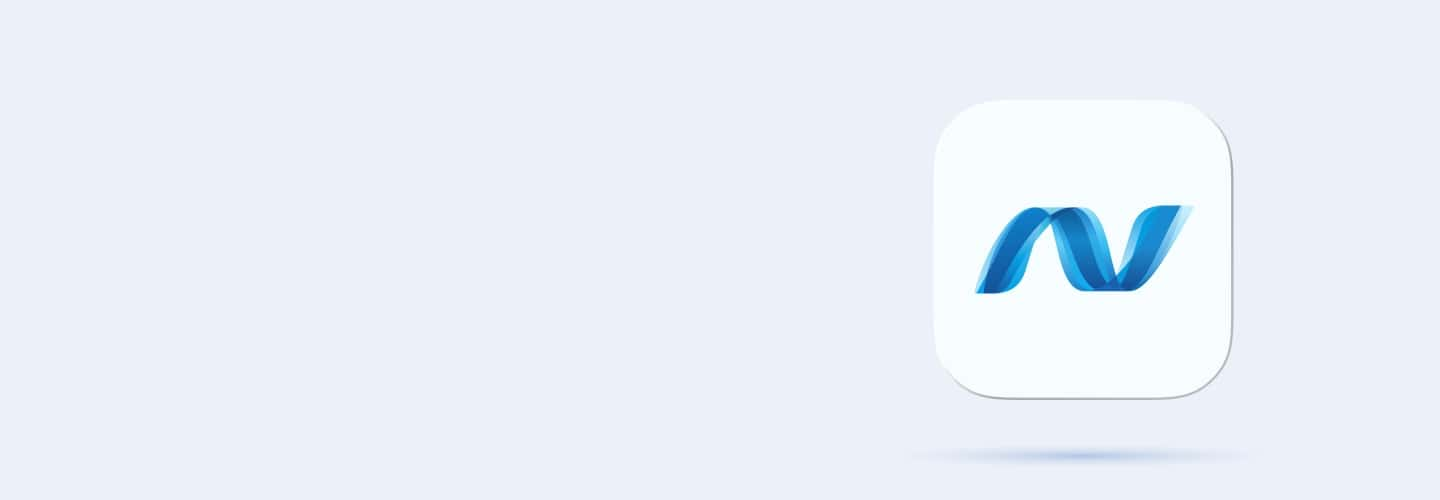
Q121
Q121 How do you log an error using the Trace class in ASP.NET?
Trace.Write("Error");
Trace.Log("Error");
Trace.Error("Error");
Trace.Add("Error");
Q122
Q122 How can you handle an application error globally in ASP.NET?
By using try-catch blocks in every method
By handling the Application_Error event in Global.asax
By setting custom error pages in the web.config
By logging the error to a database
Q123
Q123 Which tool is commonly used for debugging ASP.NET applications?
Fiddler
Postman
Visual Studio Debugger
SQL Profiler
Q124
Q124 How can you enable debugging in an ASP.NET application?
By setting debug="true" in the web.config
By setting debug="false" in the web.config
By enabling debugging in IIS
By using the Debug class
Q125
Q125 What is the use of the Debug class in ASP.NET?
To compile the application
To optimize performance
To provide debugging and tracing methods
To manage database connections
Q126
Q126 How do you use the Elmah library for error handling in ASP.NET?
By configuring Elmah in the web.config
By writing custom error handling code
By enabling Elmah in IIS
By adding Elmah to the Global.asax
Q127
Q127 What is the primary purpose of unit testing in ASP.NET?
To test the user interface
To test individual components of the application
To test database performance
To test network security
Q128
Q128 Which framework is commonly used for unit testing in ASP.NET?
NUnit
MSTest
XUnit
All of the above
Q129
Q129 What is integration testing in the context of ASP.NET?
Testing individual components
Testing the user interface
Testing the interaction between different components
Testing network security
Q130
Q130 How do you create a unit test method in MSTest?
[Test] public void TestMethod() {}
[TestMethod] public void TestMethod() {}
[UnitTest] public void TestMethod() {}
[TestClass] public void TestMethod() {}
Q131
Q131 How do you assert that a method throws an exception in NUnit?
Assert.Throws
Assert.Catch
Assert.Error
Assert.Fails
Q132
Q132 Which tool is commonly used for debugging unit tests in ASP.NET?
Visual Studio Debugger
Postman
SQL Profiler
Fiddler
Q133
Q133 How can you run unit tests in Visual Studio?
Using the Command Line
Using the Test Explorer window
Using the Solution Explorer window
Using the Output window
Q134
Q134 What is a mock object and how is it used in unit testing?
A real object used to test performance
A simulated object that mimics the behavior of real objects
A database connection
A security token
Q135
Q135 What is the primary purpose of the web.config file in deployment?
To manage database connections
To configure application settings
To handle user sessions
To optimize performance
Q136
Q136 What is the benefit of using a Web Deploy package for ASP.NET applications?
Simplifies deployment process
Improves code readability
Enhances security
Reduces development time
Q137
Q137 What is the role of IIS in hosting ASP.NET applications?
To manage databases
To handle user authentication
To serve web applications to users
To compile code
Q138
Q138 How do you publish an ASP.NET application to IIS using Visual Studio?
Use the "Compile" option
Use the "Build" option
Use the "Publish" option
Use the "Run" option
Q139
Q139 How do you configure a custom domain for an ASP.NET application in IIS?
Edit the hosts file
Add a binding for the domain in IIS
Modify the web.config file
Change the application settings in Visual Studio
Q140
Q140 Which tool can be used to diagnose deployment issues in ASP.NET applications?
Visual Studio Debugger
Web Deploy
Event Viewer
Postman
Q141
Q141 How can you troubleshoot HTTP 500 errors in an ASP.NET application hosted on IIS?
Check the application code
Review the IIS logs
Use browser developer tools
Restart the application pool
Q142
Q142 What is the purpose of the Application Pool in IIS?
To manage multiple websites
To isolate applications for better security and reliability
To serve static files
To handle DNS resolution
Q143
Q143 What is the primary goal of performance tuning in ASP.NET applications?
To improve code readability
To optimize application speed and efficiency
To enhance security
To simplify deployment
Q144
Q144 Which of the following can help reduce the load time of an ASP.NET web page?
Using inline CSS
Using image sprites
Using multiple external CSS files
Using large images
Q145
Q145 What is the purpose of output caching in ASP.NET?
To store user sessions
To reduce server load by caching the generated output of pages
To handle authentication
To manage database connections
Q146
Q146 How do you enable output caching for a page in ASP.NET?
Add the [OutputCache] attribute to the page directive
Set cache="true" in the web.config
Use the Cache object
Enable caching in IIS
Q147
Q147 How do you implement asynchronous programming in ASP.NET to improve performance?
Use the async and await keywords
Use synchronous methods
Use the Thread.Sleep method
Use the Task.Delay method
Q148
Q148 Which tool is commonly used to analyze the performance of an ASP.NET application?
Fiddler
Postman
SQL Profiler
Visual Studio Profiler
Q149
Q149 How can you identify performance bottlenecks in an ASP.NET application?
By reviewing code for syntax errors
By using performance profiling tools
By checking the application logs
By using a higher bandwidth connection
Q150
Q150 What is a common technique to improve database query performance in ASP.NET?
Use inline queries
Use complex queries
Use parameterized queries
Use indexing