Top Vue JS Interview Questions for Freshers
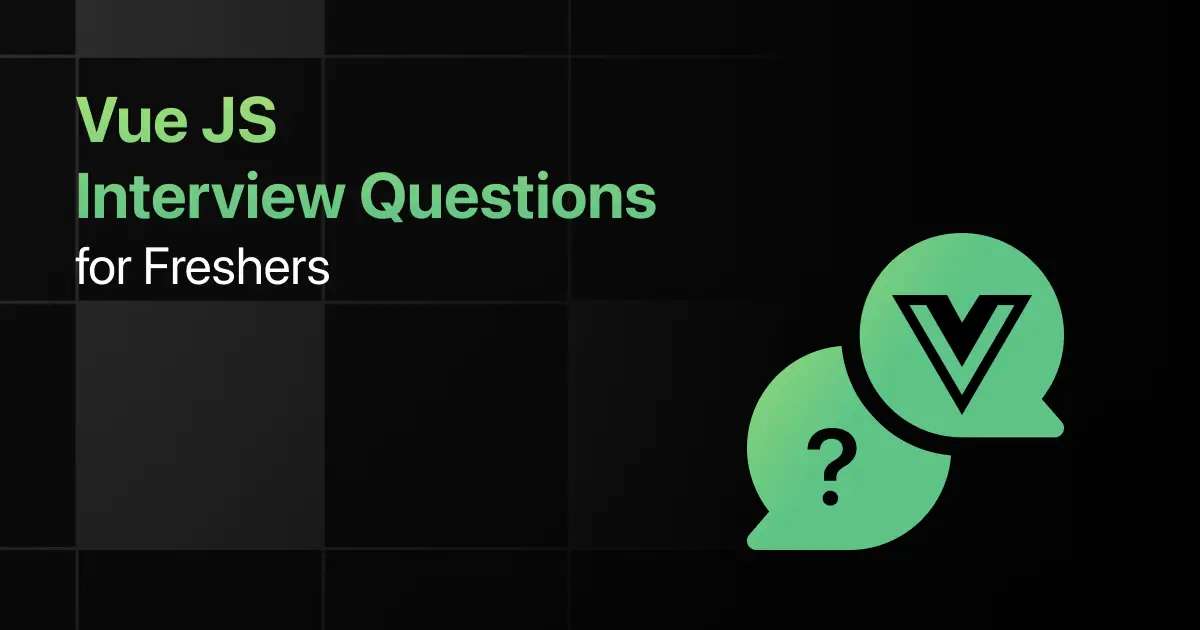
Are you preparing for your first Vue.js interview and wondering what questions you might face?
Understanding the key Vue.js interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Vue.js interview questions and answers for freshers and make a strong impression in your interview.
Practice Vue JS Interview Questions and Answers
Below are the top 50 Vue JS interview questions for freshers with answers:
1. What is Vue.js?
Answer:
Vue.js is a progressive JavaScript framework for building user interfaces. It focuses on the view layer and can easily be integrated into large projects.
2. What are the key features of Vue.js?
Answer:
Some key features are its reactive data binding, component-based architecture, and support for directives like v-if, v-for, and v-model.
3. How do you create a Vue.js instance?
Answer:
You can create a Vue instance by initializing it using the new Vue() constructor.
el: ‘#app’,
data: {
message: ‘Hello Vue!’
}
});
4. What is the virtual DOM in Vue.js?
Answer:
The virtual DOM is a lightweight copy of the real DOM. Vue.js uses it to optimize rendering by only updating parts of the DOM that need changes.
5. What is a Vue component?
Answer:
Components in Vue.js are reusable pieces of code that encapsulate HTML, CSS, and JavaScript.
template: ‘<p>Hello from the component!</p>’
});
6. How does two-way data binding work in Vue.js?
Answer:
Two-way data binding in Vue.js is achieved using the v-model directive, which binds the form input with the Vue instance data.
<p>{{ message }}</p>
7. What are Vue.js directives?
Answer:
Directives are special tokens in the markup that tell the library to do something to a DOM element.
8. What is v-if in Vue.js?
Answer:
The v-if directive is used to conditionally render elements based on a boolean value.
9. What is v-for in Vue.js?
Answer:
The v-for directive is used to loop over an array or object and render elements based on each item.
10. What is v-bind in Vue.js?
Answer:
v-bind is used to bind HTML attributes to data in Vue.js.
11. What is v-model in Vue.js?
Answer:
v-model provides two-way data binding between form inputs and the component’s data.
12. How can you pass data between components in Vue.js?
Answer:
Props allow data to be passed from a parent to a child component.
13. What is a computed property in Vue.js?
Answer:
Computed properties are cached based on their dependencies and only re-evaluate when necessary.
reversedMessage() {
return this.message.split(”).reverse().join(”);
}
}
14. What is a watcher in Vue.js?
Answer:
Watchers observe reactive data and execute a function when the data changes.
question: function (newQuestion) {
this.getAnswer();
}
}
15. What is Vue Router?
Answer:
Vue Router is the official router for Vue.js used to create navigation and routes in a Vue app.
16. What is the Vue CLI?
Answer:
Vue CLI is a command-line tool that helps to set up a Vue.js project with build configurations.
17. How do you define methods in Vue.js?
Answer:
Methods are defined inside the methods object of a Vue instance.
greet() {
return ‘Hello Vue.js!’;
}
}
18. How do you handle events in Vue.js?
Answer:
Events are handled using the v-on directive or @ shorthand.
19. What are lifecycle hooks in Vue.js?
Answer:
Lifecycle hooks are functions that are executed at specific stages of a component’s lifecycle, such as mounted, created, or updated.
20. What is the difference between v-show and v-if?
Answer:
v-show toggles the visibility of an element, while v-if actually adds or removes the element from the DOM.
21. What is Vuex?
Answer:
Vuex is a state management library for Vue.js that helps manage the state of an application.
22. How does Vue.js handle form validation?
Answer:
Vue.js can handle form validation by using v-model with custom validation logic inside methods.
23. How do you create a dynamic component in Vue.js?
Answer:
Dynamic components are created using the <component> tag with is attribute to switch between components.
24. How can you achieve lazy loading in Vue.js?
Answer:
Lazy loading can be implemented by using dynamic imports and Vue Router.
25. What is a slot in Vue.js?
Answer:
Slots allow components to accept dynamic content from the parent component.
26. What is a mixin in Vue.js?
Answer:
Mixins allow reusing a piece of functionality across components.
data() {
return { message: ‘Hello!’ };
}
}
27. How do you access DOM elements in Vue.js?
Answer:
DOM elements can be accessed using template refs.
28. What are filters in Vue.js?
Answer:
Filters are used to format text and are applied in the template syntax.
29. What are transitions in Vue.js?
Answer:
Transitions provide animations when elements enter or leave the DOM.
Copy code
<transition name=”fade”>
<p v-if=”show”>Hello</p>
</transition>
30. What is a scoped slot in Vue.js?
Answer:
Scoped slots allow passing data from child components to slot content in the parent component.
<p>{{ slotProps.data }}</p>
</template>
31. How do you communicate between components?
Answer:
You can use props for parent-to-child communication and emit events for child-to-parent communication.
32. What is a single-file component in Vue.js?
Answer:
A single-file component encapsulates template, script, and style in a .vue file.
<div>Hello World</div>
</template>
33. How do you install Vue.js using npm?
Answer:
You can install Vue.js using npm with the following command:
34. What is the purpose of the `key` attribute in Vue.js?
Answer:
The `key` attribute helps Vue.js track elements and efficiently update the DOM by reusing components.
35. How do you handle user input in Vue.js?
Answer:
User input can be handled using the v-model directive, which binds input elements with data properties.
36. How do you update the state in Vuex?
Answer:
You can update the state in Vuex using mutations.
37. How do you set up Vue.js with TypeScript?
Answer:
You can set up Vue.js with TypeScript using the Vue CLI by selecting TypeScript during project creation.
vue create my-project
38. What is a reactive property in Vue.js?
Answer:
Reactive properties in Vue.js automatically trigger view updates when the data changes.
39. How do you handle errors in Vue.js?
Answer:
Errors can be handled using errorCaptured lifecycle hook or by wrapping code in try-catch blocks.
40. What are watchers used for in Vue.js?
Answer:
Watchers are used to react to changes in data properties and execute code based on the change.
41. What is the role of the render function in Vue.js?
Answer:
The render function allows you to write raw JavaScript to define the structure of a Vue component instead of using the template syntax.
42. What is Vue Devtools?
Answer:
Vue Devtools is a browser extension that allows you to inspect and debug Vue.js applications.
43. What are Vue.js filters and how do you use them?
Answer:
Filters are functions that format the value of an expression in a template.
44. How do you optimize the performance of a Vue.js application?
Answer:
You can optimize performance by lazy loading components, using Vuex for state management, and avoiding unnecessary reactivity.
45. What are asynchronous components in Vue.js?
Answer:
Asynchronous components are components that are loaded only when needed, improving performance.
46. How do you fetch data in Vue.js?
Answer:
Data can be fetched using axios or fetch inside lifecycle hooks like mounted.
axios.get(‘/api/data’).then(response => {
this.data = response.data;
});
}
47. What is v-pre directive in Vue.js?
Answer:
The v-pre directive is used to skip compilation for this element and its children.
48. How do you implement a simple pagination in Vue.js?
Answer:
You can implement pagination by slicing an array of data and displaying it in chunks based on the current page.
paginatedData() {
return this.items.slice(this.startIndex, this.endIndex);
}
}
49. What is the purpose of Vue.js nextTick?
Answer:
nextTick is used to defer the execution of a callback after the DOM has been updated.
console.log(‘DOM updated’);
});
50. How do you deploy a Vue.js application?
Answer:
You can deploy a Vue.js application by building it using npm run build and hosting the output files on a web server like Nginx or Firebase Hosting.
Final Words
Getting ready for an interview can feel overwhelming, but going through these Vue.js fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Vue.js interview but don’t forget to practice Vue component basics, directives, and reactivity system-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for Vue JS?
Common Vue.js interview questions are :
- Vue components
- directives
- lifecycle hooks
- data binding
2. What are the important Vue JS topics freshers should focus on for interviews?
Freshers should focus on Vue directives, state management (Vuex), routing (Vue Router), and component-based architecture.
3. How should freshers prepare for Vue JS technical interviews?
Freshers should practice building projects, understanding component interactions, and learning Vue CLI and single-file components.
4. What strategies can freshers use to solve Vue JS coding questions during interviews?
Freshers can break down coding tasks, structure components modularly, and debug efficiently using Vue DevTools.
5. Should freshers prepare for advanced Vue JS topics in interviews?
Yes, freshers should prepare for advanced Vue topics like server-side rendering (Nuxt.js) and state management if required for the role.
Explore More Vue JS Resources
Explore More Interview Questions
- Python
- Java
- SQL
- React
- JavaScript
- C Programming
- HTML
- CSS
- Angular
- C++
- Spring Boot
- Node JS
- Excel
- C#
- DBMS
- PHP
- Linux
- Operating System
- MySQL
- Spring
- Flutter
- MongoDB
- Django
- React Native
- jQuery
- Bootstrap
- Embedded C
- DSA
- R Programming
- Hadoop
- .NET
- Power BI
- ASP.NET
- ASP.NET MVC
- Android
- Tableau
- MVC
- WordPress
- TypeScript
- Spark
- Kotlin
- Swift
- Golang
- Shell Scripting
- iOS
- Spring MVC
- Next JS
- AWS
- Kubernetes
- Docker
- Cyber Security
- Azure
- Terraform
Related Posts
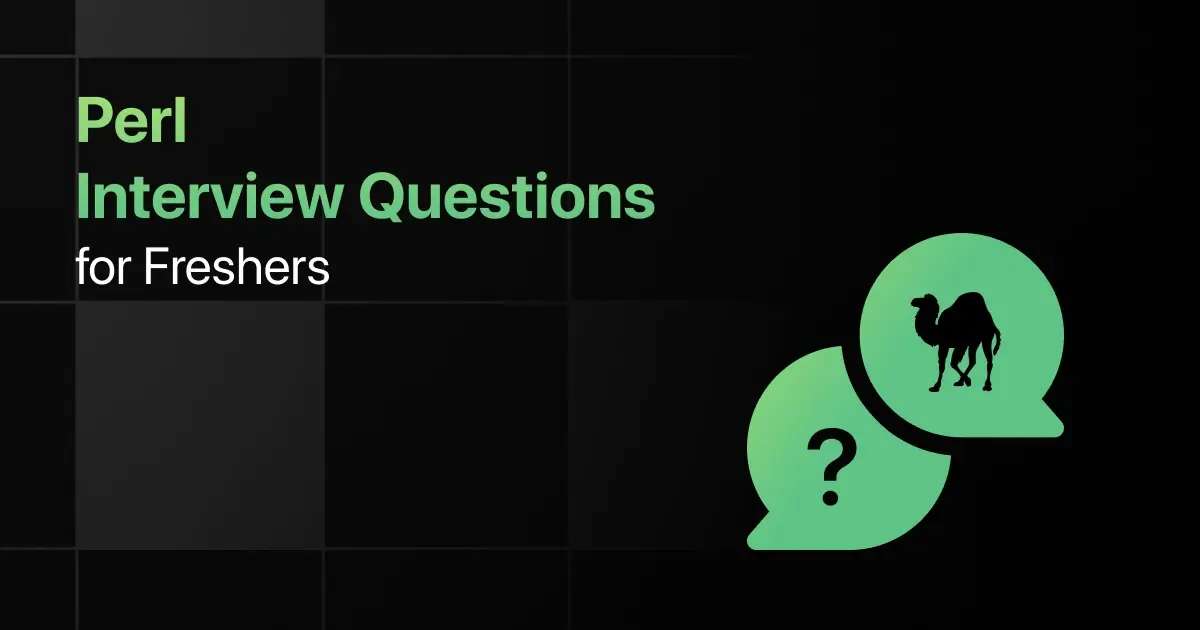
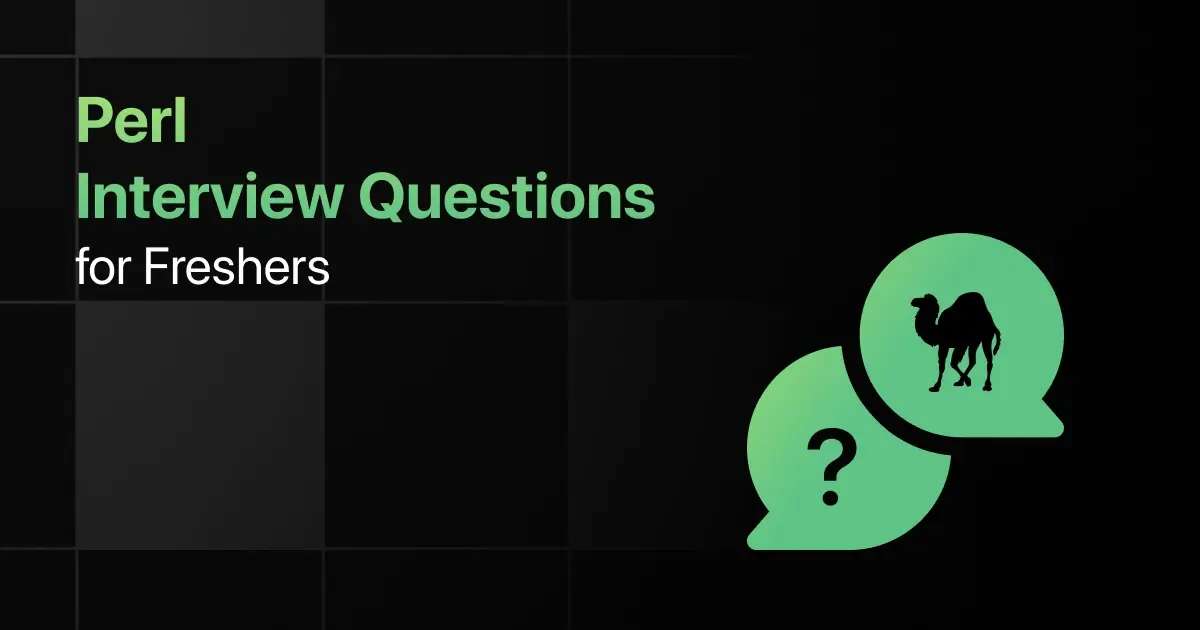
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …