Top Shell Scripting Interview Questions for Freshers
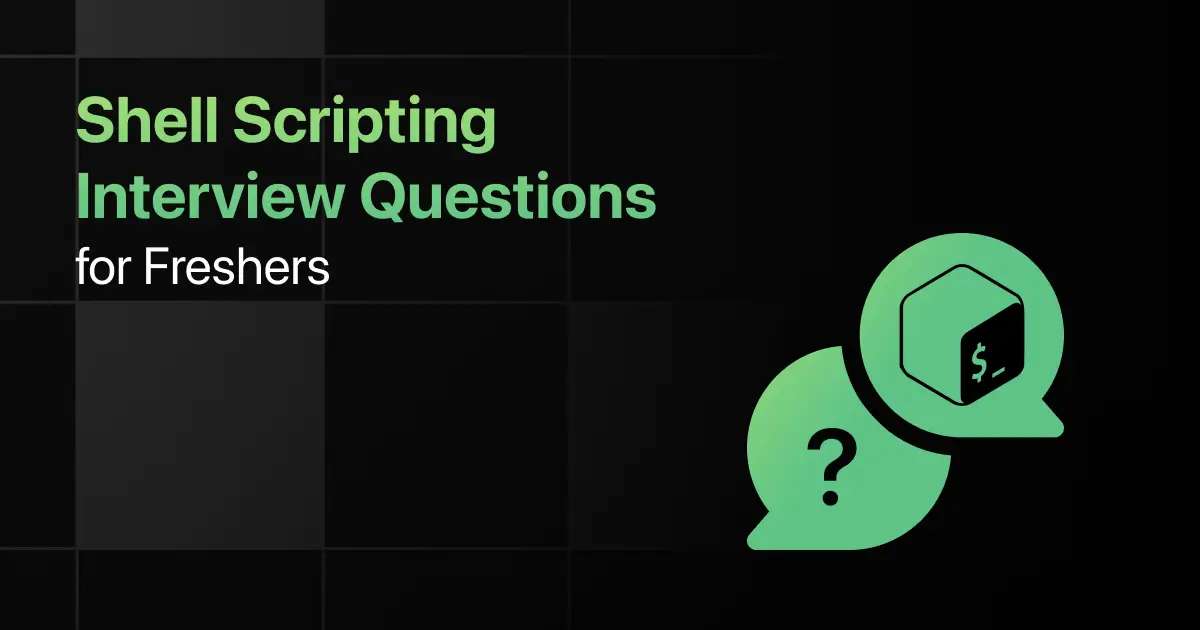
Are you preparing for your first Shell Scripting interview and wondering what questions you might face?
Understanding the key Shell Scripting interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Shell Scripting interview questions and answers for freshers and make a strong impression in your interview.
Practice Shell Scripting Interview Questions and Answers
Below are the top 50 Shell scripting interview questions for freshers with answers:
1. What is a Shell Script?
Answer:
A shell script is a text file containing a series of commands for the shell to execute. It is typically used to automate repetitive tasks in Unix/Linux systems. These scripts can include loops, conditionals, and functions.
2. How do you create a shell script?
Answer:
To create a shell script, use a text editor to write the script, starting with the shebang (#!/bin/bash). Save the file with a .sh extension, and give it executable permissions using chmod +x script.sh.
echo “Hello, World!”
3. What is the shebang (#!) in a shell script?
Answer:
The shebang (#!) indicates the interpreter that should execute the script. For example, #!/bin/bash tells the system to use the Bash shell. It must be the first line in the script for the script to execute correctly.
4. How do you execute a shell script?
Answer:
To execute a shell script, navigate to its directory and run it by typing ./script_name.sh. Make sure it has the correct executable permissions (chmod +x).
5. What are shell variables?
Answer:
Shell variables store data that can be reused within the script. Variables can store strings, numbers, and command outputs, and are typically assigned using VAR=value.
echo $my_var
6. What is the difference between local and global variables?
Answer:
A local variable is confined to the function or script it is declared in, while a global variable can be accessed throughout the script or by other scripts. Local variables are declared using the local keyword inside a function.
7. How do you pass arguments to a shell script?
Answer:
You can pass arguments to a shell script by specifying them after the script name. These arguments can be accessed inside the script using $1, $2, and so on.
echo “First argument: $1”
8. What is $0 in shell scripting?
Answer:
$0 holds the name of the script itself. This is useful when you need to refer to the script’s name within itself for logging or help messages.
9. How do you read user input in a shell script?
Answer:
Use the read command to capture user input during script execution. This allows interaction between the script and the user.
read -p “Enter your name: ” name
echo “Hello, $name!”
10. How do you create a function in a shell script?
Answer:
Functions in shell scripts allow you to group commands into reusable blocks. A function is declared using the function keyword or just the function name followed by ().
echo “Hello, $1”
}
greet “John”
11. How do you check if a file exists in a shell script?
Answer:
Use the -e operator with an if statement to check if a file exists. This is useful for handling files in conditional logic.
echo “File exists”
else
echo “File does not exist”
fi
12. What is the difference between [ ] and [[ ]] in shell scripting?
Answer:
[ ] is the test command that evaluates expressions, while [[ ]] is an enhanced test command that supports pattern matching and regular expressions, making it more powerful for conditionals.
13. How do you perform string comparison in a shell script?
Answer:
String comparison can be done using = and != for equality and inequality checks. Use -z to check if a string is empty and -n to check if it is non-empty.
echo “Strings are equal”
fi
14. What is the use of echo command in shell scripting?
Answer:
The echo command is used to print text or variables to the terminal. It is widely used for displaying messages, outputting the result of commands, or logging information during script execution.
15. How do you use loops in shell scripting?
Answer:
Shell scripts support for, while, and until loops for repetitive execution of commands. Loops help in tasks like iterating through file lists or processing user input.
echo “Iteration $i”
done
16. What is the difference between while and until loops?
Answer:
A while loop executes as long as the condition is true, while an until loop executes as long as the condition is false. The logic is inverted between the two.
17. What is the significance of exit codes in shell scripting?
Answer:
Exit codes determine the success or failure of a command. By convention, 0 represents success, and any non-zero code indicates an error. Scripts can use exit to specify custom exit codes.
echo “Success”
else
echo “Failure”
fi
18. How do you handle errors in shell scripting?
Answer:
You can handle errors using conditional checks (if, &&, ||), or by using the set -e option, which makes the script exit on the first error. It ensures reliable script execution by halting on errors.
19. How do you write comments in shell scripts?
Answer:
Comments are written with a # symbol and are ignored by the shell. They are useful for documenting the script and making it easier to understand.
20. What is a positional parameter in shell scripting?
Answer:
Positional parameters allow you to pass arguments to a script. $1, $2, etc., represent the first, second, and subsequent arguments passed to the script. These help in handling user input or data processing.
21. How do you redirect output to a file in shell scripting?
Answer:
Output can be redirected to a file using > for overwriting and >> for appending. You can redirect both stdout and stderr for logging purposes.
22. What is the pipe (|) operator in shell scripting?
Answer:
The pipe operator allows the output of one command to be used as input for another command. This helps in chaining commands together for complex data processing.
23. What is a subshell in shell scripting?
Answer:
A subshell is a child shell process created to execute commands in isolation. Commands in parentheses () are executed in a subshell, allowing temporary scope for variables or commands.
echo “$result”
24. What is command substitution in shell scripting?
Answer:
Command substitution allows you to capture the output of a command and assign it to a variable or use it directly. This can be done using backticks (`) or $(…).
echo “Today’s date: $date”
25. What are conditional statements in shell scripting?
Answer:
Conditional statements like if, elif, else, and case are used to execute different blocks of code based on the evaluation of an expression or variable.
echo “Condition met”
fi
26. How do you schedule a shell script to run at a specific time using cron?
Answer:
You can schedule a shell script using the cron utility. Edit the crontab with crontab -e and add a line to define the schedule and the script to execute.
27. What are environment variables in shell scripting?
Answer:
Environment variables are system-wide variables that affect the behavior of running processes. Common environment variables include $PATH, $HOME, and $USER. They can be accessed and modified within a script.
28. What is the difference between = and == in shell scripting?
Answer:
In shell scripting, = is used for assigning values to variables, while == is used for string comparison in some shells like Bash. In [ ], use a single = for comparison.
29. How do you terminate a shell script early?
Answer:
Use the exit command to terminate a shell script at any point. You can optionally provide an exit code to indicate the reason for termination.
exit 1
fi
30. How do you check the length of a string in shell scripting?
Answer:
You can check the length of a string using ${#string} syntax. This is useful for validation, like ensuring user input meets length requirements.
echo ${#str} # Outputs 5
31. What is the use of break and continue in loops?
Answer:
break exits the loop immediately, while continue skips the current iteration and moves to the next one. They control loop flow based on conditions.
Copy code
for i in {1..5}; do
if [ $i -eq 3 ]; then
continue
fi
echo “N
32. How do you declare and use arrays in shell scripting?
Answer:
Arrays store multiple values in a single variable. They are declared using parentheses, and individual elements are accessed using their index.
echo ${arr[1]} # Outputs banana
33. How do you debug a shell script?
Answer:
You can debug a shell script using bash -x script.sh, which prints each command before executing it. Alternatively, use set -x within the script to debug specific sections.
34. What is a here document in shell scripting?
Answer:
A here document (<<) allows you to redirect multiple lines of input into a command. It’s useful for feeding blocks of text or commands into scripts.
Hello, World!
EOF
35. How do you trap signals in shell scripting?
Answer:
The trap command allows you to catch and handle signals like SIGINT (Ctrl+C). This is useful for cleaning up resources before a script terminates.
36. How do you check if a directory exists in shell scripting?
Answer:
Use the -d operator in an if statement to check if a directory exists. This is helpful when managing directories within scripts.
echo “Directory exists”
fi
37. What is the purpose of shift in shell scripting?
Answer:
shift is used to shift positional parameters to the left. After a shift, $2 becomes $1, $3 becomes $2, and so on. This is useful for handling arguments in loops.
38. How do you perform arithmetic operations in shell scripting?
Answer:
Arithmetic operations can be performed using the expr command or double parentheses (( )). This allows you to handle basic math within scripts.
echo $result # Outputs 8
39. What is the use of the let command in shell scripting?
Answer:
The let command is used for performing arithmetic operations on shell variables. It simplifies numeric calculations in scripts.
echo $result # Outputs 15
40. How do you search for a pattern in a file using shell scripting?
Answer:
Use the grep command to search for a pattern in a file. It is commonly used for filtering text and finding specific strings in files.
41. How do you copy a file in shell scripting?
Answer:
The cp command is used to copy files from one location to another. You can specify options like -r to copy directories.
42. What is the purpose of the find command in shell scripting?
Answer:
The find command searches for files or directories within a specified path based on criteria like name, size, or modification time.
43. How do you compress files in shell scripting?
Answer:
Use the tar command to compress files into a single archive. You can combine it with gzip to compress the archive further.
44. What is the difference between > and >> in shell scripting?
Answer:
> overwrites the contents of a file, while >> appends to the file. This distinction is important when logging or saving output.
45. How do you extract a substring in shell scripting?
Answer:
You can extract a substring using ${string:position:length}. This is helpful when processing parts of a string.
echo ${str:0:5} # Outputs Hello
46. How do you split a string in shell scripting?
Answer:
Use IFS (Internal Field Separator) to split a string by a delimiter and store the parts in an array.
echo ${array[1]} # Outputs banana
47. How do you remove a file in shell scripting?
Answer:
Use the rm command to remove files or directories. For directories, use the -r option to remove them recursively.
48. What is the use of eval in shell scripting?
Answer:
The eval command executes a string as a command. It’s useful for dynamically constructing and running commands based on variables or input.
eval $cmd
49. How do you handle multiple commands in a single line in shell scripting?
Answer:
You can use ; to run multiple commands in a single line. This allows you to sequence commands together, even if they are unrelated.
50. What is the difference between && and || in shell scripting?
Answer:
&& executes the next command only if the previous command succeeds, while || executes the next command only if the previous command fails. These are used for conditional execution of commands.
Final Words
Getting ready for an interview can feel overwhelming, but going through these Shell Scripting fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Shell Scripting interview but don’t forget to practice shell commands, file manipulation, process management, and scripting-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for Shell scripting?
Common Shell scripting interview questions include file handling commands, loops, conditionals, process control (e.g., ps, kill), scripting with awk and sed, variable usage, writing functions, and understanding the difference between various shells like bash, sh, and zsh.
2. What are the important Shell scripting topics freshers should focus on for interviews?
Freshers should focus on basic commands, control flow (loops, conditionals), working with files (reading, writing, appending), string manipulation, process control, regular expressions, text processing utilities like awk, sed, and basic automation scripts.
3. How should freshers prepare for Shell scripting technical interviews?
Freshers should practice writing small scripts that automate common tasks, such as file handling, system monitoring, and backups. Understanding how to use basic Shell utilities and writing clean, efficient scripts with proper comments will be beneficial.
4. What strategies can freshers use to solve Shell scripting coding questions during interviews?
Freshers should carefully analyze the problem, use the correct commands for the task, and break down the solution into manageable steps. Testing the script incrementally and handling edge cases, such as empty files or incorrect input, is key.
5. Should freshers prepare for advanced Shell scripting topics in interviews?
Yes, freshers should prepare advanced topics like process management, signal handling, background processes, and performance tuning can be explored once they have a solid grasp of the fundamentals.
Explore More Shell Scripting Resources
Explore More Interview Questions
- Python
- Java
- SQL
- React
- JavaScript
- C Programming
- HTML
- CSS
- Angular
- C++
- Spring Boot
- Node JS
- Excel
- C#
- DBMS
- PHP
- Linux
- Operating System
- MySQL
- Spring
- Flutter
- MongoDB
- Django
- React Native
- jQuery
- Bootstrap
- Embedded C
- DSA
- R Programming
- Hadoop
- .NET
- Power BI
- ASP.NET
- ASP.NET MVC
- Android
- Tableau
- MVC
- WordPress
- TypeScript
- Spark
- Kotlin
- Swift
- Golang
Related Posts
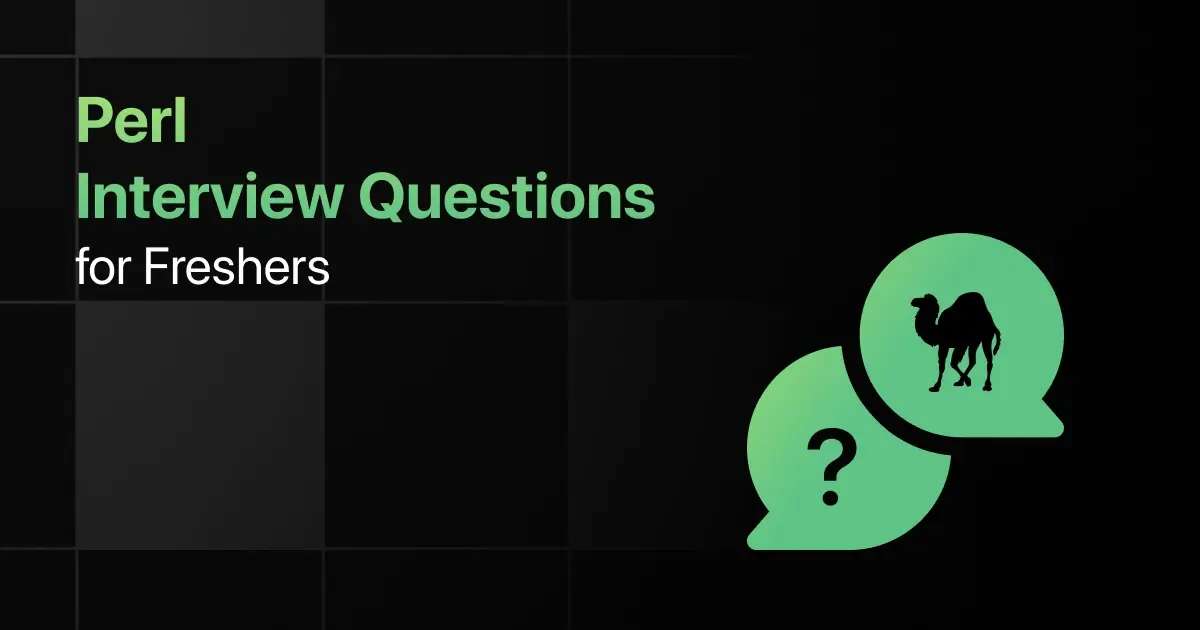
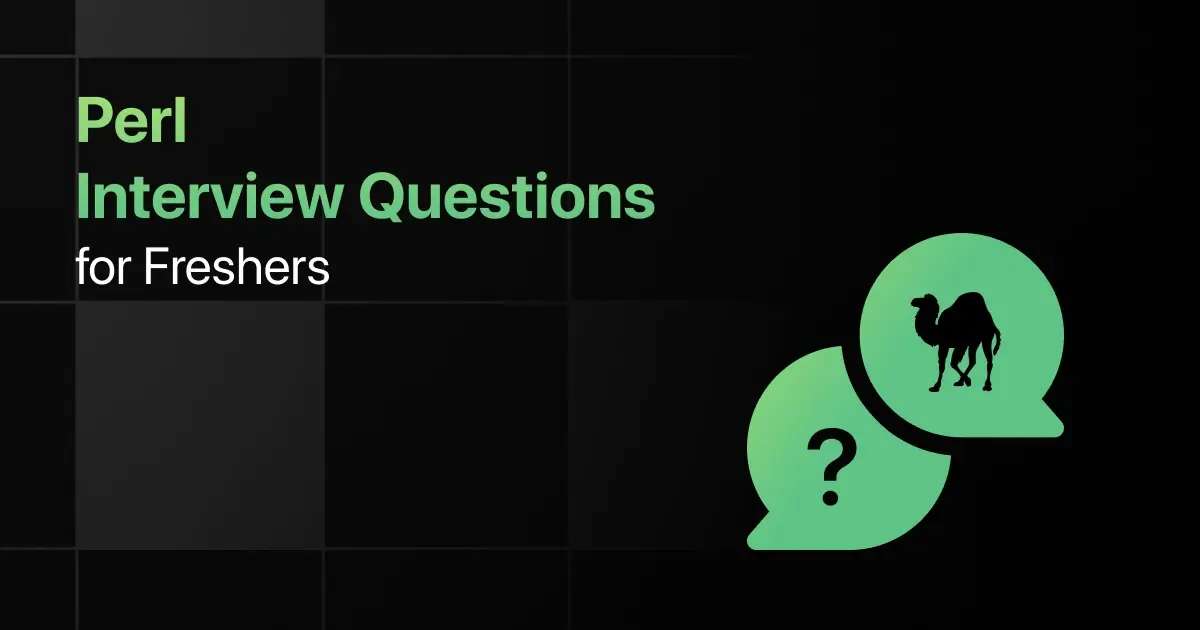
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …