Top Angular Interview Questions for Freshers
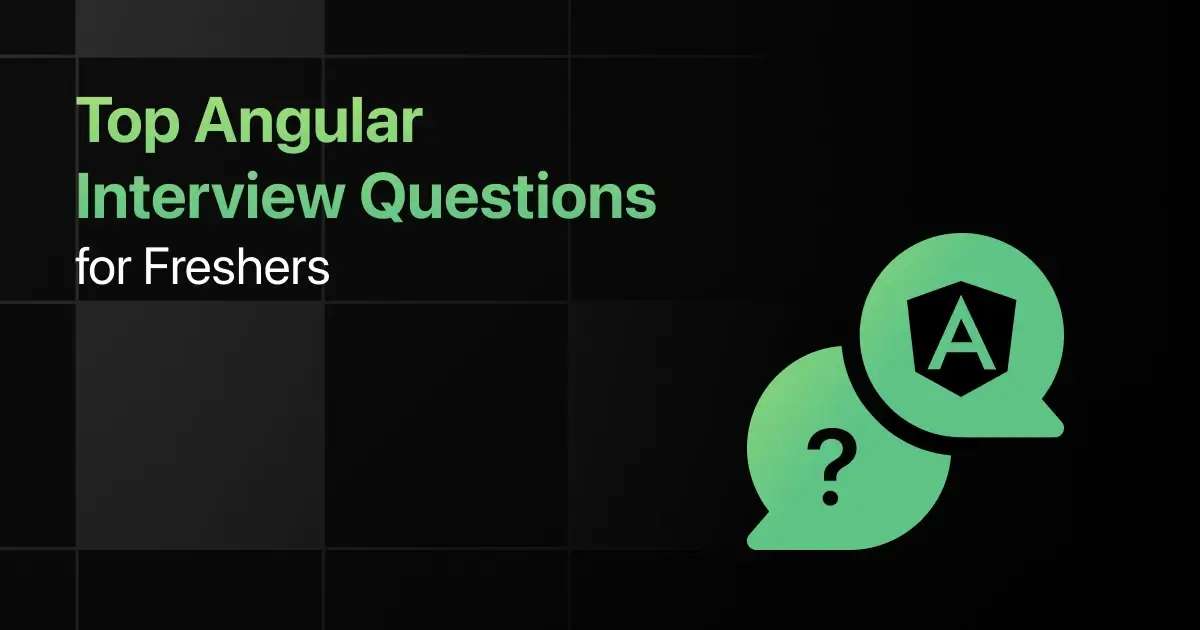
Are you preparing for your first Angular interview and wondering what questions you might face? Understanding the key Angular interview questions for freshers can give you more clarity.
This blog is here to help you get ready with practical questions that test your real-world problem-solving skills. We’ve gathered some of the most common basic Angular interview questions that freshers often encounter.
With this guide, you’ll be well-prepared to tackle these Angular interview questions and answers for freshers and make a strong impression in your interview.
Practice Angular Interview Questions and Answers
Below are the top 50 Angular interview questions for freshers with answers:
1. What is AngularJS, and what are its key features? Explain how AngularJS differs from other JavaScript frameworks.
Answer:
AngularJS is a structural framework for building dynamic web applications, offering features like two-way data binding, dependency injection, and directives.
2. How do you bootstrap an AngularJS application? Provide an example of an HTML page that bootstraps AngularJS manually.
Answer:
You can bootstrap an AngularJS application manually using angular.bootstrap() or automatically using the ng-app directive.
<!DOCTYPE html>
<html ng-app=”myApp”>
<head>
<script src=”https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js”></script>
<script>
var app = angular.module(‘myApp’, []);
</script>
</head>
<body>
<div>
<h1>Hello, AngularJS!</h1>
</div>
</body>
</html>
3. Explain the concept of the MVC architecture in AngularJS and how it benefits the development process.
Answer:
AngularJS follows the Model-View-Controller (MVC) architecture, separating concerns and making the code more modular and maintainable.
4. How do you initialize a new AngularJS module? Write the code to create a module named appModule.
Answer:
Initialize a module using angular.module().
var app = angular.module(‘appModule’, []);
5. What are the advantages of using AngularJS for web development? Discuss how AngularJS improves productivity and code maintainability.
Answer:
Advantages include two-way data binding, modularization, reusable components, and dependency injection, all of which improve productivity and maintainability.
6. What are AngularJS directives, and how do they differ from HTML attributes? Provide an example of a built-in directive.
Answer:
Directives extend HTML by adding behavior to elements; they differ from attributes by being dynamic and interactive.
<div ng-repeat=”item in items”>{{ item.name }}</div>
7. How do you create a custom directive in AngularJS? Provide an example that creates a custom directive to display a greeting message.
Answer:
Use the directive method to create a custom directive.
app.directive(‘greet’, function() {
return {
template: ‘<h1>Hello, {{ name }}!</h1>’,
scope: {
name: ‘@’
}
};
});
8. Explain the difference between element, attribute, and class directives in AngularJS. Provide examples for each.
Answer:
Element directives are used as HTML elements, attribute directives as attributes, and class directives as classes.
<!– Element directive –>
<my-directive></my-directive>
<!– Attribute directive –>
<div my-directive></div>
<!– Class directive –>
<div class=”my-directive”></div>
9. What is the role of the link function in an AngularJS directive? Provide an example where the link function manipulates the DOM.
Answer:
The link function is used to manipulate the DOM or register event listeners after the directive is linked to the element.
app.directive(‘highlight’, function() {
return {
link: function(scope, element, attrs) {
element.on(‘mouseenter’, function() {
element.css(‘color’, ‘blue’);
});
}
};
});
10. How do you use the restrict option in a directive to control how it can be used in an HTML document? Provide examples for E, A, C, and M restrictions.
Answer:
The restrict option specifies whether a directive is used as an element (E), attribute (A), class (C), or comment (M).
app.directive(‘myDirective’, function() {
return {
restrict: ‘E’, // Restrict as an element
template: ‘<div>Element Directive</div>’
};
});
11. What is the purpose of a controller in AngularJS? Explain how a controller interacts with the view and the model.
Answer:
Controllers handle the business logic and interact with the view and model by updating the view when the model changes.
12. How do you define a controller in AngularJS? Write an example of a simple controller that manages a list of items.
Answer:
Use controller method to define a controller.
app.controller(‘ItemController’, function($scope) {
$scope.items = [
{ name: ‘Item 1’ },
{ name: ‘Item 2’ }
];
});
13. Explain the concept of $scope in AngularJS. How does it enable two-way data binding between the controller and the view?
Answer:
$scope is an object that connects the controller and the view, enabling two-way data binding so that changes in the model are reflected in the view and vice versa.
14. How do you pass data to a controller in AngularJS? Provide an example of a controller that accepts a list of names as input.
Answer:
Data can be passed to a controller via the $scope object.
app.controller(‘NameController’, function($scope) {
$scope.names = [‘John’, ‘Jane’, ‘Doe’];
});
15. What is the $rootScope in AngularJS, and how does it differ from $scope? Provide an example where you use $rootScope to share data across multiple controllers.
Answer:
$rootScope is the parent scope of all other scopes and can be used to share data across multiple controllers.
app.controller(‘FirstController’, function($scope, $rootScope) {
$rootScope.sharedData = ‘Shared Value’;
});
app.controller(‘SecondController’, function($scope, $rootScope) {
$scope.data = $rootScope.sharedData;
});
16. Explain the concept of two-way data binding in AngularJS. How does it differ from one-way data binding?
Answer:
Two-way data binding automatically updates the view when the model changes and vice versa, while one-way data binding only updates the view when the model changes.
17. How do you implement one-way data binding in AngularJS? Provide an example where a change in the model updates the view.
Answer:
Use the {{ }} syntax for one-way data binding, which reflects model changes in the view.
<div>{{ item.name }}</div>
18. Write a simple AngularJS application that uses two-way data binding to update a user’s input in real time.
Answer:
Use the ng-model directive to bind the input field to a model.
<div ng-controller=”UserController”>
<input type=”text” ng-model=”user.name”>
<p>Hello, {{ user.name }}!</p>
</div>
19. How does AngularJS handle data binding for complex objects or nested properties? Provide an example of binding to a nested object.
Answer:
AngularJS allows binding to nested properties using dot notation.
<div ng-controller=”UserController”>
<input type=”text” ng-model=”user.details.name”>
<p>Name: {{ user.details.name }}</p>
</div>
20. What are AngularJS expressions, and how do they differ from JavaScript expressions? Provide examples of both.
Answer:
AngularJS expressions are written in double curly braces {{ }} and are evaluated against the $scope, while JavaScript expressions are evaluated in the global context.
<!– AngularJS Expression –>
<div>{{ 1 + 2 }}</div>
<!– JavaScript Expression –>
<script>document.write(1 + 2);</script>
21. What is an AngularJS module, and why is it important? Explain how modules help in organizing an AngularJS application.
Answer:
Modules are containers for different parts of an AngularJS application (controllers, services, filters, etc.), helping to organize code and manage dependencies.
22. How do you create a module in AngularJS, and how do you use it to encapsulate application components? Provide an example.
Answer:
Create a module using angular.module() and use it to define controllers, services, etc.
var app = angular.module(‘myApp’, []);
app.controller(‘MainController’, function($scope) {
$scope.message = ‘Hello, World!’;
});
23. Explain how to include one AngularJS module into another. Provide an example where appModule depends on sharedModule.
Answer:
Use the dependency injection mechanism to include one module into another.
var sharedModule = angular.module(‘sharedModule’, []);
var appModule = angular.module(‘appModule’, [‘sharedModule’]);
24. How do you configure a module in AngularJS? Provide an example of setting up a module configuration using the config method.
Answer:
Use the config method to configure a module during the configuration phase.
appModule.config(function($routeProvider) {
$routeProvider
.when(‘/’, {
templateUrl: ‘home.html’,
controller: ‘HomeController’
});
});
25. What are the different ways to bootstrap an AngularJS application? Discuss the pros and cons of each method.
Answer:
AngularJS can be bootstrapped manually using angular.bootstrap() or automatically using the ng-app directive. Automatic bootstrapping is simpler but less flexible, while manual bootstrapping offers more control.
26. What are AngularJS services, and how do they differ from controllers? Explain the role of services in an AngularJS application.
Answer:
Services in AngularJS are reusable singleton objects or functions used to organize and share code across the application, while controllers handle specific tasks and interact with the view.
27. How do you create a custom service in AngularJS? Provide an example of a service that calculates the square of a number.
Answer:
Create a custom service using the service or factory method.
app.service(‘MathService’, function() {
this.square = function(x) {
return x * x;
};
});
28. Explain the difference between a service, a factory, and a provider in AngularJS. Provide examples for each.
Answer:
A service is a constructor function, a factory returns an object or function, and a provider allows more configuration during the configuration phase.
// Service
app.service(‘MyService’, function() {
this.getData = function() {
return ‘Service Data’;
};
});
// Factory
app.factory(‘MyFactory’, function() {
return {
getData: function() {
return ‘Factory Data’;
}
};
});
// Provider
app.provider(‘MyProvider’, function() {
this.$get = function() {
return {
getData: function() {
return ‘Provider Data’;
}
};
};
});
29. How do you inject a service into a controller in AngularJS? Provide an example where a service is used to fetch data in a controller.
Answer:
Inject the service as a dependency in the controller.
app.controller(‘DataController’, function($scope, DataService) {
$scope.data = DataService.getData();
});
30. What are built-in AngularJS services, and how do they enhance application development? Discuss examples like $http, $q, and $timeout.
Answer:
Built-in services like $http for AJAX requests, $q for promise management, and $timeout for delayed execution, simplify common tasks and improve productivity.
31. What is dependency injection in AngularJS, and why is it important? Explain how dependency injection improves the testability and modularity of applications.
Answer:
Dependency injection (DI) in AngularJS automatically injects required services or objects into components, promoting modularity, reusability, and ease of testing.
32. How do you inject dependencies into an AngularJS controller? Provide an example where you inject both a custom service and a built-in service.
Answer:
Dependencies are injected into the controller function as arguments.
app.controller(‘MainController’, function($scope, $http, CustomService) {
// Use $http and CustomService in the controller
});
33. Explain the $injector service in AngularJS and how it is used for manual dependency injection. Provide an example of using $injector to get a service instance.
Answer:
The $injector service is used for manual dependency injection when automatic injection is not possible.
var injector = angular.injector([‘ng’, ‘myApp’]);
var myService = injector.get(‘MyService’);
34. How do you prevent minification issues with dependency injection in AngularJS? Provide an example using array syntax.
Answer:
Use the array syntax to prevent dependency injection issues during minification.
app.controller(‘MainController’, [‘$scope’, ‘$http’, function($scope, $http) {
// Controller code
}]);
35. What is the role of the $provide service in AngularJS, and how do you use it to create and configure services?
Answer:
$provide is used to create and configure services during the module configuration phase.
app.config(function($provide) {
$provide.service(‘MyService’, function() {
this.getData = function() {
return ‘Data’;
};
});
});
36. What is routing in AngularJS, and how does it enhance single-page application (SPA) development? Explain how routing works with views and controllers.
Answer:
Routing in AngularJS allows for navigation between different views within a single-page application without reloading the entire page.
37. How do you configure routes in AngularJS? Provide an example of a simple route configuration with two routes.
Answer:
Use the $routeProvider service to configure routes.
app.config(function($routeProvider) {
$routeProvider
.when(‘/home’, {
templateUrl: ‘home.html’,
controller: ‘HomeController’
})
.when(‘/about’, {
templateUrl: ‘about.html’,
controller: ‘AboutController’
})
.otherwise({
redirectTo: ‘/home’
});
});
38. Explain how to pass parameters in AngularJS routes. Provide an example where a route accepts an ID as a parameter.
Answer:
Use :param in the route path to define route parameters.
$routeProvider
.when(‘/user/:id’, {
templateUrl: ‘user.html’,
controller: ‘UserController’
});
39. How do you implement route guards in AngularJS to restrict access to certain routes? Provide an example using $routeChangeStart.
Answer:
Use the $routeChangeStart event to implement route guards.
app.run(function($rootScope, AuthService) {
$rootScope.$on(‘$routeChangeStart’, function(event, next, current) {
if (next.access && !AuthService.isLoggedIn()) {
event.preventDefault();
// Redirect to login
}
});
});
40. What is lazy loading in AngularJS, and how can it be implemented in routing to load modules on demand? Provide an example.
Answer:
Lazy loading in AngularJS defers the loading of modules until they are needed, improving performance.
$routeProvider
.when(‘/lazy’, {
templateUrl: ‘lazy.html’,
resolve: {
loadModule: function($ocLazyLoad) {
return $ocLazyLoad.load(‘lazyModule.js’);
}
}
});
41. What are AngularJS filters, and how do they enhance data presentation? Explain how filters can be used in expressions and directives.
Answer:
Filters format data before displaying it in the view, enhancing data presentation.
<div>{{ price | currency }}</div>
42. How do you create a custom filter in AngularJS? Provide an example of a filter that converts a string to uppercase.
Answer:
Use the filter method to create a custom filter.
app.filter(‘uppercase’, function() {
return function(input) {
return input.toUpperCase();
};
});
43. Explain the usage of built-in AngularJS filters like currency, date, and filter. Provide examples of each.
Answer:
Built-in filters provide common formatting functionalities like currency conversion, date formatting, and array filtering.
<div>{{ amount | currency }}</div>
<div>{{ date | date:’shortDate’ }}</div>
<div>{{ items | filter:searchText }}</div>
44. How do you apply multiple filters to a single expression in AngularJS? Provide an example that formats a number as currency and limits it to two decimal places.
Answer:
Chain multiple filters together using the pipe (|) symbol.
<div>{{ amount | currency | number:2 }}</div>
45. What is the filter filter in AngularJS, and how can it be used to filter an array of objects based on a search criteria? Provide an example.
Answer:
The filter filter searches through an array and returns elements that match the criteria.
<div ng-repeat=”item in items | filter:searchText”>{{ item.name }}</div>
46. How do you create a form in AngularJS and bind form inputs to the model using ng-model? Provide an example of a simple form with two input fields.
Answer:
Use ng-model to bind form inputs to the model.
<form ng-controller=”FormController”>
<input type=”text” ng-model=”user.name”>
<input type=”email” ng-model=”user.email”>
</form>
47. What are AngularJS form validation directives, and how do they simplify form validation? Discuss examples like ng-required, ng-minlength, and ng-pattern.
Answer:
Validation directives automatically apply validation rules to form inputs, reducing the need for custom validation logic.
<input type=”text” ng-model=”user.name” ng-required=”true”>
<input type=”email” ng-model=”user.email” ng-pattern=”/^[a-z]+@[a-z]+\.[a-z]{2,3}$/”>
48. How do you display validation error messages in AngularJS forms? Provide an example of a form with a required field that shows an error message if the field is empty.
Answer:
Use the ng-show directive to display error messages based on the form’s validation state.
<form name=”myForm”>
<input type=”text” name=”username” ng-model=”user.name” required>
<span ng-show=”myForm.username.$error.required”>This field is required.</span>
</form>
49. Explain the use of ng-submit in AngularJS forms. How does it differ from the standard submit event? Provide an example where ng-submit is used to handle form submission.
Answer:
ng-submit binds a function to the form’s submit event and prevents the default form submission behavior.
<form ng-submit=”submitForm()”>
<input type=”text” ng-model=”user.name”>
<button type=”submit”>Submit</button>
</form>
50. How do you handle form submission in AngularJS and reset the form after successful submission? Provide an example that resets the form fields after submission.
Answer:
Use ng-submit to handle submission and $setPristine to reset the form.
$scope.submitForm = function() {
if ($scope.myForm.$valid) {
// Submit the form data
$scope.user = {}; // Reset form data
$scope.myForm.$setPristine(); // Reset form state
}
};
Final Words
Getting ready for an interview can feel overwhelming, but going through these Angular fresher interview questions can help you feel more confident. This guide focuses on the kinds of Angular developer interview questions for fresher roles that you’re likely to face.
Don’t forget to practice the basic Angular coding interview questions too! With the right preparation, you’ll ace your Angular interview and take that important step in your career.
Frequently Asked Questions
1. What are the most common interview questions for Angular?
The most common interview questions for Angular typically cover topics like Angular components, data binding, directives, services, dependency injection, routing, and lifecycle hooks.
2. What are the important Angular topics freshers should focus on for interviews?
The important Angular topics freshers should focus on for interviews include understanding components, modules, services, routing, forms, RxJS (Reactive Extensions), and how to manage state and data flow in Angular applications.
3. How should freshers prepare for Angular technical interviews?
Freshers should prepare for Angular technical interviews by building small projects, practicing with common coding challenges, understanding core Angular concepts, and reviewing documentation and best practices.
4. What strategies can freshers use to solve Angular coding questions during interviews?
The strategies freshers can use to solve Angular coding questions during interviews include breaking down the problem, using Angular CLI commands efficiently, and writing modular, reusable code.
5. Should freshers prepare for advanced Angular topics in interviews?
Freshers should prepare for advanced Angular topics in interviews if time permits, but focusing on mastering the basics and understanding how to apply core concepts effectively should be the priority.
Explore More Angular Resources
Explore More Interview Questions
Related Posts
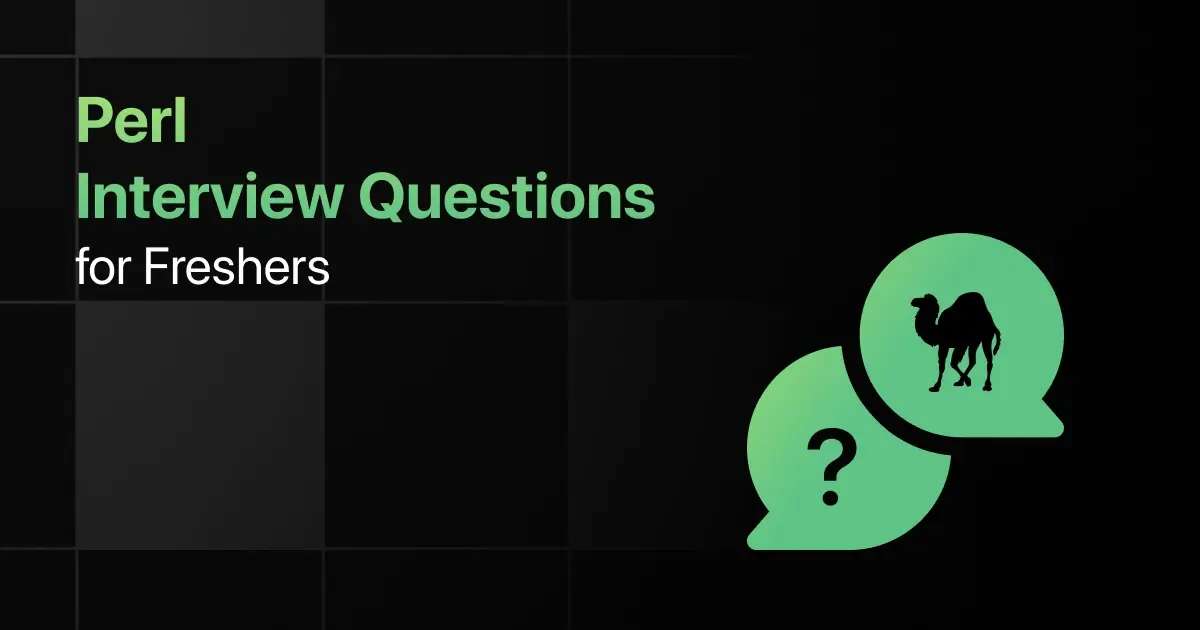
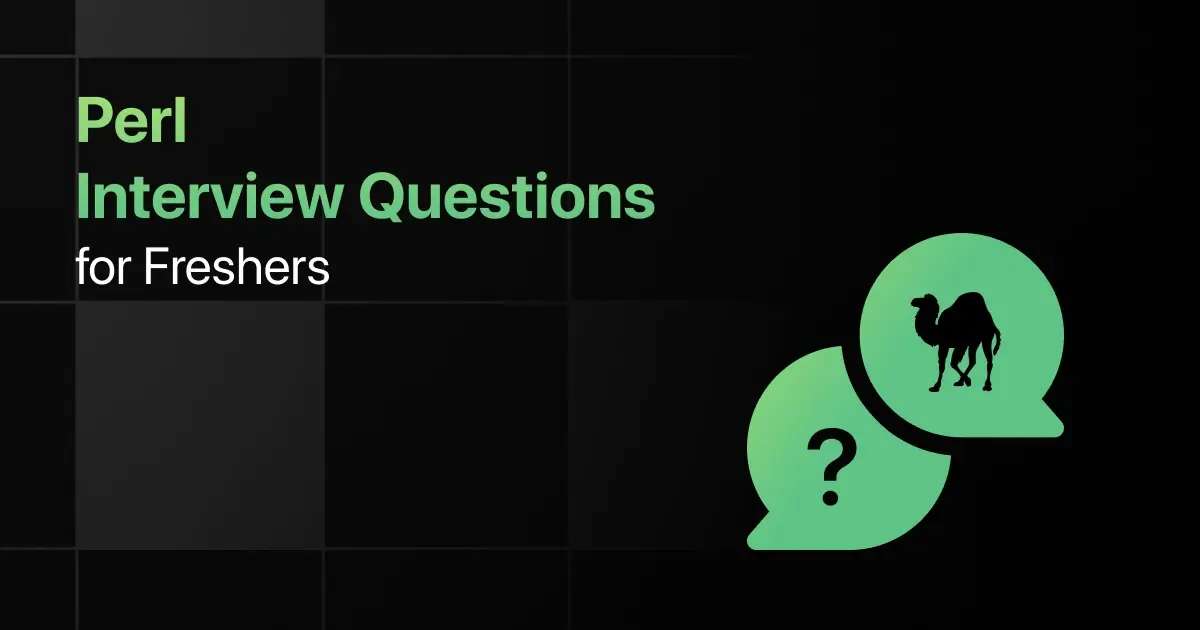
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …