Top Node JS Interview Questions for Freshers
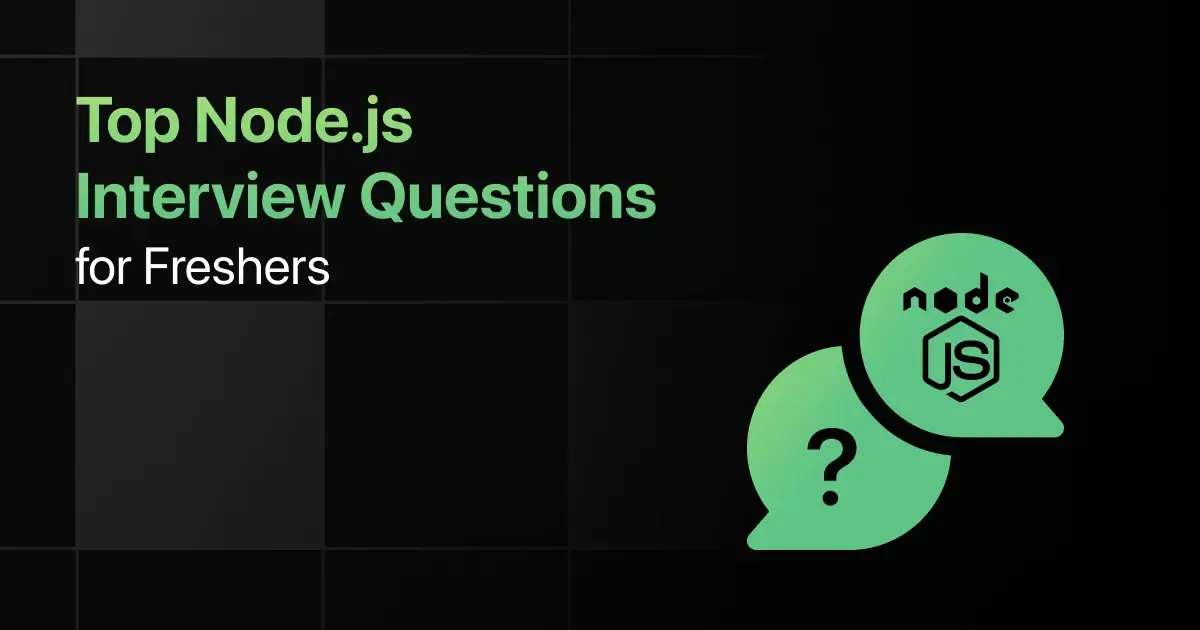
Are you preparing for your first Node.js interview and wondering what questions you might face?
Understanding the key Node.js interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Node.js interview questions and answers for freshers and make a strong impression in your interview.
Practice Node JS Interview Questions and Answers
Below are the top 50 Node JS interview questions for freshers with answers:
1. How do you create a basic HTTP server in Node.js?
Answer:
Use the http module to create a server that listens on a specified port and responds to client requests.
const http = require(‘http’);
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader(‘Content-Type’, ‘text/plain’);
res.end(‘Hello, World!’);
});
server.listen(3000);
2. What is the event loop in Node.js, and how does it handle asynchronous operations?
Answer:
The event loop in Node.js manages asynchronous operations by executing callbacks when tasks are completed, ensuring non-blocking I/O operations.
3. How do you handle uncaught exceptions in a Node.js application?
Answer:
Use the process.on(‘uncaughtException’) event to catch and handle uncaught exceptions globally.
process.on(‘uncaughtException’, (err) => {
console.error(‘Uncaught Exception:’, err.message);
process.exit(1);
});
4. Write a Node.js script that reads the content of a file asynchronously and logs it to the console.
Answer:
Use the fs.readFile method to read the file content asynchronously and handle the result in a callback.
const fs = require(‘fs’);
fs.readFile(‘example.txt’, ‘utf8’, (err, data) => {
if (err) throw err;
console.log(data);
});
5. How do you implement a simple promise-based function in Node.js?
Answer:
Use the Promise constructor to create a function that returns a promise and resolves or rejects based on a condition.
function myPromiseFunction(value) {
return new Promise((resolve, reject) => {
if (value) resolve(‘Success’);
else reject(‘Failure’);
});
}
6. How do you create and export a custom module in Node.js?
Answer:
Define the module’s functionality in a separate file and export it using
module.exports for use in other files.
// myModule.js
module.exports = function greet(name) {
return `Hello, ${name}`;
};
// main.js
const greet = require(‘./myModule’);
console.log(greet(‘John’));
7. What is the difference between require and import in Node.js?
Answer:
require is used in CommonJS modules, while import is used in ES6 modules. import provides better support for static analysis and tree-shaking.
8. How do you install a specific version of an NPM package?
Answer:
Use npm install followed by the package name and version to install a specific version of an NPM package.
npm install [email protected]
9. Write a Node.js script that uses an NPM package to make an HTTP request.
Answer:
Install the axios package and use it to make a GET request to an external API.
const axios = require(‘axios’);
axios.get(‘https://api.example.com/data’)
.then(response => console.log(response.data))
.catch(error => console.error(error));
10. How do you handle dependencies in a Node.js project to avoid version conflicts?
Answer:
Use a package-lock.json file to lock dependency versions and npm ci to install exact versions from the lock file.
11. How do you handle multiple asynchronous operations in Node.js using async/await?
Answer:
Use async functions and await to handle asynchronous operations sequentially, improving code readability and error handling.
async function fetchData() {
try {
const response = await axios.get(‘https://api.example.com/data’);
console.log(response.data);
} catch (error) {
console.error(error);
}
}
12. Write a Node.js function that executes two promises in parallel and returns the combined result.
Answer:
Use Promise.all to execute multiple promises in parallel and return their results as an array.
async function fetchData() {
const [data1, data2] = await Promise.all([
axios.get(‘https://api.example.com/data1’),
axios.get(‘https://api.example.com/data2’)
]);
return { data1: data1.data, data2: data2.data };
}
13. How do you convert a callback-based function to a promise-based one in Node.js?
Answer:
Use util.promisify to convert callback-based functions to return promises, enabling async/await usage.
const fs = require(‘fs’);
const util = require(‘util’);
const readFileAsync = util.promisify(fs.readFile);
readFileAsync(‘example.txt’, ‘utf8’)
.then(data => console.log(data))
.catch(err => console.error(err));
14. How do you handle errors in an async/await function?
Answer:
Use a try…catch block to handle errors in async/await functions, ensuring that errors are properly caught and handled.
async function fetchData() {
try {
const response = await axios.get(‘https://api.example.com/data’);
console.log(response.data);
} catch (error) {
console.error(‘Error fetching data:’, error);
}
}
15. Write a Node.js function that uses setTimeout to delay an operation by 2 seconds using a promise.
Answer:
Wrap setTimeout in a promise to create a delay function that can be awaited in an async function.
function delay(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function delayedOperation() {
await delay(2000);
console.log(‘Operation after delay’);
}
16. How do you create a basic Express.js application with a single route?
Answer:
Use express() to create an app and define a route with app.get that responds to client requests.
const express = require(‘express’);
const app = express();
app.get(‘/’, (req, res) => {
res.send(‘Hello, Express!’);
});
app.listen(3000);
17. What is middleware in Express.js, and how do you use it?
Answer:
Middleware functions in Express.js process requests before they reach the route handlers, and they can modify the request, response, or end the request-response cycle.
app.use((req, res, next) => {
console.log(`${req.method} ${req.url}`);
next();
});
18. How do you handle form data submission in an Express.js application?
Answer:
Use the express.urlencoded middleware to parse URL-encoded form data from the request body.
app.use(express.urlencoded({ extended: true }));
app.post(‘/submit’, (req, res) => {
res.send(`Received: ${req.body.name}`);
});
19. Write an Express.js route that serves static files from a directory.
Answer:
Use the express.static middleware to serve static files like HTML, CSS, and JavaScript from a public directory.
app.use(express.static(‘public’));
20. How do you implement error handling in an Express.js application?
Answer:
Define an error-handling middleware function with four parameters (err, req, res, next) to handle errors globally.
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send(‘Something broke!’);
});
21. How do you create a RESTful API in Express.js with CRUD operations?
Answer:
Define routes using GET, POST, PUT, and DELETE methods to perform CRUD operations on resources like users.
app.get(‘/users’, (req, res) => { /* Fetch users */ });
app.post(‘/users’, (req, res) => { /* Create user */ });
app.put(‘/users/:id’, (req, res) => { /* Update user */ });
app.delete(‘/users/:id’, (req, res) => { /* Delete user */ });
22. How do you implement route parameters in an Express.js application?
Answer:
Use :parameter in the route path to define route parameters, which can be accessed via req.params.
app.get(‘/users/:id’, (req, res) => {
res.send(`User ID: ${req.params.id}`);
});
23. Write an Express.js route that validates the request body using a middleware function.
Answer:
Create a middleware function to validate the request body before passing control to the route handler.
function validateBody(req, res, next) {
if (!req.body.name) return res.status(400).send(‘Name is required’);
next();
}
app.post(‘/users’, validateBody, (req, res) => {
res.send(‘User created’);
});
24. How do you handle CORS in a Node.js application?
Answer:
Use the cors middleware to enable Cross-Origin Resource Sharing, allowing your API to be accessed from different domains.
const cors = require(‘cors’);
app.use(cors());
25. Write a Node.js script that connects to a REST API and logs the response.
Answer:
Use axios or node-fetch to send a GET request to a REST API and log the response.
const axios = require(‘axios’);
axios.get(‘https://jsonplaceholder.typicode.com/posts’)
.then(response => console.log(response.data))
.catch(error => console.error(error));
26. How do you connect a Node.js application to a MongoDB database using Mongoose?
Answer:
Use Mongoose to define a schema, connect to the MongoDB database, and interact with collections.
const mongoose = require(‘mongoose’);
mongoose.connect(‘mongodb://localhost/mydb’, { useNewUrlParser: true, useUnifiedTopology: true });
27. Write a Mongoose schema for a User model with fields for name, email, and password.
Answer:
Define a schema using Mongoose and create a model to interact with the User collection.
const userSchema = new mongoose.Schema({
name: String,
email: String,
password: String
});
const User = mongoose.model(‘User’, userSchema);
28. How do you perform CRUD operations on a MongoDB collection using Mongoose in Node.js?
Answer:
Use Mongoose model methods like find, create, update, and delete to perform CRUD operations.
User.find({}, (err, users) => { /* Fetch users */ });
User.create({ name: ‘John’ }, (err, user) => { /* Create user */ });
User.updateOne({ _id: id }, { name: ‘Jane’ }, (err) => { /* Update user */ });
User.deleteOne({ _id: id }, (err) => { /* Delete user */ });
29. How do you handle database errors in a Node.js application using Mongoose?
Answer:
Use Mongoose’s built-in error handling and validate data before saving it to handle errors gracefully.
user.save((err) => {
if (err) return console.error(‘Error saving user:’, err);
console.log(‘User saved successfully’);
});
30. Write a Node.js function that fetches data from a PostgreSQL database using pg module.
Answer:
Use the pg module to connect to a PostgreSQL database, execute a query, and return the results.
const { Client } = require(‘pg’);
const client = new Client();
client.connect();
client.query(‘SELECT * FROM users’, (err, res) => {
console.log(res.rows);
client.end();
});
31. How do you hash passwords in a Node.js application using bcrypt?
Answer:
Use bcrypt to hash user passwords before storing them in the database to enhance security.
const bcrypt = require(‘bcrypt’);
const saltRounds = 10;
bcrypt.hash(‘myPassword’, saltRounds, (err, hash) => {
// Store hash in your password database
});
32. Write a Node.js middleware function to authenticate API requests using JWT.
Answer:
Use jsonwebtoken to verify the JWT in the request header and authenticate the user.
