Top React Redux Interview Questions for Freshers
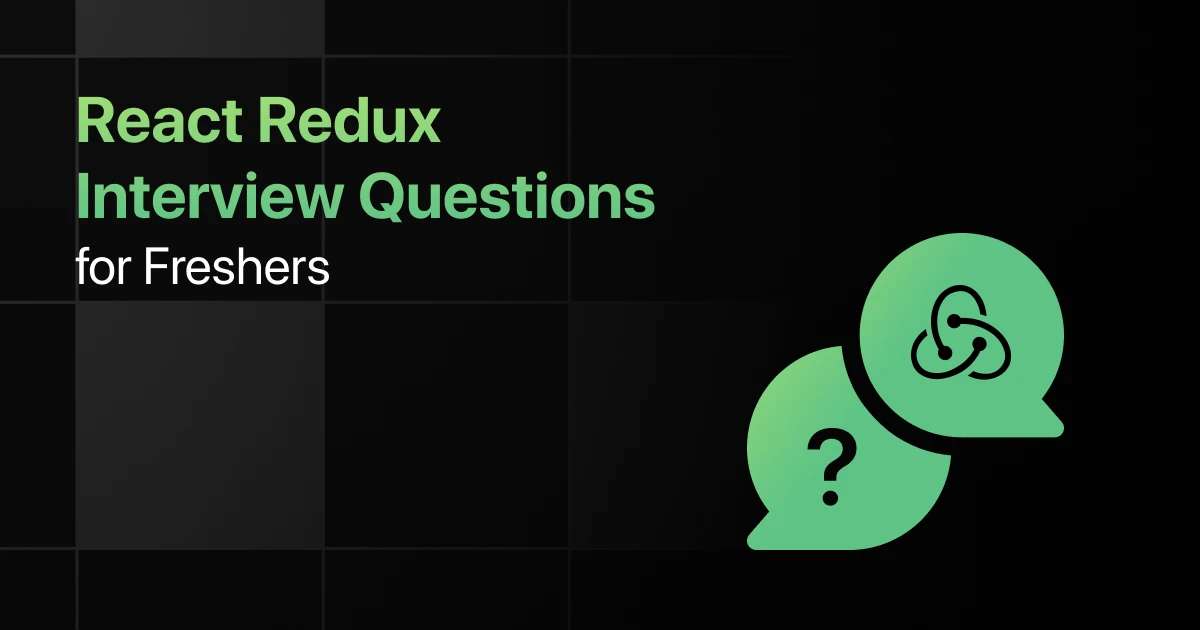
Are you preparing for your first React Redux interview and wondering what questions you might face?
Understanding the key React Redux interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these React Redux interview questions and answers for freshers and make a strong impression in your interview.
Practice React Redux Interview Questions and Answers
Below are the top 50 React Redux interview questions for freshers with answers:
1. What is Redux and why is it used in React applications?
Answer:
Redux is a state management library that centralizes application state, making it predictable and easier to debug. It helps manage complex states by providing a single source of truth for all state-related operations.
2. What are the core principles of Redux?
Answer:
Redux is based on three core principles: Single Source of Truth (the state is stored in one place), State is Read-Only (the state can only be changed by dispatching actions), and Pure Functions (reducers are pure functions).
3. How does the Redux data flow work?
Answer:
The Redux data flow is unidirectional: actions are dispatched to trigger changes, reducers handle these actions and return new state, and the store updates the view based on the new state.
4. What is an action in Redux?
Answer:
An action is a plain JavaScript object that describes a change or event in the application. Actions must have a type property, and they may also carry additional data in the form of payload.
5. What is a reducer in Redux?
Answer:
A reducer is a pure function that takes the current state and an action as arguments and returns a new state. Reducers handle how the state is updated in response to actions.
switch (action.type) {
case ‘INCREMENT’:
return state + 1;
case ‘DECREMENT’:
return state – 1;
default:
return state;
}
};
6. What is the Redux store?
Answer:
The Redux store holds the entire application state. It provides methods like getState() to access the current state, dispatch() to send actions, and subscribe() to listen for state updates.
7. How do you dispatch an action in Redux?
Answer:
Actions are dispatched using the dispatch() method of the store. Dispatching an action triggers the reducers to calculate a new state.
8. What is combineReducers() in Redux?
Answer:
combineReducers() is a utility function that merges multiple reducers into one. This is useful for managing different parts of the state with separate reducers.
9. How do you access the Redux state in a React component?
Answer:
Use the useSelector() hook to access the Redux state in a React component. It selects a slice of the state to use within the component.
10. What is useDispatch() in React Redux?
Answer:
useDispatch() is a hook that returns the Redux dispatch() function, which allows you to send actions from within a React component.
dispatch({ type: ‘INCREMENT’ });
11. How do you connect a class component to the Redux store?
Answer:
Use the connect() function from react-redux to connect a class component to the Redux store. mapStateToProps and mapDispatchToProps are used to map state and actions to the component’s props.
const mapDispatchToProps = (dispatch) => ({
increment: () => dispatch({ type: ‘INCREMENT’ })
});
export default connect(mapStateToProps, mapDispatchToProps)(CounterComponent);
12. What are middleware in Redux, and why are they important?
Answer:
Middleware in Redux are functions that sit between actions and reducers. They enable additional functionality such as logging, handling asynchronous actions, and modifying dispatched actions before they reach the reducers.
13. What is redux-thunk?
Answer:
redux-thunk is a middleware that allows you to write action creators that return a function instead of an action. This enables handling asynchronous operations like API calls.
return (dispatch) => {
fetch(‘api/data’)
.then(response => response.json())
.then(data => dispatch({ type: ‘DATA_LOADED’, payload: data }));
};
};
14. How do you handle side effects in Redux?
Answer:
Side effects, such as API calls, can be handled in Redux by using middleware like redux-thunk or redux-saga. These tools allow actions to trigger asynchronous logic while keeping the reducers pure.
15. What is the Provider component in React Redux?
Answer:
The Provider component wraps your React app and provides access to the Redux store for all connected components. It allows the React components to access the Redux state.
<App />
</Provider>
16. How can you optimize performance in Redux with useMemo()?
Answer:
Using useMemo() ensures that derived or computed values in a component are memoized, reducing unnecessary recalculations when the state changes.
computeExpensiveValue(counter), [counter]);
17. What is the reselect library and how does it improve performance in Redux?
Answer:
reselect is a library that helps create memoized selectors, which prevent unnecessary recalculations of derived state unless the inputs to the selector change. This improves performance when selecting data from the state.
[state => state.todos, state => state.filter],
(todos, filter) => todos.filter(todo => todo.status === filter)
);
18. How do you handle complex state in Redux?
Answer:
To handle complex state, divide the state into smaller slices, each managed by its reducer. Use combineReducers() to merge them into one root reducer.
19. What are selectors in Redux, and how are they used?
Answer:
Selectors are functions that extract and return specific pieces of state from the Redux store. They help keep component logic clean and reusable.
20. How do you update a nested state in Redux?
Answer:
To update nested state, use the spread operator or Object.assign() to maintain immutability. Always create new objects for the parts of the state that need updating.
switch (action.type) {
case ‘UPDATE_USER’:
return {
…state,
address: { …state.address, city: action.payload }
};
default:
return state;
}
};
21. What are action creators in Redux?
Answer:
Action creators are functions that return action objects. They encapsulate the creation of actions, making the code more reusable and reducing redundancy.
22. How do you reset the Redux state?
Answer:
You can reset the state by creating a root reducer that checks for a specific action type (RESET) and returns the initial state, effectively resetting the store.
if (action.type === ‘RESET’) return appInitialState;
return appReducer(state, action);
};
23. How do you persist Redux state across sessions?
Answer:
You can persist Redux state by using middleware like redux-persist. This library saves the Redux store to local storage or session storage and rehydrates it on app reload.
24. What is the difference between the local state and the Redux state?
Answer:
Local state is managed within individual React components using useState(), while Redux state is global, managed by the Redux store, and can be accessed by any component in the app.
25. How do you implement undo/redo functionality in Redux?
Answer:
To implement undo/redo, you can store the history of state changes in an array and create actions to revert or advance the state based on the history.
26. How does Redux handle immutability?
Answer:
Redux relies on immutability to ensure that state changes are predictable and easy to track. Reducers return new state objects instead of mutating the current state.
27. How do you batch multiple actions in Redux?
Answer:
You can batch multiple actions using libraries like redux-batched-actions or by dispatching them sequentially. Alternatively, middleware like redux-thunk allows action creators to dispatch multiple actions.
28. How do you handle errors in Redux?
Answer:
Errors can be handled by dispatching actions that represent failure states. For example, in an async action, dispatch an action like FETCH_FAILURE with an error message as the payload.
29. How do you manage multiple stores in Redux?
Answer:
While Redux encourages using a single store, in advanced cases, you can use multiple stores. However, this adds complexity to the app’s state management and should be avoided if possible.
30. How do you handle derived state in Redux?
Answer:
Derived state can be computed using selectors, often with libraries like reselect, to avoid duplicating logic and keeping the Redux state minimal.
31. What is time travel in Redux DevTools?
Answer:
Time travel is a feature in Redux DevTools that allows you to step forward and backward through dispatched actions, inspecting how the state changes over time.
32. How do you handle the authentication state in Redux?
Answer:
Authentication state, like isAuthenticated and user, can be managed in Redux by creating separate actions and reducers for login and logout, storing tokens or session information as part of the state.
33. How does Redux handle large datasets?
Answer:
To manage large datasets in Redux, you can implement pagination, lazy loading, or virtual scrolling to fetch and display data incrementally, reducing the memory footprint.
34. How do you structure a Redux project?
Answer:
A Redux project is typically structured with separate directories for actions, reducers, components, and selectors. Grouping related functionality together helps maintain code organization.
35. How do you use Redux with TypeScript?
Answer:
With TypeScript, you define types for actions, reducers, and state to ensure type safety. ActionCreators and Reducers can use TypeScript interfaces for better code maintainability.
36. What is the purpose of mapDispatchToProps in Redux?
Answer:
mapDispatchToProps is a function used with connect() to bind action creators to dispatch(), making them available as props to a React component.
37. What are higher-order reducers in Redux?
Answer:
Higher-order reducers are functions that take a reducer as an argument and return a new reducer. They can be used to add common behavior like resetting the state or logging actions.
38. How do you handle real-time updates in Redux?
Answer:
For real-time updates, such as WebSocket connections, you can dispatch actions inside WebSocket event handlers to update the Redux state with new data.
39. How do you avoid prop drilling with Redux?
Answer:
Redux eliminates the need for prop drilling by providing a global store that any component can connect to, bypassing the need to pass props through multiple levels of the component tree.
40. How do you implement optimistic updates in Redux?
Answer:
Optimistic updates involve updating the UI immediately before receiving a server response. You dispatch an optimistic action, and in case of failure, you revert the state with a rollback action.
41. How do you implement form handling in Redux?
Answer:
Form data can be managed in Redux by storing form input values in the state and dispatching actions on input changes or form submissions.
42. What is lazy loading in Redux, and why is it useful?
Answer:
Lazy loading allows you to load reducers and state only when needed, improving performance by reducing the initial load time of the app. You can add reducers dynamically when required.
43. How do you handle complex nested forms in Redux?
Answer:
For complex nested forms, you can manage each nested field as a separate slice of state, or use libraries like redux-form or formik for more advanced form handling.
44. How do you handle debouncing in Redux?
Answer:
To debounce actions, you can implement a middleware that delays action dispatches by a specified time interval, ensuring that actions are not dispatched too frequently.
45. What are atomic updates in Redux?
Answer:
Atomic updates ensure that only one piece of state is updated per action. This improves predictability and avoids race conditions when dealing with asynchronous actions.
46. How do you handle pagination in Redux?
Answer:
Pagination can be managed by storing the current page and total pages in the Redux state. Dispatch actions to fetch data for each page and update the state accordingly.
47. How do you handle data normalization in Redux?
Answer:
Data normalization flattens deeply nested structures into a normalized shape using libraries like normalizr, making it easier to manage complex state in Redux.
48. What is the duck pattern in Redux?
Answer:
The duck pattern groups action types, actions, and reducers into a single module. It organizes Redux code by feature rather than function, making it more modular and maintainable.
49. How do you implement undoable actions in Redux?
Answer:
Undoable actions can be implemented by keeping a history of past states and dispatching UNDO or REDO actions to traverse through the history stack.
50. What are store enhancers in Redux?
Answer:
Store enhancers are higher-order functions that extend or modify the Redux store’s functionality. Common examples include applying middleware or integrating DevTools.
Final Words
Getting ready for an interview can feel overwhelming, but going through these React Redux fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your React Redux interview but don’t forget to practice Redux architecture, actions, reducers, and state management-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for React Redux?
The most common React Redux interview questions focus on state management, actions, reducers, and the Redux data flow cycle.
2. What are the important React Redux topics freshers should focus on for interviews?
Freshers should focus on key React Redux topics like Redux architecture, connecting React components to the Redux store, and handling asynchronous actions with middleware like Redux Thunk.
3. How should freshers prepare for React Redux technical interviews?
Freshers should prepare by building simple React apps with Redux, practicing connecting state and dispatch to components, and understanding how to work with reducers and actions.
4. What strategies can freshers use to solve React Redux coding questions during interviews?
Freshers can solve React Redux coding questions by breaking down the state flow, focusing on managing state updates efficiently and debugging actions and reducers properly.
5. Should freshers prepare for advanced React Redux topics in interviews?
Yes, freshers should prepare for advanced React Redux topics like using Redux middleware, handling side effects, and optimizing performance with tools like Redux DevTools.
Explore More React Redux Resources
Explore More Interview Questions
- Python
- Java
- SQL
- React
- JavaScript
- C Programming
- HTML
- CSS
- Angular
- C++
- Spring Boot
- Node JS
- Excel
- C#
- DBMS
- PHP
- Linux
- Operating System
- MySQL
- Spring
- Flutter
- MongoDB
- Django
- React Native
- jQuery
- Bootstrap
- Embedded C
- DSA
- R Programming
- Hadoop
- .NET
- Power BI
- ASP.NET
- ASP.NET MVC
- Android
- Tableau
- MVC
- WordPress
- TypeScript
- Spark
- Kotlin
- Swift
- Golang
- Shell Scripting
- iOS
- Spring MVC
- Next JS
- AWS
- Kubernetes
- Docker
- Cyber Security
- Azure
- Terraform
- Vue JS
- UiPath
- Scala
- Flask
- RPA
- MATLAB
- Figma
Related Posts
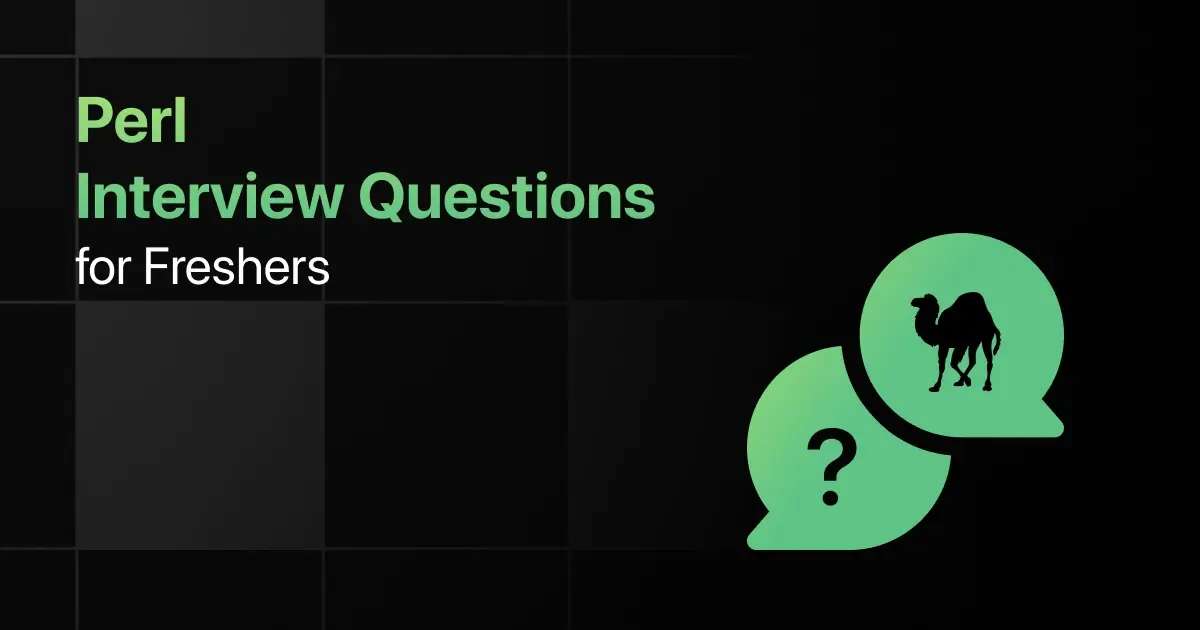
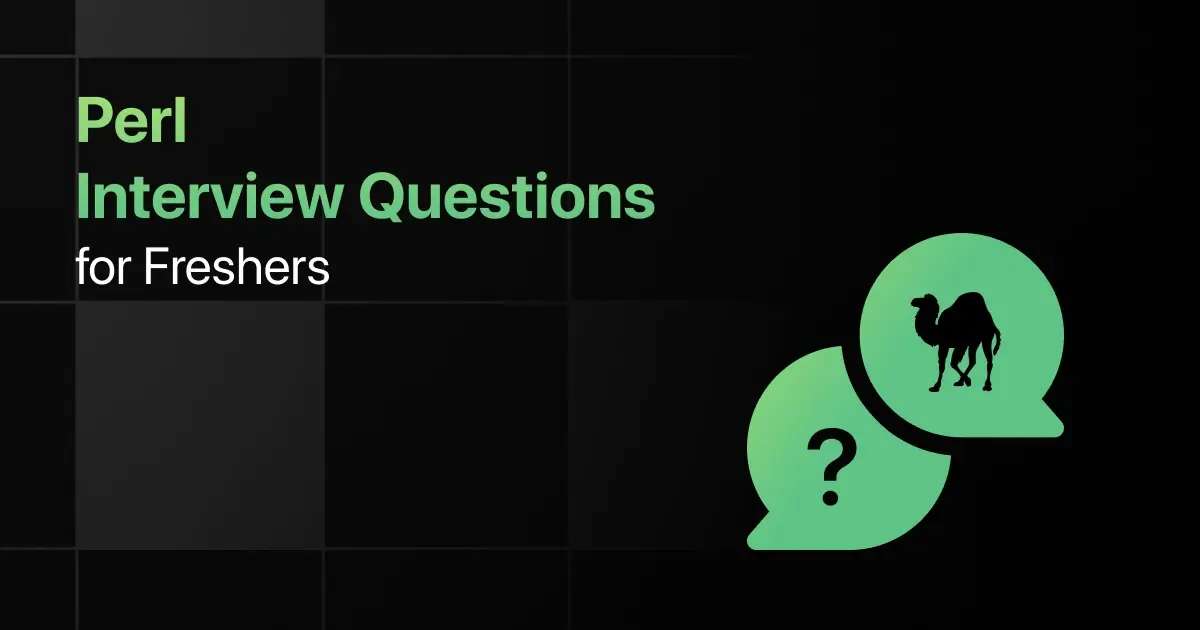
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …