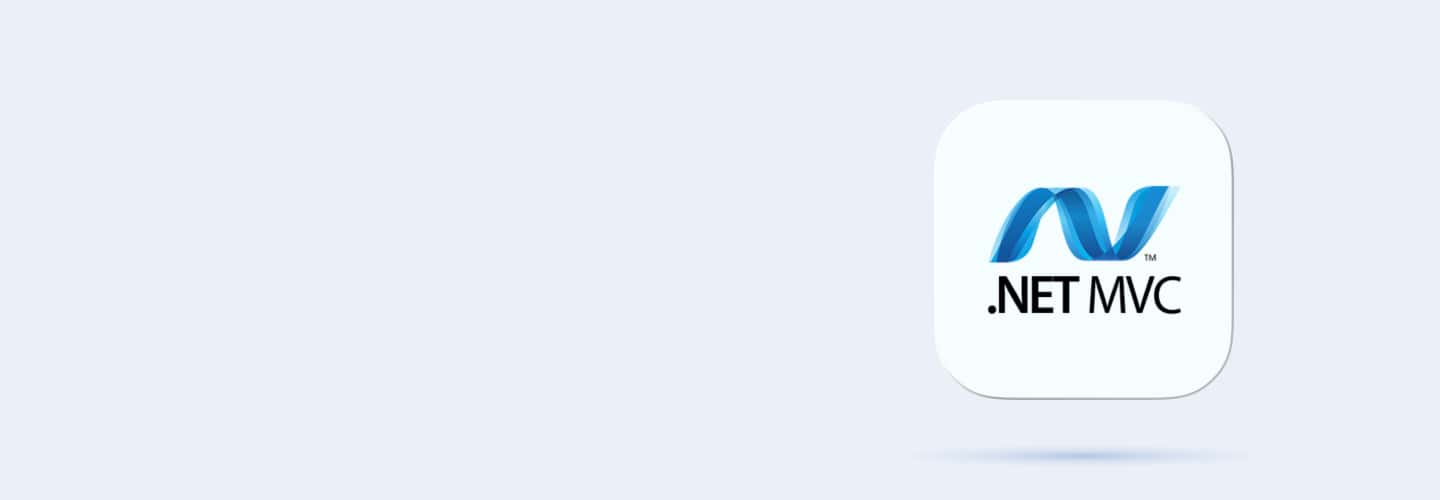
Q61
Q61 How do you create a custom route in the RouteConfig.cs file?
routes.MapRoute("name", "url");
routes.AddRoute("name", "url");
routes.CreateRoute("name", "url");
routes.CustomRoute("name", "url");
Q62
Q62 How can you specify a default value for a route parameter?
Add it to the RouteConfig file
Use an attribute on the controller
Set it in the route template
Use a query string
Q63
Q63 How do you implement route constraints in ASP.NET MVC?
Define regex in the route template
Use a custom constraint class
Define a route handler
Use inline constraints
Q64
Q64 A route is not matching. What is the likely issue?
Incorrect route order
Invalid controller
Action method missing
HTTP method mismatch
Q65
Q65 A route parameter is not being passed to the controller action. Why?
Parameter name mismatch
Missing ViewBag
Route is not registered
The controller is invalid
Q66
Q66 A custom route throws a 404 error. What could be the issue?
Invalid template syntax
Default route conflicts
Controller is not found
HTTP method is missing
Q67
Q67 What is the primary purpose of HTML Helpers in ASP.NET MVC?
Generate HTML elements
Handle routing
Define controller actions
Manage data binding
Q68
Q68 Which method is used to create a form in ASP.NET MVC using HTML Helpers?
Html.BeginForm()
Html.CreateForm()
Html.RenderForm()
Html.NewForm()
Q69
Q69 What is the role of Data Annotations in ASP.NET MVC?
Defining routes
Validating model properties
Handling authentication
Creating Razor views
Q70
Q70 What does the [Required] attribute enforce in a model?
Field must not be null
Field must be unique
Field must be a number
Field must be a string
Q71
Q71 How do you create a custom HTML Helper in ASP.NET MVC?
Extend the HtmlHelper class
Override Razor syntax
Create a custom controller
Modify the ViewBag
Q72
Q72 How do you create a text box using HTML Helpers?
@Html.TextBox("name")
@Html.CreateTextBox("name")
@Html.NewTextBox()
@Html.RenderTextBox()
Q73
Q73 How do you add a placeholder to an input field using Html.TextBoxFor?
Use the placeholder attribute in htmlAttributes
Add a [Display] annotation
Use a custom helper
Modify the Razor layout
Q74
Q74 How do you apply a CSS class to a form element using HtmlHelper?
Use the class attribute in htmlAttributes
Add the CSS class in Razor
Define it in the controller
Use the @ClassFor method
Q75
Q75 How do you render a dropdown list with specific values using HTML Helpers?
Html.DropDownList("name", selectList)
Html.SelectList("name")
Html.CreateDropDown()
Html.NewDropDown()
Q76
Q76 A HtmlHelper method throws a null reference exception. What is the issue?
The model is null
Route is missing
View is not rendered
Controller is invalid
Q77
Q77 A [Required] validation attribute is not working. Why?
Missing DataAnnotations namespace
Invalid controller
Route not defined
View is missing
Q78
Q78 A custom HTML Helper is not rendering correctly. What could be the issue?
The helper is not registered
Controller is invalid
ViewBag is not used
Invalid Razor syntax
Q79
Q79 What is the purpose of model validation in ASP.NET MVC?
Validate routes
Ensure data integrity
Secure the database
Render Razor views
Q80
Q80 Which namespace is required for Data Annotations in ASP.NET MVC?
System.Web.Mvc
System.Data
System.ComponentModel.DataAnnotations
System.IO
Q81
Q81 How does [StringLength] differ from [MaxLength] in validation?
It validates Razor syntax
It applies both client and server-side validation
It ensures a fixed length
It specifies a maximum and minimum length
Q82
Q82 What is the role of [Authorize] in ASP.NET MVC?
To define routes
To restrict access to actions
To validate models
To render views
Q83
Q83 How can you implement custom authentication in ASP.NET MVC?
Create a custom AuthorizeAttribute
Use HttpContext.User
Modify RouteConfig
Customize ViewBag
Q84
Q84 How do you enable client-side validation in ASP.NET MVC?
Add @Html.EnableClientValidation()
Include jQuery Validation library
Define rules in the Controller
Both A and B
Q85
Q85 How do you check if a user is authenticated in a Controller?
Use HttpContext.IsAuthenticated
Use User.Identity.IsAuthenticated
Use Session["Authenticated"]
Use Authorize.IsValid()
Q86
Q86 How do you validate a model manually in an action?
Use TryValidateModel()
Call ModelState.Clear()
Use ModelState.IsValid
Override the Validate method
Q87
Q87 A validation error is not displayed in the view. What could be the issue?
Validation scripts are missing
Controller is invalid
Route is not defined
Model is null
Q88
Q88 A [Required] validation is ignored on the server-side. What could be wrong?
Model binding failed
DataAnnotations namespace is missing
Client-side validation is disabled
Validation attribute is not applied
Q89
Q89 The [Authorize] attribute is not working as expected. What could be wrong?
Custom attribute is not registered
Authentication middleware is missing
Route is invalid
User role is not defined
Q90
Q90 What is Dependency Injection in ASP.NET MVC?
A pattern for database access
A technique for managing dependencies
A routing mechanism
A way to validate models