Top Next JS Interview Questions for Freshers
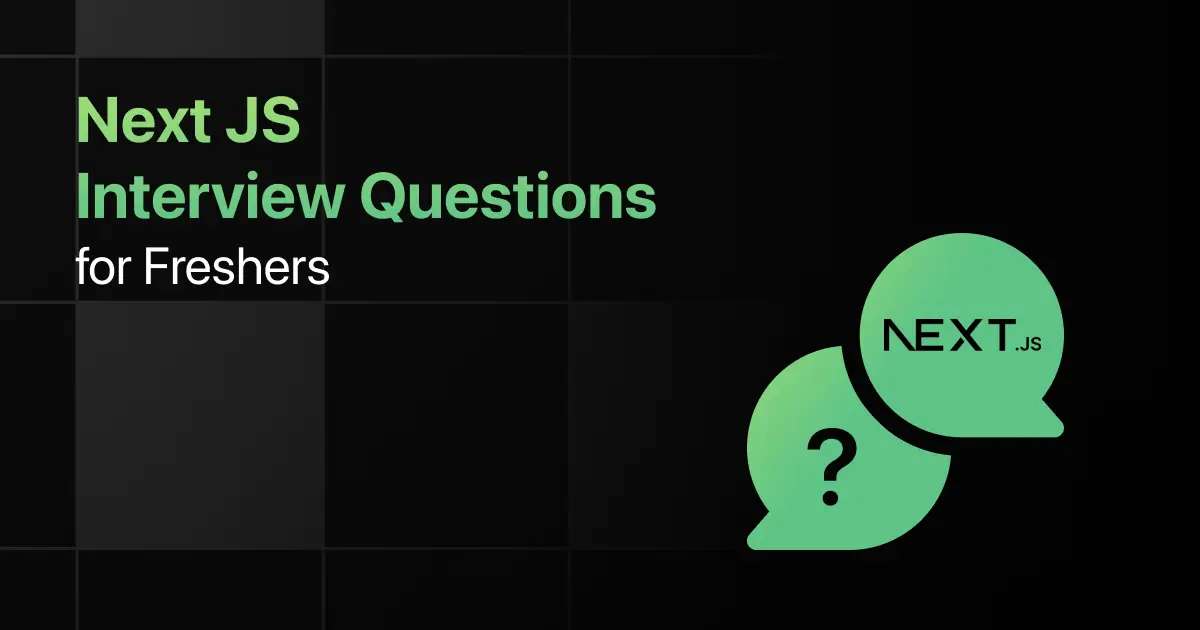
Are you preparing for your first Next.js interview and wondering what questions you might face?
Understanding the key Next.js interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Next.js interview questions and answers for freshers and make a strong impression in your interview.
Practice Next JS Interview Questions and Answers
Below are the top 50 Next JS interview questions for freshers with answers:
1. What is Next.js?
Answer:
Next.js is a React framework that enables server-side rendering, static site generation, and building full-stack applications. It simplifies the process of creating performant and SEO-friendly web applications by offering built-in support for routing, server-side rendering, and API routes.
2. What are the core features of Next.js?
Answer:
Core features of Next.js include server-side rendering (SSR), static site generation (SSG), API routes, automatic code splitting, and optimized performance. These features make Next.js suitable for building scalable and high-performance web applications.
3. How do you create a new Next.js project?
Answer:
To create a new Next.js project, you use the create-next-app command. This sets up a new Next.js application with a default configuration and example files.
cd my-next-app
npm run dev
4. What is server-side rendering (SSR) in Next.js?
Answer:
Server-side rendering (SSR) in Next.js refers to the process of rendering a web page on the server instead of the client. This means the HTML is generated on the server and sent to the client, improving SEO and initial load performance.
return { props: { data: ‘Server-side rendered data’ } };
}
5. What is static site generation (SSG) in Next.js?
Answer:
Static site generation (SSG) generates HTML at build time, creating static files that are served to users. This approach is ideal for content that doesn’t change frequently and improves performance by serving pre-rendered pages.
return { props: { data: ‘Static site generated data’ } };
}
6. How does Next.js handle routing?
Answer:
Next.js handles routing based on the file system. Each file in the pages directory automatically becomes a route. For dynamic routes, file names can include brackets to signify dynamic segments.
export default function About() {
return <div>About Page</div>;
}
// pages/post/[id].js
export default function Post({ query }) {
return <div>Post ID: {query.id}</div>;
}
7. What is getStaticProps in Next.js?
Answer:
getStaticProps is a function used in Next.js to fetch data at build time for static site generation. It allows you to pass data as props to a page component.
const data = await fetchData();
return { props: { data } };
}
8. What is getServerSideProps in Next.js?
Answer:
getServerSideProps is a function used to fetch data on each request for server-side rendering. It runs on the server and provides data as props to the page component.
const data = await fetchData();
return { props: { data } };
}
9. What is getStaticPaths used for in Next.js?
Answer:
getStaticPaths is used with getStaticProps to specify which dynamic routes should be pre-rendered at build time. It returns an array of path objects that Next.js will use to generate static pages.
const paths = [{ params: { id: ‘1’ } }, { params: { id: ‘2’ } }];
return { paths, fallback: false };
}
10. How does Next.js handle API routes?
Answer:
Next.js allows you to create API routes within the pages/api directory. Each file in this directory defines a serverless function that handles HTTP requests and responses.
export default function handler(req, res) {
res.status(200).json({ message: ‘Hello, world!’ });
}
11. What is the Link component in Next.js?
Answer:
The Link component in Next.js is used to enable client-side navigation between pages. It prefetches linked pages for faster navigation and ensures that your links are optimized for performance.
export default function Home() {
return (
<Link href=”/about”>
<a>Go to About Page</a>
</Link>
);
}
12. What are custom _app.js and _document.js files in Next.js?
Answer:
Custom _app.js and _document.js files are used to customize the default App and Document components. _app.js allows you to initialize pages and manage global styles, while _document.js is used to augment the server-rendered document markup.
export default function MyApp({ Component, pageProps }) {
return <Component {…pageProps} />;
}
// _document.js
import Document, { Html, Head, Main, NextScript } from ‘next/document’;
class MyDocument extends Document {
render() {
return (
<Html>
<Head />
<body>
<Main />
<NextScript />
</body>
</Html>
);
}
}
export default MyDocument;
13. How can you add global CSS to a Next.js project?
Answer:
Global CSS can be added by importing the CSS file in the _app.js file. This ensures that the styles are applied across all pages.
import ‘../styles/global.css’;
export default function MyApp({ Component, pageProps }) {
return <Component {…pageProps} />;
}
14. How do you use environment variables in Next.js?
Answer:
Environment variables in Next.js are defined in a .env.local file and accessed using process.env in your code. Variables must start with NEXT_PUBLIC_ to be exposed to the browser.
NEXT_PUBLIC_API_URL=https://api.example.com
const apiUrl = process.env.NEXT_PUBLIC_API_URL;
15. What is the purpose of next.config.js?
Answer:
next.config.js is used to configure various settings in a Next.js application, such as custom webpack configurations, environment variables, and redirects. It allows for advanced customization of the Next.js build process.
module.exports = {
env: {
CUSTOM_API_URL: ‘https://api.example.com’,
},
webpack(config) {
// Customize webpack configuration
return config;
},
};
16. How do you handle image optimization in Next.js?
Answer:
Next.js provides an Image component for optimized image handling. It automatically optimizes images for performance and provides features like lazy loading and responsive images.
export default function Profile() {
return (
<Image
src=”/profile.jpg”
alt=”Profile Picture”
width={500}
height={500}
/>
);
}
17. How do you use dynamic imports in Next.js?
Answer:
Dynamic imports in Next.js allow you to load modules asynchronously, which can improve performance by splitting code into smaller chunks. Use the next/dynamic package for this purpose.
const DynamicComponent = dynamic(() => import(‘../components/HeavyComponent’));
export default function Home() {
return <DynamicComponent />;
}
18. What are Next.js Middleware functions?
Answer:
Middleware functions in Next.js allow you to execute code before a request is completed. They can be used for tasks like authentication, logging, and modifying the request or response.
import { NextResponse } from ‘next/server’;
export function middleware(req) {
console.log(‘Request made to:’, req.nextUrl.pathname);
return NextResponse.next();
}
19. How do you handle authentication in Next.js?
Answer:
Authentication in Next.js can be handled using API routes for login/logout operations and middleware for protecting routes. You can use third-party libraries like next-auth for easier integration.
import NextAuth from ‘next-auth’;
import Providers from ‘next-auth/providers’;
export default NextAuth({
providers: [
Providers.Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
],
});
20. What are getStaticProps and getServerSideProps used for?
Answer:
getStaticProps is used for generating static pages at build time, while getServerSideProps is used for server-side rendering, generating pages on each request. Both methods provide data to a page component as props.
21. How do you perform client-side navigation in Next.js?
Answer:
Client-side navigation is performed using the Link component from next/link, enabling navigation without full page reloads. This provides a smoother user experience, and the linked pages are prefetched for faster performance.
export default function Home() {
return (
<Link href=”/about”>
<a>Go to About Page</a>
</Link>
);
}
22. How do you add custom metadata (e.g., title, meta tags) to a Next.js page?
Answer:
Next.js provides the Head component to insert custom metadata, like titles and meta tags, in the page head section. This is useful for SEO purposes and customizing individual pages.
export default function Home() {
return (
<>
<Head>
<title>My Custom Title</title>
<meta name=”description” content=”My custom description” />
</Head>
<div>Welcome to my page</div>
</>
);
}
23. What is Incremental Static Regeneration (ISR) in Next.js?
Answer:
Incremental Static Regeneration (ISR) allows you to update static content after the build process without rebuilding the entire site. It revalidates pages at a specific interval and serves updated content when needed.
return {
props: { data: ‘Sample data’ },
revalidate: 10, // Revalidates every 10 seconds
};
}
24. What is the difference between SSR and SSG in Next.js?
Answer:
SSR (Server-Side Rendering) generates the HTML on the server for each request, improving SEO and performance for dynamic content. SSG (Static Site Generation) builds HTML at compile time, serving pre-rendered pages for better performance in static scenarios.
25. How does API routing work in Next.js?
Answer:
Next.js provides API routes that allow you to build a back-end using Node.js within the framework. API routes are defined in the pages/api directory, and each file becomes an endpoint.
export default function handler(req, res) {
res.status(200).json({ message: ‘Hello World’ });
}
26. How does automatic static optimization work in Next.js?
Answer:
Automatic static optimization allows Next.js to automatically pre-render static pages if no getServerSideProps or getInitialProps functions are used. This improves performance and load time by serving static content.
27. What is dynamic routing in Next.js, and how is it implemented?
Answer:
Dynamic routing allows you to create routes based on dynamic segments, such as blog posts or user IDs. It’s implemented using brackets in the pages directory file name.
export default function Post({ params }) {
return <div>Post ID: {params.id}</div>;
}
28. How do you protect a page in Next.js with authentication?
Answer:
To protect a page, use Next.js middleware or redirect users based on authentication status. Libraries like next-auth help manage session-based authentication.
const session = await getSession(context);
if (!session) {
return {
redirect: {
destination: ‘/login’,
permanent: false,
},
};
}
return { props: { session } };
}
29. What are Next.js “API routes,” and how are they used?
Answer:
API routes allow you to build APIs directly within your Next.js application. Each file in the pages/api directory is treated as an API route, handling HTTP requests.
export default function handler(req, res) {
res.status(200).json({ name: ‘John Doe’ });
}
30. What is the difference between getInitialProps and getServerSideProps?
Answer:
getInitialProps can run on both the server and client, fetching data for both initial load and subsequent navigations. getServerSideProps runs only on the server, ensuring server-rendered data on every request.
31. How do you create an API in Next.js?
Answer:
In Next.js, API routes are defined within the pages/api directory. Each file becomes an API endpoint, allowing you to build serverless functions easily.
export default function handler(req, res) {
res.status(200).json({ message: ‘Hello from API!’ });
}
32. How do you manage environment variables in Next.js?
Answer:
Environment variables in Next.js are stored in .env.local and accessed via process.env. Variables starting with NEXT_PUBLIC_ are accessible on the client side.
NEXT_PUBLIC_API_URL=https://api.example.com
const apiUrl = process.env.NEXT_PUBLIC_API_URL;
33. How does code splitting work in Next.js?
Answer:
Code splitting in Next.js is automatic. It ensures that only the necessary JavaScript for the current page is loaded, improving performance by reducing the amount of code that needs to be downloaded.
34. What is fallback rendering in Next.js?
Answer:
Fallback rendering is used in dynamic SSG pages. If a page doesn’t exist during the build, Next.js can serve a fallback version while generating the content on demand.
return {
paths: [],
fallback: true,
};
}
35. How does Next.js handle page pre-rendering?
Answer:
Next.js pre-renders pages by default, generating the HTML for each page at either build time (SSG) or on each request (SSR). This improves performance and SEO.
36. What is next/head, and how is it used?
Answer:
next/head is a component used to manage metadata like titles, descriptions, and link tags in the head of your HTML document. It’s essential for improving SEO and customizing individual page metadata.
export default function Home() {
return (
<>
<Head>
<title>My Custom Title</title>
</Head>
<div>Home Page</div>
</>
);
}
37. How do you handle redirects in Next.js?
Answer:
Redirects can be configured in next.config.js to programmatically route users to different URLs. You can also use server-side or client-side methods to redirect dynamically.
async redirects() {
return [
{
source: ‘/old-route’,
destination: ‘/new-route’,
permanent: true,
},
];
},
};
38. How do you handle rewrites in Next.js?
Answer:
Rewrites allow you to map URLs to different destination paths without changing the URL. This is useful for cleaner URLs or proxying API routes.
async rewrites() {
return [
{
source: ‘/api/:path*’,
destination: ‘https://external-api.com/:path*’,
},
];
},
};
39. How do you use the Image component in Next.js?
Answer:
The Image component in Next.js automatically optimizes images, providing lazy loading, resizing, and responsive images. It improves performance by serving optimized images.
export default function Home() {
return <Image src=”/image.jpg” alt=”Image” width={500} height={500} />;
}
40. What is Next.js “middleware”?
Answer:
Middleware in Next.js runs before a request is completed, enabling you to modify requests and responses, handle authentication, or add logging functionality. It can be defined in the middleware.js file.
export function middleware(req) {
const url = req.nextUrl.clone();
url.pathname = ‘/new-path’;
return NextResponse.redirect(url);
}
41. How can you deploy a Next.js application?
Answer:
Next.js applications can be deployed to platforms like Vercel, AWS, or any Node.js hosting service. Vercel offers one-click deployment specifically for Next.js apps, making it one of the easiest methods.
42. What are getStaticPaths and getStaticProps used for in dynamic pages?
Answer:
getStaticPaths defines the dynamic routes that should be pre-rendered, while getStaticProps fetches the necessary data at build time for those dynamic routes. Together, they enable dynamic static pages.
43. What is Fast Refresh in Next.js?
Answer:
Fast Refresh is a feature in Next.js that provides instant feedback when editing React components. It allows you to see changes immediately in the browser without losing the state of your application.
44. How does Next.js handle client-side data fetching?
Answer:
Client-side data fetching in Next.js is typically done using React hooks like useEffect or third-party libraries like SWR (Stale-While-Revalidate). This is useful when fetching data after the page has been rendered.
function ClientSideData() {
const [data, setData] = useState(null);
useEffect(() => {
fetch(‘/api/data’)
.then((res) => res.json())
.then((data) => setData(data));
}, []);
return <div>Data: {data}</div>;
}
45. How can you use getServerSideProps for server-side data fetching?
Answer:
getServerSideProps allows fetching data on every request. This method runs on the server, making it ideal for dynamic content that needs to be fresh with every page load, like user-specific data.
const res = await fetch(`https://api.example.com/data`);
const data = await res.json();
return { props: { data } };
}
function Page({ data }) {
return <div>Data: {data}</div>;
}
46. What is a fallback page in Next.js, and how does it work?
Answer:
Fallback pages in Next.js are used in conjunction with getStaticPaths and getStaticProps for dynamic SSG pages. If a page is not yet generated, Next.js can render a fallback version until the data is fetched and the page is fully generated.
return { paths: [], fallback: ‘blocking’ };
}
export async function getStaticProps({ params }) {
const data = await fetch(`https://api.example.com/${params.id}`);
return { props: { data } };
}
47. How do you optimize Next.js apps for performance?
Answer:
Next.js offers several built-in performance optimizations like automatic code splitting, image optimization, lazy loading, and static generation. Additionally, using server-side caching and Content Delivery Networks (CDNs) improves performance.
48. What is SWR in Next.js, and how is it used?
Answer:
SWR (Stale-While-Revalidate) is a data-fetching library developed by Vercel, allowing you to fetch, cache, and revalidate data on the client side. It provides real-time data fetching with automatic updates.
const fetcher = (url) => fetch(url).then((res) => res.json());
function Profile() {
const { data, error } = useSWR(‘/api/user’, fetcher);
if (error) return <div>Error loading data</div>;
if (!data) return <div>Loading…</div>;
return <div>Hello {data.name}</div>;
}
49. How do you handle global state management in Next.js?
Answer:
For global state management, you can use React’s Context API, Redux, or libraries like Zustand and Recoil. State can be shared across components and pages using these tools, ensuring consistent data across your app.
const GlobalContext = createContext();
export function GlobalProvider({ children }) {
const [state, setState] = useState(‘Some global state’);
return (
<GlobalContext.Provider value={{ state, setState }}>
{children}
</GlobalContext.Provider>
);
}
export function useGlobalContext() {
return useContext(GlobalContext);
}
50. How do you configure internationalization (i18n) in Next.js?
Answer:
Next.js supports internationalization (i18n) out of the box, allowing you to serve different locales for different languages. You can configure it in next.config.js.
module.exports = {
i18n: {
locales: [‘en’, ‘fr’, ‘es’],
defaultLocale: ‘en’,
},
};
In your components, you can switch between locales using the router.
import { useRouter } from ‘next/router’;
function LanguageSwitcher() {
const router = useRouter();
const changeLanguage = (locale) => {
router.push(router.pathname, router.asPath, { locale });
};
return (
<div>
<button onClick={() => changeLanguage(‘fr’)}>French</button>
<button onClick={() => changeLanguage(‘es’)}>Spanish</button>
</div>
);
}
Final Words
Getting ready for an interview can feel overwhelming, but going through these Next.js fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Next.js interview but don’t forget to practice server-side rendering (SSR), static site generation (SSG), API routes, and Next.js routing-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for JS?
Common questions often focus on understanding JavaScript fundamentals, such as closures, promises, asynchronous programming, and the event loop. You might also encounter questions about scope, hoisting, and prototypal inheritance.
2. What are the important JS topics freshers should focus on for interviews?
Freshers should focus on core concepts such as variables and data types, functions, scope, closures, callbacks, promises, and async/await. Understanding the DOM, event handling, and basic algorithms is also crucial.
3. How should freshers prepare for JS technical interviews?
Freshers should practice coding problems on platforms like LeetCode or HackerRank, review JavaScript concepts through online tutorials, and build small projects to gain practical experience.
4. What strategies can freshers use to solve JS coding questions during interviews?
Freshers should carefully read the problem statement, break it down into smaller parts, and use a step-by-step approach to solve it. Writing clean, efficient code and testing edge cases are important.
5. Should freshers prepare for advanced JS topics in interviews?
Yes, freshers should prepare advanced topics like event loops, memory management, and performance optimization.
Explore More Next JS Resources
Explore More Interview Questions
- Python
- Java
- SQL
- React
- JavaScript
- C Programming
- HTML
- CSS
- Angular
- C++
- Spring Boot
- Node JS
- Excel
- C#
- DBMS
- PHP
- Linux
- Operating System
- MySQL
- Spring
- Flutter
- MongoDB
- Django
- React Native
- jQuery
- Bootstrap
- Embedded C
- DSA
- R Programming
- Hadoop
- .NET
- Power BI
- ASP.NET
- ASP.NET MVC
- Android
- Tableau
- MVC
- WordPress
- TypeScript
- Spark
- Kotlin
- Swift
- Golang
- Shell Scripting
- iOS
- Spring MVC
Related Posts
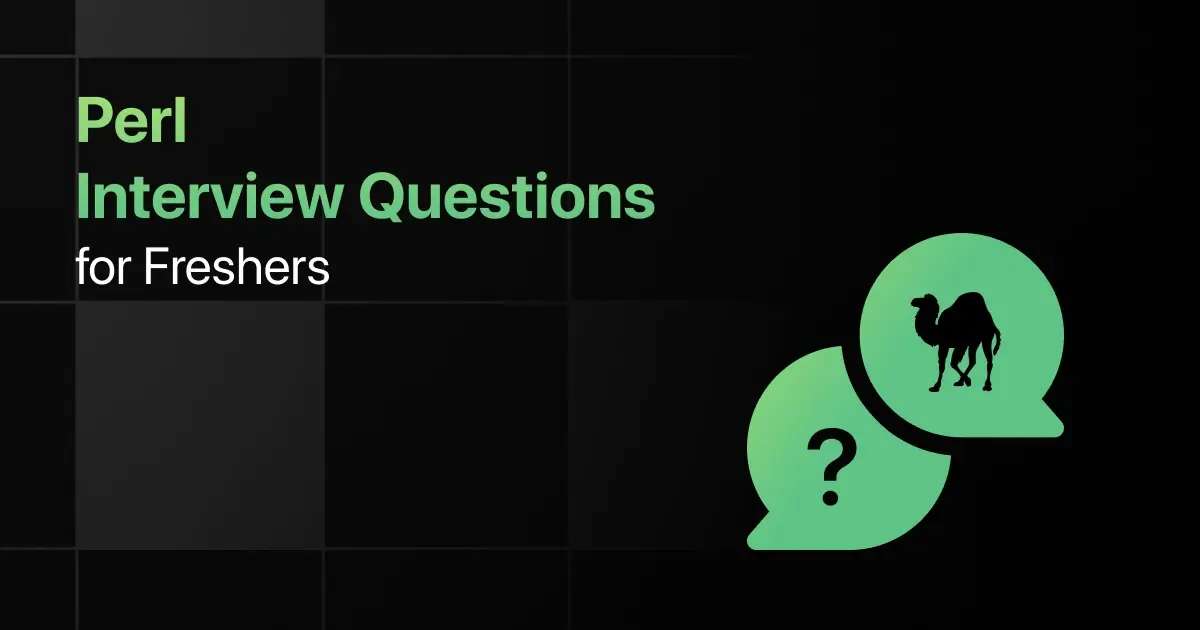
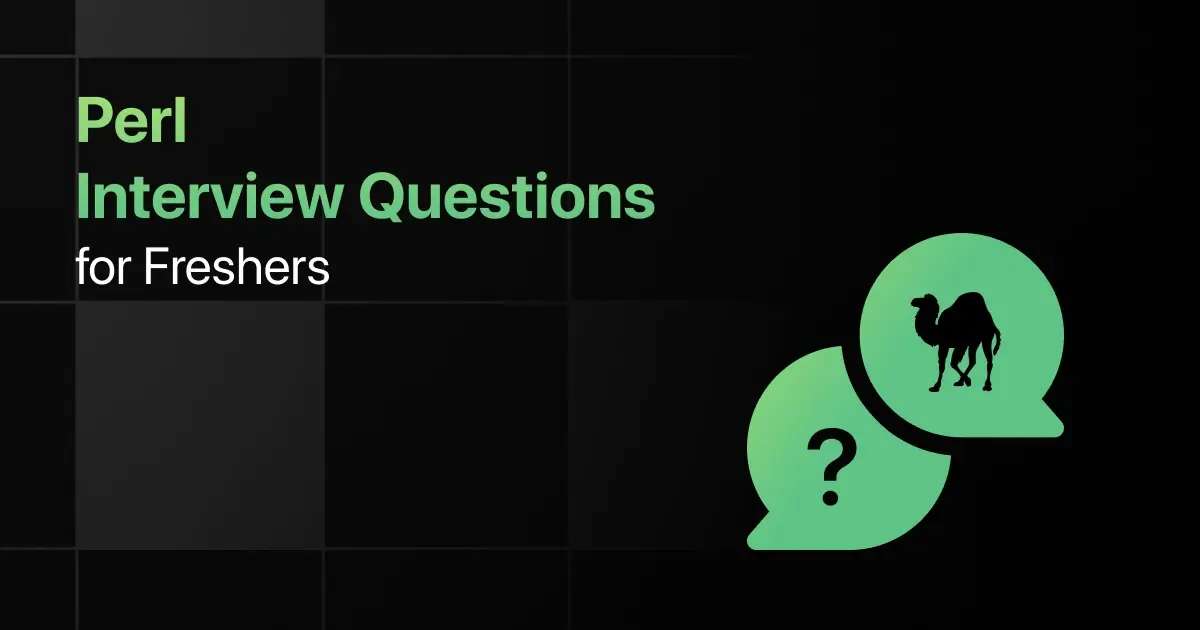
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …