Top MATLAB Interview Questions for Freshers
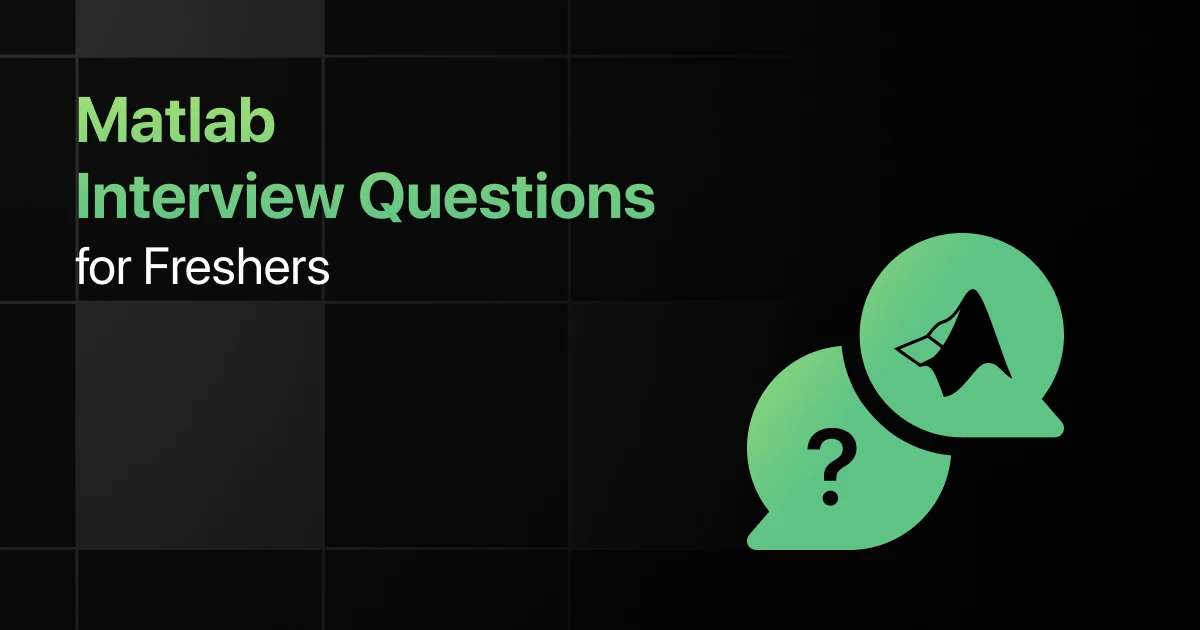
Are you preparing for your first MATLAB interview and wondering what questions you might face?
Understanding the key MATLAB interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these MATLAB interview questions and answers for freshers and make a strong impression in your interview.
Practice MATLAB Interview Questions and Answers
Below are the top 50 MATLAB interview questions for freshers with answers:
1. What is MATLAB used for?
Answer:
MATLAB is a high-level programming language and environment primarily used for numerical computing, data analysis, algorithm development, and visualization.
2. How do you define a variable in MATLAB?
Answer:
Variables are assigned using the = operator. MATLAB is dynamically typed, so no need to declare the variable type.
x = 5;
3. How do you create a 1D array (vector) in MATLAB?
Answer:
You create a row vector using square brackets with space or comma as separators.
v = [1, 2, 3, 4, 5];
4. What function is used to plot data in MATLAB?
Answer:
The plot() function is used to create 2D plots.
plot(x, y);
5. How do you create a 2D matrix in MATLAB?
Answer:
You can create a matrix using square brackets, where each row is separated by a semicolon.
M = [1, 2; 3, 4];
6. What are cell arrays in MATLAB?
Answer:
Cell arrays store data of varying types in MATLAB, where each cell can contain different data types.
C = {1, ‘text’, [3, 4]};
7. How do you find the size of a matrix?
Answer:
The size() function returns the dimensions of a matrix.
8. How do you transpose a matrix in MATLAB?
Answer:
The transpose operator (‘) is used to swap the rows and columns of a matrix.
T = M’;
9. How do you write a for-loop in MATLAB?
Answer:
For-loops are defined using the for keyword.
for i = 1:10
disp(i);
end
10. What is the difference between .* and * operators in MATLAB?
Answer:
.* performs element-wise multiplication, while * is used for matrix multiplication.
11. How do you define a function in MATLAB?
Answer:
Functions are defined using the function keyword.
function output = myFunction(input)
output = input * 2;
end
12. How do you read data from a CSV file in MATLAB?
Answer:
The readmatrix() function reads data from a CSV file.
data = readmatrix(‘file.csv’);
13. How do you add a title to a plot?
Answer:
Use the title() function to add a title to the plot.
title(‘My Plot’);
14. How do you concatenate two matrices vertically?
Answer:
You can concatenate matrices vertically using the semicolon ;.
A = [1, 2]; B = [3, 4];
C = [A; B];
15. How do you remove NaN values from a dataset?
Answer:
The isnan() function detects NaN values, and you can filter them out.
data = data(~isnan(data));
16. What is a script in MATLAB?
Answer:
A script is a file containing a sequence of MATLAB commands that can be executed in order.
17. How do you solve a system of linear equations in MATLAB?
Answer:
You can solve using the backslash operator \.
x = A \ b;
18. How do you generate random numbers in MATLAB?
Answer:
Use the rand() function to generate random numbers between 0 and 1.
r = rand(1, 5);
19. How do you use if-else conditions in MATLAB?
Answer:
If-else conditions are handled using the if, elseif, and else keywords.
if x > 0
disp(‘Positive’);
elseif x < 0
disp(‘Negative’);
else
disp(‘Zero’);
end
20. What is the purpose of hold on and hold off in MATLAB?
Answer:
hold on allows multiple plots to be overlaid on the same figure, while hold off ends this mode.
21. How do you calculate the mean of a vector?
Answer:
Use the mean() function to calculate the average of a vector.
avg = mean(v);
22. How do you clear all variables in the MATLAB workspace?
Answer:
The clear command removes all variables from the workspace.
clear;
23. How do you find the eigenvalues of a matrix?
Answer:
Use the eig() function to compute eigenvalues.
eigenValues = eig(A);
24. How do you append elements to an array in MATLAB?
Answer:
You can append using square brackets.
v = [v, 6];
25. How do you apply a function to each element of an array?
Answer:
Use the arrayfun() function to apply a function to each array element.
result = arrayfun(@(x) x^2, v);
26. What is the difference between length() and size() in MATLAB?
Answer:
length() returns the length of the largest dimension, while size() returns the size of each dimension.
27. How do you define global variables in MATLAB?
Answer:
Use the global keyword to declare a global variable.
global x;
28. How do you save variables to a file in MATLAB?
Answer:
The save() function stores variables to a file.
save(‘data.mat’, ‘x’);
29. How do you find the maximum value in a matrix?
Answer:
Use the max() function to find the maximum value.
maxValue = max(M(:));
30. How do you calculate the standard deviation of a vector?
Answer:
Use the std() function to compute the standard deviation.
s = std(v);
31. What is vectorization in MATLAB?
Answer:
Vectorization involves rewriting loops to operate on entire arrays, enhancing performance.
32. How do you find the inverse of a matrix?
Answer:
The inv() function computes the inverse of a matrix.
invA = inv(A);
33. How do you create a subplot in MATLAB?
Answer:
Use the subplot() function to create multiple plots in a figure.
subplot(2, 1, 1); plot(x1, y1);
subplot(2, 1, 2); plot(x2, y2);
34. How do you calculate the dot product of two vectors?
Answer:
Use the dot() function to compute the dot product.
result = dot(a, b);
35. How do you define a structure in MATLAB?
Answer:
Structures are created using the struct() function.
s = struct(‘field1’, 10, ‘field2’, ‘text’);
36. How do you create a 3D plot in MATLAB?
Answer:
Use the plot3() function to create 3D plots.
plot3(x, y, z);
37. How do you reshape a matrix in MATLAB?
Answer:
The reshape() function changes the dimensions of a matrix.
B = reshape(A, 2, 5);
38. What is the colon operator in MATLAB used for?
Answer:
The colon operator : is used for creating ranges and slicing arrays.
v = 1:10;
39. How do you calculate the cumulative sum of an array?
Answer:
Use the cumsum() function to compute the cumulative sum.
cumulativeSum = cumsum(v);
40. How do you create a logarithmic plot in MATLAB?
Answer:
Use the semilogx() or semilogy() functions for logarithmic plots.
semilogx(x, y);
41. How do you normalize data in MATLAB?
Answer:
Use the normalize() function to scale data.
normalizedData = normalize(data);
42. What is the linspace() function used for?
Answer:
linspace() generates linearly spaced vectors.
v = linspace(0, 1, 10);
43. How do you perform element-wise division in MATLAB?
Answer:
Use the ./ operator for element-wise division.
C = A ./ B;
44. How do you concatenate strings in MATLAB?
Answer:
Use square brackets or the strcat() function to concatenate strings.
s = strcat(‘Hello’, ‘ World’);
45. How do you calculate the FFT (Fast Fourier Transform) in MATLAB?
Answer:
Use the fft() function to compute the Fast Fourier Transform.
fftData = fft(signal);
46. How do you extract the diagonal elements of a matrix?
Answer:
Use the diag() function to extract or create diagonal matrices.
d = diag(A);
47. How do you generate a mesh grid in MATLAB?
Answer:
Use the meshgrid() function to create 2D grids for 3D plotting.
48. How do you export a figure as an image?
Answer:
Use the saveas() function to save a figure as an image.
saveas(gcf, ‘plot.png’);
49. How do you compute numerical integration in MATLAB?
Answer:
Use the integral() function to compute definite integrals.
result = integral(@(x) x.^2, 0, 1);
50. How do you find the roots of a polynomial in MATLAB?
Answer:
Use the roots() function to find the polynomial’s roots.
r = roots([1, -3, 2]);
Final Words
Getting ready for an interview can feel overwhelming, but going through these MATLAB fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your MATLAB interview but don’t forget to practice MATLAB’s programming environment, matrix manipulations, and data visualization-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for MATLAB?
The most common MATLAB interview questions are matrix manipulations, plotting functions, and control flow structures.
2. What are the important MATLAB topics freshers should focus on for interviews?
Freshers should focus on MATLAB basics like arrays, matrices, data visualization, and script writing.
3. How should freshers prepare for MATLAB technical interviews?
Freshers should prepare by practicing common MATLAB commands, solving numerical problems, and working with MATLAB’s built-in functions.
4. What strategies can freshers use to solve MATLAB coding questions during interviews?
Freshers can solve MATLAB coding questions by first understanding the problem, leveraging built-in functions, and breaking the solution into smaller steps.
5. Should freshers prepare for advanced MATLAB topics in interviews?
Yes, freshers should prepare for advanced topics like Simulink, toolboxes, and MATLAB’s optimization techniques.
Explore More MATLAB Resources
Explore More Interview Questions
- Python
- Java
- SQL
- React
- JavaScript
- C Programming
- HTML
- CSS
- Angular
- C++
- Spring Boot
- Node JS
- Excel
- C#
- DBMS
- PHP
- Linux
- Operating System
- MySQL
- Spring
- Flutter
- MongoDB
- Django
- React Native
- jQuery
- Bootstrap
- Embedded C
- DSA
- R Programming
- Hadoop
- .NET
- Power BI
- ASP.NET
- ASP.NET MVC
- Android
- Tableau
- MVC
- WordPress
- TypeScript
- Spark
- Kotlin
- Swift
- Golang
- Shell Scripting
- iOS
- Spring MVC
- Next JS
- AWS
- Kubernetes
- Docker
- Cyber Security
- Azure
- Terraform
- Vue JS
- UiPath
- Scala
- Flask
- RPA
Related Posts
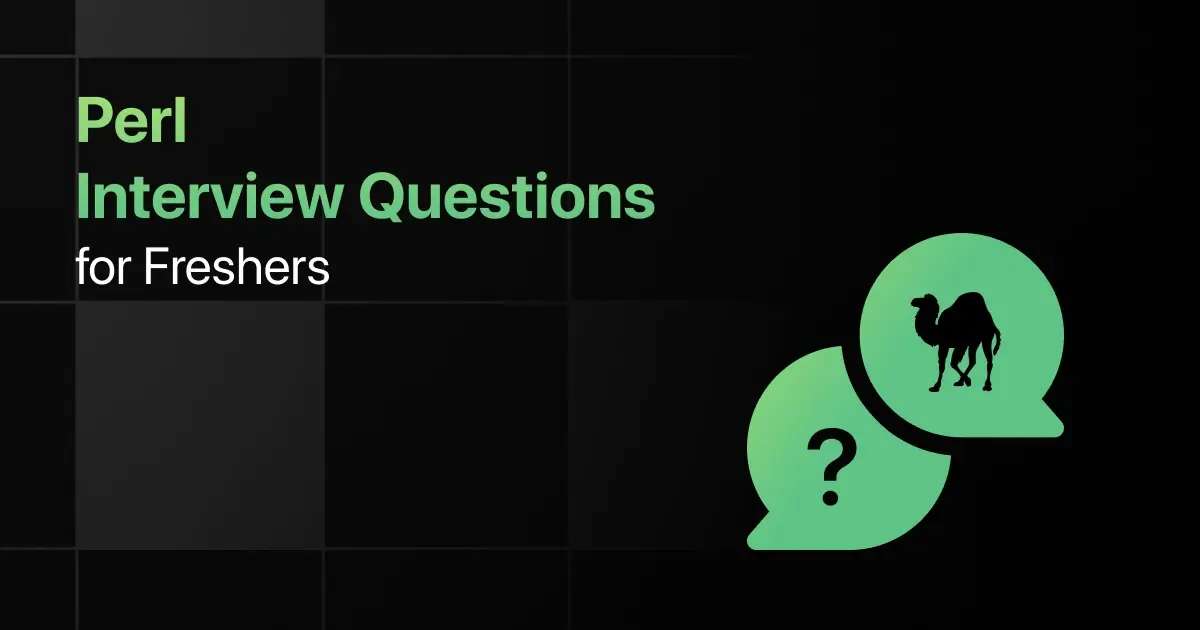
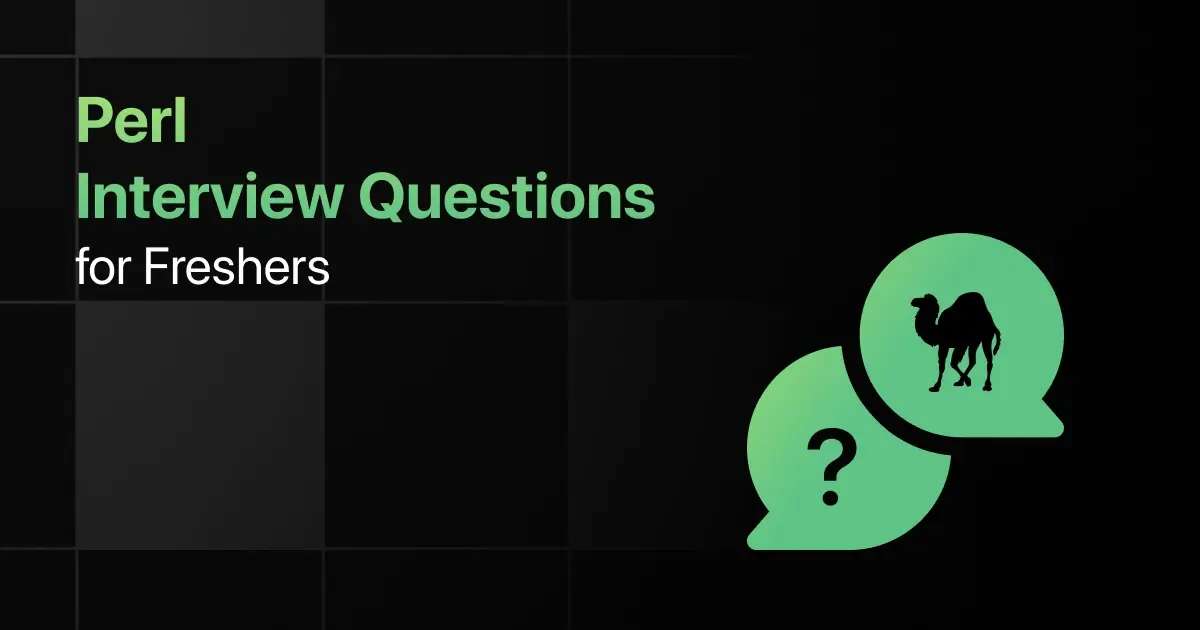
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …