Top Scala Interview Questions for Freshers
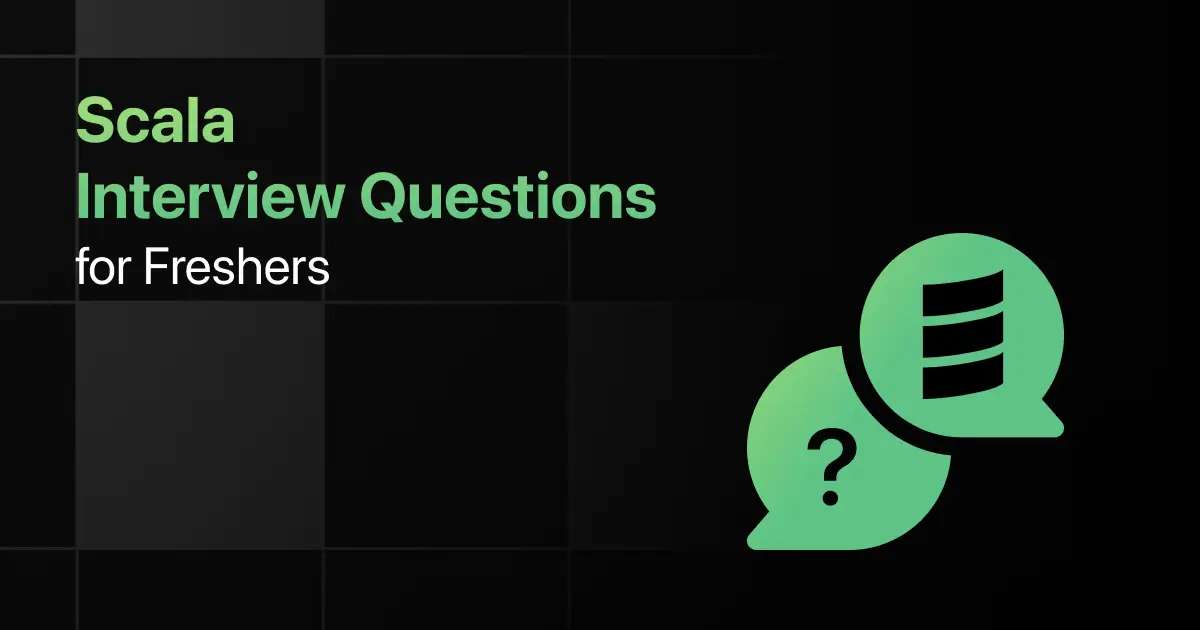
Are you preparing for your first Scala interview and wondering what questions you might face?
Understanding the key Scala interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Scala interview questions and answers for freshers and make a strong impression in your interview.
Practice Scala Interview Questions and Answers
Below are the top 50 Scala interview questions for freshers with answers:
1. What is Scala?
Answer:
Scala is a high-level programming language that combines object-oriented and functional programming paradigms. It is designed to be concise, elegant, and to work seamlessly with Java.
2. What are the main features of Scala?
Answer:
Scala supports both object-oriented and functional programming, provides type inference, has a powerful pattern-matching feature, and integrates with Java libraries seamlessly. It also offers immutable collections and concurrency support through actors.
3. What are the traits of Scala? What are their benefits?
Answer:
Traits are a fundamental unit of code reuse in Scala, similar to interfaces in Java but can also contain concrete implementations. They can be mixed into multiple classes, allowing for multiple inheritance, and improving code modularity.
4. What is a case class in Scala, and what are its benefits?
Answer:
A case class in Scala is a special type of class that is immutable and provides a default implementation of methods such as equals, hashCode, and toString. They simplify the creation of immutable data structures and enable pattern matching.
case class Person(name: String, age: Int)
5. What is pattern matching in Scala?
Answer:
Pattern matching is a powerful feature in Scala that allows checking a value against a pattern. It can be used in various scenarios, including deconstructing data structures and handling multiple cases in a clean and readable way.
val num = 2
num match {
case 1 => println(“One”)
case 2 => println(“Two”)
case _ => println(“Other”)
}
6. Explain the difference between val and var in Scala.
Answer:
val is used to declare immutable variables, while var is used for mutable variables. Once a val is assigned a value, it cannot be changed, whereas a var can be reassigned.
val x = 10 // Immutable
var y = 20 // Mutable
y = 30 // Allowed
7. What is a higher-order function in Scala?
Answer:
A higher-order function is a function that can take other functions as parameters or return a function as a result. This allows for more abstract and reusable code.
def applyFunc(f: Int => Int, x: Int): Int = f(x)
8. What are implicits in Scala, and how do they work?
Answer:
Implicits are a powerful feature in Scala that allows the compiler to automatically pass parameters or convert types when they are not explicitly provided. They help in reducing boilerplate code and enhancing readability.
implicit val multiplier: Int = 2
def multiply(x: Int)(implicit factor: Int): Int = x * factor
9. What is the purpose of the @tailrec annotation?
Answer:
The @tailrec annotation is used to indicate that a function is a tail-recursive function, allowing the compiler to optimize this by reusing the current function’s stack frame.
@tailrec
def factorial(n: Int, acc: Int = 1): Int = {
if (n <= 1) acc
else factorial(n – 1, n * acc)
}
10. What is a closure in Scala?
Answer:
A closure is a function that captures the environment in which it is defined. It can access variables from its containing scope, even after that scope has finished executing.
def makeIncrementer(increment: Int): Int => Int = {
(x: Int) => x + increment
}
11. How does Scala handle null values?
Answer:
Scala encourages the use of Option types instead of null values to represent the presence or absence of a value. This helps avoid NullPointerExceptions and encourages safer code practices.
val maybeValue: Option[String] = Some(“value”)
12. How do you implement exception handling in Scala?
Answer:
Exception handling in Scala can be done using try, catch, and finally blocks. This allows for catching exceptions and executing code to handle errors gracefully.
try {
// code that might throw an exception
} catch {
case e: Exception => println(e.getMessage)
}
13. What is a type alias in Scala?
Answer:
A type alias allows you to create an alternative name for an existing type, making code more readable and easier to manage.
type StringList = List[String]
14. What are the differences between List and Array in Scala?
Answer:
List is an immutable linked list that provides a functional programming approach, while Array is a mutable, fixed-size collection. Lists are more suited for functional transformations, whereas Arrays are better for performance-sensitive operations.
15. How can you create an immutable list in Scala?
Answer:
An immutable list can be created using the List class. The elements of the list cannot be changed after it is created, promoting functional programming practices.
val numbers = List(1, 2, 3, 4)
16. How can you convert a list to a set in Scala?
Answer:
You can convert a list to a set using the toSet method, which removes duplicates and creates an unordered collection.
val list = List(1, 2, 3, 3, 4)
val set = list.toSet
17. What is tail recursion, and why is it important?
Answer:
Tail recursion occurs when a function calls itself as its last action, allowing the compiler to optimize the call stack. This is important for preventing stack overflow in recursive functions.
18. How do you define a function with default parameters in Scala?
Answer:
A function can have default parameters defined in its signature, allowing the caller to omit those arguments if they wish to use the default values.
def greet(name: String = “World”): String = s”Hello, $name!”
19. What is the significance of the final keyword in Scala?
Answer:
The final keyword prevents a class from being subclassed or a method from being overridden, ensuring that the behavior remains consistent and unchangeable.
20. How do you define an abstract class in Scala?
Answer:
An abstract class is defined using the abstract keyword, allowing it to have abstract methods that subclasses must implement. It can also contain concrete methods.
abstract class Animal {
def makeSound(): Unit
}
21. What is the function of the super keyword in Scala?
Answer:
The super keyword is used to refer to the superclass of the current class, allowing access to overridden methods and members. It is useful for calling a parent class’s implementation.
22. What are the key differences between trait and abstract class in Scala?
Answer:
Traits can be mixed into multiple classes and do not have constructor parameters, while abstract classes can have constructor parameters and can only be inherited once. Traits are preferred for defining interfaces and shared behavior.
23. What is the function of the @deprecated annotation in Scala?
Answer:
The @deprecated annotation is used to mark methods or classes as deprecated, signaling that they should not be used and may be removed in future versions.
24. How do you define a function that takes variable arguments in Scala?
Answer:
A function can take variable arguments using the * syntax, allowing the caller to pass any number of arguments of a specified type.
def printNumbers(numbers: Int*): Unit = {
numbers.foreach(println)
}
25. What is a stack overflow in Scala?
Answer:
A stack overflow occurs when a program uses more stack space than is allocated, usually due to deep or infinite recursion. It can be mitigated by using tail recursion or iterative approaches.
26. How can you achieve concurrency in Scala?
Answer:
Concurrency can be achieved using the Future and Promise constructs, as well as through Akka actors, which provide a model for building concurrent and distributed systems.
27. What is a Monad in Scala?
Answer:
A Monad is a design pattern that allows for the composition of functions and chaining operations. In Scala, Option, List, and Future are examples of monadic types.
28. How do you define a function that returns another function in Scala?
Answer:
A function can return another function by defining the return type as a function type. This enables higher-order functions and enhances functional programming capabilities.
def createMultiplier(factor: Int): Int => Int = (x: Int) => x * factor
29. What is the significance of sealed classes in Scala?
Answer:
A sealed class restricts the inheritance of subclasses to the same file, providing exhaustiveness in pattern matching and making it easier to maintain and understand the hierarchy.
30. What is the purpose of the @volatile annotation?
Answer:
The @volatile annotation indicates that a variable’s value may be modified by different threads, ensuring that changes are visible across threads and preventing caching issues.
31. How can you create a custom collection in Scala?
Answer:
A custom collection can be created by extending existing collection traits and implementing necessary methods, allowing for tailored behavior and data structures.
32. What is a PartialFunction in Scala?
Answer:
A PartialFunction is a function that is not defined for all possible inputs. It can be used to define functions that only operate on a subset of input values.
val evenNumbers: PartialFunction[Int, String] = {
case x if x % 2 == 0 => s”$x is even”
}
33. How do you implement dependency injection in Scala?
Answer:
Dependency injection can be implemented using constructor parameters or frameworks like Guice or MacWire, allowing for better code separation and testing capabilities.
34. What is a companion trait in Scala?
Answer:
A companion trait is a trait that is defined alongside a class or object with the same name, allowing for a more modular design and the ability to define behavior associated with that class or object.
35. How can you test Scala code?
Answer:
Scala code can be tested using frameworks like ScalaTest or Specs2, which provide a rich set of features for unit testing, integration testing, and behavior-driven development.
36. What is the apply method in Scala?
Answer:
The apply method allows an object to be called as if it were a function. It’s commonly used in companion objects to create instances of a class without using the new keyword.
class Point(val x: Int, val y: Int)
object Point {
def apply(x: Int, y: Int): Point = new Point(x, y)
}
37. What are implicit parameters in Scala?
Answer:
Implicit parameters allow for the automatic passing of arguments to functions when they are not explicitly provided. This helps reduce boilerplate code and makes functions more flexible.
def greet(implicit name: String): Unit = println(s”Hello, $name!”)
implicit val name: String = “Alice”
greet() // Output: Hello, Alice!
38. What is the difference between map and flatMap in Scala?
Answer:
map transforms each element in a collection, returning a new collection, while flatMap applies a function that returns a collection for each element and then flattens the result into a single collection.
val numbers = List(1, 2, 3)
val mapped = numbers.map(x => List(x, x * 2)) // List(List(1, 2), List(2, 4), List(3, 6))
val flatMapped = numbers.flatMap(x => List(x, x * 2)) // List(1, 2, 2, 4, 3, 6)
39. What are higher-kinded types in Scala?
Answer:
Higher-kinded types are types that take other types as parameters. They enable the creation of abstractions that can operate on type constructors rather than concrete types.
def process[F[_]](data: F[Int]): F[Int] = ???
40. What is the with keyword used for in Scala?
Answer:
The with keyword is used to mix in traits to classes or objects. It allows a class to inherit behavior from multiple traits, enabling multiple inheritance.
trait A { def a: String = “A” }
trait B { def b: String = “B” }
class C extends A with B
41. What is the difference between == and === in Scala?
Answer:
== is used to compare values for equality, while === is often used in functional programming libraries to compare structural equality, especially in the context of Option types.
42. How do you handle optional values in Scala?
Answer:
Optional values in Scala are handled using the Option type, which can either be Some(value) or None. This encourages safe handling of potentially absent values.
val maybeValue: Option[Int] = Some(5)
maybeValue match {
case Some(v) => println(v)
case None => println(“No value”)
}
43. What is a for-comprehension in Scala?
Answer:
A for-comprehension is a syntactic sugar for chaining operations on collections and monads. It provides a concise way to work with collections, making the code more readable.
val numbers = List(1, 2, 3)
val result = for (n <- numbers if n % 2 == 0) yield n * 2 // List(4)
44. What is the purpose of the trait keyword?
Answer:
The trait keyword is used to define a trait, which is a special kind of class that can be mixed into other classes. Traits can contain both abstract and concrete methods, promoting code reuse.
45. How can you create an enumeration in Scala?
Answer:
You can create an enumeration by extending the Enumeration class and defining values as members of the enumeration. This provides a type-safe way to represent a fixed set of constants.
object Color extends Enumeration {
val Red, Green, Blue = Value
}
46. What is the significance of the @main annotation in Scala 3?
Answer:
The @main annotation in Scala 3 is used to define the entry point of a Scala program, simplifying the way to write the main method without boilerplate code.
@main def hello(): Unit = println(“Hello, world!”)
47. What is a structural type in Scala?
Answer:
A structural type is a type defined by the presence of certain methods rather than a specific class or trait. It allows for more flexible code that can work with any object that has the required methods.
type HasLength = { def length: Int }
def printLength(x: HasLength): Unit = println(x.length)
48. What is a partial function in Scala, and how is it different from a total function?
Answer:
A partial function is defined only for a subset of input values, whereas a total function is defined for all possible inputs. Partial functions can handle inputs safely using isDefinedAt to check if they apply.
49. How do you implement a singleton object in Scala?
Answer:
A singleton object can be created using the object keyword, which ensures that only one instance of the object exists throughout the program, allowing for easy access to shared functionality.
object Database {
def connect(): Unit = println(“Connected to the database”)
}
50. What are the differences between Set, Map, and List in Scala?
Answer:
Set is a collection of unique elements, Map is a collection of key-value pairs, and List is an ordered collection of elements. Each collection has different characteristics and use cases depending on the requirements of the application.
Final Words
Getting ready for an interview can feel overwhelming, but going through these Scala fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Scala interview but don’t forget to practice functional programming concepts, Scala collections, and type system-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for Scala?
Common Scala interview questions include functional programming, collections, and pattern matching.
2. What are the important Scala topics freshers should focus on for interviews?
Freshers should focus on topics like immutability, case classes, higher-order functions, and the Scala-type system.
3. How should freshers prepare for Scala technical interviews?
Freshers should prepare by practicing Scala syntax, functional concepts, and coding problems on platforms like LeetCode.
4. What strategies can freshers use to solve Scala coding questions during interviews?
Freshers can use strategies like breaking problems into smaller functions and using Scala’s built-in collection methods effectively.
5. Should freshers prepare for advanced Scala topics in interviews?
Yes, freshers should prepare for advanced Scala topics if the job role requires expertise in frameworks like Akka or Play.
Explore More Scala Resources
Explore More Interview Questions
- Python
- Java
- SQL
- React
- JavaScript
- C Programming
- HTML
- CSS
- Angular
- C++
- Spring Boot
- Node JS
- Excel
- C#
- DBMS
- PHP
- Linux
- Operating System
- MySQL
- Spring
- Flutter
- MongoDB
- Django
- React Native
- jQuery
- Bootstrap
- Embedded C
- DSA
- R Programming
- Hadoop
- .NET
- Power BI
- ASP.NET
- ASP.NET MVC
- Android
- Tableau
- MVC
- WordPress
- TypeScript
- Spark
- Kotlin
- Swift
- Golang
- Shell Scripting
- iOS
- Spring MVC
- Next JS
- AWS
- Kubernetes
- Docker
- Cyber Security
- Azure
- Terraform
- Vue JS
- UiPath
Related Posts
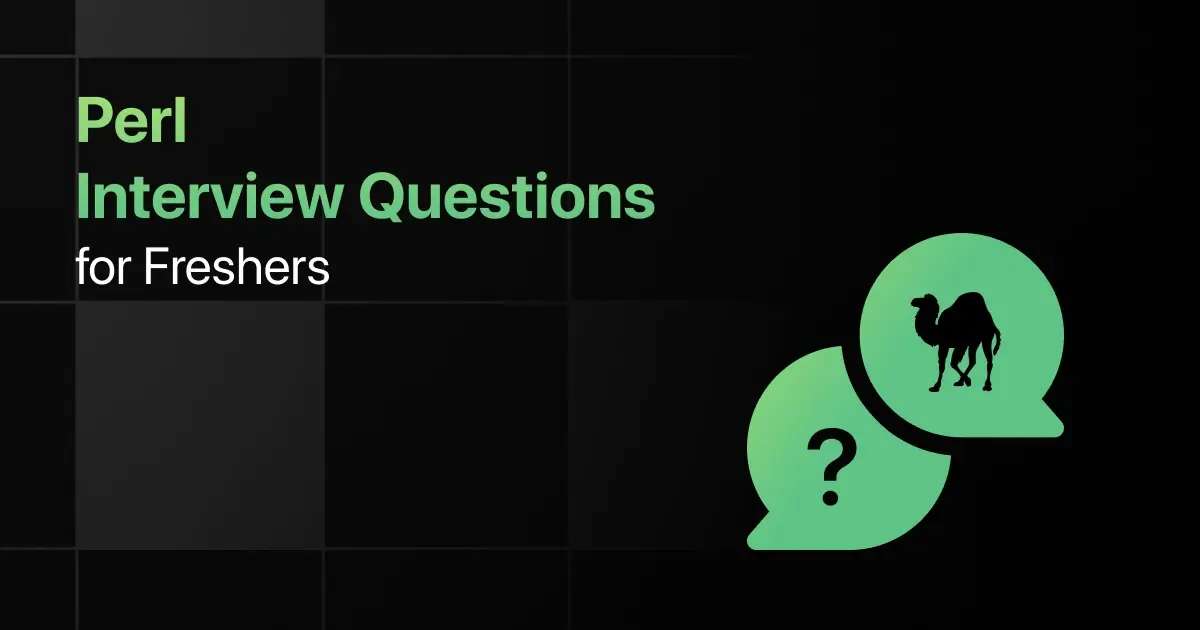
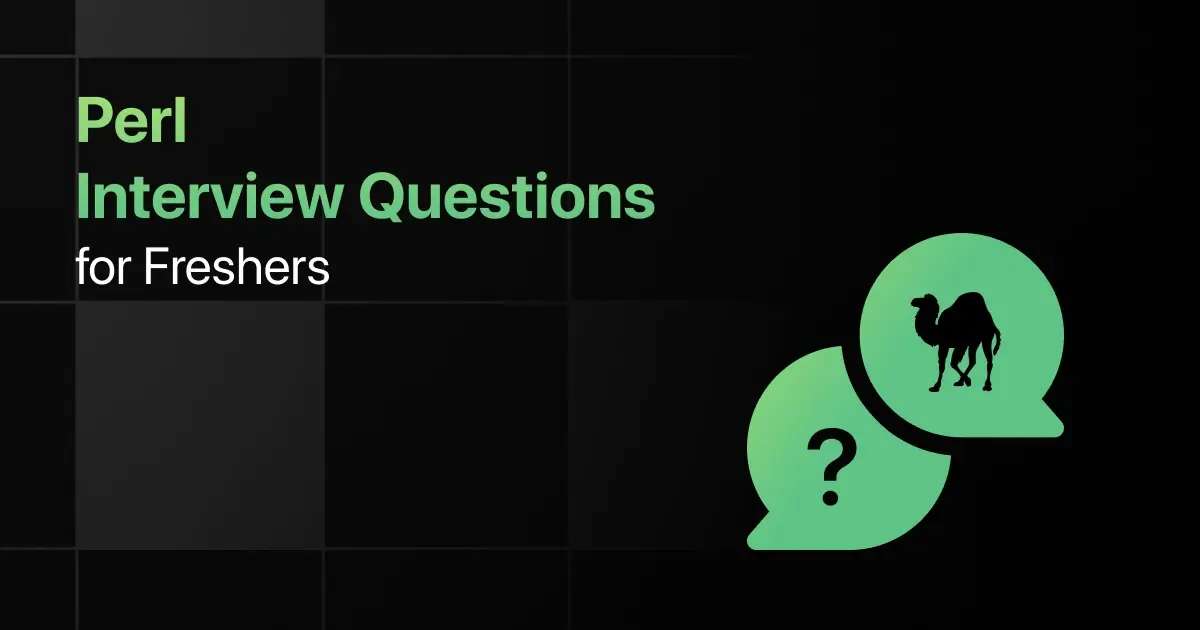
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …