Top Android Interview Questions for Freshers
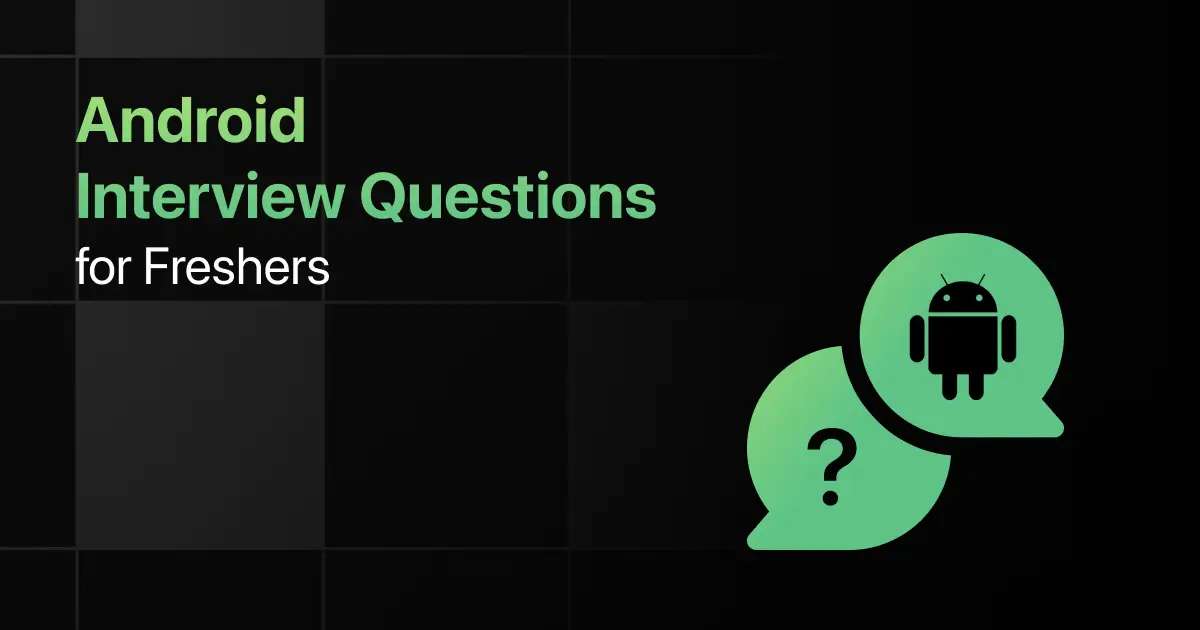
Are you preparing for your first Android interview and wondering what questions you might face?
Understanding the key Android interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Android interview questions and answers for freshers and make a strong impression in your interview.
Practice Android Interview Questions and Answers
Below are the top 50 Android interview questions for freshers with answers:
1. What is Android?
Answer:
Android is an open-source, Linux-based operating system primarily designed for mobile devices like smartphones and tablets. It provides a rich development environment for app developers, including a complete set of tools for building applications.
2. What is the Android Architecture?
Answer:
Android architecture is a stack of software components organized into four layers:
- Linux Kernel: Core services like memory management, security, and networking.
- Libraries: Android-specific libraries like OpenGL, WebKit, etc.
- Android Runtime (ART): Includes core libraries and the Dalvik/ART virtual machine.
- Application Framework: Exposes system services to apps.
- Applications: End-user apps like phones, email, browsers.
3. What is an Activity in Android?
Answer:
An Activity in Android represents a single screen with a user interface. It’s like a window in desktop applications and is the primary building block for creating an Android app.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
4. What is an Intent in Android?
Answer:
An Intent in Android is a messaging object used to request an action from another component (Activity, Service, etc.). It can be used for starting new activities, broadcasting messages, or communicating between components.
5. What is the difference between Implicit and Explicit Intent?
Answer:
- Explicit Intent: Directly specifies the target component (activity or service) to start.
- Implicit Intent: Declares an action to be performed, and the Android system determines which component to call based on available apps or services.
Intent intent = new Intent(this, SecondActivity.class);
startActivity(intent);
// Implicit Intent
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse(“http://www.google.com”));
startActivity(intent);
6. What is the Android Manifest file?
Answer:
The Android Manifest file (AndroidManifest.xml) is the configuration file that contains essential information about your app, like app components (activities, services, receivers), permissions, themes, and hardware/software features.
package=”com.example.app”>
<application
android:label=”@string/app_name”
android:icon=”@mipmap/ic_launcher”>
<activity android:name=”.MainActivity”>
<intent-filter>
<action android:name=”android.intent.action.MAIN” />
<category android:name=”android.intent.category.LAUNCHER” />
</intent-filter>
</activity>
</application>
</manifest>
7. What is a Service in Android?
Answer:
A Service is a background component that performs long-running operations without providing a user interface. It continues to run even when the user switches to another app.
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
// service code
return START_STICKY;
}
@Override
public IBinder onBind(Intent intent) {
return null;
}
}
8. What is the difference between ConstraintLayout and RelativeLayout in Android, and when would you use one over the other?
Answer:
ConstraintLayout is more flexible and allows you to create complex layouts with less nesting compared to RelativeLayout. It allows you to define relationships between elements using constraints. RelativeLayout relies on the relative positioning of views to one another.
ConstraintLayout is preferred when you need a flat hierarchy to optimize performance and want to create responsive UIs that adapt to different screen sizes without deeply nested layouts.
9. What is a Content Provider in Android?
Answer:
Content Providers manage access to a structured set of data and enable sharing data between apps. They use URIs to identify data and ContentResolver to query and manipulate data.
10. What is a Broadcast Receiver?
Answer:
A Broadcast Receiver responds to system-wide broadcast announcements, such as a system boot, network changes, or incoming SMS messages.
@Override
public void onReceive(Context context, Intent intent) {
// code to execute on broadcast
}
}
11. What is an Android Fragment?
Answer:
A Fragment is a modular section of an activity that has its own lifecycle and UI. Multiple fragments can be combined within one activity to create a dynamic UI.
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_layout, container, false);
}
}
12. What are SharedPreferences in Android?
Answer:
SharedPreferences are used to store small amounts of primitive data (key-value pairs) in an Android app. They are typically used to store user preferences or settings.
SharedPreferences.Editor editor = sharedPref.edit();
editor.putString(“username”, “JohnDoe”);
editor.apply();
13. Explain the Android Activity Lifecycle.
Answer:
The Android Activity Lifecycle includes the following key methods:
- onCreate(): Called when the activity is first created.
- onStart(): Called when the activity becomes visible to the user.
- onResume(): Called when the activity is in the foreground and interactive.
- onPause(): Called when another activity comes into the foreground.
- onStop(): Called when the activity is no longer visible.
- onDestroy(): Called when the activity is about to be destroyed.
14. What is the difference between onPause() and onStop()?
Answer:
- onPause(): Called when the activity is partially obscured by another activity, but still partially visible. The app is still active, but in the background.
- onStop(): Called when the activity is no longer visible, and the user has navigated away completely.
15. What is RecyclerView in Android?
Answer:
RecyclerView is a more advanced and flexible version of ListView. It provides an efficient way to display large sets of data with the help of ViewHolder pattern, which reduces the number of views inflated by reusing views.
recyclerView.setLayoutManager(new LinearLayoutManager(this));
recyclerView.setAdapter(new MyAdapter(myDataList));
16. What is a ViewHolder in RecyclerView?
Answer:
A ViewHolder holds references to the views for a single data item, preventing repeated calls to findViewById(). It improves the performance of RecyclerView by reusing views.
public TextView myTextView;
public MyViewHolder(View itemView) {
super(itemView);
myTextView = itemView.findViewById(R.id.textView);
}
}
17. What is a Handler in Android?
Answer:
A Handler is used to communicate between threads. It processes messages and runnable objects passed from other threads to update the UI thread.
handler.post(new Runnable() {
@Override
public void run() {
// update UI
}
});
18. What is the use of Looper in Android?
Answer:
Looper is a class used to run a message loop for a thread. It continuously loops and processes messages in a thread, which is especially useful for long-running tasks.
19. What is the ANR (Application Not Responding) error in Android?
Answer:
ANR occurs when the UI thread is blocked for too long (usually over 5 seconds). To prevent ANR, long-running operations (e.g., network requests) should be performed on a background thread rather than the main thread.
20. What is an AsyncTask?
Answer:
AsyncTask allows you to perform background operations and publish results on the UI thread without directly manipulating threads. It’s typically used for short background operations.
@Override
protected String doInBackground(Void… params) {
// background work
return “Result”;
}
@Override
protected void onPostExecute(String result) {
// update UI
}
}
21. What is Room Database in Android?
Answer:
Room is an abstraction layer over SQLite that simplifies database access in Android. It handles database creation, queries, and lifecycle management with annotations and DAO (Data Access Objects).
22. What is LiveData in Android?
Answer:
LiveData is an observable data holder class that respects the Android lifecycle. It ensures that UI components only observe data when they are active (started/resumed).
23. What is a ViewModel in Android?
Answer:
A ViewModel is designed to store and manage UI-related data in a lifecycle-conscious way. It survives configuration changes (e.g., screen rotation) and keeps the data for the activity or fragment.
private MutableLiveData text;
public LiveData getText() {
if (text == null) {
text = new MutableLiveData<>();
}
return text;
}
}
24. What is ViewStub and how does it improve layout performance in Android?
Answer:
ViewStub is an invisible, zero-sized view that can be used to load other layouts lazily. It is used to defer the loading and inflation of views until they are needed, which helps reduce initial layout inflation overhead.
Use ViewStub to optimize performance when you have parts of the layout that are rarely displayed.
android:id=”@+id/viewStub”
android:layout=”@layout/my_view”
android:layout_width=”wrap_content”
android:layout_height=”wrap_content”/>
25. What is Coroutines in Android?
Answer:
Coroutines are lightweight threads used for managing background tasks like network calls or database access in a non-blocking way. Coroutines simplify asynchronous programming in Kotlin.
26. What is the difference between LinearLayout and RelativeLayout?
Answer:
- LinearLayout: Arranges child views in a single row or column.
- RelativeLayout: Arranges child views relative to each other or to the parent container.
27. What is ConstraintLayout in Android?
Answer:
ConstraintLayout allows you to position and size widgets in a flexible way. It is more efficient than RelativeLayout because it reduces the hierarchy of views.
28. What are Broadcast Intent and Sticky Broadcast?
Answer:
- Broadcast Intent: A system-wide intent used to notify all interested apps when something interesting happens (e.g., battery low).
- Sticky Broadcast: Deprecated method used to keep the intent around after the broadcast is finished.
29. What is ProGuard in Android?
Answer:
ProGuard is a tool used to shrink, obfuscate, and optimize Android application code to make it smaller and harder to reverse engineer.
30. What is the difference between Service and Broadcast Receiver?
Answer:
- Service: Runs in the background to perform long-running operations without UI.
- Broadcast Receiver: Responds to system-wide broadcast messages or announcements.
31. What is a PendingIntent in Android?
Answer:
PendingIntent is a token that you give to another app (like a system service) to perform an action on your app’s behalf at a later time. It is commonly used with notifications and alarms.
32. What is Parcelable in Android?
Answer:
Parcelable is an Android interface used to serialize a class so its objects can be passed between activities via intents or saved in a bundle.
private String name;
protected MyModel(Parcel in) {
name = in.readString();
}
public static final Creator CREATOR = new Creator() {
public MyModel createFromParcel(Parcel in) {
return new MyModel(in);
}
};
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeString(name);
}
}
33. What is the difference between Parcelable and Serializable?
Answer:
- Parcelable: More efficient than Serializable for Android, but requires manual implementation.
- Serializable: Slower and more memory-intensive because it uses reflection.
34. What is Retrofit in Android?
Answer:
Retrofit is a popular HTTP client library for Android that simplifies network requests and RESTful API interaction by allowing you to convert JSON responses directly into POJOs.
35. What is Glide in Android?
Answer:
Glide is a powerful image loading library for Android. It fetches, decodes, and displays images from remote or local sources.
36. What is WorkManager in Android?
Answer:
WorkManager is a library for managing deferrable, asynchronous tasks that need guaranteed execution. It handles background work with constraints like network access or battery levels.
37. What is the difference between startActivity and startActivityForResult?
Answer:
- startActivity(): Starts a new activity without expecting any result in return.
- startActivityForResult(): Starts an activity and expects a result to be returned to the calling activity.
38. What is a ContentResolver in Android?
Answer:
ContentResolver provides access to content providers. It allows Android apps to query or modify data provided by content providers (e.g., querying contacts or media).
39. What are Loaders in Android?
Answer:
Loaders are used to load data asynchronously in activities and fragments. They automatically monitor data source changes and reconnect to the data when necessary.
40. What is the Android Jetpack?
Answer:
Android Jetpack is a suite of libraries, tools, and guidance to help developers write high-quality apps more easily. It includes components like LiveData, ViewModel, Navigation, and Room.
41. What is MVVM architecture in Android?
Answer:
MVVM (Model-View-ViewModel) is a design pattern that separates the UI (View), the business logic (ViewModel), and the data (Model). It promotes a clear separation of concerns and testability.
42. What are Annotations in Android?
Answer:
Annotations are metadata added to Java code to provide additional information to the compiler. They are used for things like dependency injection (@Inject), nullability checks (@NonNull), and database schemas (@Entity).
43. What is DataStore in Android?
Answer:
DataStore is a newer, faster, and more robust solution to SharedPreferences for storing small amounts of data in key-value pairs or typed objects.
44. What is a Snackbar in Android?
Answer:
Snackbar is a lightweight feedback message that briefly shows at the bottom of the screen. It’s an alternative to Toast but allows actions like “Undo.”
45. What is FusedLocationProviderClient?
Answer:
FusedLocationProviderClient is part of Google Play Services and provides a unified and battery-efficient API for getting location data on Android devices.
46. What is the difference between Service and IntentService?
Answer:
- Service: Runs on the main thread.
- IntentService: Runs on a separate worker thread, handling intents one by one.
47. What is the ActionBar?
Answer:
The ActionBar provides app branding, navigation, and access to actions such as the overflow menu. It can be customized or replaced with a Toolbar.
48. What is Deep Linking in Android?
Answer:
Deep linking is the process of directing a user to a specific activity or content within your app through a URL.
49. What is Navigation Component in Android?
Answer:
The Navigation Component is a part of Android Jetpack that simplifies navigation between fragments, activities, and deep links using a visual navigation graph.
50. What is Data Binding in Android?
Answer:
Data binding allows you to bind UI elements directly to data sources, making it easier to update the UI automatically when the data changes.
android:text=”@{viewModel.data}” />
Final Words
Getting ready for an interview can feel overwhelming, but going through these Android fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Android interview, but don’t forget to practice Android development basics, activity lifecycle, intents, and Android UI/UX-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for Android?
Common questions include topics like Android activity lifecycle, intents, fragments, services, and handling user inputs.
2. What are the important Android topics freshers should focus on for interviews?
Freshers should focus on topics like activities, fragments, intents, recyclerview, services, and handling data with SQLite or Room.
3. How should freshers prepare for Android technical interviews?
Freshers should build small Android projects, revise key Android components, and practice coding challenges related to layouts, activity lifecycle, and data management.
4. What strategies can freshers use to solve Android coding questions during interviews?
Understand the problem requirements, focus on breaking the problem into manageable parts, and use proper Android components like activities, fragments, and view models.
5. Should freshers prepare for advanced Android topics in interviews?
Yes, freshers should be familiar with advanced topics like Android Jetpack components, coroutines, and architecture patterns (e.g., MVVM) for more technical roles.
Explore More Interview Questions
Related Posts
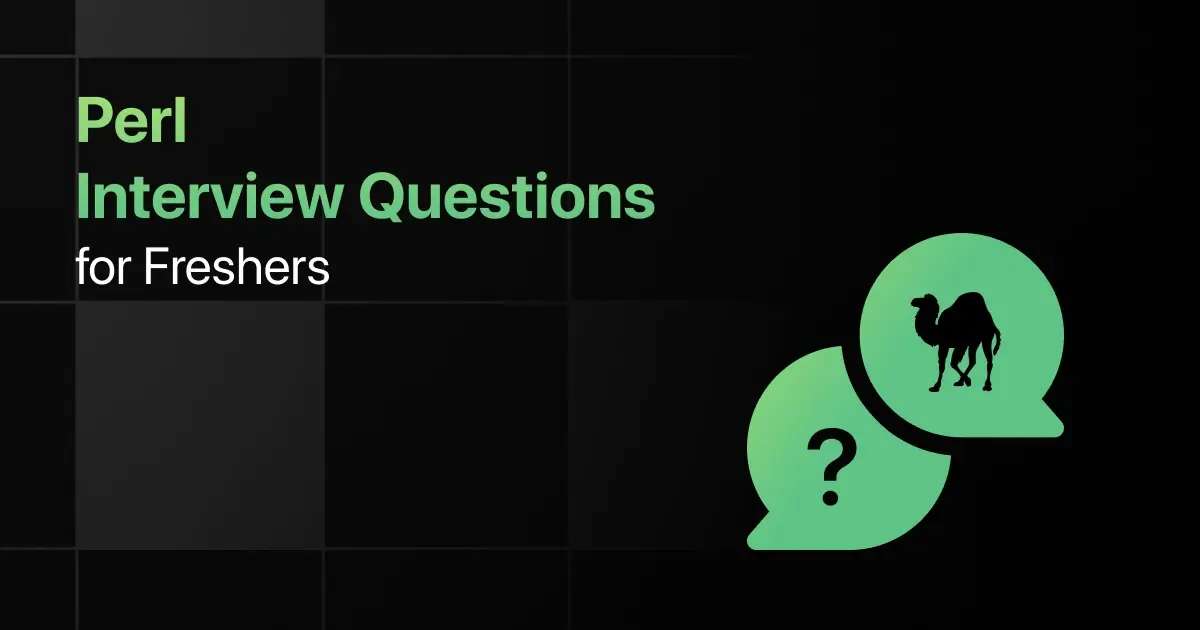
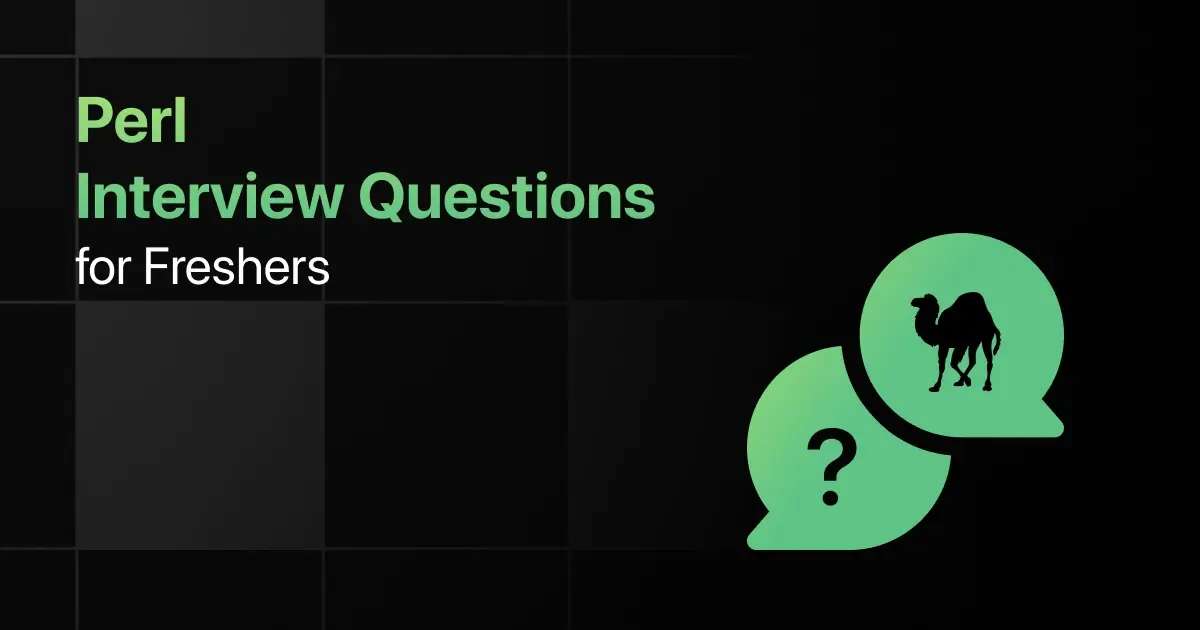
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …