Top Spark Interview Questions for Freshers
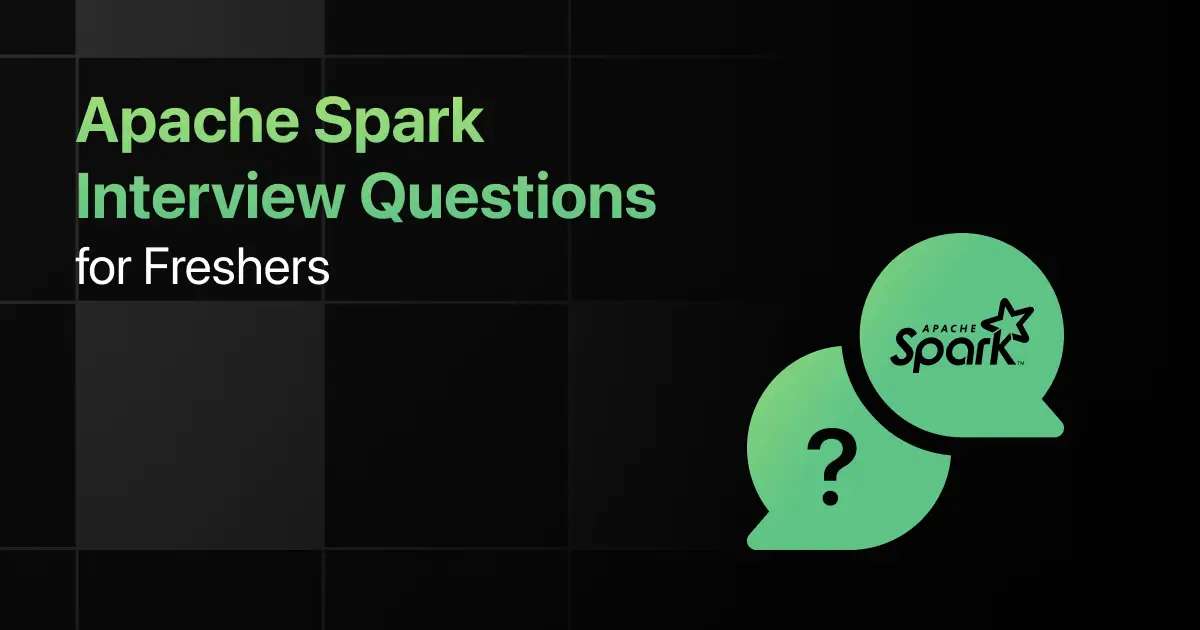
Are you preparing for your first Spark interview and wondering what questions you might face?
Understanding the key Apache Spark interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Spark interview questions and answers for freshers and make a strong impression in your interview.
Practice Spark Interview Questions and Answers
Below are the top 50 Spark interview questions for freshers with answers:
1. What is Apache Spark?
Answer:
- Apache Spark is an open-source, distributed computing system designed for fast processing of large datasets. It provides an interface for programming entire clusters with implicit data parallelism and fault tolerance.
- It supports various languages such as Python, Scala, Java, and R, and is widely used for big data analytics, machine learning, and streaming.
2. What are the key features of Apache Spark?
Answer:
Apache Spark’s key features include in-memory computation, fault tolerance, distributed data processing, support for various data sources (e.g., HDFS, Cassandra), and a rich set of APIs for SQL, streaming, machine learning, and graph processing.
3. What is an RDD in Spark?
Answer:
- RDD stands for Resilient Distributed Dataset. It is the fundamental data structure of Apache Spark, which represents a fault-tolerant collection of elements distributed across many nodes in the cluster.
- RDDs support two types of operations: transformations and actions.
4. Explain the concept of lazy evaluation in Spark.
Answer:
- Lazy evaluation means that Spark doesn’t immediately execute the transformations when they’re called. Instead, it builds a logical execution plan and waits until an action is called to actually compute the result.
- This helps optimize the execution by minimizing unnecessary data movement and computation.
5. What are transformations in Spark?
Answer:
- Transformations are operations that define a new RDD by applying a function to each element of the original RDD. They are lazily evaluated, meaning they don’t immediately compute results.
- Examples of transformations include map(), filter(), and flatMap().
6. What are actions in Spark?
Answer:
- Actions are operations that trigger the execution of transformations and return results to the driver program or save data to external storage.
- Common actions include collect(), count(), saveAsTextFile().
7. What is the difference between map() and flatMap() in Spark?
Answer:
- map() applies a function to each element of the RDD and returns a new RDD with the same number of elements.
- flatMap() applies a function that returns a sequence, and then it flattens the results, resulting in more or fewer elements than the original RDD.
rdd_map = rdd.map(lambda x: [x, x*2]) # [[1, 2], [2, 4], [3, 6]] rdd_flatmap = rdd.flatMap(lambda x: [x, x*2]) # [1, 2, 2, 4, 3, 6]
8. How does Spark achieve fault tolerance?
Answer:
Spark achieves fault tolerance through RDDs by tracking the lineage of transformations. If any partition of an RDD is lost, Spark can recompute it using the original data and the sequence of transformations applied.
9. What is a SparkContext?
Answer:
SparkContext is the entry point for any Spark application. It represents the connection to a Spark cluster, allowing the application to interact with the cluster to create RDDs, accumulators, and broadcast variables.
10. What is a DAG in Spark?
Answer:
A DAG (Directed Acyclic Graph) represents a sequence of computations that Spark must perform on the data. When you run actions, Spark builds a DAG of stages that will execute transformations and minimize data shuffling.
11. What are the different cluster managers supported by Spark?
Answer:
Spark supports four cluster managers: Standalone mode, Apache Mesos, Hadoop YARN, and Kubernetes. Each offers different levels of resource management and scalability.
12. Explain the Spark execution model.
Answer:
Spark’s execution model is based on the master-slave architecture. The driver (master) is responsible for running the main program and controlling the workers (slaves). Workers execute tasks assigned by the driver on the distributed data.
13. What is a partition in Spark?
Answer:
A partition is a logical division of data, and Spark operates on partitions of RDDs in parallel. Partitions improve performance by allowing distributed processing of large datasets.
14. How can you create an RDD in Spark?
Answer:
RDDs can be created in two ways: by loading external datasets (e.g., from HDFS, S3) or by parallelizing existing collections in the driver program.
rdd = sc.parallelize([1, 2, 3, 4, 5])
# From file
rdd_from_file = sc.textFile(“hdfs://path/to/file”)
15. What is the difference between textFile() and wholeTextFiles() in Spark?
Answer:
- textFile() reads a file line by line and returns an RDD of strings.
- wholeTextFiles() reads a directory and returns a pair RDD, where each key is the filename and each value is the file content.
16. Explain reduceByKey() in Spark.
Answer:
reduceByKey() is a transformation applied to pair RDDs (key-value pairs). It combines values with the same key using a specified associative and commutative reduction function.
rdd_reduce = rdd.reduceByKey(lambda a, b: a + b) # Output: [(“a”, 2), (“b”, 1)]
17. What is a Broadcast variable?
Answer:
Broadcast variables allow you to cache a read-only variable on each worker node rather than shipping a copy of it with each task. This is useful when you have large, read-only data that you need to use across tasks.
18. What is an Accumulator in Spark?
Answer:
- Accumulators are variables used for aggregating information across the cluster. They allow workers to update values, and only the driver can read them.
- They are commonly used for counting and summing operations.
19. Explain how Spark handles memory management.
Answer:
Spark’s memory management consists of two regions: storage memory and execution memory. Storage memory is used to cache RDDs and execution memory for computing tasks like shuffles and joins. Spark dynamically manages these two regions to avoid memory overflow.
20. What is the difference between Spark SQL and Hive?
Answer:
Spark SQL is a module of Apache Spark for structured data processing, supporting SQL queries and DataFrame API. Hive is a data warehouse infrastructure that provides SQL-like querying on Hadoop. While both tools support SQL, Spark SQL is generally faster due to in-memory computation.
21. What is a DataFrame in Spark?
Answer:
A DataFrame is a distributed collection of data organized into named columns. It is conceptually equivalent to a table in a relational database or a data frame in Python/R, and it provides more optimizations than RDDs.
22. How do you create a DataFrame in Spark?
Answer:
A DataFrame can be created from RDDs, files (JSON, CSV, Parquet), or from Hive tables.
23. What is the difference between select() and filter() in DataFrames?
Answer:
- select() is used to select specific columns from a DataFrame.
- filter() is used to filter rows based on a condition.
24. What is the difference between DataFrame and Dataset?
Answer:
DataFrame is a collection of rows and columns of data. Dataset is a strongly-typed API for structured data, providing both compile-time type safety and an object-oriented interface in Scala and Java.
25. What are the different ways to save a DataFrame in Spark?
Answer:
DataFrames can be saved in various formats such as Parquet, JSON, CSV, and ORC.
26. Explain the Catalyst optimizer in Spark SQL.
Answer:
Catalyst is an extensible query optimization framework used by Spark SQL. It helps in optimizing the execution of queries, transforming logical plans into optimized physical plans, ensuring high performance for SQL operations.
27. What is the difference between cache() and persist() in Spark?
Answer:
Both cache() and persist() store RDDs or DataFrames in memory to speed up future computations. cache() is equivalent to persist() with the default storage level of MEMORY_ONLY.vWith persist(), you can specify different storage levels such as MEMORY_AND_DISK.
28. Explain how to perform joins in DataFrames.
Answer:
Spark DataFrames support SQL-like joins including inner, outer, left, and right joins.
29. What is a SparkSession?
Answer:
SparkSession is the entry point to a Spark application and provides access to all functionalities of Spark. It replaced the SQLContext and HiveContext from previous Spark versions.
30. What are the common file formats supported by Spark?
Answer:
Spark supports several file formats including text, CSV, JSON, Parquet, ORC, Avro, and Sequence files. These formats are widely used for structured and semi-structured data processing.
31. Explain groupByKey() in Spark.
Answer:
groupByKey() groups the values for each key into a single sequence but can lead to performance degradation as it can shuffle large amounts of data.
32. How does Spark Streaming work?
Answer:
Spark Streaming works by dividing input data into micro-batches and processing each batch in near real-time. It uses DStreams (Discretized Streams), which are sequences of RDDs representing each time interval.
33. What are DStreams in Spark?
Answer:
DStreams are a high-level abstraction in Spark Streaming that represents continuous streams of data. Internally, it is a sequence of RDDs, with each RDD representing data for a particular batch interval.
34. What is the role of a checkpoint in Spark Streaming?
Answer:
Checkpointing in Spark Streaming allows you to save the state of the stream to a reliable storage system like HDFS. It helps in fault recovery by recomputing lost data.
35. What is the default batch interval in Spark Streaming?
Answer:
The default batch interval in Spark Streaming is 500 milliseconds. However, it can be configured based on the use case.
36. Explain how Spark handles shuffling.
Answer:
Shuffling in Spark is the process of redistributing data across partitions. It happens when transformations like groupByKey() or reduceByKey() are performed. Shuffling can be expensive due to disk IO and network costs.
37. What are the most common causes of performance bottlenecks in Spark?
Answer:
Common causes include unnecessary shuffling, too many small tasks, insufficient memory allocation, and improper partitioning. Optimizing data storage and avoiding wide transformations can help alleviate bottlenecks.
38. What is a narrow transformation in Spark?
Answer:
A narrow transformation is when each partition of the parent RDD is used by at most one partition of the child RDD. Examples include map() and filter().
39. What is a wide transformation in Spark?
Answer:
A wide transformation requires shuffling data across multiple partitions. This includes operations like groupByKey() or reduceByKey(), which result in a larger amount of data movement.
40. How can you avoid shuffling in Spark?
Answer:
You can avoid shuffling by minimizing wide transformations, using combiner functions with reduceByKey(), partitioning the data appropriately, and caching frequently used RDDs.
41. What is mapPartitions() in Spark?
Answer:
mapPartitions() allows you to apply a function to each partition of the RDD, giving you more control over the computation compared to map(). It’s more efficient when you want to avoid creating too many individual tasks.
42. What are coalesce and repartition in Spark?
Answer:
coalesce() reduces the number of partitions, and is used when you want to optimize computations without performing a full shuffle. repartition() increases the number of partitions and performs a full shuffle.
43. Explain foreach() in Spark.
Answer:
foreach() is an action that applies a function to each element of an RDD or DataFrame, but unlike transformations, it does not return an RDD. It’s mainly used for side effects, like saving data or printing results.
44. What is a shuffle partition in Spark?
Answer:
A shuffle partition is created during wide transformations that require shuffling data between nodes. The default number of shuffle partitions can be controlled by the spark.sql.shuffle.partitions configuration.
45. How do you control parallelism in Spark?
Answer:
Parallelism in Spark can be controlled by adjusting the number of partitions when creating an RDD or DataFrame, or using repartition() and coalesce() to change the number of partitions for computations.
46. What is speculative execution in Spark?
Answer:
Speculative execution is a feature in Spark that detects slow-running tasks and runs duplicate copies on different nodes to minimize job completion time.
47. What are Tungsten and Project Tungsten in Spark?
Answer:
Tungsten is an optimization project in Spark focused on improving the performance of Spark’s execution engine, by utilizing memory management, code generation, and better CPU utilization.
48. What is the Catalyst Optimizer in Spark?
Answer:
The Catalyst Optimizer is Spark SQL’s query optimizer. It optimizes queries by transforming logical plans into optimized physical plans that can be executed more efficiently.
49. What is the use of glom() in Spark?
Answer:
glom() transforms each partition into an array, creating an RDD of arrays. It’s useful for collecting data partition-wise rather than element-wise.
50. Explain partitioning in Spark.
Answer:
Partitioning is the process of dividing data across different nodes of a cluster. Proper partitioning improves data locality, minimizes shuffling, and increases parallelism.
Final Words
Getting ready for an interview can feel overwhelming, but going through these Spark fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Spark interview, but don’t forget to practice distributed computing concepts, RDDs, transformations, and Spark SQL-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for Spark?
Common Spark interview questions include topics like RDDs vs DataFrames, Spark architecture, transformations and actions, fault tolerance, Spark SQL, and Spark’s role in big data processing.
2. What are the important Spark topics freshers should focus on for interviews?
Freshers should focus on Spark’s core concepts like RDDs, DataFrames, transformations, actions, Spark architecture (driver and executor), fault tolerance, and how Spark handles large datasets.
3. How should freshers prepare for Spark technical interviews?
Freshers should understand Spark’s key concepts (RDDs, DataFrames), be comfortable with Spark SQL, practice coding transformations and actions, and study the internal workings of Spark, including its fault-tolerant mechanism.
4. What strategies can freshers use to solve Spark coding questions during interviews?
Freshers should break down the problem to identify necessary transformations and actions, use Spark APIs effectively, and focus on optimizing performance (e.g., using caching, partitioning). Understanding distributed computing fundamentals will help.
5. Should freshers prepare for advanced Spark topics in interviews?
Yes, freshers should prepare for advanced topics like performance tuning, optimization techniques (e.g., Catalyst optimizer), handling skewed data, and understanding Spark Streaming for real-time data processing.
Explore More Interview Questions
- Python
- Java
- SQL
- React
- JavaScript
- C Programming
- HTML
- CSS
- Angular
- C++
- Spring Boot
- Node JS
- Excel
- C#
- DBMS
- PHP
- Linux
- Operating System
- MySQL
- Spring
- Flutter
- MongoDB
- Django
- React Native
- jQuery
- Bootstrap
- Embedded C
- DSA
- R Programming
- Hadoop
- .NET
- Power BI
- ASP.NET
- ASP.NET MVC
- Android
- Tableau
- MVC
- WordPress
- TypeScript
Related Posts
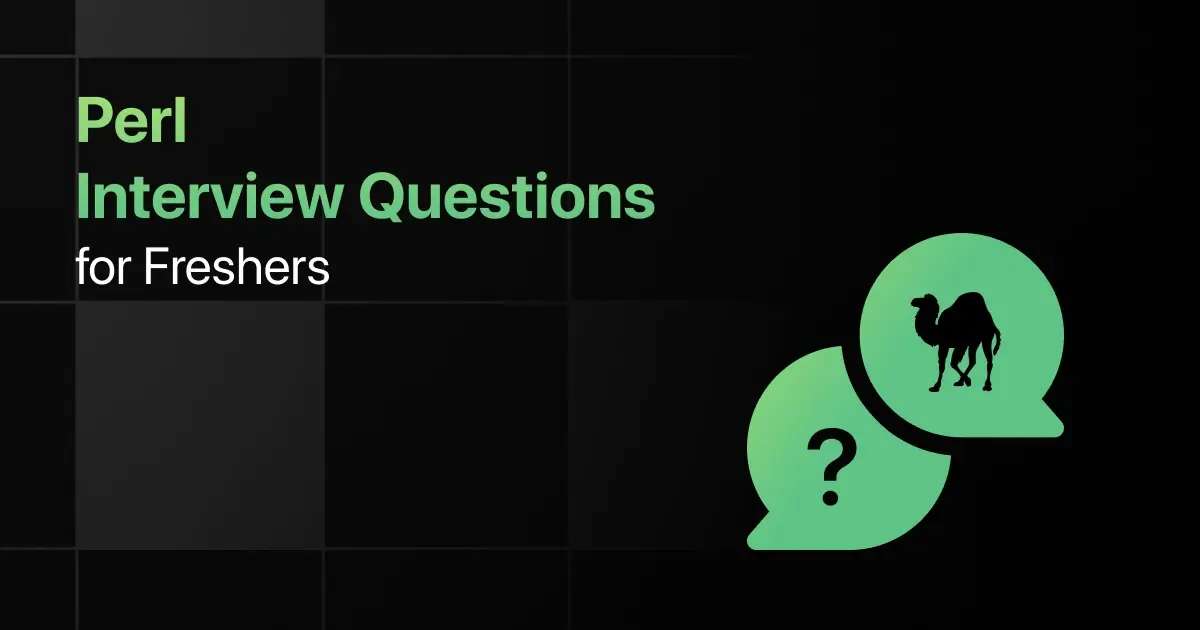
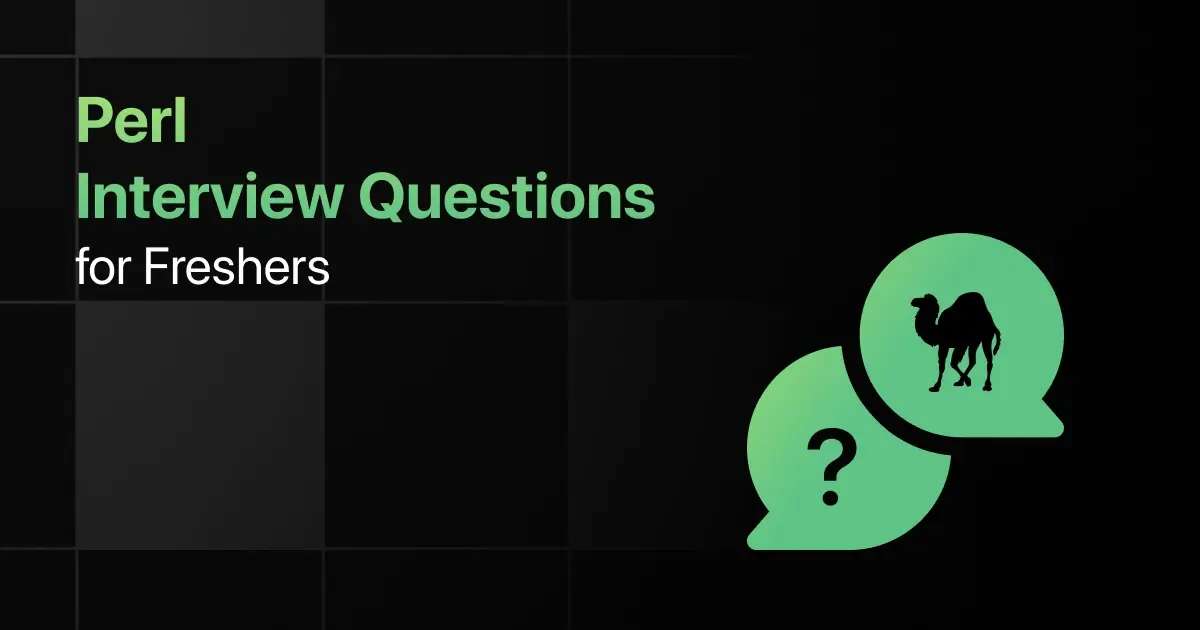
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …