Top Python Interview Questions for Freshers
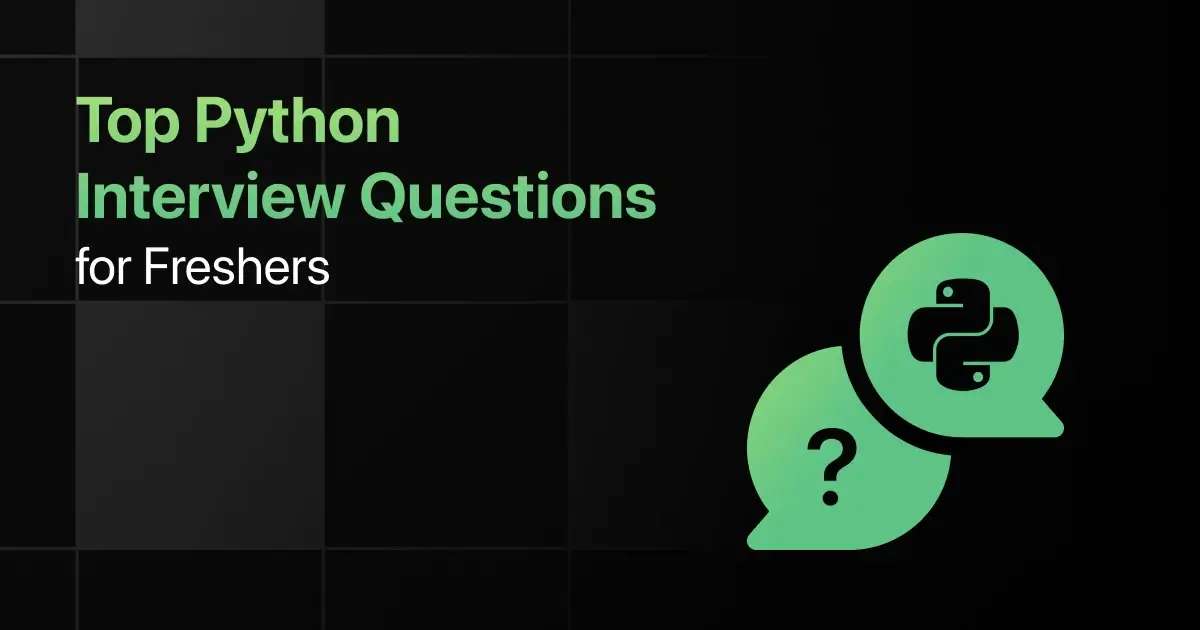
Are you preparing for your first Python interview and wondering what questions you might face? Understanding the key Python interview questions for freshers can give you more clarity.
This blog is here to help you get ready with practical questions that test your real-world problem-solving skills. We’ve gathered some of the most common basic Python interview questions that freshers often encounter.
With this guide, you’ll be well-prepared to tackle these Python interview questions and answers for freshers and make a strong impression in your interview.
Practice Python Interview Questions and Answers
Below are the 50 Python interview questions for freshers with answers:
1. Write a Python program to check if a given number is even or odd using the modulo operator.
Answer:
The solution checks if the number is divisible by 2 using the modulo operator. If the result is 0, the number is even; otherwise, it’s odd.
def check_even_odd(num):
if num % 2 == 0:
return “Even”
else:
return “Odd”
number = int(input(“Enter a number: “))
print(check_even_odd(number))
2. How would you swap two variables in Python without using a temporary variable?
Answer:
You can swap two variables using tuple unpacking.
a = 5
b = 10
a, b = b, a
print(a, b) # Output: 10 5
3. Write a Python program to find the maximum of three numbers using comparison operators.
Answer:
The solution compares three numbers using nested if-else statements to determine the maximum value.
def find_maximum(a, b, c):
if a >= b and a >= c:
return a
elif b >= a and b >= c:
return b
else:
return c
print(find_maximum(10, 20, 15))
4. Write a Python program to check if a given number lies within a specific range (10 to 50).
Answer:
The solution uses the logical and operator to check if the number lies between 10 and 50.
def is_within_range(num):
if 10 <= num <= 50:
return True
else:
return False
print(is_within_range(25))
5. Write a Python program to increment a variable by 5 using a compound assignment operator.
Answer:
The solution demonstrates the use of the += operator to add 5 to a given variable.
def increment_by_five(n):
n += 5
return n
number = 10
print(increment_by_five(number))
6. What is the difference between == and is in Python?
Answer:
- == checks if the values of two objects are equal.
- is checks if two objects are the same instance (i.e., they have the same memory address).
a = [1, 2, 3]
b = [1, 2, 3]
print(a == b) # Output: True
print(a is b) # Output: False
7. How can you check if a string is a palindrome in Python?
Answer:
A palindrome is a string that reads the same forward and backward. You can check this by comparing the string with its reverse.
def is_palindrome(s):
return s == s[::-1]
print(is_palindrome(“radar”)) # Output: True
print(is_palindrome(“hello”)) # Output: False
8. Write a Python program to check whether a number is positive, negative, or zero.
Answer:
The solution uses if-elif-else statements to determine the sign of the number.
def check_sign(num):
if num > 0:
return “Positive”
elif num < 0:
return “Negative”
else:
return “Zero”
number = int(input(“Enter a number: “))
print(check_sign(number))
9. Write a Python program to find the sum of the digits of a number.
Answer:
The solution uses a for loop to iterate through each digit of the number and accumulate the sum.
def sum_of_digits(n):
total = 0
for digit in str(n):
total += int(digit)
return total
print(sum_of_digits(1234))
10. What are Python decorators, and how do they work?
Answer:
Decorators are a way to modify the behavior of a function or method. They allow you to wrap another function to extend its behavior.
def my_decorator(func):
def wrapper():
print(“Something is happening before the function is called.”)
func()
print(“Something is happening after the function is called.”)
return wrapper
@my_decorator
def say_hello():
print(“Hello!”)
say_hello()
# Output:
# Something is happening before the function is called.
# Hello!
# Something is happening after the function is called.
11. How would you define a function that calculates the factorial of a number?
Answer:
The function takes a number as input and returns its factorial using a loop or recursion.
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
print(factorial(5)) # Output: 120
12. What happens if you pass more arguments to a function than it is defined to accept?
Answer:
Python will raise a TypeError indicating that the function received too many arguments.
13. How can you define a function with both positional and keyword arguments?
Answer:
You define a function where some arguments are positional, and others have default values.
def greet(name, message=”Hello”):
return f”{message}, {name}!”
print(greet(“John”)) # Output: Hello, John!
14. What is the purpose of the *args and **kwargs in function arguments?
Answer:
*args allows you to pass a variable number of positional arguments, while **kwargs allows for keyword arguments.
def func(*args, **kwargs):
print(args)
print(kwargs)
func(1, 2, 3, a=4, b=5) # Output: (1, 2, 3) {‘a’: 4, ‘b’: 5}
15. How would you create a lambda function that squares a number?
Answer:
A lambda function is a short anonymous function defined using the lambda keyword.
square = lambda x: x**2
print(square(4)) # Output: 16
16. How can you return multiple values from a function?
Answer:
You can return multiple values by separating them with commas.
def get_min_max(numbers):
return min(numbers), max(numbers)
print(get_min_max([1, 2, 3, 4])) # Output: (1, 4)
17. What happens when you define a function inside another function?
Answer:
The inner function is locally scoped to the outer function and can only be called within it.
18. How does Python handle default mutable arguments in functions?
Answer:
Default mutable arguments (like lists) retain their state across function calls, leading to unexpected behavior.
19. How would you modify a global variable inside a function?
Answer:
Use the global keyword to modify a global variable within a function.
x = 10
def modify_global():
global x
x = 20
modify_global()
print(x) # Output: 20
20. How would you use the nonlocal keyword in nested functions?
Answer:
nonlocal allows you to modify a variable in the nearest enclosing scope that is not global.
def outer():
x = 5
def inner():
nonlocal x
x = 10
inner()
return x
print(outer()) # Output: 10
21. How do you create a list of even numbers from 1 to 10 using list comprehension?
Answer:
List comprehension is a concise way to create lists in Python.
evens = [x for x in range(1, 11) if x % 2 == 0] print(evens) # Output: [2, 4, 6, 8, 10]
22. How would you remove the last element from a list in Python?
Answer:
You can use the pop() method without an argument to remove the last element.
my_list = [1, 2, 3]
my_list.pop()
print(my_list) # Output: [1, 2]
23. How can you merge two dictionaries in Python?
Answer:
You can merge two dictionaries using the update() method or the {**dict1, **dict2} syntax.
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
dict1.update(dict2)
print(dict1) # Output: {‘a’: 1, ‘b’: 3, ‘c’: 4}
24. What is the difference between a list and a tuple?
Answer:
A list is mutable, while a tuple is immutable. Lists use square brackets [], and tuples use parentheses ().
25. How would you check if a key exists in a dictionary?
Answer:
Use the in keyword to check if a key is in a dictionary.
my_dict = {‘a’: 1, ‘b’: 2}
print(‘a’ in my_dict) # Output: True
26. How can you sort a list of tuples based on the second element in each tuple?
Answer:
Use the sorted() function with a lambda function as the key.
my_list = [(1, 3), (2, 2), (3, 1)]
sorted_list = sorted(my_list, key=lambda x: x[1])
print(sorted_list) # Output: [(3, 1), (2, 2), (1, 3)]
27. How would you remove duplicate elements from a list?
Answer:
Convert the list to a set and back to a list to remove duplicates.
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = list(set(my_list))
print(unique_list) # Output: [1, 2, 3, 4, 5]
28. How do you count the occurrences of each element in a list?
Answer:
Use a dictionary to count the occurrences of each element.
my_list = [1, 2, 2, 3, 3, 3]
count_dict = {}
for item in my_list:
count_dict[item] = count_dict.get(item, 0) + 1
print(count_dict) # Output: {1: 1, 2: 2, 3: 3}
29. How would you create a set from a list?
Answer:
Convert the list to a set using the set() function.
my_list = [1, 2, 3, 4, 4, 5]
my_set = set(my_list)
print(my_set) # Output: {1, 2, 3, 4, 5}
30. How can you create a dictionary with default values for keys?
Answer:
Use the defaultdict from the collections module to create a dictionary with default values.
from collections import defaultdict
my_dict = defaultdict(int)
my_dict[‘a’] += 1
print(my_dict) # Output: {‘a’: 1}
31. How do you define a class in Python?
Answer:
Use the class keyword followed by the class name and a colon.
class MyClass:
pass
32. How would you initialize an object with specific attributes?
Answer:
Use the __init__ method to initialize an object’s attributes when it is created.
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
my_car = Car(“Toyota”, “Corolla”)
print(my_car.make, my_car.model) # Output: Toyota Corolla
33. What is the difference between instance variables and class variables?
Answer:
Instance variables are unique to each instance, while class variables are shared across all instances of the class.
34. How would you implement inheritance in Python?
Answer:
Define a new class that inherits from an existing class by passing the parent class as an argument.
class Animal:
def speak(self):
return “Animal speaks”
class Dog(Animal):
def speak(self):
return “Dog barks”
my_dog = Dog()
print(my_dog.speak()) # Output: Dog barks
35. What is polymorphism in Python?
Answer:
Polymorphism allows different classes to be treated as instances of the same class through inheritance. The method is overridden in derived classes.
36. How do you achieve encapsulation in Python?
Answer:
Encapsulation is achieved by using private variables and methods, typically prefixed with an underscore (_).
class Example:
def __init__(self):
self._private_var = 10
37. What is abstraction, and how do you implement it in Python?
Answer:
Abstraction hides implementation details and shows only the essential features. It can be achieved using abstract classes and methods.
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def sound(self):
pass
38. How would you override the __str__ method in a class?
Answer:
Override the __str__ method to define how the object should be represented as a string.
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
def __str__(self):
return f”{self.make} {self.model}”
my_car = Car(“Toyota”, “Corolla”)
print(my_car) # Output: Toyota Corolla
39. How would you implement a class method in Python?
Answer:
Use the @classmethod decorator to define a method that belongs to the class rather than an instance.
class MyClass:
count = 0
@classmethod
def increment_count(cls):
cls.count += 1
MyClass.increment_count()
print(MyClass.count) # Output: 1
40. What is the purpose of the __len__ magic method?
Answer:
The __len__ method is used to return the length of an object when len() is called on it.
class MyList:
def __init__(self, items):
self.items = items
def __len__(self):
return len(self.items)
my_list = MyList([1, 2, 3])
print(len(my_list)) # Output: 3
41. How can you append data to an existing file?
Answer:
Use the mode ‘a’ with the open() function to append data to a file.
with open(‘file.txt’, ‘a’) as file:
file.write(“\nAppended text”)
42. How do you read a file line by line in Python?
Answer:
Use a loop to iterate over the file object.
with open(‘file.txt’, ‘r’) as file:
for line in file:
print(line, end=”)
43. How would you read a binary file in Python?
Answer:
Use the mode ‘rb’ with the open() function to read a binary file.
with open(‘image.jpg’, ‘rb’) as file:
data = file.read()
44. How can you move a file in Python?
Answer:
Use the os.rename() function to move a file to a new location.
import os
os.rename(‘old_path/file.txt’, ‘new_path/file.txt’)
45. How do you delete a file in Python?
Answer:
Use the os.remove() function to delete a file.
import os
os.remove(‘file.txt’)
46. How would you list all files in a directory with a specific extension?
Answer:
Use os.listdir() combined with a list comprehension to filter files by extension.
import os
files = [f for f in os.listdir(‘.’) if f.endswith(‘.txt’)]
print(files)
47. How do you copy a file in Python?
Answer:
Use the shutil.copy() function from the shutil module to copy a file.
import shutil
shutil.copy(‘source.txt’, ‘destination.txt’)
48. How do you raise an exception in Python?
Answer:
Use the raise keyword to raise an exception manually.
raise ValueError(“Invalid value provided”)
49. How would you create a custom exception in Python?
Answer:
Define a new class that inherits from the built-in Exception class.
class MyCustomError(Exception):
pass
raise MyCustomError(“This is a custom exception”)
50. How can you handle exceptions in a loop without breaking the loop?
Answer:
Use a try-except block inside the loop to handle exceptions for each iteration.
for i in range(5):
try:
print(1 / i)
except ZeroDivisionError:
print(“Cannot divide by zero”)
Final Words
Getting ready for an interview can feel overwhelming, but going through these Python fresher interview questions can help you feel more confident. This guide focuses on the kinds of Python developer interview questions for fresher roles that you’re likely to face.
Don’t forget to practice the Python basic coding interview questions too! With the right preparation, you’ll ace your Python interview and take that important step in your career.
Frequently Asked Questions
1. What are the most common interview questions for Python?
The most common interview questions for Python are usually centered around basic syntax, control flow, data structures, and simple algorithms.
2. What are the important Python topics freshers should focus on for interviews?
Freshers should focus on data types, loops, functions, OOP concepts, and basic problem-solving.
3. How should freshers prepare for Python technical interviews?
Freshers should practice coding regularly, understand core concepts, and work on sample interview questions.
4. What strategies can freshers use to solve Python coding questions during interviews?
Freshers should break down problems into smaller parts, write clear and simple code, and test their solutions thoroughly.
5. Should freshers prepare for advanced Python topics in interviews?
Yes, freshers should be familiar with advanced topics like file handling, exceptions, and libraries, depending on the job requirements.
Explore More Python Resources
- Python Learning Websites
- Python Practicing Websites
- Python YouTube Channels
- Python Project Ideas
- Python Frameworks
- Python Apps
- Python IDEs
- Python MCQ
Explore More Interview Questions
Related Posts
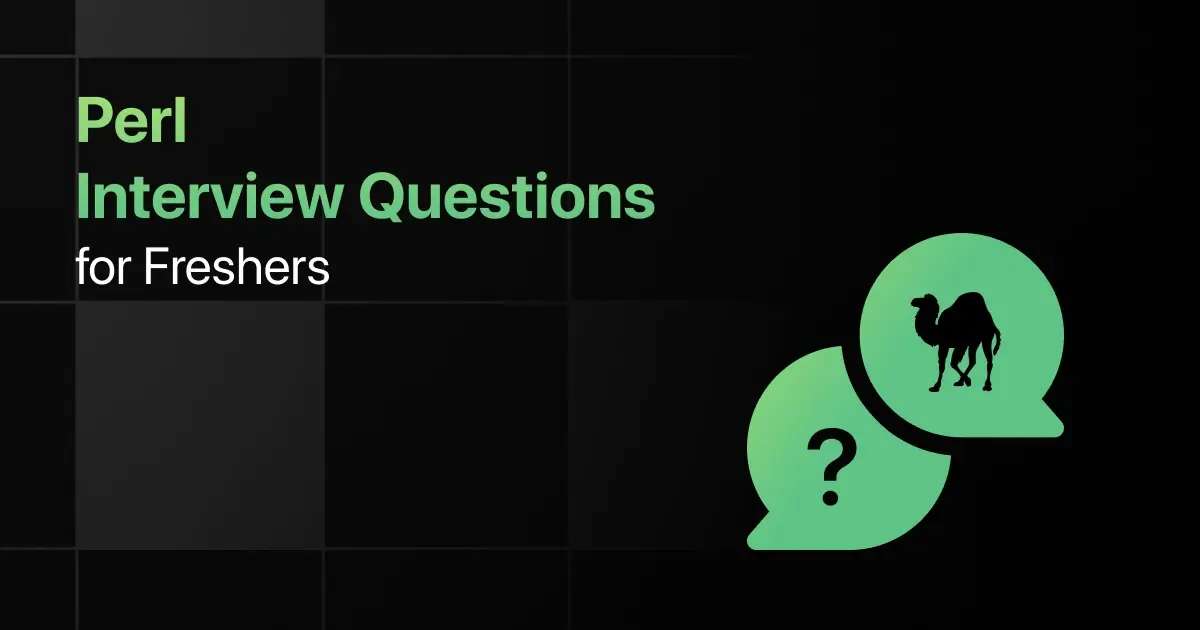
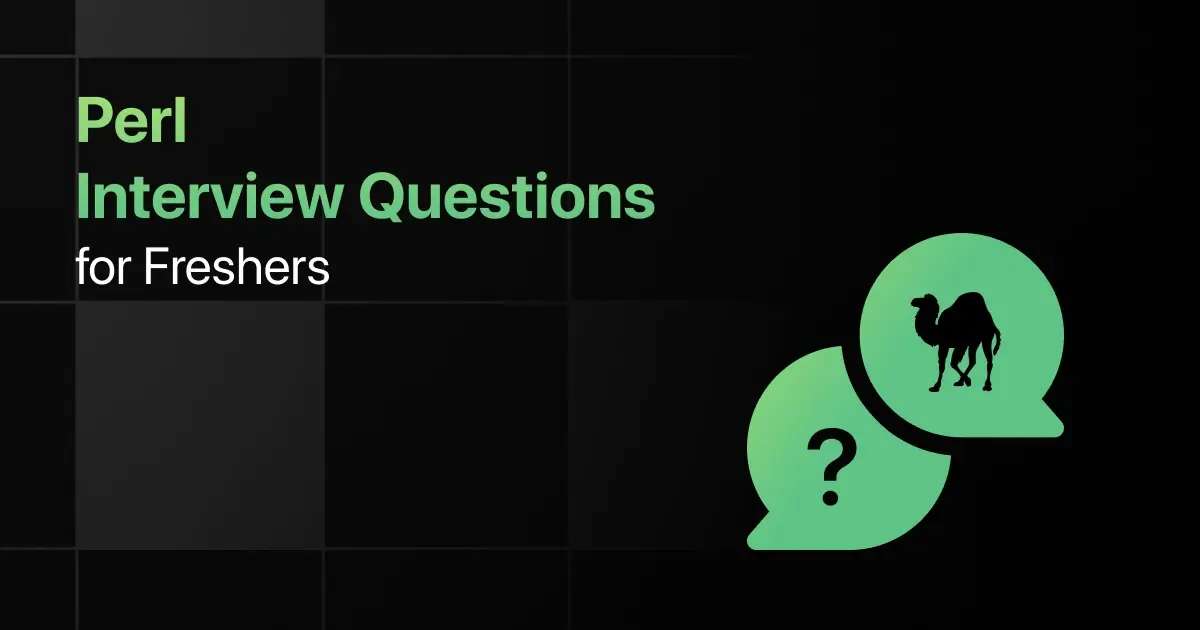
Top Perl Interview Questions for Freshers
Are you preparing for your first Perl interview and wondering what questions you might face? Understanding the key Perl interview questions …