Top ASP.NET MVC Interview Questions for Freshers
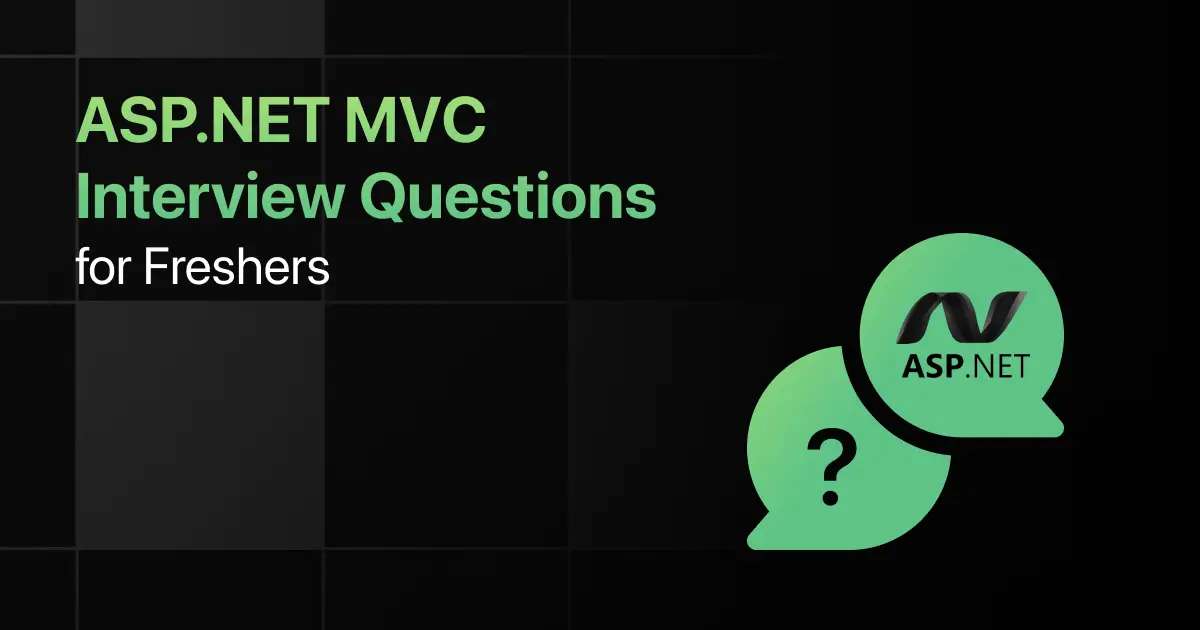
Are you preparing for your first ASP.NET MVC interview and wondering what questions you might face?
Understanding the key ASP.NET MVC interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these ASP.NET MVC interview questions and answers for freshers and make a strong impression in your interview.
Practice ASP.NET MVC Interview Questions and Answers
Below are the top 50 ASP NET MVC interview questions for freshers with answers:
1. What is ASP.NET MVC?
Answer:
ASP.NET MVC is a framework for building web applications using the Model-View-Controller architectural pattern. It separates an application into three main components: Model, View, and Controller.
This pattern helps to organize and manage complexity in large web applications by promoting loose coupling and separation of concerns.
2. What are the key components of the MVC architecture?
Answer:
The key components of MVC architecture are:
- Model: Represents the application’s data and business logic.
- View: Displays the data and user interface.
- Controller: Handles user requests, processes data via the model, and returns the view. This separation helps in managing the application efficiently and makes testing easier.
3. Explain the MVC request life cycle.
Answer:
The MVC request life cycle involves the following stages:
- Routing: The request is matched to a route in RouteConfig.
- Controller Initialization: The appropriate controller is selected based on the request.
- Action Execution: The controller’s action method is executed.
- View Rendering: The view associated with the action method is rendered.
- Response: The final HTML is returned to the browser.
4. What is Routing in MVC?
Answer:
Routing in ASP.NET MVC is used to map incoming browser requests to controller actions. The RouteConfig.cs file defines routes in the application, specifying patterns for URLs and associating them with the appropriate controller and action.
name: “Default”,
url: “{controller}/{action}/{id}”,
defaults: new { controller = “Home”, action = “Index”, id = UrlParameter.Optional }
);
5. What is the difference between ViewData, ViewBag, and TempData?
Answer:
- ViewData: A dictionary that stores data that is passed from the controller to the view. It is available only for the current request.
- ViewBag: A dynamic wrapper around ViewData. It allows you to pass data without casting or boxing.
- TempData: Stores data temporarily for the duration of a single request, allowing data to be passed between two actions.
6. What is a Partial View in MVC?
Answer:
A Partial View in MVC is a reusable portion of a web page. It is like a regular view but is intended to be rendered as part of another view. It allows you to reuse common components such as headers, footers, or menus across different views.
7. What is Model Binding in ASP.NET MVC?
Answer:
Model binding in MVC allows automatic mapping of data from HTTP requests (like form data, query strings) to action method parameters or model properties. When a user submits a form, the MVC framework binds the posted form values to the corresponding model properties or method parameters.
8. What is the purpose of ActionResult in MVC?
Answer:
ActionResult is the return type for controller actions that return results to the browser. It can represent different types of responses, such as views, JSON, files, or redirects. The flexibility of ActionResult allows controllers to return different types of responses without changing the method signature.
9. Explain the difference between ActionResult and ViewResult.
Answer:
ActionResult is a base class for various result types that a controller action can return, such as ViewResult, JsonResult, FileResult, etc.
ViewResult is a derived class of ActionResult specifically used for rendering a view.
{
return View(); // Returns a ViewResult
}
10. What is Html.BeginForm() in MVC?
Answer:
Html.BeginForm() is used to create a form in MVC views that posts data back to a controller action. It generates the HTML form element and accepts parameters like the controller name, action method, and form method.
{
// form elements
}
11. What is the purpose of ValidateAntiForgeryToken in MVC?
Answer:
[ValidateAntiForgeryToken] is used to prevent Cross-Site Request Forgery (CSRF) attacks. It ensures that form submissions come from the correct user by generating a token that is validated on form submission.
{
// form processing logic
}
12. What is Dependency Injection in ASP.NET MVC?
Answer:
Dependency Injection (DI) in MVC is a design pattern that allows objects to be passed as dependencies to other objects, promoting loose coupling. In MVC, DI is commonly used to inject services, such as logging or database contexts, into controllers.
{
private readonly IMyService _service;
public HomeController(IMyService service)
{
_service = service;
}
}
13. What is the difference between RedirectToAction and RedirectToRoute?
Answer:
- RedirectToAction: Redirects to a specified action method in a controller.
- RedirectToRoute: Redirects to a specified route, which may include a controller, action, and route parameters.
return RedirectToRoute(“Default”, new { controller = “Home”, action = “Index” });
14. What are Filters in ASP.NET MVC?
Answer:
Filters in MVC allow code to run before or after certain stages in the request processing pipeline. The common filter types include:
- Authorization Filters: Used to implement authentication and authorization.
- Action Filters: Used for pre- and post-processing of action methods.
- Result Filters: Applied before or after the execution of the action result.
- Exception Filters: Used to handle exceptions.
15. What is an Area in ASP.NET MVC?
Answer:
Areas are used to divide an ASP.NET MVC application into smaller functional units, like modules. Each area contains its own set of controllers, views, and models, making it easier to manage large applications by organizing related functionalities.
16. Explain Action Filters in MVC.
Answer:
Action Filters are custom attributes that allow logic to be executed before or after an action method is called. Examples include:
- OnActionExecuting: Runs before the action method.
- OnActionExecuted: Runs after the action method.
{
public override void OnActionExecuting(ActionExecutingContext filterContext)
{
// Code before action executes
}
}
17. What is the role of View Engine in ASP.NET MVC?
Answer:
A View Engine is responsible for rendering HTML from views in MVC. The default view engine in ASP.NET MVC is Razor (.cshtml files). View engines process view templates and generate HTML to send back to the client.
18. Explain Razor View Engine in ASP.NET MVC.
Answer:
Razor is the default view engine in ASP.NET MVC. It provides a streamlined syntax for embedding server-side code in HTML. Razor uses @ to transition from HTML to C# code. Razor views have .cshtml extensions and support C# and VB.NET.
19. How do you handle exceptions in ASP.NET MVC?
Answer:
Exceptions can be handled in MVC using:
- Try-catch blocks in the controller actions.
- Global filters like [HandleError] for handling unhandled exceptions.
- Custom error pages configured in web.config.
{
public ActionResult Index()
{
throw new Exception(“An error occurred”);
}
}
20. How do you implement authentication in ASP.NET MVC?
Answer:
Authentication in MVC can be implemented using:
- Forms Authentication: Configured in web.config.
- Windows Authentication: Integrated with Active Directory.
- OAuth/OpenID: External authentication providers like Google, Facebook, etc.
21. What is the difference between TempData, ViewData, and Session in MVC?
Answer:
- TempData: Stores data between two requests, mostly used for redirection.
- ViewData: Passes data from the controller to the view within a single request.
- Session: Stores user data throughout the user’s session, across multiple requests.
22. What are Bundling and Minification in MVC?
Answer:
Bundling and minification improve performance by reducing the number of HTTP requests and optimizing the size of files. Bundling combines multiple files (e.g., CSS or JS), while minification removes unnecessary characters, like whitespaces and comments.
23. What is AntiForgeryToken and why is it used in ASP.NET MVC?
Answer:
AntiForgeryToken is used to prevent CSRF (Cross-Site Request Forgery) attacks. It generates a hidden form field and a cookie that must match during form submissions, ensuring the request is legitimate.
24. What are strongly-typed views in MVC?
Answer:
A strongly-typed view in MVC is bound to a model, meaning the view expects a specific model type, allowing the use of IntelliSense and compile-time checking of the model properties.
<h1>@Model.Name</h1>
25. What is the difference between Html.RenderPartial and Html.Partial?
Answer:
- Html.Partial: Renders a partial view as a string and returns the output to the view.
- Html.RenderPartial: Renders a partial view directly to the response stream for better performance.
@{ Html.RenderPartial(“_PartialView”); }
26. What is the purpose of scaffolding in ASP.NET MVC?
Answer:
Scaffolding automatically generates code for the basic CRUD operations (Create, Read, Update, Delete) based on a model. It simplifies the creation of controllers and views for data operations.
27. What is ViewModel in ASP.NET MVC?
Answer:
A ViewModel is a model specifically created for a view. It contains data and properties that are required by the view but are not necessarily part of the database model. This allows decoupling the view from the domain model.
{
public string Name { get; set; }
public string Department { get; set; }
}
28. What is the role of Global.asax in ASP.NET MVC?
Answer:
Global.asax is used to define application-level events such as Application_Start, Application_End, and error handling. It is the entry point for the application and can be used to configure routing, filters, and global settings.
29. What is Unobtrusive JavaScript in MVC?
Answer:
Unobtrusive JavaScript in MVC separates JavaScript from the HTML markup by using data-* attributes in the markup. It enhances the clarity of the HTML and ensures cleaner code.
30. What is the purpose of the @Html.ValidationMessageFor helper method?
Answer:
@Html.ValidationMessageFor displays a validation message for a specific model property if the validation fails. It is used in forms to show field-specific error messages.
31. How do you implement AJAX in ASP.NET MVC?
Answer:
AJAX in MVC can be implemented using:
- jQuery AJAX: Using $.ajax() method to send asynchronous requests.
- AJAX Helpers: Built-in helpers like Html.BeginForm with AjaxOptions for creating asynchronous forms.
url: ‘/Controller/Action’,
type: ‘GET’,
success: function (data) {
// handle data
}
32. What is Remote Validation in ASP.NET MVC?
Answer:
Remote Validation is used to validate data asynchronously by sending a request to the server. It is commonly used for checking if a value already exists, like checking for duplicate usernames.
33. Explain the purpose of ViewStart.cshtml.
Answer:
_ViewStart.cshtml is used to define common layout settings for all views within a folder or an application. It is executed before any view is rendered, allowing you to set a common layout for the entire application.
34. What is ViewBag in ASP.NET MVC?
Answer:
ViewBag is a dynamic object used to pass data from a controller to a view. It does not require casting or boxing like ViewData and is useful for transferring small amounts of temporary data.
35. What is the role of ViewStart.cshtml in an MVC application?
Answer:
ViewStart.cshtml is a special file that defines a common layout for all views in an MVC application. It is executed before any other view file, making it the ideal place to specify the layout file.
36. How do you handle client-side validation in MVC?
Answer:
Client-side validation in MVC can be handled using:
- Data Annotations: Attributes on model properties.
- jQuery Unobtrusive Validation: Built-in jQuery library that automatically performs client-side validation based on data annotations.
37. What is the role of Data Annotations in MVC?
Answer:
Data annotations are used to validate user input. They are attributes applied to model properties to enforce validation rules, such as Required, StringLength, Range, etc.
38. What is a Layout Page in ASP.NET MVC?
Answer:
A layout page in MVC is similar to a master page in WebForms. It provides a consistent look and feel across multiple views. Content from the individual views is rendered within the layout’s content sections.
39. How do you implement authorization in ASP.NET MVC?
Answer:
Authorization in MVC can be implemented using:
- [Authorize] Attribute: To restrict access to certain actions or controllers based on the user’s authentication and roles.
- Custom Authorization Filters: For more granular access control.
40. Explain the purpose of RenderSection in MVC.
Answer:
RenderSection is used to define a section in a layout page that can be overridden by child views. Sections allow child views to inject content into specific parts of the layout.
}
41. What is the difference between @Html.Partial and @Html.RenderPartial?
Answer:
- @Html.Partial: Returns the rendered HTML string of a partial view and is used when you need to capture the result.
- @Html.RenderPartial: Writes directly to the response stream and is used for better performance when capturing the output is not necessary.
42. How does ASP.NET MVC handle sessions?
Answer:
Sessions in ASP.NET MVC can be handled using the HttpContext.Session object. It allows storing and retrieving user-specific data across requests.
43. What is the purpose of Authorize attribute in MVC?
Answer:
The [Authorize] attribute restricts access to actions or controllers based on user authentication and roles. Only authenticated users or those with specific roles can access certain parts of the application.
{
return View();
}
44. How do you implement file upload in ASP.NET MVC?
Answer:
File upload in MVC can be implemented using an HTML form with enctype=”multipart/form-data” and an HttpPostedFileBase parameter in the action method.
{
<input type=”file” name=”file” />
<input type=”submit” value=”Upload” />
}
45. What is TempData and how is it used?
Answer:
TempData is used to store data temporarily between two requests. It is mainly used for redirection scenarios, where data needs to persist between two different action methods.
return RedirectToAction(“Index”);
46. How do you manage large applications in ASP.NET MVC?
Answer:
In large applications, MVC promotes separation of concerns and modularity. Features like areas allow breaking down the application into smaller functional units, making the codebase easier to manage and scale.
47. What is the purpose of OutputCache in MVC?
Answer:
The OutputCache attribute caches the result of an action method and returns the cached output for subsequent requests, reducing the load on the server and improving performance.
{
return View();
}
48. How do you pass data from the controller to the view in ASP.NET MVC?
Answer:
Data can be passed from the controller to the view using:
- ViewData: Dictionary for passing data (requires casting).
- ViewBag: Dynamic object for passing data (no casting required).
- Model: Strongly typed object passed to the view.
49. Explain the difference between synchronous and asynchronous actions in MVC.
Answer:
Synchronous actions block the thread until the operation completes, while asynchronous actions allow the server to handle other requests while waiting for the operation to finish. Asynchronous actions are implemented using async and await keywords in MVC.
50. What are the advantages of using ASP.NET MVC over WebForms?
Answer:
- Separation of Concerns: MVC separates the model, view, and controller, making the application easier to manage.
- Testability: MVC is easier to test, especially with unit tests.
- Better control over HTML: MVC provides more control over HTML, CSS, and JavaScript.
- Supports TDD: MVC supports Test-Driven Development more effectively than WebForms.
Final Words
Getting ready for an interview can feel overwhelming, but going through these ASP NET MVC fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your ASP.NET MVC interview but don’t forget to practice ASP.NET MVC basics, routing, controller actions, and model-binding-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for ASP.NET MVC?
Common questions cover topics like the MVC architecture, routing, action methods, filters, and state management.
2. What are the important ASP.NET MVC topics freshers should focus on for interviews?
Freshers should focus on topics like MVC architecture, Razor views, routing, model binding, and dependency injection.
3. How should freshers prepare for ASP.NET MVC technical interviews?
Freshers should practice building simple MVC applications, understand the request lifecycle, and revise key concepts like models, views, and controllers.
4. What strategies can freshers use to solve ASP.NET MVC coding questions during interviews?
Break the problem into smaller parts, use clear code for controllers and views, and focus on understanding model binding and routing.
5. Should freshers prepare for advanced ASP.NET MVC topics in interviews?
Yes, freshers should also understand advanced topics like filters, dependency injection, and asynchronous actions for technical interviews.
Explore More Interview Questions
Related Posts
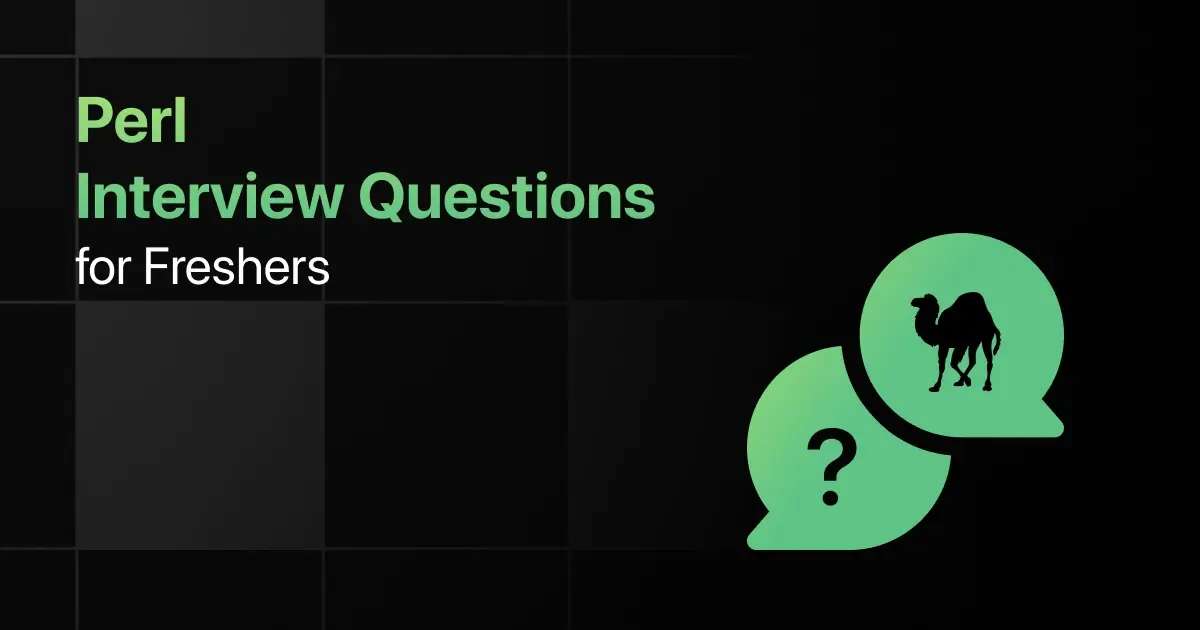
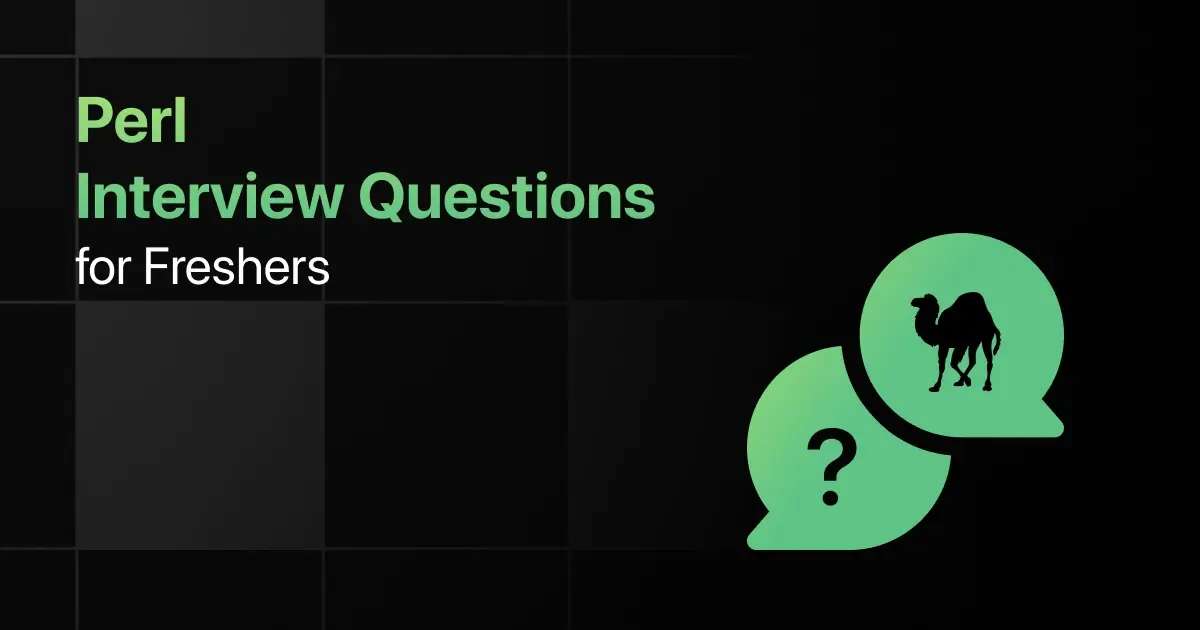
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …