Top Flask Interview Questions for Freshers
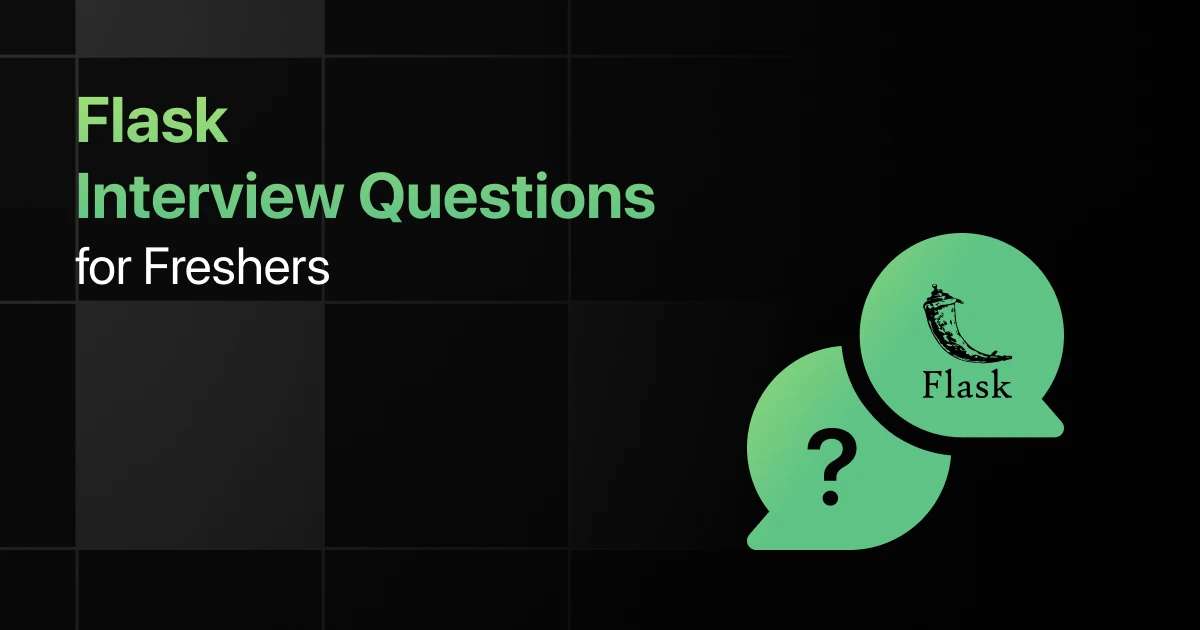
Are you preparing for your first Flask interview and wondering what questions you might face?
Understanding the key Flask interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Flask interview questions and answers for freshers and make a strong impression in your interview.
Practice Flask Interview Questions and Answers
Below are the top 50 Flask interview questions for freshers with answers:
1. What is Flask and why is it called a microframework?
Answer:
Flask is a lightweight web framework in Python that provides essential features to build web applications. It’s called a micro framework because it doesn’t require any particular tools or libraries beyond what is part of the core of Flask.
from flask import Flask
app = Flask(__name__)
2. How do you create a basic route in Flask?
Answer:
A basic route in Flask can be created using the @app.route() decorator, which maps a URL to a function that handles requests to that URL.
@app.route(‘/’)
def home():
return “Hello, Flask!”
3. What are HTTP methods in Flask?
Answer:
HTTP methods like GET, POST, PUT, DELETE, etc., are used in Flask to define how data is sent and retrieved from the server. You specify the methods in the @app.route() decorator.
@app.route(‘/submit’, methods=[‘POST’])
4. How do you pass parameters to routes in Flask?
Answer:
URL parameters can be passed to Flask routes using angle brackets <> in the route. The parameters are then available in the view function.
@app.route(‘/user/<username>’)
def show_user(username):
return f”User: {username}”
5. What is the difference between GET and POST methods in Flask?
Answer:
GET retrieves data from the server, while POST sends data to the server. GET requests appear in the URL, while POST hides data in the body of the request.
6. How do you handle form data in Flask?
Answer:
Form data in Flask is accessed using request.form when a POST request is sent from a form.
from flask import request
@app.route(‘/submit’, methods=[‘POST’])
def submit():
name = request.form[‘name’]
return f”Name: {name}”
7. How do you redirect users to another route in Flask?
Answer:
Flask provides the redirect() function to redirect users to another route. It is typically combined with url_for() to generate dynamic URLs.
from flask import redirect, url_for
@app.route(‘/go-home’)
def go_home():
return redirect(url_for(‘home’))
8. How do you handle JSON data in Flask?
Answer:
Flask allows you to handle JSON data using request.json for incoming data and jsonify() for sending JSON responses.
from flask import jsonify
@app.route(‘/data’)
def data():
return jsonify({“name”: “Flask”, “version”: 1.1})
9. What are templates in Flask and how do you render them?
Answer:
Templates in Flask are used to create dynamic HTML pages. Flask uses Jinja2 as its template engine, and templates are rendered using render_template().
from flask import render_template
@app.route(‘/hello/<name>’)
def hello(name):
return render_template(‘hello.html’, name=name)
10. How do you manage static files in Flask?
Answer:
Flask automatically serves static files from a folder named static. You can refer to them in your templates using the url_for() function.
<img src=”{{ url_for(‘static’, filename=’image.jpg’) }}”>
11. What are sessions in Flask, and how do you use them?
Answer:
Sessions in Flask allow you to store information across requests. Session data is stored on the server and signed with a secret key for security.
from flask import session
session[‘user’] = ‘Alice’
12. How do you use blueprints in Flask?
Answer:
Blueprints allow you to organize your Flask app into reusable modules. They help in structuring larger Flask applications.
from flask import Blueprint
admin = Blueprint(‘admin’, __name__)
13. What is Flask-RESTful, and how do you create a simple API using it?
Answer:
Flask-RESTful is an extension that simplifies the creation of REST APIs. Resources are created as classes, and methods like GET and POST are defined.
from flask_restful import Resource
class HelloWorld(Resource):
def get(self):
return {‘hello’: ‘world’}
14. How do you configure a Flask app?
Answer:
Configuration settings are added using app.config[]. You can load configurations from a file or environment variables.
app.config[‘DEBUG’] = True
15. How do you handle file uploads in Flask?
Answer:
Flask uses request.files to handle file uploads, and you can use the secure_filename() function to ensure file safety.
from werkzeug.utils import secure_filename
file = request.files[‘file’]
file.save(secure_filename(file.filename))
16. What is Flask-SQLAlchemy?
Answer:
Flask-SQLAlchemy is an extension that integrates SQLAlchemy ORM into Flask applications, simplifying database management with models and queries.
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy(app)
17. How do you create a database model using Flask-SQLAlchemy?
Answer:
You create a model by subclassing db.Model and defining columns using db.Column().
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True)
18. How do you perform database migrations in Flask?
Answer:
Flask-Migrate is used to handle database migrations, which allows you to update your database schema incrementally.
19. How do you create a REST API with Flask?
Answer:
You can create a REST API by defining routes with HTTP methods (GET, POST, etc.) and using jsonify() to return responses.
@app.route(‘/api/data’, methods=[‘GET’])
def get_data():
return jsonify({“key”: “value”})
20. How do you secure a Flask app with HTTPS?
Answer:
You can secure a Flask app with HTTPS by configuring an SSL certificate with your web server (like Nginx or Apache) or using ssl_context when running Flask in development.
21. How do you implement authentication in Flask?
Answer:
You can implement authentication using Flask extensions like Flask-Login, which manages user sessions and authentication flows.
22. What is Flask-Caching, and how do you use it?
Answer:
Flask-Caching provides a simple way to cache responses and improve performance in your application by storing data temporarily.
from flask_caching import Cache
cache = Cache(app)
23. How do you handle 404 errors in Flask?
Answer:
You can create custom error pages for 404 errors by using @app.errorhandler(404) decorator.
@app.errorhandler(404)
def page_not_found(e):
return “Page Not Found”, 404
24. What is the purpose of middleware in Flask?
Answer:
Middleware processes requests before they reach the view or responses before they are sent to the client, adding functionality like logging or authentication.
25. How do you serve large files in Flask?
Answer:
Flask provides send_file() and send_from_directory() functions for serving large files efficiently.
26. What are Flask’s request hooks?
Answer:
Request hooks are functions that run before or after the request processing. These include before_request, after_request, teardown_request.
27. How do you handle cross-origin requests (CORS) in Flask?
Answer:
Flask uses Flask-CORS to handle cross-origin requests by enabling specific domains or headers to access your resources.
28. What is the g object in Flask?
Answer:
The g object is used to store and share data across different functions during the lifetime of a request.
29. How do you implement role-based access control (RBAC) in Flask?
Answer:
Role-based access control can be implemented using Flask extensions like Flask-Principal or by manually checking user roles in routes.
30. How do you set and get cookies in Flask?
Answer:
Flask provides set_cookie() to set cookies and request.cookies to retrieve them.
31. What is a WSGI server, and why is it important in Flask?
Answer:
WSGI (Web Server Gateway Interface) is the standard interface between web servers and Python web applications like Flask, enabling deployment with production servers like Gunicorn.
32. How do you deploy a Flask application?
Answer:
Flask apps can be deployed using platforms like Heroku, AWS, or Docker, usually combined with WSGI servers like Gunicorn or uWSGI.
33. How do you log errors in Flask?
Answer:
Flask uses Python’s built-in logging module to log errors. You can configure different logging levels like ERROR, WARNING, etc.
34. How do you create a background task in Flask?
Answer:
Background tasks can be handled using task queues like Celery, which runs tasks asynchronously outside the request-response cycle.
35. How do you enable CSRF protection in Flask?
Answer:
Flask-WTF provides CSRF protection for Flask forms, ensuring that forms are submitted from trusted sources.
36. How do you run a scheduled task in Flask?
Answer:
Flask-APScheduler can be used to run scheduled tasks in a Flask application, allowing for cron-like jobs.
37. What are context processors in Flask?
Answer:
Context processors run before rendering a template and make certain variables globally available to all templates.
38. How do you manage background processes in Flask?
Answer:
Background processes can be managed using Celery for asynchronous tasks or threading for simple concurrency.
39. How do you secure an API in Flask?
Answer:
APIs in Flask can be secured using authentication mechanisms like OAuth, JWT (JSON Web Tokens), or API key-based authentication.
40. How do you handle internationalization in Flask?
Answer:
Flask-Babel is used for internationalization and localization in Flask, allowing you to manage multiple languages in your app.
41. What is the difference between Flask and Django?
Answer:
Flask is a microframework, offering flexibility and simplicity, while Django is a full-stack framework with more built-in features like an admin panel, ORM, and authentication.
42. How do you implement email functionality in Flask?
Answer:
Flask-Mail is an extension used to send emails from a Flask application, enabling SMTP support and HTML emails.
43. How do you create a custom CLI command in Flask?
Answer:
You can create custom CLI commands using Flask-Script or the built-in click module to extend the Flask command-line interface.
44. How do you implement websockets in Flask?
Answer:
Flask-SocketIO is used to implement WebSockets, enabling real-time communication between the client and server.
45. How do you handle large file uploads in Flask?
Answer:
You can handle large file uploads by configuring the maximum request size with app.config[‘MAX_CONTENT_LENGTH’].
46. What is the abort() function in Flask?
Answer:
The abort() function is used to stop request processing and return a specific HTTP error code, such as 404 or 403.
47. How do you throttle API requests in Flask?
Answer:
You can throttle API requests using Flask-Limiter, which provides rate-limiting functionalities for Flask applications.
48. How do you generate API documentation in Flask?
Answer:
You can generate API documentation using Swagger and Flask-RESTPlus, which provide a user-friendly interface for documenting REST APIs.
49. How do you integrate Flask with a front-end framework like React or Vue.js?
Answer:
Flask can serve a REST API that a front-end framework like React or Vue.js can consume, usually communicating via HTTP requests to fetch and display data.
50. What are middleware components in Flask, and how do you use them?
Answer:
Middleware components sit between the server and the Flask application, processing requests before they reach the app. You can implement custom middleware to log requests or add custom headers.
Final Words
Getting ready for an interview can feel overwhelming, but going through these Flask fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Flask interview but don’t forget to practice Flask routing, templates, and request handling-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for Flask?
The most common Flask interview questions revolve around routing, handling HTTP requests, and integrating databases with Flask.
2. What are the important Flask topics freshers should focus on for interviews?
Freshers should focus on Flask topics like request handling, templates, sessions, and Flask extensions.
3. How should freshers prepare for Flask technical interviews?
Freshers should prepare by building simple Flask applications, practicing API development, and understanding how to manage templates and routes.
4. What strategies can freshers use to solve Flask coding questions during interviews?
Freshers can solve Flask coding questions by focusing on designing routes logically, efficiently handling HTTP methods, and ensuring proper data validation.
5. Should freshers prepare for advanced Flask topics in interviews?
Yes, freshers should prepare for advanced Flask topics like authentication, using Flask extensions, and optimizing Flask for production environments.
Explore More Flask Resources
Explore More Interview Questions
- Python
- Java
- SQL
- React
- JavaScript
- C Programming
- HTML
- CSS
- Angular
- C++
- Spring Boot
- Node JS
- Excel
- C#
- DBMS
- PHP
- Linux
- Operating System
- MySQL
- Spring
- Flutter
- MongoDB
- Django
- React Native
- jQuery
- Bootstrap
- Embedded C
- DSA
- R Programming
- Hadoop
- .NET
- Power BI
- ASP.NET
- ASP.NET MVC
- Android
- Tableau
- MVC
- WordPress
- TypeScript
- Spark
- Kotlin
- Swift
- Golang
- Shell Scripting
- iOS
- Spring MVC
- Next JS
- AWS
- Kubernetes
- Docker
- Cyber Security
- Azure
- Terraform
- Vue JS
- UiPath
- Scala
Related Posts
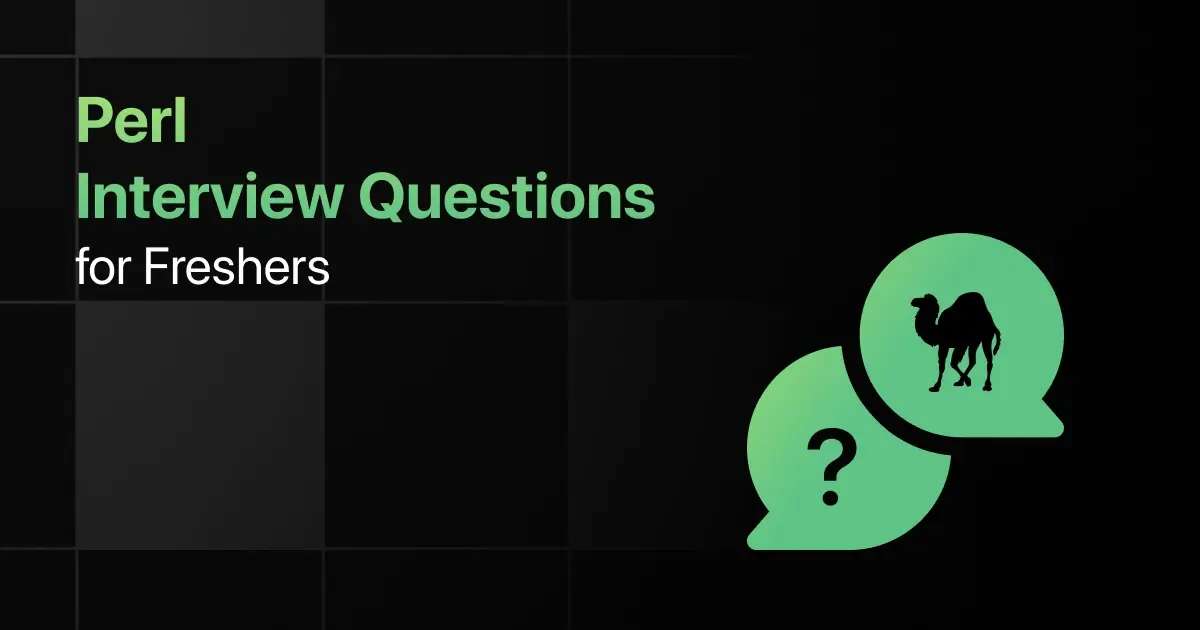
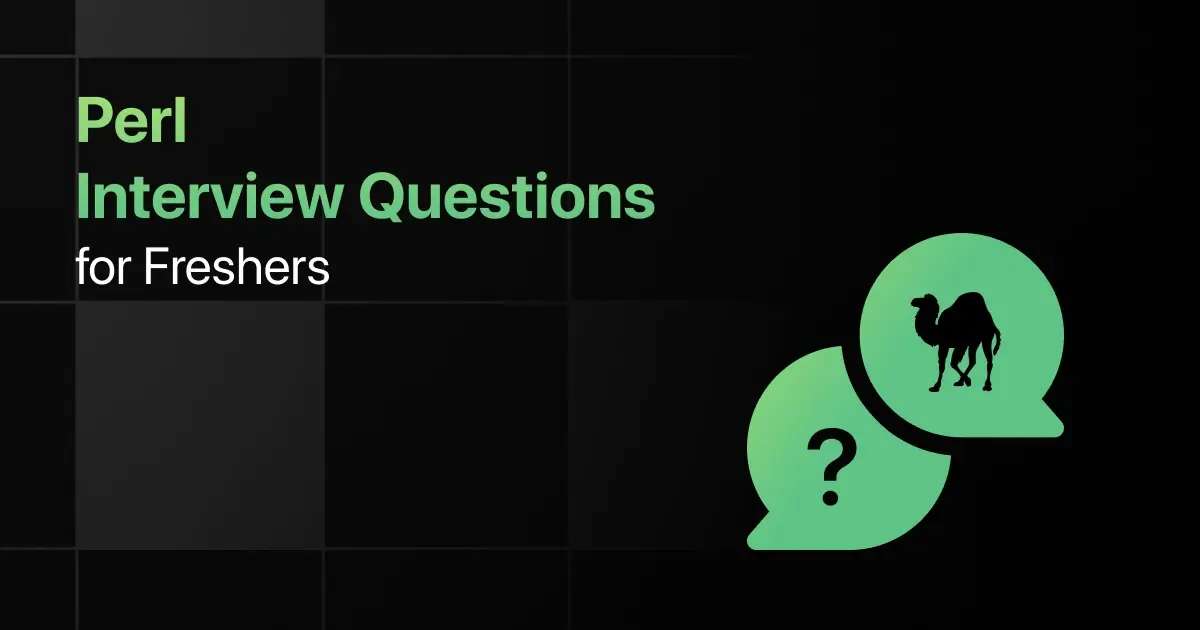
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …