := 10;
int x -> 10;
Q30
Q30 How do you declare a character variable in C#?
char letter = 'A';
char letter = "A";
char letter = A;
char letter = 65;