Top C++ Interview Questions for Freshers
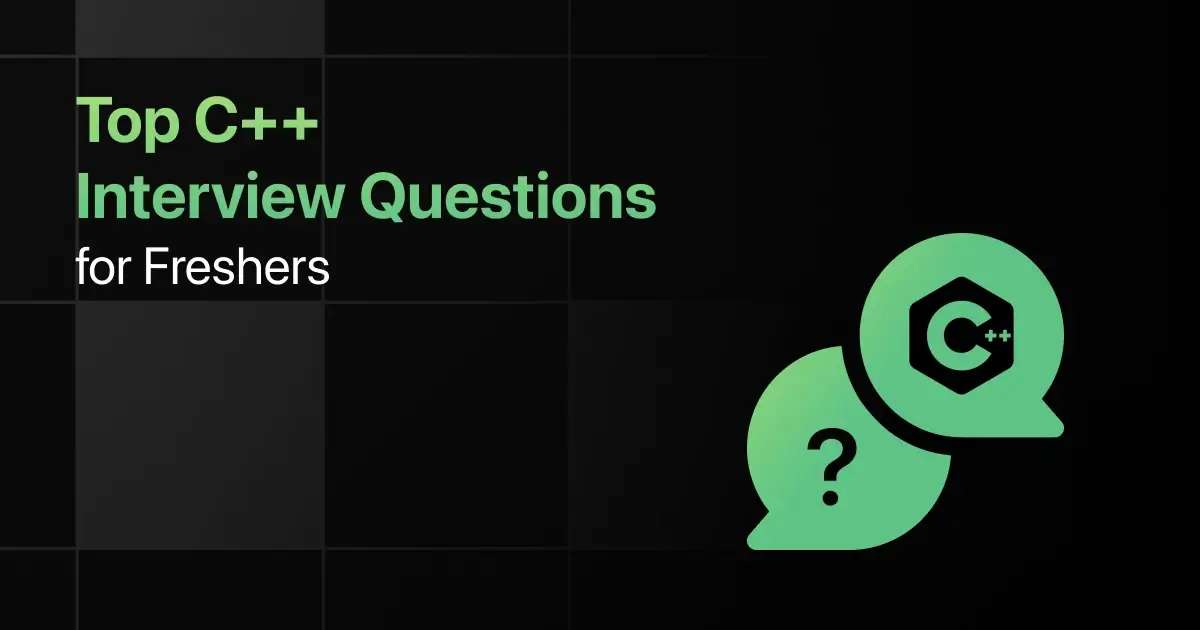
Are you preparing for your first C++ interview and wondering what questions you might face? Understanding the key C++ interview questions for freshers can give you more clarity.
This blog is here to help you get ready with practical questions that test your real-world problem-solving skills. We’ve gathered some of the most common basic C++ interview questions that freshers often encounter.
With this guide, you’ll be well-prepared to tackle these C++ interview questions and answers for freshers and make a strong impression in your interview.
Practice C++ Interview Questions and Answers
Below are the top 50 C++ interview questions for freshers with answers:
1. Write a C++ program to swap two numbers without using a third variable.
Answer:
Use arithmetic operations to swap values directly without needing extra space.
int a = 5, b = 10;
a = a + b;
b = a – b;
a = a – b;
2. How would you reverse a given string in C++ without using the reverse() function?
Answer:
Use a loop to swap characters from the beginning and end of the string until you reach the middle.
std::string str = “Hello”;
int n = str.length();
for (int i = 0; i < n / 2; i++) {
std::swap(str[i], str[n – i – 1]);
}
3. Write a C++ function to check if a given number is a palindrome.
Answer:
Reverse the number and compare it with the original to check for palindrome property.
bool isPalindrome(int num) {
int original = num, reversed = 0;
while (num > 0) {
int digit = num % 10;
reversed = reversed * 10 + digit;
num /= 10;
}
return original == reversed;
}
4. How do you calculate the factorial of a number using recursion in C++?
Answer:
Define a recursive function that multiplies the current number by the factorial of the previous number until it reaches 1.
int factorial(int n) {
if (n <= 1) return 1;
return n * factorial(n – 1);
}
5. Write a C++ program to find the largest of three numbers using a simple if-else construct.
Answer:
Compare the numbers pairwise and store the largest one at each step.
int largest(int a, int b, int c) {
if (a > b && a > c) return a;
else if (b > c) return b;
else return c;
}
6. How do you implement a basic class in C++ to represent a Student with attributes like name and roll number?
Answer:
Define a class with private attributes and public methods to set and get the attribute values.
class Student {
private:
std::string name;
int rollNumber;
public:
void setName(std::string n) { name = n; }
void setRollNumber(int r) { rollNumber = r; }
std::string getName() { return name; }
int getRollNumber() { return rollNumber; }
};
7. Write a C++ program to demonstrate constructor overloading with an example.
Answer:
Create multiple constructors within a class to initialize objects in different ways.
class Rectangle {
private:
int width, height;
public:
Rectangle() : width(0), height(0) {}
Rectangle(int w, int h) : width(w), height(h) {}
int area() { return width * height; }
};
8. How would you implement single inheritance in C++? Demonstrate with a Base and Derived class.
Answer:
Derive a new class from an existing class using the public inheritance specifier.
class Base {
public:
void show() { std::cout << “Base class\n”; }
};
class Derived : public Base {
public:
void display() { std::cout << “Derived class\n”; }
};
9. Write a C++ program that demonstrates the concept of function overloading.
Answer:
Define multiple functions with the same name but different parameters.
class Math {
public:
int add(int a, int b) { return a + b; }
double add(double a, double b) { return a + b; }
};
10. How would you implement polymorphism in C++ using a base class pointer and virtual functions?
Answer:
Create a base class with a virtual function and derive classes that override this function.
class Base {
public:
virtual void show() { std::cout << “Base class\n”; }
};
class Derived : public Base {
public:
void show() override { std::cout << “Derived class\n”; }
};
Base* basePtr = new Derived();
basePtr->show(); // Outputs “Derived class”
11. Write a C++ program to dynamically allocate memory for an array and deallocate it.
Answer:
Use new to allocate memory and delete[] to deallocate it.
int* arr = new int[10];
// Use the array
delete[] arr;
12. How do you implement a simple linked list in C++ using pointers? Write the code to insert a node at the beginning.
Answer:
Define a Node structure and use pointers to link the nodes together.
struct Node {
int data;
Node* next;
};
void insertAtBeginning(Node*& head, int newData) {
Node* newNode = new Node();
newNode->data = newData;
newNode->next = head;
head = newNode;
}
13. Write a C++ program to demonstrate the concept of pointer arithmetic by iterating over an array.
Answer:
Use pointer increment to traverse the array.
int arr[] = {1, 2, 3, 4, 5};
int* ptr = arr;
for (int i = 0; i < 5; ++i) {
std::cout << *(ptr + i) << ” “;
}
14. How do you implement a smart pointer in C++? Write a basic unique_ptr implementation.
Answer:
Use the std::unique_ptr from the C++ Standard Library to manage dynamic memory automatically.
#include <memory>
std::unique_ptr<int> ptr(new int(5));
// No need to manually delete the memory
15. What is a memory leak, and how can it be avoided in C++? Demonstrate a situation that could lead to a memory leak and how to prevent it.
Answer:
A memory leak occurs when allocated memory is not properly deallocated. It can be avoided by ensuring that all allocated memory is deleted.
int* ptr = new int(10);
// Forgot to delete the memory
// delete ptr; // Prevent memory leak
16. How do you implement a stack using STL in C++? Provide an example of basic stack operations like push and pop.
Answer:
Use the std::stack container adapter to implement a stack.
#include <stack>
std::stack<int> s;
s.push(10);
s.push(20);
int top = s.top(); // 20
s.pop(); // Removes 20
17. Write a C++ program to find the maximum element in a vector using STL.
Answer:
Use the std::max_element algorithm to find the maximum element.
#include <vector>
#include <algorithm>
std::vector<int> v = {10, 20, 30, 40};
int maxElem = *std::max_element(v.begin(), v.end());
18. How do you sort a list of strings in descending order using STL in C++?
Answer:
Use std::sort with a custom comparator.
#include <vector>
#include <algorithm>
std::vector<std::string> vec = {“apple”, “banana”, “cherry”};
std::sort(vec.begin(), vec.end(), std::greater<std::string>());
19. Write a C++ program to count the occurrences of a specific element in a list using STL.
Answer:
Use the std::count algorithm to count occurrences.
#include <list>
#include <algorithm>
std::list<int> lst = {1, 2, 3, 2, 4, 2};
int count = std::count(lst.begin(), lst.end(), 2); // 3 occurrences of 2
20. How would you merge two sets in C++ using STL? Provide a code example that merges two std::set containers.
Answer:
Use std::set_union to merge two sets.
#include <set>
#include <algorithm>
std::set<int> set1 = {1, 2, 3};
std::set<int> set2 = {3, 4, 5};
std::set<int> result;
std::set_union(set1.begin(), set1.end(), set2.begin(), set2.end(),
std::inserter(result, result.begin()));
21. Write a C++ program to implement binary search on a sorted array.
Answer:
Use a loop to repeatedly divide the array and narrow down the search range.
int binarySearch(int arr[], int size, int key) {
int left = 0, right = size – 1;
while (left <= right) {
int mid = left + (right – left) / 2;
if (arr[mid] == key) return mid;
if (arr[mid] < key) left = mid + 1;
else right = mid – 1;
}
return -1;
}
22. How do you implement a basic queue using an array in C++? Provide the code for enqueue and dequeue operations.
Answer:
Use an array to store elements and manage front and rear indices for queue operations.
class Queue {
private:
int arr[100];
int front, rear;
public:
Queue() : front(0), rear(0) {}
void enqueue(int data) { arr[rear++] = data; }
int dequeue() { return arr[front++]; }
};
23. Write a C++ program to sort an array using the quicksort algorithm.
Answer:
Use a divide-and-conquer approach to partition the array and recursively sort the subarrays.
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi – 1);
quickSort(arr, pi + 1, high);
}
}
int partition(int arr[], int low, int high) {
int pivot = arr[high];
int i = (low – 1);
for (int j = low; j <= high – 1; j++) {
if (arr[j] < pivot) {
i++;
std::swap(arr[i], arr[j]);
}
}
std::swap(arr[i + 1], arr[high]);
return (i + 1);
}
24. How would you implement a binary tree in C++? Write the code for inserting a node into a binary tree.
Answer:
Define a Node structure and write a function to insert nodes based on value comparison.
struct Node {
int data;
Node* left, *right;
Node(int val) : data(val), left(nullptr), right(nullptr) {}
};
Node* insert(Node* root, int data) {
if (root == nullptr) return new Node(data);
if (data < root->data) root->left = insert(root->left, data);
else root->right = insert(root->right, data);
return root;
}
25. Write a C++ program to find the shortest path in a graph using Dijkstra’s algorithm.
Answer:
Use a priority queue to explore the graph and update the shortest paths to each node.
#include <vector>
#include <queue>
#include <climits>
void dijkstra(std::vector<std::vector<std::pair<int, int>>> &graph, int src, int V) {
std::vector<int> dist(V, INT_MAX);
dist[src] = 0;
std::priority_queue<std::pair<int, int>, std::vector<std::pair<int, int>>, std::greater<>> pq;
pq.push({0, src});
while (!pq.empty()) {
int u = pq.top().second;
pq.pop();
for (auto &neighbor : graph[u]) {
int v = neighbor.first, weight = neighbor.second;
if (dist[u] + weight < dist[v]) {
dist[v] = dist[u] + weight;
pq.push({dist[v], v});
}
}
}
}
26. How do you read and write to a text file in C++? Provide an example that reads a file line by line and writes the content to another file.
Answer:
Use ifstream for reading and ofstream for writing.
#include <fstream>
#include <string>
void copyFile(const std::string &inputFile, const std::string &outputFile) {
std::ifstream inFile(inputFile);
std::ofstream outFile(outputFile);
std::string line;
while (std::getline(inFile, line)) {
outFile << line << “\n”;
}
}
27. Write a C++ program to count the number of words in a text file.
Answer:
Use file streams to read the file word by word and count the occurrences.
#include <fstream>
#include <string>
int countWords(const std::string &filename) {
std::ifstream inFile(filename);
std::string word;
int count = 0;
while (inFile >> word) {
count++;
}
return count;
}
28. How do you handle binary files in C++? Write a program to read and write binary data.
Answer:
Use ifstream and ofstream with the ios::binary flag to handle binary files.
#include <fstream>
struct Data {
int id;
char name[20];
};
void writeBinary(const std::string &filename) {
std::ofstream outFile(filename, std::ios::binary);
Data d = {1, “Example”};
outFile.write(reinterpret_cast<char*>(&d), sizeof(Data));
}
void readBinary(const std::string &filename) {
std::ifstream inFile(filename, std::ios::binary);
Data d;
inFile.read(reinterpret_cast<char*>(&d), sizeof(Data));
}
29. Write a C++ program to append text to an existing file without overwriting its content.
Answer:
Use ofstream with the ios::app flag to append data to a file.
#include <fstream>
#include <string>
void appendToFile(const std::string &filename, const std::string &text) {
std::ofstream outFile(filename, std::ios::app);
outFile << text << “\n”;
}
30. How do you serialize and deserialize an object in C++ using file handling? Provide an example of saving and loading a class object to/from a file.
Answer:
Use file streams to serialize (write) and deserialize (read) an object’s data.
#include <fstream>
class MyClass {
public:
int id;
char name[20];
void serialize(const std::string &filename) {
std::ofstream outFile(filename, std::ios::binary);
outFile.write(reinterpret_cast<char*>(this), sizeof(MyClass));
}
void deserialize(const std::string &filename) {
std::ifstream inFile(filename, std::ios::binary);
inFile.read(reinterpret_cast<char*>(this), sizeof(MyClass));
}
};
31. How do you create and manage threads in C++ using the Standard Library? Write a program to run two threads concurrently.
Answer:
Use std::thread to create and manage threads.
#include <thread>
#include <iostream>
void printNumbers() {
for (int i = 1; i <= 5; i++) {
std::cout << i << ” “;
}
}
void printLetters() {
for (char c = ‘A’; c <= ‘E’; c++) {
std::cout << c << ” “;
}
}
int main() {
std::thread t1(printNumbers);
std::thread t2(printLetters);
t1.join();
t2.join();
return 0;
}
32. Write a C++ program that uses a mutex to ensure thread-safe access to a shared variable.
Answer:
Use std::mutex to protect access to the shared resource.
#include <thread>
#include <mutex>
#include <iostream>
int counter = 0;
std::mutex mtx;
void incrementCounter() {
std::lock_guard<std::mutex> lock(mtx);
++counter;
}
int main() {
std::thread t1(incrementCounter);
std::thread t2(incrementCounter);
t1.join();
t2.join();
std::cout << “Counter: ” << counter << std::endl;
return 0;
}
33. How do you implement a producer-consumer problem in C++ using threads? Provide a simple implementation using condition variables.
Answer:
Use std::condition_variable to synchronize the producer and consumer threads.
#include <queue>
#include <thread>
#include <mutex>
#include <condition_variable>
#include <iostream>
std::queue<int> q;
std::mutex mtx;
std::condition_variable cv;
void producer() {
for (int i = 1; i <= 5; i++) {
std::unique_lock<std::mutex> lock(mtx);
q.push(i);
cv.notify_one();
}
}
void consumer() {
for (int i = 1; i <= 5; i++) {
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [] { return !q.empty(); });
int val = q.front();
q.pop();
std::cout << “Consumed: ” << val << std::endl;
}
}
int main() {
std::thread t1(producer);
std::thread t2(consumer);
t1.join();
t2.join();
return 0;
}
34. Write a C++ program that demonstrates the use of a thread pool for parallel processing.
Answer:
Create a pool of worker threads that can execute tasks from a task queue.
#include <iostream>
#include <vector>
#include <thread>
#include <queue>
#include <functional>
#include <condition_variable>
class ThreadPool {
public:
ThreadPool(size_t numThreads);
~ThreadPool();
void enqueueTask(std::function<void()> task);
private:
std::vector<std::thread> workers;
std::queue<std::function<void()>> tasks;
std::mutex mtx;
std::condition_variable cv;
bool stop;
void workerThread();
};
ThreadPool::ThreadPool(size_t numThreads) : stop(false) {
for (size_t i = 0; i < numThreads; ++i) {
workers.emplace_back([this] { workerThread(); });
}
}
ThreadPool::~ThreadPool() {
{
std::unique_lock<std::mutex> lock(mtx);
stop = true;
}
cv.notify_all();
for (std::thread &worker : workers) {
worker.join();
}
}
void ThreadPool::enqueueTask(std::function<void()> task) {
{
std::unique_lock<std::mutex> lock(mtx);
tasks.push(task);
}
cv.notify_one();
}
void ThreadPool::workerThread() {
while (true) {
std::function<void()> task;
{
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [this] { return stop || !tasks.empty(); });
if (stop && tasks.empty()) return;
task = std::move(tasks.front());
tasks.pop();
}
task();
}
}
int main() {
ThreadPool pool(4);
pool.enqueueTask([] { std::cout << “Task 1\n”; });
pool.enqueueTask([] { std::cout << “Task 2\n”; });
pool.enqueueTask([] { std::cout << “Task 3\n”; });
pool.enqueueTask([] { std::cout << “Task 4\n”; });
return 0;
}
35. How do you use futures and promises in C++ to manage asynchronous operations? Write a program that demonstrates their use.
Answer:
Use std::future and std::promise to handle asynchronous operations and retrieve their results.
#include <iostream>
#include <future>
int asyncTask(std::promise<int> &promiseObj) {
int result = 10 * 2;
promiseObj.set_value(result);
return result;
}
int main() {
std::promise<int> promiseObj;
std::future<int> futureObj = promiseObj.get_future();
std::thread t(asyncTask, std::ref(promiseObj));
std::cout << “Result: ” << futureObj.get() << std::endl;
t.join();
return 0;
}
36. How do you implement a template function in C++? Write a template function that swaps two values of any data type.
Answer:
Define a function template using the template keyword.
template <typename T>
void swapValues(T &a, T &b) {
T temp = a;
a = b;
b = temp;
}
37. Write a C++ program that demonstrates the use of template classes by creating a generic stack class.
Answer:
Use a class template to define a stack that works with any data type.
template <typename T>
class Stack {
private:
std::vector<T> elements;
public:
void push(const T &element) { elements.push_back(element); }
void pop() { elements.pop_back(); }
T top() const { return elements.back(); }
bool isEmpty() const { return elements.empty(); }
};
38. How do you implement multiple inheritance in C++? Write a program that demonstrates multiple inheritance with two base classes and one derived class.
Answer:
Define a derived class that inherits from multiple base classes.
class Base1 {
public:
void show() { std::cout << “Base1\n”; }
};
class Base2 {
public:
void display() { std::cout << “Base2\n”; }
};
class Derived : public Base1, public Base2 {
};
int main() {
Derived d;
d.show();
d.display();
return 0;
}
39. Write a C++ program that demonstrates the use of the std::function to store and invoke a callback function.
Answer:
Use std::function to store a callable entity and invoke it later.
#include <iostream>
#include <functional>
void myCallback(int x) {
std::cout << “Callback called with value: ” << x << std::endl;
}
int main() {
std::function<void(int)> callback = myCallback;
callback(10);
return 0;
}
40. How do you use lambda expressions in C++? Write a program that captures variables by value and by reference in a lambda.
Answer:
Use lambda expressions to define inline functions that can capture variables.
#include <iostream>
int main() {
int a = 10, b = 20;
auto add = [a, &b]() {
b = b + a;
return b;
};
std::cout << “Sum: ” << add() << std::endl;
return 0;
}
41. Write a C++ program that demonstrates exception handling by throwing and catching a custom exception.
Answer:
Define a custom exception class and use try, catch, and throw to handle exceptions.
class MyException : public std::exception {
public:
const char *what() const noexcept override {
return “My custom exception”;
}
};
int main() {
try {
throw MyException();
} catch (const MyException &e) {
std::cout << e.what() << std::endl;
}
return 0;
}
42. How do you handle multiple exceptions in C++? Provide an example where you catch different types of exceptions.
Answer:
Use multiple catch blocks to handle different types of exceptions.
int main() {
try {
throw 10;
} catch (int e) {
std::cout << “Caught integer exception: ” << e << std::endl;
} catch (double e) {
std::cout << “Caught double exception: ” << e << std::endl;
}
return 0;
}
43. Write a C++ program that uses a try-catch-finally like mechanism to ensure that resources are released even if an exception is thrown.
Answer:
Use RAII (Resource Acquisition Is Initialization) to manage resources safely.
class ResourceGuard {
public:
ResourceGuard() { std::cout << “Acquiring resource\n”; }
~ResourceGuard() { std::cout << “Releasing resource\n”; }
};
int main() {
try {
ResourceGuard rg;
throw std::runtime_error(“An error occurred”);
} catch (const std::exception &e) {
std::cout << e.what() << std::endl;
}
return 0;
}
44. How do you implement a custom error code handling mechanism in C++? Write a program that uses a custom error code enum to represent different error states.
Answer:
Define an enum for error codes and return these from functions to indicate success or failure.
enum class ErrorCode {
Success,
NotFound,
PermissionDenied
};
ErrorCode performOperation(int data) {
if (data < 0) return ErrorCode::NotFound;
if (data == 0) return ErrorCode::PermissionDenied;
return ErrorCode::Success;
}
45. Write a C++ program that demonstrates the use of std::exception_ptr to rethrow an exception in a different thread.
Answer:
Use std::exception_ptr to capture and propagate exceptions across threads.
#include <iostream>
#include <thread>
#include <exception>
std::exception_ptr eptr;
void threadFunc() {
try {
throw std::runtime_error(“Error in thread”);
} catch (…) {
eptr = std::current_exception();
}
}
int main() {
std::thread t(threadFunc);
t.join();
if (eptr) {
try {
std::rethrow_exception(eptr);
} catch (const std::exception &e) {
std::cout << e.what() << std::endl;
}
}
return 0;
}
46. How do you implement the Singleton pattern in C++? Provide a thread-safe implementation using std::mutex.
Answer:
Use a static function to return the single instance of the class and protect it with a std::mutex.
class Singleton {
private:
static Singleton *instance;
static std::mutex mtx;
Singleton() {}
public:
Singleton(const Singleton &) = delete;
Singleton &operator=(const Singleton &) = delete;
static Singleton *getInstance() {
std::lock_guard<std::mutex> lock(mtx);
if (!instance) {
instance = new Singleton();
}
return instance;
}
};
Singleton *Singleton::instance = nullptr;
std::mutex Singleton::mtx;
47. Write a C++ program that demonstrates the Factory design pattern by creating different types of objects based on input parameters.
Answer:
Use a factory method to create and return different types of objects.
class Shape {
public:
virtual void draw() = 0;
};
class Circle : public Shape {
public:
void draw() override { std::cout << “Drawing Circle\n”; }
};
class Square : public Shape {
public:
void draw() override { std::cout << “Drawing Square\n”; }
};
class ShapeFactory {
public:
static Shape *createShape(const std::string &type) {
if (type == “Circle”) return new Circle();
if (type == “Square”) return new Square();
return nullptr;
}
};
int main() {
Shape *shape = ShapeFactory::createShape(“Circle”);
shape->draw();
delete shape;
return 0;
}
48. How do you implement the Observer pattern in C++? Write a program where observers are notified of state changes in the subject.
Answer:
Use a subject class to maintain a list of observers and notify them of state changes.
#include <iostream>
#include <vector>
class Observer {
public:
virtual void update(int state) = 0;
};
class Subject {
private:
std::vector<Observer *> observers;
int state;
public:
void attach(Observer *observer) { observers.push_back(observer); }
void setState(int newState) {
state = newState;
notifyAll();
}
void notifyAll() {
for (Observer *observer : observers) {
observer->update(state);
}
}
};
class ConcreteObserver : public Observer {
public:
void update(int state) override { std::cout << “State changed to: ” << state << std::endl; }
};
int main() {
Subject subject;
ConcreteObserver observer1, observer2;
subject.attach(&observer1);
subject.attach(&observer2);
subject.setState(10);
return 0;
}
49. Write a C++ program that demonstrates the Strategy design pattern by implementing different sorting algorithms that can be selected at runtime.
Answer:
Define a strategy interface and implement different strategies that can be selected dynamically.
class SortStrategy {
public:
virtual void sort(std::vector<int> &data) = 0;
};
class BubbleSort : public SortStrategy {
public:
void sort(std::vector<int> &data) override {
for (size_t i = 0; i < data.size(); ++i) {
for (size_t j = 0; j < data.size() – i – 1; ++j) {
if (data[j] > data[j + 1]) std::swap(data[j], data[j + 1]);
}
}
}
};
class QuickSort : public SortStrategy {
public:
void sort(std::vector<int> &data) override {
std::sort(data.begin(), data.end());
}
};
class SortContext {
private:
SortStrategy *strategy;
public:
void setStrategy(SortStrategy *s) { strategy = s; }
void executeStrategy(std::vector<int> &data) { strategy->sort(data); }
};
int main() {
std::vector<int> data = {5, 2, 9, 1, 5, 6};
SortContext context;
context.setStrategy(new BubbleSort());
context.executeStrategy(data);
for (int val : data) std::cout << val << ” “;
return 0;
}
50. How do you implement the Decorator pattern in C++? Write a program that extends the functionality of an object dynamically.
Answer:
Use a base class and concrete decorators to add functionality to objects at runtime.
class Coffee {
public:
virtual std::string getDescription() = 0;
virtual double cost() = 0;
};
class SimpleCoffee : public Coffee {
public:
std::string getDescription() override { return “Simple Coffee”; }
double cost() override { return 2.0; }
};
class MilkDecorator : public Coffee {
private:
Coffee *coffee;
public:
MilkDecorator(Coffee *c) : coffee(c) {}
std::string getDescription() override { return coffee->getDescription() + “, Milk”; }
double cost() override { return coffee->cost() + 0.5; }
};
class SugarDecorator : public Coffee {
private:
Coffee *coffee;
public:
SugarDecorator(Coffee *c) : coffee(c) {}
std::string getDescription() override { return coffee->getDescription() + “, Sugar”; }
double cost() override { return coffee->cost() + 0.2; }
};
int main() {
Coffee *coffee = new SimpleCoffee();
coffee = new MilkDecorator(coffee);
coffee = new SugarDecorator(coffee);
std::cout << coffee->getDescription() << ” costs $” << coffee->cost() << std::endl;
delete coffee;
return 0;
}
Final Words
Getting ready for an interview can feel overwhelming, but going through these C++ fresher interview questions can help you feel more confident. This guide focuses on the kinds of C++ developer interview questions for fresher roles that you’re likely to face.
Don’t forget to practice the C++ basic coding interview questions too! With the right preparation, you’ll ace your C++ interview and take that important step in your career.
Frequently Asked Questions
1. What are the most common interview questions for C++?
Common interview questions for C++ often focus on object-oriented programming concepts, memory management, pointers, data structures, and algorithms.
2. What are the important C++ topics freshers should focus on for interviews?
Freshers should focus on understanding classes and objects, inheritance, polymorphism, pointers, references, STL, and basic algorithms.
3. How should freshers prepare for C++ technical interviews?
Freshers should practice coding problems, review key concepts, and understand the application of C++ in solving real-world problems.
4. What strategies can freshers use to solve C++ coding questions during interviews?
Freshers should break down problems, write clean code, test with different inputs, and focus on time and space complexity.
5. Should freshers prepare for advanced C++ topics in interviews?
Yes, depending on the job role, it’s beneficial to know advanced topics like smart pointers, multithreading, templates, and memory management techniques.
Explore More C++ Resources
Explore More Interview Questions
Related Posts
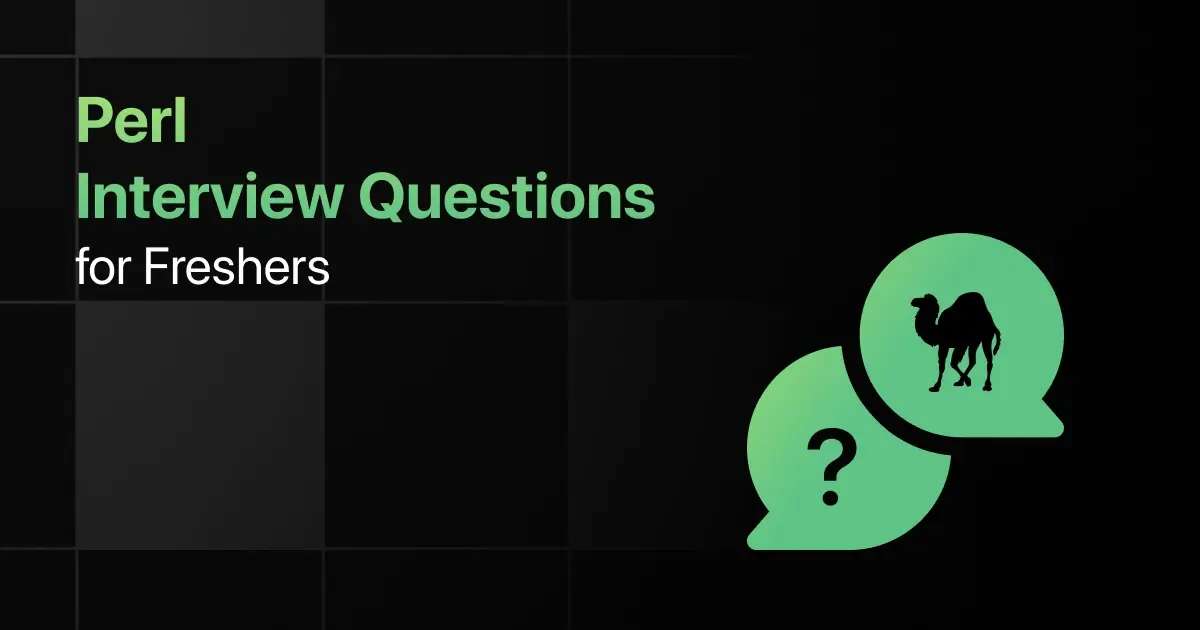
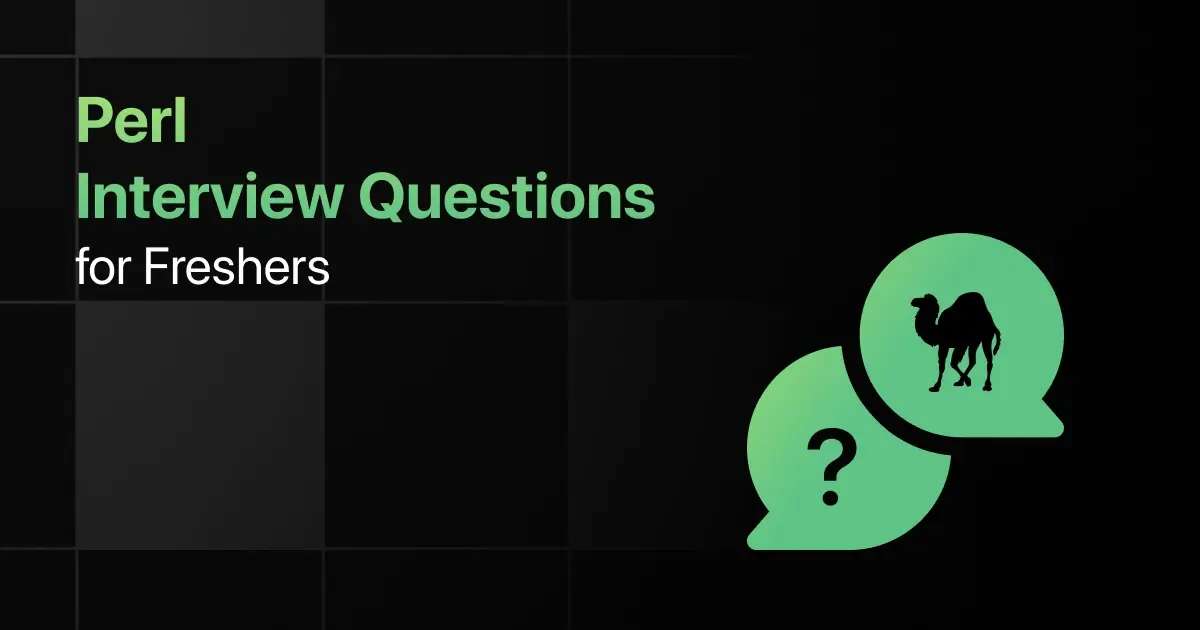
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …