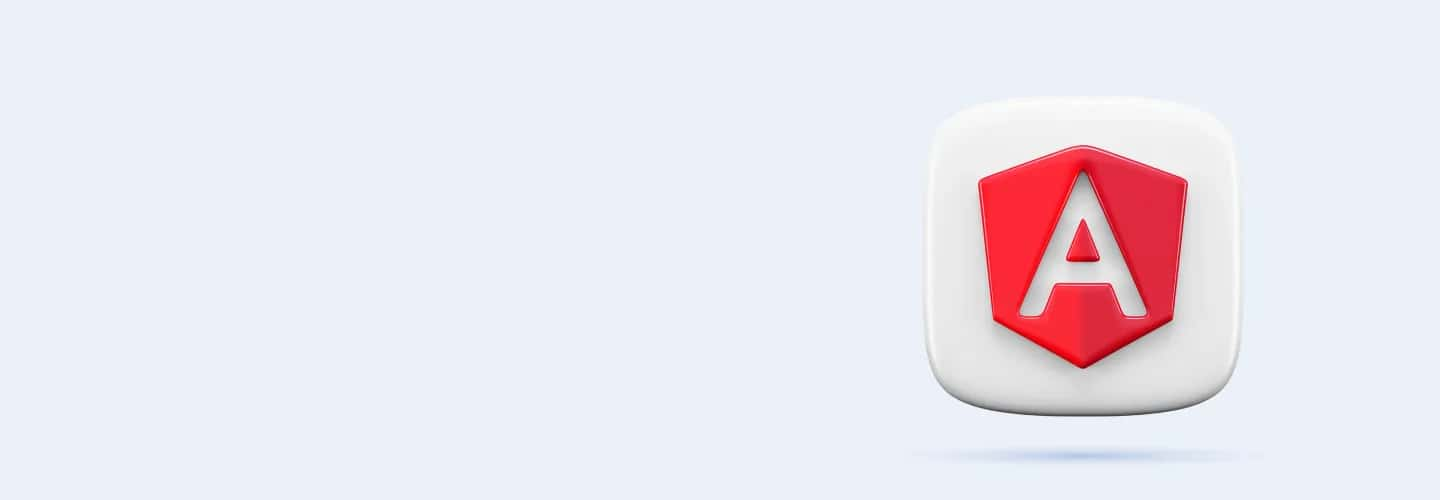
Q91
Q91 When a component's view does not update as expected after data changes, which lifecycle hook can help diagnose the issue?
ngOnChanges()
ngAfterViewChecked()
ngOnInit()
ngAfterViewInit()
Q92
Q92 What is the primary role of a reducer in NgRx?
To dispatch actions
To mutate the state in response to actions
To select data from the store
To handle side effects
Q93
Q93 In NgRx, what is the purpose of selectors?
To dispatch actions to the store
To reduce the state based on the action
To select and derive data from the store
To handle asynchronous operations
Q94
Q94 How does NgRx's Effect class contribute to state management?
By directly mutating the state
By synchronizing the state with a backend service
By handling side effects of actions
By dispatching new actions
Q95
Q95 How do you create an action in NgRx?
Using the createAction function
By defining a class that implements the Action interface
By dispatching a string to the store
By calling a service method
Q96
Q96 How do you handle asynchronous operations like API calls in NgRx?
Using reducers
With selectors
Through effects
By subscribing to the store
Q97
Q97 If an action does not trigger the expected state change in NgRx, what should you investigate first?
The action type in the reducer
The state mutation logic in the reducer
The action dispatching process
The store configuration
Q98
Q98 In NgRx, when an effect does not emit an action or causes an error, what is a common debugging approach?
Check the effect's source observable
Verify the action types the effect is listening for
Inspect the effect's error handling logic
Review the overall state management architecture
Q99
Q99 What is Angular Material primarily used for?
Creating RESTful services
Implementing complex algorithms
Designing responsive UI components
Managing application state
Q100
Q100 How do you apply a theme in Angular Material?
By using a CSS file
Through a JavaScript configuration
By importing a pre-built theme in styles.scss
By creating a custom service
Q101
Q101 What is the purpose of the MatDialog component in Angular Material?
To create a service
To display a modal dialog
To build a navigation menu
To show notifications
Q102
Q102 Which Angular Material module should be imported to use form field components?
MatFormFieldModule
MatInputModule
MatButtonModule
MatToolbarModule
Q103
Q103 How do you create a responsive sidebar using Angular Material?
Using the MatSidenavModule
With the MatToolbarModule
By applying CSS media queries
Through the MatMenuModule
Q104
Q104 If Angular Material components are not displaying correctly, what is a common thing to check first?
The version of Angular Material
The browser compatibility
The import of Angular Material modules in the app module
The network connection
Q105
Q105 When an Angular Material dialog is not opening as expected, what should you investigate?
The MatDialog configuration in the component
The version of the Angular framework
The CSS styles applied to the dialog
The HTML structure of the application
Q106
Q106 In Angular, what is the purpose of Jasmine and Karma in the context of testing?
Jasmine is for unit testing and Karma for integration testing
Jasmine provides the framework and Karma runs the tests in a browser environment
Jasmine and Karma both provide testing frameworks
Jasmine and Karma are both used for mocking dependencies
Q107
Q107 What is a 'spy' in the context of Angular testing?
A tool to simulate user interaction
A function that records calls, arguments, and returns values
A type of unit test
An assertion method
Q108
Q108 How does Angular's TestBed utility aid in testing components and services?
By providing a simplified way of creating components
By mocking Angular's dependency injection system
By automatically running tests
By simulating user interactions
Q109
Q109 How do you test an Angular service that depends on HttpClient?
By using the real HttpClient module
By mocking HttpClient using TestBed
By manually creating a fake HttpClient instance
By only testing the service logic, ignoring HttpClient dependencies
Q110
Q110 In an Angular test, how do you verify that a method has been called on a component's class?
Using expect().toHaveBeenCalled()
By checking the return value of the method
By observing changes in the component's template
By monitoring network requests
Q111
Q111 When a unit test fails unexpectedly in Angular, what is the first step in debugging?
Reviewing the test code for syntax errors
Checking the component or service logic
Verifying the test setup and dependencies
Looking at the error message in the test output
Q112
Q112 If an Angular component test is failing due to missing dependencies, how do you resolve it?
By importing all required modules into the test
By mocking all dependencies
By adding the missing dependencies to the TestBed configuration
By removing the dependencies from the component
Q113
Q113 What is the primary advantage of using Angular Universal for server-side rendering?
Faster initial page load times
Easier debugging
Reduced client-side scripting
Simplified component design
Q114
Q114 How does Angular Universal enhance SEO for Angular applications?
By increasing page load speed
By generating static HTML pages
By optimizing JavaScript execution
By reducing CSS usage
Q115
Q115 In what scenario is Angular Universal not recommended?
For applications with real-time dynamic content
For simple Angular applications
For applications with heavy user interaction
For content-heavy websites
Q116
Q116 How do you enable server-side rendering for an existing Angular application?
By adding the @angular/platform-server module
By running ng add @nguniversal/express-engine
By modifying the main.ts file
By updating the angular.json file
Q117
Q117 When implementing Angular Universal, how do you handle browser-specific APIs like localStorage or window?
By avoiding their use entirely
By providing polyfills
By using conditional checks for platform
By mocking these APIs in the server environment
Q118
Q118 What common issue should you check for if your Angular Universal app isn't rendering properly on the server?
Incorrect Angular Universal configuration
Module not found errors
Mismatched versions of Angular and Angular Universal
Routing issues
Q119
Q119 How do you troubleshoot a scenario where an Angular Universal app works locally but not when deployed?
Check server logs for errors
Verify the server environment configuration
Ensure all dependencies are installed
Compare local and server environments
Q120
Q120 What is the main benefit of lazy loading modules in Angular?
Reducing the size of the root module
Faster initial load time of the application
Simplifying the development process
Improving server-side rendering performance