Top Django Interview Questions for Freshers
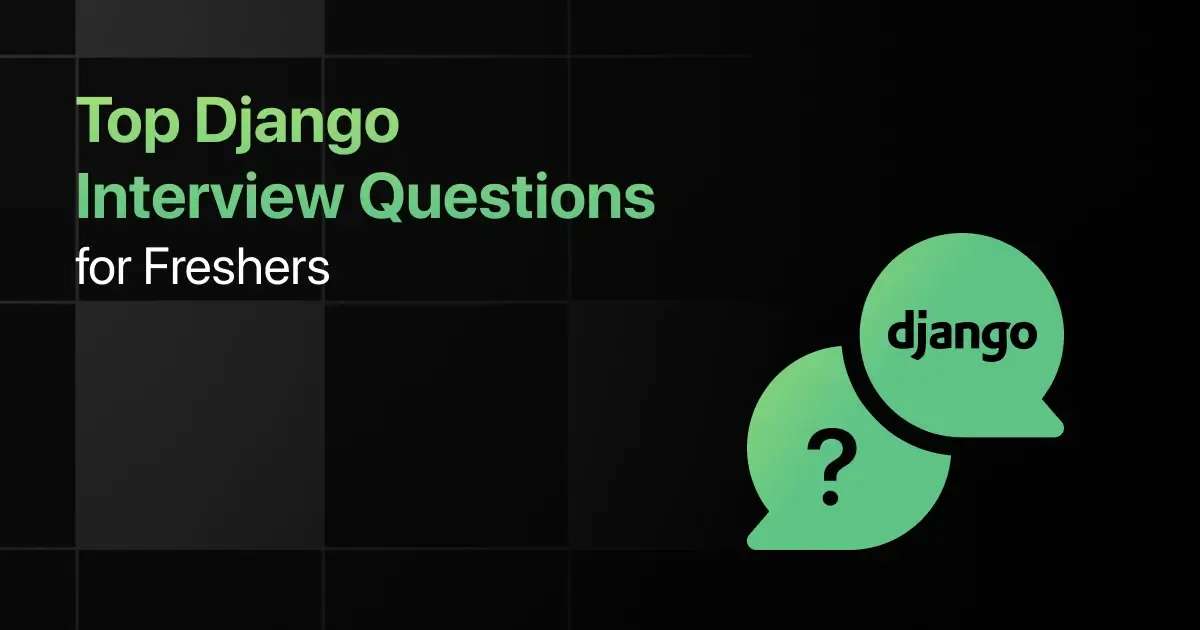
Are you preparing for your first Django interview and wondering what questions you might face? Understanding the key Django interview questions for freshers can give you more clarity.
This blog is here to help you get ready with practical questions that test your real-world problem-solving skills. We’ve gathered some of the most common basic Django interview questions that freshers often encounter.
With this guide, you’ll be well-prepared to tackle these Django interview questions and answers for freshers and make a strong impression in your interview.
Practice Django Interview Questions and Answers
Here are the top 50 Django interview questions for freshers with answers:
1. How do you create a new Django project?
Answer:
Use the django-admin startproject command followed by the project name to create a new Django project.
django-admin startproject myproject
2. How do you start a new Django app within a project?
Answer:
Use the python manage.py startapp command followed by the app name to create a new app within your project.
python manage.py startapp myapp
3. How do you run the Django development server?
Answer:
Use the python manage.py runserver command to start the Django development server.
python manage.py runserver
4. How do you configure your Django app to connect to a database?
Answer:
Modify the DATABASES setting in the settings.py file of your project to include the database engine, name, user, password, host, and port.
DATABASES = {
‘default’: {
‘ENGINE’: ‘django.db.backends.postgresql’,
‘NAME’: ‘mydatabase’,
‘USER’: ‘myuser’,
‘PASSWORD’: ‘mypassword’,
‘HOST’: ‘localhost’,
‘PORT’:
5. How do you create database migrations in Django?
Answer:
Use the python manage.py makemigrations command to create new migrations based on the changes detected in your models.
python manage.py makemigrations
6. How do you define a model in Django?
Answer:
Create a class that inherits from models.Model and define fields as class attributes.
from django.db import models
class Author(models.Model):
name = models.CharField(max_length=100)
age = models.IntegerField()
7. How do you create a one-to-many relationship in Django models?
Answer:
Use a ForeignKey field in one model to reference another model.
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.ForeignKey(Author, on_delete=models.CASCADE)
8. How do you perform a simple query to retrieve all objects of a model?
Answer:
Use the all() method on the model’s manager to retrieve all objects.
authors = Author.objects.all()
9. How do you filter objects in Django based on a condition?
Answer:
Use the filter() method on the model’s manager with keyword arguments representing the filter criteria.
young_authors = Author.objects.filter(age__lt=30)
10. How do you retrieve a single object from a model using a primary key?
Answer:
Use the get() method with the primary key as a keyword argument.
author = Author.objects.get(pk=1)
11. How do you create a simple view that returns an HTTP response?
Answer:
Define a function in your views.py file that takes an HttpRequest object as an argument and returns an HttpResponse.
from django.http import HttpResponse
def index(request):
return HttpResponse(“Hello, world!”)
12. How do you create a view that renders a template?
Answer:
Use the render() function to return an HttpResponse object with the rendered template.
from django.shortcuts import render
def index(request):
return render(request, ‘index.html’)
13. How do you pass context data to a template from a view?
Answer:
Pass a dictionary as the third argument to the render() function, where the keys represent variable names in the template.
def index(request):
context = {‘name’: ‘John’}
return render(request, ‘index.html’, context)
14. How do you handle 404 errors in Django?
Answer:
Use the get_object_or_404() function to retrieve an object or raise a Http404 exception if the object does not exist.
from django.shortcuts import get_object_or_404
def detail(request, pk):
author = get_object_or_404(Author, pk=pk)
return render(request, ‘detail.html’, {‘author’: author})
15. How do you redirect to another view in Django?
Answer:
Use the redirect() function to return an HttpResponseRedirect to a specified URL.
from django.shortcuts import redirect
def my_view(request):
return redirect(‘index’)
16. How do you create a Django form based on a model?
Answer:
Use the ModelForm class to create a form that automatically includes fields from the specified model.
from django import forms
from .models import Author
class AuthorForm(forms.ModelForm):
class Meta:
model = Author
fields = [‘name’, ‘age’]
17. How do you manually validate a form in Django?
Answer:
Call the is_valid() method on the form instance to check if the form data is valid, and use the errors attribute to inspect any validation errors.
def my_view(request):
form = AuthorForm(request.POST)
if form.is_valid():
# Process form data
form.save()
else:
print(form.errors)
18. How do you handle file uploads in Django forms?
Answer:
Use the FileField or ImageField in your form and handle file uploads using request.FILES.
class UploadForm(forms.Form):
file = forms.FileField()
def upload_view(request):
if request.method == ‘POST’:
form = UploadForm(request.POST, request.FILES)
if form.is_valid():
handle_uploaded_file(request.FILES[‘file’])
return HttpResponse(“File uploaded successfully”)
else:
form = UploadForm()
return render(request, ‘upload.html’, {‘form’: form})
19. How do you display form validation errors in a template?
Answer:
Use the {{ form.field_name.errors }} template tag to display validation errors for a specific field.
{% if form.name.errors %}
<ul>
{% for error in form.name.errors %}
<li>{{ error }}</li>
{% endfor %}
</ul>
{% endif %}
20. How do you prepopulate a form with existing data in Django?
Answer:
Pass an instance of the model to the form’s instance parameter when instantiating the form.
author = Author.objects.get(pk=1)
form = AuthorForm(instance=author)
21. How do you use template inheritance in Django?
Answer:
Use the {% extends %} tag to inherit from a base template and the {% block %} tag to define content blocks.
<!– base.html –>
<!DOCTYPE html>
<html>
<head>
<title>{% block title %}My Site{% endblock %}</title>
</head>
<body>
{% block content %}{% endblock %}
</body>
</html>
<!– index.html –>
{% extends “base.html” %}
{% block title %}Home{% endblock %}
{% block content %}
<h1>Welcome to the Home Page</h1>
22. How do you include one template inside another in Django?
Answer:
Use the {% include %} tag to include another template within the current template.
{% include “header.html” %}
23. How do you loop through a list in a Django template?
Answer:
Use the {% for %} tag to iterate over a list and display each item.
<ul>
{% for author in authors %}
<li>{{ author.name }}</li>
{% endfor %}
</ul>
24. How do you apply filters to variables in Django templates?
Answer:
Use the | character followed by the filter name to apply filters to variables.
<p>{{ author.name|upper }}</p>
25. How do you display a default value for a variable in a Django template?
Answer:
Use the default filter to provide a fallback value if the variable is not defined.
<p>{{ author.bio|default:”Bio not available” }}</p>
26. How do you create a simple API view using Django REST Framework?
Answer:
Use the APIView class and define get(), post(), put(), and delete() methods to handle requests.
from rest_framework.views import APIView
from rest_framework.response import Response
class HelloWorld(APIView):
def get(self, request):
return Response({“message”: “Hello, world!”})
27. How do you serialize a Django model in Django REST Framework?
Answer:
Create a serializer class that inherits from serializers.ModelSerializer and define the fields to be serialized.
from rest_framework import serializers
from .models import Author
class AuthorSerializer(serializers.ModelSerializer):
class Meta:
model = Author
fields =
28. How do you handle authentication in Django REST Framework?
Answer:
Use built-in authentication classes such as BasicAuthentication, SessionAuthentication, or TokenAuthentication.
from rest_framework.authentication import TokenAuthentication
class MyView(APIView):
authentication_classes = [TokenAuthentication]
…
29. How do you create a viewset in Django REST Framework?
Answer:
Create a viewset class that inherits from ModelViewSet and specify the queryset and serializer class.
from rest_framework import viewsets
from .models import Author
from .serializers import AuthorSerializer
class AuthorViewSet(viewsets.ModelViewSet):
queryset = Author.objects.all()
serializer_class = AuthorSerializer
30. How do you handle pagination in Django REST Framework?
Answer:
Use the built-in pagination classes like PageNumberPagination and configure them in the settings or directly in the view.
from rest_framework.pagination import PageNumberPagination
class MyPagination(PageNumberPagination):
page_size = 10
class AuthorViewSet(viewsets.ModelViewSet):
queryset = Author.objects.all()
serializer_class = AuthorSerializer
pagination_class = MyPagination
31. How do you create a custom user model in Django?
Answer:
Inherit from AbstractBaseUser and PermissionsMixin, and define the required fields and methods.
from django.contrib.auth.models import AbstractBaseUser, BaseUserManager, PermissionsMixin
class CustomUserManager(BaseUserManager):
def create_user(self, email, password=None, **extra_fields):
user = self.model(email=email, **extra_fields)
user.set_password(password)
user.save()
return user
class CustomUser(AbstractBaseUser, PermissionsMixin):
email = models.EmailField(unique=True)
name = models.CharField(max_length=100)
objects = CustomUserManager()
USERNAME_FIELD = ’email’
REQUIRED_FIELDS = [‘name’]
32. How do you implement user registration in Django?
Answer:
Create a form for user registration, validate the input, and save the new user using the custom or default user model.
class RegisterForm(forms.ModelForm):
password = forms.CharField(widget=forms.PasswordInput)
class Meta:
model = CustomUser
fields = [’email’, ‘name’, ‘password’]
def register(request):
if request.method == ‘POST’:
form = RegisterForm(request.POST)
if form.is_valid():
user = form.save(commit=False)
user.set_password(form.cleaned_data[‘password’])
user.save()
return redirect(‘login’)
else:
form = RegisterForm()
return render(request, ‘register.html’,
33. How do you implement login functionality in Django?
Answer:
Use Django’s built-in authenticate() and login() methods to validate the user and create a session.
from django.contrib.auth import authenticate, login
def login_view(request):
if request.method == ‘POST’:
email = request.POST[’email’]
password = request.POST[‘password’]
user = authenticate(request, email=email, password=password)
if user is not None:
login(request, user)
return redirect(‘home’)
return render(request, ‘login.html’)
34. How do you restrict access to a view to authenticated users only?
Answer:
Use the login_required decorator to restrict access to authenticated users.
from django.contrib.auth.decorators import login_required
@login_required
def my_view(request):
return render(request, ‘my_view.html’)
35. How do you implement password reset functionality in Django?
Answer:
Use Django’s built-in password reset views and forms, and configure the email backend to send reset emails.
from django.contrib.auth.views import PasswordResetView
class MyPasswordResetView(PasswordResetView):
template_name = ‘password_reset.html’
36. How do you create custom middleware in Django?
Answer:
Create a class that implements __call__ or process_request and process_response methods.
class MyCustomMiddleware:
def __init__(self, get_response):
self.get_response = get_response
def __call__(self, request):
response = self.get_response(request)
return response
37. How do you add custom middleware to a Django project?
Answer:
Add the middleware class path to the MIDDLEWARE setting in settings.py.
MIDDLEWARE = [
‘django.middleware.security.SecurityMiddleware’,
‘django.contrib.sessions.middleware.SessionMiddleware’,
…
‘myapp.middleware.MyCustomMiddleware’,
]
38. How do you modify the request object in middleware?
Answer:
Override the process_request method to modify the request object before passing it to the view.
def process_request(self, request):
request.custom_attribute = ‘value’
39. How do you modify the response object in middleware?
Answer:
Override the process_response method to modify the response object before returning it to the client.
def process_response(self, request, response):
response[‘Custom-Header’] = ‘Value’
return response
40. How do you handle exceptions in Django middleware?
Answer:
Use the process_exception method in your middleware to handle exceptions raised by views.
def process_exception(self, request, exception):
# Log the exception or handle it in some way
return HttpResponse(“An error occurred”)
41. How do you use signals to perform actions after a model is saved?
Answer:
Connect a signal handler to the post_save signal of the model.
from django.db.models.signals import post_save
from django.dispatch import receiver
@receiver(post_save, sender=Author)
def after_author_saved(sender, instance, **kwargs):
# Perform an action
print(f”Author {instance.name} was saved.”)
42. How do you disconnect a signal in Django?
Answer:
Use the disconnect() method to remove a signal handler.
post_save.disconnect(after_author_saved, sender=Author)
43. How do you create a custom signal in Django?
Answer:
Use the Signal class to define a custom signal and connect handlers to it.
from django.dispatch import Signal
my_signal = Signal()
def my_handler(sender, **kwargs):
print(“Signal received”)
my_signal.connect(my_handler)
my_signal.send(sender=None)
44. How do you ensure that a signal handler runs only once for a particular instance?
Answer:
Use a flag or check the created parameter in the post_save signal handler to ensure the code runs only for newly created instances.
@receiver(post_save, sender=Author)
def after_author_saved(sender, instance, created, **kwargs):
if created:
# Perform action only for new instances
print(f”New author {instance.name} was created.”)
45. How do you handle database transaction issues with signals?
Answer:
Use the transaction.on_commit() function to delay the signal handler until after the transaction is committed.
from django.db import transaction
@receiver(post_save, sender=Author)
def after_author_saved(sender, instance, **kwargs):
transaction.on_commit(lambda: print(f”Author {instance.name} was saved.”))
46. How do you create a simple unit test for a Django view?
Answer:
Use the Django TestCase class to create a test case and define a test method to check the view’s response.
from django.test import TestCase
from django.urls import reverse
class MyViewTest(TestCase):
def test_index_view(self):
response = self.client.get(reverse(‘index’))
self.assertEqual(response.status_code, 200)
47. How do you test a Django model’s method?
Answer:
Create a test case in which you instantiate the model, call its method, and verify the result.
class AuthorTest(TestCase):
def test_author_age(self):
author = Author(name=’John Doe’, age=30)
self.assertEqual(author.age, 30)
48. How do you mock external services in Django tests?
Answer:
Use the unittest.mock module to mock external services or API calls in your tests.
from unittest.mock import patch
class MyTest(TestCase):
@patch(‘myapp.views.requests.get’)
def test_external_service(self, mock_get):
mock_get.return_value.status_code = 200
response = self.client.get(reverse(‘myview’))
self.assertEqual(response.status_code, 200)
49. How do you test Django forms?
Answer:
Instantiate the form with test data and use the is_valid() method to check if the form validates correctly.
class AuthorFormTest(TestCase):
def test_valid_form(self):
data = {‘name’: ‘John Doe’, ‘age’: 30}
form = AuthorForm(data=data)
self.assertTrue(form.is_valid())
50. How do you test Django signals?
Answer:
Use the Signal.disconnect() method to disable other handlers and manually send the signal in your test case.
from django.test import TestCase
from django.db.models.signals import post_save
class SignalTest(TestCase):
def test_signal(self):
post_save.disconnect(after_author_saved, sender=Author)
author = Author(name=’Test Author’, age=40)
author.save()
post_save.connect(after_author_saved, sender=Author)
Final Words
Getting ready for an interview can feel overwhelming, but going through these Django fresher interview questions can help you feel more confident. This guide focuses on the kinds of Django-related interview questions for fresher roles that you’re likely to face.
Don’t forget to practice the Django core concepts, models, and Django REST framework-related interview questions too! With the right preparation, you’ll ace your Django interview and take that important step in your career.
Frequently Asked Questions
1. What are the most common interview questions for Django?
The most common interview questions for Django often cover topics like models, views, forms, ORM, and the Django REST framework.
2. What are the important Django topics freshers should focus on for interviews?
The important Django topics freshers should focus on include Django models, views, URL routing, templates, forms, and Django ORM.
3. How should freshers prepare for Django technical interviews?
Freshers should prepare for Django technical interviews by building small projects, practicing common Django patterns, and understanding the request-response cycle.
4. What strategies can freshers use to solve Django coding questions during interviews?
Strategies freshers can use include breaking down the problem, leveraging Django’s built-in features, and writing clean, modular code.
5. Should freshers prepare for advanced Django topics in interviews?
Yes, freshers should prepare for advanced Django topics like middleware, signals, custom user models, and the Django REST framework if the role requires in-depth knowledge.
Explore More Django Resources
Explore More Interview Questions
Related Posts
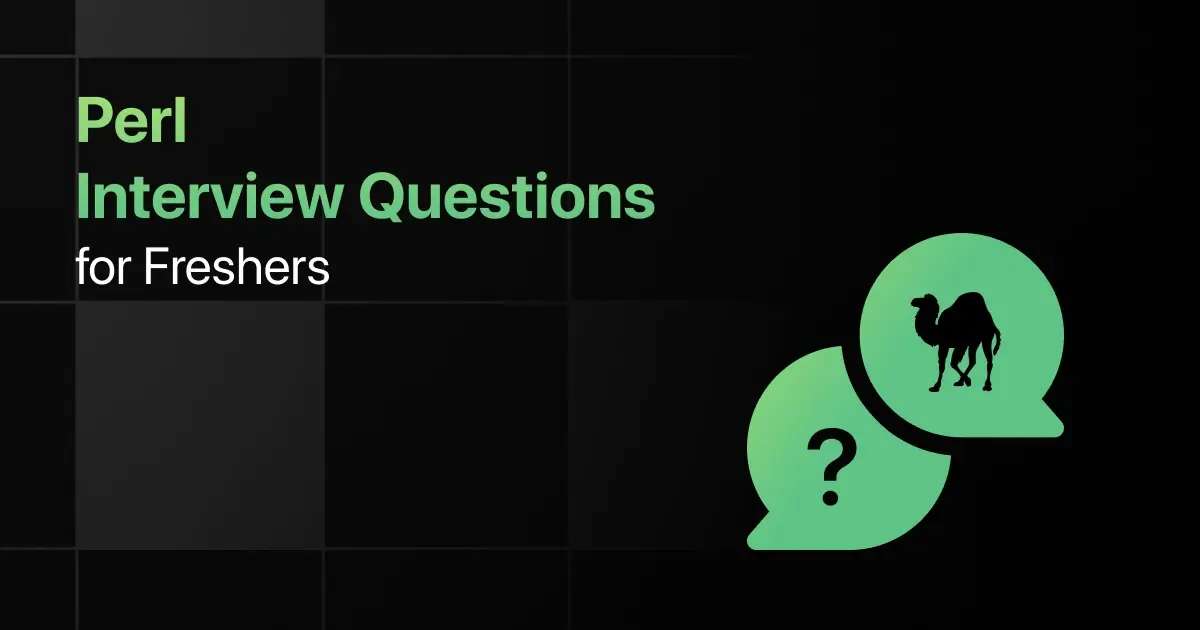
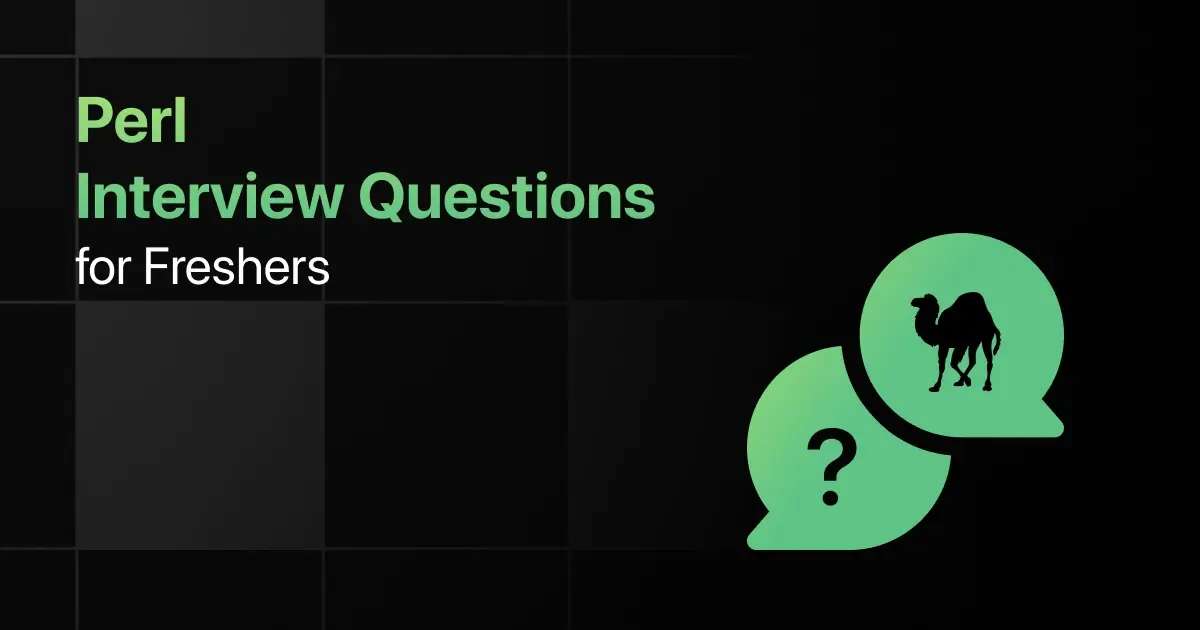
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …