Top C# Interview Questions for Freshers
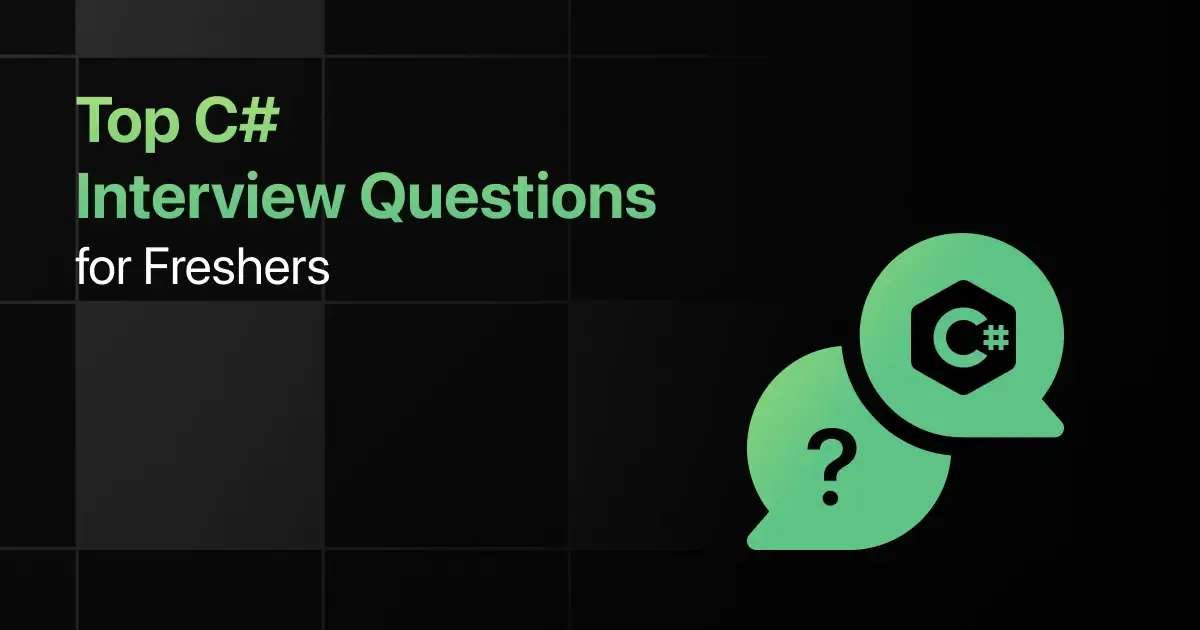
Are you preparing for your first C# interview and wondering what questions you might face?
Understanding the key C# interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these C# interview questions and answers for freshers and make a strong impression in your interview.
Practice C# Interview Questions and Answers
Below are the top 50 C# interview questions for freshers with answers:
1. How do you declare and initialize a variable in C#?
Answer:
Declare a variable by specifying its type and name, followed by initialization with a value using the assignment operator.
int number = 5;
2. What is the difference between == and Equals() in C#?
Answer:
== compares the reference or value type of objects, while Equals() compares the actual content of the objects.
string a = “hello”;
string b = “hello”;
bool result = a == b; // True
bool result2 = a.Equals(b); // True
3. How do you create a constant in C#?
Answer:
Use the const keyword to declare a constant, which means its value cannot be changed after initialization.
const double Pi = 3.14159;
4. What is the purpose of the using statement in C#?
Answer:
The using statement is used to include namespaces and ensure that resources are disposed of correctly at the end of their usage.
using System.IO;
5. How do you define an enum in C#?
Answer:
Define an enum using the enum keyword, followed by the enum name and a list of named constants.
enum Days { Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday }
6. How do you create a class in C# and instantiate an object of that class?
Answer:
Define a class using the class keyword and instantiate it using the new keyword.
class Car {
public string Make { get; set; }
}
Car myCar = new Car();
7. What is the difference between a class and an object in C#?
Answer:
A class is a blueprint or template for objects, while an object is an instance of a class, containing data and methods defined in the class.
Car myCar = new Car(); // `myCar` is an object, `Car` is the class
8. How do you implement encapsulation in C#?
Answer:
Encapsulation is implemented using access modifiers like private, protected, and public to restrict access to class members.
class Account {
private decimal balance;
public void Deposit(decimal amount) {
balance += amount;
}
}
9. What is inheritance, and how do you implement it in C#?
Answer:
Inheritance allows a class to inherit members from another class using the : symbol. The derived class inherits properties and methods from the base class.
class Vehicle {
public string Brand { get; set; }
}
class Car : Vehicle {
public int Wheels { get; set; }
}
10. What is polymorphism in C#, and how do you achieve it?
Answer:
Polymorphism allows methods to have different implementations in derived classes. It is achieved using method overriding with the virtual and override keywords.
class Animal {
public virtual void Speak() {
Console.WriteLine(“Animal speaks”);
}
}
class Dog : Animal {
public override void Speak() {
Console.WriteLine(“Dog barks”);
}
}
11. How do you implement an interface in C#?
Answer:
An interface is implemented by a class using the : symbol. The class must provide implementations for all interface members.
interface IDriveable {
void Drive();
}
class Car : IDriveable {
public void Drive() {
Console.WriteLine(“Driving a car”);
}
}
12. What is the difference between an abstract class and an interface in C#?
Answer:
An abstract class can have method implementations and fields, while an interface can only have method declarations. A class can inherit multiple interfaces but only one abstract class.
abstract class Animal {
public abstract void MakeSound();
}
interface IRun {
void Run();
}
13. How do you handle exceptions in C#?
Answer:
Use try, catch, and finally blocks to handle exceptions, allowing you to manage runtime errors gracefully.
try {
int result = 10 / 0;
} catch (DivideByZeroException ex) {
Console.WriteLine(“Cannot divide by zero”);
} finally {
Console.WriteLine(“End of try-catch block”);
}
14. What is a delegate in C#, and how do you declare and use it?
Answer:
A delegate is a type-safe function pointer. It is declared using the delegate keyword and can point to methods with a matching signature.
public delegate void PrintMessage(string message);
PrintMessage pm = new PrintMessage(Console.WriteLine);
pm(“Hello, Delegates!”);
15. How do you implement a generic class in C#?
Answer:
A generic class is defined using a type parameter that allows the class to operate on different data types.
class GenericClass<T> {
public T Field;
public GenericClass(T value) {
Field = value;
}
}
GenericClass<int> myClass = new GenericClass<int>(10);
16. How do you use LINQ to filter and sort a list of integers?
Answer:
LINQ allows you to query collections using query syntax or method syntax, enabling powerful filtering, sorting, and aggregation operations.
int[] numbers = { 5, 3, 9, 1 };
var sortedNumbers = from n in numbers
where n > 2
orderby n
select n;
17. What is the purpose of the List<T> class, and how do you add elements to it?
Answer:
List<T> is a generic collection that provides dynamic array-like storage for elements. Use the Add method to add elements.
List<int> numbers = new List<int>();
numbers.Add(1);
numbers.Add(2);
18. How do you find the maximum value in a list using LINQ?
Answer:
Use the Max() method provided by LINQ to find the maximum value in a list or collection.
List<int> numbers = new List<int> { 1, 5, 3 };
int maxValue = numbers.Max();
19. What is the difference between IEnumerable and IQueryable in C#?
Answer:
IEnumerable is used for in-memory collections, while IQueryable is designed for querying data from out-of-memory sources like databases, allowing for deferred execution and remote querying.
IEnumerable<int> numbers = new List<int> { 1, 2, 3 };
20. How do you group data using LINQ in C#?
Answer:
Use the group by clause in LINQ to group elements in a collection based on a specific key.
var grouped = from n in numbers
group n by n % 2 into g
select g;
21. How do you read the contents of a file line by line in C#?
Answer:
Use the StreamReader class in a using block to read the contents of a file line by line.
using (StreamReader sr = new StreamReader(“file.txt”)) {
string line;
while ((line = sr.ReadLine()) != null) {
Console.WriteLine(line);
}
}
22. What is the method to write text to a file in C#?
Answer:
Use the StreamWriter class to write text to a file, which creates a new file or appends to an existing one.
using (StreamWriter sw = new StreamWriter(“file.txt”)) {
sw.WriteLine(“Hello, World!”);
}
23. How do you check if a file exists in C#?
Answer:
Use the File.Exists() method to check whether a specific file exists in the specified path.
bool exists = File.Exists(“file.txt”);
24. What is the method to copy a file from one location to another in C#?
Answer:
Use the File.Copy() method to copy a file from one directory to another, specifying the source and destination paths.
File.Copy(“source.txt”, “destination.txt”);
25. How do you delete a file in C#?
Answer:
Use the File.Delete() method to delete a file from the filesystem by providing its path.
File.Delete(“file.txt”);
26. What is an event in C#, and how do you declare one?
Answer:
An event is a message that objects send to signal the occurrence of an action. Declare an event using the event keyword and a delegate type.
public event EventHandler MyEvent;
27. How do you subscribe to an event in C#?
Answer:
Subscribe to an event by attaching an event handler method using the += operator.
MyEvent += new EventHandler(EventHandlerMethod);
28. What is a multicast delegate in C#?
Answer:
A multicast delegate can reference multiple methods. When invoked, all methods in the delegate chain are called in sequence.
public delegate void Notify();
Notify del = Method1;
del += Method2;
29. How do you remove a method from a delegate’s invocation list in C#?
Answer:
Use the -= operator to remove a method from a delegate’s invocation list.
del -= Method1;
30. How do you raise an event in C#?
Answer:
Raise an event by invoking it, typically using a protected virtual method to ensure it’s properly raised by derived classes.
protected virtual void OnMyEvent() {
MyEvent?.Invoke(this, EventArgs.Empty);
}
31. How do you create a custom exception in C#?
Answer:
Create a custom exception by deriving a class from Exception and implementing appropriate constructors.
public class MyCustomException : Exception {
public MyCustomException(string message) : base(message) { }
}
32. What is the difference between throw and throw ex in C#?
Answer:
throw preserves the original stack trace, while throw ex resets the stack trace, making it harder to trace the original source of the exception.
try {
// Code that throws an exception
} catch (Exception ex) {
throw; // Preserves stack trace
}
33. How do you implement a try-finally block in C#?
Answer:
Use a try-finally block to execute code that must run whether or not an exception is thrown, such as resource cleanup.
try {
// Code that may throw an exception
} finally {
// Code that always runs
}
34. What is the purpose of the StackTrace class in C#?
Answer:
The StackTrace class provides information about the current call stack, useful for debugging and logging.
StackTrace st = new StackTrace();
Console.WriteLine(st.ToString());
35. How do you log exceptions in C#?
Answer:
Log exceptions by writing them to a file, database, or logging framework like log4net or NLog, typically inside a catch block.
try {
// Code that may throw an exception
} catch (Exception ex) {
File.WriteAllText(“log.txt”, ex.ToString());
}
36. How do you create a new thread in C#?
Answer:
Create a new thread using the Thread class, passing a ThreadStart delegate or lambda expression as the method to run.
Thread myThread = new Thread(new ThreadStart(MyMethod));
myThread.Start();
37. What is the Task class in C#, and how do you use it for asynchronous operations?
Answer:
The Task class represents an asynchronous operation. Use Task.Run() to execute a method asynchronously.
Task.Run(() => {
// Code to run asynchronously
});
38. How do you use the async and await keywords in C#?
Answer:
Mark a method with async and use await to asynchronously wait for the completion of a task.
public async Task<int> GetDataAsync() {
int result = await Task.Run(() => {
// Simulate long-running task
return 42;
});
return result;
}
39. What is a ThreadPool, and how do you use it in C#?
Answer:
A ThreadPool manages a pool of worker threads, reducing the overhead of thread creation. Use ThreadPool.QueueUserWorkItem() to queue tasks.
ThreadPool.QueueUserWorkItem(state => {
// Code to run in the thread pool
});
40. How do you implement a cancellation token to cancel an ongoing task in C#?
Answer:
Use the CancellationTokenSource and CancellationToken to signal and check for cancellation in a task.
CancellationTokenSource cts = new CancellationTokenSource();
Task.Run(() => {
while (!cts.Token.IsCancellationRequested) {
// Long-running task
}
}, cts.Token);
// Cancel the task
cts.Cancel();
41. How do you create a model class for a database table in Entity Framework?
Answer:
Define a C# class with properties corresponding to the database table columns, and annotate it with data annotations if needed.
public class Product {
public int ProductId { get; set; }
public string Name { get; set; }
}
42. How do you perform a basic CRUD operation using Entity Framework?
Answer:
Use the DbContext to perform Create, Read, Update, and Delete operations on the database.
using (var context = new MyDbContext()) {
var product = new Product { Name = “Laptop” };
context.Products.Add(product); // Create
context.SaveChanges();
var products = context.Products.ToList(); // Read
product.Name = “Updated Laptop”; // Update
context.SaveChanges();
context.Products.Remove(product); // Delete
context.SaveChanges();
}
43. What is lazy loading in Entity Framework, and how do you enable or disable it?
Answer:
Lazy loading delays the loading of related data until it is explicitly accessed. Enable or disable it using LazyLoadingEnabled in the DbContext.
context.Configuration.LazyLoadingEnabled = false;
[/su_note]44. How do you handle database migrations in Entity Framework?
Answer:
Use Add-Migration and Update-Database commands in the Package Manager Console to handle schema changes and apply them to the database.
Add-Migration InitialCreate
Update-Database
45. How do you execute a raw SQL query in Entity Framework?
Answer:
Use the context.Database.SqlQuery<T>() method to execute a raw SQL query and return the result as a collection of entities.
var products = context.Database.SqlQuery<Product>(“SELECT * FROM Products”).ToList();
46. What is the Singleton pattern, and how do you implement it in C#?
Answer:
The Singleton pattern ensures a class has only one instance and provides a global point of access to it. Implement it using a private constructor and a static instance.
public class Singleton {
private static readonly Singleton instance = new Singleton();
private Singleton() { }
public static Singleton Instance {
get { return instance; }
}
}
47. How do you implement the Dependency Injection pattern in C#?
Answer:
Implement Dependency Injection by injecting dependencies through constructors, properties, or methods, often managed by an IoC container like Autofac or Unity.
public class MyClass {
private readonly IService _service;
public MyClass(IService service) {
_service = service;
}
}
48. What is the purpose of the SOLID principles in C# development?
Answer:
SOLID principles are guidelines for writing maintainable and scalable code, focusing on single responsibility, open-closed, Liskov substitution, interface segregation, and dependency inversion.
49. How do you implement the Factory Method pattern in C#?
Answer:
The Factory Method pattern defines an interface for creating an object but lets subclasses alter the type of objects that will be created.
public abstract class Creator {
public abstract IProduct FactoryMethod();
}
public class ConcreteCreator : Creator {
public override IProduct FactoryMethod() {
return new ConcreteProduct();
}
}
50. How do you ensure that a class implements multiple interfaces with the same method signature in C#?
Answer:
Use explicit interface implementation to ensure that each interface method is implemented separately, avoiding conflicts.
public class MyClass : IInterface1, IInterface2 {
void IInterface1.Method() {
// Implementation for IInterface1
}
void IInterface2.Method() {
// Implementation for IInterface2
}
}
Final Words
Getting ready for an interview can feel overwhelming, but going through these C# fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your C# interview but don’t forget to practice the C# basic syntax, object-oriented programming concepts, and .NET framework-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for C#?
Common interview questions for C# typically focus on object-oriented programming, syntax, exception handling, and the .NET framework.
2. What are the important C# topics freshers should focus on for interviews?
Important C# topics freshers should focus on include classes and objects, inheritance, polymorphism, delegates, and LINQ.
3. How should freshers prepare for C# technical interviews?
Freshers should prepare for C# technical interviews by practicing coding problems, reviewing OOP principles, and understanding the .NET framework.
4. What strategies can freshers use to solve C# coding questions during interviews?
Freshers can use strategies like breaking down the problem, using proper OOP practices, and testing edge cases to solve C# coding questions during interviews.
5. Should freshers prepare for advanced C# topics in interviews?
Yes, freshers should prepare for advanced C# topics in interviews if the role demands in-depth knowledge or if specified in the job description.
Explore More C# Resources
Explore More Interview Questions
Related Posts
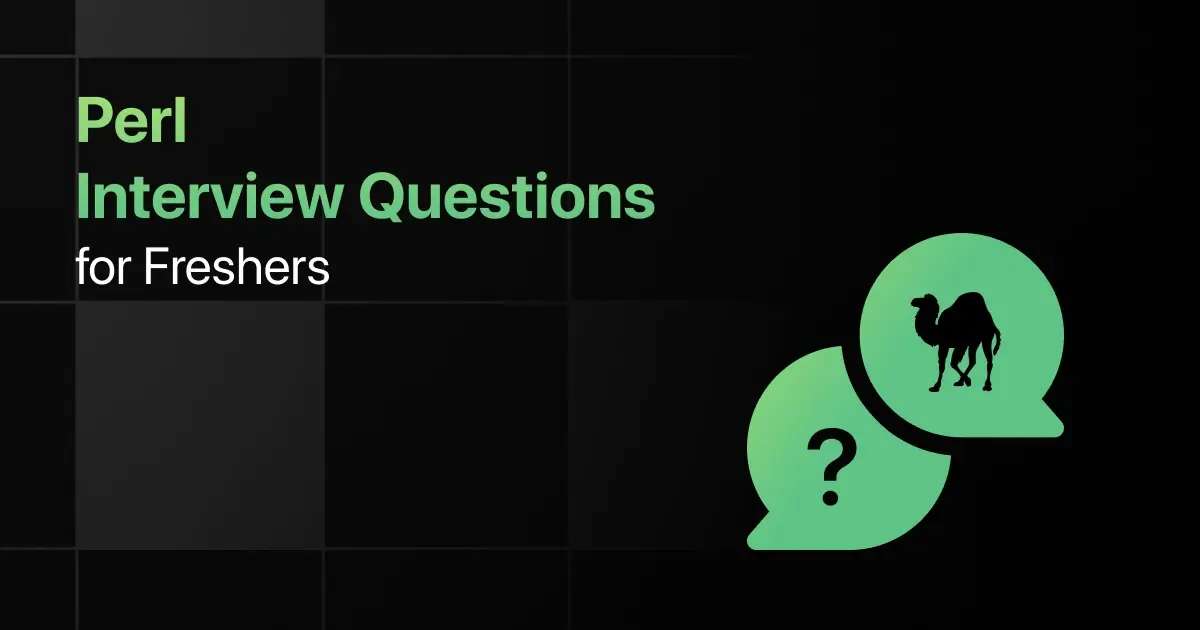
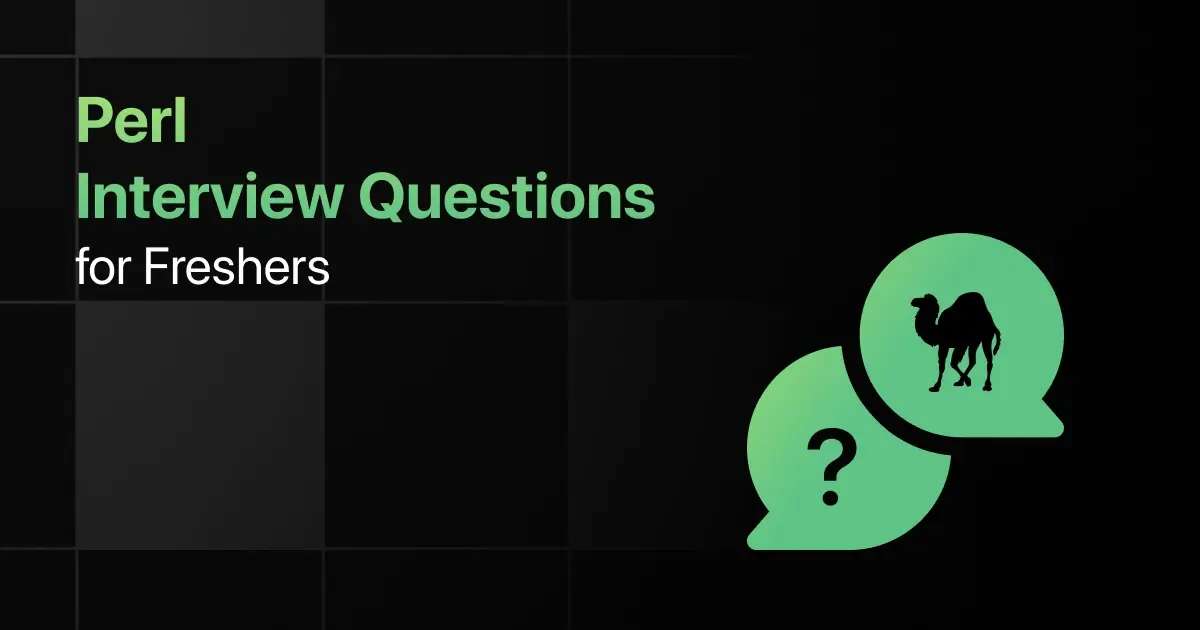
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …