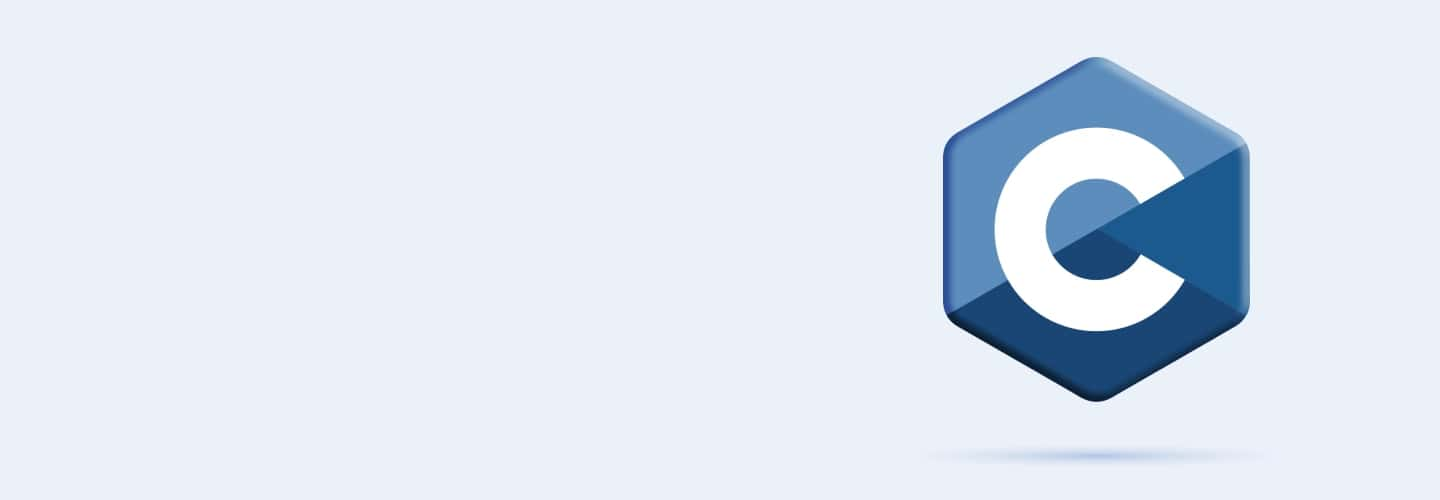
Q91
Q91 How do you access the value pointed to by a pointer in C?
Using the * operator
Using the & operator
Directly by the pointer name
Using the -> operator
Q92
Q92 What does passing a pointer to a function allow the function to do?
Read the value of the variable
Change the value of the variable
Copy the variable
None of the above
Q93
Q93 How does dynamic memory allocation differ between malloc and calloc?
malloc initializes memory to zero, calloc does not
calloc initializes memory to zero, malloc does not
They don't differ
malloc allocates more efficiently
Q94
Q94 What will be the output of the following C code?
int main() {
int a = 10;
int *p = &a;
printf("%d", *p);
return 0;
}
10
Address of a
Error
0
Q95
Q95 What does the following C code do?
int main() {
int arr[] = {1, 2, 3};
int *p = arr;
printf("%d", *(p + 1));
return 0;
}
Prints the first element of arr
Prints the second element of arr
Prints the third element of arr
Error
Q96
Q96 Complete the C code:
int main() {
int a = 10;
int *p = &a; ___;
printf("%d", a);
return 0;
}
to make the output 20.
*p = 20;
p = 20;
a = 20;
&a = 20;
Q97
Q97 Pseudocode:
SET a TO 10, POINTER p TO ADDRESS OF a, PRINT VALUE AT p
Prints 10
Prints the address of a
Error
None of the above
Q98
Q98 Pseudocode:
FUNCTION Increment(VALUE POINTER p) INCREMENT VALUE AT p END FUNCTION CALL Increment(ADDRESS OF num)
Increments num
Prints num
Returns num's address
Error
Q99
Q99 Identify the error in this C code:
int main() {
int *p; *p = 10;
printf("%d", *p);
return 0;
}
Uninitialized pointer
Syntax error
No error
Wrong printf format
Q100
Q100 Spot the mistake:
int main() {
int a = 10, *p = &a;
*p++;
printf("%d", a);
return 0;
}
Incrementing a instead of *p
Syntax error
No error
Incorrect use of increment operator
Q101
Q101 Find the error:
int main() {
int arr[3] = {1, 2, 3};
int *p = arr;
printf("%d", *p + 2);
return 0;
}
Pointer arithmetic error
Syntax error
No error
Wrong printf format
Q102
Q102 How is a string represented in C?
As a series of characters followed by a null character
As an array of integers
As a single character
As a special data type
Q103
Q103 What is the purpose of the strcpy function in C?
To compare two strings
To concatenate two strings
To copy one string to another
To find the length of a string
Q104
Q104 What does the strcat function do in C?
Concatenates two strings
Compares two strings
Converts a string to an integer
Splits a string into tokens
Q105
Q105 Which function is used to determine the length of a string in C?
strlen
strcmp
strncpy
strchr
Q106
Q106 What is the return value of strcmp when the two strings are equal in C?
0
1
-1
The length of the strings
Q107
Q107 How do you declare an array of strings in C?
char *arr[];
string arr[];
char arr[][];
char **arr;
Q108
Q108 What happens if a string copied using strcpy is larger than the destination array?
Syntax error
Compilation error
Runtime error
Undefined behavior
Q109
Q109 In C, which function is used to reverse a string?
strrev
strinvert
reverse
invert
Q110
Q110 How does the strncpy function differ from strcpy?
strncpy limits the number of characters copied
strncpy reverses the string
strncpy concatenates the string
No difference
Q111
Q111 What is the purpose of the strchr function in C?
Finds the first occurrence of a character in a string
Concatenates two strings
Reverses a string
Compares two strings
Q112
Q112 What is the output of this C code?
int main() {
char str[] = "Hello World";
printf("%d", strlen(str));
return 0;
}
11
12
10
Error
Q113
Q113 Complete the C code:
int main() {
char str[20];
___;
printf("%s", str);
return 0;
}
to copy "Hello" into str.
strcpy(str, "Hello");
str = "Hello";
strncpy(str, "Hello", 5);
strcat(str, "Hello");
Q114
Q114 What does the following C code do?
int main() {
char str1[] = "Hello", str2[20];
strcpy(str2, str1);
strrev(str2);
printf("%s", str2);
return 0;
}
Copies str1 to str2 and reverses it
Concatenates str1 and str2
Compares str1 and str2
None of the above
Q115
Q115 Pseudocode:
FUNCTION ConcatStrings(STRING str1, STRING str2) RETURN str1 PLUS str2 END FUNCTION
Concatenates two strings and returns the result
Finds the length of the two strings
Reverses the two strings
Compares the two strings
Q116
Q116 Pseudocode:
FUNCTION FindChar(STRING str, CHAR ch) FOR EACH character IN str IF character EQUALS ch RETURN True END FOR RETURN False
Searches for a character in a string and returns true if found
Reverses the string
Concatenates the string
None of the above
Q117
Q117 Pseudocode:
FUNCTION CopyString(STRING source, STRING destination) FOR EACH character IN source SET destination TO source END FOR
Copies one string to another
Compares two strings
Concatenates two strings
Reverses a string
Q118
Q118 Identify the error in this C code:
int main() {
char str[] = "Hello";
str[0] = 'M';
printf("%s", str);
return 0;
}
Cannot modify a string literal
No error
Syntax error
Wrong printf format
Q119
Q119 Spot the mistake:
int main() {
char *str = "Hello";
str[0] = 'M';
printf("%s", str);
return 0;
}
Cannot modify a string literal
Syntax error
No error
Wrong printf format
Q120
Q120 What is a structure in C?
A collection of variables under a single name
A method for looping
A data type for characters
A control flow statement