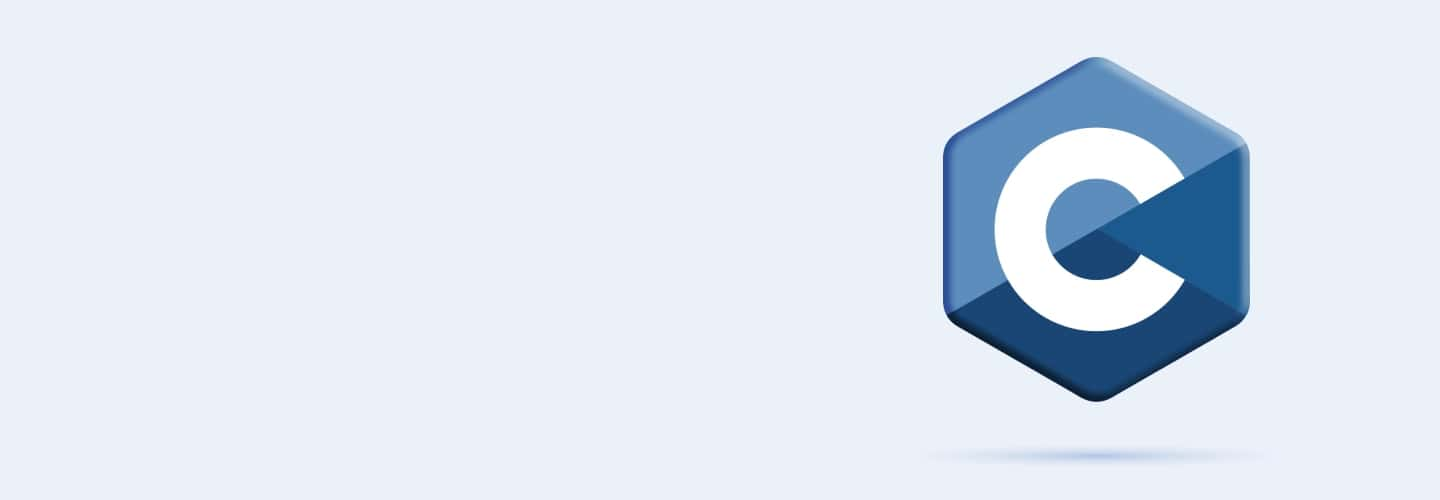
Q61
Q61 What will be the output of the following C code?
int main() {
int num = 10;
increment(num);
printf("%d", num);
return 0;
} void increment(int n) {
n++;
}
10
11
Error
Nothing
Q62
Q62 What is the output of this recursive function call?
int main() {
printf("%d ", factorial(5));
return 0;
}
int factorial(int n) {
if (n==0)
return 1;
else
return n * factorial(n - 1);
}
120
24
5
Error
Q63
Q63 Pseudocode:
FUNCTION Add(a, b) RETURN a PLUS b END FUNCTION CALL Add(5, 10)
15
5
10
Error
Q64
Q64 Pseudocode:
FUNCTION CheckEven(number) IF number MOD 2 EQUALS 0 THEN RETURN True ELSE RETURN False END FUNCTION CALL CheckEven(4)
True
False
Error
None
Q65
Q65 Pseudocode:
FUNCTION Fibonacci(n) IF n LESS THAN OR EQUAL TO 1 THEN RETURN n ELSE RETURN Fibonacci(n-1) PLUS Fibonacci(n-2) END FUNCTION CALL Fibonacci(5)
5
8
Error
None
Q66
Q66 Identify the error in this C code:
int main() {
printHello();
return 0;
}
void printHello() {
printf("Hello");
}
Missing declaration of printHello
Syntax error
No error
Wrong function call
Q67
Q67 Spot the mistake:
int main() {
int result = sum(5, 10);
printf("%d", result);
return 0;
}
int sum(int a, int b) {
return;
}
Missing return value in sum
Syntax error
No error
Incorrect function parameters
Q68
Q68 Find the error:
int main() {
int x = 5;
increment(&x);
printf("%d", x);
return 0;
}
void increment(int* n) {
*n++;
}
Incorrect pointer usage in increment
Syntax error
No error
Wrong printf format
Q69
Q69 How do you declare an array of integers in C?
int arr[10];
array int[10];
int array 10;
[10]int array;
Q70
Q70 Which index is used to access the first element of an array in C?
0
1
-1
First
Q71
Q71 What happens when you try to access an array element outside its bounds in C?
Syntax error
Compilation error
Runtime error
Undefined behavior
Q72
Q72 How do you initialize an array in C?
With any value
With 0s
With 1s
Cannot be initialized
Q73
Q73 Which of the following is a correct way to pass an array to a function in C?
By specifying the size of the array
By passing the first element
By passing the array name
By passing each element individually
Q74
Q74 What is the size of a 2-dimensional array int arr[3][4]; in C?
3
4
12
Cannot be determined
Q75
Q75 How can you access the third element in the second row of a 2D array int arr[3][4]; in C?
arr[1][2]
arr[2][3]
arr[3][2]
arr[2][1]
Q76
Q76 What is the default value of an uninitialized array in C?
0
1
Random memory value
-1
Q77
Q77 How does C handle array index out of bounds errors during runtime?
Generates a compile-time error
Automatically corrects the index
Generates a runtime error
Does not check for bounds
Q78
Q78 In C, can an array be resized after its declaration?
Yes
No
Only if it's a dynamic array
Only in C99 standard
Q79
Q79 What will be the output of the following C code?
int main() {
int arr[] = {1, 2, 3, 4, 5};
printf("%d", arr[3]);
return 0;
}
1
2
3
4
Q80
Q80 What is the output of this C code?
int main() {
int arr[5] = {1, 2, 3};
printf("%d", arr[4]);
return 0;
}
0
3
Random value
Error
Q81
Q81 What does the following C code do?
int main() {
int arr[3][4] = {{1, 2}, {3, 4}};
printf("%d", arr[1][1]);
return 0;
}
Prints 2
Prints 3
Prints 4
Prints 0
Q82
Q82 Complete the C code:
int main() {
int arr[5];
for(int i = 0; i < 5; i++) { ___ }
printf("%d", arr[2]);
return 0;
}
arr[i] = i;
arr[i] = i * 2;
arr[i] = i + 1;
arr[i] = 0;
Q83
Q83 Pseudocode:
ARRAY numbers[5] SET numbers[0] TO 1 PRINT numbers[0]
Prints 1
Prints 0
Prints random value
Syntax error
Q84
Q84 Pseudocode:
ARRAY arr[10] FOR i FROM 0 TO 9 SET arr[i] TO i TIMES 2 END FOR PRINT arr[6]
Prints 6
Prints 12
Prints 7
Syntax error
Q85
Q85 Pseudocode:
FUNCTION SumArray(ARRAY arr, SIZE) SET sum TO 0 FOR i FROM 0 TO SIZE-1 INCREMENT sum BY arr[i] RETURN sum
Calculates the sum of array elements
Returns the size of the array
Returns the first element
Syntax error
Q86
Q86 Pseudocode:
ARRAY matrix[2][3] SET matrix[0][0] TO 1, matrix[0][1] TO 2, matrix[0][2] TO 3, matrix[1][0] TO 4, matrix[1][1] TO 5, matrix[1][2] TO 6 PRINT matrix[1][2]
Prints 1
Prints 5
Prints 6
Syntax error
Q87
Q87 Identify the error in this C code:
int main() {
int arr[3];
arr[3] = 10;
printf("%d", arr[3]);
return 0;
}
Array index out of bounds
Syntax error
No error
Wrong printf format
Q88
Q88 Find the mistake:
int main() {
int arr[5] = {1, 2, 3, 4, 5};
for(int i = 0; i <= 5; i++) {
printf("%d ", arr[i]);
}
return 0;
}
Off-by-one error in loop bounds
Syntax error
No error
Incorrect array initialization
Q89
Q89 What is a pointer in C?
A variable that stores a memory address
A function
An array
A data type
Q90
Q90 What is the output of sizeof(pointer) where pointer is an integer pointer in C?
4 on all systems
8 on all systems
Depends on the system architecture
Depends on the compiler