const jwt = require(‘jsonwebtoken’);
function authenticateToken(req, res, next) {
const token = req.header(‘Authorization’).split(‘ ‘)[1];
if (!token) return res.sendStatus(401);
jwt.verify(token, ‘secretkey’, (err, user) => {
if (err) return res.sendStatus(403);
req.user = user;
next();
});
}
33. How do you prevent SQL injection attacks in a Node.js application?
Answer:
Use parameterized queries or ORM libraries like Sequelize to safely execute SQL queries, preventing SQL injection attacks.
client.query(‘SELECT * FROM users WHERE id = $1’, [userId], (err, res) => {
// Handle result
});
34. Write a Node.js function to sanitize user input before saving it to the database.
Answer:
Use libraries like validator to sanitize and validate user input, ensuring data integrity and security.
const validator = require(‘validator’);
const sanitizedInput = validator.escape(req.body.input);
35. How do you implement HTTPS in a Node.js application?
Answer:
Use the https module to create an HTTPS server with SSL/TLS certificates, ensuring secure communication.
const https = require(‘https’);
const fs = require(‘fs’);
const options = {
key: fs.readFileSync(‘server.key’),
cert: fs.readFileSync(‘server.cert’)
};
https.createServer(options, (req, res) => {
res.writeHead(200);
res.end(‘Secure connection’);
}).listen(443);
36. How do you write a unit test for a Node.js function using Mocha and Chai?
Answer:
Use Mocha as the testing framework and Chai for assertions to write and run unit tests for Node.js functions.
const { expect } = require(‘chai’);
describe(‘My Function’, () => {
it(‘should return true’, () => {
const result = myFunction();
expect(result).to.be.true;
});
});
37. Write a Node.js test case that mocks a database call using Sinon.
Answer:
Use Sinon to create a mock for the database method and test the function without hitting the actual database.
const sinon = require(‘sinon’);
const db = require(‘./db’);
sinon.stub(db, ‘find’).returns([{ id: 1, name: ‘Test’ }]);
38. How do you handle exceptions and errors in a Node.js application?
Answer:
Use try…catch blocks to handle synchronous errors and the process.on(‘uncaughtException’) event for global error handling.
try {
const data = JSON.parse(invalidJson);
} catch (error) {
console.error(‘JSON parsing error:’, error);
}
39. How do you debug a Node.js application?
Answer:
Use the built-in Node.js debugger or tools like node-inspect and Chrome DevTools to set breakpoints and inspect variables during execution.
node –inspect-brk app.js
40. Write a Node.js function with proper logging using a logging library like winston.
Answer:
Use winston to log messages at different levels (info, error) and output them to console or files.
const winston = require(‘winston’);
const logger = winston.createLogger({
transports: [new winston.transports.Console()]
});
logger.info(‘This is an info message’);
41. How do you deploy a Node.js application to a cloud platform like Heroku?
Answer:
Create a Procfile and push your code to Heroku using Git to deploy your Node.js application.
web: node app.js
42. How do you manage environment variables in a Node.js application?
Answer:
Use the dotenv package to load environment variables from a .env file into process.env for use in your application.
require(‘dotenv’).config();
const port = process.env.PORT || 3000;
43. How do you implement clustering in Node.js to improve performance?
Answer:
Use the cluster module to create multiple worker processes that share the same port, improving performance on multi-core systems.
const cluster = require(‘cluster’);
if (cluster.isMaster) {
for (let i = 0; i < 4; i++) cluster.fork();
} else {
require(‘./app’);
}
44. Write a Node.js script to measure the execution time of a function.
Answer:
Use console.time and console.timeEnd to measure and log the execution time of a function.
console.time(‘executionTime’);
myFunction();
console.timeEnd(‘executionTime’);
45. How do you optimize a Node.js application for high concurrency?
Answer:
Optimize for high concurrency by using non-blocking I/O operations, clustering, and efficiently managing memory and resources.
46. How do you implement WebSockets in a Node.js application for real-time communication?
Answer:
Use the ws library to create a WebSocket server that handles real-time bidirectional communication with clients.
const WebSocket = require(‘ws’);
const wss = new WebSocket.Server({ port: 8080 });
wss.on(‘connection’, ws => {
ws.on(‘message’, message => console.log(`Received: ${message}`));
ws.send(‘Hello, client!’);
});
47. Write a Node.js function that uses child_process to execute a shell command and capture the output.
Answer:
Use the exec function from the child_process module to run a shell command and handle the output asynchronously.
const { exec } = require(‘child_process’);
exec(‘ls’, (error, stdout, stderr) => {
if (error) console.error(`Error: ${error.message}`);
console.log(`Output: ${stdout}`);
});
48. How do you implement a rate limiter in an Express.js application to prevent abuse?
Answer:
Use the express-rate-limit middleware to limit the number of requests from a single IP address.
const rateLimit = require(‘express-rate-limit’);
const limiter = rateLimit({ windowMs: 15 * 60 * 1000, max: 100 });
app.use(limiter);
49. How do you create a RESTful API that supports pagination in Node.js?
Answer:
Use query parameters to handle pagination and return a subset of results based on limit and offset.
app.get(‘/users’, (req, res) => {
const limit = parseInt(req.query.limit, 10) || 10;
const offset = parseInt(req.query.offset, 10) || 0;
User.find().skip(offset).limit(limit).exec((err, users) => {
res.json(users);
});
});
50. How do you implement a custom event emitter in Node.js?
Answer:
Extend the EventEmitter class to create a custom event emitter and emit custom events.
const EventEmitter = require(‘events’);
class MyEmitter extends EventEmitter {}
const myEmitter = new MyEmitter();
myEmitter.on(‘event’, () => console.log(‘Event triggered’));
myEmitter.emit(‘event’);
Final Words
Getting ready for an interview can feel overwhelming, but going through these Node.js fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Node.js interview but don’t forget to practice the Node.js basic coding and API-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for Node JS?
The most common interview questions for Node.js often cover topics like asynchronous programming, event-driven architecture, Express.js, middleware, and RESTful API development.
2. What are the important Node JS topics freshers should focus on for interviews?
The important Node.js topics freshers should focus on include the event loop, callbacks, promises, modules, and handling asynchronous operations.
3. How should freshers prepare for Node JS technical interviews?
Freshers should prepare for Node.js technical interviews by building small projects, practicing common coding patterns, and understanding core concepts like middleware and API routing.
4. What strategies can freshers use to solve Node JS coding questions during interviews?
Strategies freshers can use include breaking down the problem, leveraging Node.js built-in modules, and writing clean, modular code.
5. Should freshers prepare for advanced Node JS topics in interviews?
Yes, freshers should prepare for advanced Node.js topics like microservices, real-time communication with WebSockets, and working with databases, depending on the job role.
Explore More Node JS Resources
Explore More Interview Questions
Related Posts
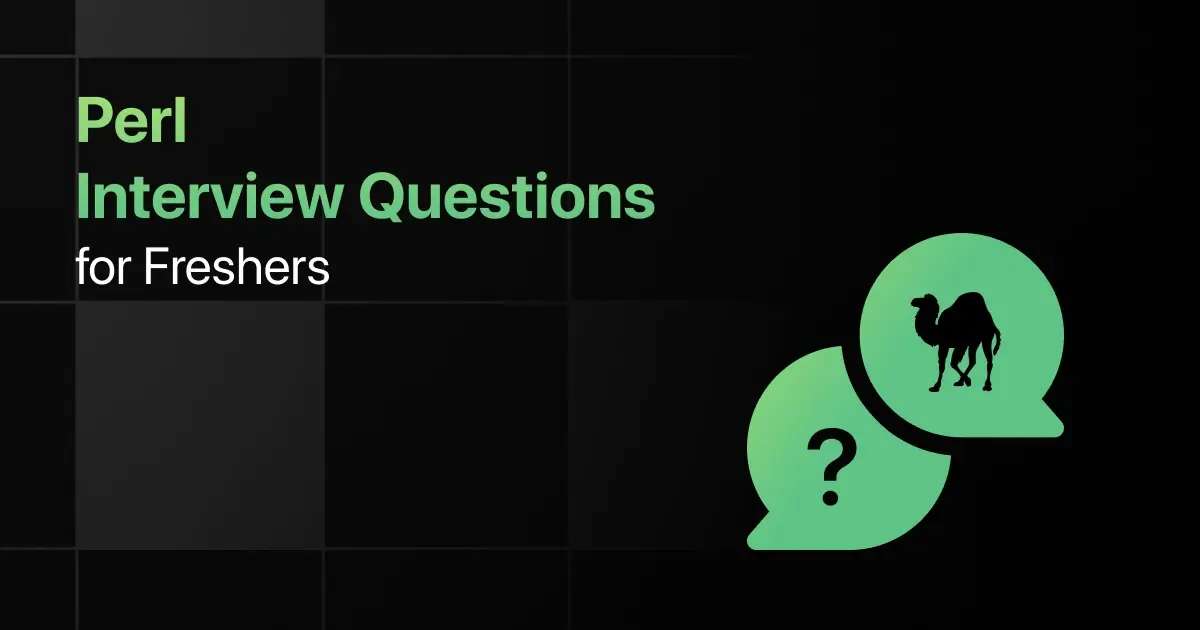
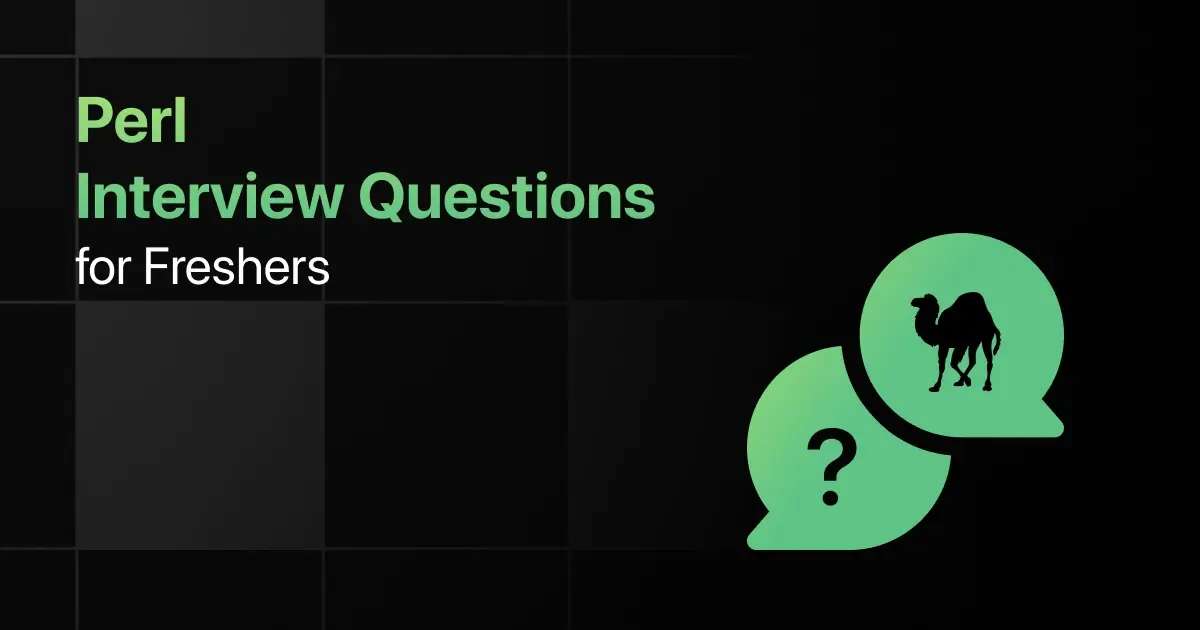
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …