Top Kotlin Interview Questions for Freshers
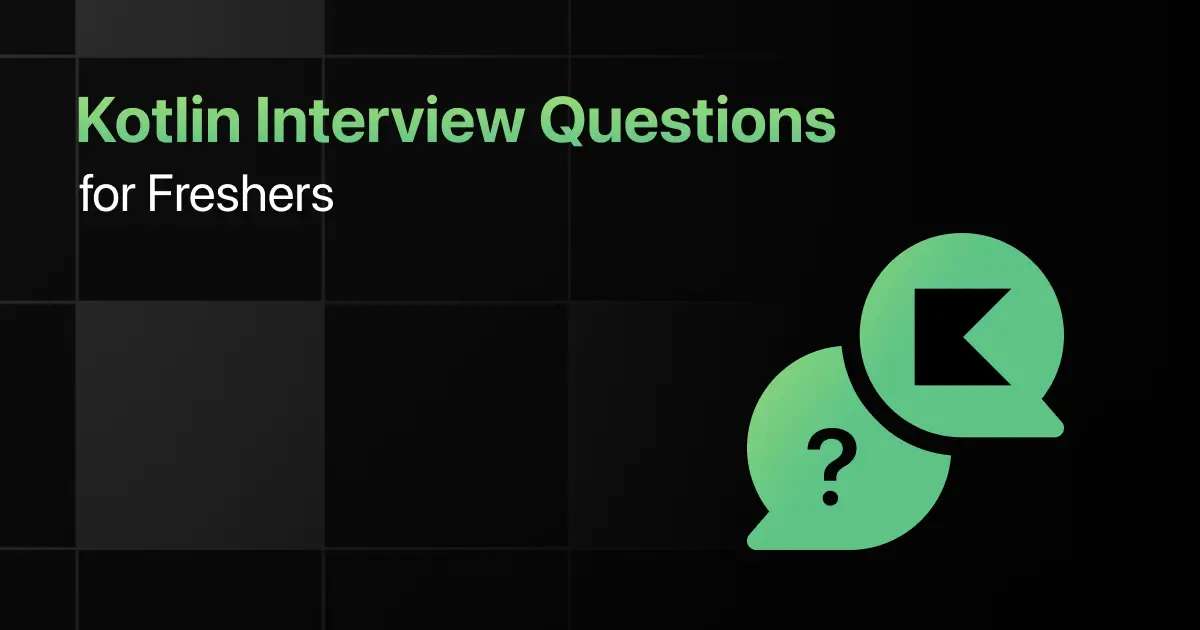
Are you preparing for your first Kotlin interview and wondering what questions you might face?
Understanding the key Kotlin interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Kotlin interview questions and answers for freshers and make a strong impression in your interview.
Practice Kotlin Interview Questions and Answers
Below are the top 50 Kotlin interview questions for freshers with answers:
1. What is Kotlin?
Answer:
Kotlin is a statically typed programming language developed by JetBrains. It is fully interoperable with Java and is used to build modern Android apps. Kotlin provides concise syntax and aims to increase productivity with features like null safety and lambda expressions.
2. What are the main features of Kotlin?
Answer:
Kotlin features include null safety, type inference, extension functions, smart casts, and coroutines for asynchronous programming. It supports both object-oriented and functional programming paradigms. Kotlin is also fully interoperable with Java, making it easy to use alongside existing Java codebases.
3. How do you declare a variable in Kotlin?
Answer:
In Kotlin, variables can be declared using val for immutable variables and var for mutable variables. Immutable variables cannot be reassigned, while mutable variables can be changed later.
var age: Int = 25 // Mutable
4. What is the difference between val and var in Kotlin?
Answer:
val declares a read-only variable, meaning it cannot be reassigned after its initial value is set. var is used for mutable variables, which can be reassigned multiple times. Using val promotes immutability and helps avoid potential side effects.
5. What is a null safety feature in Kotlin?
Answer:
Null safety in Kotlin prevents the occurrence of null pointer exceptions. By default, a variable cannot be assigned null, and you must explicitly declare a nullable type using a ?. This feature helps ensure that the code is less prone to runtime crashes.
6. How do you handle null values in Kotlin?
Answer:
You can handle null values in Kotlin using safe calls (?.), the Elvis operator (?:), and !! for asserting non-null values. Safe calls are used to execute an operation only if the variable is not null, and the Elvis operator provides a default value if the variable is null.
7. What is a data class in Kotlin?
Answer:
A data class in Kotlin is a class used primarily to store data. It automatically generates methods like equals(), hashCode(), toString(), and copy() based on the properties. Data classes are useful for modeling immutable data.
8. How do you define a function in Kotlin?
Answer:
Functions in Kotlin are defined using the fun keyword followed by the function name, parameter list, and return type. Functions can have default parameter values and return types can be inferred.
return a + b
}
9. What are extension functions in Kotlin?
Answer:
Extension functions allow you to add functionality to existing classes without modifying their code. They are defined using the class name as a receiver followed by the function.
return this + “!”
}
10. What is a companion object in Kotlin?
Answer:
A companion object is an object associated with a class, allowing you to define static methods and properties. It acts like a singleton within the class and can be accessed without creating an instance of the class.
companion object {
fun printMessage() {
println(“Hello”)
}
}
}
11. What is the use of lateinit in Kotlin?
Answer:
lateinit is used to declare a variable that will be initialized later. It is primarily used for variables that cannot be initialized when the object is created. lateinit can only be used with mutable (var) non-nullable variables.
12. What is a sealed class in Kotlin?
Answer:
A sealed class in Kotlin is used to represent a restricted hierarchy of classes. Subclasses of a sealed class must be declared within the same file. This allows exhaustive when expressions, as all possible subclasses are known at compile time.
class Circle(val radius: Double) : Shape()
class Square(val side: Double) : Shape()
13. What is a coroutine in Kotlin?
Answer:
Coroutines are lightweight threads used for asynchronous programming in Kotlin. They allow code to be suspended and resumed, making it easier to write non-blocking code. Coroutines are more efficient than traditional threads because they require less memory and CPU resources.
delay(1000L)
println(“Hello from coroutine!”)
}
14. What is the difference between == and === in Kotlin?
Answer:
In Kotlin, == checks for structural equality, meaning it compares the values of two objects. === checks for referential equality, meaning it checks whether two references point to the same object in memory.
15. How do you create a singleton class in Kotlin?
Answer:
In Kotlin, you can create a singleton class using the object keyword. This ensures that only one instance of the class is created and shared.
val name = “Singleton”
}
16. What is the Elvis operator in Kotlin?
Answer:
The Elvis operator (?:) is used to return a default value if the expression on the left is null. It provides a concise way to handle nullable values without needing to write lengthy null checks.
17. How does Kotlin handle checked exceptions?
Answer:
Kotlin does not have checked exceptions. Unlike Java, Kotlin treats all exceptions as unchecked, meaning you are not forced to declare or catch exceptions. This simplifies error handling and reduces boilerplate code.
18. What is smart casting in Kotlin?
Answer:
Smart casting in Kotlin automatically casts a variable to a specific type after a successful type check. It eliminates the need for manual casting and simplifies the code.
println(obj.length) // Smart cast to String
}
19. What are higher-order functions in Kotlin?
Answer:
Higher-order functions are functions that take other functions as parameters or return them as results. They allow more functional programming patterns, such as lambdas and function composition.
return op(x, y)
}
20. How do you create a lambda function in Kotlin?
Answer:
A lambda function in Kotlin is an anonymous function defined using curly braces. Lambda functions can be passed as arguments or stored in variables. They are useful for inline functions and functional programming.
println(sum(2, 3))
21. What is the default visibility modifier in Kotlin?
Answer:
The default visibility modifier in Kotlin is public, which means the class or function is visible to all other code. If no modifier is specified, it is assumed to be public.
22. How do you define a class in Kotlin?
Answer:
A class in Kotlin is defined using the class keyword followed by the class name. Kotlin classes can have properties, methods, constructors, and more.
23. What is an inline function in Kotlin?
Answer:
An inline function in Kotlin requests the compiler to insert the function’s code directly into the calling site, avoiding function call overhead. This is commonly used with higher-order functions to improve performance.
println(“Hello, $name”)
}
24. What is a companion object used for in Kotlin?
Answer:
A companion object is used to define members that are shared among all instances of a class, similar to static members in Java. It allows you to group functions and properties within the class scope, without creating an instance.
companion object {
const val CONSTANT = 10
}
}
25. How do you implement inheritance in Kotlin?
Answer:
Inheritance in Kotlin is implemented using the : symbol followed by the parent class. The parent class must be marked as open to allow inheritance.
class Dog(name: String) : Animal(name)
26. What is the by keyword in Kotlin used for?
Answer:
The by keyword is used in Kotlin for delegation. It allows a class to delegate the implementation of a property or method to another object. This simplifies code by avoiding boilerplate.
fun printMessage()
}
class BaseImpl(val x: Int) : Base {
override fun printMessage() {
println(x)
}
}
class Derived(b: Base) : Base by b
27. What is the difference between open and final in Kotlin?
Answer:
In Kotlin, classes and methods are final by default, meaning they cannot be overridden or inherited. The open keyword is used to allow inheritance or method overriding. A final class or method cannot be extended or overridden.
28. How does Kotlin handle static members?
Answer:
Kotlin does not have static members. Instead, you can use companion objects to define functions and properties that belong to the class rather than an instance. Companion objects serve a similar purpose to static members in Java.
29. How do you handle exceptions in Kotlin?
Answer:
Exceptions in Kotlin are handled using try, catch, and finally blocks, similar to Java. Kotlin has no checked exceptions, so the compiler does not force you to catch exceptions, making error handling more flexible.
val result = 10 / 0
} catch (e: ArithmeticException) {
println(“Cannot divide by zero!”)
} finally {
println(“Cleanup if necessary”)
}
30. How do you create an interface in Kotlin?
Answer:
An interface in Kotlin is created using the interface keyword. It can contain both abstract methods and method implementations. A class can implement one or more interfaces.
fun drive()
}
class Car : Drivable {
override fun drive() {
println(“Driving a car”)
}
}
31. What are type aliases in Kotlin?
Answer:
Type aliases in Kotlin allow you to create an alternative name for an existing type. This can improve code readability and shorten long type names, especially when dealing with generic types.
32. How do you declare a constant in Kotlin?
Answer:
Constants in Kotlin are declared using the const keyword. They are immutable and must be initialized at compile time. Constants are often declared in companion objects.
33. What are destructuring declarations in Kotlin?
Answer:
Destructuring declarations allow you to unpack a data class or a collection into multiple variables. This improves readability when working with data objects or map entries
34. What is the use of init block in Kotlin?
Answer:
The init block is used to initialize code in a Kotlin class. It is executed immediately after the primary constructor. You can have multiple init blocks in a class.
init {
println(“Person initialized with name: $name”)
}
}
35. How do you define a secondary constructor in Kotlin?
Answer:
A secondary constructor in Kotlin is defined using the constructor keyword. It allows you to create additional constructors with different parameter sets.
constructor(name: String, age: Int) {
println(“Name: $name, Age: $age”)
}
}
36. What is infix notation in Kotlin?
Answer:
Infix notation in Kotlin allows functions with a single parameter to be called using an infix operator, making the syntax cleaner. The function must be marked with the infix keyword.
println(2 times “Hello”)
37. What is the difference between mapOf and mutableMapOf in Kotlin?
Answer:
mapOf creates a read-only (immutable) map, whereas mutableMapOf creates a mutable map that allows adding, removing, and updating elements after creation.
val mutableMap = mutableMapOf(“key1” to “value1”)
38. How do you make a property read-only in Kotlin?
Answer:
A property in Kotlin is made read-only by declaring it with the val keyword. Once initialized, its value cannot be changed.
39. What is the difference between arrayOf and intArrayOf in Kotlin?
Answer:
arrayOf creates an array of generic type elements, while intArrayOf creates a primitive array of integers. Primitive arrays are more memory-efficient as they store values directly.
val intArray = intArrayOf(1, 2, 3)
40. How do you create an empty list in Kotlin?
Answer:
You can create an empty list in Kotlin using the emptyList() function for immutable lists or mutableListOf() for mutable lists.
val emptyMutableList = mutableListOf<String>()
41. What is lazy initialization in Kotlin?
Answer:
Lazy initialization is used to delay the initialization of a variable until it is accessed for the first time. This is achieved using the lazy function, which returns the value only when requested.
println(“Lazy initialization”)
“Hello”
}
42. How do you use when in Kotlin?
Answer:
when in Kotlin is similar to a switch statement in Java, but more powerful. It can be used as an expression or a statement and supports various data types and conditions.
1 -> println(“x is 1”)
2 -> println(“x is 2”)
else -> println(“x is neither 1 nor 2”)
}
43. How do you iterate over a collection in Kotlin?
Answer:
You can iterate over a collection in Kotlin using a for loop or the forEach function. Both approaches are concise and provide access to each element in the collection.
for (item in list) {
println(item)
}
list.forEach { println(it) }
44. How do you convert a Kotlin list to a mutable list?
Answer:
A Kotlin list can be converted to a mutable list using the toMutableList() function. This allows you to add or modify elements in the list.
val mutableList = list.toMutableList()
45. How do you concatenate strings in Kotlin?
Answer:
Strings in Kotlin can be concatenated using the + operator or string templates. String templates allow embedding variables or expressions directly within the string.
val greeting = “Hello, $name”
46. What is an enum class in Kotlin?
Answer:
An enum class in Kotlin represents a fixed set of constants. Each constant is an object, and additional properties or methods can be added to the enum class.
NORTH, SOUTH, EAST, WEST
}
47. How do you create a range in Kotlin?
Answer:
A range in Kotlin is created using the .. operator. Ranges are useful for iterating over numbers or checking if a value is within a specified range.
48. How do you define a function with a default parameter in Kotlin?
Answer:
In Kotlin, functions can have default parameter values, which are used if no argument is provided. This eliminates the need for multiple overloaded methods.
println(“Hello, $name”)
}
49. How do you create a thread in Kotlin?
Answer:
A thread can be created in Kotlin using the Thread class and overriding its run method or by using the Runnable interface. This allows for concurrent execution of code. Here’s an example:
println(“Thread is running”)
}
thread.start()
50. What is a blocking function in Kotlin?
Answer:
A blocking function in Kotlin is a function that halts the execution of the thread until the operation is complete, which can lead to performance issues in asynchronous programming. Coroutines or non-blocking techniques should be used to avoid blocking.
Final Words
Getting ready for an interview can feel overwhelming, but going through these Kotlin fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Kotlin interview but don’t forget to practice Kotlin basics, coroutines, object-oriented principles, and Android-specific Kotlin development questions too.
Frequently Asked Questions
1. What are the most common interview questions for Kotlin?
Common Kotlin interview questions include topics like Kotlin’s interoperability with Java, data classes, coroutines for asynchronous programming, extension functions, null safety, and the difference between var and val.
2. What are the important Kotlin topics freshers should focus on for interviews?
Freshers should focus on Kotlin fundamentals like null safety, data classes, object-oriented features (inheritance, interfaces), collections, higher-order functions, coroutines, and Android development-related topics if relevant.
3. How should freshers prepare for Kotlin technical interviews?
Freshers should study Kotlin’s core syntax and features (e.g., lambdas, null safety, coroutines), understand differences with Java, practice writing code for common algorithms, and review key Android topics if the role involves mobile development.
4. What strategies can freshers use to solve Kotlin coding questions during interviews?
Freshers should focus on writing clean, efficient, and concise Kotlin code using idiomatic constructs (e.g., when expressions, scope functions), breaking problems into smaller tasks, and ensuring that null safety is properly handled.
5. Should freshers prepare for advanced Kotlin topics in interviews?
Yes, freshers should prepare for advanced topics like Kotlin coroutines for concurrency, using Kotlin in a functional programming style, understanding Kotlin Multiplatform (KMP), and optimization techniques for performance.
Explore More Kotlin Resources
Explore More Interview Questions
- Python
- Java
- SQL
- React
- JavaScript
- C Programming
- HTML
- CSS
- Angular
- C++
- Spring Boot
- Node JS
- Excel
- C#
- DBMS
- PHP
- Linux
- Operating System
- MySQL
- Spring
- Flutter
- MongoDB
- Django
- React Native
- jQuery
- Bootstrap
- Embedded C
- DSA
- R Programming
- Hadoop
- .NET
- Power BI
- ASP.NET
- ASP.NET MVC
- Android
- Tableau
- MVC
- WordPress
- TypeScript
- Spark
Related Posts
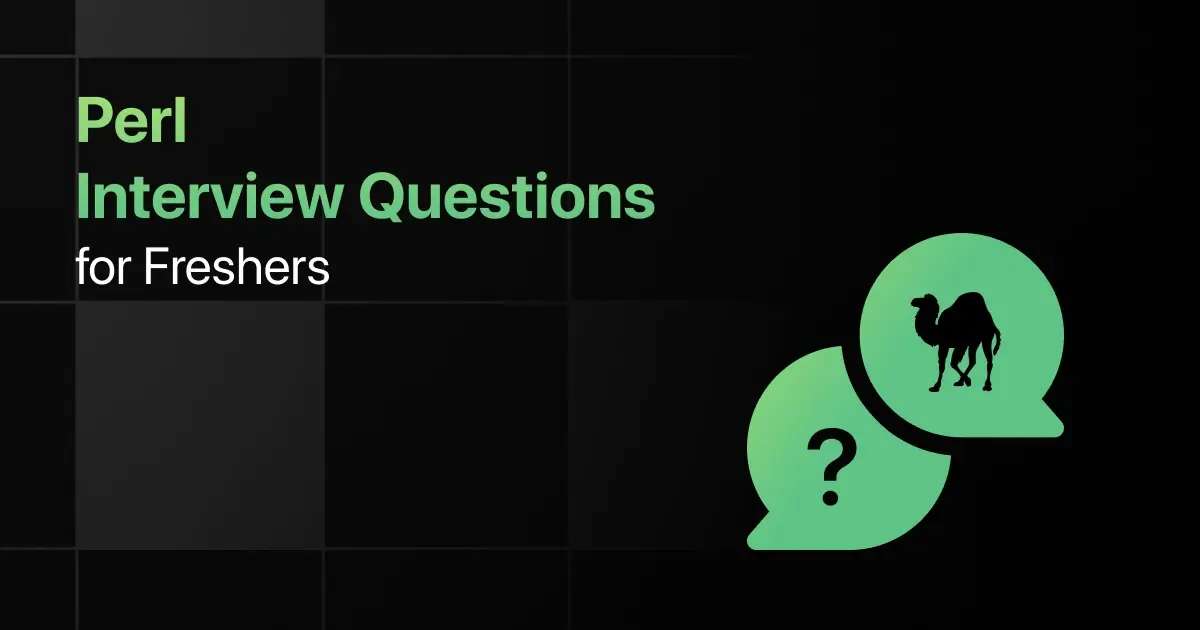
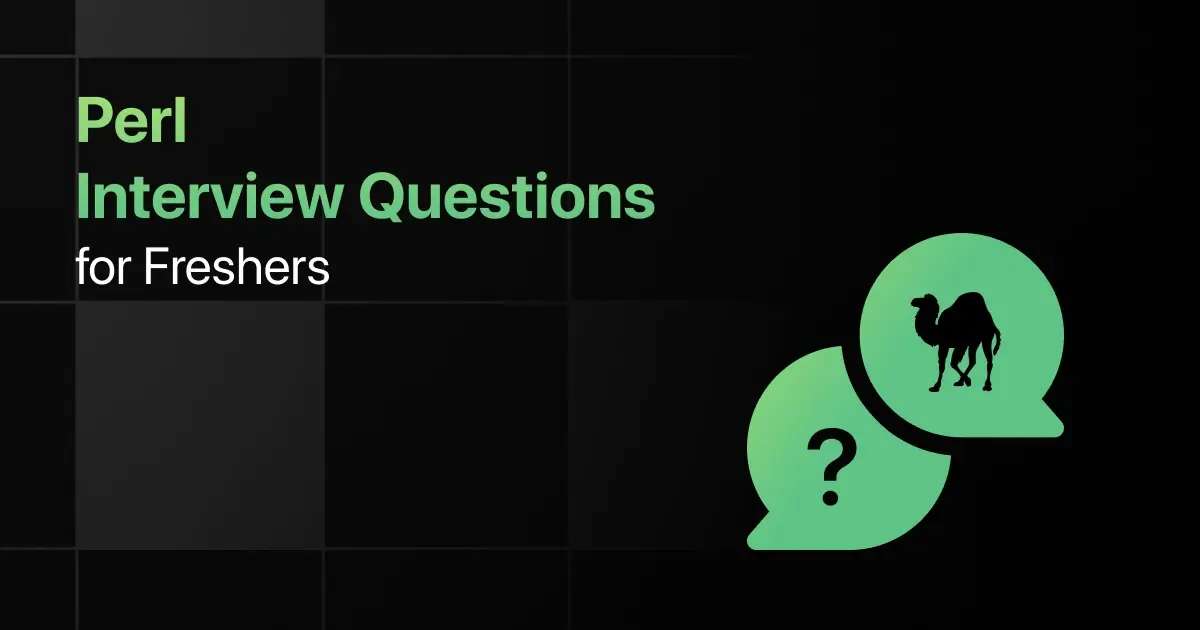
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …