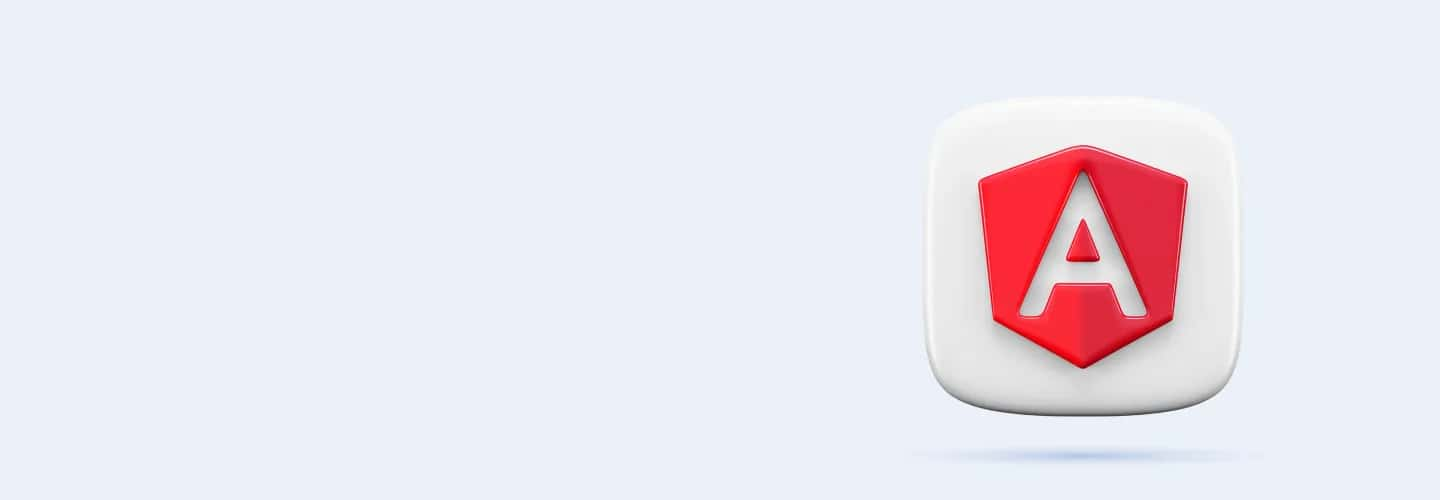
Q61
Q61 What is the purpose of the FormBuilder service in Angular's reactive forms?
To automatically generate form components
To create complex form validation rules
To provide a shorthand for creating form groups and form controls
To synchronize form data with the backend
Q62
Q62 Which directive is used to bind a template-driven form input to a component property in Angular?
ngModel
ngControl
formControl
ngForm
Q63
Q63 In Angular's reactive forms, which class represents a group of FormControls?
FormGroup
FormControlArray
FormControl
FormBuilder
Q64
Q64 If a form input bound with ngModel is not updating the model in a template-driven form, what could be a reason?
The ngModel directive is not imported
The form is not linked to the component
The input type is incompatible with ngModel
The component property is not correctly bound to ngModel
Q65
Q65 When a reactive form control is always invalid, what is a common issue to investigate?
Incorrect form control validation rules
Missing form control in the group
Incorrect data type for the form control
Form control not bound to the form
Q66
Q66 What is the primary use of the HttpClient service in Angular?
Managing local state
Interacting with RESTful APIs
Styling components
Handling authentication
Q67
Q67 In Angular, what are Observables primarily used for?
To observe changes in form control values
To handle asynchronous data such as HTTP responses
To watch changes in component properties
To monitor routing events
Q68
Q68 How do you subscribe to an Observable returned by an HttpClient method in Angular?
Using the .subscribe() method
With the async pipe in the template
Through a service
Using a callback function
Q69
Q69 What is the purpose of the 'pipe' function in RxJS Observables used in Angular's HttpClient?
To execute the Observable
To transform the data emitted by the Observable
To debug the Observable
To create a new Observable
Q70
Q70 Which method of the HttpClient service in Angular is used to send a GET request?
.get()
.post()
.send()
.fetch()
Q71
Q71 In Angular, how do you handle errors when making an HTTP request with HttpClient?
Using the catchError operator in the pipe function
With a try-catch block
By checking the status code in the response
Using an error event handler
Q72
Q72 If an HttpClient request in Angular fails to return data, what is a common thing to check first?
The URL of the request
The headers of the request
The status of the server
The configuration of HttpClient
Q73
Q73 When an Observable does not emit any values, what might be a reason in the context of Angular's HttpClient?
The Observable is not subscribed to
The server is not responding
There's a syntax error in the Observable creation
The response type is incorrect
Q74
Q74 What is the primary use of pipes in Angular?
To navigate between different components
To transform data for display
To manage state
To handle user input
Q75
Q75 Which built-in pipe in Angular is used for formatting dates?
numberPipe
datePipe
currencyPipe
jsonPipe
Q76
Q76 How do you apply a pipe to a value in an Angular template?
{{ value | pipeName }}
[value | pipeName]
{{ value } & pipeName}
{{ value: pipeName }}
Q77
Q77 What is the primary advantage of creating a custom pipe in Angular?
To add new features to the Angular framework
To create reusable transformations specific to the application
To replace the built-in pipes
To optimize the application's performance
Q78
Q78 Which pipe would you use to convert a string to uppercase in an Angular template?
uppercasePipe
lowercasePipe
stringPipe
capitalizePipe
Q79
Q79 How do you create a custom pipe in Angular?
Using the @Pipe decorator and implementing the PipeTransform interface
By extending a built-in pipe
By declaring it in a module
Using a service
Q80
Q80 If a pipe does not update the displayed value when the input changes, what could be the issue?
The pipe is not stateful
The input is not correctly bound
The pipe is not declared in a module
The pipe's transform method is incorrect
Q81
Q81 When a custom pipe throws an error in Angular, what is a common cause to investigate?
Incorrect implementation of the PipeTransform interface
Syntax error in the template
Incorrect input types for the pipe
The pipe is not included in the module declarations
Q82
Q82 Which lifecycle hook in Angular is called after Angular has initialized all data-bound properties of a directive?
ngOnInit()
ngOnChanges()
ngAfterViewInit()
ngOnDestroy()
Q83
Q83 In Angular, which lifecycle hook is used to respond to changes in @Input properties?
ngOnChanges()
ngOnInit()
ngAfterContentChecked()
ngAfterViewChecked()
Q84
Q84 What is the purpose of the ngAfterViewInit lifecycle hook in Angular?
To clean up the component before its destruction
To react to the initialization of the component's views
To handle changes after the view is checked
To execute code after the component's content is initialized
Q85
Q85 How does the ngOnDestroy lifecycle hook help in Angular applications?
It provides a method to store component state
It is used to perform custom initialization logic
It is called before Angular destroys the component
It initializes the component's child elements
Q86
Q86 In which scenario would you use the ngAfterContentChecked lifecycle hook in Angular?
When you need to react after Angular checks the content projected into the component
To modify component properties after they are checked
Before the view is initialized
After the component is destroyed
Q87
Q87 Which Angular lifecycle hook should be used to unsubscribe from an Observable to prevent memory leaks?
ngOnInit()
ngOnChanges()
ngOnDestroy()
ngAfterViewInit()
Q88
Q88 In Angular, how do you detect when a component's view has been fully initialized?
Implement the ngAfterViewInit lifecycle hook
Use the ngOnInit lifecycle hook
Check the view in the ngOnChanges hook
Observe changes in the DOM
Q89
Q89 How can you respond to changes in a component's @Input properties in Angular?
Implement the ngOnChanges lifecycle hook
Use a setter for the input property
Use the ngOnInit lifecycle hook
Observe changes in the component's state
Q90
Q90 If changes to data are not reflected in the view, which lifecycle hook might be incorrectly implemented?
ngOnChanges()
ngOnInit()
ngAfterViewInit()
ngDoCheck()