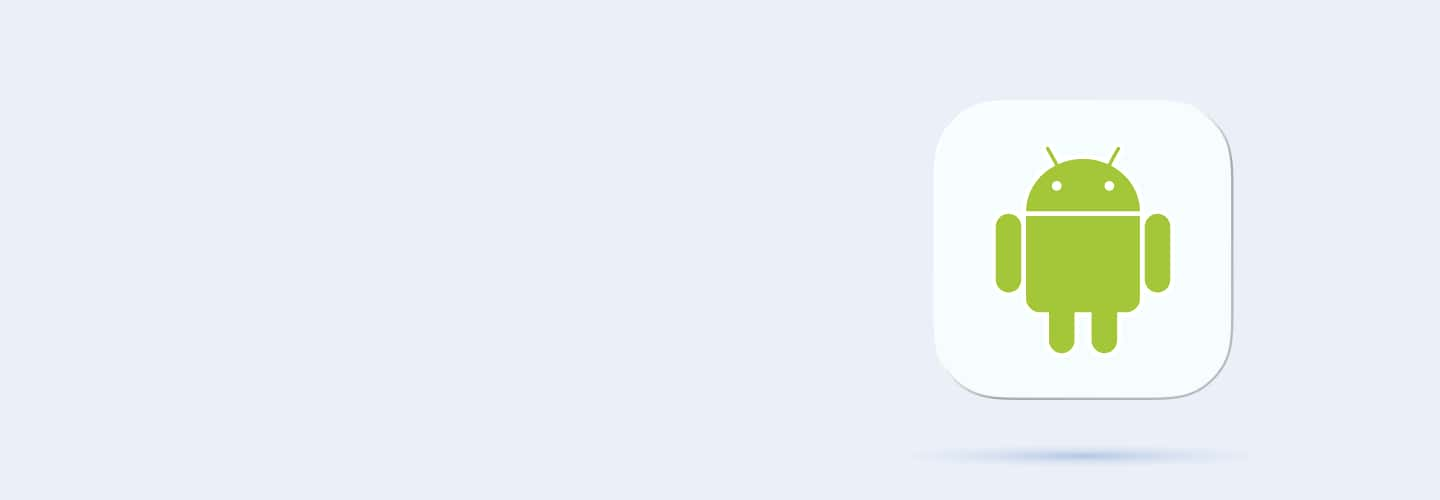
Q31
Q31 Android Studio throws a 'Gradle sync failed' error. What is the most probable cause?
Gradle is not installed
The project files are missing
The Gradle version is incompatible or network issues
The AndroidManifest is corrupted
Q32
Q32 What is an Activity in Android?
A component that allows background services
A component that represents a single screen with a user interface
A component to handle database queries
A background process handler
Q33
Q33 What is an Intent in Android?
A message passing mechanism between components
A method for managing lifecycle states
A tool to manage UI layouts
A debugging tool
Q34
Q34 Which method is used to launch a new Activity in Android?
startActivity()
launchIntent()
startApp()
openActivity()
Q35
Q35 What is the purpose of the onCreate() method in an Android Activity?
To manage user permissions
To perform operations when the Activity is first created
To close the Activity
To handle background services
Q36
Q36 How do you pass data between two Activities in Android?
Using a Service
Using an Intent
Using a Fragment
Using a Content Provider
Q37
Q37 Which lifecycle method is called when an Activity becomes visible to the user?
onStart()
onCreate()
onResume()
onPause()
Q38
Q38 Which method is used to send data back from an Activity to its parent?
setResult()
finishActivity()
sendIntent()
returnResult()
Q39
Q39 How do you retrieve data from an Intent in the receiving Activity?
getIntent().getData()
getIntent().getExtras()
startIntent().getData()
getExtrasFromIntent()
Q40
Q40 How do you create an implicit Intent to open a web page in Android?
Intent intent = new Intent(Intent.ACTION_WEB_SEARCH);
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse("http://example.com"));
Intent intent = new Intent(Intent.ACTION_SEARCH);
Intent intent = new Intent(Intent.ACTION_MAIN);
Q41
Q41 An Activity crashes after being started. What is the first place to check for possible issues?
MainActivity.java
AndroidManifest.xml
Gradle build file
Logcat
Q42
Q42 What could cause an Activity to not appear when you try to start it?
The Activity is not declared in the AndroidManifest.xml
The device's battery is low
The layout file is missing
The app has no internet access
Q43
Q43 An Intent fails to pass data between two Activities. What is the most likely cause?
The wrong key was used in the putExtra() method
The Intent is declared in the manifest
The Activities are not visible
The device is in airplane mode
Q44
Q44 Which layout is the simplest for arranging UI components in a single row or column in Android?
RelativeLayout
ConstraintLayout
LinearLayout
FrameLayout
Q45
Q45 Which layout allows positioning of UI elements relative to each other or parent?
FrameLayout
ConstraintLayout
TableLayout
GridLayout
Q46
Q46 What is the purpose of the 'wrap_content' attribute in Android layouts?
To match the parent's width or height
To make the view as large as its parent
To make the view just large enough to fit its content
To hide the view
Q47
Q47 Which layout is best suited for creating overlapping UI components?
FrameLayout
TableLayout
LinearLayout
GridLayout
Q48
Q48 What does the 'match_parent' value for layout width or height signify?
The view will expand to fill the parent
The view will be hidden
The view will wrap around its content
The view will be centered
Q49
Q49 In a ConstraintLayout, which attribute is used to define the connection between two UI components?
layout_alignParent
layout_constraintEnd_toEndOf
layout_relativeTo
layout_connect
Q50
Q50 How do you set the margin of a Button in XML?
android:layout_margin="16dp"
layout_margin="16dp"
android:margin="16dp"
view_margin="16dp"
Q51
Q51 How do you set a background color for a TextView in XML?
android:background="@color/colorPrimary"
android:layout_backgroundColor="@color/colorPrimary"
android:backgroundColor="blue"
android:background="@colorPrimary"
Q52
Q52 How do you align a Button to the center of its parent in a RelativeLayout?
android:layout_centerInParent="true"
android:layout_alignParentCenter="true"
android:layout_alignInCenter="true"
android:layout_center="true"
Q53
Q53 A TextView is not visible in the app, although it is present in the layout file. What is the most likely cause?
The TextView has no ID
The TextView's text color is the same as the background
The TextView is not initialized
The TextView is in a FrameLayout
Q54
Q54 A Button is not aligned as expected in a ConstraintLayout. What is the most likely cause?
The Button's constraints are not properly set
The Button has no text
The Button has no ID
The Button is disabled
Q55
Q55 An app crashes when trying to inflate a custom view layout. What is a common cause?
Incorrect view ID
Layout file not found
The layout is missing required attributes
The app has no permissions
Q56
Q56 Which Android widget is used to display a scrollable list of items?
TextView
ListView
Button
ImageView
Q57
Q57 What is the function of the Spinner widget in Android?
To display text
To display a list of selectable options
To trigger background services
To display images
Q58
Q58 What does the CheckBox widget allow users to do?
Select a single option
Select multiple options
Open a new activity
Submit a form
Q59
Q59 Which widget is used to create a button that can trigger a user action?
TextView
Button
ImageButton
SeekBar
Q60
Q60 What is the purpose of the Android ProgressBar widget?
To display text
To display the progress of an operation
To show a list of items
To select multiple options