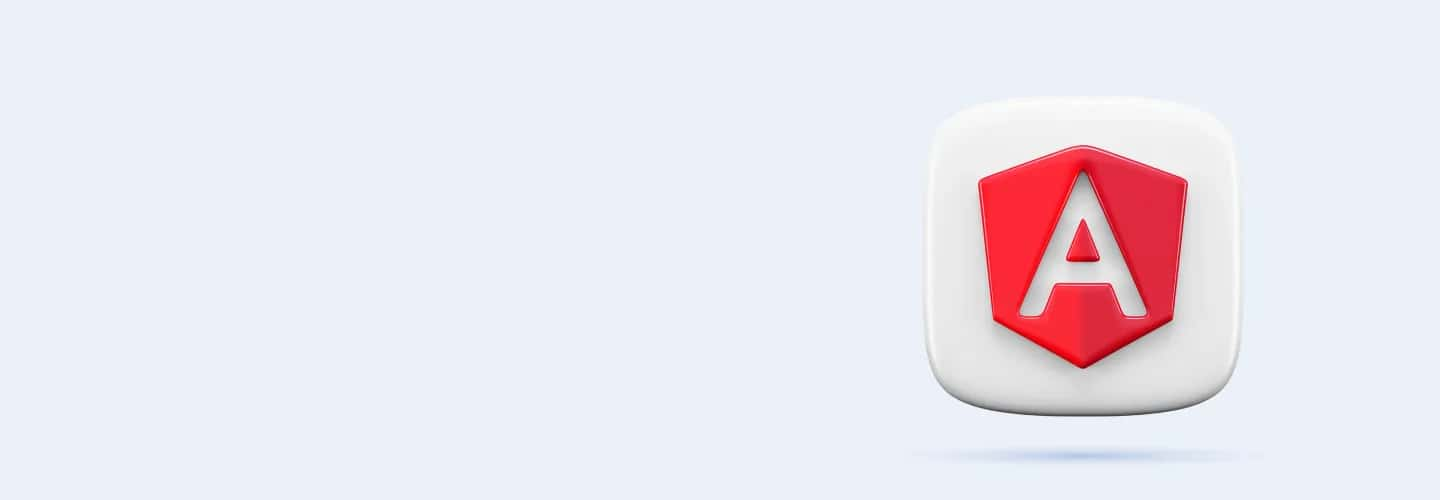
Q31
Q31 What is the main use of the *ngFor directive in Angular?
To iterate over a set of items
To check a condition
To bind a property
To catch an event
Q32
Q32 What is the purpose of the trackBy function in an *ngFor directive?
To optimize performance by tracking items with a unique identifier
To sort items
To filter items
To duplicate items
Q33
Q33 What will be the displayed value when using the following Angular template syntax: {{ 5 + 3 }}?
8
"5 + 3"
53
Undefined
Q34
Q34 What is the result of the following binding in Angular:
[src]="user.imageUrl"?
The user.imageUrl string will be set as the text content of the element
The src attribute of the element will dynamically bind to the user.imageUrl property
The image will be displayed as a user avatar
An error, as it's an incorrect syntax
Q35
Q35 In Angular, what is the output of if the message property is 'Hello World'?
A paragraph with 'Hello World'
A paragraph with 'message'
A blank paragraph
An error
Q36
Q36 If a binding like {{username}} displays nothing, what might be the issue?
The username property is not defined in the component
Incorrect syntax
The data type of username is wrong
username is a private variable in the component
Q37
Q37 When the console shows an error "Can't bind to 'ngModel' since it isn't a known property of 'input'",
what is a possible cause?
The component is missing an import for FormsModule or ReactiveFormsModule
The ngModel directive is spelled incorrectly
There's a syntax error in the template
The input element is not properly closed
Q38
Q38 What is the primary purpose of services in Angular?
To manage data state
To handle user interactions
To facilitate communication between components
To style the application
Q39
Q39 In Angular, what is Dependency Injection (DI)?
A design pattern for data binding
A method for creating responsive layouts
A technique for creating services
A design pattern that allows a class to request dependencies from external sources
Q40
Q40 Which decorator is used to inject a service into an Angular component or another service?
@Inject
@Injectable
@Input
@Output
Q41
Q41 How does Angular's hierarchical injector system work?
Child injectors can override instances of services from parent injectors
Services are global and shared across the entire application
Injectors do not follow a hierarchy
Each component has its own injector
Q42
Q42 What is the significance of the 'providedIn' property in Angular services?
It specifies the module in which the service should be provided
It determines the scope of the service
It defines the dependencies of the service
It sets the initialization time of the service
Q43
Q43 What is the correct syntax to inject a service into an Angular component?
constructor(private myService: MyService) {}
constructor(public myService: MyService) {}
myService: new MyService()
Inject(MyService)
Q44
Q44 In Angular, how do you provide a service at the component level?
Add the service to the 'providers' array of the @NgModule decorator
Declare the service in the component's 'providers' array
Import the service in the component's constructor
Declare the service as a global variable
Q45
Q45 How can a shared service be used to communicate between unrelated components in Angular?
By using a shared module
By setting global variables
By using an event emitter in the service
By passing data through URL parameters
Q46
Q46 If a service injected into an Angular component is undefined, what is a possible cause?
The service is not provided in any module
The service is not imported in the component
The service does not have the @Injectable decorator
The service is provided in multiple modules
Q47
Q47 When encountering "NullInjectorError:
No provider for MyService", what should be checked first?
If MyService is imported correctly in the component
If MyService is declared in the @NgModule decorator
If MyService is provided in the module where it's injected
If MyService is annotated with @Injectable
Q48
Q48 What is the primary purpose of the Angular Router?
Managing state within the application
Styling and animations
Navigating between views within an application
Data binding between components
Q49
Q49 In Angular, how is a route defined in the routing module?
Using a @Route decorator
With an array of path and component pairs
Through a routing service
In the component metadata
Q50
Q50 What is the purpose of the
To define where to render the component of the active route
To display a list of available routes
To create a new route
To link to different parts of the application
Q51
Q51 How do you pass data to a route in Angular?
Using query parameters
Through services
By directly accessing the component properties
By using local storage
Q52
Q52 What is a 'Route Guard' in Angular?
A method to optimize route performance
A feature for styling routes
A tool for debugging routes
A service that controls navigation to a route based on certain conditions
Q53
Q53 Which Angular directive is used for linking to a different route?
Q54
Q54 How do you programmatically navigate to a different route in Angular?
Using the navigate() method of the Router service
By changing the window.location URL
Using the redirectTo property in a route definition
Through an HTTP request to the server
Q55
Q55 In Angular, how do you specify a parameterized route, like for a user profile?
/user/:id in the route path
/user/{id}
/user/?id=
/user/id=
Q56
Q56 If an Angular application shows a blank page instead of the desired route, what is a common issue to check?
Incorrect base href in index.html
Syntax error in the template
Missing import for the component
Wrong path in the service
Q57
Q57 When encountering "Cannot match any routes" error in Angular, what should you check first?
If the routes are defined correctly in the routing module
If the components are declared in the app module
If the server is running
If the URL is correctly formed in the browser
Q58
Q58 In Angular, what is the primary difference between template-driven and reactive forms?
The way they handle data binding
The way they are created in the template
The way they manage form control objects
The modules they belong to
Q59
Q59 Which module must be imported to use reactive forms in Angular?
FormsModule
HttpClientModule
BrowserModule
ReactiveFormsModule
Q60
Q60 In a reactive form, how do you access a form control's value in an Angular component?
Using the value property of the form control
Through the ngModel binding
By accessing the DOM directly
Using an event binding