Top Spring MVC Interview Questions for Freshers
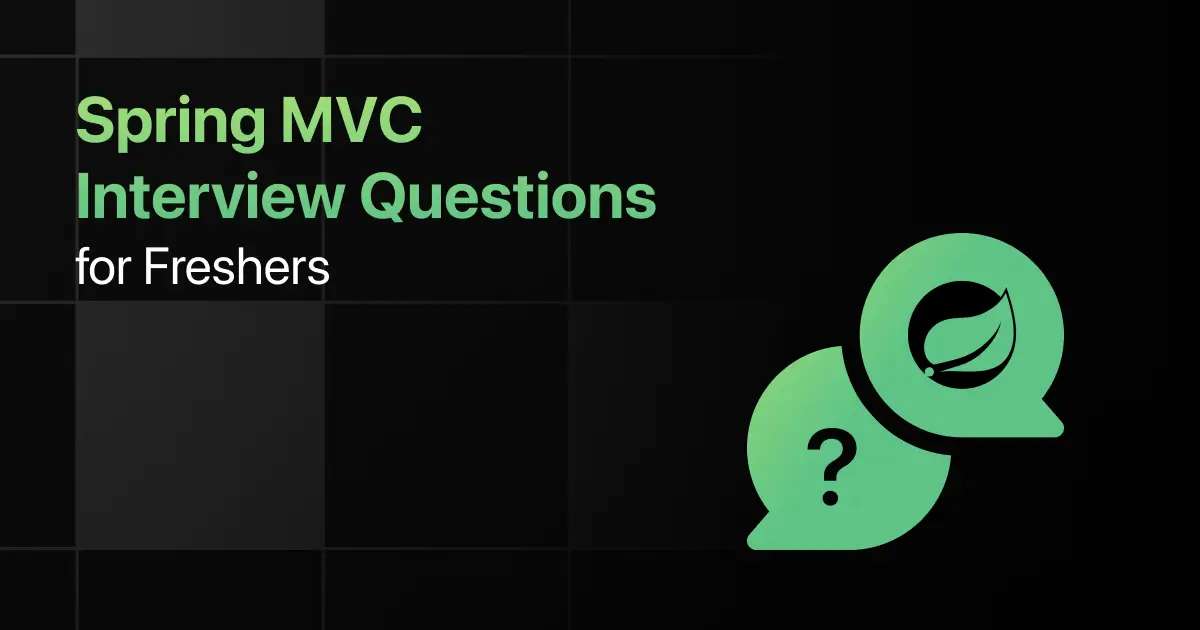
Are you preparing for your first Spring MVC interview and wondering what questions you might face?
Understanding the key Spring MVC interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Spring MVC interview questions and answers for freshers and make a strong impression in your interview.
Practice Spring MVC Interview Questions and Answers
Below are the top 50 Spring MVC interview questions for freshers with answers:
1. What is Spring MVC?
Answer:
Spring MVC is a web framework based on the Model-View-Controller (MVC) design pattern. It simplifies the development of web applications by separating the application logic (controller), user interface (view), and data handling (model). This modular structure enhances testability and maintainability.
2. What are the main components of the Spring MVC framework?
Answer:
The main components are:
- DispatcherServlet: Front controller that routes requests.
- Controller: Handles user requests and processes data.
- ViewResolver: Determines which view to return.
- Model: Holds application data and state.
3. Explain the DispatcherServlet in Spring MVC.
Answer:
The DispatcherServlet acts as the front controller in Spring MVC. It receives all incoming requests, delegates them to the appropriate controllers, and processes their responses before sending them back to the client. It also works closely with view resolvers to handle view rendering.
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
</servlet>
4. How does the @RequestMapping annotation work?
Answer:
@RequestMapping is used to map web requests to specific handler methods in Spring MVC. It can map a request path to a method or controller, allowing flexibility in handling various HTTP methods like GET, POST, PUT, DELETE.
public String hello() {
return “hello”;
}
5. What is the role of a ViewResolver in Spring MVC?
Answer:
A ViewResolver resolves logical view names returned by controllers into actual views. It maps a view name to a specific view implementation, such as JSP or Thymeleaf, allowing Spring MVC to separate view rendering from business logic.
public InternalResourceViewResolver viewResolver() {
InternalResourceViewResolver resolver = new InternalResourceViewResolver();
resolver.setPrefix(“/WEB-INF/views/”);
resolver.setSuffix(“.jsp”);
return resolver;
}
6. How does Spring MVC handle form data?
Answer:
Spring MVC uses @ModelAttribute and @RequestParam annotations to bind form data to model objects. The framework automatically binds the submitted form fields to the corresponding object attributes, handling both primitive and complex data types.
public String handleForm(@ModelAttribute(“user”) User user) {
// Handle form data
return “result”;
}
7. Explain @ModelAttribute in Spring MVC.
Answer:
@ModelAttribute is used to bind form data to a model object or to make pre-populated objects available to the view. It can be used at the method or parameter level and allows Spring to automatically bind request parameters to object properties.
public User populateUser() {
return new User();
}
8. What is the difference between @Controller and @RestController?
Answer:
- @Controller: Used in Spring MVC to define a controller that returns a view (HTML, JSP).
- @RestController: A specialized version of @Controller that returns data (usually JSON or XML) instead of a view.
9. How do you configure Spring MVC using Java-based configuration?
Answer:
Spring MVC can be configured using Java-based configuration by extending WebMvcConfigurer and overriding necessary methods like addViewControllers, addResourceHandlers, etc. This eliminates the need for XML configuration.
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Override
public void addViewControllers(ViewControllerRegistry registry) {
registry.addViewController(“/home”).setViewName(“home”);
}
}
10. What is the role of the ModelAndView class in Spring MVC?
Answer:
ModelAndView is used to return both the model (data) and the view (UI) from a Spring MVC controller. It holds the view name and the data that the view will display.
public ModelAndView welcome() {
ModelAndView mav = new ModelAndView(“welcome”);
mav.addObject(“message”, “Welcome to Spring MVC!”);
return mav;
}
11. How do you handle exceptions in Spring MVC?
Answer:
Spring MVC provides several ways to handle exceptions, such as using @ExceptionHandler at the controller level or @ControllerAdvice to handle exceptions globally across controllers.
public String handleException() {
return “error”;
}
12. What is the role of @RequestParam in Spring MVC?
Answer:
@RequestParam is used to extract query parameters, form data, or path variables from an HTTP request and map them to controller method parameters.
public String greet(@RequestParam(“name”) String name) {
return “Hello, ” + name;
}
13. Explain the concept of validation in Spring MVC.
Answer:
Spring MVC uses annotations like @Valid and @NotNull to validate model attributes. Validation errors can be captured using the BindingResult interface, allowing the developer to handle and display validation messages.
public String save(@Valid @ModelAttribute(“user”) User user, BindingResult result) {
if (result.hasErrors()) {
return “form”;
}
return “success”;
}
14. How can you upload a file using Spring MVC?
Answer:
Spring MVC provides support for file uploads through the MultipartFile interface. To handle file uploads, you must configure multipart support in the Spring configuration and handle the file in the controller.
public String handleFileUpload(@RequestParam(“file”) MultipartFile file) {
// Handle the file
return “uploadSuccess”;
}
15. What are Interceptors in Spring MVC?
Answer:
Interceptors in Spring MVC are used to intercept HTTP requests before or after they reach the controller. They allow pre-processing and post-processing of the request, such as logging, authentication, or modifying the request/response.
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) {
// Pre-process the request
return true;
}
}
16. How does Spring MVC support internationalization (i18n)?
Answer:
Spring MVC supports internationalization through the LocaleResolver and ResourceBundleMessageSource. By defining different message bundles for each language, Spring can dynamically change content based on the user’s locale.
public LocaleResolver localeResolver() {
SessionLocaleResolver resolver = new SessionLocaleResolver();
resolver.setDefaultLocale(Locale.US);
return resolver;
}
17. How do you implement pagination in Spring MVC?
Answer:
Pagination in Spring MVC can be implemented using query parameters like page and size in a controller. You can fetch data in chunks based on these parameters from a database or service layer.
public String list(@RequestParam(“page”) int page, @RequestParam(“size”) int size) {
// Fetch data with pagination
return “listView”;
}
18. What is the role of @PathVariable in Spring MVC?
Answer:
@PathVariable is used to extract values from the URI path. It allows mapping dynamic values from the URL to controller method parameters.
public String getUser(@PathVariable(“id”) int id) {
// Fetch user by id
return “userView”;
}
19. What is ContentNegotiation in Spring MVC?
Answer:
ContentNegotiation allows Spring MVC to determine the response format (JSON, XML, HTML) based on the Accept header in the HTTP request. This ensures that the same URL can serve different response formats based on client preferences.
20. How can you configure static resource handling in Spring MVC?
Answer:
You can configure Spring MVC to serve static resources (CSS, JS, images) by overriding the addResourceHandlers method in the WebMvcConfigurer interface.
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler(“/resources/**”).addResourceLocations(“/public/”);
}
21. How do you configure CORS (Cross-Origin Resource Sharing) in Spring MVC?
Answer:
You can configure CORS in Spring MVC using the @CrossOrigin annotation at the controller or method level. Alternatively, you can configure CORS globally using the addCorsMappings method in WebMvcConfigurer.
@RequestMapping(“/greet”)
public String greet() {
return “Hello!”;
}
22. What is the purpose of the @SessionAttributes annotation in Spring MVC?
Answer:
@SessionAttributes is used to store model attributes in the HTTP session between multiple requests. This can be useful for maintaining user-specific data, like a shopping cart, across different controller methods.
@Controller
public class CartController {
// Cart logic here
}
23. How do you bind a list of values in Spring MVC?
Answer:
You can bind a list of values from a form to a model object using @ModelAttribute. The list can be directly populated using a form element like a checkbox or select, with Spring automatically mapping it to the model object.
public String handleSubmit(@ModelAttribute(“user”) User user) {
// user.getRoles() will return the list of roles
return “result”;
}
24. How do you configure custom error pages in Spring MVC?
Answer:
You can configure custom error pages by defining error codes in your web.xml or using the ErrorController interface for programmatic handling in Spring Boot applications.
<error-code>404</error-code>
<location>/WEB-INF/views/error/404.jsp</location>
</error-page>
25. What is Spring’s Flash Attributes?
Answer:
Flash Attributes in Spring MVC are used to pass temporary data (e.g., success or error messages) across redirects. They are typically used when a POST-Redirect-GET pattern is implemented to avoid form resubmission issues.
26. What is the role of @ResponseBody in Spring MVC?
Answer:
@ResponseBody indicates that the return value of a method should be bound to the web response body rather than being interpreted as a view name. It is used when sending raw data (like JSON or XML) in a RESTful service.
@ResponseBody
public User getUser() {
return new User();
}
27. What is the role of @RequestBody in Spring MVC?
Answer:
@RequestBody is used to bind the HTTP request body to a method parameter in Spring MVC. It is commonly used in REST APIs to map incoming JSON or XML payloads to Java objects.
public String addUser(@RequestBody User user) {
// Process user
return “success”;
}
28. How can you configure message converters in Spring MVC?
Answer:
Spring MVC uses HttpMessageConverter to convert HTTP request and response bodies into Java objects and vice versa. You can configure custom message converters in configureMessageConverters method of WebMvcConfigurer.
public void configureMessageConverters(List<HttpMessageConverter<?>> converters) {
converters.add(new MappingJackson2HttpMessageConverter());
}
29. How do you implement method-level security in Spring MVC?
Answer:
Spring MVC integrates with Spring Security to provide method-level security. You can secure methods using annotations like @PreAuthorize, @Secured, and @RolesAllowed.
@RequestMapping(“/admin”)
public String adminPage() {
return “admin”;
}
30. What is @ControllerAdvice in Spring MVC?
Answer:
@ControllerAdvice is a global annotation for handling exceptions, binding data, or adding common model attributes across multiple controllers. It helps in centralizing error handling or logic that is common across controllers.
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public String handleException() {
return “error”;
}
}
31. What is the difference between @PathVariable and @RequestParam?
Answer:
@PathVariable extracts values from the URI path, while @RequestParam extracts query parameters or form data from the request.
@RequestMapping(“/users/{id}”)
public String getUserById(@PathVariable(“id”) int id) {
// Logic
}
// RequestParam
@RequestMapping(“/search”)
public String search(@RequestParam(“q”) String query) {
// Logic
}
32. How can you customize the default error handling in Spring MVC?
Answer:
You can customize error handling in Spring MVC by implementing HandlerExceptionResolver or using the @ExceptionHandler annotation in a controller or globally using @ControllerAdvice.
33. What is the use of @RequestPart in Spring MVC?
Answer:
@RequestPart is used to bind a specific part of a multipart request (file, form field) to a method parameter. It’s typically used in file uploads.
public String uploadFile(@RequestPart(“file”) MultipartFile file) {
// Handle file upload
return “success”;
}
34. What is @RequestHeader in Spring MVC?
Answer:
@RequestHeader is used to extract a header value from the HTTP request and bind it to a method parameter in the controller.
public String greet(@RequestHeader(“User-Agent”) String userAgent) {
return “User-Agent: ” + userAgent;
}
35. How do you test Spring MVC controllers?
Answer:
Spring MVC controllers can be tested using MockMvc provided by the Spring Test framework. It simulates HTTP requests and tests the controller logic without deploying the application to a web server.
@WebMvcTest(MyController.class)
public class MyControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void testGet() throws Exception {
mockMvc.perform(get(“/greet”))
.andExpect(status().isOk())
.andExpect(content().string(“Hello”));
}
}
36. How can you configure asynchronous request handling in Spring MVC?
Answer:
Spring MVC supports asynchronous request handling using DeferredResult or Callable. This allows you to free up the request thread and return a result later.
public Callable<String> handleAsync() {
return () -> {
// Simulate long task
return “asyncResponse”;
};
}
37. What is a @ResponseStatus annotation?
Answer:
@ResponseStatus is used to specify the HTTP status code for a method’s response. It can be applied to methods or exceptions, making it easier to return specific status codes in error scenarios.
public class ResourceNotFoundException extends RuntimeException {
// Custom exception
}
38. What is the purpose of @CookieValue in Spring MVC?
Answer:
@CookieValue is used to bind the value of an HTTP cookie to a method parameter in a controller. It allows easy access to cookie values.
public String getCookie(@CookieValue(“sessionId”) String sessionId) {
return “Session ID: ” + sessionId;
}
39. What is a @ModelAttribute annotation at the method level?
Answer:
When applied at the method level, @ModelAttribute adds an object to the model that will be accessible to the view. It can be used for pre-populating forms or sharing common objects across multiple views.
public User createUser() {
return new User();
}
40. How does Spring MVC handle multipart file uploads?
Answer:
Spring MVC handles multipart file uploads by using the MultipartResolver interface. Once configured, Spring automatically binds the uploaded file to a MultipartFile object in the controller.
41. How does the @SessionAttribute annotation work?
Answer:
@SessionAttribute is used to retrieve session attributes in a controller method. This ensures that session-scoped data is available across multiple requests.
public String getCart(@SessionAttribute(“cart”) Cart cart) {
return “cartView”;
}
42. What is the purpose of @InitBinder in Spring MVC?
Answer:
@InitBinder is used to customize data binding for specific request parameters or model attributes in Spring MVC. It allows defining custom editors for data types or handling validation.
public void initBinder(WebDataBinder binder) {
binder.registerCustomEditor(Date.class, new CustomDateEditor(new SimpleDateFormat(“dd-MM-yyyy”), false));
}
43. How do you handle 404 errors in Spring MVC?
Answer:
You can handle 404 errors by defining a custom error page in the web.xml or by configuring an ErrorController in Spring Boot. Alternatively, you can define a global exception handler for NoHandlerFoundException.
44. What is the @RequestMapping annotation used for?
Answer:
@RequestMapping maps web requests to specific controller methods in Spring MVC. It can map a URL, HTTP method, headers, and other properties to a handler method.
45. How can you handle JSON data in Spring MVC?
Answer:
To handle JSON data, Spring MVC uses Jackson to automatically convert Java objects to JSON and vice versa. You can configure the MappingJackson2HttpMessageConverter for this purpose.
46. How do you use @MatrixVariable in Spring MVC?
Answer:
@MatrixVariable is used to extract matrix variables from URI paths. It is rarely used, but Spring MVC supports it for cases where URL path segments contain additional information.
public String getCarDetails(@MatrixVariable(“color”) String color) {
return “Car color: ” + color;
}
47. How do you handle form validation in Spring MVC?
Answer:
Form validation can be handled using annotations like @Valid or @Validated, along with BindingResult to capture validation errors and display messages to the user.
48. How do you configure a view resolver in Spring MVC?
Answer:
You can configure a view resolver by defining a ViewResolver bean, like InternalResourceViewResolver, which resolves view names to actual view templates such as JSP files.
49. What is the role of @Valid in Spring MVC?
Answer:
@Valid is used to trigger validation on a model object in Spring MVC. It ensures that the object complies with the validation annotations applied to its fields.
50. How can you use @RestController in Spring MVC?
Answer:
@RestController is a convenience annotation that combines @Controller and @ResponseBody, making it easier to build RESTful web services. It automatically serializes objects returned from handler methods into JSON or XML.
Final Words
Getting ready for an interview can feel overwhelming, but going through these Spring MVC fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Spring MVC interview, but don’t forget to practice MVC architecture, Spring controllers, dependency injection, and Spring Data JPA-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for Spring MVC?
Common Spring MVC interview questions often cover topics like the architecture of Spring MVC, the DispatcherServlet, request handling lifecycle, annotations like @Controller, @RequestMapping, dependency injection, and the role of Model, View, and Controller.
2. What are the important Spring MVC topics freshers should focus on for interviews?
Freshers should focus on Spring MVC architecture, the role of DispatcherServlet, request mapping, form handling, Spring’s dependency injection, bean lifecycle, exception handling in Spring, and how Spring MVC integrates with databases using Spring Data or Hibernate.
3. How should freshers prepare for Spring MVC technical interviews?
Freshers should understand the fundamental concepts of Spring MVC, practice building simple web applications, and be familiar with request/response handling, annotations, the lifecycle of Spring beans, and how Spring integrates with databases and security.
4. What strategies can freshers use to solve Spring MVC coding questions during interviews?
Freshers should break down the problem, identify the correct annotations and controllers needed, and focus on writing clean, modular code with proper request mappings, services, and exception handling. They should also be able to explain the flow of data between the client and server.
5. Should freshers prepare for advanced Spring MVC topics in interviews?
While it’s good to know topics like Spring Security, AOP, and integrating Spring MVC with microservices, freshers should first focus on understanding the core concepts of Spring MVC and basic web development. Advanced topics can be tackled once the basics are clear.
Explore More Interview Questions
- Python
- Java
- SQL
- React
- JavaScript
- C Programming
- HTML
- CSS
- Angular
- C++
- Spring Boot
- Node JS
- Excel
- C#
- DBMS
- PHP
- Linux
- Operating System
- MySQL
- Spring
- Flutter
- MongoDB
- Django
- React Native
- jQuery
- Bootstrap
- Embedded C
- DSA
- R Programming
- Hadoop
- .NET
- Power BI
- ASP.NET
- ASP.NET MVC
- Android
- Tableau
- MVC
- WordPress
- TypeScript
- Spark
- Kotlin
- Swift
- Golang
- Shell Scripting
- iOS
Related Posts
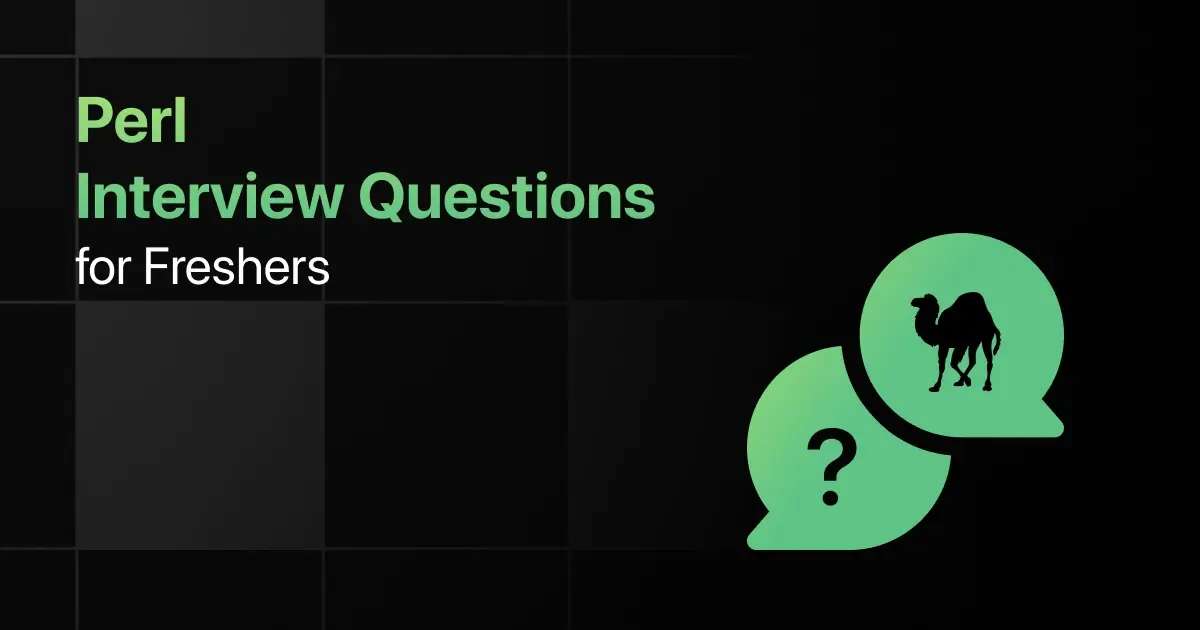
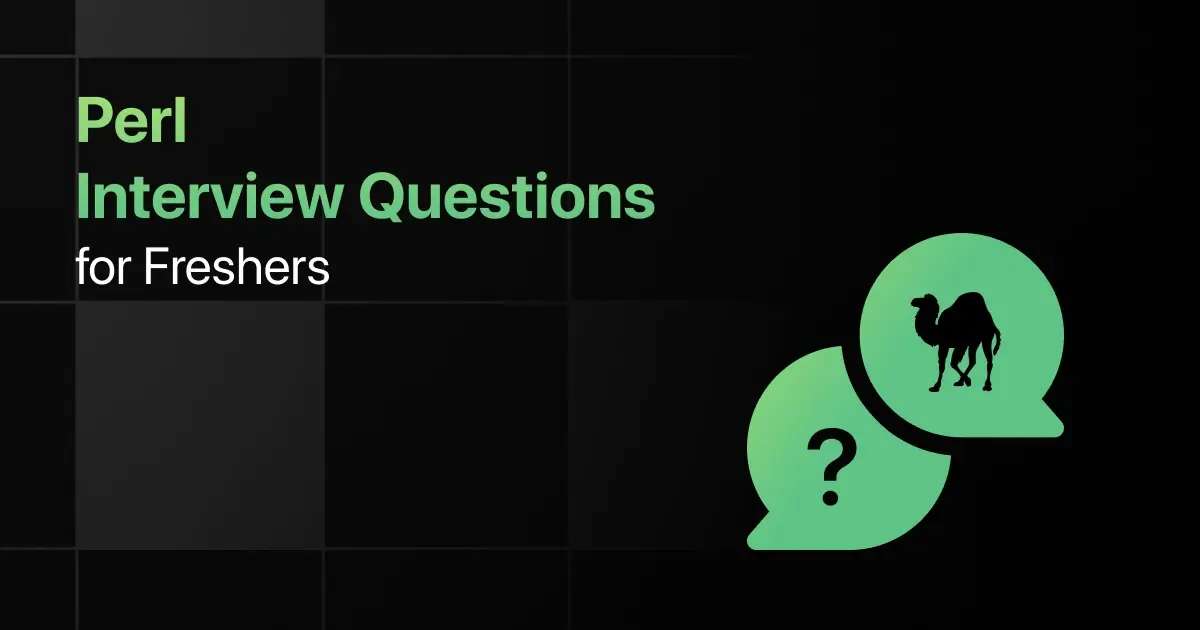
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …