Top R Programming Interview Questions for Freshers
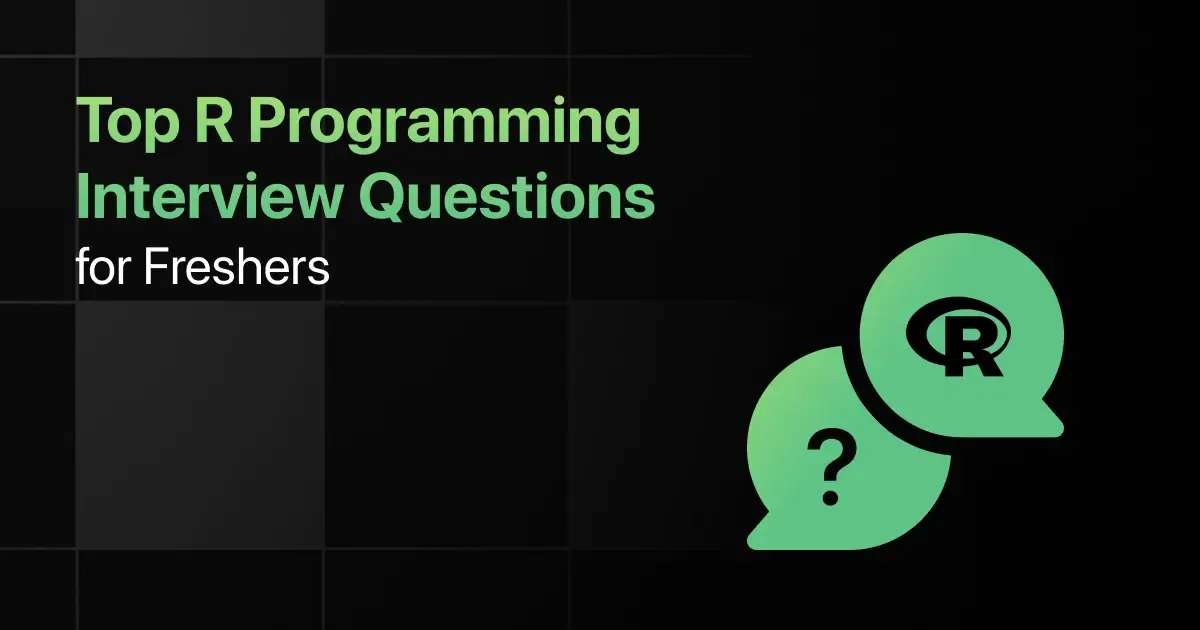
Are you preparing for your first R programming interview and wondering what questions you might face?
Understanding the key R programming interview questions for freshers can
give you more clarity.
With this guide, you’ll be well-prepared to tackle these R programming interview questions and answers for freshers and make a strong impression in your interview.
Practice R Programming Interview Questions and Answers
Below are the top 50 R programming interview questions for freshers with answers:
1. What is R programming?
Answer:
R is a programming language used for statistical computing, data analysis, and graphical representation.
R is particularly useful for data science, with numerous built-in functions for statistical tests, modeling, and visualization.
mean(x) # Outputs mean of the vector
2. How do you create a vector in R?
Answer:
A vector is created using the c() function, which combines values into a single vector.
Vectors are the most basic data structures in R and can store numeric, character, or logical data.
3. What is the difference between a matrix and a data frame in R?
Answer:
A matrix contains only one type of data (numeric or character), whereas a data frame can store multiple data types (numeric, character, etc.) in its columns.
A matrix is used for homogeneous data, while a data frame is more versatile, especially for datasets with mixed data types.
df <- data.frame(Name=c(“John”, “Alice”), Age=c(30, 25))
4. How can you check the structure of a data frame in R?
Answer:
You can use the str() function to display the internal structure of an R object.
This function is especially useful for understanding the types of data within a data frame.
5. How do you handle missing values in R?
Answer:
R represents missing values with NA. You can use is.na() to identify or remove them, or na.omit() to remove rows with missing values.
Handling missing values is crucial for ensuring the accuracy of statistical analysis.
is.na(data) # Returns TRUE for missing values
6. How do you install and load a package in R?
Answer:
You install a package using install.packages(“package_name”) and load it with library(package_name).
R has a vast repository of packages, making it powerful for specific tasks such as data manipulation or machine learning.
library(dplyr)
7. What is the purpose of the apply() function in R?
Answer:
The apply() function applies a function to the rows or columns of a matrix or data frame.
This function is useful for operations across rows or columns without explicitly writing loops.
8. How do you subset a data frame in R?
Answer:
You can subset a data frame by using indexing ([]), the subset() function, or dplyr functions like filter().
Subsetting helps focus on specific data by extracting relevant rows or columns.
df[df$Age > 25, ]
9. What are factors in R, and how are they useful?
Answer:
Factors are variables in R that categorize data and store it as levels. They are used for categorical data like gender or educational level.
Factors help with efficient storage and enable statistical modeling for categorical data.
levels(gender)
10. How do you merge two data frames in R?
Answer:
You can use the merge() function to combine two data frames by common columns or row names.
Merging data frames is essential for combining datasets from different sources.
df2 <- data.frame(ID=1:3, Age=c(25, 30, 35))
merge(df1, df2, by=”ID”)
11. Explain how to create a function in R.
Answer:
In R, functions are created using the function() keyword. A function is a block of code designed to perform a specific task.
Functions make code reusable and modular.
return(x + y)
}
add(2, 3)
12. How do you plot data in R?
Answer:
You can use the plot() function to create simple plots or the ggplot2 package for advanced visualizations.
Visualization is a key strength of R, helping to understand data patterns.
13. How do you read a CSV file in R?
Answer:
Use read.csv() to import CSV files into R as a data frame.
Reading data from files is one of the first steps in data analysis.
14. How do you write data to a CSV file in R?
Answer:
You can write data to a CSV file using write.csv().
This function is useful for exporting processed or analyzed data.
15. What is a list in R?
Answer:
A list is a collection of objects in R, such as vectors, data frames, or matrices.
Lists are useful for storing data of different types in a single object.
16. What is the use of lapply() and sapply() in R?
Answer:
Both lapply() and sapply() apply a function to each element of a list. lapply() returns a list, while sapply() simplifies the result to a vector or matrix.
These functions are ideal for applying the same operation to multiple elements.
sapply(my_list, mean)
17. How do you sort data in R?
Answer:
You can use sort() to sort vectors and order() for data frames.
Sorting is often necessary for organizing data before analysis.
18. What is the difference between NA and NaN in R?
Answer:
NA represents missing values, while NaN refers to computational errors (e.g., 0/0).
It’s essential to differentiate between the two during data cleaning.
is.na(x) # Identifies missing values
19. What is a dataframe in R?
Answer:
A dataframe is a table where columns can have different data types. It is similar to a spreadsheet or SQL table.
Dataframes are used to store datasets in a structured way for analysis.
20. How do you combine two vectors in R?
Answer:
Use the c() function to concatenate vectors.
Combining vectors is useful when merging data from different sources.
v2 <- c(4, 5, 6)
combined <- c(v1, v2)
21. How do you rename columns in a data frame in R?
Answer:
You can rename columns using the names() function or colnames() function.
This is useful for making column names more descriptive or aligning them with dataset standards.
22. What is the purpose of grep() in R?
Answer:
grep() is used to search for patterns within strings in vectors.
This function is valuable for data cleaning and extraction based on specific patterns.
23. How do you filter rows in a data frame using dplyr?
Answer:
You use the filter() function from dplyr to select rows based on conditions.
Filtering is essential for narrowing down data to the most relevant observations.
filtered_df <- filter(df, Age > 25)
24. How do you generate sequences in R?
Answer:
You can generate sequences using the seq() function.
This is often used to create evenly spaced intervals or repetitive data.
25. What is the difference between data.frame() and tibble() in R?
Answer:
data.frame() creates a regular data frame, while tibble() (from the tibble package) provides a more modern, user-friendly version.
Tibbles offer better error messages and do not convert strings to factors by default.
my_tibble <- tibble(Name=c(“Alice”, “Bob”), Age=c(25, 30))
26. What is the use of mutate() in dplyr?
Answer:
mutate() adds new variables or modifies existing ones within a data frame.
It simplifies the process of creating new columns based on existing data.
27. How do you calculate summary statistics in R?
Answer:
Functions like mean(), median(), sd(), and summary() provide summary statistics for a vector or data frame.
These functions are key for understanding data distributions and variability.
28. What is a for loop in R, and how do you use it?
Answer:
A for loop repeats a block of code a set number of times based on a defined sequence.
For loops are helpful for iterating through elements of a vector or list.
print(i)
}
29. How do you perform a t-test in R?
Answer:
You can perform a t-test using the t.test() function, which compares means between two groups.
T-tests are commonly used in hypothesis testing for comparing group differences.
30. What is the use of paste() in R?
Answer:
paste() is used to concatenate strings or variables into a single character string.
This is useful for generating labels or combining text with variable values.
31. How do you create a bar plot in R?
Answer:
You can create a bar plot using the barplot() function.
Bar plots are useful for visualizing categorical data distributions.
barplot(counts)
32. What is the difference between sapply() and lapply() in R?
Answer:
sapply() simplifies the output to a vector or matrix, while lapply() returns a list.
These functions allow you to apply a function over lists or vectors without writing loops.
sapply(1:3, sqrt)
33. How do you find the correlation between two variables in R?
Answer:
You can use the cor() function to compute the correlation between two numeric variables.
Correlation is essential for understanding relationships between continuous variables.
34. How do you create a scatter plot in R?
Answer:
You use the plot() function to create scatter plots for visualizing relationships between two continuous variables.
Scatter plots help reveal trends, correlations, or outliers in data.
35. What is the difference between plot() and ggplot()?
Answer:
plot() is a base R function for simple plots, while ggplot() from the ggplot2 package allows more advanced and customizable visualizations.
ggplot() is preferred for creating layered, aesthetic plots.
ggplot(df, aes(x=Age, y=Height)) + geom_point()
36. How do you reshape data in R?
Answer:
You can reshape data using functions like reshape(), gather(), or spread() from tidyverse.
Reshaping is useful for converting data from wide to long format or vice versa.
df_long <- gather(df, key=”Variable”, value=”Value”, -ID)
37. What is the purpose of setwd() and getwd() in R?
Answer:
setwd() sets the working directory, while getwd() returns the current working directory.
These functions are important for managing file paths and accessing datasets.
getwd()
38. How do you create a boxplot in R?
Answer:
You can create a boxplot using the boxplot() function, which visualizes the distribution of data and highlights outliers.
Boxplots are commonly used in exploratory data analysis.
39. How do you apply a function to every row in a data frame in R?
Answer:
You can use apply() to apply a function to rows of a matrix or data frame.
This is useful when performing row-wise calculations.
40. What is the use of summary() in R?
Answer:
summary() provides summary statistics, including the minimum, maximum, mean, and quartiles, for each variable in a data frame.
This function is essential for quickly understanding a dataset.
41. How do you calculate the variance and standard deviation in R?
Answer:
Use var() for variance and sd() for standard deviation.
These metrics are crucial for measuring data dispersion.
sd(df$Age)
42. What are the different types of joins in R using dplyr?
Answer:
The common joins include inner_join(), left_join(), right_join(), and full_join(). These join functions are used to merge datasets based on common keys.
43. How do you create a histogram in R?
Answer:
Use the hist() function to create a histogram, which shows the frequency distribution of a numeric variable.
Histograms are helpful for understanding the shape of data distributions.
44. How do you append rows to a data frame in R?
Answer:
You can append rows using rbind() or bind_rows() from dplyr.
This is often used when adding new observations to an existing dataset.
45. How do you remove duplicates from a data frame in R?
Answer:
Use the distinct() function from dplyr to remove duplicate rows.
This function ensures data integrity by eliminating repeated observations.
46. How do you calculate cumulative sums in R?
Answer:
Use the cumsum() function to calculate cumulative sums of a vector or column.
Cumulative sums are useful for tracking running totals or progressive data.
47. How do you handle dates in R?
Answer:
You can handle dates using as.Date() to convert strings to date objects, or use the lubridate package for more advanced date manipulations.
Dates are essential for time-series analysis.
48. How do you check the data type of an object in R?
Answer:
Use the class() function to check the data type of any object.
Knowing the data type is important for selecting the right operations.
49. How do you check the data type of an object in R?
Answer:
Use the class() function to check the type of any object in R, such as numeric, character, or factor.
Understanding the data type is crucial for performing appropriate operations, as R handles different data types with different methods.
50. How do you create and manipulate factors in R?
Answer:
You can create a factor using the factor() function and modify its levels using levels().
Factors are useful for categorical data, such as gender or education level, which can be ordered or unordered.
levels(factor_data)
51. How do you remove a column from a data frame in R?
Answer:
You can remove a column by setting it to NULL or using the select() function from dplyr.
Removing unnecessary columns is essential for optimizing data analysis and storage.
# Or using dplyr
df <- select(df, -Age)
Final Words
Getting ready for an interview can feel overwhelming, but going through these R programming fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your R programming interview, but don’t forget to practice R basics, data manipulation, and data visualization-related interview questions too.
Frequently Asked Questions
1. What are the most common interview questions for R programming?
Common R programming interview questions focus on data manipulation, data visualization, R syntax, using libraries like dplyr and ggplot2, basic statistical functions, and handling data frames, lists, and vectors.
2. What are the important R programming topics freshers should focus on for interviews?
Freshers should focus on topics such as R data types (vectors, lists, data frames), control structures (loops, if-else), functions, data wrangling with dplyr, data visualization with ggplot2, statistical analysis, and importing/exporting data.
3. How should freshers prepare for R programming technical interviews?
Freshers should practice by solving data manipulation problems, working on projects that involve data cleaning and visualization, getting comfortable with R’s statistical functions, and reviewing key libraries (dplyr, tidyverse, ggplot2) and packages for data analysis.
4. What strategies can freshers use to solve R programming coding questions during interviews?
Freshers should break problems into smaller steps, use R’s vectorized operations to improve efficiency, understand how to manipulate data frames effectively, and utilize libraries like dplyr and tidyr for cleaner and more concise code.
5. Should freshers prepare for advanced R programming topics in interviews?
Yes, freshers should have a basic understanding of advanced topics like statistical modeling, machine learning algorithms using caret, working with large datasets, and optimizing R code for performance, as these can make them stand out.
Explore More R Programming Resources
Explore More Interview Questions
Related Posts
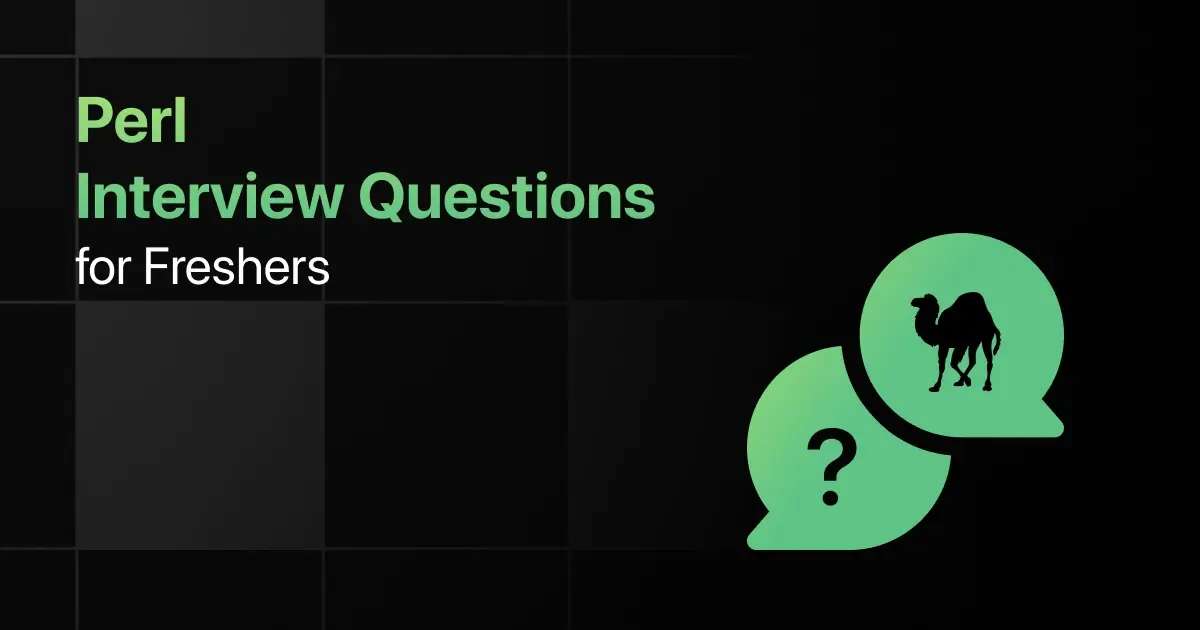
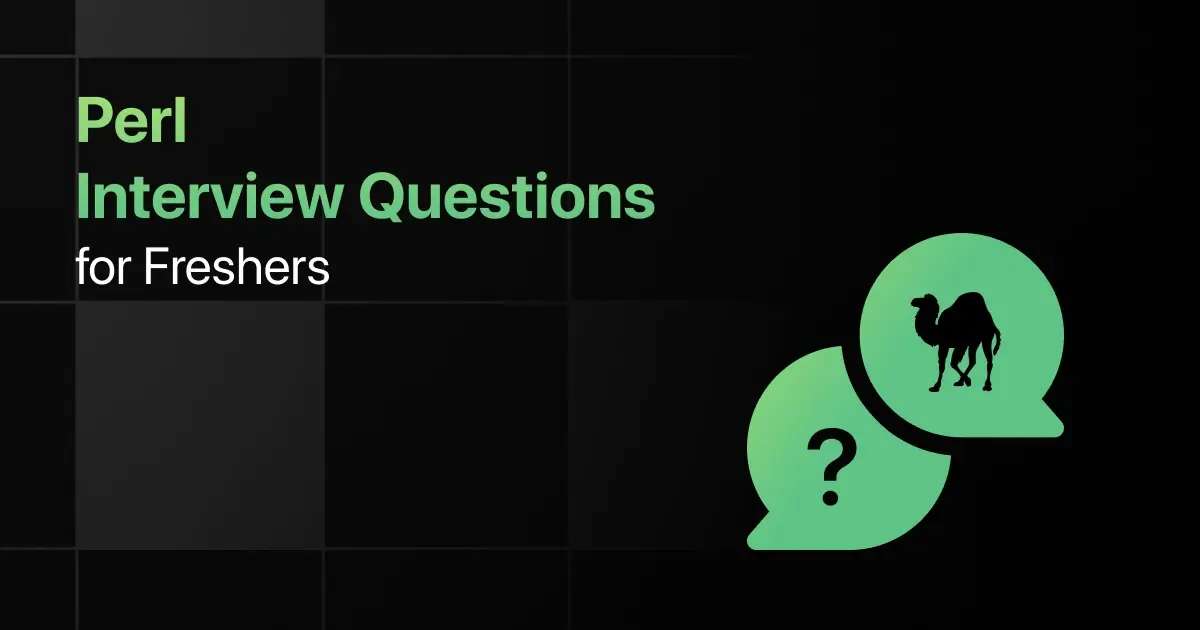
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …