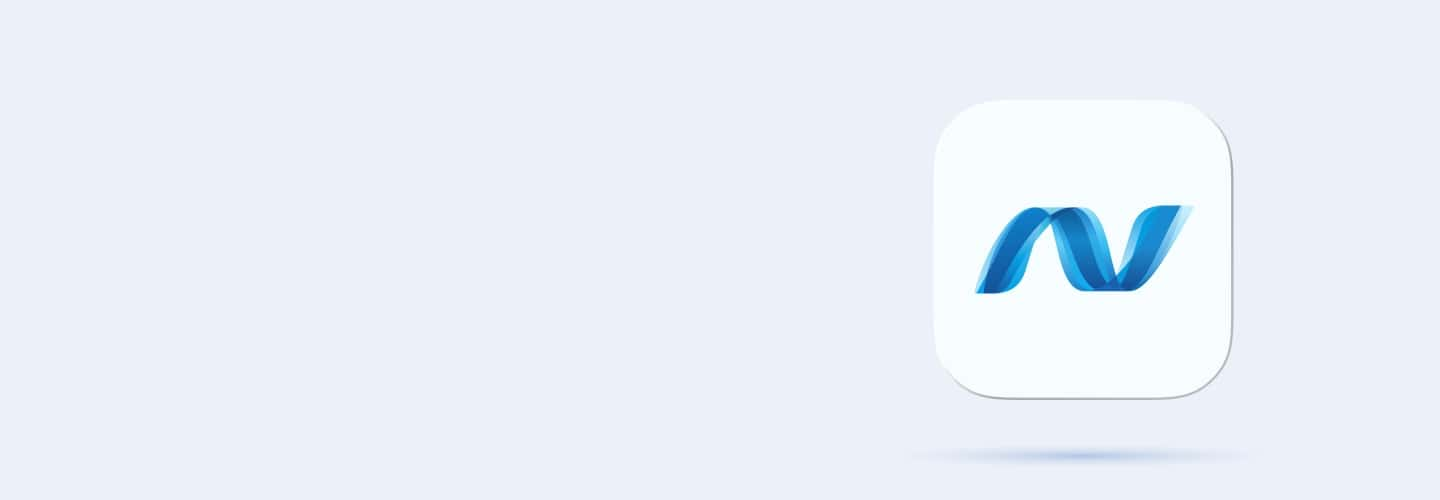
Q91
Q91 How do you ensure security in a Web API?
By using SSL
By storing passwords in plaintext
By disabling authentication
By allowing all IPs
Q92
Q92 How do you return a JSON response from a Web API controller action?
return Json(object);
return new JsonResult(object);
return Ok(object);
return JsonResponse(object);
Q93
Q93 How do you specify an HTTP GET method in a Web API controller?
[HttpGet]
[Get]
[HttpVerb("GET")]
[HttpRetrieve]
Q94
Q94 How do you handle exceptions in a Web API?
By using try-catch blocks
By using exception filters
By using middleware
All of the above
Q95
Q95 Which tool can be used to test Web API endpoints?
Fiddler
Postman
SQL Profiler
Route Debugger
Q96
Q96 How can you enable tracing in a Web API application?
By using the System.Diagnostics.Trace class
By setting Trace="true" in Web.config
By adding a trace listener in Global.asax
By using tracing middleware
Q97
Q97 What is the primary purpose of SignalR in ASP.NET?
To manage databases
To create real-time web applications
To handle user authentication
To optimize performance
Q98
Q98 What is a Hub in SignalR?
A database component
A security feature
A server-side component for handling client connections
A caching mechanism
Q99
Q99 Which protocol is preferred for SignalR when available?
Long Polling
WebSockets
Server-Sent Events
HTTP
Q100
Q100 How does SignalR handle fallback transport methods?
By using only WebSockets
By disconnecting the client
By automatically switching to supported transport methods
By throwing an error
Q101
Q101 What is the main use of the PersistentConnection class in SignalR?
To manage database connections
To create reusable connections that handle low-level communication details
To optimize performance
To manage user authentication
Q102
Q102 How do you define a Hub in SignalR?
public class MyHub : SignalRHub
public class MyHub : Hub
public class MyHub : SignalR.Connection
public class MyHub : SignalR.Component
Q103
Q103 How do you call a client method from a SignalR Hub using a specific client ID?
Clients.Caller.methodName()
Clients.Client("clientId").methodName()
Clients.Group("groupName").methodName()
Clients.All.methodName()
Q104
Q104 How do you handle disconnections in SignalR?
By overriding the OnDisconnectedAsync method
By using the OnDisconnect event
By implementing the IDisconnectHandler interface
By using the Disconnect attribute
Q105
Q105 Which tool can be used to monitor SignalR traffic?
Fiddler
Postman
SQL Profiler
Browser Developer Tools
Q106
Q106 What is a recommended approach to troubleshoot connection issues in SignalR?
By checking server logs
By enabling detailed client-side logging
By using browser developer tools
By testing different transport methods
Q107
Q107 What is the primary purpose of ASP.NET Identity?
To manage sessions
To handle HTTP requests
To provide authentication and authorization
To manage database connections
Q108
Q108 What is a role in ASP.NET Identity?
A type of database connection
A type of user
A set of permissions assigned to a user
A method of session management
Q109
Q109 Which class is used to manage users in ASP.NET Identity?
UserManager
RoleManager
SignInManager
IdentityManager
Q110
Q110 What is the purpose of the SignInManager class in ASP.NET Identity?
To create roles
To manage user sign-ins
To handle database connections
To manage user sessions
Q111
Q111 How does ASP.NET Identity store user information by default?
In a SQL database
In memory
In a text file
In an XML file
Q112
Q112 How do you create a new user in ASP.NET Identity?
userManager.AddUserAsync(user);
userManager.CreateAsync(user, password);
userManager.AddAsync(user);
userManager.NewUserAsync(user, password);
Q113
Q113 How do you add a user to a role in ASP.NET Identity?
roleManager.AddUserToRole(user, role);
userManager.AddToRoleAsync(user, role);
signInManager.AddToRoleAsync(user, role);
identityManager.AddUserToRoleAsync(user, role);
Q114
Q114 How do you verify a user password in ASP.NET Identity?
userManager.VerifyPassword(user, password);
signInManager.CheckPassword(user, password);
userManager.CheckPasswordAsync(user, password);
identityManager.VerifyPasswordAsync(user, password);
Q115
Q115 Which tool can be used to monitor and debug authentication issues in ASP.NET Identity?
Fiddler
Postman
SQL Profiler
Browser Developer Tools
Q116
Q116 How do you handle errors when creating a user in ASP.NET Identity?
By logging the errors
By showing detailed error messages to the user
By using try-catch blocks and checking IdentityResult
By ignoring the errors
Q117
Q117 What is the primary purpose of error handling in ASP.NET?
To enhance performance
To manage user sessions
To handle and log application errors
To manage databases
Q118
Q118 What is a common method for handling exceptions in ASP.NET?
Using if-else statements
Using try-catch blocks
Using loops
Using break statements
Q119
Q119 What is the role of the Global.asax file in error handling?
To define CSS styles
To handle application-level events and errors
To manage database connections
To optimize performance
Q120
Q120 What is the purpose of the customErrors element in Web.config?
To customize the appearance of a website
To manage user roles
To define custom error pages
To handle database queries