Top .NET Interview Questions for Freshers
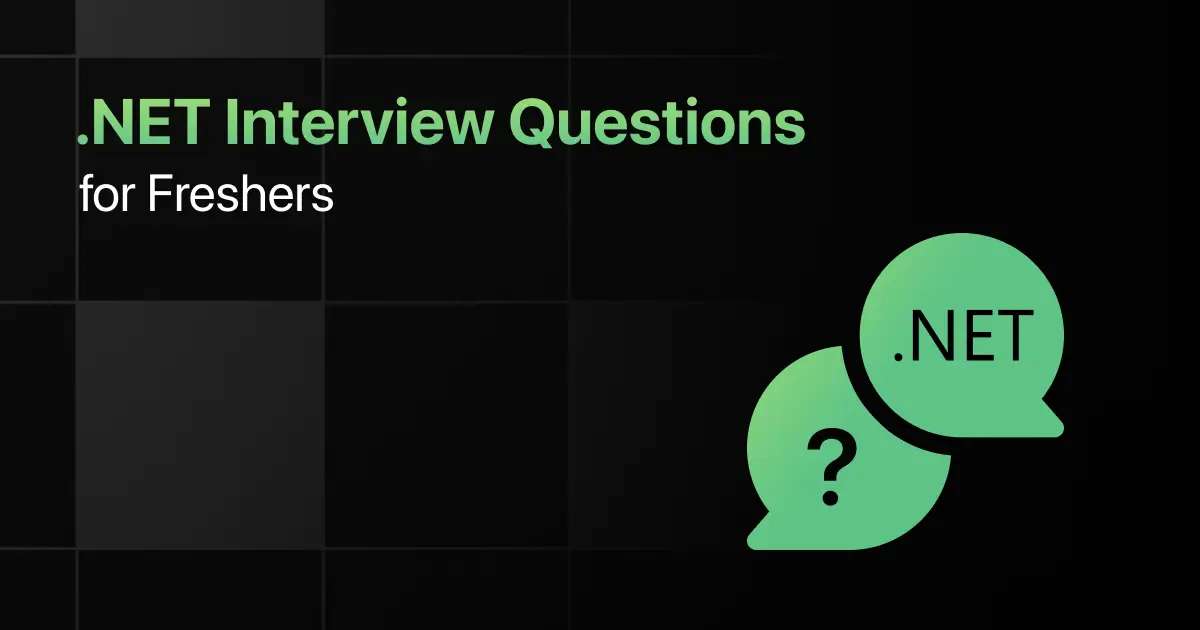
Are you preparing for your first .NET interview and wondering what questions you might face?
Understanding the key .NET interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these .NET interview questions and answers for freshers and make a strong impression in your interview.
Practice .NET Interview Questions and Answers
Below are the top 50 .NET interview questions for freshers with answers:
1. What is .NET Framework?
Answer:
.NET Framework is a software development framework created by Microsoft for building and running applications on Windows. It provides a consistent object-oriented programming environment with a rich set of libraries for building desktop, web, and mobile applications.
It supports multiple languages like C#, VB.NET, and F#, and includes the Common Language Runtime (CLR) for memory management, exception handling, and security.
Console.WriteLine(“Hello, .NET Framework!”);
2. What is the CLR in .NET?
Answer:
The Common Language Runtime (CLR) is the runtime environment in the .NET Framework that handles the execution of code. It provides services like memory management, garbage collection, security, and exception handling.
The CLR ensures that .NET applications run efficiently and securely by managing resources and enforcing code safety.
int number = 10;
3. What is the difference between .NET Core and .NET Framework?
Answer:
.NET Framework is designed for building Windows applications, while .NET Core is a cross-platform framework for building modern cloud, IoT, and mobile applications. .NET Core is more modular and supports Linux, macOS, and Windows, unlike .NET Framework which is limited to Windows.
.NET Core offers better performance and scalability for microservices and cloud applications.
dotnet new console
dotnet run
4. What is the purpose of the Garbage Collector (GC) in .NET?
Answer:
The Garbage Collector (GC) in .NET automatically manages memory by freeing up unused objects from the heap. It reduces memory leaks and eliminates the need for developers to manually allocate and deallocate memory.
The GC works in generations (0, 1, and 2) to optimize the collection of short-lived and long-lived objects.
var obj = new MyClass();
5. What are assemblies in .NET?
Answer:
An assembly is the building block of a .NET application. It is a compiled code library used by the CLR for deployment, versioning, and security. Assemblies can be DLLs (Dynamic Link Libraries) or EXE files.
Assemblies contain metadata and IL (Intermediate Language) code, and can be either private or shared (GAC – Global Assembly Cache).
dotnet build
6. What is the difference between managed and unmanaged code?
Answer:
Managed code is executed by the CLR and benefits from features like garbage collection, security, and type safety. Unmanaged code is executed directly by the OS, without CLR intervention, and requires manual memory management.
Examples of unmanaged code include C++ programs or COM components.
int x = 5;
7. Explain the concept of boxing and unboxing in .NET.
Answer:
Boxing is the process of converting a value type to an object type, while unboxing converts an object type back to a value type. Boxing wraps the value inside an object, and unboxing extracts the value from the object.
Boxing and unboxing are expensive operations, as they involve memory allocation and copying.
int x = 10;
object obj = x; // Boxing
int y = (int)obj; // Unboxing
8. What is the difference between an interface and an abstract class in .NET?
Answer:
An interface defines a contract with no implementation, while an abstract class can contain both abstract methods (without implementation) and non-abstract methods (with implementation).
Interfaces support multiple inheritances, whereas a class can only inherit from one abstract class.
public interface IAnimal {
void MakeSound();
}
// Abstract class example
public abstract class Animal {
public abstract void MakeSound();
public void Eat() {
Console.WriteLine(“Eating”);
}
}
9. What is LINQ in .NET?
Answer:
LINQ (Language Integrated Query) is a query syntax in .NET for querying collections, databases, XML, and more. It provides a unified approach to querying different data sources using a consistent syntax.
LINQ allows developers to write queries in C# or VB.NET in a readable and efficient way.
int[] numbers = { 1, 2, 3, 4, 5 };
var evenNumbers = from num in numbers
where num % 2 == 0
select num;
10. What is the role of the JIT compiler in .NET?
Answer:
The Just-In-Time (JIT) compiler in .NET compiles the Intermediate Language (IL) code into machine code just before execution. This allows .NET applications to be platform-independent until runtime, where the IL code is converted to native machine code for the target platform.
The JIT optimizes performance by compiling only the code needed for execution.
Console.WriteLine(“JIT compiling…”);
11. What is ADO.NET in .NET?
Answer:
ADO.NET is a data access technology in .NET for interacting with databases. It provides classes to connect to databases, execute commands, retrieve results, and manage data.
ADO.NET supports connected and disconnected architectures using SqlConnection, SqlCommand, DataSet, and other classes.
using (SqlConnection connection = new SqlConnection(connectionString)) {
connection.Open();
SqlCommand cmd = new SqlCommand(“SELECT * FROM Users”, connection);
SqlDataReader reader = cmd.ExecuteReader();
}
12. What is .NET Standard?
Answer:
.NET Standard is a specification that defines a set of APIs that must be implemented by all .NET platforms (e.g., .NET Core, .NET Framework, Xamarin). It ensures compatibility across different .NET platforms, enabling developers to create libraries that work everywhere.
.NET Standard allows for greater code sharing between different platforms.
public class MyClass {
public string Greet() {
return “Hello, .NET Standard!”;
}
}
13. What are generics in .NET?
Answer:
Generics in .NET allow you to define classes, methods, and interfaces with type parameters. They provide type safety and performance by allowing code reusability without the need for boxing/unboxing.
Generics are used in collections like List<T>, Dictionary<TKey, TValue>, etc.
public class Box<T> {
public T Value { get; set; }
}
14. What is the Global Assembly Cache (GAC) in .NET?
Answer:
The Global Assembly Cache (GAC) is a repository in .NET that stores shared assemblies that can be used by multiple applications. Assemblies in the GAC are versioned, enabling applications to use specific versions of shared libraries.
Assemblies must be strongly named to be added to the GAC.
gacutil -i MyAssembly.dll
15. What is Entity Framework in .NET?
Answer:
Entity Framework (EF) is an Object-Relational Mapper (ORM) that allows developers to interact with databases using .NET objects instead of SQL queries. EF supports LINQ to query the database and simplifies CRUD operations.
Entity Framework makes data access more abstract, allowing developers to work with data as objects and properties.
using (var context = new MyDbContext()) {
var users = context.Users.ToList();
}
16. What is the difference between Task and Thread in .NET?
Answer:
Task represents an asynchronous operation, while Thread represents a separate path of execution. Tasks in .NET are used for managing asynchronous operations and work at a higher level of abstraction than threads.
Tasks make it easier to handle parallelism and are optimized for resource management.
Task.Run(() => Console.WriteLine(“Running asynchronously”));
17. What is the role of async and await in .NET?
Answer:
async and await are used to write asynchronous code in .NET. The async modifier is applied to methods that perform asynchronous operations, and await is used to pause the execution until the awaited task is completed.
Async programming helps prevent blocking the main thread and improves responsiveness in applications.
public async Task<string> FetchDataAsync() {
await Task.Delay(1000);
return “Data fetched”;
}
18. What is the difference between IEnumerable and IQueryable in .NET?
Answer:
IEnumerable is used for iterating over in-memory collections and works well with LINQ-to-Objects. IQueryable is used for querying data sources, like databases, and performs queries on the server-side.
IQueryable provides better performance for large datasets because it generates database-specific queries, while IEnumerable fetches all data into memory before processing.
var query = dbContext.Users.Where(u => u.Age > 30);
19. What is .NET Core, and how is it different from .NET Framework?
Answer:
.NET Core is a cross-platform, open-source framework for building modern applications, whereas .NET Framework is limited to Windows.
.NET Core supports cloud-based, IoT, and microservice architectures and offers better performance and scalability compared to .NET Framework.
.NET Core is modular and lightweight, making it suitable for containerized applications.
dotnet new console
dotnet run
20. What is ASP.NET Core?
Answer:
ASP.NET Core is a cross-platform framework for building modern web applications. It is a modular framework that allows for high-performance and scalable applications. ASP.NET Core unifies MVC and Web API into a single framework.
ASP.NET Core supports dependency injection, middleware, and cross-platform deployment.
public void Configure(IApplicationBuilder app) {
app.UseRouting();
app.UseEndpoints(endpoints => {
endpoints.MapControllers();
});
}
}
21. What is Dependency Injection (DI) in .NET?
Answer:
Dependency Injection (DI) is a design pattern in which dependencies (services) are injected into a class, rather than being created inside the class. This promotes loose coupling and enhances testability and maintainability.
ASP.NET Core has built-in support for DI, allowing you to register services in the Startup.ConfigureServices() method.
public void ConfigureServices(IServiceCollection services) {
services.AddScoped<IMyService, MyService>();
}
}
22. What is the purpose of the appsettings.json file in .NET Core?
Answer:
appsettings.json is used to store configuration settings for a .NET Core application. It contains settings like connection strings, API keys, and environment-specific configurations. The contents are loaded into the application’s configuration at runtime and can be accessed using the IConfiguration interface.
.NET Core supports environment-specific configurations through files like appsettings.Development.json.
“ConnectionStrings”: {
“DefaultConnection”: “Server=localhost;Database=mydb;Trusted_Connection=True;”
}
}
23. What are extension methods in .NET?
Answer:
Extension methods allow you to add new methods to existing types without modifying the original class or creating a new derived type. They are defined as static methods in static classes, and the first parameter of the method specifies the type it extends.
Extension methods improve code readability and maintainability by adding functionality to existing types.
public static class StringExtensions {
public static bool IsNullOrEmpty(this string value) {
return string.IsNullOrEmpty(value);
}
}
24. What is the Task Parallel Library (TPL) in .NET?
Answer:
The Task Parallel Library (TPL) in .NET provides a framework for parallel programming and efficient task management. It simplifies writing concurrent and asynchronous code by using Task objects to represent asynchronous operations.
TPL improves performance by utilizing multi-core processors and simplifying code structure for parallel operations.
Task.Run(() => DoWork());
25. What is MVC in .NET?
Answer:
MVC (Model-View-Controller) is a design pattern used in ASP.NET Core and .NET Framework to separate an application’s concerns. The Model represents the data, the View displays the UI, and the Controller handles user input and interactions.
The MVC pattern promotes modularity and makes applications more maintainable by separating logic, UI, and data access.
public class HomeController : Controller {
public IActionResult Index() {
return View();
}
}
26. What is SignalR in .NET?
Answer:
SignalR is a library in .NET used for adding real-time web functionality to applications. It enables the server to push content to connected clients instantly, supporting features like chat applications, live dashboards, and notifications.
SignalR abstracts communication technologies like WebSockets and Long Polling to provide real-time communication.
public class ChatHub : Hub {
public async Task SendMessage(string user, string message) {
await Clients.All.SendAsync(“ReceiveMessage”, user, message);
}
}
27. What is a delegate in .NET?
Answer:
A delegate in .NET is a type that represents references to methods with a specific signature. Delegates are used to pass methods as arguments to other methods and are the foundation of events and callback methods.
Delegates provide flexibility by allowing methods to be treated as first-class objects.
public delegate void MyDelegate(string message);
public void PrintMessage(string message) {
Console.WriteLine(message);
}
28. What is a lambda expression in .NET?
Answer:
A lambda expression is a concise way to represent anonymous methods in .NET. Lambda expressions are used extensively in LINQ queries, event handling, and asynchronous programming.
They provide a more readable and functional style of writing code, allowing inline definitions of small methods.
Func<int, int, int> add = (a, b) => a + b;
Console.WriteLine(add(5, 3)); // Output: 8
29. What is an event in .NET, and how is it used?
Answer:
An event in .NET is a way for objects to notify other objects when something of interest occurs. Events are based on delegates and follow the publisher-subscriber model, where an event is raised by one object (publisher), and other objects (subscribers) respond to it.
Events are commonly used in GUIs, where user actions like button clicks trigger events.
public class Button {
public event EventHandler Clicked;
public void OnClick() {
Clicked?.Invoke(this, EventArgs.Empty);
}
}
30. What is REST, and how do you implement it in .NET?
Answer:
REST (Representational State Transfer) is an architectural style for building web services that interact using HTTP methods like GET, POST, PUT, DELETE. RESTful services are stateless and use URLs to represent resources.
In .NET, REST APIs are typically implemented using ASP.NET Core or ASP.NET Web API, with methods mapped to HTTP verbs.
[HttpGet(“{id}”)] public IActionResult GetItem(int id) {
var item = _repository.GetItemById(id);
return Ok(item);
}
31. What is async and await in .NET, and why are they important?
Answer:
async and await are used to simplify asynchronous programming in .NET. They allow methods to run asynchronously without blocking the main thread, making the application more responsive, especially for I/O-bound tasks like network requests.
await is used to wait for the completion of an asynchronous operation, while async marks the method that contains asynchronous code.
csharp
Copy code
public async Task<string> GetDataAsync() {
await Task.Delay(1000);
return “Data loaded”;
}
32. What is a struct in .NET?
Answer:
A struct in .NET is a value type used to encapsulate small groups of related variables, such as the coordinates of a point. Unlike classes, structs are stored on the stack and have value semantics, meaning they are copied when passed to functions.
structs are suitable for small data objects and can be used for performance-critical operations.
public struct Point {
public int X;
public int Y;
}
33. What is the difference between const and readonly in .NET?
Answer:
const defines a compile-time constant, while readonly defines a runtime constant that can only be assigned in the constructor. const fields are static and cannot be changed after compilation, while readonly fields can be initialized at runtime.
readonly is often used when the value needs to be constant after object creation but is not known at compile time.
public const int MaxValue = 100;
public readonly int MinValue;
public MyClass(int minValue) {
MinValue = minValue;
}
34. What are attributes in .NET?
Answer:
Attributes in .NET are used to add metadata to code elements such as classes, methods, or properties. Attributes provide additional information to the runtime or compiler, such as indicating obsolete code or requiring special permissions.
Attributes are placed directly above the code they affect and are processed at runtime via reflection.
// Old code
}
35. What is reflection in .NET?
Answer:
Reflection in .NET allows programs to inspect and manipulate their own metadata at runtime. It provides the ability to dynamically discover types, methods, properties, and fields in assemblies. Reflection is often used in frameworks, serialization, and for accessing private members of classes.
Reflection can also be used to create instances of types dynamically.
Type type = typeof(MyClass);
MethodInfo method = type.GetMethod(“MyMethod”);
object result = method.Invoke(myClassInstance, null);
36. What is the difference between finalize and dispose in .NET?
Answer:
Finalize is used for cleanup by the garbage collector and is automatically called when an object is being collected, whereas Dispose is explicitly called to release unmanaged resources before the object is collected.
Dispose is part of the IDisposable interface and provides a deterministic way to clean up resources like file handles or database connections.
public void Dispose() {
// Clean up resources
}
37. What are the key differences between List and Array in .NET?
Answer:
Arrays have a fixed size, while List<T> is a dynamic collection that can grow or shrink as needed. Arrays can store elements of a specific type, whereas List<T> is a generic class that allows for type-safe storage of elements with more flexible operations like Add and Remove.
List<T> also provides more built-in functionality for operations like searching, sorting, and filtering.
List<int> numbers = new List<int> { 1, 2, 3 };
numbers.Add(4);
38. What is a namespace in .NET, and why is it important?
Answer:
A namespace is a way to organize and group related classes, interfaces, enums, and other elements in .NET. It helps prevent naming conflicts by distinguishing between classes with the same name but in different namespaces.
Namespaces provide a logical hierarchy and make code easier to maintain by grouping related functionality together.
namespace MyApplication {
public class MyClass {
public void MyMethod() {
Console.WriteLine(“Hello from MyClass”);
}
}
}
39. What is a sealed class in .NET?
Answer:
A sealed class in .NET cannot be inherited by other classes. It is useful when you want to prevent further derivation, typically for reasons related to security, performance, or design.
Sealing a class ensures that the class cannot be extended, which may enhance stability and prevent accidental overrides.
public sealed class MyClass {
public void DisplayMessage() {
Console.WriteLine(“This is a sealed class”);
}
}
40. What is the IDisposable interface in .NET?
Answer:
The IDisposable interface defines a method Dispose() that is used to release unmanaged resources, such as file handles or database connections, when they are no longer needed.
It provides a standardized way to perform cleanup operations, and objects that use unmanaged resources should implement IDisposable.
public class ResourceHolder : IDisposable {
public void Dispose() {
// Clean up resources
}
}
41. What is asynchronous programming, and how is it implemented in .NET?
Answer:
Asynchronous programming allows for non-blocking execution, meaning tasks can run in the background without stopping the main thread. It is implemented using async and await keywords in .NET.
This is especially useful for I/O-bound operations, such as reading from files or making HTTP requests, as it prevents UI freezing or unnecessary thread blocking.
public async Task<string> GetDataAsync() {
await Task.Delay(1000); // Simulates async work
return “Data fetched”;
}
42. What are properties in .NET?
Answer:
Properties in .NET are members of a class that provide a flexible mechanism to access and modify data fields. They use get and set accessors to encapsulate the fields of a class.
Properties are a safer way to expose class data compared to public fields because you can control the logic in the get or set methods.
public class Person {
private string _name;
public string Name {
get { return _name; }
set { _name = value; }
}
}
43. What is polymorphism in .NET?
Answer:
Polymorphism in .NET is the ability of different classes to be treated as instances of the same base class. It allows one method to have different implementations based on the derived class.
Polymorphism can be achieved through method overriding (runtime polymorphism) and method overloading (compile-time polymorphism).
public class Animal {
public virtual void MakeSound() {
Console.WriteLine(“Animal sound”);
}
}
public class Dog : Animal {
public override void MakeSound() {
Console.WriteLine(“Bark”);
}
}
44. What is an enum in .NET?
Answer:
An enum is a special value type in .NET that defines a set of named constants. Enums are used to represent a collection of related constants, such as days of the week, user roles, or states.
Enums improve code readability by giving meaningful names to numeric values.
public enum DaysOfWeek {
Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday
}
45. What is an exception in .NET, and how is it handled?
Answer:
An exception in .NET is an error that occurs during the execution of a program. Exceptions are handled using try-catch-finally blocks. In the catch block, you can define the logic for dealing with the exception, while the finally block is used for code that must execute whether an exception occurs or not.
Proper exception handling ensures that your application can gracefully handle errors and continue running.
try {
int result = 10 / 0;
} catch (DivideByZeroException ex) {
Console.WriteLine(“Error: ” + ex.Message);
} finally {
Console.WriteLine(“Operation completed”);
}
46. What are the access modifiers in .NET?
Answer:
Access modifiers in .NET define the scope and visibility of classes and their members. The main access modifiers are:
- public: Accessible from anywhere.
- private: Accessible only within the same class.
- protected: Accessible within the same class and by derived classes.
- internal: Accessible only within the same assembly.
- protected internal: Accessible by derived classes or within the same assembly.
public class MyClass {
private int privateVar;
public int publicVar;
}
47. What is the difference between overloading and overriding in .NET?
Answer:
Overloading occurs when two or more methods in the same class have the same name but different parameters (method signatures).
Overriding happens when a derived class provides a specific implementation for a method that is already defined in the base class.
Overloading is a compile-time feature, while overriding is a runtime feature.
public class Calculator {
public int Add(int a, int b) => a + b;
public double Add(double a, double b) => a + b;
}
// Overriding example
public class BaseClass {
public virtual void Display() {
Console.WriteLine(“Base class display”);
}
}
public class DerivedClass : BaseClass {
public override void Display() {
Console.WriteLine(“Derived class display”);
}
}
48. What is the purpose of the default keyword in .NET?
Answer:
The default keyword in .NET is used to assign default values to value types and reference types. For value types like int, default assigns 0, and for reference types, it assigns null.
This keyword is useful in generic programming to handle cases where you don’t know the type at compile-time but still want to initialize it.
T value = default(T); // T is a generic type
49. What is an Indexer in .NET?
Answer:
An indexer allows instances of a class to be indexed like arrays. It lets you define how to access and set values within an object using array-like syntax, improving usability.
Indexers are useful when you want a class to behave like a collection.
public class MyCollection {
private int[] arr = new int[100];
public int this[int index] {
get { return arr[index]; }
set { arr[index] = value; }
}
}
50. What is a Nullable type in .NET?
Answer:
A Nullable type allows value types, such as int or bool, to represent null values. In .NET, the Nullable<T> structure or the shorthand T? is used to allow value types to have null as a valid value.
Nullable types are useful when working with databases or scenarios where a value may not always be present.
int? nullableInt = null;
if (nullableInt.HasValue) {
Console.WriteLine(nullableInt.Value);
}
Final Words
Getting ready for an interview can feel overwhelming, but going through these .NET fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your .NET interview, but don’t forget to practice .NET framework basics, CLR, and OOP principles-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for .NET?
Common .NET interview questions revolve around the .NET framework, CLR (Common Language Runtime), garbage collection, C# programming concepts, exception handling, and object-oriented programming (OOP) principles like inheritance, polymorphism, and encapsulation.
2. What are the important .NET topics freshers should focus on for interviews?
Freshers should focus on core topics such as the .NET framework, CLR, memory management, basic C# syntax, OOP concepts, assemblies, and namespaces. Knowledge of the .NET lifecycle and understanding how ASP.NET and Entity Framework work within the .NET ecosystem is also essential.
3. How should freshers prepare for .NET technical interviews?
Freshers should prepare by revising core C# programming concepts, practicing coding problems, understanding the .NET framework architecture, and building small projects using .NET. Familiarity with Visual Studio, debugging, and version control will also be useful.
4. What strategies can freshers use to solve .NET coding questions during interviews?
Freshers should approach .NET coding questions by understanding the problem clearly, applying appropriate OOP principles, and using common .NET libraries. They should also follow clean coding practices, utilize exception handling, and make use of .NET debugging tools to troubleshoot issues.
5. Should freshers prepare for advanced .NET topics in interviews?
Yes, while the basics are important, freshers may also benefit from understanding advanced .NET topics such as asynchronous programming (async/await), LINQ, delegates, events, and Entity Framework for database interaction, especially for more technical roles.
Explore More .NET Resources
Explore More Interview Questions
Related Posts
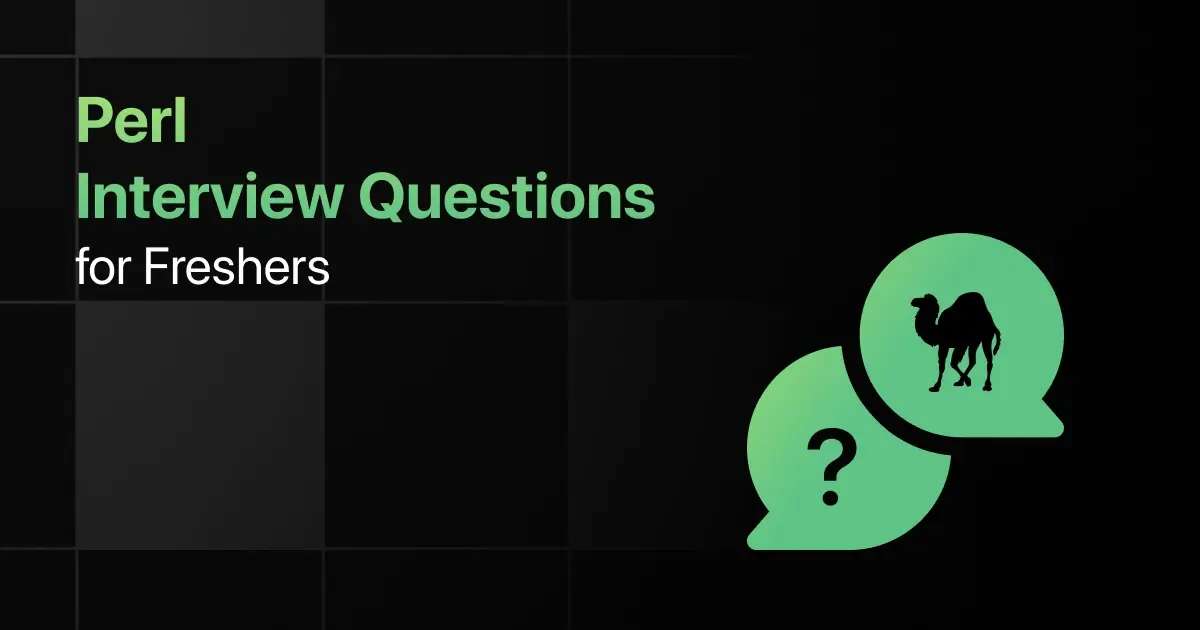
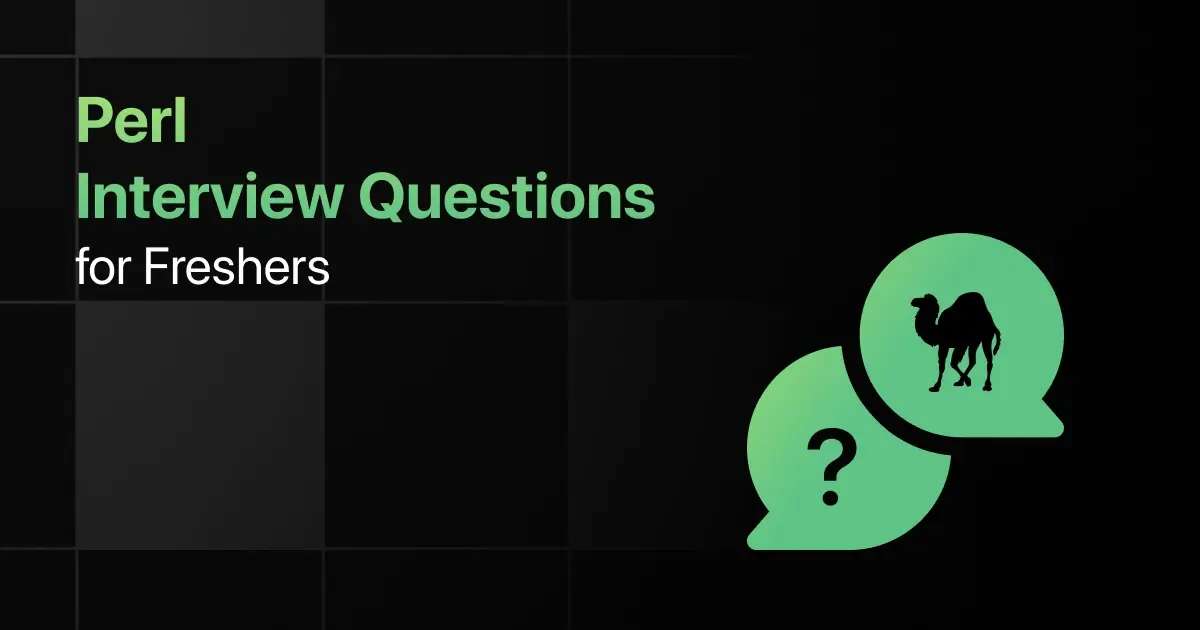
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …