Top Swift Interview Questions for Freshers
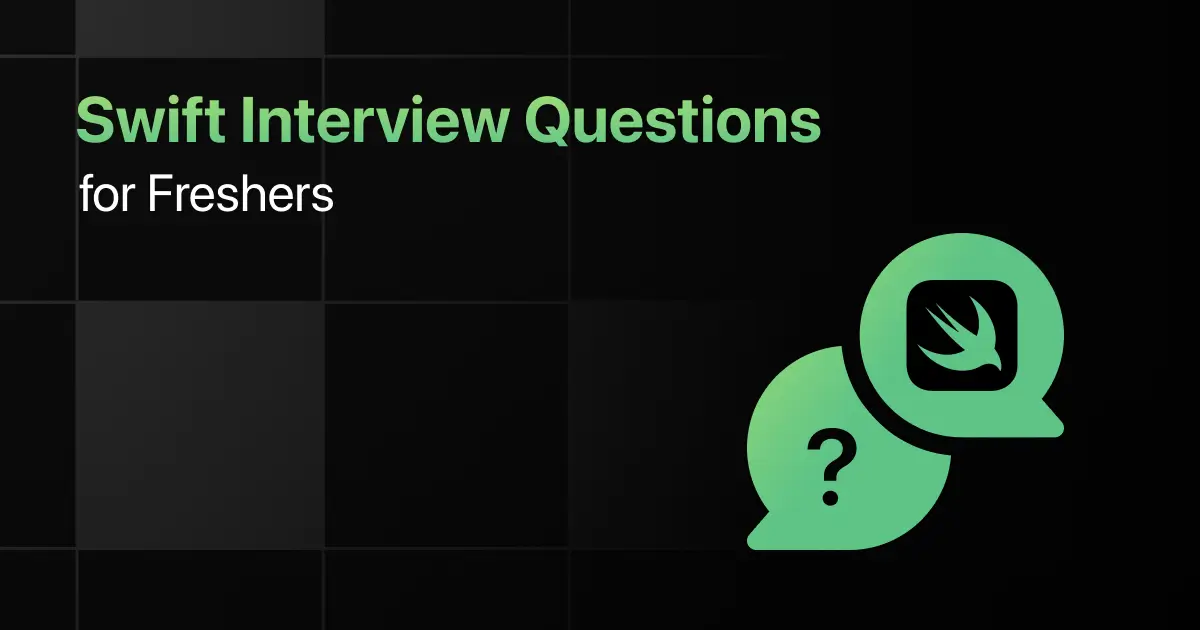
Are you preparing for your first Swift interview and wondering what questions you might face?
Understanding the key Swift interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Swift interview questions and answers for freshers and make a strong impression in your interview.
Practice Swift Interview Questions and Answers
Below are the top 50 Swift interview questions for freshers with answers:
1. What is Swift and why was it created?
Answer:
Swift is a programming language developed by Apple for iOS, macOS, watchOS, and tvOS development. It was created to provide a safer, more performant, and modern alternative to Objective-C. Swift combines powerful features with ease of use, aiming to improve both performance and developer productivity.
2. What are some key features of Swift?
Answer:
Swift includes features like type inference, optionals, and automatic memory management. It also offers modern syntax, strong typing, and error handling capabilities. Swift is designed for safety and performance, which helps developers write robust and efficient code.
3. How does Swift handle optionals?
Answer:
Swift uses optionals to handle the absence of a value. An optional is a type that can hold either a value or nil. Optionals are declared with a ? and can be safely unwrapped using optional binding (if let or guard let) or forced unwrapping (!).
if let unwrappedName = name {
print(unwrappedName)
}
4. How do you perform unit testing in Swift?
Answer:
Unit testing in Swift is performed using the XCTest framework, which provides tools for writing and executing tests. You can create test cases by subclassing XCTestCase, and each test method must start with test. This enables you to validate functionality and ensure code quality over time.
class MyTests: XCTestCase {
func testExample() {
XCTAssertEqual(2 + 2, 4)
}
}
5. What is a closure in Swift?
Answer:
A closure is a self-contained block of code that can be passed around and used in your code. Closures can capture and store references to variables and constants from the surrounding context in which they are defined. Closures in Swift are similar to lambdas in other languages.
return “Hello, \(name)!”
}
print(greet(“Alice”))
6. What are the benefits of using Swift for iOS development?
Answer:
Swift offers several benefits, including safety features that prevent null pointer exceptions, high performance with modern language constructs, and an expressive syntax that enhances developer productivity. It also has a strong community and is continually updated by Apple. Swift’s interoperability with Objective-C allows developers to integrate it into existing projects seamlessly
7. What is the purpose of the defer statement in Swift?
Answer:
The defer statement is used to execute code just before leaving the current scope, regardless of whether the scope is exited due to an error, a return statement, or other control flow changes. It is often used for cleanup tasks, such as closing files or releasing resources.
let file = openFile()
defer {
closeFile(file)
}
// Process the file
}
8. How do you define a class in Swift?
Answer:
A class in Swift is defined using the class keyword followed by the class name. Classes can have properties, methods, and initializers. Swift supports inheritance, allowing one class to inherit properties and methods from another.
var name: String
var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
func greet() {
print(“Hello, my name is \(name).”)
}
}
9. What is a protocol in Swift?
Answer:
A protocol in Swift is a blueprint of methods, properties, and other requirements that suit a particular task or piece of functionality. Protocols can be adopted by classes, structs, or enums to provide implementations of these requirements.
func greet()
}
class Person: Greetable {
func greet() {
print(“Hello!”)
}
}
10. What is the purpose of the enum type in Swift?
Answer:
The enum type in Swift is used to define a group of related values in a type-safe way. Enums can have associated values and methods, providing a way to handle multiple cases and states more effectively.
case north, south, east, west
}
func move(to direction: Direction) {
switch direction {
case .north:
print(“Moving north”)
case .south:
print(“Moving south”)
case .east:
print(“Moving east”)
case .west:
print(“Moving west”)
}
}
11. What is @available in Swift?
Answer:
The @available attribute in Swift is used to mark APIs or types that are available in specific OS versions or platforms. It helps developers manage code compatibility across different iOS versions. Using this attribute can prevent crashes by ensuring that deprecated APIs are not accessed on unsupported versions.
func newFeature() { }
12. What are higher-order functions in Swift?
Answer:
Higher-order functions are functions that can take other functions as parameters or return functions as results. They enable functional programming techniques, such as mapping and filtering collections. Common higher-order functions include map, filter, and reduce.
13. What is the difference between class and struct in Swift?
Answer:
In Swift, class and struct are both used to define data types, but they have some differences. class is a reference type, meaning instances are passed by reference, and they support inheritance. struct is a value type, meaning instances are passed by value and do not support inheritance.
var value: Int
init(value: Int) { self.value = value }
}
struct MyStruct {
var value: Int
}
14. What is an optional chaining in Swift?
Answer:
Optional chaining is a technique to safely access properties, methods, and subscripts on optional that might currently be nil. It allows you to call methods or access properties on an optional without having to check if it is nil first.
var address: Address?
}
class Address {
var city: String
init(city: String) { self.city = city }
}
let person = Person()
let city = person.address?.city
15. What are SwiftUI and UIKit?
Answer:
SwiftUI and UIKit are frameworks for building user interfaces in iOS applications. UIKit is the traditional framework, providing a robust set of components and controllers. SwiftUI, introduced later, allows for a more declarative approach to UI design, enabling real-time previews and easier state management.
16. What is the purpose of the @escaping keyword in Swift?
Answer:
The @escaping keyword is used to indicate that a closure can escape the function it is passed into. It is necessary when a closure is stored for later execution or executed asynchronously.
DispatchQueue.global().async {
completion()
}
}
17. What are computed properties in Swift?
Answer:
Computed properties are properties that do not store a value but instead provide a getter and optionally a setter to compute a value. They are defined with a var keyword and calculated on-the-fly.
var width: Double
var height: Double
var area: Double {
return width * height
}
}
18. Explain the concept of inheritance in Swift.
Answer:
Inheritance allows a class to inherit properties, methods, and other characteristics from another class. The class that inherits is called a subclass, and the class it inherits from is the superclass. Swift supports single inheritance, meaning a class can inherit from only one superclass.
func makeSound() {
print(“Animal sound”)
}
}
class Dog: Animal {
override func makeSound() {
print(“Woof”)
}
}
19. What is a lazy property in Swift?
Answer:
A lazy property is a property whose initial value is not calculated until the first time it is accessed. Lazy properties are useful when the initial value is complex to create or when the property is not always needed.
lazy var data: [String] = {
return [“Data”, “Fetched”] }()
}
20. How do you define a generic type in Swift?
Answer:
Generics in Swift allow you to write flexible and reusable code by defining functions and types that can work with any type, subject to certain constraints. Generics are defined using angle brackets (<>).
let temp = a
a = b
b = temp
}
21. What is the purpose of the final keyword in Swift?
Answer:
The final keyword is used to prevent a class from being subclassed, or a method from being overridden. It helps to ensure that the implementation of a class or method remains unchanged.
// Class implementation
}
22. How do you handle optional values in Swift?
Answer:
Optional values in Swift are handled using optional binding, optional chaining, and forced unwrapping. Optional binding uses if let or guard let to safely unwrap optionals, while optional chaining allows for safe method calls on optionals.
if let unwrappedValue = optionalValue {
print(unwrappedValue)
}
23. What is a tuple in Swift?
Answer:
A tuple in Swift is a group of multiple values that can be of different types. Tuples can be used to return multiple values from functions or to encapsulate related data. They can be named or unnamed for better clarity.
24. What are struct and enum in Swift and how do they differ?
Answer:
struct and enum are value types in Swift. struct is used for creating complex data types with properties and methods, while enum is used to define a set of related values and can include associated values. struct is generally used for modeling data, and enum for defining options or states.
var x: Int
var y: Int
}
enum CompassDirection {
case north, south, east, west
}
25. What is the difference between self and super in Swift?
Answer:
self refers to the current instance of a class or struct, while super refers to the superclass of the current instance. self is used to access properties and methods of the current instance, and super is used to call methods or access properties of the superclass.
func greet() {
print(“Hello from Parent”)
}
}
class Child: Parent {
override func greet() {
super.greet()
print(“Hello from Child”)
}
}
26. What is a protocol extension in Swift?
Answer:
Protocol extensions allow you to provide default implementations of methods, properties, and other requirements in a protocol. This helps to add functionality to multiple types that conform to the protocol without having to repeat code.
func describe() -> String
}
extension Describable {
func describe() -> String {
return “This is a describable item.”
}
}
27. How do you create a singleton in Swift?
Answer:
A singleton in Swift can be created using a static constant that is lazily initialized. This ensures that only one instance of the class is created and shared throughout the application. Singleton classes are often used for shared resources or configurations.
static let shared = Singleton()
private init() { }
}
28. What is the @escaping closure in Swift and when is it used?
Answer:
The @escaping closure is used when a closure is allowed to escape the function it was passed into, meaning it can be stored and called later. This is necessary for closures used with asynchronous operations or completion handlers.
DispatchQueue.global().async {
// Perform async task
completion()
}
}
29. What is the purpose of the didSet and willSet property observers in Swift?
Answer:
didSet and willSet are property observers in Swift that allow you to run custom code when a property’s value changes. willSet is called before the property’s value is set, and didSet is called immediately after the new value is set.
willSet {
print(“Will set temperature to \(newValue)”)
}
didSet {
print(“Did set temperature from \(oldValue) to \(temperature)”)
}
}
30. How do you create and use an extension in Swift?
Answer:
An extension in Swift is used to add new functionality to existing classes, structs, enums, or protocols. Extensions can add computed properties, methods, and initializers.
func reversed() -> String {
return String(self.reversed())
}
}
let original = “Swift”
let reversed = original.reversed()
31. What is the difference between @IBInspectable and @IBDesignable?
Answer:
@IBInspectable is used to expose a property to Interface Builder so that you can modify it directly in the Attributes Inspector. @IBDesignable is used to allow Interface Builder to render a custom view in real-time, reflecting any custom drawing or modifications.
@IBInspectable var borderColor: UIColor = .black {
didSet {
layer.borderColor = borderColor.cgColor
}
}
}
32. What is the role of func in Swift?
Answer:
The func keyword is used to declare functions in Swift. Functions can have parameters and return values, and they are used to encapsulate code into reusable blocks.
return “Hello, \(name)!”
}
33. What are lazy properties and when should you use them?
Answer:
lazy properties are properties that are not initialized until they are first accessed. They are useful for properties that require significant computation or resources to initialize and are not needed immediately
lazy var data: [String] = {
// Load and return data
return [“Data”, “Loaded”] }()
}
34. How do you use the override keyword in Swift?
Answer:
The override keyword is used to indicate that a method, property, or initializer is intended to override a method, property, or initializer in a superclass. It ensures that you are correctly overriding functionality from the superclass.
func makeSound() {
print(“Generic sound”)
}
}
class Dog: Animal {
override func makeSound() {
print(“Woof”)
}
}
35. What is a computed property and how does it differ from a stored property?
Answer:
A computed property is a property that does not store a value but calculates it on demand. Unlike stored properties, which keep their value in memory, computed properties perform calculations every time they are accessed.
var width: Double
var height: Double
var area: Double {
return width * height
}
}
36. How do you handle asynchronous code in Swift?
Answer:
Asynchronous code in Swift can be handled using Grand Central Dispatch (GCD) or Operation Queues. Swift 5.5 introduced async/await syntax for more readable asynchronous code, allowing functions to be marked with async and awaited with await.
// Asynchronous fetch
return “Data”
}
37. What are typealiases and how are they used?
Answer:
typealias in Swift is used to create a new name for an existing type. This helps to simplify complex type definitions and improve code readability.
38. How do you use the @escaping attribute with closures?
Answer:
The @escaping attribute is used with closures that can be called after the function they were passed into has returned. This is common with asynchronous operations where the closure is executed later.
DispatchQueue.global().async {
completion()
}
}
39. What is the difference between synchronous and asynchronous programming?
Answer:
Synchronous programming executes tasks one after another, blocking the current thread until a task completes. Asynchronous programming allows tasks to run concurrently, freeing the main thread to perform other operations while waiting for tasks to finish. This leads to a more responsive application.
// Perform asynchronous task
}
40. What is the @objc attribute used for in Swift?
Answer:
The @objc attribute is used to expose Swift code to Objective-C. This allows Swift classes, methods, and properties to be accessed from Objective-C code. It’s often used when you need to interact with legacy Objective-C APIs or when using features like KVO (Key-Value Observing) and selectors.
@objc func myMethod() {
print(“This method is exposed to Objective-C”)
}
}
41. How do you define a method that accepts a closure as a parameter in Swift?
Answer:
You define a method that accepts a closure by specifying the closure type as a parameter. The closure type is defined with a list of parameters and a return type. For example, a closure that takes an Int and returns Void can be defined as (Int) -> Void.
let result = 10
completion(result)
}
42. What is the difference between let and var in Swift?
Answer:
In Swift, let is used to declare constants whose values cannot be changed after they are set. var is used to declare variables whose values can be modified. Using let ensures that the variable’s value remains constant throughout its lifetime.
var variableValue = 20
variableValue = 30 // This is allowed
43. What is type inference in Swift and how does it work?
Answer:
Type inference in Swift allows the compiler to automatically deduce the type of a variable or constant based on the assigned value. This means you do not always have to explicitly specify the type, as Swift will infer it from the context.
swift
Copy code
44. How do you handle errors in Swift?
Answer:
Error handling in Swift is done using do-catch blocks. Functions that can throw errors are marked with throws, and errors are caught and handled in the catch block. You can also use try? for optional error handling and try! for forced unwrapping.
case fileNotFound
}
func readFile() throws -> String {
throw FileError.fileNotFound
}
do {
let content = try readFile()
} catch FileError.fileNotFound {
print(“File not found”)
}
45. What is memory management in Swift and how does ARC work?
Answer:
Memory management in Swift is handled by Automatic Reference Counting (ARC). ARC automatically manages the memory of instances by keeping track of the number of references to each instance. When an instance’s reference count drops to zero, the memory occupied by the instance is deallocated.
var property: String
init(property: String) {
self.property = property
}
}
46. What is a guard statement in Swift and how is it used?
Answer:
The guard statement is used for early exits in a function or loop if certain conditions are not met. It helps to ensure that required conditions are satisfied and allows for cleaner, more readable code by handling failure cases early.
guard let unwrappedNumber = number else {
print(“Number is nil”)
return
}
print(“Number is \(unwrappedNumber)”)
}
47. How do you use @escaping and @autoclosure in Swift?
Answer:
@escaping is used with closures that are called after the function they were passed into has returned. @autoclosure is used to automatically create a closure from an expression. This is useful for lazy evaluation of expressions.
DispatchQueue.global().async {
closure()
}
}
func evaluate(_ expression: @autoclosure () -> Bool) {
if expression() {
print(“Expression is true”)
}
}
48. What is the difference between private, fileprivate, and internal access levels?
Answer:
private restricts access to the enclosing declaration and extensions, fileprivate allows access within the same file, and internal (the default) allows access within the same module. These access levels help encapsulate functionality and protect data integrity. Understanding these levels is essential for maintaining clean and safe code.
49. How do you define and use a protocol in Swift?
Answer:
A protocol in Swift defines a blueprint of methods, properties, and other requirements that can be adopted by classes, structs, and enums. Protocols are used to specify what methods or properties a conforming type must implement.
func greet() -> String
}
class Person: Greetable {
func greet() -> String {
return “Hello!”
}
}
50. What is functional programming and how does Swift support it?
Answer:
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. Swift supports functional programming with first-class functions, closures, and higher-order functions like map, filter, and reduce.
Final Words
Getting ready for an interview can feel overwhelming, but going through these Swift fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Swift interview but don’t forget to practice Swift syntax, optionals, closures, and protocol-oriented programming questions too.
Frequently Asked Questions
1. What are the most common interview questions for Swift?
Common Swift interview questions include topics such as optionals and how to handle them, differences between struct and class, memory management, Swift’s type safety, closures, and SwiftUI for interface development.
2. What are the important Swift topics freshers should focus on for interviews?
Freshers should focus on Swift fundamentals like optionals, value vs reference types, memory management (ARC), closures, protocols, error handling, generics, and if relevant, SwiftUI and UIKit for iOS app development.
3. How should freshers prepare for Swift technical interviews?
Freshers should have a strong understanding of Swift basics, practice writing code for common algorithms, understand Apple’s frameworks (e.g., UIKit, SwiftUI), and be able to explain key features like optionals, closures, and protocol-oriented programming.
4. What strategies can freshers use to solve Swift coding questions during interviews?
Start by fully understanding the problem, plan a solution, and use Swift’s strong type system, optionals, and error-handling features.
Write clean and efficient code using best practices like keeping methods short, making use of built-in Swift functions, and avoiding unnecessary force unwraps.
5. Should freshers prepare for advanced Swift topics in interviews?
Yes, freshers should prepare advanced topics like protocol-oriented programming, Swift’s memory management (ARC), async programming with Combine, Swift Concurrency (async/await), and functional programming concepts should be prepared.
Explore More Swift Resources
Explore More Interview Questions
- Python
- Java
- SQL
- React
- JavaScript
- C Programming
- HTML
- CSS
- Angular
- C++
- Spring Boot
- Node JS
- Excel
- C#
- DBMS
- PHP
- Linux
- Operating System
- MySQL
- Spring
- Flutter
- MongoDB
- Django
- React Native
- jQuery
- Bootstrap
- Embedded C
- DSA
- R Programming
- Hadoop
- .NET
- Power BI
- ASP.NET
- ASP.NET MVC
- Android
- Tableau
- MVC
- WordPress
- TypeScript
- Spark
- Kotlin
Related Posts
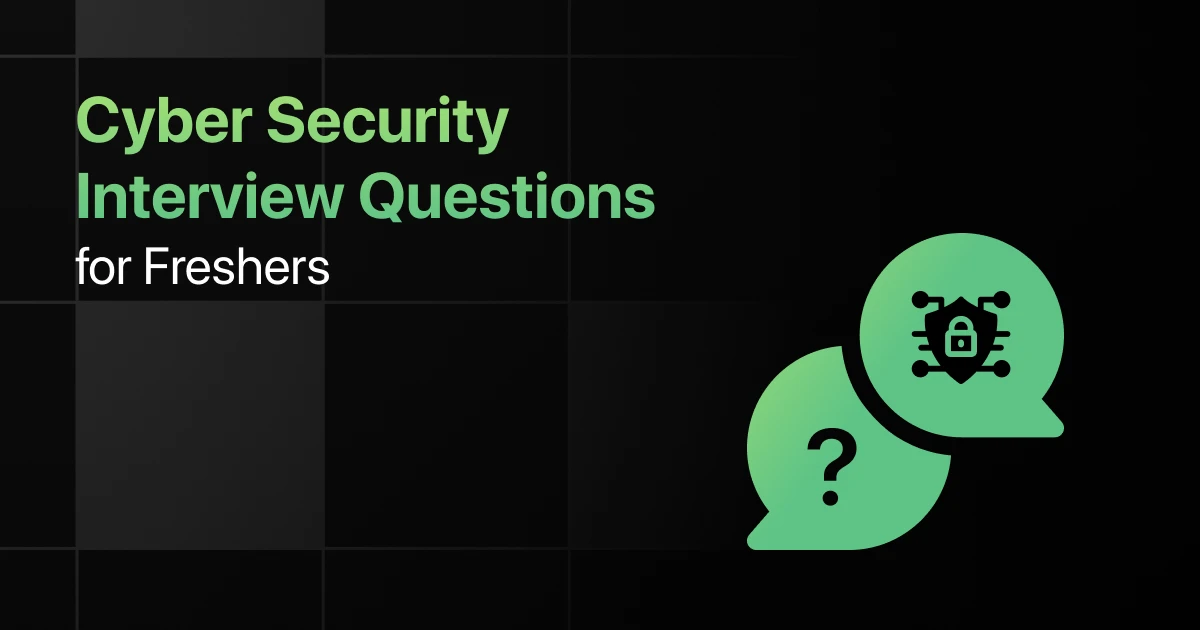
Top Cyber Security Interview Questions for Freshers
Are you preparing for your first Cyber Security interview and wondering what questions you might face? Understanding the key Cyber Security …