Top PHP Interview Questions for Freshers
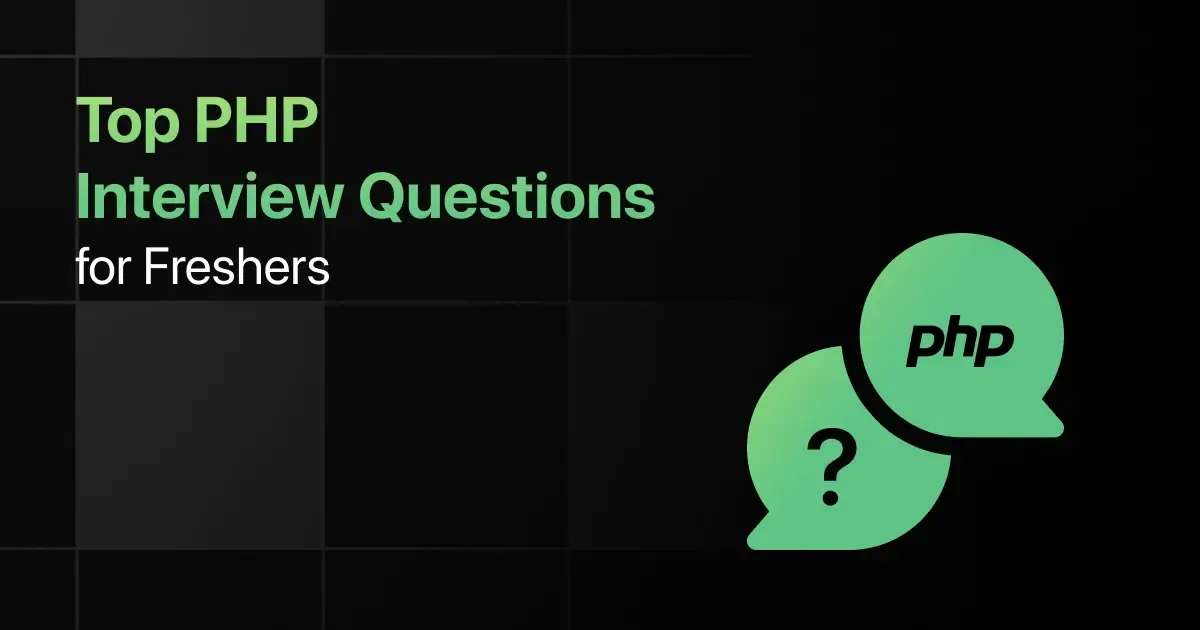
Are you preparing for your first PHP interview and wondering what questions you might face?
Understanding the key PHP interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these PHP interview questions and answers for freshers and make a strong impression in your interview.
Practice PHP Interview Questions and Answers
Below are the top 50 PHP interview questions for freshers with answers:
1. How can you connect to a MySQL database using PHP?
Answer:
To connect to a MySQL database in PHP, you can use the mysqli_connect() function, which requires parameters like host, username, password, and database name. It establishes a connection to the MySQL database server.
$connection = mysqli_connect(“localhost”, “username”, “password”, “database”);
2. What is the purpose of the isset() function in PHP, and how do you use it?
Answer:
The isset() function checks if a variable is set and is not null. It returns true if the variable exists and has a value other than null, which is useful for ensuring that variables are defined before usage.
if (isset($variable)) {
// Code to execute
}
3. How can you handle file uploads in PHP?
Answer:
To handle file uploads in PHP, you can use the $_FILES superglobal to access the uploaded file’s information, and then move the file from the temporary directory to a desired location using the move_uploaded_file() function.
move_uploaded_file($_FILES[‘file’][‘tmp_name’], ‘uploads/’ . $_FILES[‘file’][‘name’]);
4. Explain how you would implement form validation in PHP.
Answer:
Form validation in PHP involves checking that the form data meets specific criteria, such as required fields, valid email formats, or string lengths, typically done using conditional statements and regular expressions before processing the form.
if ($_SERVER[“REQUEST_METHOD”] == “POST”) {
if (empty($_POST[“name”])) {
$nameErr = “Name is required”;
}
}
5. How do you prevent SQL injection in PHP?
Answer:
To prevent SQL injection in PHP, you should use prepared statements with bound parameters provided by PDO or MySQLi. This ensures that user inputs are treated as data, not executable SQL code.
$stmt = $conn->prepare(“SELECT * FROM users WHERE username = ?”);
$stmt->bind_param(“s”, $username);
$stmt->execute();
6. What is the difference between include and require in PHP?
Answer:
The include statement will produce a warning and allow the script to continue if the file cannot be found, while require will produce a fatal error and halt script execution if the file is missing.
include ‘header.php’;
require ‘config.php’;
7. How can you create a session in PHP, and what is its purpose?
Answer:
A session in PHP is created using session_start() and is used to store user-specific data across multiple pages. Sessions allow you to preserve user state and data between page requests.
session_start();
$_SESSION[‘username’] = ‘JohnDoe’;
8. How do you send an email using PHP?
Answer:
To send an email in PHP, you can use the mail() function, which requires parameters like recipient, subject, message, and headers. This function uses the server’s mail configuration to dispatch the email.
mail(“[email protected]”, “Subject”, “Message”, “From: [email protected]”);
9. What are cookies in PHP, and how can you set and retrieve them?
Answer:
Cookies are small files stored on the client’s machine used to track user data. You set a cookie using setcookie() and retrieve it using the $_COOKIE superglobal array.
setcookie(“user”, “John Doe”, time() + (86400 * 30), “/”);
echo $_COOKIE[‘user’];
10. How can you use sessions to manage user authentication in PHP?
Answer:
In PHP, sessions can manage user authentication by storing the user’s unique identifier, like a user ID, in a session variable upon successful login. Subsequent pages check this session variable to verify the user’s authentication status.
$_SESSION[‘user_id’] = $user_id;
if (!isset($_SESSION[‘user_id’])) {
header(“Location: login.php”);
}
11. What are PHP Data Objects (PDO), and why are they important?
Answer:
PHP Data Objects (PDO) provide a consistent interface for accessing databases. They support various database types, allowing you to switch databases without changing the code. PDO also supports prepared statements, enhancing security.
$pdo = new PDO(‘mysql:host=localhost;dbname=testdb’, $user, $pass);
12. How can you implement file handling in PHP, such as reading from a file?
Answer:
File handling in PHP is done using functions like fopen() to open a file, fread() to read the content, and fclose() to close the file. It allows for operations like reading, writing, and appending to files.
$file = fopen(“example.txt”, “r”);
$content = fread($file, filesize(“example.txt”));
fclose($file);
13. What is the use of the explode() function in PHP?
Answer:
The explode() function in PHP splits a string into an array based on a specified delimiter, making it useful for parsing strings into manageable parts, such as when handling comma-separated values.
$array = explode(“,”, “apple,banana,orange”);
14. Explain how to handle errors and exceptions in PHP.
Answer:
In PHP, errors can be handled using custom error handlers with set_error_handler(), and exceptions are handled using try-catch blocks to catch and manage exceptions gracefully, ensuring the application remains stable.
try {
// Code that may throw an exception
} catch (Exception $e) {
echo ‘Caught exception: ‘, $e->getMessage(), “\n”;
}
15. How would you implement a basic search functionality in PHP?
Answer:
To implement basic search functionality in PHP, retrieve search input from a form, query the database using LIKE with wildcards in SQL, and display the results to the user.
$search = $_POST[‘search’];
$query = “SELECT * FROM products WHERE name LIKE ‘%$search%'”;
16. What is a prepared statement in PHP, and how is it used?
Answer:
A prepared statement in PHP is used to execute the same SQL query multiple times with different values securely, protecting against SQL injection by separating SQL logic from data.
$stmt = $pdo->prepare(“SELECT * FROM users WHERE email = ?”);
$stmt->execute([$email]);
17. How can you sanitize user input in PHP?
Answer:
Sanitizing user input in PHP involves using functions like filter_var() to remove or encode unwanted characters from input data, protecting the application from malicious input and ensuring data integrity.
$email = filter_var($_POST[’email’], FILTER_SANITIZE_EMAIL);
18. How do you manage file permissions in PHP?
Answer:
File permissions in PHP can be managed using the chmod() function, which changes the permissions of a specified file, controlling who can read, write, or execute the file.
chmod(“example.txt”, 0755);
19. Explain how to use foreach loops in PHP for iterating over arrays.
Answer:
foreach loops in PHP iterate over each element in an array, allowing you to access both the key and value pairs easily, making it ideal for processing arrays and associative arrays.
foreach ($array as $key => $value) {
echo “$key => $value”;
}
20. How can you manage multiple form submissions in PHP?
Answer:
To manage multiple form submissions in PHP, use unique tokens stored in sessions to check if a form has already been submitted, preventing duplicate actions such as double-posting data.
if ($_SESSION[‘token’] == $_POST[‘token’]) {
// Process form
}
21. What are traits in PHP, and how do you use them?
Answer:
Traits in PHP are reusable pieces of code that can be included in multiple classes to avoid code duplication. They allow you to share methods between classes without using inheritance.
trait Logger {
public function log($message) {
echo $message;
}
}
22. How can you implement pagination in PHP for large data sets?
Answer:
Pagination in PHP involves calculating the number of pages required, retrieving only a subset of records from the database based on the current page, and displaying navigation links to other pages.
$offset = ($page – 1) * $limit;
$query = “SELECT * FROM products LIMIT $offset, $limit”;
23. How do you handle date and time in PHP?
Answer:
Handling date and time in PHP is done using the DateTime class, which provides methods for manipulating and formatting dates and times, making it easier to work with different time zones and formats.
$date = new DateTime();
echo $date->format(‘Y-m-d H:i:s’);
24. Explain how to use header() in PHP to redirect users to another page.
Answer:
The header() function in PHP sends a raw HTTP header to the browser, allowing you to redirect users to another page by specifying the location URL, which must be called before any output is sent.
header(“Location: homepage.php”);
exit();
25. How can you generate a random string in PHP for use in passwords or tokens?
Answer:
To generate a random string in PHP, you can use the bin2hex() function in combination with random_bytes() to create a secure, random string suitable for passwords or tokens.
$token = bin2hex(random_bytes(16));
26. What is the use of json_encode() and json_decode() in PHP?
Answer:
json_encode() converts PHP arrays or objects into a JSON string, while json_decode() converts a JSON string back into a PHP array or object, facilitating data exchange between PHP and JavaScript.
$json = json_encode($array);
$array = json_decode($json, true);
27. How can you secure file uploads in PHP to prevent malicious files from being uploaded?
Answer:
Securing file uploads in PHP involves validating the file type, size, and name, checking for file extension and MIME type, and storing uploaded files outside the web root to prevent direct access.
if ($_FILES[‘file’][‘type’] == ‘image/jpeg’) {
move_uploaded_file($_FILES[‘file’][‘tmp_name’], ‘uploads/’ . $_FILES[‘file’][‘name’]);
}
28. Explain how to use sessions to store shopping cart data in PHP.
Answer:
In PHP, you can use sessions to store shopping cart data by saving product IDs and quantities in a session array, which persists across page reloads, allowing users to manage their cart without logging in.
$_SESSION[‘cart’][$product_id] = $quantity;
29. How can you create a custom error handler in PHP?
Answer:
A custom error handler in PHP is created using set_error_handler(), where you define a function to handle different error levels, logging errors to a file, or displaying user-friendly messages.
function customError($errno, $errstr) {
echo “Error: [$errno] $errstr”;
}
set_error_handler(“customError”);
30. What is the difference between == and === in PHP?
Answer:
In PHP, == checks for equality after type juggling, meaning it converts types if necessary, while === checks for both value and type equality, ensuring no type conversion occurs.
if ($a == $b) {
// Equal values, type not checked
}
if ($a === $b) {
// Equal values and types
}
31. How can you implement a login system using sessions in PHP?
Answer:
To implement a login system in PHP, verify user credentials against a database, and if valid, start a session and store the user’s ID or username in a session variable to maintain their logged-in state.
if ($isValidUser) {
$_SESSION[‘user_id’] = $user_id;
}
32. How do you handle JSON data in PHP for API responses?
Answer:
Handling JSON data in PHP for API responses involves encoding PHP data into JSON format using json_encode() and setting the appropriate content-type header to indicate that the response is JSON.
header(‘Content-Type: application/json’);
echo json_encode($response);
33. What is the significance of require_once in PHP?
Answer:
require_once in PHP includes and evaluates a file only once, preventing the same file from being included multiple times, which can avoid redeclaration errors and improve code organization.
require_once ‘config.php’;
34. Explain how you can use curl in PHP to make HTTP requests.
Answer:
curl in PHP is used to make HTTP requests by initializing a curl session with curl_init(), setting options like URL and method with curl_setopt(), executing the request with curl_exec(), and closing the session with curl_close().
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, “http://example.com”);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
$response = curl_exec($ch);
curl_close($ch);
35. How can you perform data validation using regular expressions in PHP?
Answer:
In PHP, data validation using regular expressions is done with functions like preg_match() to check if a string matches a pattern, ensuring data conforms to expected formats such as email addresses or phone numbers.
if (preg_match(“/^[a-zA-Z0-9]+@[a-zA-Z0-9]+\.[a-zA-Z]{2,6}$/”, $email)) {
echo “Valid email address”;
}
36. How would you implement a rate-limiting feature in PHP?
Answer:
To implement rate limiting in PHP, track the number of requests a user makes within a specified time frame using sessions or a database, and block further requests if the limit is exceeded.
if ($_SESSION[‘request_count’] > $limit && time() – $_SESSION[‘start_time’] < $timeWindow) {
// Block request
}
37. What are the steps to create a RESTful API using PHP?
Answer:
Creating a RESTful API in PHP involves setting up a routing mechanism to handle different HTTP methods, processing incoming requests, interacting with a database, and returning responses in JSON format.
// Example route handling
if ($_SERVER[‘REQUEST_METHOD’] == ‘GET’) {
// Fetch and return data
}
38. How do you handle time zones in PHP?
Answer:
Handling time zones in PHP involves setting the default time zone using date_default_timezone_set() and using DateTime objects to manipulate dates and times across different time zones.
date_default_timezone_set(‘Asia/Kolkata’);
$date = new DateTime(‘now’);
39. What is the importance of autoload in PHP, and how do you use it?
Answer:
autoload in PHP automatically loads classes when they are needed, avoiding manual inclusion. It’s done using spl_autoload_register() to register a function that specifies how to locate and load class files.
spl_autoload_register(function ($class_name) {
include $class_name . ‘.php’;
});
40. Explain how to use fgetcsv() to read CSV files in PHP.
Answer:
fgetcsv() in PHP reads a line from a CSV file and parses it into an array, making it useful for processing data from CSV files line by line in a structured format.
$handle = fopen(“file.csv”, “r”);
while (($data = fgetcsv($handle, 1000, “,”)) !== FALSE) {
print_r($data);
}
fclose($handle);
41. How can you implement a recursive function in PHP, and when is it useful?
Answer:
A recursive function in PHP calls itself to solve smaller instances of the same problem, useful in scenarios like traversing directories or calculating factorials, where a problem can be broken down into simpler sub-problems.
function factorial($n) {
return ($n == 0) ? 1 : $n * factorial($n – 1);
}
42. How do you work with multi-dimensional arrays in PHP?
Answer:
Working with multi-dimensional arrays in PHP involves nested loops to access or modify elements in arrays that contain other arrays, useful for managing complex data structures like matrices.
foreach ($multiArray as $row) {
foreach ($row as $element) {
echo $element;
}
}
43. What are the steps to create a file download feature in PHP?
Answer:
Creating a file download feature in PHP involves setting the appropriate headers using header(), specifying the content type, disposition, and size, then reading the file and sending it to the browser.
header(‘Content-Type: application/octet-stream’);
header(‘Content-Disposition: attachment; filename=”file.txt”‘);
readfile(‘path/to/file.txt’);
44. How can you create a secure user authentication system in PHP?
Answer:
A secure user authentication system in PHP involves using hashed passwords with password_hash() and password_verify(), storing session tokens, and implementing measures like two-factor authentication.
$hashedPassword = password_hash($password, PASSWORD_DEFAULT);
if (password_verify($password, $hashedPassword)) {
// Grant access
}
45. Explain how to use array_map() in PHP to apply a function to array elements.
Answer:
array_map() in PHP applies a callback function to each element of an array, returning a new array with the modified elements, useful for processing or transforming data in an array.
$numbers = [1, 2, 3, 4];
$squares = array_map(function($n) {
return $n * $n;
}, $numbers);
46. What is the difference between strpos() and strstr() in PHP?
Answer:
strpos() in PHP returns the position of the first occurrence of a substring in a string, while strstr() returns the portion of the string starting from the first occurrence of a substring, useful for string searching and manipulation.
$pos = strpos(“hello world”, “world”);
$substring = strstr(“hello world”, “world”);
47. How can you create a dynamic dropdown list in PHP?
Answer:
Creating a dynamic dropdown list in PHP involves fetching data from a database and populating the <option> tags in a <select> element, allowing users to select from a list of dynamically generated options.
$options = $db->query(“SELECT name FROM categories”);
echo “<select>”;
while ($row = $options->fetch_assoc()) {
echo “<option value='{$row[‘name’]}’>{$row[‘name’]}</option>”;
}
echo “</select>”;
48. How do you implement AJAX in PHP for updating parts of a webpage without reloading?
Answer:
To implement AJAX in PHP, use JavaScript to send an asynchronous request to a PHP script, process the request on the server, and return a response that is used to update the webpage dynamically.
$.ajax({
url: ‘process.php’,
type: ‘POST’,
data: { id: 1 },
success: function(response) {
$(‘#result’).html(response);
}
});
49. What are the steps to create a secure file download feature in PHP?
Answer:
A secure file download feature in PHP involves validating the user’s permissions, sanitizing the file path to prevent directory traversal attacks, and then serving the file with appropriate headers.
$file = basename($_GET[‘file’]);
$filePath = ‘/secure/path/’ . $file;
if (file_exists($filePath) && is_readable($filePath)) {
header(‘Content-Type: application/octet-stream’);
header(‘Content-Disposition: attachment; filename=”‘ . $file . ‘”‘);
readfile($filePath);
}
50. How can you use PDO::lastInsertId() in PHP, and why is it important?
Answer:
PDO::lastInsertId() in PHP retrieves the ID of the last inserted row, crucial for operations that depend on the unique ID of newly inserted records, especially in relational databases.
$pdo->exec(“INSERT INTO users (name) VALUES (‘John Doe’)”);
$lastId = $pdo->lastInsertId();
Final Words
Getting ready for an interview can feel overwhelming, but going through these PHP fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your PHP interview but don’t forget to practice the PHP basic syntax, form handling, and database integration-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for PHP?
Common interview questions for PHP typically focus on syntax, array handling, error handling, and working with databases.
2. What are the important PHP topics freshers should focus on for interviews?
Important PHP topics freshers should focus on include form handling, sessions, cookies, file handling, and MySQL integration.
3. How should freshers prepare for PHP technical interviews?
Freshers should prepare for PHP technical interviews by practicing coding problems, reviewing common functions, and understanding web development basics.
4. What strategies can freshers use to solve PHP coding questions during interviews?
Freshers can use strategies like understanding the problem requirements, writing clean and modular code, and testing for various input scenarios to solve PHP coding questions during interviews.
5. Should freshers prepare for advanced PHP topics in interviews?
Yes, freshers should prepare for advanced PHP topics in interviews if applying for roles that require in-depth knowledge or if the job description specifies them.
Explore More PHP Resources
Explore More Interview Questions
Related Posts
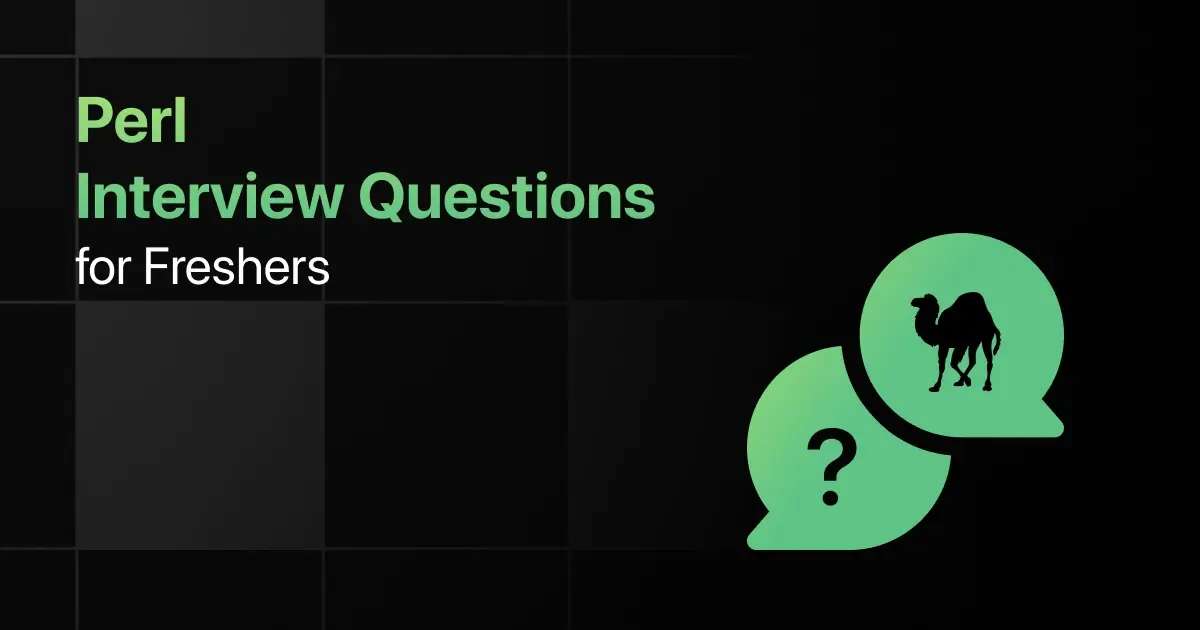
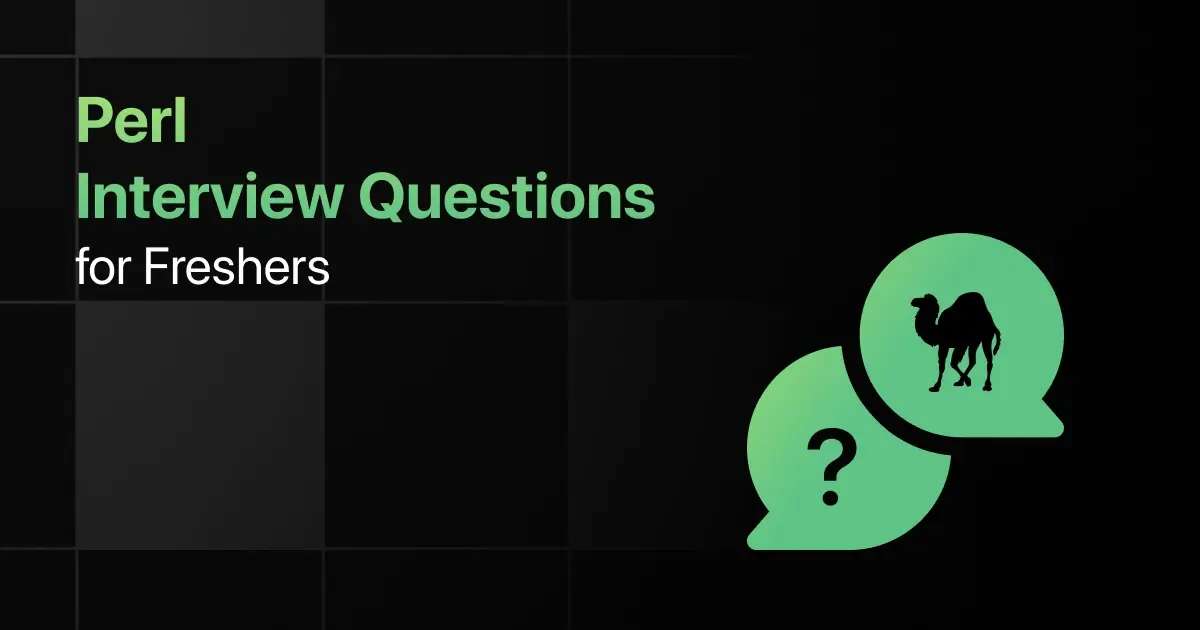
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …