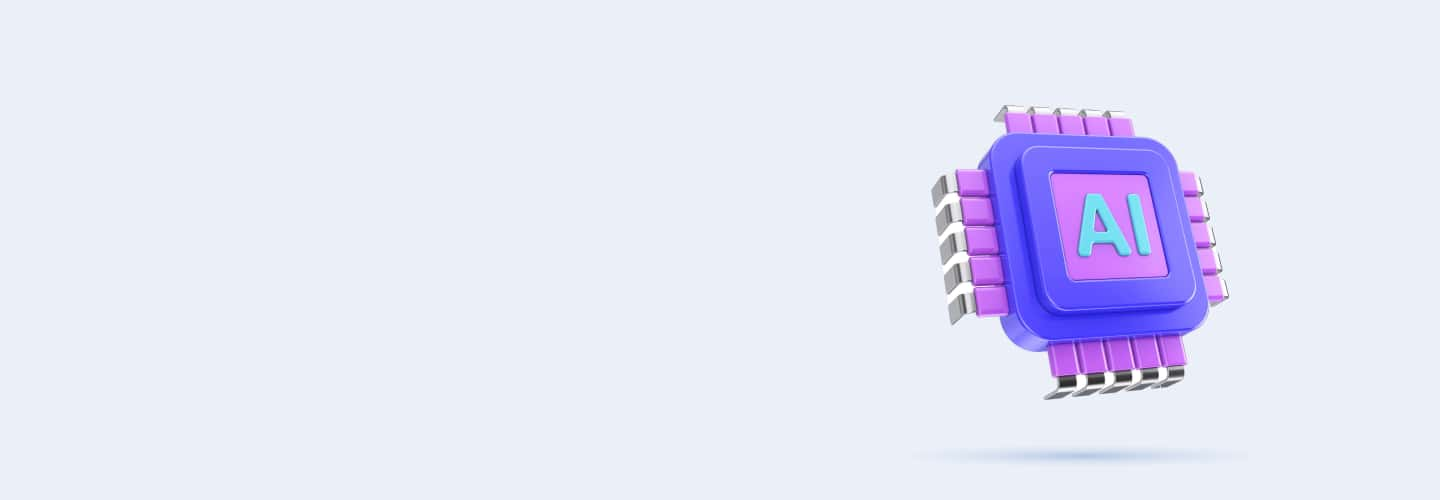
Q91
Q91 How would you create a simple feedforward neural network using TensorFlow in Python?
Use Dense() layers
Use a for loop
Use a decision tree
Use KMeans()
Q92
Q92 How do you compile a neural network in Keras?
Use the compile() function
Use the fit() function
Use the train() function
Use the evaluate() function
Q93
Q93 How would you implement dropout in a Keras model?
Use the Dropout() layer
Use regularization
Change the learning rate
Use a for loop
Q94
Q94 How would you handle the vanishing gradient problem in deep neural networks?
Increase the number of layers
Use ReLU or similar activation functions
Use fewer neurons
Use a lower learning rate
Q95
Q95 How would you implement a recurrent neural network (RNN) in TensorFlow?
Use LSTM() or GRU() layers
Use Dense() layers
Use PCA
Use KMeans()
Q96
Q96 Your neural network model is overfitting on the training data. What could be a solution?
Increase the number of neurons
Add dropout
Increase the learning rate
Remove layers
Q97
Q97 Your deep learning model is not improving despite increasing the number of layers. What could be wrong?
The model is underfitting
The learning rate is too low
The model is overfitting
The dataset is too small
Q98
Q98 Your RNN is failing to remember long sequences. What is the most likely cause?
The model is too deep
The dataset is too small
The vanishing gradient problem
The learning rate is too high
Q99
Q99 Your deep learning model is experiencing exploding gradients. What should you do?
Increase the learning rate
Use gradient clipping
Increase the number of layers
Change the activation function
Q100
Q100 What is tokenization in Natural Language Processing (NLP)?
The process of splitting text into sentences
The process of removing stopwords
The process of splitting text into smaller units like words or phrases
The process of stemming words
Q101
Q101 In NLP, what is the primary purpose of stemming?
To remove punctuation
To shorten words to their base form
To count word frequency
To group similar words together
Q102
Q102 What is the role of stop words in NLP?
Words that are removed during text processing
Words that are tokenized
Words that are analyzed for sentiment
Words that are grouped for stemming
Q103
Q103 What is the Bag of Words model in NLP?
A model that generates word embeddings
A model that captures the frequency of words without considering order
A model that groups words into clusters
A model that generates sentence embeddings
Q104
Q104 Which of the following is a key disadvantage of the Bag of Words model?
It considers word order
It captures only the frequency of words
It works well with small datasets
It is computationally expensive
Q105
Q105 What is the primary advantage of using word embeddings like Word2Vec in NLP over Bag of Words?
It captures word frequency
It captures the semantic meaning of words
It is computationally efficient
It removes stop words
Q106
Q106 What is named entity recognition (NER) in NLP?
Identifying and classifying proper names in a text
Tokenizing a sentence
Counting word frequency
Removing stop words
Q107
Q107 Which Python library would you use to implement tokenization and stop word removal in NLP?
TensorFlow
NLTK
Scikit-learn
PyTorch
Q108
Q108 How would you implement a Bag of Words model in Python using Scikit-learn?
Use CountVectorizer()
Use fit_transform()
Use tokenize()
Use transform()
Q109
Q109 How would you create word embeddings using the Gensim library in Python?
Use Word2Vec()
Use CountVectorizer()
Use KMeans()
Use tokenize()
Q110
Q110 How would you implement Named Entity Recognition (NER) using SpaCy in Python?
Use ner.pipe()
Use displacy()
Use ner.recognize()
Use entity.recognize()
Q111
Q111 Your NLP model is struggling with understanding context in sentences. What could be a solution?
Use a larger dataset
Switch from Bag of Words to word embeddings
Reduce stop words
Use regularization
Q112
Q112 Your named entity recognition (NER) model is incorrectly classifying entities. What could be the issue?
The dataset is too large
The model lacks enough training data
The stop words are not removed
The tokenizer is too complex
Q113
Q113 Your NLP model is performing poorly in sentiment analysis. What might be the problem?
The data is noisy
The model is underfitting
The stop words are too many
The word embeddings are too small
Q114
Q114 What is the primary goal of AI in robotics?
To replace human labor
To enhance robotic capabilities in decision-making
To build faster robots
To minimize costs
Q115
Q115 What is a key difference between traditional robots and AI-powered robots?
Traditional robots use sensors
AI-powered robots can make decisions based on data
AI-powered robots have no sensors
Traditional robots cannot move
Q116
Q116 In robotics, what is path planning?
The process of navigating obstacles
The process of determining an optimal path for a robot to follow
The process of assembling a robot
The process of programming a robot
Q117
Q117 Which of the following is a challenge in integrating AI with robotics?
Lack of data
High costs
Limited computing power
All of the above
Q118
Q118 In AI-powered robotics, what is the role of reinforcement learning?
To mimic human actions
To enable a robot to learn from its environment through rewards and penalties
To predict robot movements
To analyze robot failures
Q119
Q119 Which Python library is commonly used for controlling robotic systems and simulating environments?
TensorFlow
PyTorch
ROS (Robot Operating System)
NLTK
Q120
Q120 How would you implement obstacle avoidance in a robot using AI?
Use object detection and path planning algorithms
Use reinforcement learning
Use a pre-programmed path
Use a random movement strategy