Top Embedded C Interview Questions for Freshers
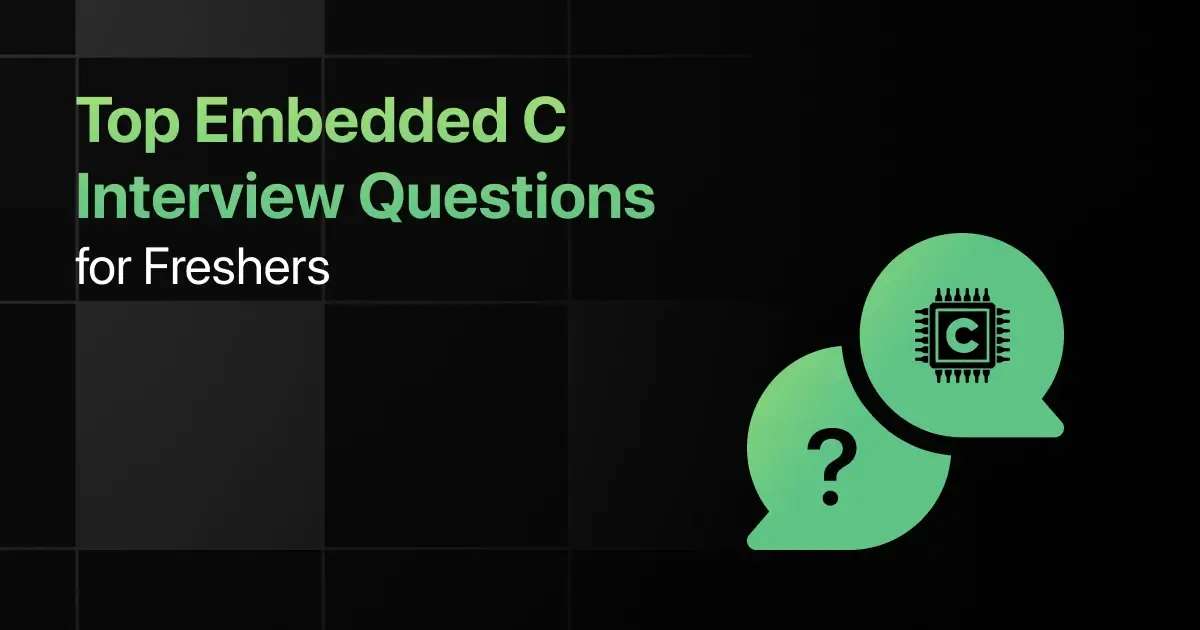
Are you preparing for your first Embedded C interview and wondering what questions you might face?
Understanding the key Embedded C interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Embedded C interview questions and answers for freshers and make a strong impression in your interview.
Practice Embedded C Interview Questions and Answers
Below are the top 50 Embedded C interview questions for freshers with answers:
1. What is Embedded C, and how does it differ from standard C?
Answer:
Embedded C is an extension of the C programming language used for programming embedded systems. It has hardware-specific optimizations, access to hardware registers, and low-level operations that are not typical in standard C programming.
#include <avr/io.h> // Example of hardware-specific headers
2. How do you set up an environment for writing Embedded C code?
Answer:
Set up a development environment by installing a compiler, IDE (such as Keil, MPLAB), and necessary hardware-specific libraries or drivers.
// Using Keil IDE for ARM microcontroller programming
#include “LPC17xx.h”
3. What are the common data types used in Embedded C?
Answer:
Common data types include int, char, float, and long, but specific microcontrollers may have hardware-specific types like uint8_t, uint16_t for unsigned 8-bit and 16-bit values.
uint8_t sensorValue = 0xFF; // Example of 8-bit unsigned integer
4. What is the difference between volatile and non-volatile variables in Embedded C?
Answer:
A volatile variable tells the compiler that its value can change unexpectedly, preventing the compiler from optimizing its value. It’s often used with hardware registers or global variables accessed by interrupt service routines.
volatile uint8_t *port = (uint8_t *)0x20; // Example of volatile register access
5. Why is memory management important in Embedded C?
Answer:
Memory management is critical due to limited resources in embedded systems. Efficient use of RAM and ROM is important, and dynamic memory allocation (malloc, free) is often avoided to prevent fragmentation.
6. What are the differences between RISC and CISC architectures in microcontrollers?
Answer:
RISC (Reduced Instruction Set Computing) has a smaller set of simpler instructions, while CISC (Complex Instruction Set Computing) has a larger set of more complex instructions. RISC architectures are more power-efficient, and often used in embedded systems.
7. How do you interface with an I/O port on a microcontroller in Embedded C?
Answer:
Interfacing with I/O ports involves reading from and writing to specific hardware registers that control the I/O pins.
DDRB = 0xFF; // Set PORTB as output
PORTB = 0x01; // Set pin 0 of PORTB to HIGH
8. What is the purpose of pull-up and pull-down resistors in microcontroller circuits?
Answer:
Pull-up and pull-down resistors are used to ensure a defined logic level (HIGH or LOW) on input pins to avoid floating states when no signal is connected.
9. How do you configure a GPIO pin as an input or output in Embedded C?
Answer:
Set the appropriate Data Direction Register (DDR) to configure the pin as input or output.
DDRB |= (1 << DDB0); // Set PORTB pin 0 as output
DDRB &= ~(1 << DDB1); // Set PORTB pin 1 as input
10. How do you implement software debouncing for a button press in Embedded C?
Answer:
Software debouncing is implemented by introducing a small delay after detecting a button press to ensure that only one press is registered.
if (button_pressed) {
_delay_ms(50); // Delay for debouncing
if (button_pressed) {
// Confirmed button press
}
}
11. What is an interrupt, and how is it used in Embedded C programming?
Answer:
An interrupt is a signal that temporarily halts the current execution flow and jumps to an interrupt service routine (ISR). It is used to handle events like timers, I/O, or communication without constantly polling.
ISR(INT0_vect) {
// Interrupt Service Routine for external interrupt 0
}
12. How do you enable and disable interrupts in Embedded C?
Answer:
Interrupts are typically enabled using specific bits in control registers, and they can be disabled globally or individually.
sei(); // Enable global interrupts
cli(); // Disable global interrupts
13. What is the difference between hardware and software interrupts?
Answer:
Hardware interrupts are triggered by external devices or peripherals, while software interrupts are triggered by the software or CPU instructions.
14. How do you prioritize interrupts in a microcontroller?
Answer:
Interrupt priority is typically set using priority registers provided by the microcontroller. Higher-priority interrupts preempt lower-priority ones.
15. What is the function of an ISR, and how is it different from a normal function?
Answer:
An ISR is a function triggered by an interrupt and executed immediately, interrupting the normal program flow. It cannot take parameters or return values and should execute quickly to avoid blocking other interrupts.
16. How do you configure a timer in Embedded C?
Answer:
Timers are configured by setting control registers for mode, prescaler, and counter value.
TCCR0A = (1 << WGM01); // Set Timer0 to CTC mode
OCR0A = 156; // Set compare value
17. What is the difference between a timer and a counter in Embedded C?
Answer:
A timer counts internal clock pulses, while a counter counts external events on a specific pin.
18. How do you generate a time delay using timers in Embedded C?
Answer:
Use a timer in conjunction with interrupts or polling to generate time delays.
while (!(TIFR0 & (1 << OCF0A))); // Wait until timer flag is set
TIFR0 |= (1 << OCF0A); // Clear the flag
19. How do you implement PWM (Pulse Width Modulation) using timers in Embedded C?
Answer:
PWM is implemented by configuring the timer in PWM mode and setting the duty cycle using the compare register.
TCCR1A |= (1 << COM1A1) | (1 << WGM11); // Configure Timer1 for PWM
OCR1A = 128; // Set duty cycle to 50%
20. What is the function of a prescaler in a timer?
Answer:
A prescaler divides the clock frequency, allowing the timer to count more slowly and generate longer delays.
21: How do you configure UART in Embedded C for serial communication?
Answer:
Configure the baud rate, data format, and enable the transmitter and receiver using the control registers.
UBRR0 = 51; // Set baud rate for 9600 bps
UCSR0B |= (1 << TXEN0) | (1 << RXEN0); // Enable TX and RX
22: How do you send and receive data over UART in Embedded C?
Answer:
Data is sent by writing to the data register and received by reading from the data register when a flag is set.
// Send data
while (!(UCSR0A & (1 << UDRE0))); // Wait for transmit buffer to be empty
UDR0 = data; // Load data into the register
// Receive data
while (!(UCSR0A & (1 << RXC0))); // Wait for data to be received
char receivedData = UDR0; // Read received data
23. What is the difference between synchronous and asynchronous serial communication?
Answer:
Synchronous communication uses a clock signal to synchronize data transmission, while asynchronous communication does not use a clock and instead relies on start/stop bits.
24. How do you implement SPI communication in Embedded C?
Answer:
SPI is configured using control registers to set the master/slave mode, clock polarity, and clock phase. Data is transmitted and received by writing and reading the data register.
SPCR |= (1 << MSTR) | (1 << SPE); // Set as master and enable SPI
SPDR = data; // Load data to transmit
while (!(SPSR & (1 << SPIF))); // Wait for transmission to complete
25. What is the role of the baud rate in serial communication?
Answer:
The baud rate defines the speed of data transmission in bits per second (bps). It must be set the same on both the transmitter and receiver.
26. What are the types of memory used in embedded systems?
Answer:
Embedded systems typically use ROM (for storing code), RAM (for storing data), and Flash memory (for persistent storage).
27. How do you manage stack and heap memory in Embedded C?
Answer:
The stack is used for local variables and function calls, while the heap is used for dynamic memory allocation (malloc, free). Proper management is crucial to avoid stack overflow and memory leaks.
28. What is the difference between static and dynamic memory allocation?
Answer:
Static memory is allocated at compile time and remains fixed, while dynamic memory is allocated at runtime using functions like malloc().
29. Why is dynamic memory allocation avoided in embedded systems?
Answer:
Dynamic memory allocation is avoided due to fragmentation and unpredictable behavior in systems with limited memory resources.
30. How do you initialize global variables in Embedded C?
Answer:
Global variables can be initialized at the time of declaration. They are stored in the data segment if initialized and in the BSS segment if uninitialized.
int globalVar = 10; // Initialized global variable
int anotherVar; // Uninitialized global variable
31. What is an RTOS, and why is it used in embedded systems?
Answer:
An RTOS (Real-Time Operating System) is used in systems that require real-time responses. It handles task scheduling, inter-task communication, and timing constraints.
32. How do you create a task in an RTOS using Embedded C?
Answer:
Tasks in RTOS are created using functions like xTaskCreate() in FreeRTOS. Each task is given a priority and stack space.
xTaskCreate(vTaskFunction, “TaskName”, 100, NULL, 1, NULL);
33. What is the difference between preemptive and cooperative multitasking in RTOS?
Answer:
In preemptive multitasking, the RTOS can interrupt tasks to switch between them, while in cooperative multitasking, tasks must yield control voluntarily.
34. How do you implement task synchronization in Embedded C using an RTOS?
Answer:
Task synchronization is achieved using mechanisms like semaphores, mutexes, or message queues to prevent race conditions.
xSemaphoreTake(mySemaphore, portMAX_DELAY);
35: How do you handle task priority in an RTOS?
Answer:
Tasks are assigned priorities during creation, and the RTOS scheduler runs the highest-priority ready task. Priority inversion can be handled using priority inheritance.
36. What are the techniques for reducing power consumption in embedded systems?
Answer:
Techniques include using low-power modes, reducing clock speeds, and minimizing active components through power gating.
37. How do you put a microcontroller into sleep mode in Embedded C?
Answer:
Microcontrollers typically have specific registers to configure and enter different sleep modes to save power.
set_sleep_mode(SLEEP_MODE_PWR_DOWN);
sleep_enable();
sleep_cpu();
38. What is the role of wake-up sources in low-power design?
Answer:
Wake-up sources like external interrupts or timers bring the microcontroller out of sleep mode when an event occurs.
39. How do you implement a watchdog timer in Embedded C?
Answer:
A watchdog timer is implemented by configuring a timer that resets the system if it is not periodically cleared.
WDTCSR |= (1 << WDE); // Enable watchdog timer
40. What is dynamic voltage and frequency scaling (DVFS)?
Answer:
DVFS is a technique that adjusts the voltage and frequency of a processor based on workload, reducing power consumption during low-demand periods.
41. How do you debug an embedded system using Embedded C?
Answer:
Debugging is done using tools like in-circuit emulators (ICE), JTAG, or using software tools like GDB for source-level debugging.
42. How do you use assertions in Embedded C for debugging?
Answer:
Assertions are used to check conditions during runtime. If a condition fails, the program halts or logs an error for debugging.
#include <assert.h>
assert(x != NULL);
43. What is the role of boundary scan in debugging embedded systems?
Answer:
Boundary scan is a method of testing interconnections on a PCB using a shift register-based test access port (TAP). It’s often used for testing embedded hardware.
44. How do you use printf() for debugging in Embedded C?
Answer:
The printf() function can be used to output debug messages through UART or other communication interfaces.
printf(“Value of variable: %d\n”, myVariable);
45. What are the challenges of debugging real-time embedded systems?
Answer:
Challenges include timing issues, limited observability of internal states, and the inability to pause time-sensitive operations.
46. How do you implement I2C communication in Embedded C?
Answer:
I2C communication is implemented by setting the clock speed, starting a communication sequence, and sending/receiving data using the data register.
TWBR = 0x02; // Set I2C clock speed
TWCR = (1 << TWINT) | (1 << TWSTA) | (1 << TWEN); // Send START condition
47. What is the difference between I2C and SPI communication?
Answer:
I2C is a multi-master, multi-slave protocol with two wires (SDA, SCL), while SPI is a faster protocol with four wires (MISO, MOSI, SCK, SS) and is typically used for short-distance, high-speed communication.
48. How do you implement CAN (Controller Area Network) in Embedded C?
Answer:
CAN is implemented by configuring the CAN control registers for baud rate and frame format, and sending/receiving messages through the data register.
49. What is the role of CRC (Cyclic Redundancy Check) in communication protocols?
Answer:
CRC is used to detect errors in transmitted data by calculating a checksum and verifying it at the receiver’s end.
50. How do you handle communication errors like noise and interference in Embedded C?
Answer:
Errors are handled by implementing error-checking mechanisms like CRC, parity bits, or using retransmission protocols like Automatic Repeat Request (ARQ).
Final Words
Getting ready for an interview can feel overwhelming, but going through these Embedded C fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Embedded C interview but don’t forget to practice the Embedded C basics, microcontroller programming, and real-time system-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for embedded C?
Common interview questions for Embedded C include topics like memory management, the use of pointers, interrupt handling, communication protocols (I2C, SPI, UART), and hardware interfacing using GPIOs.
2. What are the important embedded C topics freshers should focus on for interviews?
Freshers should focus on understanding microcontroller programming, the role of registers, timer/counter-programming, interrupts, embedded C syntax, and interfacing with peripherals like ADC, DAC, and sensors.
3. How should freshers prepare for embedded C technical interviews?
Freshers should prepare by practicing low-level programming tasks, understanding the workings of embedded systems, studying data sheets of microcontrollers, and working on real-time projects like sensor interfacing and communication protocols.
4. What strategies can freshers use to solve embedded C coding questions during interviews?
Freshers should break down the problem into hardware-specific tasks, manage memory efficiently, avoid dynamic allocation when possible, use interrupts for asynchronous tasks, and focus on efficient use of GPIO and peripheral registers.
5. Should freshers prepare for advanced embedded C topics in interviews?
Yes, freshers should be familiar with topics like real-time operating systems (RTOS), memory optimization, debugging techniques, and basic knowledge of communication protocols, which can be critical for embedded system development.
Explore More Embedded C Resources
Explore More Interview Questions
Related Posts
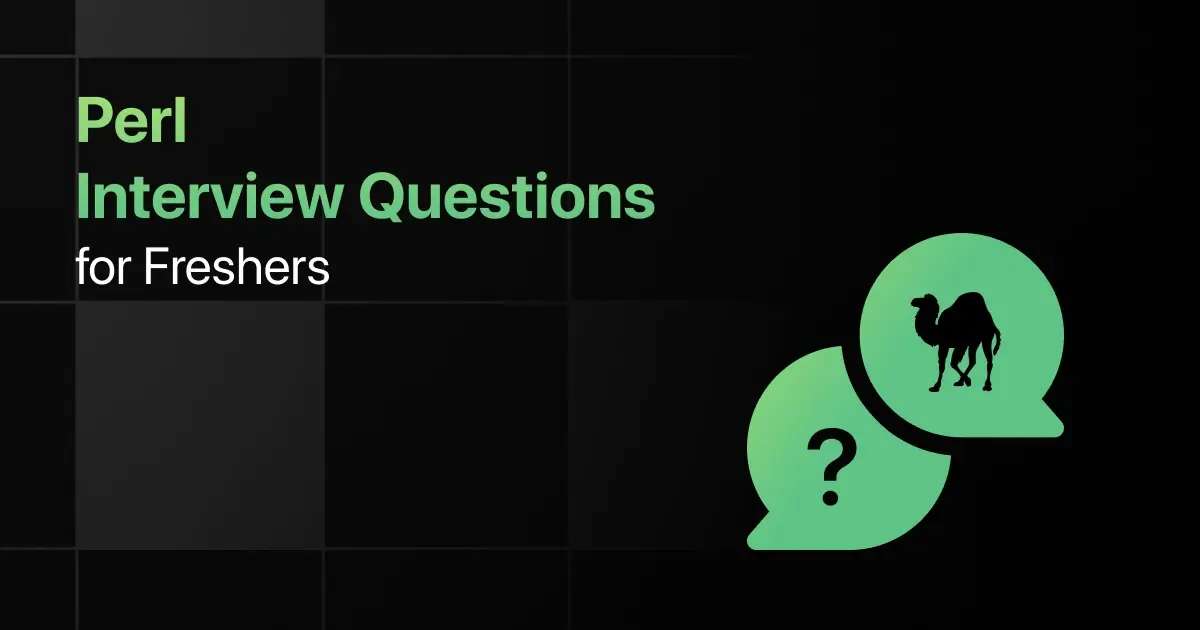
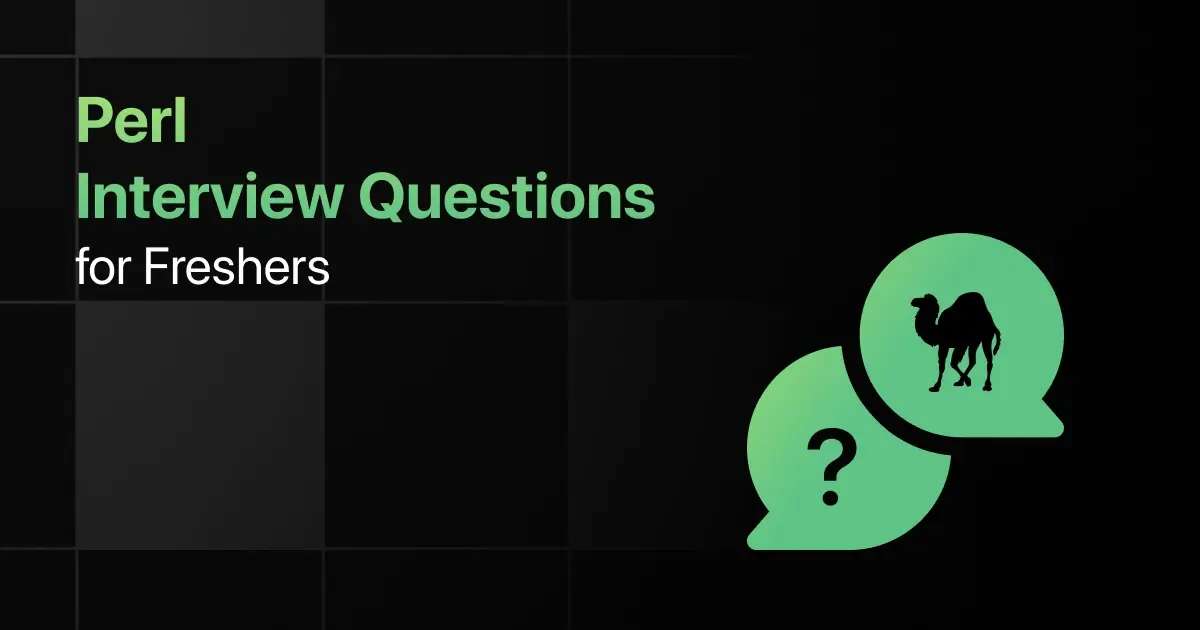
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …