Top Golang Interview Questions for Freshers
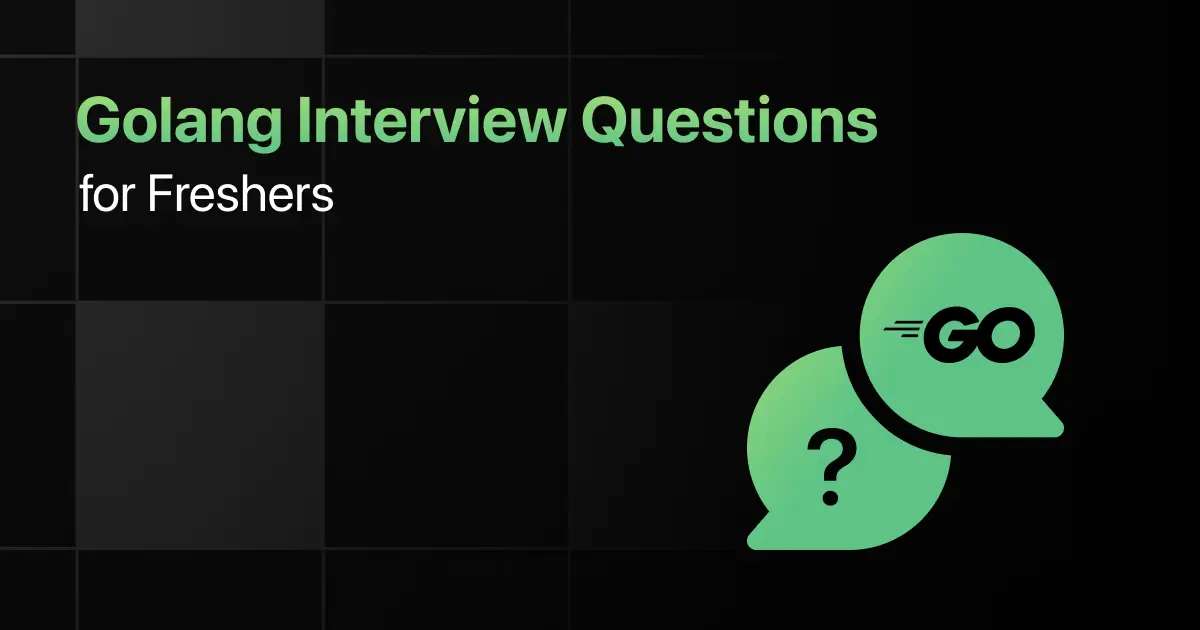
Are you preparing for your first Golang interview and wondering what questions you might face?
Understanding the key Golang interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Golang interview questions and answers for freshers and make a strong impression in your interview.
Practice Golang Interview Questions and Answers
Below are the top 50 Golang interview questions for freshers with answers:
1. What is Go language?
Answer:
Go, often referred to as Golang, is a statically typed, compiled programming language developed by Google. It is designed for simplicity, concurrency, and scalability, making it suitable for cloud and server-side development. Go is known for its clean syntax and efficient memory management.
2. What is a Go workspace?
Answer:
A Go workspace is a directory hierarchy where Go code is organized. It typically contains three subdirectories: src (source files), pkg (package objects), and bin (compiled binaries). The workspace structure allows for efficient project management in Go projects.
3. How do you define a function in Go?
Answer:
In Go, a function is defined using the func keyword, followed by the function name, parameters, and return type.
return a + b
}
This defines a function add that takes two integers and returns their sum.
4. What is a Go package?
Answer:
Packages in Go allow code to be organized into reusable units. Every Go program starts with a package declaration. The main package is used for executable programs, while other packages are for libraries.
import “fmt”
func main() {
fmt.Println(“Hello, Go!”)
}
5. What are goroutines in Go?
Answer:
Goroutines are lightweight threads in Go used for concurrent execution. They are managed by the Go runtime, making them more efficient compared to traditional threads.
fmt.Println(“Running in a goroutine”)
}()
This creates a goroutine that runs the provided function concurrently.
6. Explain Go’s defer statement.
Answer:
defer is used to postpone the execution of a function until the surrounding function returns. It’s often used for resource cleanup, such as closing files.
f, _ := os.Open(“example.txt”)
defer f.Close() // Closes the file after readFile finishes
}
7. How do you handle errors in Go?
Answer:
In Go, errors are handled by returning an error object from functions and checking it. Go encourages explicit error handling over exception-based models.
if err != nil {
log.Fatal(err) // Handle the error
}
8. What are Go interfaces?
Answer:
An interface in Go is a type that defines a set of method signatures. Any type that implements those methods automatically satisfies the interface.
Area() float64
}
type Circle struct {
Radius float64
}
func (c Circle) Area() float64 {
return 3.14 * c.Radius * c.Radius
}
9. What is the difference between var, :=, and const?
Answer:
- var is used to declare variables that can change values.
- := is shorthand for declaring and initializing variables.
- const defines constants whose values cannot change.
y := 20
const Pi = 3.14
10. Explain Go’s garbage collection.
Answer:
Go automatically manages memory through garbage collection, which frees up memory occupied by objects that are no longer in use. Go’s garbage collector is highly efficient, making it ideal for long-running applications.
11. How do Go slices work?
Answer:
A slice in Go is a dynamically-sized, flexible view into the elements of an array. Unlike arrays, slices are not fixed in size and can grow or shrink.
s = append(s, 4)
12. What is a Go struct?
Answer:
A struct is a collection of fields, and it’s used to group data together in Go. It allows the creation of user-defined types that can store different properties.
Name string
Age int
}
13. How do you declare constants in Go?
Answer:
Constants are declared using the const keyword. Their values must be determined at compile time.
14. How do you implement concurrency in Go?
Answer:
Concurrency in Go is primarily handled using goroutines and channels. Goroutines run concurrently, while channels are used for communication between them.
go func() { ch <- 1 }()
fmt.Println(<-ch)
15. What are channels in Go?
Answer:
Channels are Go’s way of allowing goroutines to communicate and synchronize execution. They can be used to send and receive values between goroutines.
go func() { ch <- “Hello” }()
msg := <-ch
fmt.Println(msg) // Outputs: Hello
16. What is Go’s select statement?
Answer:
select allows a goroutine to wait on multiple channel operations. It blocks until one of its cases can run, making it useful for handling multiple channels.
case msg1 := <-ch1:
fmt.Println(“Received”, msg1)
case msg2 := <-ch2:
fmt.Println(“Received”, msg2)
}
17. How do you define methods on types in Go?
Answer:
In Go, methods are defined by associating them with a type. Methods allow types to have behavior.
Radius float64
}
func (c Circle) Area() float64 {
return 3.14 * c.Radius * c.Radius
}
18. What is the zero value in Go?
Answer:
The zero value is the default value assigned to variables when they are declared without initialization. For example, the zero value for an int is 0, for string it’s “”, and for pointers, it’s nil.
19. How do you create a pointer in Go?
Answer:
A pointer holds the memory address of a value. Pointers in Go are declared using the * symbol.
x := 42
p = &x
20. What is Go’s type inference?
Answer:
Type inference allows Go to automatically infer the type of a variable based on its initialization. This is done using the := shorthand.
21. How do you perform client-side navigation in Next.js?
Answer:
Client-side navigation is performed using the Link component from next/link, enabling navigation without full page reloads. This provides a smoother user experience, and the linked pages are prefetched for faster performance.
import Link from ‘next/link’;
return (
<Link href=”/about”>
<a>Go to About Page</a>
</Link>
);
}
22. How do you add custom metadata (e.g., title, meta tags) to a Next.js page?
Answer:
Next.js provides the Head component to insert custom metadata, like titles and meta tags, in the page head section. This is useful for SEO purposes and customizing individual pages.
export default function Home() {
return (
<>
<Head>
<title>My Custom Title</title>
<meta name=”description” content=”My custom description” />
</Head>
<div>Welcome to my page</div>
</>
);
}
23. What is Incremental Static Regeneration (ISR) in Next.js?
Answer:
Incremental Static Regeneration (ISR) allows you to update static content after the build process without rebuilding the entire site. It revalidates pages at a specific interval and serves updated content when needed.
return {
props: { data: ‘Sample data’ },
revalidate: 10, // Revalidates every 10 seconds
};
}
24. What is the difference between SSR and SSG in Next.js?
Answer:
SSR (Server-Side Rendering) generates the HTML on the server for each request, improving SEO and performance for dynamic content. SSG (Static Site Generation) builds HTML at compile time, serving pre-rendered pages for better performance in static scenarios.
25. How does API routing work in Next.js?
Answer:
Next.js provides API routes that allow you to build a back-end using Node.js within the framework. API routes are defined in the pages/api directory, and each file becomes an endpoint.
export default function handler(req, res) {
res.status(200).json({ message: ‘Hello World’ });
}
26. How does automatic static optimization work in Next.js?
Answer:
Automatic static optimization allows Next.js to automatically pre-render static pages if no getServerSideProps or getInitialProps functions are used. This improves performance and load time by serving static content.
27. What is dynamic routing in Next.js, and how is it implemented?
Answer:
Dynamic routing allows you to create routes based on dynamic segments, such as blog posts or user IDs. It’s implemented using brackets in the pages directory file name.
export default function Post({ params }) {
return <div>Post ID: {params.id}</div>;
}
28. How do you protect a page in Next.js with authentication?
Answer:
To protect a page, use Next.js middleware or redirect users based on authentication status. Libraries like next-auth help manage session-based authentication.
const session = await getSession(context);
if (!session) {
return {
redirect: {
destination: ‘/login’,
permanent: false,
},
};
}
return { props: { session } };
}
29. What are Next.js “API routes,” and how are they used?
Answer:
API routes allow you to build APIs directly within your Next.js application. Each file in the pages/api directory is treated as an API route, handling HTTP requests.
export default function handler(req, res) {
res.status(200).json({ name: ‘John Doe’ });
}
30. What is the difference between a slice and an array in Go?
Answer:
An array in Go has a fixed size, meaning its length cannot be changed once declared. A slice, on the other hand, is a dynamically-sized view into the elements of an array and can grow or shrink as needed. Slices are more flexible and commonly used in Go.
slice := []int{1, 2, 3} // Slice with dynamic size
31. How do you copy slices in Go?
Answer:
The copy() function in Go is used to copy the elements of one slice into another. It copies up to the minimum of the length of the two slices.
dst := make([]int, 3)
copy(dst, src) // Copies elements from src to dst
32. What is a map in Go, and how is it used?
Answer:
A map in Go is a built-in data type that associates keys with values. It’s similar to hash tables or dictionaries in other languages.
m[“apple”] = 5
fmt.Println(m[“apple”]) // Outputs: 5
33. How do you iterate over a map in Go?
Answer:
You can iterate over a map using the range keyword, which provides both the key and the value during each iteration.
for key, value := range m {
fmt.Println(key, value)
}
34. What are Go tags, and how are they used?
Answer:
Go tags are annotations used in struct fields to provide additional information, such as serialization options. They are commonly used with packages like encoding/json for data parsing.
Name string `json:”name”`
Age int `json:”age”`
}
35. How do you handle panics in Go?
Answer:
In Go, a panic stops the normal execution of the program, but it can be handled using recover(). recover() is called within a deferred function to regain control after a panic.
defer func() {
if r := recover(); r != nil {
fmt.Println(“Recovered from panic:”, r)
}
}()
fmt.Println(a / b)
}
36. How do you handle time in Go?
Answer:
Go provides the time package for handling time-related operations such as creating timers, measuring duration, and formatting time.
fmt.Println(t.Format(“2006-01-02 15:04:05”))
37. What are Go’s init() functions?
Answer:
init() functions are special functions that run before the main() function and are typically used for initialization purposes. Each file can contain one or more init() functions.
fmt.Println(“This runs before main!”)
}
38. Explain Go’s sync.WaitGroup.
Answer:
sync.WaitGroup is used to wait for a collection of goroutines to finish. It allows the main program to block until all goroutines have completed their execution.
wg.Add(1)
go func() {
defer wg.Done()
fmt.Println(“Goroutine done”)
}()
wg.Wait() // Waits for all goroutines to finish
39. How do you define custom errors in Go?
Answer:
In Go, custom errors can be defined by implementing the Error() method from the error interface.
Msg string
}
func (e MyError) Error() string {
return e.Msg
}
40. How does Go handle file I/O?
Answer:
Go uses the os and io/ioutil packages for file operations. Files can be opened, read, written, and closed using methods from these packages.
if err != nil {
log.Fatal(err)
}
fmt.Println(string(data))
41. What is type assertion in Go?
Answer:
Type assertion is a way to retrieve the dynamic value of an interface. It is used to cast an interface to its concrete type.
s := i.(string) // Type assertion
fmt.Println(s) // Outputs: Hello
42. How do you achieve polymorphism in Go?
Answer:
Polymorphism in Go is achieved through interfaces. Types can implement interfaces, allowing different types to be used interchangeably based on their behavior rather than their concrete type.
Area() float64
}
type Circle struct {
Radius float64
}
func (c Circle) Area() float64 {
return 3.14 * c.Radius * c.Radius
}
func printArea(s Shape) {
fmt.Println(s.Area())
}
43. How do you use reflection in Go?
Answer:
Reflection in Go is used to inspect the type and value of variables at runtime using the reflect package. It’s particularly useful in advanced scenarios like building frameworks or tools.
t := reflect.TypeOf(x)
fmt.Println(t) // Outputs: float64
44. What is the difference between make() and new() in Go?
Answer:
make() is used to initialize slices, maps, and channels, whereas new() is used to allocate memory for other types and returns a pointer to the zero value of that type.
p := new(int) // Allocates memory and returns a pointer
45. What is a buffered channel in Go?
Answer:
A buffered channel allows sending a limited number of values without a corresponding receiver ready. When the buffer is full, sends block until some values are received.
ch <- 1
ch <- 2
fmt.Println(<-ch) // Outputs: 1
46. What are Go templates, and how are they used?
Answer:
Go templates are a powerful tool for generating textual data by combining static and dynamic content. They are commonly used for generating HTML, emails, or configuration files.
t, _ = t.Parse(“Hello, {{.Name}}!”)
t.Execute(os.Stdout, map[string]string{“Name”: “Go Developer”})
47. How do you define a multi-line string in Go?
Answer:
In Go, multi-line strings are defined using backticks `, which allow raw strings without interpreting escape sequences.
multi-line
string in Go`
48. What is Go’s build constraint?
Answer:
Build constraints are used to conditionally include files in the build process. These constraints are specified using comments at the top of Go files, allowing you to include/exclude code based on platform or custom build tags.
49. How do you test code in Go?
Answer:
Go has a built-in testing framework. Test files are created in the same package with filenames ending in _test.go. Test functions must start with Test and use the testing package.
result := add(1, 2)
if result != 3 {
t.Errorf(“Expected 3, got %d”, result)
}
}
50. How do you benchmark code in Go?
Answer:
Benchmarking in Go is done using the testing package, similar to unit tests. Benchmark functions start with Benchmark and use the B type to measure performance.
for i := 0; i < b.N; i++ {
add(1, 2)
}
}
Final Words
Getting ready for an interview can feel overwhelming, but going through these Golang fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Golang interview, but don’t forget to practice Go’s concurrency model, goroutines, channels, and Go’s standard library-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for Golang?
The most common Golang interview questions revolve around Go’s concurrency model, goroutines, channels, error handling, memory management, interfaces, and struct embedding.
2. What are the important Golang topics freshers should focus on for interviews?
Freshers should focus on Go’s syntax and core concepts like data types, arrays, slices, maps, pointers, and functions.
3. How should freshers prepare for Golang technical interviews?
Freshers should write small Go programs that cover various Go concepts like concurrency, memory allocation, and standard library functions.
4. What strategies can freshers use to solve Golang coding questions during interviews?
Freshers should break down the problem and think in terms of Go idiomatic practices.
They should focus on concurrency where applicable, optimize for performance, and avoid complex code.
5. Should freshers prepare for advanced Golang topics in interviews?
Yes, freshers should prepare advanced topics like reflection, Go’s profiling tools, and complex concurrency patterns.
Explore More Golang Resources
Explore More Interview Questions
- Python
- Java
- SQL
- React
- JavaScript
- C Programming
- HTML
- CSS
- Angular
- C++
- Spring Boot
- Node JS
- Excel
- C#
- DBMS
- PHP
- Linux
- Operating System
- MySQL
- Spring
- Flutter
- MongoDB
- Django
- React Native
- jQuery
- Bootstrap
- Embedded C
- DSA
- R Programming
- Hadoop
- .NET
- Power BI
- ASP.NET
- ASP.NET MVC
- Android
- Tableau
- MVC
- WordPress
- TypeScript
- Spark
- Kotlin
- Swift
Related Posts
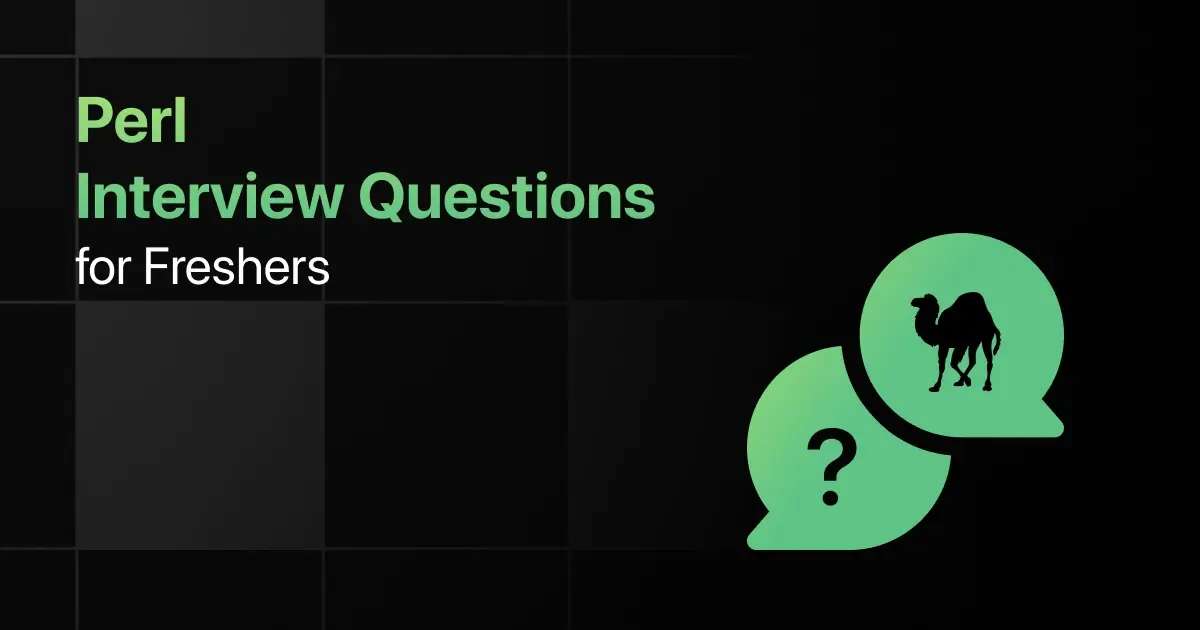
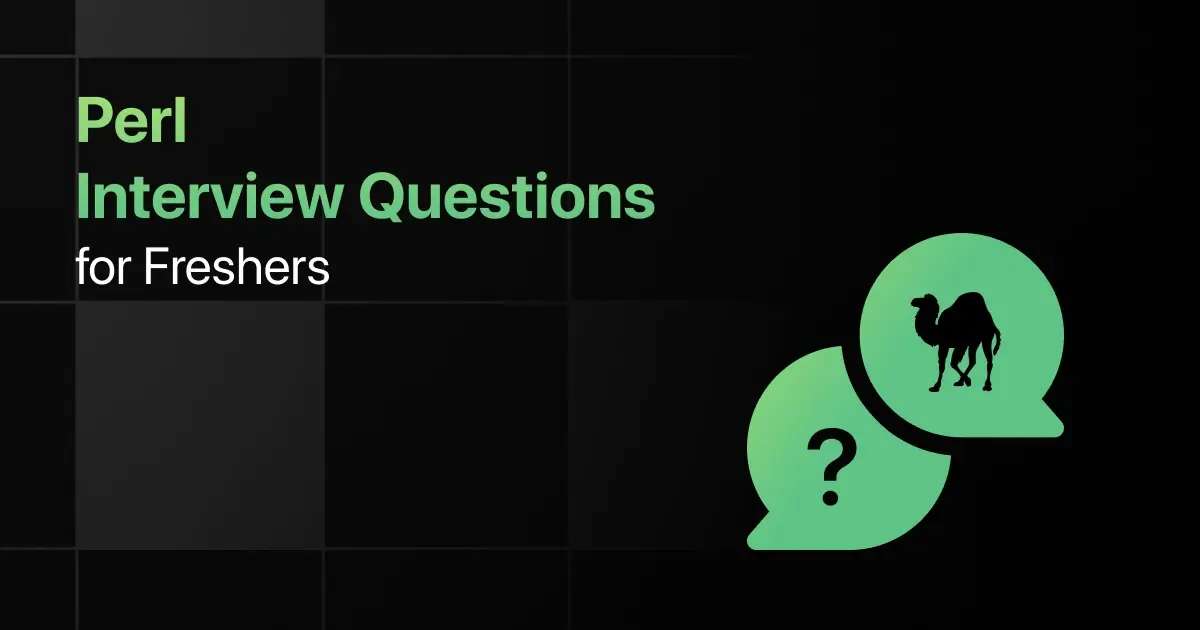
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …