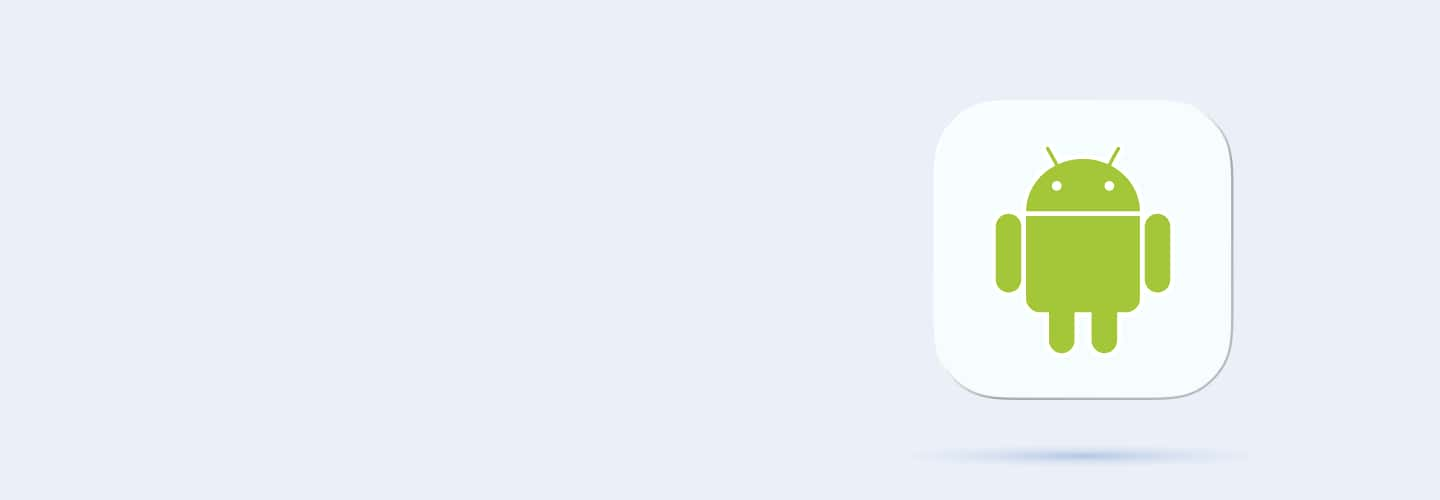
Q121
Q121 What is the purpose of the Android Keystore system?
To store sensitive data such as passwords and cryptographic keys
To manage app files
To handle database encryption
To manage UI components
Q122
Q122 What is a common risk associated with storing sensitive data in Shared Preferences?
It is encrypted by default
It can be accessed by other apps
It is too slow for real-time operations
It requires a password
Q123
Q123 Which Android component is most vulnerable to security risks if not properly secured?
Activities
Content Providers
Services
Fragments
Q124
Q124 How do you encrypt data before storing it in Shared Preferences?
Use Base64 encoding
Use Android's encryption libraries to encrypt and decrypt data
Use a third-party library
Encrypt using a password
Q125
Q125 How do you securely store a password in Android using the Keystore system?
Store the password directly in Shared Preferences
Encrypt the password using AES and store the key in Keystore
Use a hash function
Use Base64 encoding
Q126
Q126 An Android app crashes when trying to decrypt data. What is the most likely cause?
Incorrect encryption algorithm
Key used for decryption is incorrect or unavailable
The app has no internet
The data is too large
Q127
Q127 A Content Provider exposes sensitive data to unauthorized apps. What could be the cause?
The Content Provider is not encrypted
Permissions were not set properly
The device is rooted
The data is corrupted
Q128
Q128 What is the purpose of Unit Testing in Android?
To test individual units of code
To test the entire application
To check app performance
To verify UI layouts
Q129
Q129 What tool is commonly used for debugging Android apps?
Android Studio Profiler
Gradle
Logcat
JUnit
Q130
Q130 What is the difference between an Instrumented Test and a Local Unit Test in Android?
Instrumented Test runs on a local JVM
Local Unit Test runs on a device
Instrumented Test runs on a device, Local Unit Test runs on JVM
There is no difference
Q131
Q131 Which testing framework is used for writing UI tests in Android?
Espresso
JUnit
Mockito
Robolectric
Q132
Q132 How do you log messages for debugging in Android?
System.out.println()
logMessage();
Log.d("TAG", "message");
printMessage("message");
Q133
Q133 How do you write a basic unit test in Android using JUnit?
@Test
@TestUnit
@JUnitTest
@TestRun
Q134
Q134 An app crashes on launch. What is the first step in debugging this issue?
Check the manifest
Check Logcat for errors
Restart Android Studio
Check Gradle build file
Q135
Q135 A test in your Android app is failing intermittently. What could be a possible reason?
Incorrect assertions
Flaky test due to asynchronous code
The test is written correctly
The test is too fast
Q136
Q136 What is the primary purpose of performance optimization in Android apps?
To improve battery life
To reduce memory usage
To enhance user experience and app responsiveness
To increase app size
Q137
Q137 What is the effect of "overdrawing" on Android app performance?
It improves app performance
It slows down rendering and reduces frame rate
It increases battery usage
It enhances GPU usage
Q138
Q138 How does the Android garbage collector affect app performance?
It clears unnecessary data from memory
It may cause UI jank due to pauses in app execution
It boosts app speed
It optimizes CPU usage
Q139
Q139 What is the purpose of using "lazy loading" in Android app optimization?
To load resources only when they are needed
To preload all resources at the start
To keep all data in memory
To speed up UI rendering
Q140
Q140 How do you optimize a ListView for better performance when scrolling?
Use a RecyclerView
Use a custom Adapter
Enable nested scrolling
Use ViewHolder pattern
Q141
Q141 How do you reduce memory leaks in Android applications?
Use static variables
Use WeakReference when holding context
Disable garbage collection
Reduce code execution speed
Q142
Q142 An app is experiencing slow UI rendering. What is the first step in identifying the performance bottleneck?
Use Android Profiler
Clear the app's cache
Rebuild the project
Optimize the layouts
Q143
Q143 An app is consuming too much memory and crashes frequently. What is the most likely cause?
The app has too many services running
The app is not handling background tasks properly
Memory leaks
The app is performing too many network requests
Q144
Q144 What is the purpose of signing an APK in Android before publishing it?
To improve app performance
To identify the developer and ensure app integrity
To encrypt app resources
To enable app updates
Q145
Q145 Which file must be included with your Android app to ensure it can be published on Google Play?
AndroidManifest.xml
proguard-rules.pro
gradle.properties
keystore.jks
Q146
Q146 What is the significance of enabling ProGuard in an Android app before releasing it?
It reduces app size and optimizes performance
It prevents unauthorized access to the app
It encrypts sensitive user data
It obfuscates code and optimizes the APK
Q147
Q147 How do you create a signed APK in Android Studio?
Build > Sign APK
Build > Generate Signed APK
Build > Create Signed APK
Tools > Generate Signed APK
Q148
Q148 How do you enable ProGuard in an Android project?
Add proguard-rules.pro file
Enable it in AndroidManifest.xml
Add "minifyEnabled true" in build.gradle
Set it in gradle.properties
Q149
Q149 An app crashes on certain devices after being published. What could be the most likely cause?
The app is not optimized for all screen sizes
The app's permissions are not granted
Device-specific issues with certain hardware
The APK is unsigned
Q150
Q150 A published Android app fails to update on users' devices. What could be the cause?
The app was not signed correctly
The version code was not incremented
The APK size is too large
The app is no longer compatible with older devices