Top Spring Boot Interview Questions for Freshers
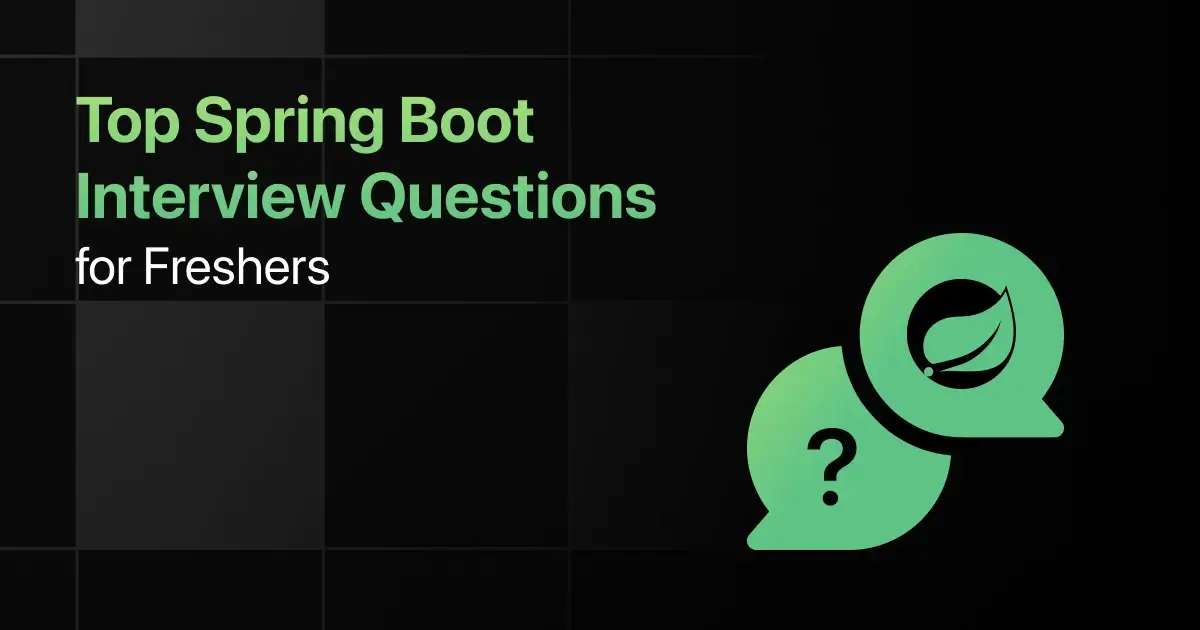
Are you preparing for your first Spring Boot interview and wondering what questions you might face?
Understanding the key Spring Boot interview questions for freshers can give you more clarity.
With this guide, you’ll be well-prepared to tackle these Spring Boot interview questions and answers for freshers and make a strong impression in your interview.
Practice Spring Boot Interview Questions and Answers
Below are the top 50 Spring Boot interview questions for freshers with answers:
1. How do you create a basic Spring Boot application using the @SpringBootApplication annotation?
Answer:
Use the @SpringBootApplication annotation to mark the main class, which serves as the entry point for the Spring Boot application.
@SpringBootApplication
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
}
2. How do you configure an embedded server’s port in a Spring Boot application?
Answer:
You can set the server port using the application.properties or application.yml file.
server.port=8081
3. Write a Spring Boot application that reads a custom configuration property and prints it.
Answer:
Use @Value to inject the custom property and print it in a @PostConstruct method.
@Value(“${my.custom.property}”)
private String customProperty;
@PostConstruct
public void printProperty() {
System.out.println(customProperty);
}
4. How do you handle application startup tasks in Spring Boot?
Answer:
Implement the CommandLineRunner interface to execute code after the application context is loaded.
@Bean
public CommandLineRunner run() {
return args -> System.out.println(“Application started”);
}
5. How do you create a simple REST controller in Spring Boot?
Answer:
Use @RestController and @RequestMapping to define endpoints that return JSON responses.
@RestController
@RequestMapping(“/api”)
public class MyController {
@GetMapping(“/hello”)
public String sayHello() {
return “Hello, World!”;
}
}
6. How do you define and use multiple profiles in a Spring Boot application?
Answer:
Use the @Profile annotation to activate different beans based on the active profile.
@Bean
@Profile(“dev”)
public MyService devService() {
return new MyService(“Development Service”);
}
7. How do you load different configuration files for different environments in Spring Boot?
Answer:
Use profile-specific configuration files like application-dev.properties and application-prod.properties.
# application-dev.properties
my.custom.property=devValue
# application-prod.properties
my.custom.property=prodValue
8. How do you externalize application properties in Spring Boot using environment variables?
Answer:
Set the environment variable and reference it in the application.properties file.
my.custom.property=${MY_ENV_VARIABLE}
9. Write a Spring Boot application that conditionally loads a bean based on a property value.
Answer:
Use the @ConditionalOnProperty annotation to conditionally load beans.
@Bean
@ConditionalOnProperty(name = “feature.enabled”, havingValue = “true”)
public MyService featureService() {
return new MyService(“Feature Service”);
}
10. How do you configure a Spring Boot application to log different levels for different packages?
Answer:
Configure logging levels in the application.properties file for specific packages.
logging.level.com.example=DEBUG
logging.level.org.springframework=WARN
11. How do you handle a POST request in a Spring Boot REST controller to create a new resource?
Answer:
Use @PostMapping to handle POST requests and @RequestBody to bind the request payload to an object.
@PostMapping(“/users”)
public User createUser(@RequestBody User user) {
return userService.save(user);
}
12. Write a Spring Boot controller method that returns a list of resources in JSON format.
Answer:
Use @GetMapping to define the endpoint and return a list of resources.
@GetMapping(“/users”)
public List<User> getAllUsers() {
return userService.findAll();
}
13. How do you handle path variables in Spring Boot REST endpoints?
Answer:
Use @PathVariable to extract values from the URI path.
@GetMapping(“/users/{id}”)
public User getUserById(@PathVariable Long id) {
return userService.findById(id);
}
14. How do you implement exception handling for RESTful services in Spring Boot?
Answer:
Use @ControllerAdvice and @ExceptionHandler to handle exceptions globally.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
public ResponseEntity<String> handleUserNotFound(UserNotFoundException ex) {
return new ResponseEntity<>(ex.getMessage(), HttpStatus.NOT_FOUND);
}
}
15. Write a Spring Boot application that validates a request body using @Valid.
Answer:
Use @Valid in the controller method and annotate the fields in the request object.
@PostMapping(“/users”)
public User createUser(@Valid @RequestBody User user) {
return userService.save(user);
}
16. How do you secure a Spring Boot REST API using basic authentication?
Answer:
Use HttpSecurity in the WebSecurityConfigurerAdapter to configure basic authentication.
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.anyRequest().authenticated()
.and().httpBasic();
}
17. How do you restrict access to certain endpoints in a Spring Boot application based on user roles?
Answer:
Use @PreAuthorize to restrict access based on roles.
@PreAuthorize(“hasRole(‘ADMIN’)”)
@GetMapping(“/admin”)
public String adminEndpoint() {
return “Admin access”;
}
18. Write a Spring Boot configuration that disables CSRF protection for a specific endpoint.
Answer:
Use csrf().disable() in the security configuration to disable CSRF protection.
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers(“/public/**”).permitAll()
.anyRequest().authenticated();
}
19. How do you implement JWT authentication in a Spring Boot application?
Answer:
Use a filter to validate JWT tokens and set the authentication in the security context.
public class JwtFilter extends OncePerRequestFilter {
protected void doFilterInternal(HttpServletRequest req, HttpServletResponse res, FilterChain chain) {
// Extract and validate JWT
}
}
20. How do you customize the login form in a Spring Boot security configuration?
Answer:
Use formLogin() to customize the login page and success/failure handlers.
@Override
protected void configure(HttpSecurity http) throws Exception {
http.formLogin()
.loginPage(“/login”)
.defaultSuccessURL(“/home”)
.failureUrl(“/login?error=true”);
}
21. Write a Spring Boot repository method to find all users with a specific role using JPA.
Answer:
Define a query method in the repository interface to fetch users by role.
List<User> findByRole(String role);
22. How do you implement pagination in a Spring Boot REST API using Spring Data JPA?
Answer:
Use Pageable in the repository method and pass it to the service layer.
Page<User> findAll(Pageable pageable);
23. How do you execute a native SQL query in Spring Boot using JPA?
Answer:
Use the @Query annotation with nativeQuery set to true.
@Query(value = “SELECT * FROM users WHERE email = ?1”, nativeQuery = true)
User findByEmail(String email);
24. Write a Spring Boot service method that updates a user’s details in the database.
Answer:
Use the repository’s save() method to update the user details.
public User updateUser(User user) {
return userRepository.save(user);
}
25. How do you handle transactions in a Spring Boot application using @Transactional?
Answer:
Annotate the service method with @Transactional to manage transactions.
@Transactional
public void transferMoney(Long fromAccountId, Long toAccountId, BigDecimal amount) {
// Transfer logic
}
26. How do you write a unit test for a Spring Boot service class using Mockito?
Answer:
Use @MockBean to mock dependencies and verify service behavior.
@MockBean
private UserRepository userRepository;
@Test
public void testFindUserById() {
when(userRepository.findById(1L)).thenReturn(Optional.of(new User(“John”)));
assertEquals(“John”, userService.findUserById(1L).getName());
}
27. Write an integration test for a Spring Boot REST controller using @SpringBootTest.
Answer:
Use @SpringBootTest and TestRestTemplate to perform integration tests.
@Test
public void testGetUser() {
ResponseEntity<User> response = restTemplate.getForEntity(“/api/users/1”, User.class);
assertEquals(HttpStatus.OK, response.getStatusCode());
assertEquals(“John”, response.getBody().getName());
}
28. How do you test a Spring Boot application with an in-memory database?
Answer:
Use H2 in-memory database and configure it in application.properties.
spring.datasource.url=jdbc:h2:mem:testdb
29. Write a Spring Boot test that verifies the loading of the application context.
Answer:
Use @SpringBootTest with a simple test method to check context loading.
@SpringBootTest
public class ApplicationContextTest {
@Test
void contextLoads() {
}
}
30. How do you test a Spring Boot service method that involves a transaction?
Answer:
Use @Transactional in the test class and verify the transaction behavior.
@Test
@Transactional
public void testTransactionalMethod() {
userService.updateUser(user);
assertEquals(“UpdatedName”, userRepository.findById(user.getId()).get().getName());
}
31. How do you send a message to a Kafka topic in a Spring Boot application?
Answer:
Use the KafkaTemplate to send messages to a Kafka topic.
@Autowired
private KafkaTemplate<String, String> kafkaTemplate;
public void sendMessage(String topic, String message) {
kafkaTemplate.send(topic, message);
}
32. Write a Spring Boot application that consumes messages from a RabbitMQ queue.
Answer:
Use @RabbitListener to consume messages from the specified queue.
@RabbitListener(queues = “myQueue”)
public void receiveMessage(String message) {
System.out.println(“Received: ” + message);
}
33. How do you configure a JMS queue in a Spring Boot application?
Answer:
Use @JmsListener to listen to a JMS queue and configure the connection factory.
@JmsListener(destination = “myQueue”)
public void receiveMessage(String message) {
System.out.println(“Received: ” + message);
}
34. Write a Spring Boot method that sends an email using JavaMailSender.
Answer:
Use JavaMailSender to compose and send an email.
@Autowired
private JavaMailSender mailSender;
public void sendEmail(String to, String subject, String text) {
SimpleMailMessage message = new SimpleMailMessage();
message.setTo(to);
message.setSubject(subject);
message.setText(text);
mailSender.send(message);
}
35. How do you configure a REST endpoint in Spring Boot to asynchronously process a request?
Answer:
Use @Async in the service method to process requests asynchronously.
@Async
public CompletableFuture<String> asyncMethod() {
return CompletableFuture.completedFuture(“Hello, Async”);
}
36. How do you expose custom metrics in a Spring Boot application using Actuator?
Answer:
Use @Component and @Timed annotations to expose custom metrics.
@Timed(value = “custom.metric.time”, description = “Time taken to execute method”)
public String customMethod() {
return “Custom Metric”;
}
37. Write a Spring Boot actuator endpoint that provides custom health check information.
Answer:
Implement HealthIndicator to create a custom health check endpoint.
@Component
public class CustomHealthIndicator implements HealthIndicator {
@Override
public Health health() {
return Health.up().withDetail(“Custom”, “Available”).build();
}
}
38. How do you customize the Spring Boot Actuator endpoints to change their default path?
Answer:
Use management.endpoints.web.base-path in application.properties.
management.endpoints.web.base-path=/manage
39. Write a Spring Boot application that uses Actuator to monitor HTTP request metrics.
Answer:
Include the micrometer-core dependency and expose HTTP request metrics using @Timed.
@Timed(value = “http.requests”, description = “HTTP Request Metrics”)
@GetMapping(“/metrics”)
public String metrics() {
return “Metrics”;
}
40. How do you secure Spring Boot Actuator endpoints?
Answer:
Configure security settings in application.properties or application.yml to restrict access.
management.endpoints.web.exposure.include=health,info
management.endpoint.health.roles=ADMIN
41. How do you implement service discovery in a Spring Boot microservice using Netflix Eureka?
Answer:
Annotate the main class with @EnableEurekaClient and configure Eureka in application.properties.
eureka.client.serviceUrl.defaultZone=http://localhost:8761/eureka/
42. Write a Spring Boot application that acts as a Netflix Zuul API Gateway.
Answer:
Use @EnableZuulProxy in the main class to create an API Gateway.
@EnableZuulProxy
@SpringBootApplication
public class ApiGatewayApplication {
public static void main(String[] args) {
SpringApplication.run(ApiGatewayApplication.class, args);
}
}
43. How do you implement client-side load balancing in a Spring Boot application using Ribbon?
Answer:
Use @LoadBalanced annotation with RestTemplate to enable Ribbon for load balancing.
@Bean
@LoadBalanced
public RestTemplate restTemplate() {
return new RestTemplate();
}
44. Write a Spring Boot application that implements circuit breaking using Netflix Hystrix.
Answer:
Use @HystrixCommand to define fallback methods for circuit-breaking.
@HystrixCommand(fallbackMethod = “fallback”)
public String callExternalService() {
return restTemplate.getForObject(“http://external-service/api”, String.class);
}
public String fallback() {
return “Fallback response”;
}
45. How do you configure distributed tracing in a Spring Boot microservice using Spring Cloud Sleuth?
Answer:
Add spring-cloud-starter-sleuth dependency and configure Sleuth in application.properties.
spring.sleuth.sampler.probability=1.0
46. How do you enable Spring Boot DevTools for automatic application restart?
Answer:
Include the spring-boot-devtools dependency in the pom.xml or build.gradle file.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
</dependency>
47. Write a Spring Boot configuration that disables DevTools in production.
Answer:
Use the spring.devtools.restart.enabled property to disable DevTools in production.
spring.devtools.restart.enabled=false
48. How do you configure LiveReload in a Spring Boot application using DevTools?
Answer:
Enable LiveReload in application.properties.
spring.devtools.livereload.enabled=true
49. Write a Spring Boot method that uses @ConfigurationProperties to map application properties to a POJO.
Answer:
Create a POJO and annotate it with @ConfigurationProperties to bind properties.
@ConfigurationProperties(prefix = “app”)
public class AppProperties {
private String name;
private String version;
// Getters and setters
}
50. How do you configure conditional beans in Spring Boot based on DevTools being active?
Answer:
Use @ConditionalOnProperty to conditionally create beans based on DevTools properties.
@Bean
@ConditionalOnProperty(name = “spring.devtools.restart.enabled”, havingValue = “true”)
public MyService devToolsService() {
return new MyService();
}
Final Words
Getting ready for an interview can feel overwhelming, but going through these Spring Boot fresher interview questions can help you feel more confident.
With the right preparation, you’ll ace your Spring Boot interview but don’t forget to practice the Spring Boot basic coding interview questions too!
Frequently Asked Questions
1. What are the most common interview questions for Spring Boot?
Common interview questions for Spring Boot typically focus on creating REST APIs, configuring Spring Boot applications, dependency injection, and working with databases using Spring Data JPA.
2. What are the important Spring Boot topics freshers should focus on for interviews?
Freshers should focus on understanding the basics of Spring Boot, RESTful web services, dependency injection, Spring Data JPA, and security configurations.
3. How should freshers prepare for Spring Boot technical interviews?
Freshers should practice building small Spring Boot projects, understand the core annotations, and familiarize themselves with key concepts like auto-configuration and Spring Boot starters.
4. What strategies can freshers use to solve Spring Boot coding questions during interviews?
Freshers should approach coding questions by clearly understanding the problem, breaking down the requirements, and using Spring Boot’s annotations and configurations effectively.
5. Should freshers prepare for advanced Spring Boot topics in interviews?
Yes, depending on the role, it’s beneficial to have a basic understanding of advanced topics like microservices, Spring Cloud, and security configurations.
Explore More Spring Boot Resources
Explore More Interview Questions
Related Posts
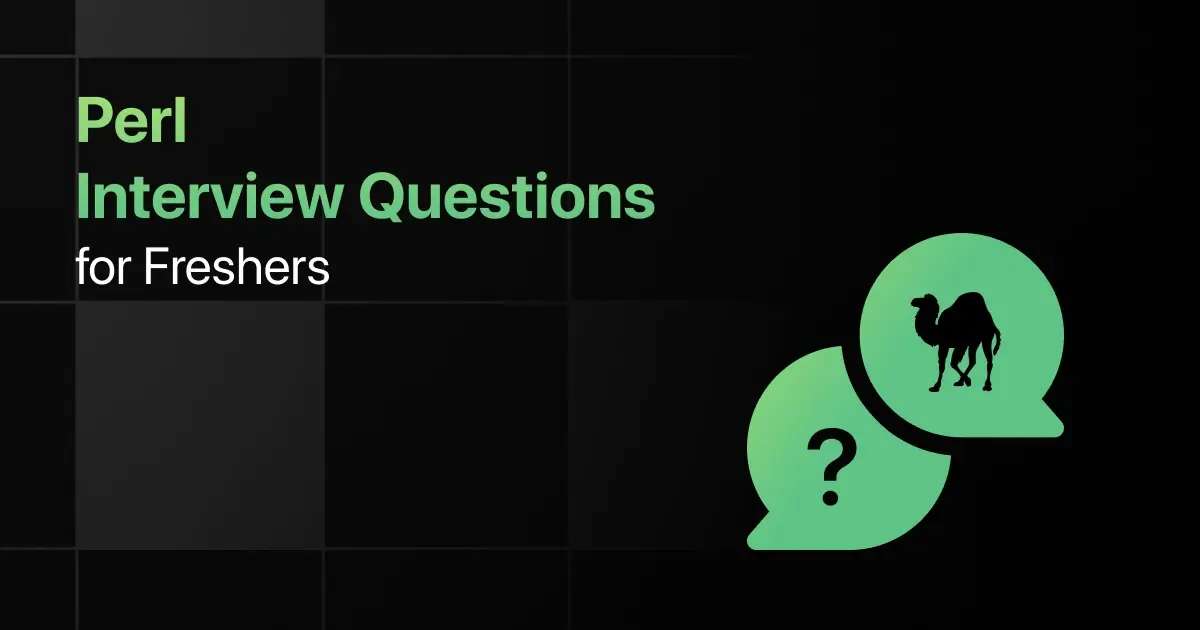
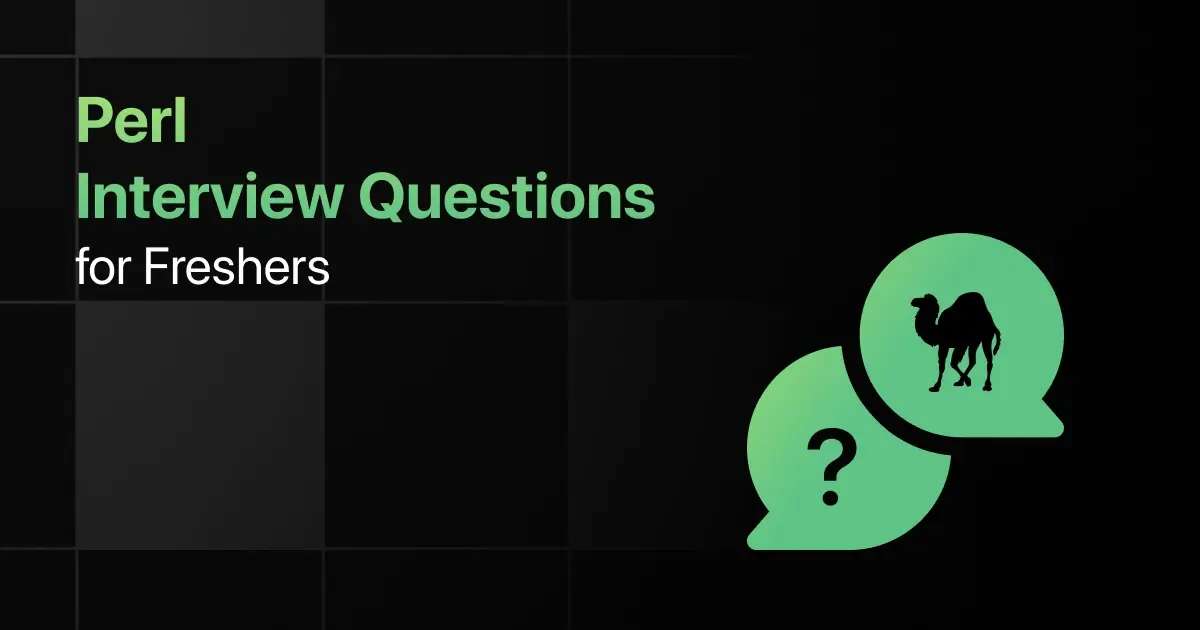
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …