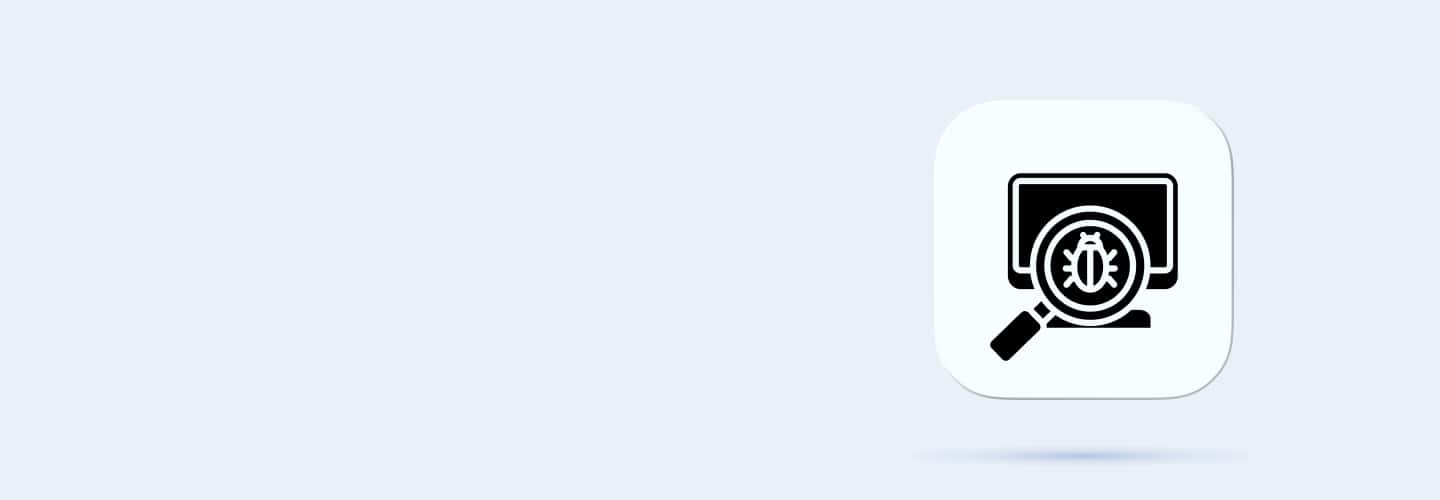
Q61
Q61 How do you run a group of tests in TestNG?
@Test(groups={"groupName"})
@RunGroup("groupName")
@TestGroup("groupName")
@IncludeGroup("groupName")
Q62
Q62 Write a JUnit annotation to execute a method after all test methods in a class.
@After
@AfterClass
@AfterTest
@Finalize
Q63
Q63 A TestNG test case fails with a "NullPointerException." What could be the issue?
Incorrect data setup
Null data returned by @DataProvider
No assertions in the test case
Unsupported annotations
Q64
Q64 A JUnit test fails with an "InitializationError." What could be the reason?
Missing @Test annotation
Unsupported test methods
Incorrect configuration
Incorrect test data
Q65
Q65 Why might a TestNG suite execution fail with a "NoClassDefFoundError"?
Incorrect test class path
Unsupported Java version
Missing dependencies
All of these
Q66
Q66 What is the main purpose of data-driven testing?
To test UI responsiveness
To execute tests with multiple data sets
To enhance debugging
To identify unique test cases
Q67
Q67 How is test data typically stored in a data-driven framework?
Within the test script
In external files
In the test runner
In browser cookies
Q68
Q68 What defines the steps to be executed in a keyword-driven framework?
Hardcoded test cases
Keywords stored in external files
Test runner configurations
Code comments
Q69
Q69 Which file format is commonly used for storing test data in data-driven testing?
PNG
Excel
JSON
HTML
Q70
Q70 What is a significant challenge in implementing keyword-driven frameworks?
Managing large keyword libraries
Integrating multiple tools
Handling unsupported browsers
Debugging test scripts
Q71
Q71 Write a command to fetch data from an Excel file in Java.
Workbook wb = WorkbookFactory.create(file);
Excel wb = new Excel(file);
Workbook wb = createWorkbook(file);
Excel wb = WorkbookFactory.open(file);
Q72
Q72 Write a Python snippet to read test data from a CSV file.
with open('data.csv', 'w') as file:
import csv; csv.writer(file)
with open('data.csv', 'r') as file: csv.reader(file)
file = open('data.csv', 'r')
Q73
Q73 Write a Selenium command to log in using data from a keyword-driven framework.
driver.findElement(By.id("username")).sendKeys(username);
driver.findElement(By.id("password")).sendKeys(password);
driver.findElement(By.id("loginButton")).click();
All of these
Q74
Q74 Write a Java function to retrieve test data from a MySQL database.
public ResultSet getData() {}
public Connection fetchDB() {}
public ResultSet fetchData(Connection conn) {}
public ResultSet getTestData() {}
Q75
Q75 A data-driven test fails due to missing data. What is the most probable cause?
Incorrect test logic
Empty data file
Unsupported file format
Invalid data mapping
Q76
Q76 Why might a test in a keyword-driven framework throw a "KeywordNotFound" error?
Unrecognized keyword
Unsupported file format
Invalid test script
All of these
Q77
Q77 Why might a data-driven test fail with a "NullPointerException"?
Missing data source
Incorrect test script
Unsupported framework version
Invalid syntax
Q78
Q78 What is the primary purpose of the Page Object Model (POM)?
To improve test readability and maintainability
To enhance debugging
To reduce test execution time
To avoid coding effort
Q79
Q79 How does POM improve test automation efficiency?
By reducing code duplication
By increasing execution speed
By simplifying assertions
By eliminating frameworks
Q80
Q80 What is a key component of a POM structure?
Page classes
Data providers
TestNG listeners
Framework annotations
Q81
Q81 Why is the @FindBy annotation used in POM?
To locate web elements
To provide data to test cases
To configure test listeners
To execute test scripts
Q82
Q82 What is a common challenge when implementing POM?
Handling dynamic elements
Writing test assertions
Integrating third-party tools
Executing parallel tests
Q83
Q83 Write a POM example to locate a web element using the @FindBy annotation.
@FindBy(id="username") WebElement userField;
@FindBy(name="username") WebElement userField;
@FindBy(xpath="//input[@id='username']") WebElement userField;
All of these
Q84
Q84 Write a Java method in POM to perform login using a username and password.
public void login() { username.sendKeys(); password.sendKeys(); loginButton.click(); }
public void login(String user, String pass) { username.sendKeys(user); password.sendKeys(pass); loginButton.click(); }
public void login(String user) { username.sendKeys(user); loginButton.click(); }
public void login(String pass) { password.sendKeys(pass); loginButton.click(); }
Q85
Q85 How do you initialize page elements in a POM class?
Using the PageFactory.initElements method
Using the constructor
Using a static block
Direct assignment
Q86
Q86 Write a POM example to handle a dynamic table row based on text content.
driver.findElement(By.xpath("//table//td[text()='DynamicText']"));
@FindBy(xpath="//table//td[text()='DynamicText']") WebElement tableRow;
public void handleRow(String text) { driver.findElement(By.xpath("//table//td[text()='"+text+"']")); }
All of these
Q87
Q87 A POM test fails because the web element is not initialized. What could be the issue?
Missing PageFactory.initElements
Incorrect @FindBy locator
Unsupported browser
Missing data provider
Q88
Q88 Why might a POM test fail with a "NoSuchElementException"?
Element is not interactable
Incorrect locator strategy
Element is not present in the DOM
All of these
Q89
Q89 Why might a test in a POM framework throw a "StaleElementReferenceException"?
Element is no longer attached to the DOM
Element locator changed
Browser driver crashed
Test script syntax is invalid
Q90
Q90 What is the main purpose of Continuous Integration (CI) in automation testing?
To execute tests manually
To integrate code frequently and test automatically
To perform UI testing
To reduce test scripts