Top React Interview Questions for Freshers
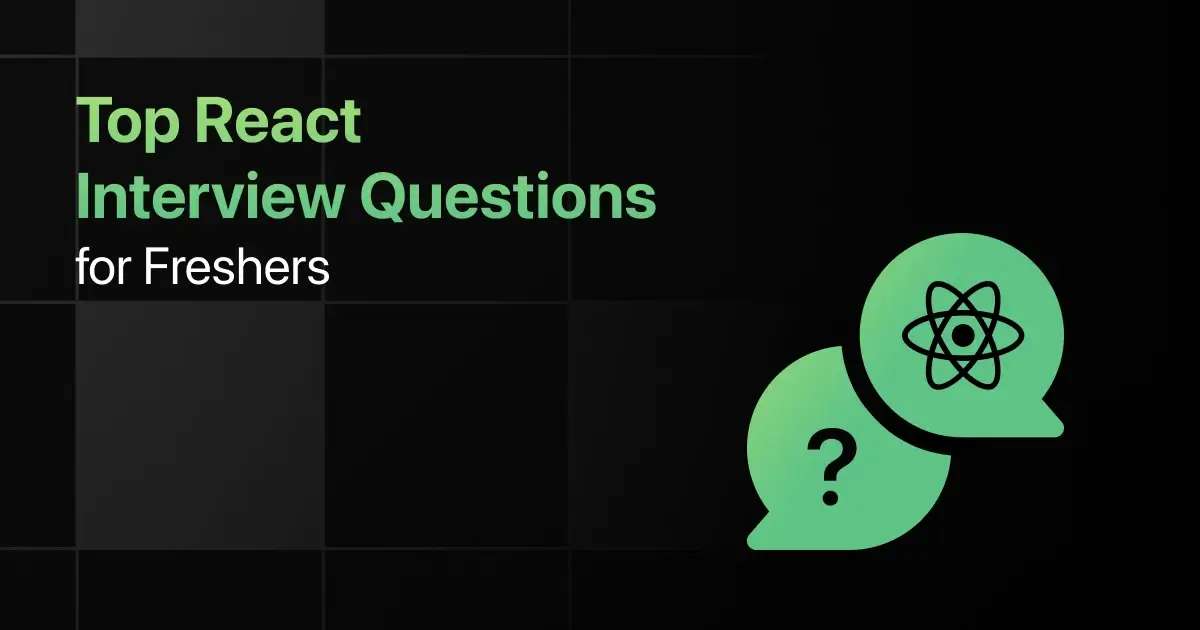
Are you preparing for your first React interview and wondering what questions you might face? Understanding the key React interview questions for freshers can give you more clarity.
This blog is here to help you get ready with practical questions that test your real-world problem-solving skills. We’ve gathered some of the most common basic React interview questions that freshers often encounter.
With this guide, you’ll be well-prepared to tackle these React interview questions and answers for freshers and make a strong impression in your interview.
Practice React Interview Questions and Answers
Below are the 50 react interview questions for freshers with answers
1. What is the purpose of ReactDOM in a React application?
Answer:
ReactDOM is used to render React components into the DOM and handle DOM-specific methods.
ReactDOM.render(<App />, document.getElementById(‘root’));
2. Explain the role of Babel in a React project.
Answer:
Babel transpiles modern JavaScript (ES6+) and JSX into a format compatible with older browsers.
// JSX code
const element = <h1>Hello, world!</h1>;
3. How does Webpack contribute to a React application?
Answer:
Webpack bundles JavaScript files and assets, optimizing them for production.
// Webpack config example
module.exports = {
entry: ‘./src/index.js’,
output: {
filename: ‘bundle.js’,
},
};
4. What is JSX, and why is it used in React?
Answer:
JSX is a syntax extension for JavaScript that allows you to write HTML elements within JavaScript.
const element = <h1>Hello, JSX!</h1>;
5. Describe the purpose of Create React App in a React project.
Answer:
Create React App is a tool that sets up a new React project with a standardized build configuration.
npx create-react-app my-app
6. How do you embed JavaScript expressions in JSX?
Answer:
JavaScript expressions can be embedded in JSX using curly braces {}.
javascript
Copy code
const name = “John”;
const element = <h1>Hello, {name}!</h1>;
7. What is the difference between JSX and HTML?
Answer:
JSX is syntactically similar to HTML but comes with some differences like the use of className instead of class.
const element = <div className=”container”></div>;
8. Can you use conditional expressions directly in JSX?
Answer:
Yes, you can use ternary operators or logical operators to conditionally render elements in JSX.
const isLoggedIn = true;
const message = <h1>{isLoggedIn ? ‘Welcome back!’ : ‘Please sign in’}</h1>;
9. How do you apply inline styles to a JSX element?
Answer:
Inline styles in JSX are applied using a JavaScript object with camelCased properties.
const style = { color: ‘blue’, fontSize: ’20px’ };
const element = <h1 style={style}>Styled Text</h1>;
10. What are the best practices for writing JSX code?
Answer:
Best practices include keeping JSX code clean, using meaningful variable names, and breaking down large components into smaller ones.
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
11. What is the difference between functional and class components?
Answer:
Functional components are simple JavaScript functions, while class components are ES6 classes with state and lifecycle methods.
// Functional component
function Greeting() {
return <h1>Hello!</h1>;
}
// Class component
class Greeting extends React.Component {
render() {
return <h1>Hello!</h1>;
}
}
12. How do you export and import a React component?
Answer:
You can export a component using export default and import it using import.
// Export
export default function Greeting() {
return <h1>Hello!</h1>;
}
// Import
import Greeting from ‘./Greeting’;
13. What are the naming conventions for React components?
Answer:
React components should be named in PascalCase, where each word starts with an uppercase letter.
function MyComponent() {
return <div>My Component</div>;
}
14. How do you pass data from a parent component to a child component in React?
Answer:
Data is passed to a child component using props.
function Parent() {
return <Child name=”John” />;
}
function Child({ name }) {
return <h1>Hello, {name}!</h1>;
}
15. What is the purpose of the render() method in a class component?
Answer:
The render() method returns the JSX that should be rendered to the DOM.
class MyComponent extends React.Component {
render() {
return <div>Hello, World!</div>;
}
}
16. How do you define state in a functional component?
Answer:
State in a functional component is managed using the useState hook.
function Counter() {
const [count, setCount] = useState(0);
return <button onClick={() => setCount(count + 1)}>Count: {count}</button>;
}
17. What are PropTypes, and how do you use them in a component?
Answer:
PropTypes are used to validate the types of props passed to a component.
import PropTypes from ‘prop-types’;
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
Greeting.propTypes = {
name: PropTypes.string.isRequired,
};
18. Explain the difference between props and state in React.
Answer:
Props are immutable and passed down from parent to child components, while state is mutable and managed within the component.
function Child({ propValue }) {
const [stateValue, setStateValue] = useState(”);
return <div>{propValue} {stateValue}</div>;
}
19. How do you manage multiple state variables in a functional component?
Answer:
Multiple state variables can be managed by calling useState multiple times.
function Form() {
const [name, setName] = useState(”);
const [email, setEmail] = useState(”);
return <div>{name} {email}</div>;
}
20. Why is it important to avoid directly mutating the state in React?
Answer:
Direct mutation of state can lead to unexpected behavior and bugs because React relies on state immutability for efficient re-rendering.
// Incorrect
this.state.value = newValue;
// Correct
this.setState({ value: newValue });
21. How do you handle click events in a React component?
Answer:
Click events are handled by passing a function to the onClick attribute in JSX.
function Button() {
const handleClick = () => alert(‘Button clicked’);
return <button onClick={handleClick}>Click me</button>;
}
22. What is event binding, and why is it needed in React?
Answer:
Event binding ensures that this refers to the correct context when an event handler is called in a class component.
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
console.log(‘Button clicked’);
}
render() {
return <button onClick={this.handleClick}>Click me</button>;
}
}
23. Explain the difference between controlled and uncontrolled components.
Answer:
Controlled components have their input values managed by React state, while uncontrolled components store their values in the DOM.
// Controlled
<input value={this.state.value} onChange={this.handleChange} />
// Uncontrolled
<input defaultValue=”default” ref={this.inputRef} />
24. How do you handle form submission in React?
Answer:
Form submission is handled by attaching an onSubmit event handler to the form element.
function MyForm() {
const handleSubmit = (event) => {
event.preventDefault();
console.log(‘Form submitted’);
};
return <form onSubmit={handleSubmit}>…</form>;
}
25. How do you prevent the default behavior of an event in React?
Answer:
Use the event.preventDefault() method to prevent the default behavior of an event.
function Link() {
const handleClick = (event) => {
event.preventDefault();
console.log(‘Link clicked’);
};
return <a href=”#” onClick={handleClick}>Click me</a>;
}
26. How do you render elements based on a condition in React?
Answer:
Conditional rendering can be achieved using if statements or ternary operators within the JSX.
function Greeting({ isLoggedIn }) {
if (isLoggedIn) {
return <h1>Welcome back!</h1>;
} else {
return <h1>Please sign in</h1>;
}
}
27. Explain how to use the ternary operator for conditional rendering in React.
Answer:
The ternary operator allows you to render different elements based on a condition in a single line of JSX.
const message = isLoggedIn ? ‘Welcome back!’ : ‘Please sign in’;
28. How do you conditionally render an element using the logical && operator in React?
Answer:
The logical && operator renders an element if the condition is true, otherwise, it renders nothing.
const isLoggedIn = true;
return <div>{isLoggedIn && <h1>Welcome back!</h1>}</div>;
29. What are the pros and cons of using if-else statements for conditional rendering in React?
Answer:
If-else statements are clear and easy to understand but can make the JSX verbose and harder to manage as conditions grow complex.
if (condition) {
return <ComponentA />;
} else {
return <ComponentB />;
}
30. How can you render a fallback UI when a certain condition is not met in React?
Answer:
You can render a fallback UI using conditional statements or ternary operators when a condition is not met.
return hasError ? <ErrorComponent /> : <MainComponent />;
31. How do you render a list of items in React?
Answer:
Use the map() function to iterate over an array and render each item as a React element.
const items = [‘Item 1’, ‘Item 2’, ‘Item 3’];
const listItems = items.map(item => <li key={item}>{item}</li>);
32. Why are keys important when rendering lists in React?
Answer:
Keys help React identify which items have changed, are added, or are removed, optimizing the rendering process.
const listItems = items.map(item => <li key={item.id}>{item.name}</li>);
33. What will happen if you forget to add a key to list items in React?
Answer:
React will still render the list, but it will warn you in the console about the missing keys, which can lead to inefficient rendering.
// Missing keys example
const listItems = items.map(item => <li>{item.name}</li>);
34. How can you handle arrays of objects when rendering lists in React?
Answer:
Use the map() function to iterate over the array and render elements based on object properties.
const items = [{ id: 1, name: ‘Item 1’ }, { id: 2, name: ‘Item 2’ }];
const listItems = items.map(item => <li key={item.id}>{item.name}</li>);
35. What is the best practice for assigning keys to dynamically generated list items in React?
Answer:
Use unique identifiers like IDs or indices as keys, but avoid using indices if the list order might change.
const listItems = items.map((item, index) => <li key={item.id}>{item.name}</li>);
36. What is the componentDidMount lifecycle method used for in React?
Answer:
componentDidMount is used to perform actions after the component has been rendered to the DOM, such as fetching data.
componentDidMount() {
fetchData().then(data => this.setState({ data }));
}
37. How do you use componentDidUpdate in a React class component?
Answer:
componentDidUpdate is used to perform actions after the component’s state or props have changed.
componentDidUpdate(prevProps, prevState) {
if (prevState.data !== this.state.data) {
this.doSomethingWithUpdatedData();
}
}
38. When is componentWillUnmount called in a React component?
Answer:
componentWillUnmount is called just before the component is removed from the DOM, allowing you to clean up resources.
componentWillUnmount() {
clearInterval(this.timer);
}
39. How can you trigger a re-render in a class component?
Answer:
A re-render is triggered by calling setState with a new state or when the component receives new props.
this.setState({ count: this.state.count + 1 });
40. What is the purpose of the shouldComponentUpdate lifecycle method?
Answer:
shouldComponentUpdate allows you to control whether a component should re-render when its state or props change.
shouldComponentUpdate(nextProps, nextState) {
return nextState.count !== this.state.count;
}
41. What are React Hooks, and why are they used?
Answer:
React Hooks allow you to use state and other React features in functional components without writing a class.
const [count, setCount] = useState(0);
42. How do you use the useEffect hook to perform side effects in a functional component?
Answer:
useEffect runs side effects like data fetching or subscriptions after every render or when specified dependencies change.
useEffect(() => {
fetchData().then(data => setData(data));
}, []);
43. Explain the rules of Hooks in React.
Answer:
Hooks must be called at the top level of a component and only inside React functional components or custom hooks.
// Correct usage
function MyComponent() {
const [count, setCount] = useState(0);
}
// Incorrect usage
if (condition) {
const [count, setCount] = useState(0);
}
44. How do you create a custom hook in React?
Answer:
A custom hook is a JavaScript function that starts with use and can call other hooks inside it.
function useCustomHook() {
const [state, setState] = useState(initialState);
// Custom logic
return [state, setState];
}
45. What is the purpose of the useReducer hook, and when should you use it?
Answer:
useReducer is used for managing complex state logic in functional components, similar to how a reducer works in Redux.
const [state, dispatch] = useReducer(reducer, initialState);
46. How do you set up React Router in a React application?
Answer:
Install React Router and wrap your app with BrowserRouter, then define routes using Route.
import { BrowserRouter as Router, Route } from ‘react-router-dom’;
function App() {
return (
<Router>
<Route path=”/” component={Home} />
</Router>
);
}
47. How do you navigate between different pages in a React application?
Answer:
Use the Link or NavLink components to navigate between different routes in a React app.
<Link to=”/about”>About</Link>
48. What are URL parameters in React Router, and how do you use them?
Answer:
URL parameters are dynamic parts of the URL that you can access in your component via useParams.
function Profile() {
let { id } = useParams();
return <h1>Profile ID: {id}</h1>;
}
49. How do you define a route that catches all unmatched paths in React Router?
Answer:
Use the Switch component with a wildcard * route as the last route to catch all unmatched paths.
<Switch>
<Route path=”/” exact component={Home} />
<Route path=”*” component={NotFound} />
</Switch>
50. What is the difference between Link and NavLink in React Router?
Answer:
NavLink is an extended version of Link that adds styling to the active route.
<NavLink to=”/about” activeClassName=”active”>About</NavLink>
Final Words
Getting ready for an interview can feel overwhelming, but going through these React fresher interview questions can help you feel more confident. This guide focuses on the kinds of React developer interview questions for fresher roles that you’re likely to face.
Don’t forget to practice the React basic coding interview questions too! With the right preparation, you’ll ace your React interview and take that important step in your career.
Frequently Asked Questions
1. What are the most common interview questions for React JS?
The most common ReactJS interview questions cover components, state management, props, lifecycle methods, and hooks.
2. What are the important React topics freshers should focus on for interviews?
Freshers should focus on components, state and props, lifecycle methods, hooks, and basic routing.
3. How should freshers prepare for React technical interviews?
Freshers should practice building simple React applications, understand core concepts, and review common interview questions.
4. What strategies can freshers use to solve React coding questions during interviews?
Freshers should break down the problem, write clean code, and use React’s debugging tools to ensure functionality.
5. Should freshers prepare for advanced React topics in interviews?
Yes, depending on the role, freshers might need to know advanced topics like Redux, context API, and performance optimization.
Explore More React Resources
Explore More Interview Questions
Related Posts
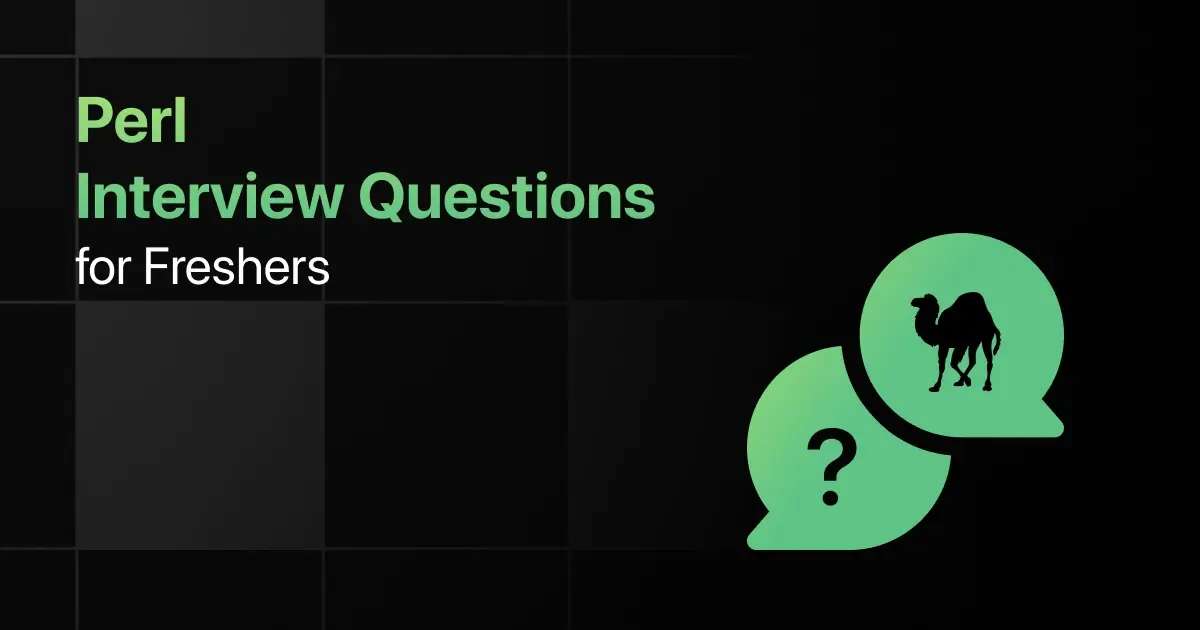
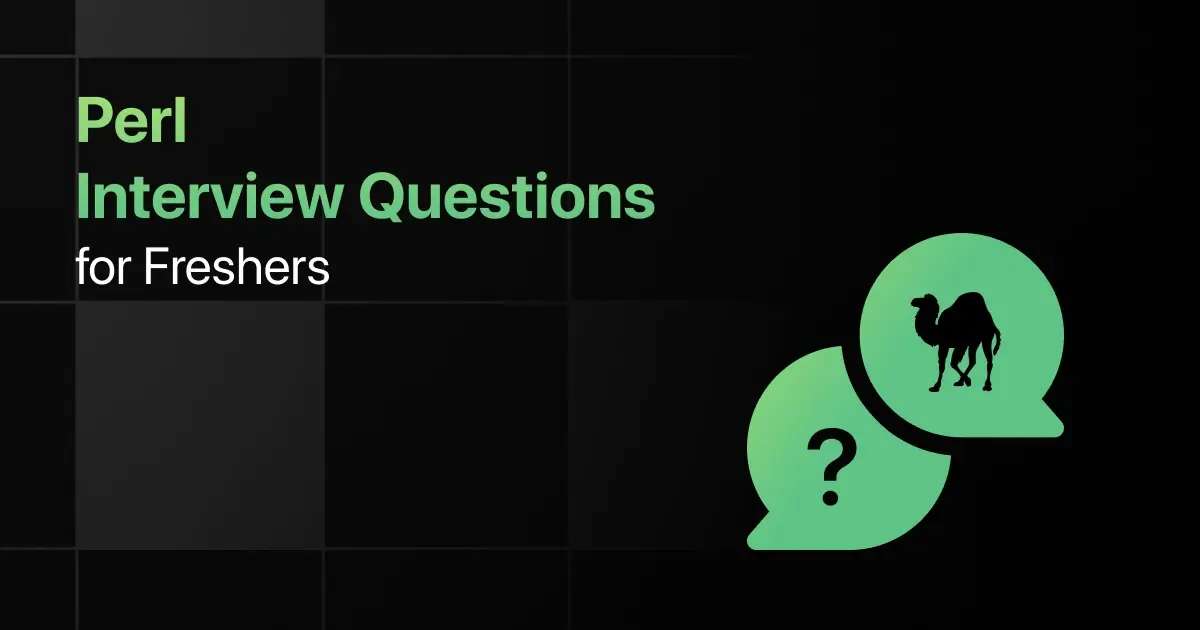
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …