Top jQuery Interview Questions for Freshers
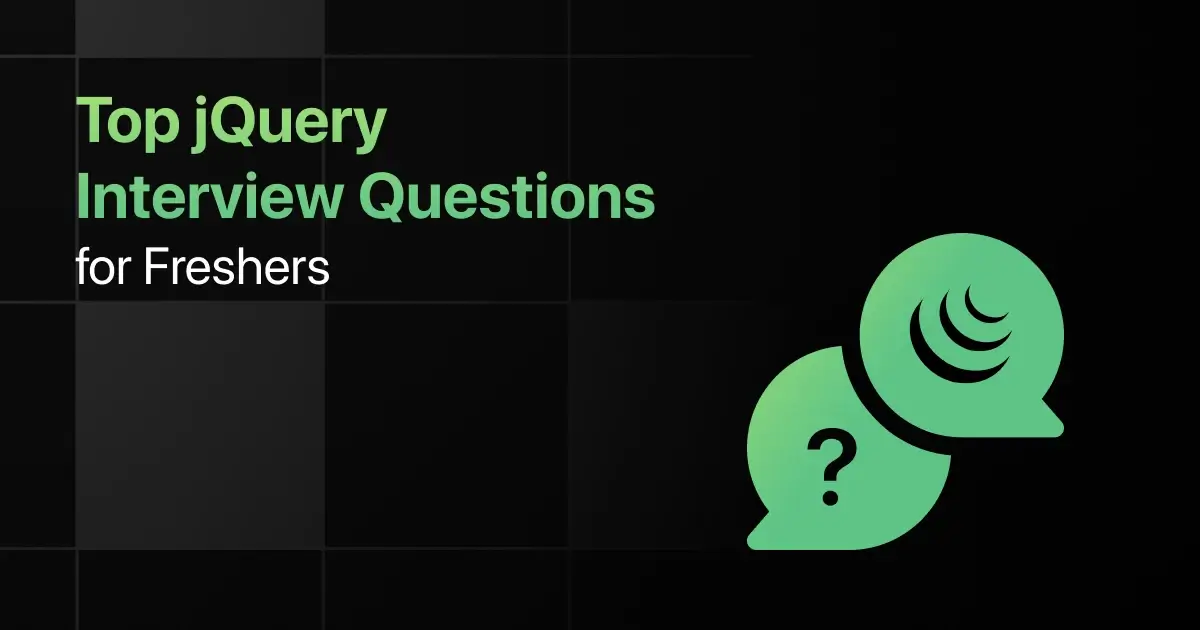
Are you preparing for your first jQuery interview and wondering what questions you might face?
Understanding the key jQuery interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these jQuery interview questions and answers for freshers and make a strong impression in your interview.
Practice jQuery Interview Questions and Answers
Below are the top 50 jQuery interview questions for freshers with answers:
1. How do you include jQuery in a web project?
Answer:
You can include jQuery by downloading the jQuery library and linking it in your HTML file, or by including it via a CDN.
<!– Using CDN –>
<script src=”https://code.jquery.com/jquery-3.6.0.min.js”></script>
2. How do you check if jQuery is loaded on a page?
Answer:
You can check if jQuery is loaded by typing $.fn.jquery in the browser console, which should return the version of jQuery.
if (typeof jQuery !== ‘undefined’) {
console.log(‘jQuery is loaded’);
} else {
console.log(‘jQuery is not loaded’);
}
3. How do you run a function when the DOM is ready in jQuery?
Answer:
Use the $(document).ready() function to execute code once the DOM is fully loaded.
$(document).ready(function() {
console.log(‘DOM is ready’);
});
4. How do you select an element by its ID in jQuery?
Answer:
Use the $(“#id”) selector to select an element by its ID.
$(‘#myElement’).css(‘color’, ‘blue’);
5. How do you select elements by their class name in jQuery?
Answer:
Use the $(“.className”) selector to select elements by their class name.
$(‘.myClass’).css(‘background-color’, ‘yellow’);
6. How do you select all paragraphs within a div with a specific class in jQuery?
Answer:
Use the $(“div.className p”) selector to target all paragraphs within a specific div.
$(‘div.myClass p’).css(‘font-weight’, ‘bold’)
7. How do you select the first element of a specific type using jQuery?
Answer:
Use the :first pseudo-class to select the first element of a specific type.
$(‘p:first’).css(‘color’, ‘red’);
8. How do you find elements by their attribute value in jQuery?
Answer:
Use the [attribute=”value”] selector to find elements with a specific attribute value.
$(‘input[type=”text”]’).css(‘border’, ‘1px solid green’);
9. How do you attach a click event handler to a button in jQuery?
Answer:
Use the .click() method to attach a click event handler to a button.
$(‘#myButton’).click(function() {
alert(‘Button clicked’);
});
10. How do you handle events on dynamically added elements in jQuery?
Answer:
Use .on() to delegate event handling to dynamically added elements.
$(document).on(‘click’, ‘.dynamicButton’, function() {
alert(‘Dynamically added button clicked’);
});
11. How do you prevent the default action of an event in jQuery?
Answer:
Use event.preventDefault() within an event handler to prevent the default action.
$(‘a’).click(function(event) {
event.preventDefault();
alert(‘Link clicked, but default action prevented’);
});
12. How do you stop the event from propagating to parent elements in jQuery?
Answer:
Use event.stopPropagation() to prevent the event from bubbling up to parent elements.
$(‘#myElement’).click(function(event) {
event.stopPropagation();
alert(‘Event propagation stopped’);
});
13. How do you trigger an event programmatically in jQuery?
Answer:
Use the .trigger() or .click() methods to trigger an event programmatically.
$(‘#myButton’).trigger(‘click’);
14. How do you change the text content of an element using jQuery?
Answer:
Use the .text() method to set or get the text content of an element.
$(‘#myElement’).text(‘New text content’);
15. How do you add a new element after an existing element in jQuery?
Answer:
Use the .after() method to insert a new element after an existing one.
$(‘#myElement’).after(‘<p>New paragraph added after the element</p>’);
16. How do you remove an element from the DOM using jQuery?
Answer:
Use the .remove() method to completely remove an element from the DOM.
$(‘#myElement’).remove();
17. How do you clone an element in jQuery?
Answer:
Use the .clone() method to create a copy of an element.
var clone = $(‘#myElement’).clone();
$(‘body’).append(clone);
18. How do you get or set the value of an input field in jQuery?
Answer:
Use the .val() method to get or set the value of an input field.
$(‘#myInput’).val(‘New Value’);
19. How do you add or remove a class from an element in jQuery?
Answer:
Use the .addClass() and .removeClass() methods to add or remove classes from elements.
$(‘#myElement’).addClass(‘highlight’);
$(‘#myElement’).removeClass(‘highlight’);
20. How do you toggle a class on an element in jQuery?
Answer:
Use the .toggleClass() method to add or remove a class based on its presence.
$(‘#myElement’).toggleClass(‘active’);
21. How do you change the CSS properties of an element using jQuery?
Answer:
Use the .css() method to set or get the value of CSS properties.
$(‘#myElement’).css(‘color’, ‘blue’);
22. How do you animate the width of an element in jQuery?
Answer:
Use the .animate() method to animate the CSS properties of an element.
$(‘#myElement’).animate({ width: ‘200px’ }, 1000);
23. How do you hide or show an element with a sliding effect in jQuery?
Answer:
Use the .slideUp() and .slideDown() methods to hide or show elements with a sliding effect.
$(‘#myElement’).slideUp();
$(‘#myElement’).slideDown();
24. How do you find the parent element of a specific element in jQuery?
Answer:
Use the .parent() method to select the immediate parent of an element.
$(‘#myElement’).parent().css(‘border’, ‘1px solid red’);
25. How do you find all child elements of a specific element in jQuery?
Answer:
Use the .children() method to select all direct child elements.
$(‘#myElement’).children().css(‘color’, ‘green’);
26. How do you find the next sibling of an element in jQuery?
Answer:
Use the .next() method to select the next sibling of an element.
$(‘#myElement’).next().css(‘background-color’, ‘yellow’);
27. How do you find all sibling elements of a specific element in jQuery?
Answer:
Use the .siblings() method to select all sibling elements.
$(‘#myElement’).siblings().css(‘opacity’, ‘0.5’);
28. How do you filter elements from a selection in jQuery?
Answer:
Use the .filter() method to filter elements based on a condition.
$(‘p’).filter(‘.highlight’).css(‘color’, ‘red’);
29. How do you create a fading effect in jQuery?
Answer:
Use the .fadeIn() and .fadeOut() methods to create a fading effect on elements.
$(‘#myElement’).fadeOut();
$(‘#myElement’).fadeIn();
30. How do you chain multiple animations together in jQuery?
Answer:
Chain multiple jQuery methods together by calling them in sequence.
$(‘#myElement’).slideUp().slideDown().fadeOut();
31. How do you stop an animation in jQuery before it completes?
Answer:
Use the .stop() method to halt an ongoing animation.
$(‘#myElement’).stop();
32. How do you delay an animation in jQuery?
Answer:
Use the .delay() method to introduce a delay before an animation starts.
$(‘#myElement’).slideUp().delay(500).slideDown();
33. How do you check if an element is currently animated in jQuery?
Answer:
Use the :animated selector to determine if an element is currently being animated.
if ($(‘#myElement’).is(‘:animated’)) {
console.log(‘Element is currently animated’);
}
34. How do you make a GET request using jQuery?
Answer:
Use the $.get() method to perform an AJAX GET request.
$.get(‘https://api.example.com/data’, function(response) {
console.log(response);
});
35. How do you make a POST request using jQuery?
Answer:
Use the $.post() method to perform an AJAX POST request.
$.post(‘https://api.example.com/data’, { key: ‘value’ }, function(response) {
console.log(response);
});
36. How do you load content from a server into a specific element using jQuery?
Answer:
Use the .load() method to load content from a server into a specific element.
$(‘#myElement’).load(‘https://example.com/page.html’);
37. How do you handle AJAX errors in jQuery?
Answer:
Use the .fail() method to handle errors in AJAX requests.
$.get(‘https://api.example.com/data’).fail(function() {
alert(‘Error occurred’);
});
38. How do you serialize form data for an AJAX request in jQuery?
Answer:
Use the .serialize() method to convert form data into a query string format.
var formData = $(‘#myForm’).serialize();
$.post(‘https://api.example.com/data’, formData);
39. How do you create a simple jQuery plugin?
Answer:
Create a jQuery plugin by extending the $.fn object.
$.fn.highlight = function() {
this.css(‘background-color’, ‘yellow’);
return this;
};
$(‘#myElement’).highlight();
40. How do you pass options to a jQuery plugin?
Answer:
Accept an options object as a parameter in the plugin function.
$.fn.customPlugin = function(options) {
var settings = $.extend({
color: ‘blue’,
fontSize: ’14px’
}, options);
return this.css({
color: settings.color,
fontSize: settings.fontSize
});
};
$(‘#myElement’).customPlugin({ color: ‘red’ });
41. How do you chain plugin methods in jQuery?
Answer:
Ensure your plugin returns this to allow method chaining.
$.fn.changeColor = function(color) {
this.css(‘color’, color);
return this;
};
$(‘#myElement’).changeColor(‘red’).hide();
42. How do you check if a jQuery plugin is applied to an element?
Answer:
You can check by using custom data attributes or flags within the plugin.
$.fn.addPlugin = function() {
return this.each(function() {
$(this).data(‘plugin_applied’, true);
});
};
if ($(‘#myElement’).data(‘plugin_applied’)) {
console.log(‘Pl
43. How do you create a jQuery plugin that supports method calls?
Answer:
Use a switch-case structure to differentiate between initialization and method calls.
$.fn.myPlugin = function(methodOrOptions) {
if (typeof methodOrOptions === ‘object’ || !methodOrOptions) {
// Initialization code here
} else if (typeof methodOrOptions === ‘string’) {
switch (methodOrOptions) {
case ‘destroy’:
// Method code here
break;
// Additional cases here
}
}
};
$(‘#myElement’).myPlugin(‘destroy’);
44. How do you optimize jQuery selectors for better performance?
Answer:
Optimize jQuery selectors by targeting IDs or caching selectors to reduce DOM traversal.
var element = $(‘#myElement’);
element.hide();
element.css(‘color’, ‘red’);
45. How do you debounce a function in jQuery to limit the rate of execution?
Answer:
Use a timer to delay the execution of a function, resetting the timer on each call.
var debounceTimer;
$(‘#myInput’).on(‘keyup’, function() {
clearTimeout(debounceTimer);
debounceTimer = setTimeout(function() {
console.log(‘Debounced event’);
}, 300);
});
46. How do you animate multiple properties simultaneously in jQuery?
Answer:
Use the .animate() method with an object containing the properties you want to animate.
$(‘#myElement’).animate({
width: ‘200px’,
opacity: 0.5
}, 1000);
47. How do you ensure cross-browser compatibility when using jQuery?
Answer:
Use jQuery’s methods instead of native JavaScript functions to ensure cross-browser compatibility.
$(‘#myElement’).css(‘opacity’, 0.5);
48. How do you handle large datasets in jQuery to prevent performance issues?
Answer:
Use techniques like pagination, lazy loading, or virtual scrolling to manage large datasets.
$(‘#loadMore’).click(function() {
// Load next batch of data
});
49. How do you test jQuery code in a unit testing framework?
Answer:
Use frameworks like QUnit or Jasmine to write unit tests for jQuery code.
QUnit.test(‘Element color change’, function(assert) {
$(‘#myElement’).css(‘color’, ‘red’);
assert.equal($(‘#myElement’).css(‘color’), ‘red’, ‘Color is red’);
});
50. How do you handle memory leaks in jQuery?
Answer:
Avoid memory leaks by unbinding event handlers, removing DOM elements properly, and cleaning up references.
$(‘#myElement’).off(‘click’);
$(‘#myElement’).remove();
Final Words
Getting ready for an interview can feel overwhelming, but going through these jQuery fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your jQuery interview but don’t forget to practice the jQuery basics, DOM manipulation, and event handling-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for jQuery?
The most common interview questions for jQuery often cover topics like DOM manipulation, event handling, AJAX, and jQuery selectors.
2. What are the important jQuery topics freshers should focus on for interviews?
The important jQuery topics freshers should focus on include understanding jQuery selectors, event handling methods, AJAX requests, and animations.
3. How should freshers prepare for jQuery technical interviews?
Freshers should prepare for jQuery technical interviews by practicing common DOM manipulation tasks, understanding how to handle events, and working with AJAX.
4. What strategies can freshers use to solve jQuery coding questions during interviews?
Strategies freshers can use include breaking down the problem into smaller tasks, using jQuery selectors effectively, and ensuring cross-browser compatibility.
5. Should freshers prepare for advanced jQuery topics in interviews?
Yes, freshers should prepare for advanced jQuery topics like plugin development, performance optimization, and complex animations if the role requires in-depth jQuery knowledge.
Explore More jQuery Resources
Explore More Interview Questions
Related Posts
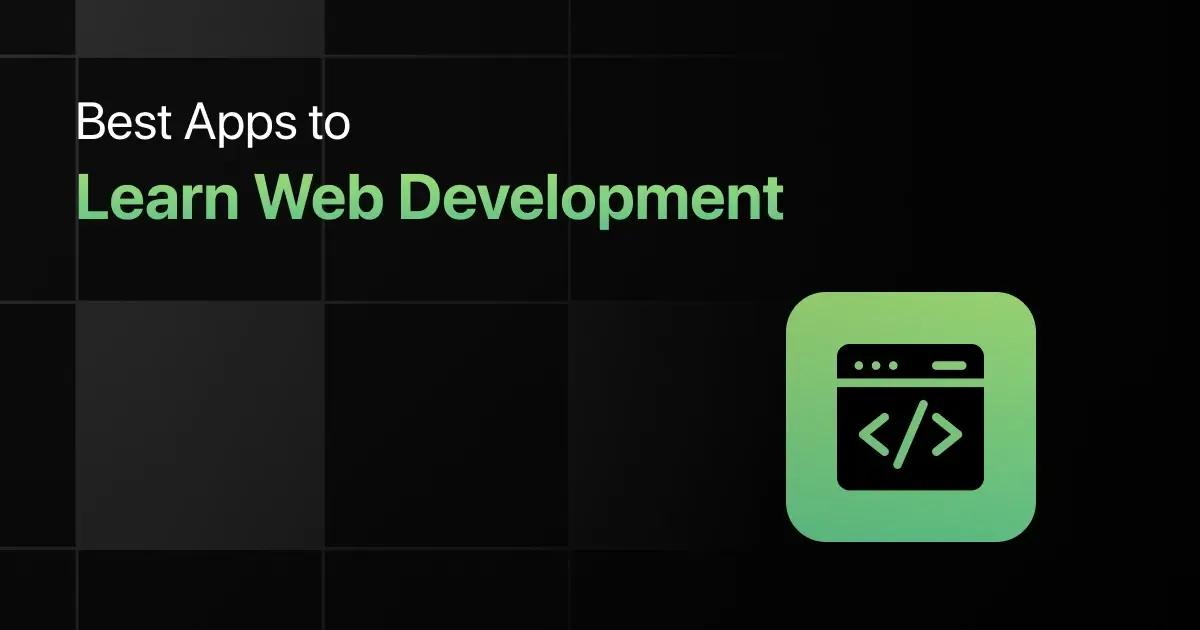
Best Apps to Learn Web Development
Ever thought about building your own website or launching a career in tech but don’t know where to start? With the …