Top React Native Interview Questions for Freshers
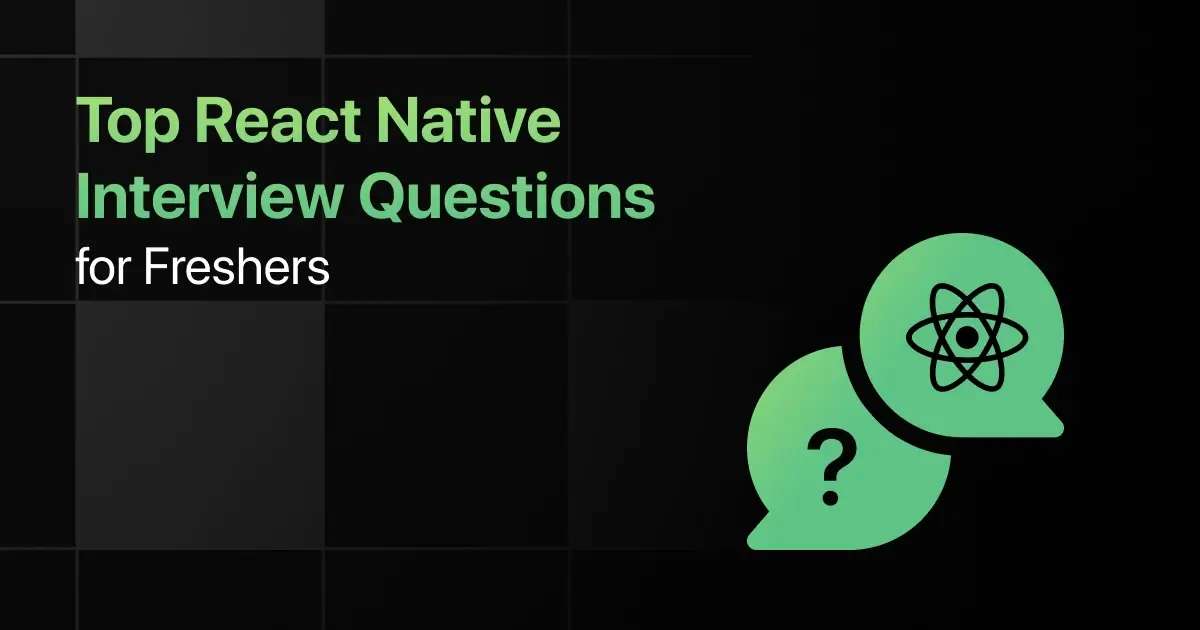
Are you preparing for your first React Native interview and wondering what questions you might face? Understanding the key React Native interview questions for freshers can give you more clarity.
This blog is here to help you get ready with practical questions that test your real-world problem-solving skills. We’ve gathered some of the most common basic React Native interview questions that freshers often encounter.
With this guide, you’ll be well-prepared to tackle these React Native interview questions and answers for freshers and make a strong impression in your interview.
Practice React Native Interview Questions and Answers
Here are the top 50 React Native Interview questions for freshers with answers:
1. What is React Native, and how does it differ from React?
Answer:
React Native is a framework for building mobile applications using React. Unlike React, which targets web browsers, React Native targets mobile platforms (iOS and Android) and uses native components instead of web components.
import { Text, View } from ‘react-native’;
2. How do you set up a new React Native project?
Answer:
Use the npx react-native init command followed by the project name to set up a new React Native project.
npx react-native init MyNewProject
3. What is the purpose of the AppRegistry in React Native?
Answer:
AppRegistry is the entry point to run a React Native application. It tells React Native which component to render as the root component.
import { AppRegistry } from ‘react-native’;
import App from ‘./App’;
AppRegistry.registerComponent(‘MyApp’, () => App);
4. How do you run a React Native app on an iOS or Android emulator?
Answer:
Use the npx react-native run-ios or npx react-native run-android commands to run the app on the respective emulator.
npx react-native run-ios
npx react-native run-android
5. What is JSX, and how is it used in React Native?
Answer:
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within JavaScript. In React Native, JSX is used to describe the UI.
const App = () => (
<View>
<Text>Hello, world!</Text>
</View>
);
6. How do you create a functional component in React Native?
Answer:
A functional component is a simple function that returns JSX to render UI elements.
const MyComponent = () => (
<View>
<Text>My Functional Component</Text>
</View>
);
7. How do you apply styles to components in React Native?
Answer:
Use the StyleSheet API or inline styles to apply styles to React Native components.
import { StyleSheet, View, Text } from ‘react-native’;
const styles = StyleSheet.create({
container: {
padding: 20,
backgroundColor: ‘lightblue’,
},
text: {
fontSize: 20,
color: ‘darkblue’,
},
});
const App = () => (
<View style={styles.container}>
<Text style={styles.text}>Styled Text</Text>
</View>
);
8. How do you handle component state in React Native?
Answer:
Use the useState hook to manage state within a functional component.
import { useState } from ‘react’;
import { View, Button, Text } from ‘react-native’;
const App = () => {
const [count, setCount] = useState(0);
return (
<View>
<Text>{count}</Text>
<Button title=”Increment” onPress={() => setCount(count + 1)} />
</View>
);
};
9. How do you pass data between components in React Native?
Answer:
Pass data between components using props. The parent component passes data to the child component as a prop.
const ChildComponent = ({ message }) => (
<Text>{message}</Text>
);
const ParentComponent = () => (
<ChildComponent message=”Hello from parent!” />
);
10. How do you conditionally render components in React Native?
Answer:
Use conditional statements like if-else or ternary operators to render components based on certain conditions.
const App = ({ isLoggedIn }) => (
<View>
{isLoggedIn ? <Text>Welcome back!</Text> : <Text>Please log in.</Text>}
</View>
);
11. How do you set up navigation in a React Native app?
Answer:
Use the react-navigation library to handle navigation. Start by installing the necessary packages and setting up a NavigationContainer.
import { NavigationContainer } from ‘@react-navigation/native’;
import { createStackNavigator } from ‘@react-navigation/stack’;
const Stack = createStackNavigator();
const App = () => (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name=”Home” component={HomeScreen} />
<Stack.Screen name=”Details” component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
12. How do you navigate between screens in React Native?
Answer:
Use the navigation.navigate() method provided by the react-navigation library to move between screens.
const HomeScreen = ({ navigation }) => (
<Button title=”Go to Details” onPress={() => navigation.navigate(‘Details’)} />
);
13. How do you pass parameters to routes in React Native?
Answer:
Pass parameters to routes using the navigate() method, and access them using route.params.
// Navigating with parameters
navigation.navigate(‘Details’, { itemId: 42 });
// Accessing parameters
const { itemId } = route.params;
14. How do you handle deep linking in React Native?
Answer:
Configure deep linking by setting up the Linking module and defining URL patterns in your navigation.
const linking = {
prefixes: [‘myapp://’],
config: {
screens: {
Home: ‘home’,
Details: ‘details/:id’,
},
},
};
const App = () => (
<NavigationContainer linking={linking}>
{/* Navigator setup */}
</NavigationContainer>
);
15. How do you implement a bottom tab navigator in React Native?
Answer:
Use the createBottomTabNavigator function from @react-navigation/bottom-tabs to implement a tab-based navigation.
import { createBottomTabNavigator } from ‘@react-navigation/bottom-tabs’;
const Tab = createBottomTabNavigator();
const App = () => (
<NavigationContainer>
<Tab.Navigator>
<Tab.Screen name=”Home” component={HomeScreen} />
<Tab.Screen name=”Settings” component={SettingsScreen} />
</Tab.Navigator>
</NavigationContainer>
);
16. What is the difference between state and props in React Native?
Answer:
State is a component’s internal data, managed within the component itself, while props are external data passed to the component by its parent.
// State (internal)
const [count, setCount] = useState(0);
// Props (external)
const ChildComponent = ({ message }) => <Text>{message}</Text>;
17. How do you use the Context API for state management in React Native?
Answer:
Create a context using React.createContext, and use Provider to pass the state to child components.
const MyContext = React.createContext();
const App = () => {
const [value, setValue] = useState(‘Hello’);
return (
<MyContext.Provider value={value}>
<ChildComponent />
</MyContext.Provider>
);
};
18. How do you use the useReducer hook in React Native?
Answer:
useReducer is an alternative to useState for managing more complex state logic in React Native components.
const reducer = (state, action) => {
switch (action.type) {
case ‘increment’:
return { count: state.count + 1 };
default:
return state;
}
};
const App = () => {
const [state, dispatch] = useReducer(reducer, { count: 0 });
return (
<View>
<Text>{state.count}</Text>
<Button title=”Increment” onPress={() => dispatch({ type: ‘increment’ })} />
</View>
);
};
19. How do you manage global state in a React Native app using Redux?
Answer:
Set up Redux by creating a store, defining reducers, and using the Provider to make the store available to the app.
import { createStore } from ‘redux’;
import { Provider } from ‘react-redux’;
const reducer = (state = { count: 0 }, action) => {
switch (action.type) {
case ‘INCREMENT’:
return { …state, count: state.count + 1 };
default:
return state;
}
};
const store = createStore(reducer);
const App = () => (
<Provider store={store}>
<MyComponent />
</Provider>
);
20. How do you connect a component to the Redux store in React Native?
Answer:
Use the connect function from react-redux to map state and dispatch to the component’s props.
import { connect } from ‘react-redux’;
const MyComponent = ({ count, increment }) => (
<View>
<Text>{count}</Text>
<Button title=”Increment” onPress={increment} />
</View>
);
const mapStateToProps = state => ({
count: state.count,
});
const mapDispatchToProps = dispatch => ({
increment: () => dispatch({ type: ‘INCREMENT’ }),
});
export default connect(mapStateToProps, mapDispatchToProps)(MyComponent);
21. How do you make API requests in React Native?
Answer:
Use the fetch API or a library like axios to make HTTP requests to a backend service.
useEffect(() => {
fetch(‘https://api.example.com/data’)
.then(response => response.json())
.then(data => console.log(data));
}, []);
22. How do you handle API request errors in React Native?
Answer:
Use try-catch blocks or .catch() method to handle errors in API requests.
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(‘https://api.example.com/data’);
const data = await response.json();
console.log(data);
} catch (error) {
console.error(‘Error fetching data:’, error);
}
};
fetchData();
}, []);
23. How do you use the useEffect hook to fetch data in React Native?
Answer:
Use the useEffect hook to fetch data when a component mounts, and update the state with the fetched data.
useEffect(() => {
fetch(‘https://api.example.com/data’)
.then(response => response.json())
.then(data => setData(data));
}, []);
24. How do you manage loading and error states while fetching data in React Native?
Answer:
Use state variables to track loading and error states, and conditionally render UI based on these states.
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
const [data, setData] = useState(null);
useEffect(() => {
fetch(‘https://api.example.com/data’)
.then(response => response.json())
.then(data => {
setData(data);
setLoading(false);
})
.catch(error => {
setError(error);
setLoading(false);
});
}, []);
25. How do you implement pagination in React Native while fetching data from an API?
Answer:
Implement pagination by maintaining a page state, fetching data based on the current page, and updating the page number for subsequent requests.
const [page, setPage] = useState(1);
const [items, setItems] = useState([]);
const fetchData = () => {
fetch(`https://api.example.com/data?page=${page}`)
.then(response => response.json())
.then(newItems => setItems([…items, …newItems]));
};
useEffect(() => {
fetchData();
}, [page]);
26. What are native modules in React Native, and why are they used?
Answer:
Native modules are custom modules written in native code (Java/Objective-C/Swift) that extend React Native’s functionality, allowing you to access platform-specific APIs.
import { NativeModules } from ‘react-native’;
const { MyNativeModule } = NativeModules;
27. How do you bridge a native module to React Native?
Answer:
Create a native module in Java/Objective-C/Swift and expose it to React Native using the ReactMethod annotation (Android) or RCT_EXPORT_MODULE macro (iOS).
// Android example
package com.myapp;
import com.facebook.react.bridge.ReactApplicationContext;
import com.facebook.react.bridge.ReactContextBaseJavaModule;
import com.facebook.react.bridge.ReactMethod;
import com.facebook.react.bridge.Promise;
public class MyModule extends ReactContextBaseJavaModule {
@Override
public String getName() {
return “MyModule”;
}
@ReactMethod
public void doSomething(Promise promise) {
promise.resolve(“Success”);
}
}
28. How do you call a native method from React Native?
Answer:
Call a native method using NativeModules after importing it into your React Native component.
import { NativeModules } from ‘react-native’;
const { MyModule } = NativeModules;
MyModule.doSomething()
.then(result => console.log(result))
.catch(error => console.error(error));
29. How do you handle permissions in React Native?
Answer:
Use the PermissionsAndroid module on Android or the react-native-permissions library to request and check permissions.
import { PermissionsAndroid } from ‘react-native’;
const requestCameraPermission = async () => {
try {
const granted = await PermissionsAndroid.request(
PermissionsAndroid.PERMISSIONS.CAMERA,
{
title: “Camera Permission”,
message: “App needs camera access”,
buttonNeutral: “Ask Me Later”,
buttonNegative: “Cancel”,
buttonPositive: “OK”
}
);
if (granted === PermissionsAndroid.RESULTS.GRANTED) {
console.log(“You can use the camera”);
} else {
console.log(“Camera permission denied”);
}
} catch (err) {
console.warn(err);
}
};
30. How do you integrate third-party native libraries in React Native?
Answer:
Integrate third-party native libraries by linking them using react-native link (for older versions) or using the pod install command for iOS and manual linking for Android.
npm install react-native-device-info
cd ios
pod install
31. How do you implement global theming in React Native?
Answer:
Use the Context API or a theming library like styled-components to manage global themes across the app.
import { ThemeProvider } from ‘styled-components’;
const theme = {
colors: {
primary: ‘#3498db’,
secondary: ‘#2ecc71’,
},
};
const App = () => (
<ThemeProvider theme={theme}>
<MyComponent />
</ThemeProvider>
);
32. How do you create responsive layouts in React Native?
Answer:
Use Dimensions, flexbox, or third-party libraries like react-native-responsive-screen to create responsive layouts.
import { Dimensions, StyleSheet, View, Text } from ‘react-native’;
const { width, height } = Dimensions.get(‘window’);
const styles = StyleSheet.create({
container: {
width: width * 0.8,
height: height * 0.5,
backgroundColor: ‘lightgray’,
},
});
const App = () => (
<View style={styles.container}>
<Text>Responsive Layout</Text>
</View>
);
33. How do you apply platform-specific styles in React Native?
Answer:
Use the Platform module to apply platform-specific styles in your React Native components.
import { Platform, StyleSheet, View, Text } from ‘react-native’;
const styles = StyleSheet.create({
text: {
…Platform.select({
ios: {
fontFamily: ‘Avenir’,
fontSize: 20,
},
android: {
fontFamily: ‘Roboto’,
fontSize: 18,
},
}),
},
});
const App = () => (
<View>
<Text style={styles.text}>Platform-specific styles</Text>
</View>
);
34. How do you use Flexbox for layout in React Native?
Answer:
Flexbox is the primary layout system in React Native, allowing you to align and distribute space among items in a container.
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: ‘row’,
justifyContent: ‘space-between’,
alignItems: ‘center’,
},
box: {
width: 50,
height: 50,
backgroundColor: ‘blue’,
},
});
const App = () => (
<View style={styles.container}>
<View style={styles.box} />
<View style={styles.box} />
<View style={styles.box} />
</View>
);
35. How do you implement dark mode in a React Native app?
Answer:
Use the useColorScheme hook or Appearance API to detect the system theme and apply dark mode styles accordingly.
import { useColorScheme } from ‘react-native’;
const App = () => {
const scheme = useColorScheme();
const backgroundColor = scheme === ‘dark’ ? ‘black’ : ‘white’;
const textColor = scheme === ‘dark’ ? ‘white’ : ‘black’;
return (
<View style={{ backgroundColor, flex: 1 }}>
<Text style={{ color: textColor }}>Hello, World!</Text>
</View>
);
};
36. How do you create simple animations in React Native?
Answer:
Use the Animated API to create simple animations by interpolating values and applying them to styles.
import { Animated } from ‘react-native’;
const App = () => {
const fadeAnim = new Animated.Value(0);
Animated.timing(fadeAnim, {
toValue: 1,
duration: 1000,
useNativeDriver: true,
}).start();
return (
<Animated.View style={{ opacity: fadeAnim }}>
<Text>Fading in</Text>
</Animated.View>
);
};
37. How do you create a looping animation in React Native?
Answer:
Use Animated.loop() to create a looping animation that repeats indefinitely or a specified number of times.
const spinValue = new Animated.Value(0);
Animated.loop(
Animated.timing(spinValue, {
toValue: 1,
duration: 2000,
useNativeDriver: true,
})
).start();
const spin = spinValue.interpolate({
inputRange: [0, 1],
outputRange: [‘0deg’, ‘360deg’],
});
return <Animated.View style={{ transform: [{ rotate: spin }] }} />;
38. How do you create staggered animations in React Native?
Answer:
Use Animated.stagger() to create a sequence of animations that start after a specified delay.
const fadeAnim1 = new Animated.Value(0);
const fadeAnim2 = new Animated.Value(0);
Animated.stagger(500, [
Animated.timing(fadeAnim1, { toValue: 1, duration: 1000, useNativeDriver: true }),
Animated.timing(fadeAnim2, { toValue: 1, duration: 1000, useNativeDriver: true }),
]).start();
return (
<View>
<Animated.View style={{ opacity: fadeAnim1 }} />
<Animated.View style={{ opacity: fadeAnim2 }} />
</View>
);
39. How do you animate list items when they appear in React Native?
Answer:
Use the LayoutAnimation module to animate changes in the layout, such as the insertion of new list items.
import { LayoutAnimation, UIManager, Platform, View, Button } from ‘react-native’;
if (Platform.OS === ‘android’) {
UIManager.setLayoutAnimationEnabledExperimental && UIManager.setLayoutAnimationEnabledExperimental(true);
}
const App = () => {
const [items, setItems] = useState([]);
const addItem = () => {
LayoutAnimation.configureNext(LayoutAnimation.Presets.spring);
setItems([…items, { id: items.length, name: `Item ${items.length}` }]);
};
return (
<View>
{items.map(item => (
<View key={item.id}>
<Text>{item.name}</Text>
</View>
))}
<Button title=”Add Item” onPress={addItem} />
</View>
);
};
40. How do you use the useNativeDriver option in React Native animations?
Answer:
The useNativeDriver option allows animations to run on the native thread for better performance, but it only works with properties like opacity and transforms.
Animated.timing(fadeAnim, {
toValue: 1,
duration: 1000,
useNativeDriver: true, // Enable native driver
}).start();
41. How do you write unit tests for React Native components?
Answer:
Use the Jest testing framework and react-test-renderer to write unit tests for React Native components.
import React from ‘react’;
import renderer from ‘react-test-renderer’;
import MyComponent from ‘../MyComponent’;
test(‘renders correctly’, () => {
const tree = renderer.create(<MyComponent />).toJSON();
expect(tree).toMatchSnapshot();
});
42. How do you perform snapshot testing in React Native?
Answer:
Snapshot testing involves rendering a component and saving its output to a file, which is then compared with future renders to ensure consistency.
import renderer from ‘react-test-renderer’;
test(‘MyComponent renders correctly’, () => {
const tree = renderer.create(<MyComponent />).toJSON();
expect(tree).toMatchSnapshot();
});
43. How do you mock API calls in React Native tests?
Answer:
Use jest.mock to mock API calls in your tests, ensuring that tests run without making actual network requests.
jest.mock(‘axios’);
test(‘fetches data successfully’, async () => {
axios.get.mockResolvedValue({ data: { id: 1, name: ‘John Doe’ } });
const data = await fetchData();
expect(data.name).toBe(‘John Doe’);
});
44. How do you test asynchronous code in React Native?
Answer:
Use async/await in your tests to handle asynchronous operations and make assertions after the async code has run.
test(‘async function returns data’, async () => {
const data = await asyncFunction();
expect(data).toBe(‘expectedData’);
});
45. How do you perform end-to-end (E2E) testing in React Native?
Answer:
Use tools like Detox to perform end-to-end testing by simulating user interactions and validating app behavior.
detox init -r jest
detox test
46. How do you optimize React Native performance by avoiding unnecessary re-renders?
Answer:
Use React.memo for functional components and PureComponent for class components to prevent unnecessary re-renders.
const MyComponent = React.memo(({ prop1 }) => {
return <Text>{prop1}</Text>;
});
47. How do you improve the performance of large lists in React Native?
Answer:
Use FlatList or SectionList with optimizations like getItemLayout, initialNumToRender, and removeClippedSubviews.
<FlatList
data={data}
renderItem={renderItem}
keyExtractor={item => item.id}
initialNumToRender={10}
getItemLayout={(data, index) => ({ length: ITEM_HEIGHT, offset: ITEM_HEIGHT * index, index })}
removeClippedSubviews={true}
/>
48. How do you reduce the app bundle size in React Native?
Answer:
Reduce app bundle size by enabling Proguard for Android, using Hermes engine, and removing unused dependencies.
// Enable Hermes in android/app/build.gradle
project.ext.react = [
enableHermes: true
]
49. How do you optimize image loading in React Native?
Answer:
Optimize images by using appropriate formats, compressing images, and using tools like react-native-fast-image for better performance.
import FastImage from ‘react-native-fast-image’;
<FastImage
style={{ width: 200, height: 200 }}
source={{
uri: ‘https://example.com/image.png’,
priority: FastImage.priority.high,
}}
resizeMode={FastImage.resizeMode.cover}
/>
50. How do you handle memory leaks in React Native applications?
Answer:
Handle memory leaks by unmounting components properly, cleaning up event listeners, and avoiding long-running timers.
useEffect(() => {
const timer = setInterval(() => {
console.log(‘Interval running’);
}, 1000);
return () => clearInterval(timer); // Cleanup
}, []);
Final Words
Getting ready for an interview can feel overwhelming, but going through these React Native fresher interview questions can help you feel more confident. This guide focuses on the kinds of React Native-related interview questions for fresher roles that you’re likely to face.
Don’t forget to practice the React Native basics, component lifecycle, and state management-related interview questions too! With the right preparation, you’ll ace your React Native interview and take that important step in your career.
Frequently Asked Questions
1. What are the most common interview questions for React Native?
The most common interview questions for React Native often cover topics like component lifecycle, state and props, navigation, and styling with React Native’s styling system.
2. What are the important React Native topics freshers should focus on for interviews?
The important React Native topics freshers should focus on include understanding components, handling state and props, navigation with React Navigation, and integrating with native modules.
3. How should freshers prepare for React Native technical interviews?
Freshers should prepare for React Native technical interviews by building sample apps, practicing common UI challenges, and understanding the differences between React and React Native.
4. What strategies can freshers use to solve React Native coding questions during interviews?
Strategies freshers can use include breaking down the problem, efficiently managing state, and testing components to ensure they render correctly on different devices.
5. Should freshers prepare for advanced React Native topics in interviews?
Yes, freshers should prepare for advanced React Native topics like performance optimization, handling animations, and integrating with native APIs if the role requires a deeper understanding of React Native.
Explore More React Native Resources
Explore More Interview Questions
Related Posts
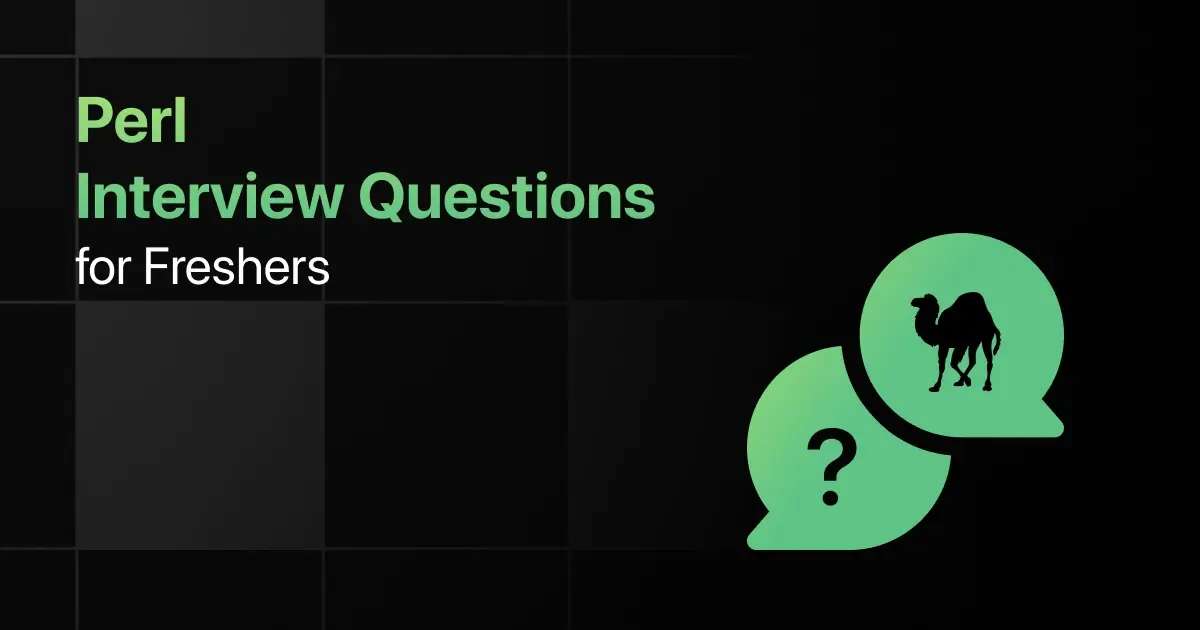
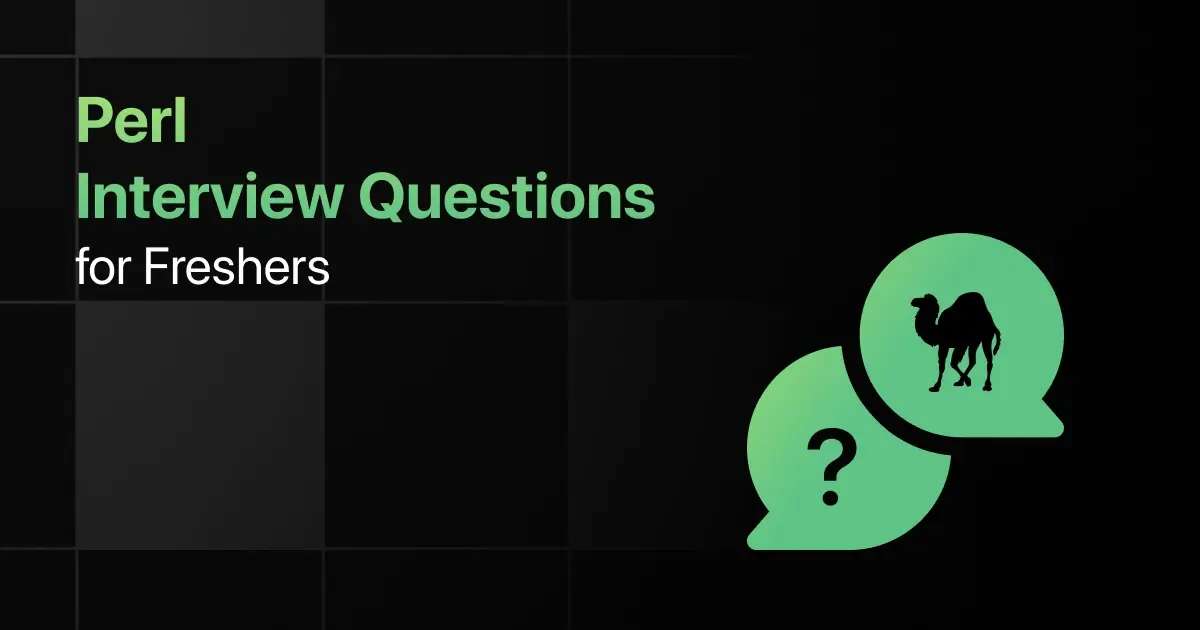
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …