Top iOS Interview Questions for Freshers
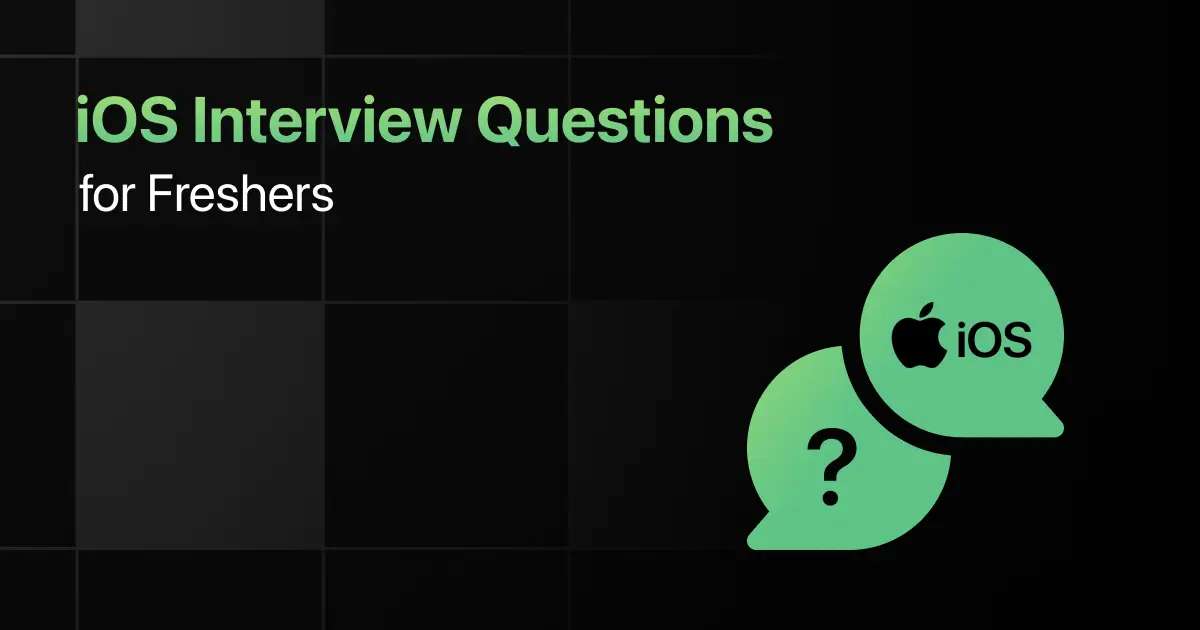
Are you preparing for your first iOS interview and wondering what questions you might face?
Understanding the key iOS interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these iOS interview questions and answers for freshers and make a strong impression in your interview.
Practice iOS Interview Questions and Answers
Below are the top 50 iOS interview questions for freshers with answers:
1. What is iOS?
Answer:
iOS is Apple’s mobile operating system that runs on iPhone, iPad, and iPod Touch devices. It is known for its security, performance, and clean design. iOS apps are built using Objective-C or Swift and are distributed through the Apple App Store.
2. What is the difference between Frame and Bounds in iOS?
Answer:
The frame represents the view’s location and size in the coordinate system of its superview. In contrast, the bounds defines the view’s location and size in its own coordinate system (starting from 0,0). Frame changes affect the position relative to the parent, while bounds affect content display inside the view.
view.bounds // Relative to the view itself
3. What is MVC in iOS?
Answer:
MVC stands for Model-View-Controller, a design pattern used in iOS development. The model represents the data, the view displays the UI, and the controller acts as an intermediary that handles logic and updates the view. It helps in organizing code and making it more maintainable.
4. What is ARC in iOS?
Answer:
ARC (Automatic Reference Counting) is a memory management feature in iOS. It automatically manages the allocation and deallocation of objects. When an object’s reference count drops to zero, ARC deallocates the object, preventing memory leaks. ARC doesn’t require manual memory management, simplifying code.
5. What is a delegate in iOS?
Answer:
A delegate is a design pattern that allows one object to send messages or events to another object. In iOS, it is commonly used in table views and collection views to handle events like selecting a row. It decouples components, making them more flexible and reusable.
func didTapButton()
}
class MyViewController: MyDelegate {
func didTapButton() {
print(“Button Tapped”)
}
}
6. Explain Retain Cycles in iOS and how to prevent them.
Answer:
A retain cycle occurs when two objects hold strong references to each other, preventing ARC from deallocating them. This results in memory leaks. To avoid this, you can use weak or unowned references. A weak reference doesn’t increase the reference count.
var b: B?
}
class B {
weak var a: A?
}
7. What is the difference between weak, strong, and unowned in Swift?
Answer:
- strong: Default reference that increases the reference count and retains the object.
- weak: Doesn’t increase the reference count. The reference is optional and automatically set to nil when the object is deallocated.
- unowned: Doesn’t increase the reference count. It’s not optional and must not be nil when accessed, else it causes a crash.
unowned var manager: Manager
8. What is the difference between @IBOutlet and @IBAction in iOS?
Answer:
- @IBOutlet: Connects the UI components like labels, buttons, and images in Interface Builder to your code.
- @IBAction: Links UI events (such as a button tap) to your code, triggering a method when the event occurs.
@IBAction func buttonPressed(_ sender: UIButton) {
print(“Button Pressed”)
}
9. Explain the concept of Optional in Swift.
Answer:
Optionals in Swift are used to represent the absence or presence of a value. An optional either holds a value or nil. You can safely unwrap an optional using if-let or guard-let to avoid crashes caused by unwrapping nil.
if let unwrappedName = name {
print(unwrappedName)
}
10. What is the use of guard statement in Swift?
Answer:
The guard statement is used to check conditions and exit early from a function if the conditions aren’t met. It helps improve code readability by eliminating nested if-else blocks. guard requires an else block to handle the failure case.
return
}
print(name)
11. What is GCD (Grand Central Dispatch) in iOS?
Answer:
GCD is a low-level API used for managing concurrent tasks in iOS. It allows you to perform background operations without blocking the main thread, ensuring a smooth user experience. It manages thread queues and can execute tasks asynchronously or synchronously.
// Background thread
DispatchQueue.main.async {
// Main thread
}
}
12. What is the difference between synchronous and asynchronous in iOS?
Answer:
- Synchronous: Blocks the current thread until the task is finished, which can cause the UI to freeze.
- Asynchronous: Runs the task in the background, allowing the main thread to remain responsive, preventing UI freezes.
13. What is the purpose of UserDefaults in iOS?
Answer:
UserDefaults is a simple storage mechanism for saving small pieces of data, like user preferences or settings. It stores data as key-value pairs and persists even after the app is closed. It’s best used for lightweight storage, such as booleans, strings, and integers.
let username = UserDefaults.standard.string(forKey: “username”)
14. What are closures in Swift?
Answer:
A closure is a self-contained block of functionality that can be passed and used in your code. Closures in Swift capture and store references to variables from their surrounding context, making them powerful for asynchronous programming.
print(“Hello, World!”)
}
myClosure()
15. What is Core Data in iOS?
Answer:
Core Data is Apple’s framework for managing an object graph and persisting data to disk. It can store large amounts of data efficiently and works well for relational data models. It supports various storage types, including SQLite and binary data.
let entity = NSEntityDescription.insertNewObject(forEntityName: “Person”, into: context)
entity.setValue(“John”, forKey: “name”)
try context.save()
16. What is the difference between Nil and NSNull in iOS?
Answer:
- nil: Represents the absence of a value in Swift and Objective-C, typically used for optional variables.
- NSNull: Represents a null value in collections like arrays and dictionaries, as these cannot store nil. It is an instance of a class in Objective-C that acts as a placeholder for nil.
17. What is KVC (Key-Value Coding) in iOS?
Answer:
KVC allows accessing and modifying an object’s properties dynamically using string keys. It bypasses the need for direct accessors (getter and setter methods). It is used in situations where properties are not known at compile time.
@objc var name: String = “John”
}
let person = Person()
person.setValue(“Jane”, forKey: “name”)
print(person.value(forKey: “name”) as! String) // Output: Jane
18. What is KVO (Key-Value Observing) in iOS?
Answer:
KVO is a mechanism that allows observing changes to a property. When the value of a property changes, the observer is notified. It is commonly used for UI updates in response to data changes.
override func observeValue(forKeyPath keyPath: String?, of object: Any?, change: [NSKeyValueChangeKey : Any]?, context: UnsafeMutableRawPointer?) {
print(“Name changed to \(change?[.newKey] ?? “”)”)
}
19. What is the difference between UITableView and UICollectionView?
Answer:
- UITableView: Displays data in a single-column, vertical scrollable list. It is good for structured data in a list format.
- UICollectionView: Displays data in a customizable grid-like structure, with multiple columns and sections. It offers more flexibility and custom layouts.
20. What is a Lazy Property in Swift?
Answer:
A lazy property is one that does not get initialized until it’s first accessed. This helps in optimizing memory usage, especially when the property’s value depends on external factors or is expensive to initialize.
func loadLargeData() -> [Int] {
return [1, 2, 3, 4, 5] }
21. What is a Protocol in Swift?
Answer:
A protocol defines a blueprint of methods, properties, and other requirements that suit a particular task or piece of functionality. Classes, structs, and enums can conform to protocols, providing implementations for the required methods and properties.
func doSomething()
}
class MyClass: MyProtocol {
func doSomething() {
print(“Doing something”)
}
}
22. What is the difference between Struct and Class in Swift?
Answer:
- Class: Reference type, meaning instances share the same memory reference. Supports inheritance, deinitializers, and reference counting (ARC).
- Struct: Value type, meaning instances are copied when assigned or passed. Does not support inheritance or deinitializers, but it’s more efficient for small, lightweight data.
class MyClass { var name: String = “” }
23. What is the purpose of DispatchGroup in iOS?
Answer:
DispatchGroup allows grouping multiple tasks together and then notifying when all the tasks in the group are complete. This is useful when performing multiple asynchronous tasks and waiting for all of them to finish before proceeding.
group.enter()
// Perform some task
group.leave()
group.notify(queue: .main) {
print(“All tasks completed”)
}
24. What is Codable in Swift?
Answer:
Codable is a protocol that allows for easy encoding and decoding of data types, typically to and from JSON, XML, or other formats. Structs and classes can conform to Codable for seamless serialization and deserialization.
var name: String
var age: Int
}
let jsonData = try? JSONEncoder().encode(Person(name: “John”, age: 30))
25. Explain the concept of Dependency Injection in iOS.
Answer:
Dependency Injection is a design pattern where an object receives its dependencies from an external source rather than creating them internally. This improves code modularity, testability, and maintainability by decoupling objects.
let engine: Engine
init(engine: Engine) {
self.engine = engine
}
}
26. What is a tuple in Swift?
Answer:
A tuple in Swift is a group of multiple values combined into a single compound value. It can contain different types and is useful for returning multiple values from a function.
print(person.name) // Output: John
Answer:
A singleton is a design pattern that restricts the instantiation of a class to one object. It ensures that a class has only one instance and provides a global point of access to it.
static let shared = Singleton()
private init() { }
}
28. What is the use of NotificationCenter in iOS?
Answer:
NotificationCenter is a communication mechanism that allows one part of an app to notify other parts about events without directly referencing them. It decouples objects, making the app more modular.
NotificationCenter.default.addObserver(self, selector: #selector(handleNotification), name: .myNotification, object: nil)
29. Explain the use of URLSession in iOS.
Answer:
URLSession is used to create and manage network tasks, such as downloading data or files from the web, uploading data, and performing background uploads or downloads. It is highly configurable and allows for synchronous and asynchronous operations.
let task = URLSession.shared.dataTask(with: url) { data, response, error in
if let data = data {
print(data)
}
}
task.resume()
30. What are generics in Swift?
Answer:
Generics allow you to write flexible, reusable functions and types that can work with any data type. They help avoid code duplication while maintaining type safety.
let temp = a
a = b
b = temp
}
31. What is the difference between flatMap and map in Swift?
Answer:
- map: Transforms each element of a collection and returns an array of results. It doesn’t flatten nested collections.
- flatMap: Transforms and flattens the result into a single collection by removing optional values or nested arrays.
32. What are Access Control Levels in Swift?
Answer:
Swift provides five access control levels:
- open: Accessible and overrideable anywhere, even outside the module.
- public: Accessible outside the module but cannot be subclassed or overridden.
- internal: Default level, accessible only within the module.
- fileprivate: Accessible only within the same file.
- private: Accessible only within the enclosing declaration (class, struct, etc.).
33. What is the difference between @escaping and non-escaping closures?
Answer:
- Non-escaping: The closure is executed before the function returns, and it is the default behavior.
- @escaping: The closure is allowed to outlive the function’s execution, meaning it can be called later (e.g., in asynchronous operations).
DispatchQueue.main.asyncAfter(deadline: .now() + 1) {
closure()
}
}
34. Explain the defer statement in Swift.
Answer:
defer is used to schedule a block of code that will be executed when the scope it is in exits. It’s useful for cleaning up resources, like closing files or releasing locks, ensuring that the code runs at the end of the function, no matter what.
defer {
print(“File closed”)
}
print(“Performing operations on file”)
}
35. What is the difference between viewDidLoad and viewWillAppear in iOS?
Answer:
- viewDidLoad: Called once when the view is loaded into memory. It is ideal for one-time setups like loading data and initializing views.
- viewWillAppear: Called every time the view is about to appear on the screen. It’s good for tasks that need to be done every time the view is shown, like refreshing data.
36. What is the difference between convenience and designated initializers in Swift?
Answer:
- Designated initializer: The main initializer of a class that fully initializes all properties. Every class must have at least one designated initializer.
- Convenience initializer: A secondary initializer that calls a designated initializer internally. It’s used to simplify the initialization process or provide default values.
var name: String
init(name: String) {
self.name = name
}
convenience init() {
self.init(name: “Default Name”)
}
}
37. What is the difference between guard and if in Swift?
Answer:
- if: Executes the code block only if the condition is true.
- guard: Exits the function early if the condition is false, ensuring the rest of the function can assume the condition is true. guard is typically used for early exits and improves readability.
guard let age = age, age > 18 else {
print(“Invalid age”)
return
}
print(“Valid age: \(age)”)
}
38. What is the purpose of the mutating keyword in Swift?
Answer:
The mutating keyword is used in structs and enums to indicate that a method can modify the properties of the instance it is called on. Structs and enums are value types, so their methods are immutable unless marked as mutating.
var value = 0
mutating func increment() {
value += 1
}
}
39. Explain what UIApplicationDelegate is.
Answer:
UIApplicationDelegate is a protocol that defines methods to handle app-wide events, such as app launch, state transitions (background, foreground), notifications, and termination. It acts as the central hub for handling these global events in an iOS app.
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
return true
}
}
40. What are dynamic and @objc used for in Swift?
Answer:
- @objc: Exposes Swift methods and properties to Objective-C runtime, which is required when using KVO, selectors, or interacting with Objective-C APIs.
- dynamic: Used with @objc to enable dynamic dispatch, ensuring that methods can be overridden and dispatched at runtime rather than at compile time.
41. What is the use of didSet and willSet in Swift?
Answer:
didSet and willSet are property observers in Swift. willSet is called just before a property’s value is changed, while didSet is called immediately after the value changes. These are used to execute custom code when properties change.
willSet {
print(“Will set new value: \(newValue)”)
}
didSet {
print(“Did set old value: \(oldValue)”)
}
}
42. What is the purpose of the @discardableResult annotation in Swift?
Answer:
@discardableResult is used to suppress warnings when the return value of a function is not used. This is helpful for functions that return a value but the caller may not always need it.
func add(_ a: Int, _ b: Int) -> Int {
return a + b
}
43. Explain the concept of typealias in Swift.
Answer:
typealias allows you to define a new name for an existing type. It is useful for improving code readability, especially when dealing with complex types like closures or long generic types.
func performTask(with completion: CompletionHandler) {
completion(true)
}
44. What is the difference between Any, AnyObject, and nil in Swift?
Answer:
- Any: Represents any type, including both value and reference types.
- AnyObject: Represents any reference type, typically classes.
- nil: Represents the absence of a value and is used for optionals.
45. Explain how threading works in iOS using GCD.
Answer:
Threading in iOS is managed using Grand Central Dispatch (GCD). It allows tasks to be scheduled on different dispatch queues (main, global, or custom). Tasks can be executed synchronously or asynchronously, improving app performance by keeping the main thread free for UI updates.
// Background thread
DispatchQueue.main.async {
// Back to main thread for UI updates
}
}
46. What is a weak reference and why is it used?
Answer:
A weak reference does not increase the reference count of an object. It is used to prevent retain cycles, which can cause memory leaks when two objects hold strong references to each other. Weak references are set to nil when the referenced object is deallocated.
weak var friend: Person?
}
47. What is Auto Layout in iOS?
Answer:
Auto Layout is a system that allows developers to define rules for how views should be arranged on the screen. It adjusts the layout dynamically based on the device’s screen size, orientation, and other constraints, making it ideal for creating responsive designs.
48. What are extensions in Swift and why are they useful?
Answer:
Extensions allow you to add functionality to existing classes, structs, enums, or protocols without modifying their source code. They can add new methods, computed properties, initializers, and more, enhancing code modularity and organization.
func reversedString() -> String {
return String(self.reversed())
}
}
49. Explain the concept of Generics in Swift.
Answer:
Generics allow writing flexible, reusable functions and types that can work with any type. This helps in avoiding code duplication while maintaining type safety.
let temp = a
a = b
b = temp
}
50. What is a @propertyWrapper in Swift?
Answer:
@propertyWrapper is a feature in Swift that provides a way to add custom logic to the getting and setting of property values. By defining a property wrapper, you can encapsulate code that runs whenever a property’s value is accessed or modified. This is useful for adding functionality like validation, default values, or formatting in a reusable way.
struct Capitalized {
private var text: String
var wrappedValue: String {
get { text }
set { text = newValue.capitalized }
}
init(wrappedValue: String) {
self.text = wrappedValue.capitalized
}
}
class Person {
@Capitalized var name: String
init(name: String) {
self.name = name
}
}
Final Words
Getting ready for an interview can feel overwhelming, but going through these iOS fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your iOS interview, but don’t forget to practice key iOS concepts, UIKit, Swift programming, memory management, and view controller lifecycle-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for iOS?
Common iOS interview questions often cover topics like the iOS app lifecycle, memory management (ARC), delegates, protocols, Swift syntax, handling UI elements with UIKit, and Swift concurrency using GCD or async/await.
2. What are the important iOS topics freshers should focus on for interviews?
Freshers should focus on the Swift programming language, UIKit, Auto Layout, view controllers, iOS app architecture (MVC, MVVM), memory management (ARC), and handling asynchronous tasks with GCD or Combine.
3. How should freshers prepare for iOS technical interviews?
Freshers should practice coding in Swift, build sample apps, understand the app lifecycle, and focus on concepts like view controllers, table views, networking (URLSession), Core Data, and handling concurrency.
4. What strategies can freshers use to solve iOS coding questions during interviews?
To solve iOS coding questions, freshers should break down the problem into small components, use Swift’s built-in libraries efficiently, write clean and readable code, and explain their thought process, especially when using design patterns or solving UI/UX issues.
5. Should freshers prepare for advanced iOS topics in interviews?
While advanced topics like Core Data, custom animations, and concurrency management (GCD, async/await) are beneficial, freshers should prioritize mastering the basics and understanding key iOS concepts before diving into advanced areas.
Explore More Interview Questions
- Python
- Java
- SQL
- React
- JavaScript
- C Programming
- HTML
- CSS
- Angular
- C++
- Spring Boot
- Node JS
- Excel
- C#
- DBMS
- PHP
- Linux
- Operating System
- MySQL
- Spring
- Flutter
- MongoDB
- Django
- React Native
- jQuery
- Bootstrap
- Embedded C
- DSA
- R Programming
- Hadoop
- .NET
- Power BI
- ASP.NET
- ASP.NET MVC
- Android
- Tableau
- MVC
- WordPress
- TypeScript
- Spark
- Kotlin
- Swift
- Golang
- Shell Scripting
Related Posts
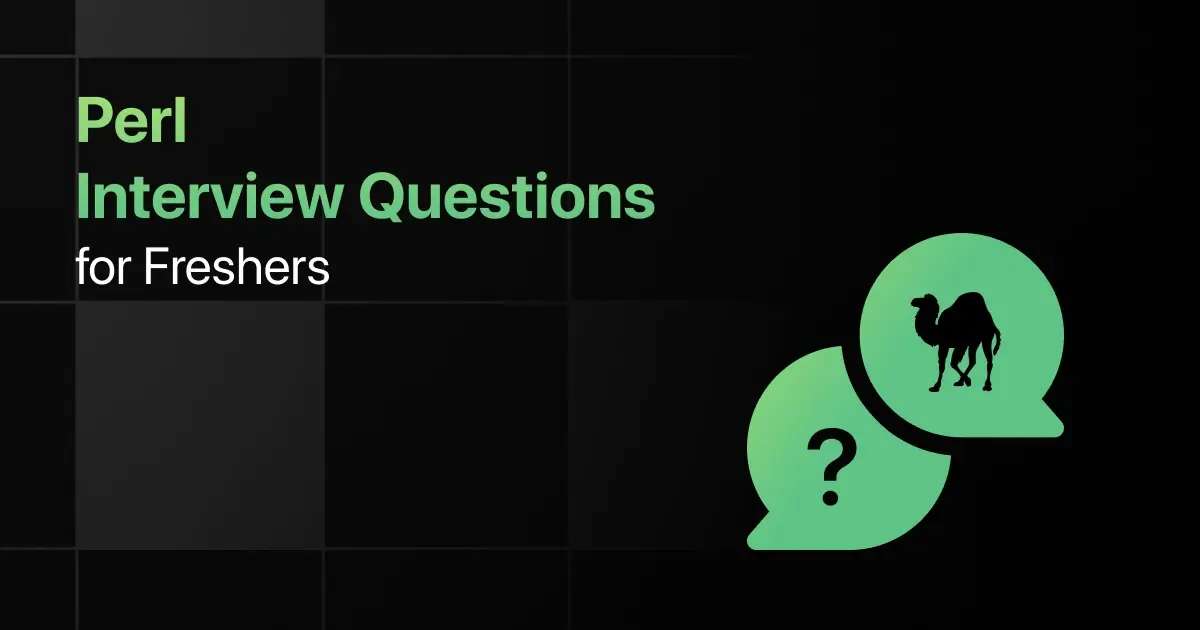
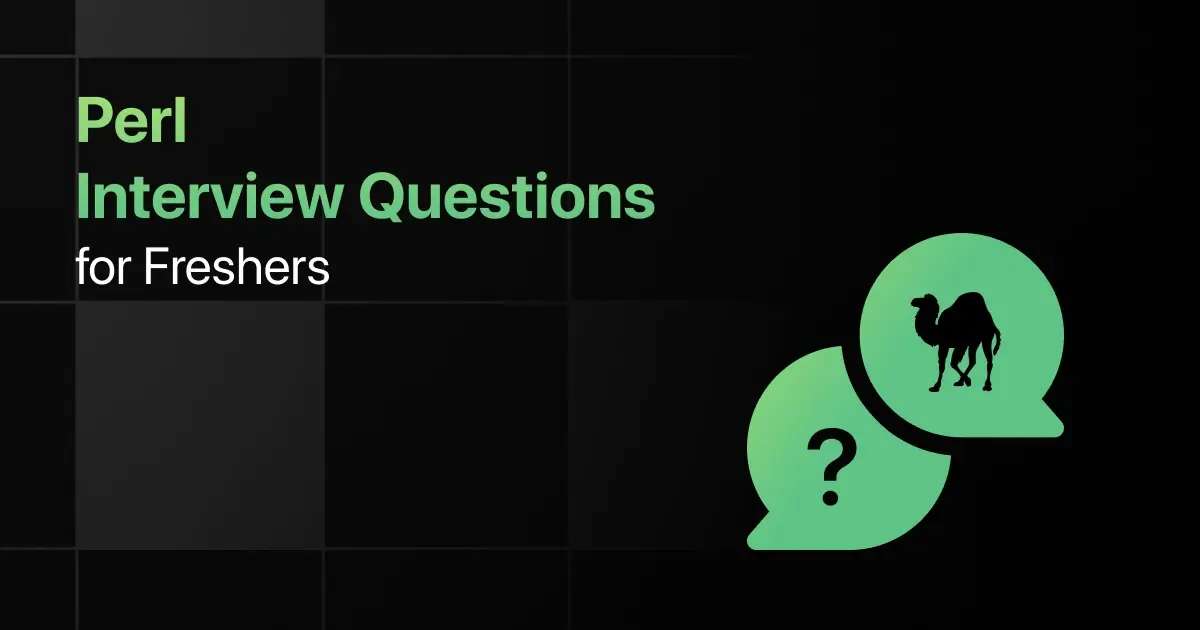
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …