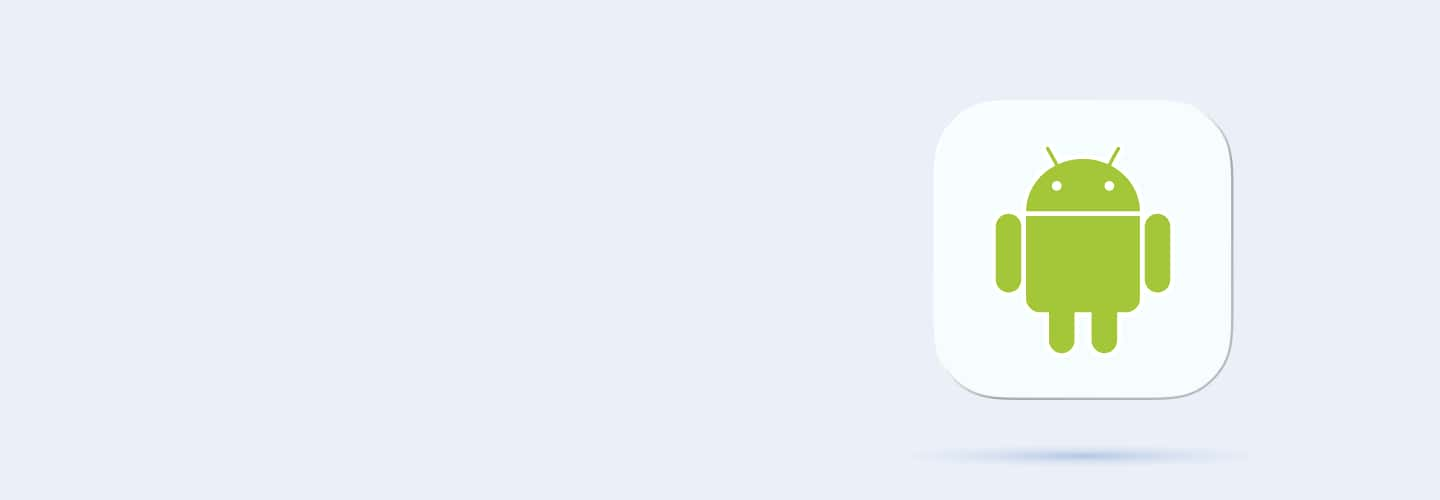
Q91
Q91 An app crashes when making a network call on the main thread. What is the most likely cause?
No internet connection
Network call is blocking the main thread
Invalid API key
Timeout error
Q92
Q92 What is the purpose of a Service in Android?
To handle UI components
To run long-running tasks in the background
To manage the app's lifecycle
To store data
Q93
Q93 What is the difference between a started Service and a bound Service in Android?
Started Service runs indefinitely, Bound Service is tied to a component
Started Service handles user input, Bound Service doesn't
Started Service has a UI, Bound Service does not
There is no difference
Q94
Q94 Which type of Service is used to perform tasks in response to explicit user interaction in Android?
Started Service
Foreground Service
Bound Service
Background Service
Q95
Q95 What is the primary benefit of using WorkManager in Android for background tasks?
It handles large file uploads
It simplifies scheduling reliable background tasks
It manages memory
It handles network requests
Q96
Q96 When should you use a JobScheduler over a Service in Android?
When the task requires UI updates
When background tasks need to be scheduled and deferred
When handling network connections
When tasks need to be run immediately
Q97
Q97 How do you start a Service in Android?
startService(new Intent(this, MyService.class));
startBackgroundService();
runService();
bindService();
Q98
Q98 How do you create a foreground Service in Android?
Start the Service and call startForeground()
Bind the Service to an Activity
Call startForegroundService()
Use a background thread
Q99
Q99 How do you stop a Service in Android?
stopService();
terminateService();
stopForegroundService();
killService();
Q100
Q100 Why would an Android Service fail to start?
The Service is not declared in AndroidManifest
The Service is missing a layout file
The app has no internet
The Service uses too much memory
Q101
Q101 An Android app crashes when trying to bind to a Service. What is a likely cause?
The Service is already running
The Service is not declared in the manifest
The Service is running on the main thread
The app has no permissions
Q102
Q102 A Foreground Service crashes after a long time. What could be a likely cause?
The Service is running on a background thread
The notification was removed
The Service was stopped manually
The system terminated it to free resources
Q103
Q103 What is a Notification in Android?
A way to store data
A pop-up window
A message displayed outside of the app's UI
A background service
Q104
Q104 Which method is used to issue a notification in Android?
createNotification()
sendNotification()
notify()
buildNotification()
Q105
Q105 What is the purpose of Notification channels in Android?
To group notifications by category
To enable multiple notifications at once
To manage background tasks
To store user settings
Q106
Q106 Which Android version introduced Notification Channels?
Android Marshmallow
Android Oreo
Android Nougat
Android Pie
Q107
Q107 How do you create a notification in Android?
NotificationCompat.Builder(context)
Notification.create()
Notification.new(context)
Notification.Builder(context)
Q108
Q108 How do you set a sound for a notification in Android?
notification.sound("file");
builder.setSound(uri);
builder.addSound(uri);
notification.setAudio(uri);
Q109
Q109 How do you create an action button in a notification?
builder.addAction(icon, title, pendingIntent);
builder.addButton(icon, title, pendingIntent);
builder.setButton(icon, title, intent);
notification.addAction(icon, title, pendingIntent);
Q110
Q110 A notification is not appearing in the status bar. What is the most likely reason?
The notification does not have a channel (Android 8.0 and above)
The app is in the background
The app has no internet connection
The device is in airplane mode
Q111
Q111 A notification sound is not playing when a new notification is received. What is a likely cause?
The app is not running
The sound file is corrupted
The notification settings are muted
The sound file is too large
Q112
Q112 What is a Fragment in Android?
A background service
A UI component that can be reused in multiple activities
A database handler
An asynchronous task
Q113
Q113 How does a Fragment communicate with its parent Activity in Android?
Through Intents
Through a direct method call
Through a Broadcast Receiver
Through interfaces
Q114
Q114 When should you use a Fragment instead of an Activity?
When you need an independent screen
When the UI needs to be dynamic and reusable within an activity
When you need background processing
When you need complex UI management
Q115
Q115 Which method is called when a Fragment is added to an activity?
onCreate()
onAttach()
onAdd()
onInflate()
Q116
Q116 How do you add a Fragment to an activity in Android?
Using startFragment()
Using FragmentTransaction.add()
Using Fragment.add()
Using startFragmentTransaction()
Q117
Q117 How do you replace an existing Fragment with another Fragment?
fragment.replace()
FragmentTransaction.replace()
FragmentManager.switch()
FragmentTransaction.switch()
Q118
Q118 A Fragment does not appear in the activity after adding it. What could be the most likely cause?
The Fragment is not declared in the manifest
The Fragment is not added to the layout file
The Fragment is not attached to the activity
The Fragment is not visible
Q119
Q119 An app crashes when trying to access a Fragment's UI component. What could be a likely cause?
The Fragment is not attached to the activity
The Fragment's view hierarchy is not initialized
The Fragment has no UI
The Fragment is null
Q120
Q120 What is the primary purpose of Android's permission system?
To ensure user privacy and security
To manage background services
To handle app updates
To improve app performance