Top Flutter Interview Questions for Freshers
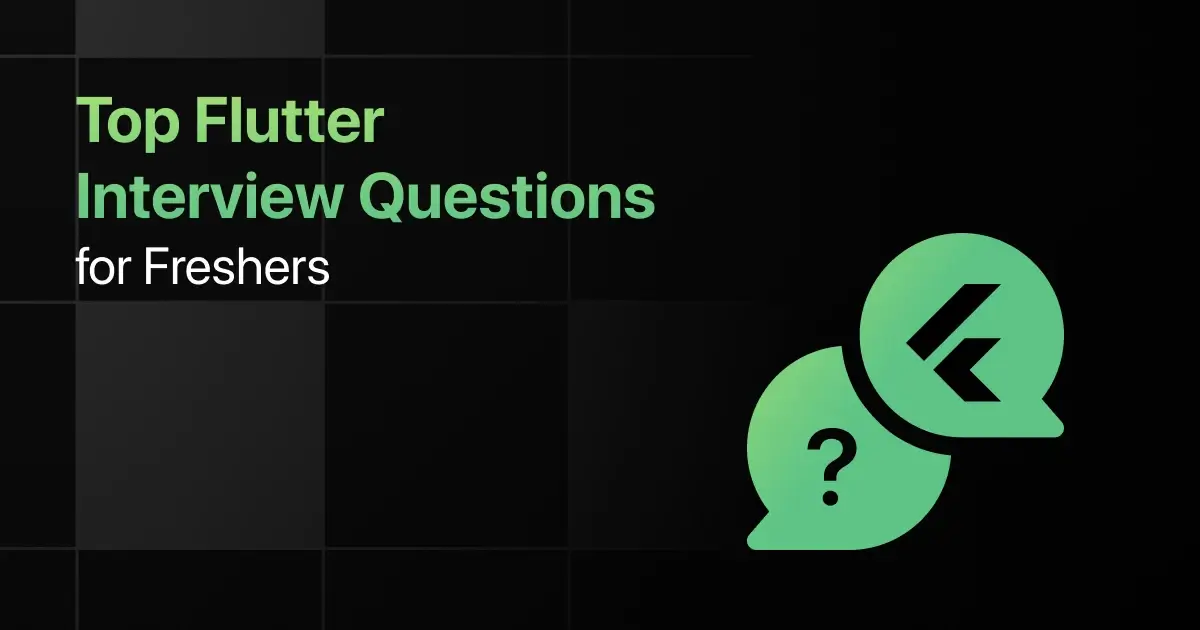
Are you preparing for your first Flutter interview and wondering what questions you might face?
Understanding the key Flutter interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Flutter interview questions and answers for freshers and make a strong impression in your interview.
Practice Flutter Interview Questions and Answers
Below are the top 50 flutter interview questions for freshers with answers:
1. How do you create a basic Flutter application with a Text widget centered on the screen?
Answer:
Use the MaterialApp and Scaffold widgets to set up the basic structure, and Center to center the Text widget.
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Center(child: Text(‘Hello, Flutter!’)),
),
);
}
}
2. How can you create a custom button widget in Flutter?
Answer:
Extend StatelessWidget or StatefulWidget and customize the UI using GestureDetector or InkWell for handling tap events.
class CustomButton extends StatelessWidget {
final String text;
final VoidCallback onPressed;
CustomButton({required this.text, required this.onPressed});
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: onPressed,
child: Container(
padding: EdgeInsets.all(10.0),
color: Colors.blue,
child: Text(text, style: TextStyle(color: Colors.white)),
),
);
}
}
3. How do you implement a dynamic list in Flutter?
Answer:
Use ListView.builder to create a dynamic list where the number of items can vary based on the data source.
class DynamicList extends StatelessWidget {
final List<String> items = List.generate(10, (index) => ‘Item $index’);
@override
Widget build(BuildContext context) {
return ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
return ListTile(title: Text(items[index]));
},
);
}
}
4. How can you create a grid view in Flutter?
Answer:
Use GridView.builder with SliverGridDelegateWithFixedCrossAxisCount to create a grid layout.
class GridViewExample extends StatelessWidget {
@override
Widget build(BuildContext context) {
return GridView.builder(
gridDelegate: SliverGridDelegateWithFixedCrossAxisCount(crossAxisCount: 2),
itemCount: 10,
itemBuilder: (context, index) {
return Card(
color: Colors.blue,
child: Center(child: Text(‘Item $index’)),
);
},
);
}
}
5. How do you handle form validation in Flutter?
Answer:
Use Form and TextFormField widgets with GlobalKey<FormState> to handle form validation.
final _formKey = GlobalKey<FormState>();
Form(
key: _formKey,
child: Column(
children: [
TextFormField(
validator: (value) {
if (value == null || value.isEmpty) {
return ‘Please enter some text’;
}
return null;
},
),
ElevatedButton(
onPressed: () {
if (_formKey.currentState!.validate()) {
// Process data
}
},
child: Text(‘Submit’),
),
],
),
);
6. How do you manage the state in a Flutter application using setState?
Answer:
Use setState within a StatefulWidget to update the UI whenever the state changes.
class Counter extends StatefulWidget {
@override
_CounterState createState() => _CounterState();
}
class _CounterState extends State<Counter> {
int _count = 0;
void _incrementCounter() {
setState(() {
_count++;
});
}
@override
Widget build(BuildContext context) {
return Column(
children: [
Text(‘Count: $_count’),
ElevatedButton(
onPressed: _incrementCounter,
child: Text(‘Increment’),
),
],
);
}
}
7. How do you implement a stateful widget that holds a counter and increments on button press?
Answer:
Create a StatefulWidget, manage the counter value in the state, and update it using setState.
class IncrementCounter extends StatefulWidget {
@override
_IncrementCounterState createState() => _IncrementCounterState();
}
class _IncrementCounterState extends State<IncrementCounter> {
int _counter = 0;
@override
Widget build(BuildContext context) {
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(‘Counter: $_counter’),
ElevatedButton(
onPressed: () {
setState(() {
_counter++;
});
},
child: Text(‘Increment’),
),
],
);
}
}
8. How do you implement global state management using the Provider package in Flutter?
Answer:
Wrap your MaterialApp in a ChangeNotifierProvider and use Consumer or Provider.of to access and update the state.
void main() {
runApp(
ChangeNotifierProvider(
create: (context) => Counter(),
child: MyApp(),
),
);
}
class Counter extends ChangeNotifier {
int _count = 0;
int get count => _count;
void increment() {
_count++;
notifyListeners();
}
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text(‘Provider Example’)),
body: Center(
child: Consumer<Counter>(
builder: (context, counter, child) => Text(‘Count: ${counter.count}’),
),
),
floatingActionButton: FloatingActionButton(
onPressed: () => context.read<Counter>().increment(),
child: Icon(Icons.add),
),
),
);
}
}
9. How do you persist state using SharedPreferences in Flutter?
Answer:
Use the SharedPreferences package to save and retrieve simple data types across app launches.
void _saveCounter(int value) async {
SharedPreferences prefs = await SharedPreferences.getInstance();
prefs.setInt(‘counter’, value);
}
Future<int> _loadCounter() async {
SharedPreferences prefs = await SharedPreferences.getInstance();
return prefs.getInt(‘counter’) ?? 0;
}
10. How do you manage state in a complex Flutter app using Riverpod?
Answer:
Use Riverpod to manage state by defining providers for state management and using ConsumerWidget or Provider.of to access state in the UI.
final counterProvider = StateProvider<int>((ref) => 0);
class CounterApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return ProviderScope(
child: MaterialApp(
home: Scaffold(
body: Center(
child: Consumer(builder: (context, watch, child) {
final count = watch(counterProvider).state;
return Text(‘$count’);
}),
),
floatingActionButton: FloatingActionButton(
onPressed: () => context.read(counterProvider).state++,
child: Icon(Icons.add),
),
),
),
);
}
}
11. How do you implement navigation between two screens in Flutter?
Answer:
Use Navigator.push and Navigator.pop to navigate between screens.
Navigator.push(
context,
MaterialPageRoute(builder: (context) => SecondScreen()),
);
12. How do you pass data between screens in Flutter?
Answer:
Pass data by including it in the constructor of the destination screen.
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => SecondScreen(data: ‘Hello’),
),
);
13. How do you implement named routes in Flutter?
Answer:
Define routes in the MaterialApp and navigate using Navigator.pushNamed.
MaterialApp(
initialRoute: ‘/’,
routes: {
‘/’: (context) => HomeScreen(),
‘/second’: (context) => SecondScreen(),
},
);
Navigator.pushNamed(context, ‘/second’);
14. How do you handle deep linking in a Flutter application?
Answer:
Use the flutter_deep_linking package or platform-specific methods to handle deep links and route users to specific parts of the app.
15. How do you implement bottom navigation with multiple pages in Flutter?
Answer:
Use BottomNavigationBar with IndexedStack or a PageView to manage navigation between different pages.
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _selectedIndex = 0;
static List<Widget> _widgetOptions = <Widget>[
Text(‘Home’),
Text(‘Business’),
Text(‘School’),
];
void _onItemTapped(int index) {
setState(() {
_selectedIndex = index;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: _widgetOptions.elementAt(_selectedIndex),
),
bottomNavigationBar: BottomNavigationBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(
icon: Icon(Icons.home),
label: ‘Home’,
),
BottomNavigationBarItem(
icon: Icon(Icons.business),
label: ‘Business’,
),
BottomNavigationBarItem(
icon: Icon(Icons.school),
label: ‘School’,
),
],
currentIndex: _selectedIndex,
onTap: _onItemTapped,
),
);
}
}
16. How do you handle asynchronous operations in Flutter using Future and async/await?
Answer:
Use async functions to return a Future, and await to pause execution until the Future completes.
Future<void> fetchData() async {
var data = await getDataFromAPI();
print(data);
}
17. How do you manage a stream of data in Flutter using StreamBuilder?
Answer:
Use StreamBuilder to listen to a stream and update the UI based on the data received.
StreamBuilder<int>(
stream: myStream,
builder: (context, snapshot) {
if (snapshot.hasData) {
return Text(‘Data: ${snapshot.data}’);
} else {
return CircularProgressIndicator();
}
},
);
18. How do you perform a background task in Flutter using Isolate?
Answer:
Use Isolate to perform heavy computations in the background, freeing up the main thread.
Isolate.spawn(doWork, ‘Hello’);
void doWork(String message) {
print(‘Isolate: $message’);
}
19. How do you handle multiple asynchronous tasks in Flutter and wait for all of them to complete?
Answer:
Use Future.wait to run multiple futures in parallel and wait for all of them to complete.
List<Future> futures = [fetchData1(), fetchData2()];
await Future.wait(futures);
20. How do you debounce an input field in Flutter to avoid excessive API calls?
Answer:
Use a Timer to delay API calls and reset the timer on each input change, only proceeding when the input stops for a set time.
Timer? _debounce;
onChanged(String text) {
if (_debounce?.isActive ?? false) _debounce!.cancel();
_debounce = Timer(const Duration(milliseconds: 500), () {
// API call
});
}
21. How do you create a simple fade transition animation in Flutter?
Answer:
Use the AnimatedOpacity widget to create a fade transition.
AnimatedOpacity(
opacity: _visible ? 1.0 : 0.0,
duration: Duration(seconds: 1),
child: Text(‘Fade In’),
);
22. How do you implement a hero animation between two screens in Flutter?
Answer:
Wrap the shared widget with a Hero widget and give it a tag, which will animate between screens.
Hero(
tag: ‘hero-tag’,
child: Image.network(‘https://example.com/image.jpg’),
);
23. How do you create a custom animation using AnimationController and Tween in Flutter?
Answer:
Use AnimationController to manage the animation and Tween to define the range of values.
AnimationController _controller = AnimationController(
duration: const Duration(seconds: 2),
vsync: this,
);
Animation<double> _animation = Tween<double>(begin: 0, end: 1).animate(_controller);
24. How do you implement a rotating animation in Flutter using Transform.rotate?
Answer:
Use Transform.rotate with an animation controller to rotate a widget.
Transform.rotate(
angle: _controller.value * 2.0 * math.pi,
child: Icon(Icons.refresh),
);
25. How do you create a bouncing button animation in Flutter?
Answer:
Use ScaleTransition with a CurvedAnimation to create a bouncing effect.
ScaleTransition(
scale: CurvedAnimation(
parent: _controller,
curve: Curves.elasticInOut,
),
child: ElevatedButton(onPressed: () {}, child: Text(‘Bounce’)),
);
26. How do you write a unit test for a function in Flutter?
Answer:
Use the test package to write unit tests for functions, ensuring they return the correct results.
test(‘adds one to input values’, () {
final calculator = Calculator();
expect(calculator.addOne(2), 3);
expect(calculator.addOne
27. How do you write a widget test in Flutter?
Answer:
Use WidgetTester to create a widget test that verifies the UI behavior.
testWidgets(‘Counter increments smoke test’, (WidgetTester tester) async {
await tester.pumpWidget(MyApp());
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
expect(find.text(‘1’), findsOneWidget);
});
28. How do you perform integration testing in Flutter?
Answer:
Use the integration_test package to perform end-to-end testing of a Flutter application.
final binding = IntegrationTestWidgetsFlutterBinding.ensureInitialized();
testWidgets(‘test description’, (tester) async {
await tester.pumpWidget(MyApp());
// Perform actions and checks
});
29. How do you mock dependencies in a Flutter test?
Answer:
Use the mockito package to create mock objects and stub methods for testing.
var mockService = MockService();
when(mockService.getData()).thenReturn(Future.value(‘mocked data’));
30. How do you test a widget that depends on asynchronous data in Flutter?
Answer:
Use pumpAndSettle to wait for all frames to be rendered after asynchronous calls.
await tester.pumpAndSettle();
expect(find.text(‘Async data’), findsOneWidget);
31. How do you make a GET request in Flutter using http package?
Answer:
Use the http.get method to fetch data from an API and handle the response.
final response = await http.get(Uri.parse(‘https://jsonplaceholder.typicode.com/posts’));
if (response.statusCode == 200) {
var data = jsonDecode(response.body);
} else {
throw Exception(‘Failed to load data’);
}
32. How do you handle JSON parsing in Flutter?
Answer:
Use the jsonDecode function to convert a JSON string into a Dart map.
Map<String, dynamic> user = jsonDecode(response.body);
33. How do you send data to a server using POST request in Flutter?
Answer:
Use http.post to send data to a server, passing the body as a JSON-encoded string.
final response = await http.post(
Uri.parse(‘https://jsonplaceholder.typicode.com/posts’),
headers: {“Content-Type”: “application/json”},
body: jsonEncode(<String, String>{‘title’: ‘foo’, ‘body’: ‘bar’, ‘userId’: ‘1’}),
);
34. How do you implement error handling for network requests in Flutter?
Answer:
Use try-catch blocks to handle exceptions and ensure proper error messages are displayed.
try {
final response = await http.get(Uri.parse(‘https://jsonplaceholder.typicode.com/posts’));
if (response.statusCode == 200) {
// Parse data
} else {
throw Exception(‘Failed to load data’);
}
} catch (e) {
print(e);
}
35. How do you display data fetched from a REST API in a ListView in Flutter?
Answer:
Fetch the data asynchronously and use a FutureBuilder to display the data in a ListView.
Future<List<Post>> fetchPosts() async {
final response = await http.get(Uri.parse(‘https://jsonplaceholder.typicode.com/posts’));
return (jsonDecode(response.body) as List).map((data) => Post.fromJson(data)).toList();
}
@override
Widget build(BuildContext context) {
return FutureBuilder<List<Post>>(
future: fetchPosts(),
builder: (context, snapshot) {
if (snapshot.hasData) {
return ListView.builder(
itemCount: snapshot.data!.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(snapshot.data![index].title),
);
},
);
} else if (snapshot.hasError) {
return Text(‘${snapshot.error}’);
}
return CircularProgressIndicator();
},
);
}
36. How do you use the url_launcher plugin to open a web URL in Flutter?
Answer:
Use url_launcher to open URLs in the default browser.
if (await canLaunch(url)) {
await launch(url);
} else {
throw ‘Could not launch $url’;
}
37. How do you implement local notifications in Flutter using flutter_local_notifications?
Answer:
Use flutter_local_notifications to schedule and display notifications.
final FlutterLocalNotificationsPlugin flutterLocalNotificationsPlugin =
FlutterLocalNotificationsPlugin();
final InitializationSettings initializationSettings = InitializationSettings(
android: AndroidInitializationSettings(‘@mipmap/ic_launcher’),
);
flutterLocalNotificationsPlugin.initialize(initializationSettings);
flutterLocalNotificationsPlugin.show(
0,
‘Hello’,
‘This is a notification’,
NotificationDetails(
android: AndroidNotificationDetails(
‘your channel id’,
‘your channel name’,
‘your channel description’,
),
),
);
38. How do you integrate Firebase with a Flutter application?
Answer:
Add Firebase to your Flutter project by including the firebase_core and other Firebase packages, and initialize Firebase in the main function.
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(MyApp());
}
39. How do you handle user authentication with Firebase in Flutter?
Answer:
Use firebase_auth to manage user authentication, including login and registration.
final FirebaseAuth _auth = FirebaseAuth.instance;
Future<User?> signIn(String email, String password) async {
final UserCredential user = await _auth.signInWithEmailAndPassword(email: email, password: password);
return user.user;
}
40. How do you add in-app purchases in a Flutter application using in_app_purchase plugin?
Answer:
Use the in_app_purchase plugin to manage product purchases and subscription services within your Flutter app.
final bool available = await InAppPurchase.instance.isAvailable();
final ProductDetailsResponse response = await InAppPurchase.instance.queryProductDetails(productIds);
InAppPurchase.instance.buyNonConsumable(purchaseParam: PurchaseParam(productDetails: productDetails));
41. How do you store data locally in Flutter using shared_preferences?
Answer:
Use shared_preferences to store key-value pairs locally in the device.
SharedPreferences prefs = await SharedPreferences.getInstance();
prefs.setString(‘username’, ‘JohnDoe’);
42. How do you implement a SQLite database in Flutter?
Answer:
Use the sqflite package to manage SQLite databases, including creating tables and performing CRUD operations.
final database = openDatabase(
join(await getDatabasesPath(), ‘demo.db’),
onCreate: (db, version) {
return db.execute(
“CREATE TABLE users(id INTEGER PRIMARY KEY, name TEXT, age INTEGER)”,
);
},
version: 1,
);
Future<void> insertUser(User user) async {
final db = await database;
await db.insert(
‘users’,
user.toMap(),
conflictAlgorithm: ConflictAlgorithm.replace,
);
}
43. How do you retrieve data from a SQLite database in Flutter?
Answer:
Use the sqflite package to query the SQLite database and retrieve the results.
Future<List<User>> users() async {
final db = await database;
final List<Map<String, dynamic>> maps = await db.query(‘users’);
return List.generate(maps.length, (i) {
return User(id: maps[i][‘id’], name: maps[i][‘name’], age: maps[i][‘age’]);
});
}
44. How do you perform database migrations in Flutter using sqflite?
Answer:
Use the onUpgrade callback in openDatabase to handle schema changes between database versions.
final database = openDatabase(
join(await getDatabasesPath(), ‘demo.db’),
onUpgrade: (db, oldVersion, newVersion) {
if (oldVersion < newVersion) {
db.execute(“ALTER TABLE users ADD COLUMN email TEXT”);
}
},
version: 2,
);
45. How do you use the hive package for storing structured data in Flutter?
Answer:
Use hive to store structured data locally with a NoSQL-like API, ideal for key-value storage and offline access.
var box = await Hive.openBox(‘myBox’);
box.put(‘name’, ‘John Doe’);
print(‘Name: ${box.get(‘name’)}’);
46. How do you optimize widget rebuilds in Flutter?
Answer:
Use const constructors, avoid unnecessary setState calls, and use ValueListenableBuilder or StreamBuilder to minimize rebuilds.
47. How do you optimize Flutter app performance for smooth scrolling in a ListView?
Answer:
Use ListView.builder to efficiently build large lists, and consider using CachedNetworkImage for optimized image loading.
ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(‘Item $index’),
);
},
);
48. How do you reduce the size of your Flutter app?
Answer:
Use tree shaking, remove unused resources, enable code shrinking, and split the APK or IPA for different target architectures.
49. How do you handle large images and optimize their loading in Flutter?
Answer:
Use cached_network_image or flutter_advanced_networkimage to cache and optimize image loading, and consider image resizing before loading.
CachedNetworkImage(
imageUrl: “https://via.placeholder.com/150”,
placeholder: (context, url) => CircularProgressIndicator(),
errorWidget: (context, url, error) => Icon(Icons.error),
);
50. How do you profile a Flutter application to identify performance bottlenecks?
Answer:
Use the Flutter DevTools suite to profile your app, monitor frame rendering, analyze memory usage, and identify performance issues.
Final Words
Getting ready for an interview can feel overwhelming, but going through these Flutter fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Flutter interview but don’t forget to practice the Flutter widgets, state management, and responsive design-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for Flutter?
The most common interview questions for Flutter often cover topics like widgets, state management, navigation, and asynchronous programming.
2. What are the important Flutter topics freshers should focus on for interviews?
The important Flutter topics freshers should focus on include understanding basic widgets, handling state with setState and Provider, and implementing responsive designs.
3. How should freshers prepare for Flutter technical interviews?
Freshers should prepare for Flutter technical interviews by building sample projects, practicing common UI challenges, and understanding how to manage the app state effectively.
4. What strategies can freshers use to solve Flutter coding questions during interviews?
Strategies freshers can use include breaking down the UI into smaller widgets, ensuring proper state management, and testing the app on different devices for responsiveness.
5. Should freshers prepare for advanced Flutter topics in interviews?
Yes, freshers should prepare for advanced Flutter topics like animations, custom widgets, and integration with backend services if the role demands in-depth Flutter knowledge.
Explore More Flutter Resources
Explore More Interview Questions
Related Posts
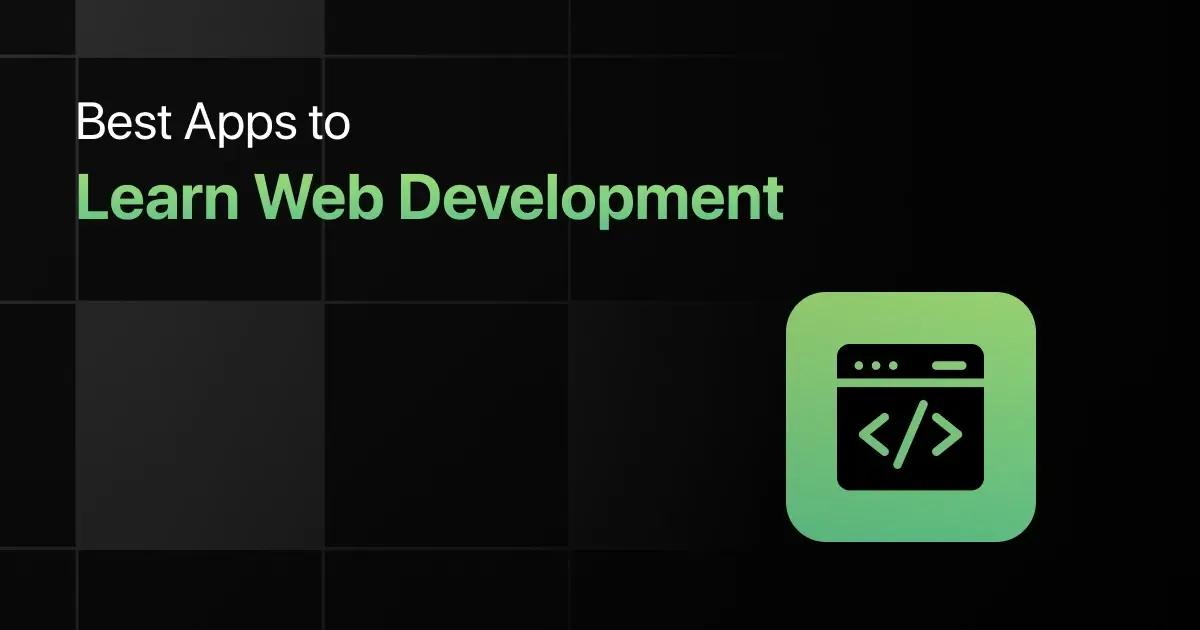
Best Apps to Learn Web Development
Ever thought about building your own website or launching a career in tech but don’t know where to start? With the …