Top ASP.NET Interview Questions for Freshers
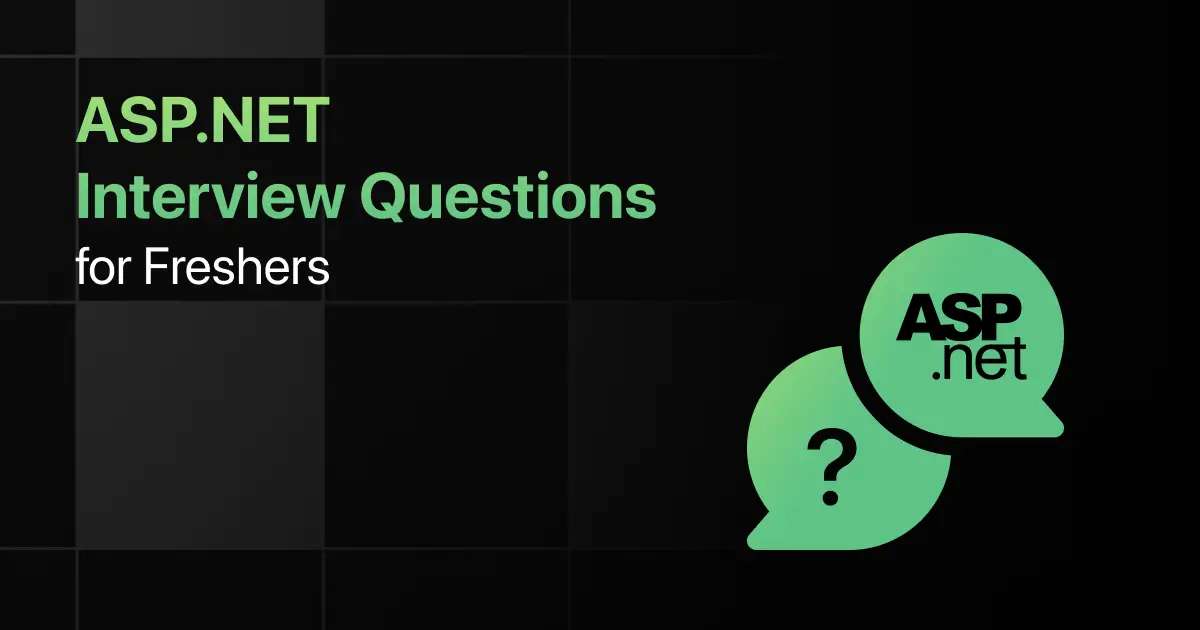
Are you preparing for your first ASP NET interview and wondering what questions you might face?
Understanding the key ASP NET interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these ASP NET interview questions and answers for freshers and make a strong impression in your interview.
Practice ASP.NET Interview Questions and Answers
Below are the top 50 ASP NET interview questions for freshers with answers:
1. What is ASP.NET?
Answer:
ASP.NET is a web framework developed by Microsoft for building web applications and services using .NET. It provides a robust environment for building dynamic websites, web services, and enterprise-level applications.
ASP.NET supports multiple languages like C# and VB.NET, and allows integration with various database systems. It also supports Model-View-Controller (MVC) architecture, which separates application logic, user interface, and input handling.
public class HomeController : Controller {
public IActionResult Index() {
return Content(“Hello World”);
}
}
2. What is the difference between ASP.NET Web Forms and ASP.NET MVC?
Answer:
ASP.NET Web Forms is an older framework for building web applications using a drag-and-drop model, while ASP.NET MVC provides a more flexible Model-View-Controller architecture.
MVC allows better separation of concerns and is easier to test compared to Web Forms. MVC also supports RESTful services and is highly customizable, while Web Forms is event-driven and ideal for RAD (Rapid Application Development).
public class ProductController : Controller {
public IActionResult List() {
return View(products);
}
}
3. What is a ViewState in ASP.NET Web Forms?
Answer:
ViewState is a method used in ASP.NET Web Forms to preserve the state of web controls between postbacks. It stores the values of controls as a hidden field within the page, allowing the state to be maintained.
Although useful, ViewState can increase page size and affect performance in large applications, making it less ideal for highly interactive pages.
ViewState[“Username”] = “JohnDoe”;
string username = ViewState[“Username”].ToString();
4. What is the purpose of a Master Page in ASP.NET?
Answer:
Master Pages in ASP.NET provide a template for the layout of a website. They allow developers to define a consistent look and feel across multiple pages while allowing individual pages to have unique content.
Master Pages help maintain uniformity and make changes easier, as any modification to the Master Page is reflected across all content pages.
<%@ Master Language=”C#” AutoEventWireup=”true” %>
<html>
<head>
<title><asp:ContentPlaceHolder ID=”TitleContent” runat=”server” /></title>
</head>
<body>
<asp:ContentPlaceHolder ID=”MainContent” runat=”server” />
</body>
</html>
5. Explain the ASP.NET page lifecycle.
Answer:
The ASP.NET page lifecycle consists of multiple stages such as initialization, loading, rendering, and unloading. Each phase provides an opportunity to manage page processing, like loading data, handling user input, and rendering output to the client.
The key stages include Page_Init, Page_Load, Page_PreRender, and Page_Unload.
protected void Page_Load(object sender, EventArgs e) {
if (!IsPostBack) {
// Code for first page load
}
}
6. What is a Postback in ASP.NET?
Answer:
A Postback in ASP.NET occurs when a web page sends data back to the server for processing, typically as a result of a form submission or control event. The server processes the request and returns a response, which updates the current page.
Postbacks can be triggered by actions like button clicks, form submissions, or other user interactions.
// Handle the Postback action
}
7. How does ASP.NET handle error handling?
Answer:
ASP.NET offers various ways to handle errors, such as using try-catch blocks, custom error pages, or global error handling via the Application_Error event in Global.asax.
Custom error pages can be configured in the web.config file, providing a user-friendly message for different error types.
try {
// Code that might throw an error
} catch (Exception ex) {
// Handle exception
}
8. What is a Web API in ASP.NET?
Answer:
A Web API in ASP.NET is a framework for building HTTP services that can be consumed by various clients like web browsers, mobile apps, and desktop applications. It allows for RESTful communication and is commonly used for building APIs for CRUD operations.
ASP.NET Web API supports various media types like JSON and XML, making it ideal for modern web services.
public class ProductsController : ApiController {
public IEnumerable<Product> GetProducts() {
return productList;
}
}
9. What are Bundling and Minification in ASP.NET MVC?
Answer:
Bundling and Minification are optimization techniques in ASP.NET MVC that reduce the number of HTTP requests and decrease file size. Bundling combines multiple files into a single file, while minification removes unnecessary characters (like whitespace) from files.
These techniques improve page load time and overall performance, especially for large-scale web applications.
bundles.Add(new ScriptBundle(“~/bundles/jquery”).Include(
“~/Scripts/jquery-{version}.js”));
10. How do you manage sessions in ASP.NET?
Answer:
Sessions in ASP.NET allow you to store user-specific data across multiple requests. You can manage session state using in-memory sessions, SQL Server, or other state providers.
Session data is often used for tracking user activity, maintaining authentication, or storing temporary information.
Session[“Username”] = “JohnDoe”;
string username = Session[“Username”].ToString();
11. What is the Global.asax file in ASP.NET?
Answer:
Global.asax is an optional file used to handle application-level events like Application_Start, Application_End, and Session_Start. It is part of the ASP.NET application lifecycle and allows you to manage global logic that applies to all users.
This file can also handle errors, logging, and other common processes.
void Application_Start(object sender, EventArgs e) {
// Code that runs on application startup
}
12. What are HTTP handlers and modules in ASP.NET?
Answer:
HTTP Handlers process HTTP requests at the lower level of the ASP.NET request pipeline. For example, they handle requests for specific resources like .aspx or .jpg files.
HTTP Modules are components that inspect or modify requests and responses. They are useful for handling security, logging, or custom header manipulation.
public class ImageHandler : IHttpHandler {
public void ProcessRequest(HttpContext context) {
context.Response.ContentType = “image/jpeg”;
context.Response.WriteFile(“image.jpg”);
}
}
13. How do you secure an ASP.NET application?
Answer:
ASP.NET applications can be secured using various techniques like authentication, authorization, HTTPS, and proper input validation. You can implement forms-based authentication, OAuth, or identity frameworks for user authentication.
In addition, it’s important to use HTTPS, handle cross-site scripting (XSS) attacks, and sanitize user inputs to prevent security vulnerabilities.
<authentication mode=”Forms”>
<forms loginUrl=”Login.aspx” timeout=”30″ />
</authentication>
14. What is the role of the web.config file in ASP.NET?
Answer:
The web.config file is an XML-based configuration file used to store application settings, database connection strings, authentication configurations, and error handling information for an ASP.NET application.
This file allows you to define settings for individual applications without modifying IIS settings.
<connectionStrings>
<add name=”MyDB” connectionString=”Data Source=server; Initial Catalog=db; Integrated Security=True” />
</connectionStrings>
</configuration>
15. What is Dependency Injection in ASP.NET Core?
Answer:
Dependency Injection (DI) is a design pattern used in ASP.NET Core to inject services or objects into classes, rather than having them created internally. It decouples dependencies from a class, making the system more modular, testable, and easier to maintain.
ASP.NET Core has built-in support for DI via its service container.
services.AddScoped<IMyService, MyService>();
16. What is the difference between ViewBag, ViewData, and TempData in ASP.NET MVC?
Answer:
ViewBag and ViewData are used to pass data from controllers to views, but ViewBag uses dynamic properties while ViewData uses key-value pairs. TempData, on the other hand, is used to pass data between two consecutive requests.
ViewBag and ViewData are for one-time transfers, while TempData persists data between actions or redirects.
ViewBag.Message = “Hello World”;
17. What is Razor in ASP.NET MVC?
Answer:
Razor is a markup syntax used in ASP.NET MVC to embed server-side code in web pages. It enables mixing C# or VB.NET with HTML.
Razor syntax simplifies development by using the @ symbol to embed code blocks, and it eliminates the need for complex directives.
var message = “Hello World”;
}
<h1>@message</h1>
18. What is the role of a Controller in ASP.NET MVC?
Answer:
A controller in ASP.NET MVC handles incoming requests, processes data, and returns the appropriate view or response. Controllers act as intermediaries between the model and view layers.
They contain action methods that correspond to user actions, such as loading pages, submitting forms, or performing CRUD operations.
public IActionResult Index() {
return View();
}
}
19. How can you perform model validation in ASP.NET MVC?
Answer:
Model validation is done using Data Annotations like [Required], [Range], or custom validation attributes. These annotations enforce rules like required fields or value ranges.
Upon submission, ASP.NET MVC checks the validity of the model, and errors can be displayed to the user if validation fails.
[Required] public string Name { get; set; }
}
20. What is the difference between TempData and Session in ASP.NET?
Answer:
TempData is used to store temporary data between consecutive requests, such as after a redirect. It is short-lived and only available during the request lifecycle.
Session, on the other hand, stores data for the entire user session and can persist between multiple requests throughout the session.
Session[“User”] = “JohnDoe”;
21. What is the purpose of middleware in ASP.NET Core?
Answer:
Middleware in ASP.NET Core is software that sits in the request pipeline and handles tasks such as authentication, logging, or error handling. Each middleware component can either process the request or pass it to the next middleware.
Middleware provides flexibility in managing the request-response process.
await next();
});
22. How do you handle AJAX requests in ASP.NET MVC?
Answer:
AJAX requests can be handled in ASP.NET MVC by using JsonResult to send JSON responses back to the client. JavaScript frameworks like jQuery can be used to make the AJAX call.
public JsonResult GetUserData(int id) {
var user = GetUserById(id);
return Json(user);
}
23. How do you prevent Cross-Site Request Forgery (CSRF) in ASP.NET MVC?
Answer:
ASP.NET MVC provides built-in protection against CSRF attacks using AntiForgery tokens. By using the @Html.AntiForgeryToken() in the form and [ValidateAntiForgeryToken] attribute in the controller, you can prevent malicious form submissions.
The token ensures that the form submission comes from the same source it was served to.
// Process form
}
24. What is the purpose of Entity Framework in ASP.NET?
Answer:
Entity Framework (EF) is an ORM (Object-Relational Mapper) that allows developers to interact with databases using .NET objects instead of writing SQL queries. It supports LINQ to query the database and allows for easier CRUD operations.
EF simplifies data access and reduces the amount of boilerplate code needed for database interactions.
public DbSet<User> Users { get; set; }
}
25. How do you enable routing in ASP.NET MVC?
Answer:
Routing in ASP.NET MVC is configured in the RouteConfig class. Routes define how URL patterns map to controller actions.
The default route follows the pattern {controller}/{action}/{id}, where controller and action names are provided as part of the URL.
name: “Default”,
url: “{controller}/{action}/{id}”,
defaults: new { controller = “Home”, action = “Index”, id = UrlParameter.Optional }
);
26. What is LINQ in ASP.NET?
Answer:
LINQ (Language Integrated Query) is a query language used to query collections, databases, XML, and more in a type-safe way. LINQ can be integrated with Entity Framework to simplify database queries.
It provides a uniform syntax across different data sources and supports filtering, ordering, and joining data.
where user.Age > 30
select user;
27. What is ASP.NET Identity?
Answer:
ASP.NET Identity is a membership system for ASP.NET applications that provides authentication, authorization, and user management features like user roles, claims, and password management.
ASP.NET Identity supports features like social login (Google, Facebook) and token-based authentication.
var user = new ApplicationUser { UserName = “JohnDoe” };
await UserManager.CreateAsync(user, “password123”);
28. What are Partial Views in ASP.NET MVC?
Answer:
Partial Views are reusable components within views, used to render sections of a webpage. They help break down complex views into smaller, manageable pieces.
Partial views are typically used for sections of a page that appear in multiple places, like a navigation bar or footer.
29. What is ASP.NET Core?
Answer:
ASP.NET Core is a cross-platform, high-performance framework for building modern, cloud-based web applications. It is a modular framework that provides more flexibility, improved performance, and can run on Windows, macOS, and Linux.
ASP.NET Core supports dependency injection, middleware, and a unified programming model for MVC and Web API.
public void ConfigureServices(IServiceCollection services) {
services.AddControllersWithViews();
}
}
30. What is a Tag Helper in ASP.NET Core?
Answer:
Tag Helpers in ASP.NET Core allow server-side code to participate in creating and rendering HTML elements. Tag Helpers are processed on the server and help make Razor views more readable and maintainable.
They allow you to work with HTML in a natural way while providing dynamic functionality like form generation, link generation, etc.
<input asp-for=”Name” />
</form>
31. What is the difference between ASP.NET Core and ASP.NET MVC 5?
Answer:
ASP.NET Core is a complete rewrite of the legacy ASP.NET framework, offering cross-platform support, better performance, and modularity. ASP.NET MVC 5, part of the .NET Framework, is limited to Windows and lacks the flexibility of Core.
ASP.NET Core merges MVC and Web API into a single framework, providing a more unified development experience.
public class Startup {
public void Configure(IApplicationBuilder app) {
app.UseRouting();
app.UseEndpoints(endpoints => {
endpoints.MapControllerRoute(“default”, “{controller=Home}/{action=Index}/{id?}”);
});
}
}
32. How do you implement logging in ASP.NET Core?
Answer:
ASP.NET Core includes built-in logging through the ILogger interface. You can log messages at various levels, such as Information, Warning, and Error.
Logging is useful for tracking the application state and diagnosing issues.
private readonly ILogger<HomeController> _logger;
public HomeController(ILogger<HomeController> logger) {
_logger = logger;
}
public IActionResult Index() {
_logger.LogInformation(“Loading home page”);
return View();
}
}
33. What is Kestrel in ASP.NET Core?
Answer:
Kestrel is a cross-platform web server for ASP.NET Core that is lightweight and fast. It is typically used as an internal server, behind a reverse proxy like Nginx or IIS, to handle requests in production environments.
Kestrel can serve HTTP requests directly and provides an option for high-performance hosting.
CreateHostBuilder(args).Build().Run();
}
34. How do you deploy an ASP.NET Core application to IIS?
Answer:
To deploy an ASP.NET Core app to IIS, you need to install the ASP.NET Core Hosting Bundle on the server. Publish the app using the `dotnet publish` command, and configure IIS to point to the `publish` folder. The app will run behind IIS, which acts as a reverse proxy to forward requests to Kestrel.
Ensure that the application pool in IIS is set to “No Managed Code” since ASP.NET Core handles its runtime.
35. What is the role of the IActionResult interface in ASP.NET Core?
Answer:
IActionResult is an interface in ASP.NET Core that defines the contract for action results returned by controllers. It allows you to return various types of responses, such as views, JSON, files, or redirects. It provides flexibility in handling different kinds of requests.
Using IActionResult, you can return different types of content based on conditions within the action method.
if (someCondition)
return View();
else
return Json(new { message = “Hello World” });
}
36. What are View Components in ASP.NET Core?
Answer:
View Components in ASP.NET Core are reusable, modular parts of a view, similar to partial views but more powerful. They allow you to encapsulate both logic and presentation. A View Component consists of a class and a corresponding view, enabling you to render dynamic content without full page reloads.
Unlike controllers, View Components don’t use routing. They are used within Razor views and return a ViewComponentResult.
public IViewComponentResult Invoke() {
var posts = GetRecentPosts();
return View(posts);
}
}
37. How does ASP.NET Core handle dependency injection (DI)?
Answer:
ASP.NET Core has built-in support for dependency injection (DI), where services are registered in the Startup.ConfigureServices() method and injected into controllers or other classes via constructors. It improves testability, modularity, and maintainability.
You can register services with different lifetimes: Transient, Scoped, and Singleton.
public void ConfigureServices(IServiceCollection services) {
services.AddScoped<IMyService, MyService>();
}
}
38. What is the purpose of appsettings.json in ASP.NET Core?
Answer:
appsettings.json is a configuration file in ASP.NET Core that stores application settings, such as database connection strings, API keys, and other environment-specific configurations. It is loaded during application startup, and its contents can be accessed via the Configuration object.
ASP.NET Core supports multiple environments and can use environment-specific configuration files like appsettings.Development.json.
“ConnectionStrings”: {
“DefaultConnection”: “Server=localhost;Database=app_db;Trusted_Connection=True;”
}
}
39. What is SignalR in ASP.NET Core?
Answer:
SignalR is a library in ASP.NET Core that enables real-time communication between server and client. It allows the server to push updates to connected clients instantly, making it ideal for chat apps, live feeds, notifications, and dashboards.
SignalR supports WebSockets, Long Polling, and Server-Sent Events, providing a fallback mechanism to ensure real-time communication regardless of the client’s capabilities.
services.AddSignalR();
app.UseEndpoints(endpoints => {
endpoints.MapHub<ChatHub>(“/chathub”);
});
40. How do you secure an ASP.NET Core Web API?
Answer:
ASP.NET Core Web APIs can be secured using techniques like JWT (JSON Web Token) authentication, OAuth, and API keys. The most common approach is using JWT Bearer tokens for stateless authentication, where the client sends a token with each request for verification.
You can also implement role-based and policy-based authorization for fine-grained access control.
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options => {
options.TokenValidationParameters = new TokenValidationParameters {
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(“YourSecretKey”))
};
});
41. What is Middleware in ASP.NET Core?
Answer:
Middleware in ASP.NET Core is a component that processes HTTP requests and responses. The middleware is executed in a pipeline, where each component can either process the request or pass it to the next middleware.
Middleware can perform tasks like authentication, logging, error handling, or serving static files. Custom middleware can be written and added to the pipeline in the Startup.Configure method.
private readonly RequestDelegate _next;
public CustomMiddleware(RequestDelegate next) {
_next = next;
}
public async Task Invoke(HttpContext context) {
// Custom logic before next middleware
await _next(context);
// Custom logic after next middleware
}
}
// Register middleware in Startup
app.UseMiddleware<CustomMiddleware>();
42. What are Filters in ASP.NET Core MVC?
Answer:
Filters in ASP.NET Core MVC allow you to execute custom logic before or after an action method executes. There are several types of filters: Authorization, Resource, Action, Exception, and Result filters. Filters are often used for logging, authentication, authorization, and error handling.
Filters can be applied globally, at the controller level, or at the action level.
public override void OnActionExecuting(ActionExecutingContext context) {
// Logic before action executes
}
public override void OnActionExecuted(ActionExecutedContext context) {
// Logic after action executes
}
}
// Apply filter to action method
[CustomActionFilter] public IActionResult Index() {
return View();
}
43. What is Docker and how can you use it to containerize an ASP.NET Core application?
Answer:
Docker is a platform for developing, shipping, and running applications in containers. Containers package an application and its dependencies, ensuring that it runs consistently across different environments.
To containerize an ASP.NET Core application, you create a Dockerfile that defines the application’s environment, build the image, and run it inside a container.
FROM mcr.microsoft.com/dotnet/aspnet:5.0 AS base
WORKDIR /app
EXPOSE 80
FROM mcr.microsoft.com/dotnet/sdk:5.0 AS build
WORKDIR /src
COPY . .
RUN dotnet restore
RUN dotnet publish -c Release -o /app
FROM base AS final
WORKDIR /app
COPY –from=build /app .
ENTRYPOINT [“dotnet”, “YourApp.dll”]
44. How do you implement global exception handling in ASP.NET Core?
Answer:
Global exception handling in ASP.NET Core can be achieved using middleware. By creating custom middleware, you can capture and log all unhandled exceptions across the entire application.
Additionally, the UseExceptionHandler() method can be used to specify a default error handling page or JSON response.
private readonly RequestDelegate _next;
public ExceptionHandlingMiddleware(RequestDelegate next) {
_next = next;
}
public async Task Invoke(HttpContext context) {
try {
await _next(context);
} catch (Exception ex) {
// Log the error
await HandleExceptionAsync(context, ex);
}
}
private Task HandleExceptionAsync(HttpContext context, Exception exception) {
context.Response.StatusCode = 500;
return context.Response.WriteAsync(“An error occurred”);
}
}
// Register middleware
app.UseMiddleware<ExceptionHandlingMiddleware>();
45. What is CORS and how do you enable it in ASP.NET Core?
Answer:
CORS (Cross-Origin Resource Sharing) is a security feature in web browsers that restricts web pages from making requests to a different domain. To enable CORS in ASP.NET Core, you use the AddCors() method in the Startup.ConfigureServices() method and configure it to allow or block specific domains, headers, and methods.
CORS is useful when building APIs that are consumed by front-end apps hosted on different domains.
services.AddCors(options => {
options.AddPolicy(“AllowSpecificOrigin”, builder =>
builder.WithOrigins(“http://example.com”)
.AllowAnyMethod()
.AllowAnyHeader());
});
app.UseCors(“AllowSpecificOrigin”);
46. What is Swagger and how do you integrate it with ASP.NET Core?
Answer:
Swagger is an open-source tool used to document and test RESTful APIs. ASP.NET Core provides the Swashbuckle package, which generates interactive API documentation based on your API’s controllers and actions.
Swagger allows users to explore and test your API endpoints directly from the browser.
app.UseSwagger();
app.UseSwaggerUI(c => {
c.SwaggerEndpoint(“/swagger/v1/swagger.json”, “My API V1”);
});
47. What is the role of ViewModel in ASP.NET MVC?
Answer:
A ViewModel is a class that represents data that you want to display on a view. It is a combination of multiple models or other data types that are tailored specifically for the view.
ViewModels help you separate logic and improve data representation by providing a custom structure for the data to be rendered in the UI.
public string Username { get; set; }
public int Age { get; set; }
}
48. How do you use Tag Helpers in ASP.NET Core?
Answer:
Tag Helpers in ASP.NET Core simplify the process of creating dynamic HTML elements. They provide server-side processing of HTML tags, allowing attributes and elements to be bound to server-side data.
Tag Helpers make Razor pages more readable and maintainable by providing a familiar HTML-like syntax for common server-side tasks, like form submission or routing.
<input asp-for=”Username” />
</form>
49. How do you perform caching in ASP.NET Core?
Answer:
ASP.NET Core supports both in-memory and distributed caching to store frequently accessed data, reducing the need to recreate or retrieve that data on every request. In-memory caching stores data in the server’s memory, while distributed caching can store it across different nodes.
Caching improves application performance by reducing the load on databases or external APIs.
_cache.Set(“cacheKey”, “cachedValue”, TimeSpan.FromMinutes(5));
50. What is Razor Pages in ASP.NET Core, and how is it different from MVC?
Answer:
Razor Pages is a new feature in ASP.NET Core that simplifies the structure of web applications by using page-based coding rather than controllers and actions. Razor Pages provides a more streamlined approach where each page has its model and view, reducing the complexity of small applications.
Unlike MVC, Razor Pages focuses on a “page” model, making it easier to develop simple or small-scale web apps.
public void OnGet() {
// Code to handle GET requests
}
}
Final Words
Getting ready for an interview can feel overwhelming, but going through these ASP.NET fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your ASP.NET interview, but don’t forget to practice ASP.NET basics, MVC architecture, and web API-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for ASP.NET?
Common ASP.NET interview questions focus on the ASP.NET lifecycle, MVC architecture, routing, controllers, views, action methods, and how ASP.NET handles sessions, authentication, and state management.
2. What are the important ASP.NET topics freshers should focus on for interviews?
Freshers should focus on topics like the ASP.NET page lifecycle, MVC architecture, routing, action filters, state management, authentication, and authorization. Understanding Web APIs and their integration with ASP.NET is also crucial.
3. How should freshers prepare for ASP.NET technical interviews?
Freshers should be prepared by understanding ASP.NET’s core concepts, coding simple MVC applications, and reviewing projects they have worked on. They should also practice coding with C# and be familiar with using Visual Studio for debugging and developing ASP.NET applications.
4. What strategies can freshers use to solve ASP.NET coding questions during interviews?
Freshers should start by understanding the problem clearly, break it down into smaller steps, and then apply appropriate ASP.NET principles such as controllers, views, and action methods. Leveraging debugging techniques in Visual Studio is key to solving ASP.NET coding problems.
5. Should freshers prepare for advanced ASP.NET topics in interviews?
Yes, while focusing on the basics is crucial, it’s helpful for freshers to also understand advanced topics such as dependency injection, middleware, asynchronous programming, and how to build RESTful APIs in ASP.NET for more technical roles.
Explore More Interview Questions
Related Posts
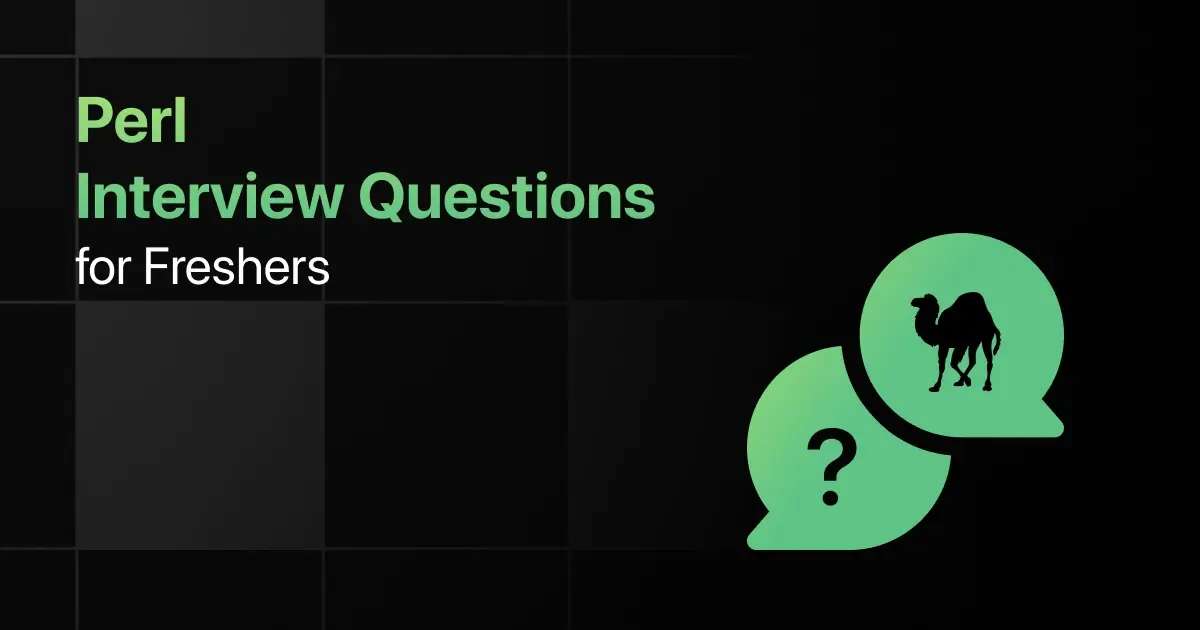
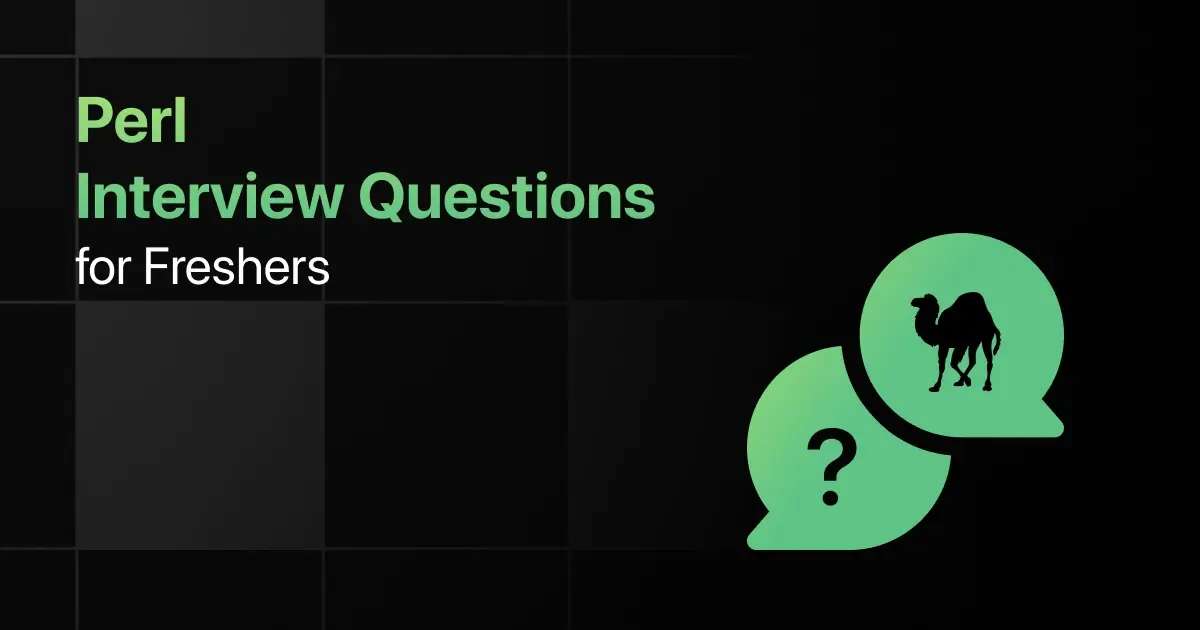
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …