Top Perl Interview Questions for Freshers
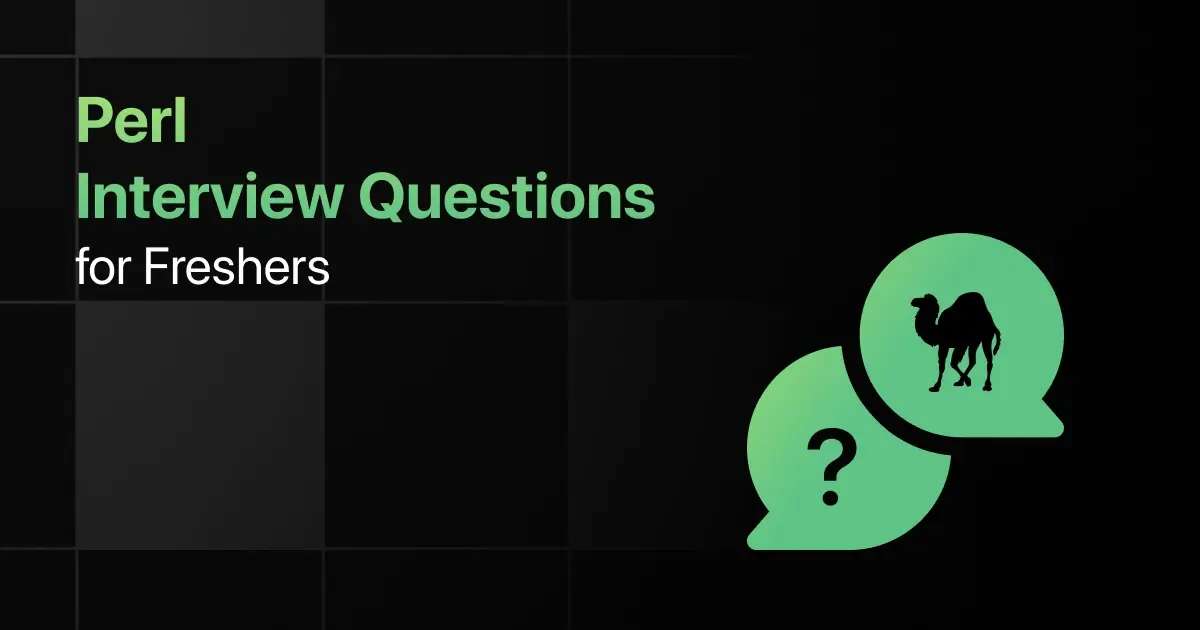
Are you preparing for your first Perl interview and wondering what questions you might face?
Understanding the key Perl interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Perl interview questions and answers for freshers and make a strong impression in your interview.
Practice Perl Interview Questions and Answers
Below are the top 50 Perl interview questions for freshers with answers:
1. What is Perl and what are its main features?
Answer:
Perl is a high-level, interpreted programming language known for its flexibility and text-processing capabilities. It supports object-oriented, procedural, and functional programming paradigms, making it versatile for various applications.
2. How do you declare a variable in Perl?
Answer:
Variables in Perl are declared using the my, our, or local keywords. The my keyword is used for lexically scoped variables.
3. What are the different types of variables in Perl?
Answer:
Perl has three main variable types: scalars (single values), arrays (ordered lists), and hashes (key-value pairs). Each type is prefixed with a different sigil: $ for scalars, @ for arrays, and % for hashes.
4. How do you create and access an array in Perl?
Answer:
An array is created by listing values in parentheses and accessed using an index. Perl arrays are zero-indexed.
print $array[0]; # Output: 1
5. How do you create a hash in Perl and access its elements?
Answer:
A hash is created using key-value pairs and accessed using the key. Keys and values are separated by the => operator.
print $hash{name}; # Output: Alice
6. What is the difference between == and eq in Perl?
Answer:
== is used for numeric comparison, while eq is used for string comparison. Using the appropriate operator is crucial to avoid unexpected behavior.
7. How do you perform regular expression matching in Perl?
Answer:
Regular expression matching in Perl is done using the m// operator. You can use modifiers like i for case-insensitive matching.
print “Match found!”;
}
8. How do you define a subroutine in Perl?
Answer:
A subroutine is defined using the sub keyword followed by the subroutine name and curly braces. Subroutines can take parameters and return values.
my ($name) = @_;
return “Hello, $name!”;
}
9. What is the purpose of the strict pragma in Perl?
Answer:
The strict pragma enforces stringent rules on variable declarations and scope, helping to catch potential errors and improve code reliability. It requires explicit declaration of variables before use.
10. How do you read a file in Perl?
Answer:
To read a file in Perl, use the open function to create a filehandle and the <$filehandle> operator to read lines from the file. Always close the filehandle after use.
while (my $line = <$fh>) {
print $line;
}
close($fh);
11. How do you write to a file in Perl?
Answer:
To write to a file, use the open function with the > mode and the print function to write data. Ensure to close the filehandle after writing.
print $fh “Hello, World!”;
close($fh);
12. What are Perl modules, and how do you use them?
Answer:
Perl modules are packages of reusable code that can be included in your scripts using the use keyword. Modules can provide additional functionality and help organize code.
use warnings;
13. How do you handle errors in Perl?
Answer:
Errors in Perl can be handled using the eval block to catch exceptions. You can check the special variable $@ to determine if an error occurred.
# Code that may cause an error
};
if ($@) {
print “Error: $@”;
}
14. What is the purpose of the warnings pragma in Perl?
Answer:
The warnings pragma enables warnings for potentially problematic code. It helps identify issues without stopping program execution, allowing for easier debugging.
15. How do you use the map function in Perl?
Answer:
The map function applies a block of code to each element of a list and returns a new list with the results. It’s useful for transforming data.
16. How do you use the grep function in Perl?
Answer:
The grep function filters a list based on a condition specified in a block, returning only the elements that satisfy the condition.
17. What is the significance of the @_ array in Perl subroutines?
Answer:
The @_ array contains the parameters passed to a subroutine. It’s automatically populated with the arguments when the subroutine is called.
18. How do you perform string interpolation in Perl?
Answer:
String interpolation occurs when a variable is included in double quotes, allowing its value to be evaluated and included in the string.
print “Hello, $name!”; # Output: Hello, Alice!
19. How do you create a package in Perl?
Answer:
A package in Perl is created using the package keyword, allowing you to define a namespace for your variables and subroutines. This helps organize code into modules.
20. How do you handle command-line arguments in Perl?
Answer:
Command-line arguments in Perl are accessed via the @ARGV array, which contains all arguments passed to the script when executed.
21. What are the different ways to loop in Perl?
Answer:
Perl supports several looping constructs, including for, foreach, while, and until. Each has its specific use case depending on the requirement.
print $item;
}
22. How do you create a multi-dimensional array in Perl?
Answer:
A multi-dimensional array in Perl is created by nesting arrays within arrays, allowing for complex data structures.
print $matrix[0][1]; # Output: 2
23. What is the difference between sort and reverse in Perl?
Answer:
sort organizes elements of an array based on specified criteria, while reverse reverses the order of elements in an array. They can be combined for more complex sorting.
my @reversed = reverse @array;
24. How do you check if a file exists in Perl?
Answer:
You can check for a file’s existence using the -e file test operator, which returns true if the file exists.
print “File exists.”;
}
25. What is the use strict pragma, and how does it help in Perl?
Answer:
The use strict pragma enforces strict variable declaration rules, which helps prevent errors due to typos and unintended variable use, leading to cleaner, more maintainable code.
26. How do you use the join function in Perl?
Answer:
The join function concatenates elements of an array into a single string, using a specified delimiter.
27. How do you use the split function in Perl?
Answer:
The split function divides a string into a list of substrings based on a specified delimiter and returns the list.
28. What is context in Perl?
Answer:
Context in Perl refers to the expected behavior of an expression, either in list context (returning a list) or scalar context (returning a single value). Functions may behave differently depending on the context in which they are called.
29. How do you create a reference in Perl?
Answer:
A reference in Perl is created using the backslash \ operator, allowing you to create complex data structures like arrays and hashes.
30. What is the difference between die and warn in Perl?
Answer:
die terminates the program and outputs an error message, while warn generates a warning message but allows the program to continue executing. Use die for fatal errors and warn for non-critical issues.
31. How do you create a regular expression that matches an email address in Perl?
Answer:
A simple regex for matching email addresses can use the m// operator to check for valid formats, though a comprehensive regex is more complex.
print “Valid email.”;
}
32. How do you implement object-oriented programming in Perl?
Answer:
Object-oriented programming in Perl is implemented using packages, constructors, and method definitions. You can create objects using the bless function.
sub new { bless {}, shift }
33. What is the AUTOLOAD mechanism in Perl?
Answer:
The AUTOLOAD mechanism allows you to create methods dynamically in a package, making it easier to handle method calls that are not explicitly defined. It helps implement features like proxies or dynamic dispatch.
34. How do you perform a hash slice in Perl?
Answer:
A hash slice allows you to retrieve multiple values from a hash at once using a list of keys.
35. What is the purpose of the use feature pragma in Perl?
Answer:
The use feature pragma enables the use of features and syntactic sugar introduced in recent Perl versions, allowing access to newer language capabilities.
say “Hello, World!”;
36. How do you handle undefined values in Perl?
Answer:
You can check for undefined values using the defined function, which returns true if a value is defined and false otherwise.
print “Variable is defined.”;
}
37. How do you create and use a custom error handling routine in Perl?
Answer:
You can create a custom error handling routine using the eval block to catch exceptions and handle them as needed.
my ($message) = @_;
print “Error: $message”;
}
38. How do you perform a database connection in Perl?
Answer:
To connect to a database, you typically use the DBI module and its connect method, providing the database source, username, and password.
my $dbh = DBI->connect(‘DBI:mysql:database’, ‘user’, ‘password’);
39. How do you execute a SQL query using Perl?
Answer:
After establishing a database connection, use the prepare and execute methods from the DBI module to run a SQL query.
$sth->execute();
40. What is the purpose of the chomp function in Perl?
Answer:
The chomp function removes the newline character from the end of a string, making it useful for cleaning up input data.
41. How do you implement a simple web server using Perl?
Answer:
You can implement a simple web server using the HTTP::Server::Simple module to handle HTTP requests and responses.
my $server = HTTP::Server::Simple->new(8080);
42. What is bless in Perl?
Answer:
The bless function associates an object (a reference) with a class, allowing you to use the object-oriented features of Perl.
43. How do you implement a singleton class in Perl?
Answer:
A singleton class can be implemented by controlling instance creation through a private constructor and storing the single instance within the class itself.
state $instance; # Only created once
return $instance //= bless {}, shift;
}
44. How do you create a CSV file in Perl?
Answer:
You can create a CSV file using the Text::CSV module, which simplifies writing and reading CSV data.
my $csv = Text::CSV->new();
$csv->print($fh, [ ‘header1’, ‘header2’ ]);
45. How do you perform a deep copy of a data structure in Perl?
Answer:
A deep copy can be performed using the Storable module, which allows you to serialize and deserialize data structures.
my $copy = dclone($original);
46. How do you use Perl for web scraping?
Answer:
You can use modules like LWP::UserAgent to make HTTP requests and HTML::TreeBuilder or Mojo::DOM to parse HTML content for web scraping.
my $ua = LWP::UserAgent->new();
my $response = $ua->get(‘http://example.com’);
47. What is the purpose of the tie function in Perl?
Answer:
The tie function allows you to bind a variable to an object that provides methods to manipulate the variable, enabling custom behaviors for built-in data types.
48. How do you use a Perl regex to validate a phone number format?
Answer:
You can use regular expressions to check if a phone number matches a specific format by using the m// operator.
print “Valid phone number.”;
}
49. How do you debug Perl scripts?
Answer:
You can use the perl -d command to run your script in debugging mode, allowing you to set breakpoints, inspect variables, and step through the code.
50. How do you implement a queue in Perl?
Answer:
A simple queue can be implemented using an array, utilizing push to enqueue and shift to dequeue elements.
push @queue, ‘item1’;
my $item = shift @queue; # Dequeue
Final Words
Getting ready for an interview can feel overwhelming, but going through these Perl fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Perl interview but don’t forget to practice regular expressions, file handling, and Perl scripting-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for Perl?
The most common Perl interview questions focus on regular expressions, file handling, and scalar vs list context.
2. What are the important Perl topics freshers should focus on for interviews?
Freshers should focus on Perl basics like data structures (arrays, hashes), control flow, and regular expressions.
3. How should freshers prepare for Perl technical interviews?
Freshers should prepare by practicing Perl scripts, learning common Perl modules, and understanding regular expressions.
4. What strategies can freshers use to solve Perl coding questions during interviews?
Freshers can solve Perl coding questions by breaking problems into manageable parts, leveraging Perl’s text processing strengths, and using built-in functions effectively.
5. Should freshers prepare for advanced Perl topics in interviews?
Yes, freshers should prepare for advanced Perl topics like object-oriented programming (OOP) in Perl and working with CPAN modules, depending on the job requirements.
Explore More Perl Resources
Explore More Interview Questions
- Python
- Java
- SQL
- React
- JavaScript
- C Programming
- HTML
- CSS
- Angular
- C++
- Spring Boot
- Node JS
- Excel
- C#
- DBMS
- PHP
- Linux
- Operating System
- MySQL
- Spring
- Flutter
- MongoDB
- Django
- React Native
- jQuery
- Bootstrap
- Embedded C
- DSA
- R Programming
- Hadoop
- .NET
- Power BI
- ASP.NET
- ASP.NET MVC
- Android
- Tableau
- MVC
- WordPress
- TypeScript
- Spark
- Kotlin
- Swift
- Golang
- Shell Scripting
- iOS
- Spring MVC
- Next JS
- AWS
- Kubernetes
- Docker
- Cyber Security
- Azure
- Terraform
- Vue JS
- UiPath
- Scala
- Flask
- RPA
- MATLAB
- Figma
- React Redux
Related Posts
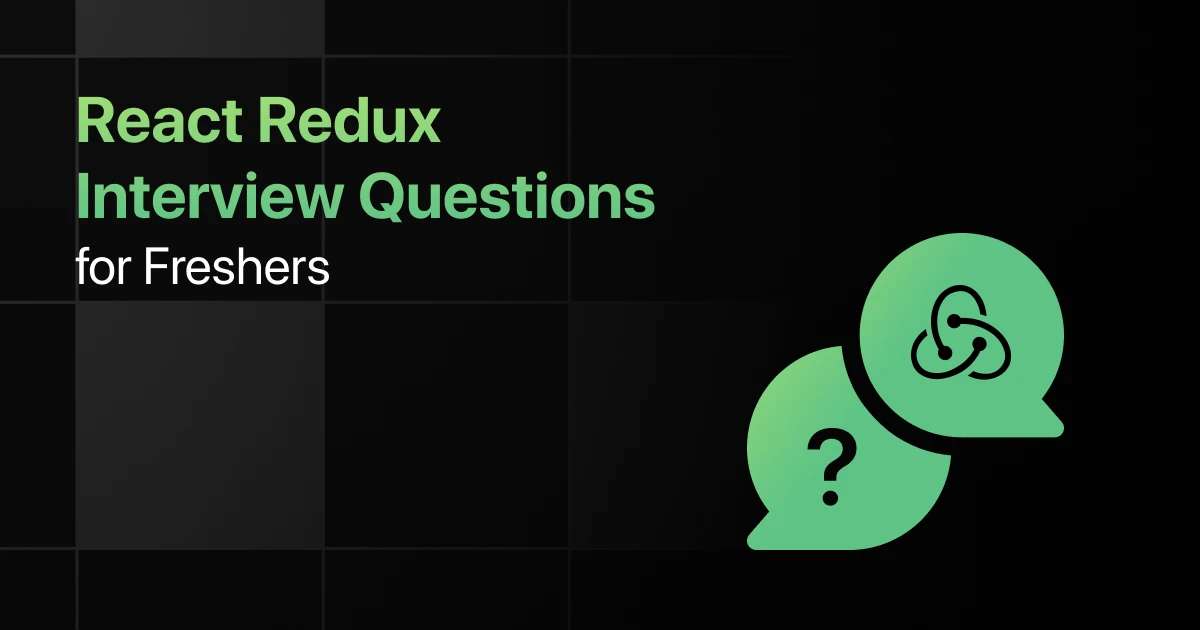
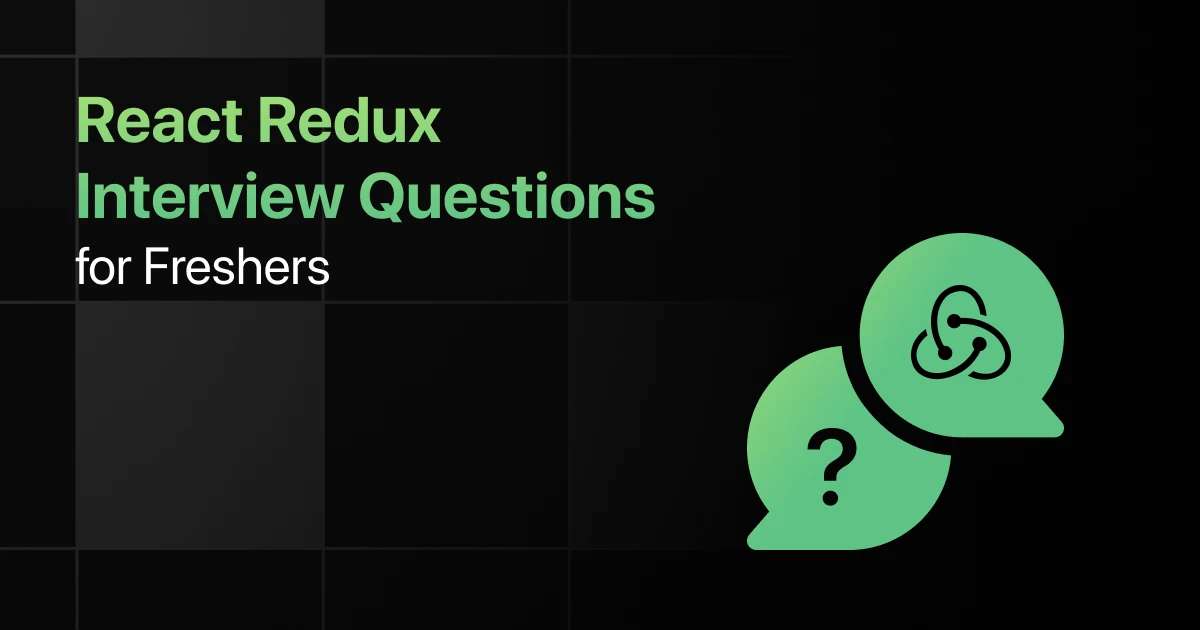
Top React Redux Interview Questions for Freshers
Are you preparing for your first React Redux interview and wondering what questions you might face? Understanding the key React Redux …