Top TypeScript Interview Questions for Freshers
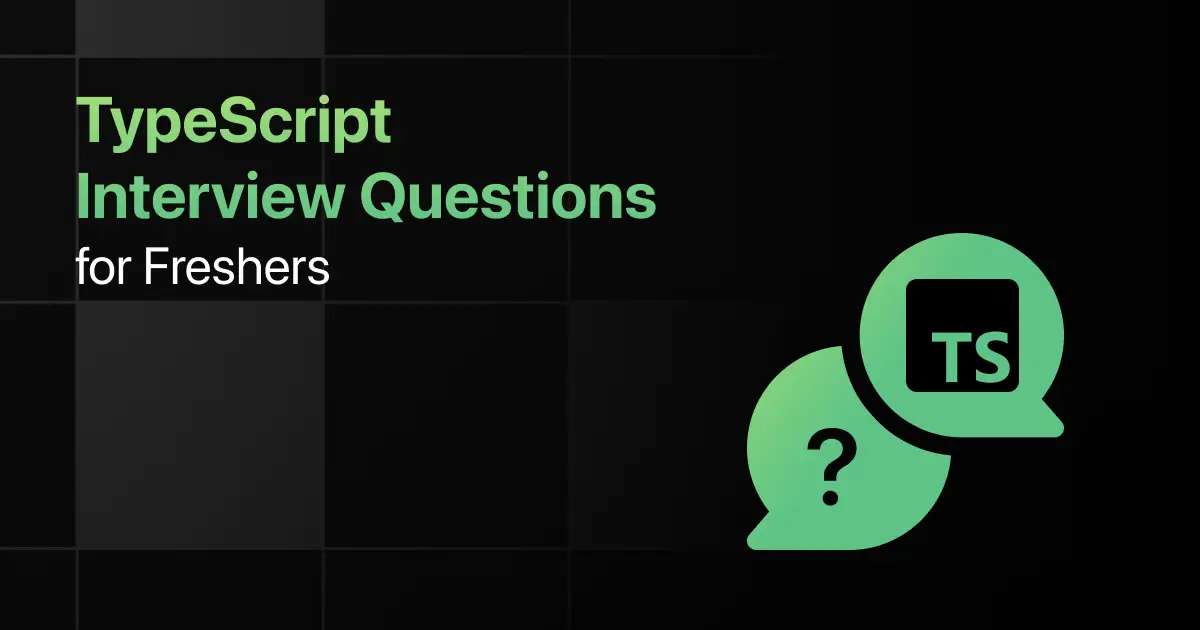
Are you preparing for your first TypeScript interview and wondering what questions you might face?
Understanding the key TypeScript interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these TypeScript interview questions and answers for freshers and make a strong impression in your interview.
Practice TypeScript Interview Questions and Answers
Below are the top 50 TypeScript interview questions for freshers with answers:
1. What is TypeScript?
Answer:
TypeScript is a strongly typed, object-oriented, compiled language. It is a superset of JavaScript, meaning any valid JavaScript is also valid TypeScript. It adds features like static typing, classes, and interfaces to JavaScript.
2. Why do we use TypeScript?
Answer:
TypeScript helps developers catch errors early during development, making the code more reliable and maintainable. It adds type definitions, which improve IDE support for autocompletion and refactoring. It also allows the use of modern JavaScript features, even on older JavaScript engines.
3. What are the key features of TypeScript?
Answer:
Some key features include static typing, interfaces, classes, and inheritance. It supports ES6 and ES7 features like async/await and modules. TypeScript also compiles to JavaScript, ensuring compatibility with various browsers.
4. How is TypeScript different from JavaScript?
Answer:
JavaScript is a loosely typed language, while TypeScript is strongly typed. TypeScript compiles to plain JavaScript and adds features like type checking, classes, and interfaces, which are not available in JavaScript by default.
5. How do you install TypeScript?
Answer:
You can install TypeScript globally using npm with the following command:
6. What is a .ts file?
Answer:
A .ts file is a TypeScript file. It contains TypeScript code, which is eventually compiled into JavaScript. These files use TypeScript syntax and must be compiled into .js files before running in a browser or Node.js environment.
7. What are types in TypeScript?
Answer:
Types define the kind of value a variable can hold. TypeScript uses static types like number, string, boolean, and custom types like interfaces or enums. They help to ensure variables are used in the intended manner.
8. What is static typing?
Answer:
Static typing means that variable types are known at compile time. This allows TypeScript to catch errors early before the code is executed. It contrasts with JavaScript’s dynamic typing, where types are checked at runtime.
9. How do you declare a variable in TypeScript?
Answer:
You can declare variables using let, const, or var. You can also explicitly specify the type during declaration.
const age: number = 30;
10. What are type annotations in TypeScript?
Answer:
Type annotations explicitly define the type of a variable or function parameter. They ensure that variables are assigned values of the correct type, making the code safer and more predictable.
let score: number = 95;
11. What is type inference in TypeScript?
Answer:
TypeScript can automatically infer the type of a variable based on the assigned value. If you declare a variable without a type, TypeScript will assign a type based on the initial value.
12. What is an interface in TypeScript?
Answer:
Interfaces define the structure of an object by specifying properties and their types. They act as a contract to ensure that objects adhere to a specific structure.
name: string;
age: number;
}
let john: Person = { name: “John”, age: 25 };
13. What are classes in TypeScript?
Answer:
Classes are blueprints for creating objects. They contain properties and methods and support inheritance. TypeScript adds strict type-checking and encapsulation to the classes.
model: string;
constructor(model: string) {
this.model = model;
}
}
let myCar = new Car(“Toyota”);
14. What are access modifiers in TypeScript?
Answer:
Access modifiers control the visibility of class members (properties or methods). TypeScript has three modifiers: public, private, and protected. public is accessible anywhere, private within the class, and protected within the class and its subclasses.
15. What is a constructor in TypeScript?
Answer:
A constructor is a special method in a class that is called when an instance of the class is created. It is used to initialize object properties and can accept parameters to set initial values.
16. What is inheritance in TypeScript?
Answer:
Inheritance allows one class to extend another class, inheriting its properties and methods. The extends keyword is used to create a subclass in TypeScript.
makeSound() {
console.log(“Animal sound”);
}
}
class Dog extends Animal {
bark() {
console.log(“Woof!”);
}
}
17. What is the difference between interface and type in TypeScript?
Answer:
Both interface and type allow you to define shapes of objects. However, interface is specifically used for object types, while type can define more complex types like unions, intersections, or primitive types.
18. What is an enum in TypeScript?
Answer:
Enums allow you to define a set of named constants, making your code more readable and easier to manage. They can be numeric or string-based.
Up = 1,
Down,
Left,
Right
}
19. What is a union type in TypeScript?
Answer:
Union types allow a variable to hold one of several types. This provides more flexibility while still enabling type safety.
value = “Hello”;
value = 10;
20. What is a tuple in TypeScript?
Answer:
A tuple is a type of array that has a fixed number of elements, where each element can have a different type. Tuples are useful when you know the exact number and type of elements in an array.
21. What is the any type in TypeScript?
Answer:
The any type allows you to assign any type of value to a variable. It disables type-checking, making it similar to JavaScript’s dynamic typing. Use it sparingly to avoid losing the benefits of TypeScript’s type system.
22. What is the unknown type in TypeScript?
Answer:
The unknown type is similar to any, but it is safer because you can’t perform operations on a value of type unknown without first narrowing the type through type checking.
23. What are generics in TypeScript?
Answer:
Generics allow you to create reusable components that work with a variety of data types. Instead of specifying a type when defining a function, class, or interface, you use a placeholder type.
return arg;
}
24. What is the void type in TypeScript?
Answer:
The void type represents the absence of a value. It is commonly used as the return type for functions that don’t return a value.
console.log(“This function returns nothing”);
}
25. What is the difference between null and undefined in TypeScript?
Answer:
null represents an intentional absence of value, while undefined indicates that a variable has been declared but has not been assigned a value. Both are subtypes of all types in TypeScript.
26. How do you create an array in TypeScript?
Answer:
Arrays in TypeScript can be created using two syntaxes: square brackets or Array<T> notation. The elements of the array must match the specified type.
let list2: Array<number> = [4, 5, 6];
27. What is type casting in TypeScript?
Answer:
Type casting allows you to tell the compiler that you know the type of a value better than TypeScript does. It helps when you want to treat a variable as a different type.
let strLength: number = (someValue as string).length;
28. What are namespaces in TypeScript?
Answer:
Namespaces provide a way to group related code together, preventing naming collisions. They help organize large codebases by grouping variables, functions, and classes into a single logical unit.
29. What are modules in TypeScript?
Answer:
Modules are a way to organize and reuse code by exporting and importing variables, functions, classes, and interfaces. They allow you to split your code into multiple files.
export const myFunction = () => “Hello”;
// main.ts
import { myFunction } from “./module”;
30. How do you handle exceptions in TypeScript?
Answer:
Exception handling in TypeScript works the same way as in JavaScript, using try, catch, and finally blocks. This helps manage runtime errors gracefully.
throw new Error(“An error occurred”);
} catch (error) {
console.error(error.message);
}
31. What is the never type in TypeScript?
Answer:
The never type represents values that never occur. It is used as a return type for functions that always throw an exception or never return.
throw new Error(“This function never returns”);
}
32. What is the Readonly modifier in TypeScript?
Answer:
The Readonly modifier ensures that the properties of an object cannot be modified after they are set. It makes the object immutable.
readonly name: string;
}
let person: Person = { name: “John” };
// person.name = “Jane”; // Error
33. What is type assertion in TypeScript?
Answer:
Type assertion is a way to override TypeScript’s inferred type and explicitly specify a different type. It is useful when you know more about the type of a value than TypeScript.
let length: number = (value as string).length;
34. What is type narrowing in TypeScript?
Answer:
Type narrowing is the process of refining the type of a variable based on control flow, such as type checks. This allows TypeScript to narrow a union type to a more specific type.
if (typeof value === “string”) {
console.log(value.length);
} else {
console.log(value);
}
}
35. What are decorators in TypeScript?
Answer:
Decorators are a special kind of declaration that can be attached to classes, methods, or properties to modify their behavior. They are widely used in frameworks like Angular.
36. What are TypeScript declaration files and how do they work?
Answer:
Declaration files (with a .d.ts extension) are used to describe the shape of JavaScript libraries, allowing TypeScript to understand the types of third-party libraries that don’t have built-in TypeScript support. They define the types and signatures of the library functions, so you can use JavaScript libraries with TypeScript type safety.
export function myFunction(a: string): void;
}
37. How do you use type guards in TypeScript?
Answer:
Type guards are a way of checking the type of a variable at runtime and allow you to narrow down the type within a conditional block. You can use typeof, instanceof, or custom type predicates to create type guards. They help make your code more predictable and safer by narrowing types.
if (typeof id === “string”) {
console.log(id.toUpperCase()); // TypeScript knows it’s a string here
} else {
console.log(id); // Here, TypeScript knows it’s a number
}
}
38. What is function overloading in TypeScript?
Answer:
Function overloading allows you to define multiple signatures for a function but with different parameters. TypeScript resolves which function signature to use based on the arguments passed to the function. However, all overloads share a common implementation.
function add(a: number, b: number): number;
function add(a: any, b: any): any {
return a + b;
}
39. What is type aliasing in TypeScript?
Answer:
Type aliasing allows you to create a new name for an existing type. This is useful for simplifying complex type declarations or creating reusable, self-documenting types. It can be used with primitive types, object types, and even union or intersection types.
let myPoint: Point = { x: 10, y: 20 };
40. How do you create a mapped type in TypeScript?
Answer:
Mapped types allow you to create new types by transforming an existing type’s properties. They are often used in scenarios where you need to make certain properties optional, readonly, or alter the type of the properties in bulk.
readonly [P in keyof T]: T[P];
};
interface Person {
name: string;
age: number;
}
const readonlyPerson: Readonly<Person> = { name: “John”, age: 25 };
// readonlyPerson.name = “Jane”; // Error: Cannot assign to ‘name’ because it is a read-only property.
41. What is the keyof operator in TypeScript?
Answer:
keyof is a TypeScript operator that returns a union of the keys of a given object type. It is commonly used in generic functions to restrict the input to known property names of an object.
model: string;
year: number;
}
type CarKeys = keyof Car; // ‘model’ | ‘year’
42. What is conditional typing in TypeScript?
Answer:
Conditional types allow you to create types that change based on a condition. They follow the pattern T extends U ? X : Y, meaning if T extends U, the type will be X; otherwise, it will be Y. This is useful for making flexible, reusable types.
let msg: Message<string>; // inferred as string
let count: Message<number>; // inferred as number
43. What is the difference between Partial and Required utility types in TypeScript?
Answer:
- Partial<T> makes all properties of type T optional.
- Required<T> makes all properties of type T required (non-optional). These utility types are useful for quickly modifying object types without rewriting the entire structure.
name: string;
age?: number;
}
let partialPerson: Partial<Person> = { name: “John” };
let requiredPerson: Required<Person> = { name: “John”, age: 25 }; // ‘age’ is required here
44. How does the Record utility type work in TypeScript?
Answer:
The Record<K, T> utility type creates an object type with a set of keys K of one type and values T of another type. It is used when you want to create an object that maps keys to specific value types.
type FruitPrices = Record<Fruit, number>;
const prices: FruitPrices = { apple: 10, banana: 5, orange: 8 };
45. What is an index signature in TypeScript?
Answer:
An index signature allows you to define the shape of an object where you don’t know all the property names, but you know the types of the keys and values. This is useful when creating dynamic or flexible objects.
[key: string]: string;
}
const user: StringMap = { name: “Alice”, city: “Paris” };
46. What is the Exclude utility type in TypeScript?
Answer:
The Exclude<T, U> utility type removes from T all types that are assignable to U. It is helpful when you want to exclude certain values from a union type.
type WithoutA = Exclude<T, “a”>; // ‘b’ | ‘c’
47. What is the Omit utility type in TypeScript?
Answer:
Omit<T, K> creates a new type by removing one or more properties K from type T. This is useful when you want to exclude certain properties from an object type.
name: string;
age: number;
city: string;
}
type PersonWithoutAge = Omit<Person, “age”>; // name and city remain
48. How do you create a custom utility type in TypeScript?
Answer:
Custom utility types allow you to create reusable types by leveraging TypeScript’s type manipulation capabilities like conditional types, mapped types, and utility types. You can create types that follow specific patterns in your codebase.
let username: Nullable<string> = null;
49. What is discriminated union in TypeScript and how do you use it?
Answer:
A discriminated union is a pattern where each type in a union has a common property (the “discriminant”) that allows TypeScript to narrow the type of an object. This is often used in conjunction with tagged unions for type-safe conditional logic.
kind: “square”;
size: number;
}
interface Circle {
kind: “circle”;
radius: number;
}
type Shape = Square | Circle;
function getArea(shape: Shape) {
if (shape.kind === “square”) {
return shape.size * shape.size;
} else if (shape.kind === “circle”) {
return Math.PI * shape.radius ** 2;
}
}
50. How do you use the infer keyword in TypeScript?
Answer:
The infer keyword is used in conditional types to infer a type within the true branch of the condition. This allows you to extract and manipulate types based on the structure of other types.
function getString(): string {
return “Hello”;
}
type StringReturnType = ReturnType<typeof getString>; // inferred as string
Final Words
Getting ready for an interview can feel overwhelming, but going through these TypeScript fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your TypeScript interview, but don’t forget to practice TypeScript syntax, classes, interfaces, and type safety-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for TypeScript?
Common TypeScript interview questions include types and interfaces, class vs interface, generics, type inference, decorators, and how TypeScript integrates with JavaScript libraries.
2. What are the important TypeScript topics freshers should focus on for interviews?
Freshers should focus on types, interfaces, classes, generics, modules, type assertions, and how to convert JavaScript code into TypeScript while ensuring type safety.
3. How should freshers prepare for TypeScript technical interviews?
Freshers should practice converting JavaScript code to TypeScript, understand static typing, explore type inference, and work with interfaces and classes. Studying common patterns used in TypeScript is also crucial.
4. What strategies can freshers use to solve TypeScript coding questions during interviews?
Freshers should carefully read the problem to understand the required types, use TypeScript’s static type-checking to prevent errors, and apply interfaces, classes, and generics to write reusable code.
5. Should freshers prepare for advanced TypeScript topics in interviews?
Yes, freshers should be ready to discuss advanced topics like generics, decorators, working with third-party libraries, handling complex types, and TypeScript’s interaction with JavaScript frameworks like React or Angular.
Explore More TypeScript Resources
Explore More Interview Questions
Related Posts
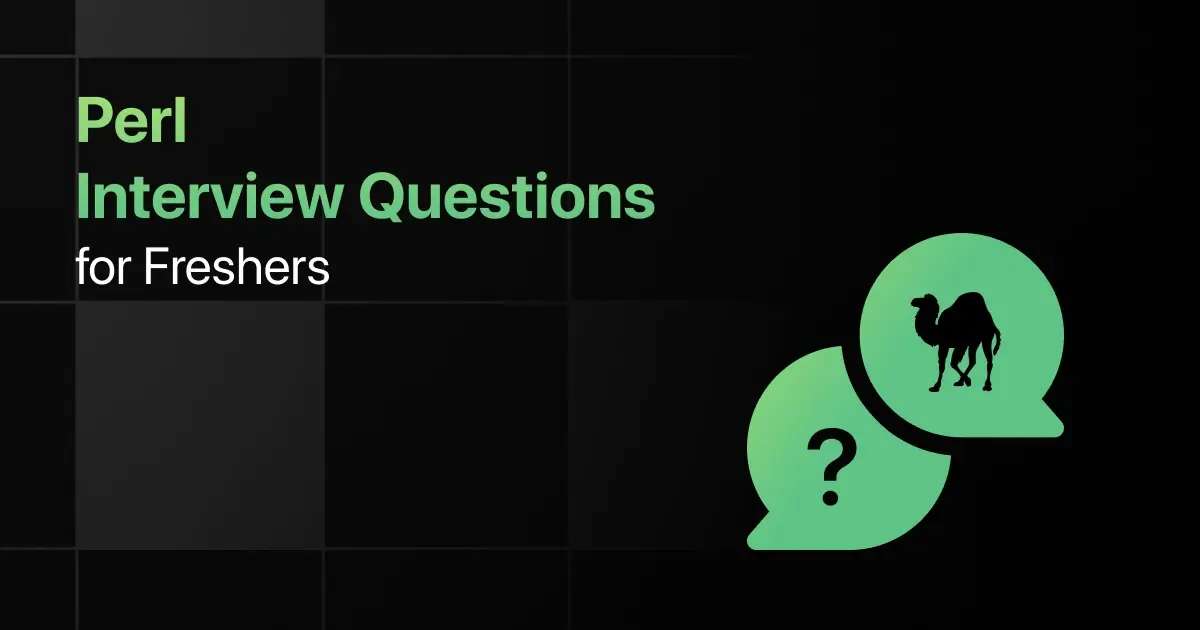
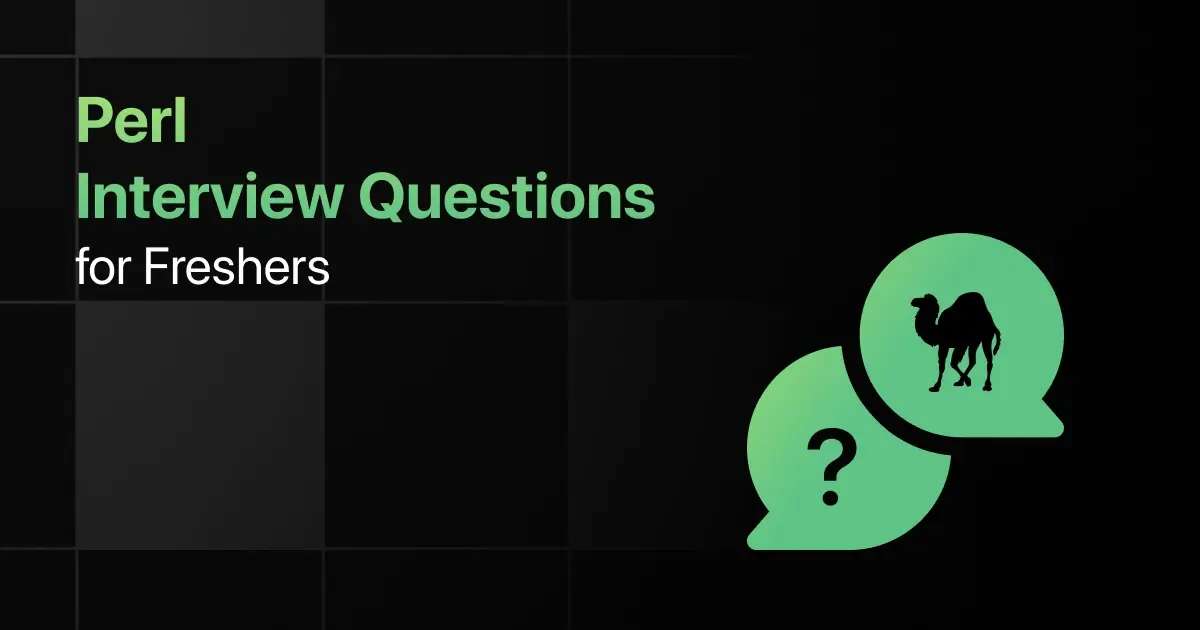
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …