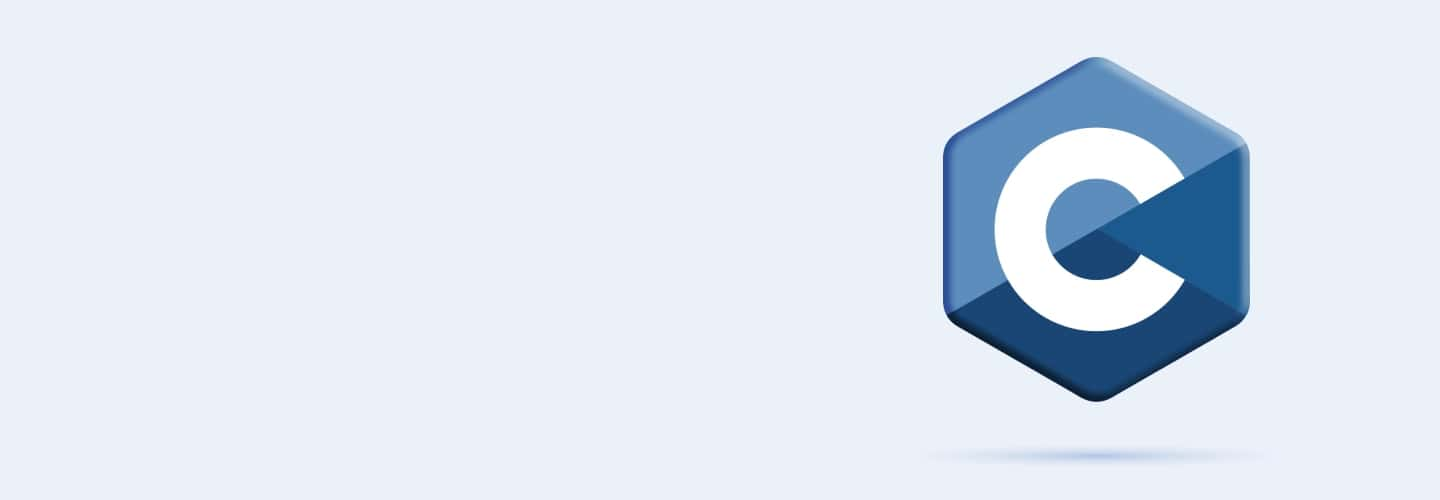
Q31
Q31 What is the output of the following C code snippet?
int main() {
int x
= 10;
printf("%d", x++ + ++x);
return 0;
}
20
21
22
23
Q32
Q32 What is the result of the following expression?
int a = 1; int b = a++ + ++a;
2
3
4
5
Q33
Q33 Pseudocode:
SET x TO 5, y TO 10 IF x LESS THAN y THEN PRINT "x is less than y" ELSE PRINT "x is not less than y"
Prints "x is less than y"
Prints "x is not less than y"
Prints nothing
Syntax error
Q34
Q34 Pseudocode:
SET a TO 10, b TO 20 PRINT a PLUS b
30
20
10
0
Q35
Q35 Pseudocode:
SET num TO 5 IF num EQUALS 5 THEN PRINT "Five" ELSE PRINT "Not Five"
Prints "Five"
Prints "Not Five"
Prints nothing
Syntax error
Q36
Q36 Pseudocode:
SET value TO 10 PRINT NOT value
-10
0
1
10
Q37
Q37 Pseudocode:
SET a TO 10, b TO 20 IF a GREATER THAN b THEN PRINT "a is greater" ELSE PRINT "b is greater or equal"
Prints "a is greater"
Prints "b is greater or equal"
Prints nothing
Syntax error
Q38
Q38 Pseudocode:
SET x TO 5 IF x GREATER THAN 0 AND x LESS THAN 10 THEN PRINT "x is between 0 and 10"
Prints "x is between 0 and 10"
Prints nothing
Syntax error
Prints "x is not between 0 and 10"
Q39
Q39 Pseudocode:
SET c TO 15 IF c MOD 2 EQUALS 0 THEN PRINT "Even" ELSE PRINT "Odd"
Prints "Even"
Prints "Odd"
Prints nothing
Syntax error
Q40
Q40 Spot the error:
int main() {
int a = 10, b = 0;
printf("%d", a / b);
return 0;
}
Division by zero
Syntax error
No error
Wrong format specifier
Q41
Q41 Identify the mistake:
int main() {
int x = 10, y; y = x == 10 ? x : 0;
printf("%d", y);
return 0;
}
Incorrect use of ternary operator
Syntax error
No error
Wrong variable used
Q42
Q42 What does the break statement do in a loop?
Exits the loop
Skips the current iteration
Repeats the loop
Does nothing
Q43
Q43 What is the difference between for and while loops?
Syntax
Execution speed
Functionality
No difference
Q44
Q44 In a switch statement, what happens if a break statement is omitted from a case?
The program exits
The case is skipped
Fall-through to the next case
Syntax error
Q45
Q45 What is the purpose of the continue statement in loops?
Terminates the loop
Skips to the next iteration
Does nothing
Exits the program
Q46
Q46 When is a do-while loop preferred over a while loop?
When the condition is complex
When the loop must execute at least once
When the loop should not execute
When the loop is infinite
Q47
Q47 What will the following C code print?
int main() {
for(int i = 0; i < 3; i++)
{ printf("%d ", i); }
return 0;
}
0 1 2
1 2 3
0 1 2 3
1 2 3 4
Q48
Q48 What does this C code do?
int main()
{ int i = 0;
while(i < 5) { if(i == 2) break;
printf("%d ", i); i++; }
return 0;
}
Prints 0 1 2
Prints 0 1
Prints all numbers less than 5
Prints nothing
Q49
Q49 What is the output of the following C code?
int main() {
int x = 0; do {
printf("%d ", x);
x++;
} while(x < 3);
return 0;
}
0 1 2
0 1
1 2 3
No output
Q50
Q50 Pseudocode:
SET num TO 10 IF num EQUALS 10 THEN PRINT "Ten" ELSE PRINT "Not Ten"
Prints "Ten"
Prints "Not Ten"
Prints nothing
Syntax error
Q51
Q51 Pseudocode:
SET x TO 5 WHILE x GREATER THAN 0 DO PRINT x DECREMENT x
Prints numbers from 5 to 1 in descending order
Prints 5
Prints 0
Syntax error
Q52
Q52 Pseudocode:
FOR i FROM 1 TO 5 PRINT i
Prints numbers from 1 to 5
Prints numbers from 1 to 4
Prints 5
Syntax error
Q53
Q53 Pseudocode:
SET count TO 0 REPEAT PRINT count INCREMENT count UNTIL count EQUALS 3
Prints 0 1 2 3
Prints 0 1 2
Prints 3
Syntax error
Q54
Q54 Pseudocode:
SET i TO 0 WHILE i LESS THAN 10 IF i MOD 2 EQUALS 0 THEN CONTINUE INCREMENT i PRINT i END WHILE
Prints odd numbers from 1 to 9
Prints even numbers from 0 to 8
Prints all numbers from 0 to 9
Syntax error
Q55
Q55 Identify the error in this C code:
int main() {
int i = 0;
while(i < 3) {
i++;
}
printf("%d ", i);
return 0;
}
Infinite loop
Syntax error
No error
Prints nothing
Q56
Q56 Spot the mistake:
int main() {
for(int i = 0; i < 5; i++) {
if(i == 2)
continue;
printf("%d ", i);
} return 0;
}
Infinite loop
Skips printing 2
Syntax error
No error
Q57
Q57 What is the primary purpose of a function in C?
Code reuse and modularity
Error handling
Memory management
User interface creation
Q58
Q58 What does 'pass by value' mean in C?
Passing a copy of the argument
Passing the argument directly
Passing the memory address
Passing the variable type
Q59
Q59 What is recursion in C?
A function calling another function
A function that has no return type
A function calling itself
A loop within a function
Q60
Q60 What is the scope of a local variable in C?
Inside the function in which it is declared
Throughout the program
Inside the file
Throughout the function block where it is declared