Top MongoDB Interview Questions for Freshers
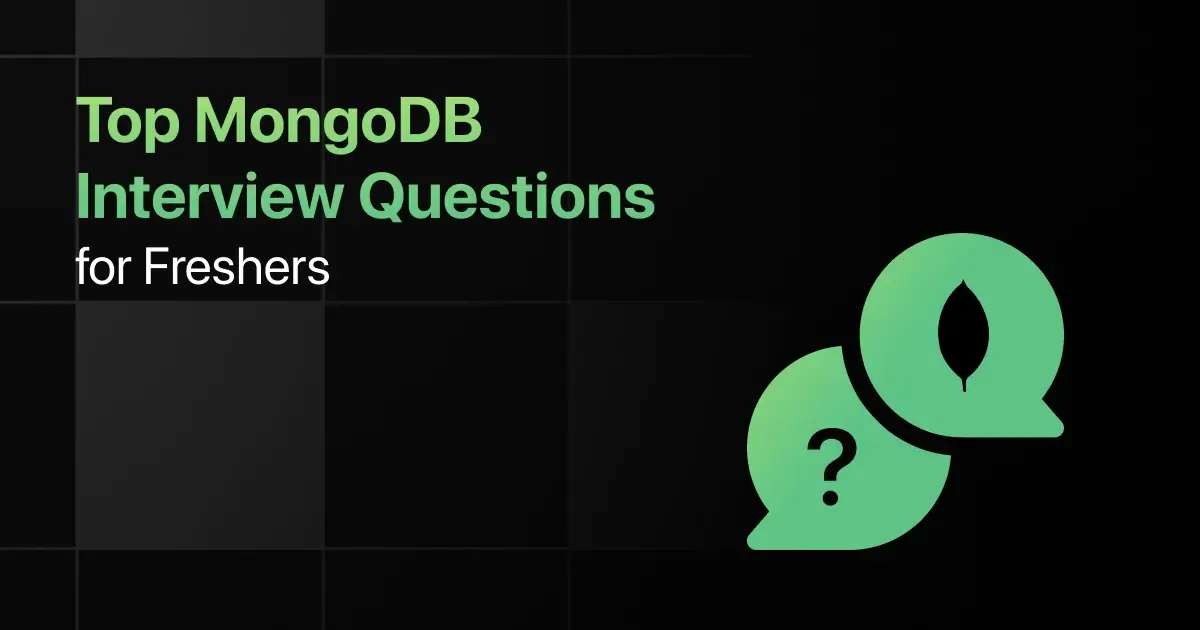
Are you preparing for your first MongoDB interview and wondering what questions you might face? Understanding the key MongoDB interview questions for freshers can give you more clarity.
This blog is here to help you get ready with practical questions that test your real-world problem-solving skills. We’ve gathered some of the most common basic MongoDB interview questions that freshers often encounter.
With this guide, you’ll be well-prepared to tackle these MongoDB interview questions and answers for freshers and make a strong impression in your interview.
Practice MongoDB Interview Questions and Answers
Here are the top 50 MongoDB interview questions with answers for freshers:
1. How do you create a new collection in MongoDB?
Answer:
Collections are created automatically in MongoDB when you insert the first document into a collection that does not exist. You can also explicitly create a collection using the db.createCollection() method.
db.createCollection(“users”)
2. How do you insert a single document into a MongoDB collection?
Answer:
Use the insertOne() method to add a single document to a collection.
db.users.insertOne({ name: “John Doe”, age: 30, email: “[email protected]” })
3. How do you insert multiple documents into a MongoDB collection?
Answer:
Use the insertMany() method to insert multiple documents at once.
db.users.insertMany([
{ name: “Jane Doe”, age: 25, email: “[email protected]” },
{ name: “Alice”, age: 28, email: “[email protected]” }
])
4. How do you find a document by a specific field in MongoDB?
Answer:
Use the findOne() method to retrieve a single document that matches a specified field.
db.users.findOne({ email: “[email protected]” })
5. How do you update a single field in a document in MongoDB?
Answer:
Use the updateOne() method with the $set operator to update a specific field in a document.
db.users.updateOne({ name: “John Doe” }, { $set: { age: 31 } })
6. How do you find all documents where a field value matches a certain condition (e.g., age greater than 25)?
Answer:
Use the find() method with a query condition, such as $gt for greater than.
db.users.find({ age: { $gt: 25 } })
7. How do you find documents with a field that contains a specific substring?
Answer:
Use the find() method with a regular expression to match documents containing a substring.
db.users.find({ name: /Doe/ })
8. How do you find documents with an array field containing a specific value?
Answer:
Use the find() method to match documents where an array field contains a specific value.
db.users.find({ hobbies: “reading” })
9. How do you find documents where an array field contains all specified values?
Answer:
Use the $all operator to match documents where an array contains all the specified values.
db.users.find({ hobbies: { $all: [“reading”, “sports”] } })
10. How do you sort the results of a query by a specific field in ascending or descending order?
Answer:
Use the sort() method to order query results by a specific field, using 1 for ascending and -1 for descending.
db.users.find().sort({ age: 1 })
11. How do you group documents by a field and calculate the count of documents in each group?
Answer:
Use the aggregate() method with the $group stage to group by a field and count the documents in each group.
db.users.aggregate([
{ $group: { _id: “$age”, count: { $sum: 1 } } }
])
12. How do you calculate the average value of a numeric field across all documents in a collection?
Answer:
Use the $group stage with the $avg operator to calculate the average value of a numeric field.
db.users.aggregate([
{ $group: { _id: null, averageAge: { $avg: “$age” } } }
])
13. How do you filter documents in an aggregation pipeline before grouping them?
Answer:
Use the $match stage before the $group stage in the aggregation pipeline to filter documents.
db.users.aggregate([
{ $match: { age: { $gt: 25 } } },
{ $group: { _id: “$age”, count: { $sum: 1 } } }
])
14. How do you use the $lookup operator to join documents from two collections?
Answer:
Use the $lookup stage in the aggregation pipeline to perform a left outer join between two collections.
db.orders.aggregate([
{
$lookup: {
from: “customers”,
localField: “customerId”,
foreignField: “_id”,
as: “customerD
15. How do you limit the number of documents returned in an aggregation pipeline?
Answer:
Use the $limit stage to restrict the number of documents returned by an aggregation pipeline.
db.users.aggregate([
{ $group: { _id: “$age”, count: { $sum: 1 } } },
{ $limit: 5 }
])
16. How do you create an index on a specific field in a MongoDB collection?
Answer:
Use the createIndex() method to create an index on a specific field to improve query performance.
db.users.createIndex({ email: 1 }
17. How do you create a compound index on multiple fields in a MongoDB collection?
Answer:
Use the createIndex() method with multiple fields specified in the index object.
db.users.createIndex({ lastName: 1, firstName: 1 })
18. How do you check the existing indexes on a collection?
Answer:
Use the getIndexes() method to list all indexes created on a collection.
db.users.getIndexes()
19. How do you drop an index from a collection in MongoDB?
Answer:
Use the dropIndex() method to remove a specific index from a collection.
db.users.dropIndex(“email_1”)
20. How do you ensure a query uses a specific index in MongoDB?
Answer:
Use the hint() method to force a query to use a specific index.
db.users.find({ email: “[email protected]” }).hint({ email: 1 }
21. How do you model a one-to-many relationship in MongoDB?
Answer:
Model a one-to-many relationship by embedding the related documents as an array or referencing them using ObjectIds.
// Embedding example
{
name: “John Doe”,
orders: [
{ orderId: 1, item: “Laptop” },
{ orderId: 2, item: “Mouse” }
]
}
// Referencing example
{
name: “John Doe”,
orders: [ObjectId(“order_id_1”), ObjectId(“order_id_2”)]
}
22. How do you model a many-to-many relationship in MongoDB?
Answer:
Model a many-to-many relationship by using arrays of ObjectIds in both related documents or creating a junction collection.
// Example with arrays of ObjectIds
{
name: “Course 101”,
students: [ObjectId(“student_id_1”), ObjectId(“student_id_2”)]
}
{
name: “John Doe”,
courses: [ObjectId(“course_id_1”), ObjectId(“course_id_2”)]
}
23. How do you design a schema in MongoDB to handle hierarchical data?
Answer:
Use an array of embedded documents or references to represent parent-child relationships, or use materialized paths.
// Example using embedded documents
{
_id: ObjectId(“category_id”),
name: “Electronics”,
children: [
{ _id: ObjectId(“subcategory_id_1”), name: “Computers” },
{ _id: ObjectId(“subcategory_id_2”), name: “Cameras” }
]
}
24. How do you model time-series data in MongoDB?
Answer:
Model time-series data by storing each time point as a document with a timestamp, or use a bucketing approach.
// Example document for a time-series
{
deviceId: “device_123”,
timestamp: ISODate(“2023-01-01T10:00:00Z”),
temperature: 22.5
}
25. How do you handle schema evolution in MongoDB?
Answer:
Handle schema evolution by using schema versioning within documents or using dynamic fields and arrays to accommodate changes.
{
_id: ObjectId(“user_id”),
schemaVersion: 2,
name: “John Doe”,
email: “[email protected]”,
preferences: { theme: “dark” }
}
26. How do you start a multi-document transaction in MongoDB?
Answer:
Use the startSession() method to create a session, then start a transaction using the session.
const session = db.getMongo().startSession();
session.startTransaction();
27. How do you commit a transaction in MongoDB?
Answer:
Use the commitTransaction() method on the session to commit all operations performed during the transaction.
session.commitTransaction();
session.endSession();
28. How do you roll back a transaction in MongoDB?
Answer:
Use the abortTransaction() method on the session to roll back all operations performed during the transaction.
session.abortTransaction();
session.endSession();
29. How do you ensure atomicity in MongoDB for a single document update?
Answer:
MongoDB operations on a single document are atomic by default, ensuring that partial updates are not visible.
db.users.updateOne({ _id: ObjectId(“user_id”) }, { $set: { age: 31 } }
30. How do you handle errors during a transaction in MongoDB?
Answer:
Use a try-catch block to handle errors and roll back the transaction if an error occurs.
try {
session.startTransaction();
db.users.updateOne({ _id: ObjectId(“user_id”) }, { $set: { age: 31 } }, { session });
session.commitTransaction();
} catch (error) {
session.abortTransaction();
throw error;
} final
31. How do you create a new user in MongoDB with specific roles?
Answer:
Use the createUser() method to create a user and assign roles such as readWrite, dbAdmin, etc.
db.createUser({
user: “new_user”,
pwd: “secure_password”,
roles: [{ role: “readWrite”, db: “test_db” }]
})
32. How do you enable authentication in MongoDB?
Answer:
Enable authentication by configuring the security.authorization option in the MongoDB configuration file and restarting the MongoDB server.
security:
authorization: “enabled”
33. How do you restrict access to a collection for a specific user in MongoDB?
Answer:
Create a role with specific privileges for the collection and assign that role to the user.
db.createRole({
role: “restrictedRole”,
privileges: [
{ resource: { db: “test_db”, collection: “users” }, actions: [“find”, “update”] }
],
roles: []
})
db.createUser({
user: “restricted_user”,
pwd: “password”,
roles: [“restrictedRole”]
})
34. How do you check the roles assigned to a user in MongoDB?
Answer:
Use the rolesInfo command to view the roles assigned to a specific user.
db.getUser(“restricted_user”)
35. How do you change a user’s password in MongoDB?
Answer:
Use the updateUser() method to change the user’s password.
db.updateUser(“restricted_user”, { pwd: “new_secure_password” })
36. How do you enable sharding for a database in MongoDB?
Answer:
Enable sharding on a database using the enableSharding() method.
sh.enableSharding(“test_db”)
37. How do you shard a collection in MongoDB?
Answer:
Use the shardCollection() method to shard a collection, specifying the shard key.
sh.shardCollection(“test_db.users”, { email: 1 })
38. How do you check the shard distribution of data in MongoDB?
Answer:
Use the sh.status() command to check the distribution of data across shards.
sh.status()
39. How do you add a new shard to an existing MongoDB cluster?
Answer:
Use the addShard() method to add a new shard to the cluster.
sh.addShard(“shard_name/shard_host:port”)
40. How do you remove a shard from a MongoDB cluster?
Answer:
Use the removeShard() method to remove a shard, and monitor the data migration.
sh.remove
41. How do you set up a replica set in MongoDB?
Answer:
Initialize a replica set using the rs.initiate() method and add secondary members using rs.add().
rs.initiate({
_id: “myReplicaSet”,
members: [
{ _id: 0, host: “localhost:27017” },
{ _id: 1, host: “localhost:27018” },
{ _id: 2, host: “localhost:27019” }
]
})
42. How do you promote a secondary member to primary in a replica set?
Answer:
Use the rs.stepDown() method on the current primary to force an election, promoting a secondary to primary.
rs.stepDown()
43. How do you check the status of a replica set in MongoDB?
Answer:
Use the rs.status() command to view the status and health of all members in the replica set.
rs.status()
44. How do you change the priority of a member in a replica set?
Answer:
Use the rs.reconfig() method to change the priority of a member, influencing which member is elected as primary.
cfg = rs.conf()
cfg.members[1].priority = 2
rs.reconfig(cfg)
45. How do you handle a network partition in a MongoDB replica set?
Answer:
Monitor the rs.status() output, ensure network connectivity is restored, and allow the replica set to resolve the partition automatically, or manually reconfigure the set if needed.
46. How do you create a backup of a MongoDB database using mongodump?
Answer:
Use the mongodump command to create a backup of a MongoDB database, specifying the output directory.
mongodump –db test_db –out /path/to/backup/
47. How do you restore a MongoDB database from a backup using mongorestore?
Answer:
Use the mongorestore command to restore a MongoDB database from a backup.
mongorestore –db test_db /path/to/backup/test_db/
48. How do you perform a point-in-time recovery in MongoDB?
Answer:
Use the combination of a backup created by mongodump and the oplog to restore the database to a specific point in time.
mongorestore –oplogReplay –dir /path/to/backup/
49. How do you schedule regular backups of a MongoDB database?
Answer:
Use a cron job on Linux or Task Scheduler on Windows to schedule regular mongodump commands.
0 2 * * * mongodump –db test_db –out /path/to/backup/
50. How do you restore specific collections from a backup in MongoDB?
Answer:
Use the –collection option with mongorestore to restore specific collections from a backup.
mongorestore –db test_db –collection users /path/to/backup/test_db/users.bson
Final Words
Getting ready for an interview can feel overwhelming, but going through these MongoDB fresher interview questions can help you feel more confident. This guide focuses on the kinds of MongoDB-related interview questions for fresher roles that you’re likely to face.
Don’t forget to practice the MongoDB basics, aggregation framework, and performance optimization-related interview questions too! With the right preparation, you’ll ace your MongoDB interview and take that important step in your career.
Frequently Asked Questions
1. What are the most common interview questions for MongoDB?
The most common interview questions for MongoDB often cover topics like CRUD operations, indexing, aggregation framework, and data modeling.
2. What are the important MongoDB topics freshers should focus on for interviews?
The important MongoDB topics freshers should focus on include understanding collections, documents, queries, indexing, and the aggregation pipeline.
3. How should freshers prepare for MongoDB technical interviews?
Freshers should prepare for MongoDB technical interviews by practicing query writing, understanding data modeling principles, and working with aggregation pipelines.
4. What strategies can freshers use to solve MongoDB coding questions during interviews?
Strategies freshers can use include understanding the problem requirements, efficiently using indexes, and leveraging MongoDB’s built-in operators.
5. Should freshers prepare for advanced MongoDB topics in interviews?
Yes, freshers should prepare for advanced MongoDB topics like sharding, replication, and performance optimization if the role demands a deep understanding of MongoDB.
Explore More MongoDB Resources
Explore More Interview Questions
Related Posts
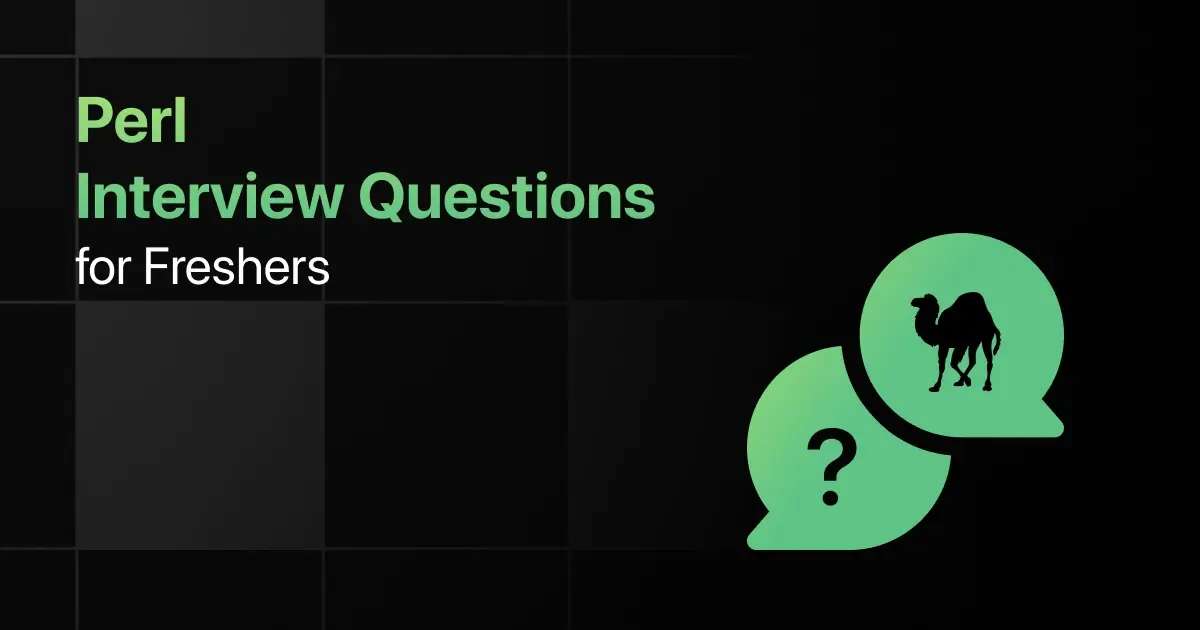
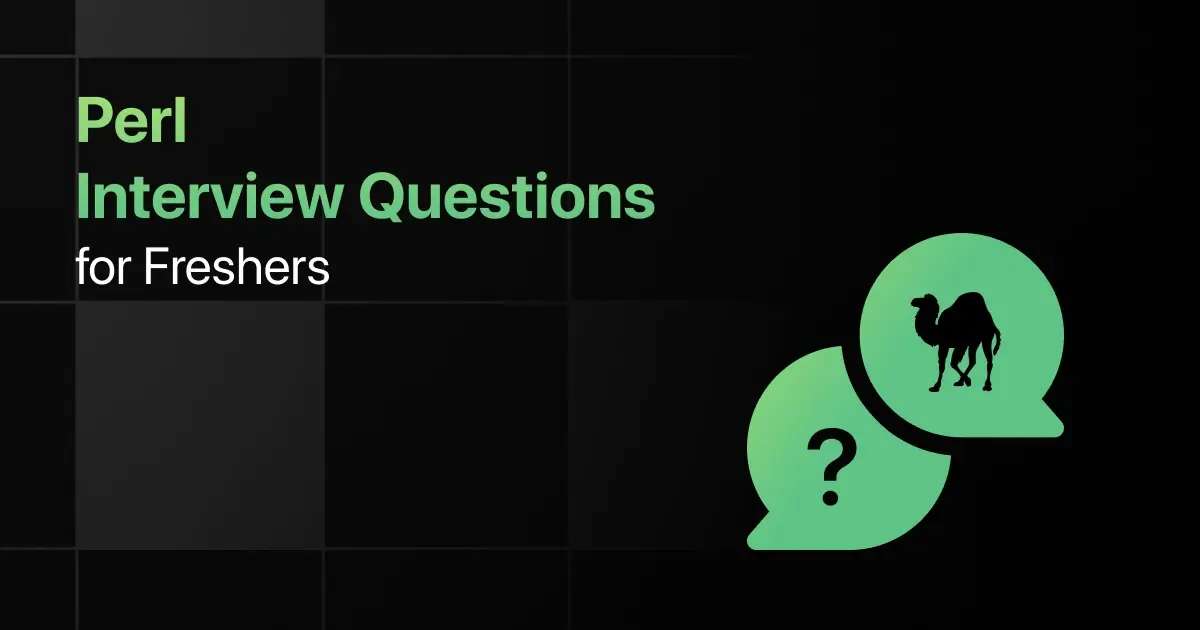
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …