Top JavaScript Interview Questions for Freshers
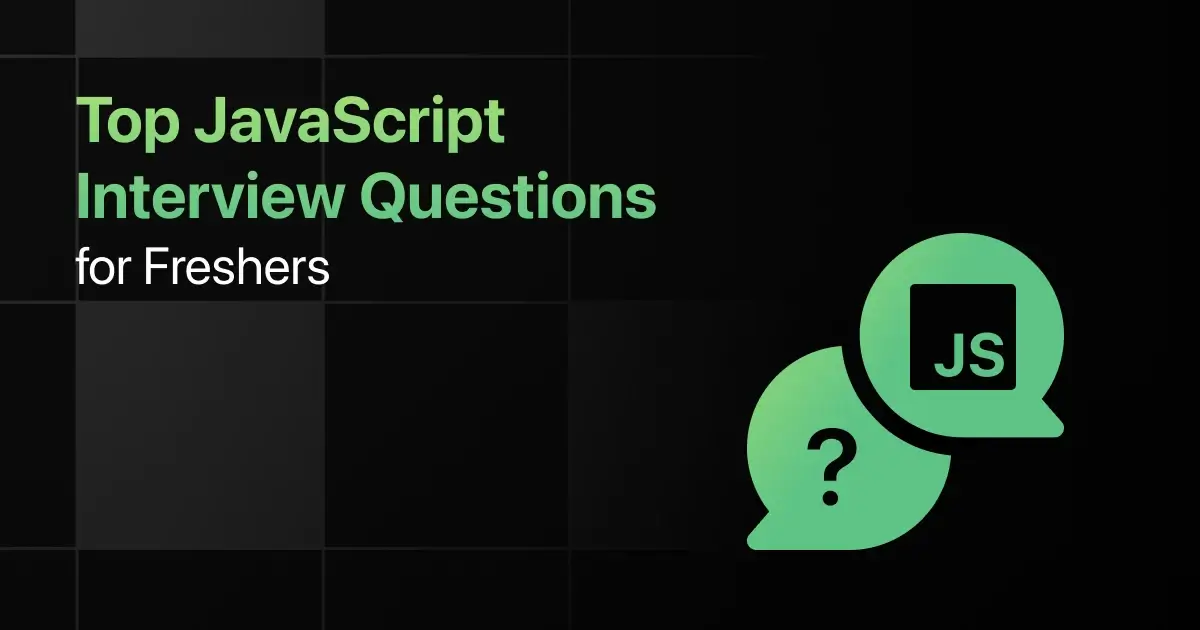
Are you preparing for your first JavaScript interview and wondering what questions you might face? Understanding the key JavaScript interview questions for freshers can give you more clarity.
This blog is here to help you get ready with practical questions that test your real-world problem-solving skills. We’ve gathered some of the most common basic JavaScript interview questions that freshers often encounter.
With this guide, you’ll be well-prepared to tackle these JavaScript interview questions and answers for freshers and make a strong impression in your interview.
Practice JavaScript Interview Questions and Answers
Below are the top 50 Javascript interview questions for freshers with answers:
1. What is JavaScript, and how is it different from Java?
Answer:
JavaScript is a scripting language primarily used for web development, whereas Java is a full-fledged programming language.
// Example of JavaScript code
console.log(“Hello, JavaScript!”);
2. How did JavaScript evolve over time?
Answer:
JavaScript evolved from a simple scripting language to a powerful tool with features like ES6, async programming, and modules.
3. How do you set up a basic JavaScript development environment?
Answer:
Install Node.js for server-side development and use a code editor like VSCode for writing JavaScript code.
node –version
4. What is the difference between running JavaScript in the browser and in Node.js?
Answer:
JavaScript in the browser is used for client-side interactions, while Node.js runs JavaScript on the server side.
5. Write a simple JavaScript program that prints “Hello, World!” to the console.
Answer:
Use console.log() to print messages to the console.
console.log(“Hello, World!”);
6. How do you declare a variable in JavaScript, and what are the differences between var, let, and const?
Answer:
var is function-scoped, let and const are block-scoped, with const being immutable.
let name = “John”;
const age = 30;
7. Explain the difference between == and === in JavaScript.
Answer:
== checks for value equality, while === checks for both value and type equality.
console.log(5 == ‘5’); // true
console.log(5 === ‘5’); // false
8. How do you create an alert, prompt, and confirm dialog in JavaScript?
Answer:
Use alert() to show messages, prompt() to get user input, and confirm() to get confirmation.
alert(“Hello!”);
let name = prompt(“What’s your name?”);
let isConfirmed = confirm(“Are you sure?”);
9. What are primitive data types in JavaScript?
Answer:
Primitive data types include string, number, boolean, null, undefined, and symbol.
let num = 42;
let isActive = true;
10. Write a JavaScript program to calculate the sum of two numbers using arithmetic operators.
Answer:
Use the + operator to sum two numbers.
let a = 5;
let b = 10;
let sum = a + b;
console.log(sum);
11. How do you use an if-else statement to check if a number is positive or negative?
Answer:
Use if-else to conditionally check and execute code based on the number’s value.
let num = -5;
if (num > 0) {
console.log(“Positive”);
} else {
console.log(“Negative”);
}
12. Explain the use of the switch statement in JavaScript.
Answer:
switch is used for multiple conditions based on a single variable, offering a cleaner alternative to multiple if-else statements.
let day = 2;
switch (day) {
case 1:
console.log(“Monday”);
break;
case 2:
console.log(“Tuesday”);
break;
default:
console.log(“Invalid day”);
}
13. How do you write a for loop that prints numbers from 1 to 10?
Answer:
Use a for loop to iterate from 1 to 10 and print each number.
for (let i = 1; i <= 10; i++) {
console.log(i);
}
14. What is the difference between while and do-while loops in JavaScript?
Answer:
while loops check the condition before execution, while do-while loops execute the block at least once before checking the condition.
let i = 1;
do {
console.log(i);
i++;
} while (i <= 5);
15. Write a JavaScript program to print all even numbers from 1 to 20 using a loop and the continue statement.
Answer:
Use continue to skip odd numbers and print only even numbers.
for (let i = 1; i <= 20; i++) {
if (i % 2 !== 0) continue;
console.log(i);
}
16. What is the difference between function declaration and function expression in JavaScript?
Answer:
Function declarations are hoisted, while function expressions are not.
function greet() {
return “Hello!”;
}
const greetExpression = function() {
return “Hello!”;
};
17. How do you use parameters and arguments in a JavaScript function?
Answer:
Parameters are placeholders in the function definition, while arguments are the actual values passed to the function.
function add(a, b) {
return a + b;
}
console.log(add(5, 10)); // 15
18. Explain how the return statement works in a function.
Answer:
The return statement exits the function and optionally returns a value to the caller.
function multiply(a, b) {
return a * b;
}
let result = multiply(3, 4); // 12
19. What are arrow functions in JavaScript, and how do they differ from regular functions?
Answer:
Arrow functions are a shorter syntax for functions and do not have their own this context.
const sum = (a, b) => a + b;
20. Write a JavaScript program using an Immediately Invoked Function Expression (IIFE).
Answer:
IIFEs are functions that execute immediately after they are defined.
(function() {
console.log(“IIFE executed”);
})();
21. How do you create an object in JavaScript, and how can you access its properties?
Answer:
Create an object using curly braces {} and access properties using dot notation or bracket notation.
let person = { name: “John”, age: 30 };
console.log(person.name); // John
22. Explain the use of the this keyword in JavaScript.
Answer:
this refers to the object that is currently calling the method or the global object in a function.
let person = {
name: “John”,
greet: function() {
console.log(this.name);
}
};
person.greet(); // John
23. How do you add and remove elements from an array using push, pop, shift, and unshift methods?
Answer:
push adds to the end, pop removes from the end, shift removes from the beginning, and unshift adds to the beginning of an array.
let arr = [1, 2, 3];
arr.push(4); // [1, 2, 3, 4]
arr.pop(); // [1, 2, 3]
24. What is the difference between map() and forEach() when working with arrays?
Answer:
map returns a new array with the results of calling a function on every element, while forEach executes a function on each element without returning a new array.
let arr = [1, 2, 3];
let doubled = arr.map(x => x * 2); // [2, 4, 6]
25. Write a JavaScript program to filter an array of numbers and return only the even numbers.
Answer:
Use the filter() method to create a new array with elements that pass a test.
let numbers = [1, 2, 3, 4, 5, 6];
let evenNumbers = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers); // [2, 4, 6]
26. How do you find the length of a string in JavaScript?
Answer:
Use the length property to find the number of characters in a string.
let str = “Hello, World!”;
console.log(str.length); // 13
27. Write a JavaScript program to convert a string to uppercase.
Answer:
Use the toUpperCase() method to convert a string to uppercase.
let str = “hello”;
console.log(str.toUpperCase()); // HELLO
28. How do you extract a substring from a string using slice() and substring()?
Answer:
Both slice() and substring() extract parts of a string, but slice() can accept negative indices.
let str = “Hello, World!”;
console.log(str.slice(0, 5)); // Hello
console.log(str.substring(0, 5)); // Hello
29. What is the use of template literals in JavaScript?
Answer:
Template literals allow embedding expressions inside strings using backticks “ and ${}.
let name = “John”;
let greeting = `Hello, ${name}!`;
console.log(greeting); // Hello, John!
30. Write a JavaScript program to replace all occurrences of a substring in a string.
Answer:
Use the replace() method with a regular expression to replace all occurrences of a substring.
let str = “foo bar foo”;
let newStr = str.replace(/foo/g, “baz”);
console.log(newStr); // baz bar baz
31. How do you select an HTML element by its ID in JavaScript?
Answer:
Use document.getElementById() to select an element by its ID.
let element = document.getElementById(“myElement”);
32. Write a JavaScript program to change the text content of an HTML element.
Answer:
Use the textContent property to change the text inside an HTML element.
let element = document.getElementById(“myElement”);
element.textContent = “New Text”;
33. How do you add an event listener to an HTML element in JavaScript?
Answer:
Use addEventListener() to attach an event handler to an HTML element.
let button = document.getElementById(“myButton”);
button.addEventListener(“click”, () => {
alert(“Button clicked!”);
});
34. What is event propagation, and how does it work in JavaScript?
Answer:
Event propagation refers to the order in which events are handled, including capturing, target, and bubbling phases.
Document. getElementById(“parent”).addEventListener(“click”, () => {
console.log(“Parent clicked”);
}, true); // Capturing phase
35. Write a JavaScript program to dynamically create and append an HTML element.
Answer:
Use document.createElement() and appendChild() to dynamically create and add elements to the DOM.
let newElement = document.createElement(“div”);
newElement.textContent = “Hello, World!”;
document.body.appendChild(newElement);
36. Explain function hoisting in JavaScript.
Answer:
Function declarations are hoisted to the top of their scope, allowing them to be called before they are defined.
console.log(greet()); // Hello
function greet() {
return “Hello”;
}
37. What is recursion, and how do you implement it in JavaScript?
Answer:
Recursion is when a function calls itself, used to solve problems that can be broken down into similar sub-problems.
function factorial(n) {
return n <= 1 ? 1 : n * factorial(n – 1);
}
console.log(factorial(5)); // 120
38. What are closures in JavaScript, and why are they important?
Answer:
Closures are functions that remember the scope in which they were created, allowing them to access variables from that scope.
function outer() {
let count = 0;
return function() {
return ++count;
};
}
const increment = outer();
console.log(increment()); // 1
39. How do you use bind(), call(), and apply() methods in JavaScript?
Answer:
These methods are used to change the this context of a function, with call() and apply() invoking the function immediately.
let obj = { num: 2 };
function multiply(a, b) {
return this.num * a * b;
}
console.log(multiply.call(obj, 3, 4)); // 24
40. What is function currying, and how do you implement it in JavaScript?
Answer:
Currying transforms a function with multiple arguments into a series of functions that each take a single argument.
function add(a) {
return function(b) {
return a + b;
};
}
console.log(add(2)(3)); // 5
41. How do you handle errors in JavaScript using try, catch, and finally?
Answer:
Wrap code that may throw errors in a try block, handle the error in catch, and optionally execute code in finally.
try {
let result = someUndefinedFunction();
} catch (error) {
console.log(“An error occurred: ” + error.message);
} finally {
console.log(“This runs no matter what”);
}
42. Write a JavaScript program that throws a custom error if a number is negative.
Answer:
Use throw to create and throw a custom error based on a condition.
function checkPositive(num) {
if (num < 0) {
throw new Error(“Number is negative”);
}
return num;
}
try {
console.log(checkPositive(-5));
} catch (error) {
console.log(error.message);
}
43. What are the different types of errors in JavaScript?
Answer:
Common error types include SyntaxError, ReferenceError, TypeError, and RangeError.
44. How do you debug JavaScript code in the browser?
Answer:
Use the browser’s developer tools to set breakpoints, inspect variables, and step through code.
45. Explain the concept of stack trace in JavaScript error handling.
Answer:
A stack trace shows the sequence of function calls that led to an error, helping in debugging.
function a() { b(); }
function b() { c(); }
function c() { throw new Error(“Oops!”); }
a(); // Stack trace will show a -> b -> c
46. What is a callback function, and how is it used in asynchronous JavaScript?
Answer:
A callback function is passed as an argument to another function and executed after the completion of a task.
function fetchData(callback) {
setTimeout(() => {
callback(“Data received”);
}, 1000);
}
fetchData((data) => {
console.log(data);
});
47. How do you create and use a promise in JavaScript?
Answer:
A promise represents the eventual completion (or failure) of an asynchronous operation and its resulting value.
let promise = new Promise((resolve, reject) => {
setTimeout(() => resolve(“Success”), 1000);
});
promise.then(result => console.log(result));
48. What is the purpose of async and await in JavaScript?
Answer:
async and await simplify asynchronous code, making it look and behave more like synchronous code.
async function fetchData() {
let data = await new Promise((resolve) => setTimeout(() => resolve(“Data received”), 1000));
console.log(data);
}
fetchData();
49. Write a JavaScript program to fetch data from an API using fetch and handle errors.
Answer:
Use the fetch API for making HTTP requests and handle errors with .catch().
fetch(“https://api.example.com/data”)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(“Error fetching data:”, error));
50. How do you handle errors in asynchronous code using try-catch with async-await?
Answer:
Wrap the await calls in a try-catch block to handle errors in asynchronous functions.
async function fetchData() {
try {
let response = await fetch(“https://api.example.com/data”);
let data = await response.json();
console.log(data);
} catch (error) {
console.error(“Error fetching data:”, error);
}
}
fetchData();
Final Words
Getting ready for an interview can feel overwhelming, but going through these JavaScript fresher interview questions can help you feel more confident. This guide focuses on the kinds of JavaScript developer interview questions for fresher roles that you’re likely to face.
Don’t forget to practice the JavaScript basic coding interview questions too! With the right preparation, you’ll ace your JavaScript interview and take that important step in your career.
Frequently Asked Questions
1. What are the most common interview questions for JavaScript?
The most common JavaScript interview questions often cover topics like closures, hoisting, event handling, and basic DOM manipulation.
2. What are the important JavaScript topics freshers should focus on for interviews?
Freshers should focus on understanding concepts like scope, functions, ES6 features, promises, and async/await.
3. How should freshers prepare for JavaScript technical interviews?
Freshers should practice writing clean, efficient code, understand core JavaScript concepts, and solve a variety of coding problems.
4. What strategies can freshers use to solve JavaScript coding questions during interviews?
Freshers should break down problems, write pseudocode first, and thoroughly test their code with different inputs.
5. Should freshers prepare for advanced JavaScript topics in interviews?
Yes, depending on the role, freshers might need to know advanced topics like closures, asynchronous programming, and design patterns.
Explore More JavaScript Resources
- JavaScript Learning Websites
- JavaScript Practice Websites
- JavaScript YouTube Channels
- JavaScript Project Ideas
- JavaScript Frameworks
- JavaScript Apps
- JavaScript IDEs
- JavaScript MCQ
Explore More Interview Questions
Related Posts
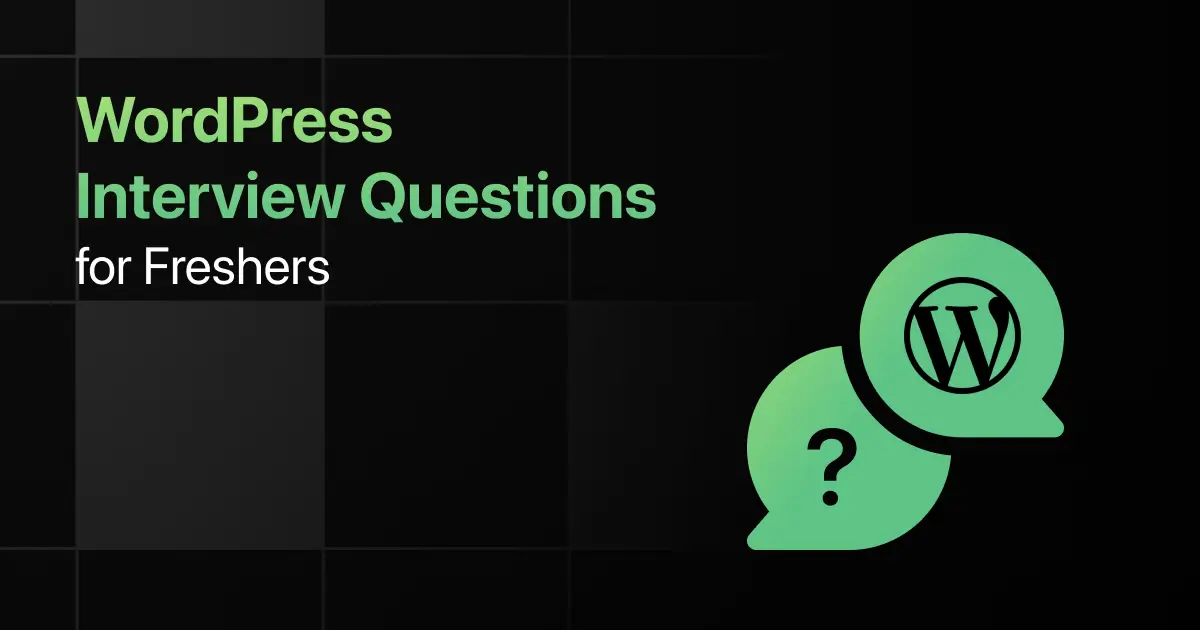
Top WordPress Interview Questions for Freshers
Are you preparing for your first WordPress interview and wondering what questions you might face? Understanding the key WordPress interview questions …